Generate States/Choices/Shocks Grids, get Functions (Interpolated + Risky + Safe Asset)
back to Fan's Dynamic Assets Repository Table of Content.
Contents
- FFS_AKZ_GET_FUNCGRID get funcs, params, states choices shocks grids
- Default
- Parse Parameters
- Get Asset and Choice Grid
- Get Shock Grids
- Get Equations
- Generate Cash-on-Hand/State Matrix
- IWKZ Interpolation Cash-on-hand Interpolation Grid
- IWKZ Interpolation Consumption Grid
- Store
- Graph
- Graph 1: a and k choice grid graphs
- Graph 2: coh by shock
- Graph 3: coh by index
- Graph 4: coh by coh
- Display
function [armt_map, func_map] = ffs_akz_get_funcgrid(varargin)
FFS_AKZ_GET_FUNCGRID get funcs, params, states choices shocks grids
Centralized gateway for retrieving parameters, and solution grids and functions.
This function services both m_akz as well as m_akbz functions. This means that the function here support solving both the borrowing as well as the savings problems.
Note that while we have several versions of algorithms, one version requires interpolation, so there are several matrixes and vectors below that are specifically for that. These interpolation related matrixes and arrays are not used when we solve the wkz or akz problem, but are used when we solve the iwkz problem.
For the ikwz problem, we have two cash-on-hand matrixes: mt_coh_wkb
Note that in the m_abz problem's funcgrid function, we also called ffs_abz_gen_borrsave_grid function, which generates borrowing grid depending on if default is allowed or not. In the problem here, the situation is more complicated, because now the maximum borrowing level is a function of the risky investment choice when default is not allowed. We impose a exogenous borrowing bound here.
@param param_map container parameter container
@param support_map container support container
@param bl_input_override boolean if true varargin contained param_map and support_map fully overrides local default. Local default is not invoked. This could be important for speed if this function is getting invoked within certain loops. Default is 0.
@return armt_map container container with states, choices and shocks grids that are inputs for grid based solution algorithm. keys included in armt_map are described below for each group of vectors when they are stored into armt_map.
@return func_map container container with function handles for consumption cash-on-hand etc.
@example
it_param_set = 2; bl_input_override = true; [param_map, support_map] = ffs_akz_set_default_param(it_param_set); [armt_map, func_map] = ffs_akz_get_funcgrid(param_map, support_map, bl_input_override);
@include
* ffs_akz_set_functions * ffto_gen_tauchen_jhl * fft_gen_grid_loglin
Default
if (~isempty(varargin)) % override when called from outside [param_map, support_map] = varargin{:}; else % default internal run [param_map, support_map] = ffs_akz_set_default_param(); support_map('bl_graph_funcgrids') = true; support_map('bl_display_funcgrids') = true; default_maps = {param_map, support_map}; % Run Default with Borrowing param_map('fl_b_bd') = -20; % numvarargs is the number of varagin inputted [default_maps{1:length(varargin)}] = varargin{:}; param_map = [param_map; default_maps{1}]; support_map = [support_map; default_maps{2}]; end
Parse Parameters
params_group = values(param_map, {'it_z_n', 'fl_z_mu', 'fl_z_rho', 'fl_z_sig'}); [it_z_n, fl_z_mu, fl_z_rho, fl_z_sig] = params_group{:}; params_group = values(param_map, {'fl_b_bd', 'fl_w_max', 'it_w_n', 'fl_coh_interp_grid_gap'}); [fl_b_bd, fl_w_max, it_w_n, fl_coh_interp_grid_gap] = params_group{:}; params_group = values(param_map, {'fl_k_min', 'it_ak_n'}); [fl_k_min, it_ak_n] = params_group{:}; params_group = values(param_map, {'fl_crra', 'fl_c_min', 'it_c_interp_grid_gap'}); [fl_crra, fl_c_min, it_c_interp_grid_gap] = params_group{:}; params_group = values(param_map, {'fl_Amean', 'fl_alpha', 'fl_delta'}); [fl_Amean, fl_alpha, fl_delta] = params_group{:}; params_group = values(param_map, {'fl_r_save', 'fl_r_borr', 'fl_w'}); [fl_r_save, fl_r_borr, fl_w] = params_group{:}; params_group = values(support_map, {'bl_graph_funcgrids', 'bl_display_funcgrids'}); [bl_graph_funcgrids, bl_display_funcgrids] = params_group{:};
Get Asset and Choice Grid
This generate triangular choice structure. Household choose total aggregate savings, and within that how much to put into risky capital and how much to put into safe assets. See ffs_akz_set_default_param for details.
fl_w_min = param_map('fl_b_bd'); fl_k_max = (param_map('fl_w_max') - param_map('fl_b_bd')); ar_w = linspace(fl_w_min, fl_w_max, it_w_n); ar_k = linspace(fl_k_min, fl_k_max, it_ak_n); mt_a = ar_w - ar_k'; mt_k = ar_w - mt_a; mt_bl_constrained = (mt_a < fl_b_bd); % this generates NAN, some NAN have -Inf Util mt_a_wth_na = mt_a; mt_k_wth_na = mt_k; mt_a_wth_na(mt_bl_constrained) = nan; mt_k_wth_na(mt_bl_constrained) = nan; ar_a_mw_wth_na = mt_a_wth_na(:); ar_k_mw_wth_na = mt_k_wth_na(:); ar_a_meshk = ar_a_mw_wth_na(~isnan(ar_a_mw_wth_na)); ar_k_mesha = ar_k_mw_wth_na(~isnan(ar_k_mw_wth_na));
Get Shock Grids
[~, mt_z_trans, ar_stationary, ar_z] = ffto_gen_tauchen_jhl(fl_z_mu,fl_z_rho,fl_z_sig,it_z_n);
Get Equations
[f_util_log, f_util_crra, f_util_standin, f_prod, f_inc, f_coh, f_cons] = ...
ffs_akz_set_functions(fl_crra, fl_c_min, fl_Amean, fl_alpha, fl_delta, fl_r_save, fl_r_borr, fl_w);
Generate Cash-on-Hand/State Matrix
The endogenous state variable is cash-on-hand, it has it_z_n*it_a_n number of points, covering all reachable points when ar_a is the choice vector and ar_z is the shock vector. requires inputs from get Asset and choice grids, get shock grids, and get equations above.
mt_coh_wkb = f_coh(ar_z, ar_a_meshk, ar_k_mesha); mt_z_mesh_coh_wkb = repmat(ar_z, [size(mt_coh_wkb,1),1]); if (bl_display_funcgrids) % Generate Aggregate Variables ar_aplusk_mesh = ar_a_meshk + ar_k_mesha; % Genereate Table tab_ak_choices = array2table([ar_aplusk_mesh, ar_k_mesha, ar_a_meshk]); cl_col_names = {'ar_aplusk_mesh', 'ar_k_mesha', 'ar_a_meshk'}; tab_ak_choices.Properties.VariableNames = cl_col_names; % Label Table Variables tab_ak_choices.Properties.VariableDescriptions{'ar_aplusk_mesh'} = ... '*ar_aplusk_mesh*: ar_aplusk_mesh = ar_a_meshk + ar_k_mesha;'; tab_ak_choices.Properties.VariableDescriptions{'ar_k_mesha'} = ... '*ar_k_mesha*:'; tab_ak_choices.Properties.VariableDescriptions{'ar_a_meshk'} = ... '*ar_a_meshk*:'; cl_var_desc = tab_ak_choices.Properties.VariableDescriptions; for it_var_name = 1:length(cl_var_desc) disp(cl_var_desc{it_var_name}); end disp('----------------------------------------'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp('tab_ak_choices'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); it_rows_toshow = length(ar_w)*2; disp(size(tab_ak_choices)); disp(head(array2table(tab_ak_choices), it_rows_toshow)); disp(tail(array2table(tab_ak_choices), it_rows_toshow)); disp('----------------------------------------'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp('mt_coh_wkb'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp(size(mt_coh_wkb)); disp(head(array2table(mt_coh_wkb), it_rows_toshow)); disp(tail(array2table(mt_coh_wkb), it_rows_toshow)); end
*ar_aplusk_mesh*: ar_aplusk_mesh = ar_a_meshk + ar_k_mesha; *ar_k_mesha*: *ar_a_meshk*: ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx tab_ak_choices xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx 1275 3 tab_ak_choices1 tab_ak_choices2 tab_ak_choices3 ar_aplusk_mesh ar_k_mesha ar_a_meshk _______________ _______________ _______________ -20 0 -20 -18.571 0 -18.571 -18.571 1.4286 -20 -17.143 0 -17.143 -17.143 1.4286 -18.571 -17.143 2.8571 -20 -15.714 0 -15.714 -15.714 1.4286 -17.143 -15.714 2.8571 -18.571 -15.714 4.2857 -20 -14.286 0 -14.286 -14.286 1.4286 -15.714 -14.286 2.8571 -17.143 -14.286 4.2857 -18.571 -14.286 5.7143 -20 -12.857 0 -12.857 -12.857 1.4286 -14.286 -12.857 2.8571 -15.714 -12.857 4.2857 -17.143 -12.857 5.7143 -18.571 -12.857 7.1429 -20 -11.429 0 -11.429 -11.429 1.4286 -12.857 -11.429 2.8571 -14.286 -11.429 4.2857 -15.714 -11.429 5.7143 -17.143 -11.429 7.1429 -18.571 -11.429 8.5714 -20 -10 0 -10 -10 1.4286 -11.429 -10 2.8571 -12.857 -10 4.2857 -14.286 -10 5.7143 -15.714 -10 7.1429 -17.143 -10 8.5714 -18.571 -10 10 -20 -8.5714 0 -8.5714 -8.5714 1.4286 -10 -8.5714 2.8571 -11.429 -8.5714 4.2857 -12.857 -8.5714 5.7143 -14.286 -8.5714 7.1429 -15.714 -8.5714 8.5714 -17.143 -8.5714 10 -18.571 -8.5714 11.429 -20 -7.1429 0 -7.1429 -7.1429 1.4286 -8.5714 -7.1429 2.8571 -10 -7.1429 4.2857 -11.429 -7.1429 5.7143 -12.857 -7.1429 7.1429 -14.286 -7.1429 8.5714 -15.714 -7.1429 10 -17.143 -7.1429 11.429 -18.571 -7.1429 12.857 -20 -5.7143 0 -5.7143 -5.7143 1.4286 -7.1429 -5.7143 2.8571 -8.5714 -5.7143 4.2857 -10 -5.7143 5.7143 -11.429 -5.7143 7.1429 -12.857 -5.7143 8.5714 -14.286 -5.7143 10 -15.714 -5.7143 11.429 -17.143 -5.7143 12.857 -18.571 -5.7143 14.286 -20 -4.2857 0 -4.2857 -4.2857 1.4286 -5.7143 -4.2857 2.8571 -7.1429 -4.2857 4.2857 -8.5714 -4.2857 5.7143 -10 -4.2857 7.1429 -11.429 -4.2857 8.5714 -12.857 -4.2857 10 -14.286 -4.2857 11.429 -15.714 -4.2857 12.857 -17.143 -4.2857 14.286 -18.571 -4.2857 15.714 -20 -2.8571 0 -2.8571 -2.8571 1.4286 -4.2857 -2.8571 2.8571 -5.7143 -2.8571 4.2857 -7.1429 -2.8571 5.7143 -8.5714 -2.8571 7.1429 -10 -2.8571 8.5714 -11.429 -2.8571 10 -12.857 -2.8571 11.429 -14.286 -2.8571 12.857 -15.714 -2.8571 14.286 -17.143 -2.8571 15.714 -18.571 -2.8571 17.143 -20 -1.4286 0 -1.4286 -1.4286 1.4286 -2.8571 -1.4286 2.8571 -4.2857 -1.4286 4.2857 -5.7143 -1.4286 5.7143 -7.1429 -1.4286 7.1429 -8.5714 -1.4286 8.5714 -10 -1.4286 10 -11.429 -1.4286 11.429 -12.857 tab_ak_choices1 tab_ak_choices2 tab_ak_choices3 ar_aplusk_mesh ar_k_mesha ar_a_meshk _______________ _______________ _______________ 47.143 67.143 -20 48.571 0 48.571 48.571 1.4286 47.143 48.571 2.8571 45.714 48.571 4.2857 44.286 48.571 5.7143 42.857 48.571 7.1429 41.429 48.571 8.5714 40 48.571 10 38.571 48.571 11.429 37.143 48.571 12.857 35.714 48.571 14.286 34.286 48.571 15.714 32.857 48.571 17.143 31.429 48.571 18.571 30 48.571 20 28.571 48.571 21.429 27.143 48.571 22.857 25.714 48.571 24.286 24.286 48.571 25.714 22.857 48.571 27.143 21.429 48.571 28.571 20 48.571 30 18.571 48.571 31.429 17.143 48.571 32.857 15.714 48.571 34.286 14.286 48.571 35.714 12.857 48.571 37.143 11.429 48.571 38.571 10 48.571 40 8.5714 48.571 41.429 7.1429 48.571 42.857 5.7143 48.571 44.286 4.2857 48.571 45.714 2.8571 48.571 47.143 1.4286 48.571 48.571 0 48.571 50 -1.4286 48.571 51.429 -2.8571 48.571 52.857 -4.2857 48.571 54.286 -5.7143 48.571 55.714 -7.1429 48.571 57.143 -8.5714 48.571 58.571 -10 48.571 60 -11.429 48.571 61.429 -12.857 48.571 62.857 -14.286 48.571 64.286 -15.714 48.571 65.714 -17.143 48.571 67.143 -18.571 48.571 68.571 -20 50 0 50 50 1.4286 48.571 50 2.8571 47.143 50 4.2857 45.714 50 5.7143 44.286 50 7.1429 42.857 50 8.5714 41.429 50 10 40 50 11.429 38.571 50 12.857 37.143 50 14.286 35.714 50 15.714 34.286 50 17.143 32.857 50 18.571 31.429 50 20 30 50 21.429 28.571 50 22.857 27.143 50 24.286 25.714 50 25.714 24.286 50 27.143 22.857 50 28.571 21.429 50 30 20 50 31.429 18.571 50 32.857 17.143 50 34.286 15.714 50 35.714 14.286 50 37.143 12.857 50 38.571 11.429 50 40 10 50 41.429 8.5714 50 42.857 7.1429 50 44.286 5.7143 50 45.714 4.2857 50 47.143 2.8571 50 48.571 1.4286 50 50 0 50 51.429 -1.4286 50 52.857 -2.8571 50 54.286 -4.2857 50 55.714 -5.7143 50 57.143 -7.1429 50 58.571 -8.5714 50 60 -10 50 61.429 -11.429 50 62.857 -12.857 50 64.286 -14.286 50 65.714 -15.714 50 67.143 -17.143 50 68.571 -18.571 50 70 -20 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx mt_coh_wkb xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx 1275 15 mt_coh_wkb1 mt_coh_wkb2 mt_coh_wkb3 mt_coh_wkb4 mt_coh_wkb5 mt_coh_wkb6 mt_coh_wkb7 mt_coh_wkb8 mt_coh_wkb9 mt_coh_wkb10 mt_coh_wkb11 mt_coh_wkb12 mt_coh_wkb13 mt_coh_wkb14 mt_coh_wkb15 ___________ ___________ ___________ ___________ ___________ ___________ ___________ ___________ ___________ ____________ ____________ ____________ ____________ ____________ ____________ -20.056 -20.056 -20.056 -20.056 -20.056 -20.056 -20.056 -20.056 -20.056 -20.056 -20.056 -20.056 -20.056 -20.056 -20.056 -18.592 -18.592 -18.592 -18.592 -18.592 -18.592 -18.592 -18.592 -18.592 -18.592 -18.592 -18.592 -18.592 -18.592 -18.592 -18.347 -18.286 -18.216 -18.136 -18.043 -17.935 -17.811 -17.668 -17.503 -17.313 -17.094 -16.841 -16.549 -16.212 -15.823 -17.128 -17.128 -17.128 -17.128 -17.128 -17.128 -17.128 -17.128 -17.128 -17.128 -17.128 -17.128 -17.128 -17.128 -17.128 -16.883 -16.822 -16.752 -16.671 -16.578 -16.471 -16.347 -16.204 -16.039 -15.849 -15.63 -15.376 -15.084 -14.748 -14.359 -16.921 -16.843 -16.753 -16.65 -16.53 -16.392 -16.233 -16.05 -15.838 -15.594 -15.312 -14.988 -14.613 -14.18 -13.682 -15.663 -15.663 -15.663 -15.663 -15.663 -15.663 -15.663 -15.663 -15.663 -15.663 -15.663 -15.663 -15.663 -15.663 -15.663 -15.418 -15.358 -15.288 -15.207 -15.114 -15.007 -14.883 -14.74 -14.575 -14.385 -14.165 -13.912 -13.62 -13.283 -12.895 -15.457 -15.379 -15.289 -15.185 -15.066 -14.928 -14.769 -14.585 -14.374 -14.13 -13.848 -13.523 -13.148 -12.716 -12.218 -15.527 -15.437 -15.333 -15.213 -15.075 -14.915 -14.731 -14.519 -14.274 -13.991 -13.666 -13.29 -12.856 -12.356 -11.779 -14.199 -14.199 -14.199 -14.199 -14.199 -14.199 -14.199 -14.199 -14.199 -14.199 -14.199 -14.199 -14.199 -14.199 -14.199 -13.954 -13.894 -13.824 -13.743 -13.65 -13.542 -13.418 -13.275 -13.111 -12.92 -12.701 -12.448 -12.156 -11.819 -11.43 -13.992 -13.914 -13.825 -13.721 -13.601 -13.464 -13.305 -13.121 -12.91 -12.665 -12.384 -12.059 -11.684 -11.252 -10.753 -14.063 -13.972 -13.869 -13.749 -13.61 -13.451 -13.267 -13.055 -12.81 -12.527 -12.201 -11.825 -11.392 -10.892 -10.315 -14.149 -14.049 -13.933 -13.8 -13.647 -13.47 -13.266 -13.031 -12.759 -12.446 -12.084 -11.667 -11.186 -10.632 -9.9915 -12.735 -12.735 -12.735 -12.735 -12.735 -12.735 -12.735 -12.735 -12.735 -12.735 -12.735 -12.735 -12.735 -12.735 -12.735 -12.49 -12.429 -12.359 -12.279 -12.185 -12.078 -11.954 -11.811 -11.646 -11.456 -11.237 -10.984 -10.692 -10.355 -9.9662 -12.528 -12.45 -12.36 -12.257 -12.137 -11.999 -11.84 -11.657 -11.445 -11.201 -10.919 -10.595 -10.22 -9.7876 -9.2889 -12.598 -12.508 -12.404 -12.284 -12.146 -11.987 -11.803 -11.59 -11.345 -11.063 -10.737 -10.361 -9.9275 -9.4273 -8.8502 -12.684 -12.584 -12.469 -12.336 -12.183 -12.006 -11.802 -11.566 -11.295 -10.981 -10.62 -10.203 -9.7221 -9.1672 -8.5272 -12.78 -12.672 -12.547 -12.403 -12.236 -12.045 -11.823 -11.568 -11.274 -10.934 -10.543 -10.091 -9.5698 -8.9686 -8.2751 -11.271 -11.271 -11.271 -11.271 -11.271 -11.271 -11.271 -11.271 -11.271 -11.271 -11.271 -11.271 -11.271 -11.271 -11.271 -11.026 -10.965 -10.895 -10.814 -10.721 -10.614 -10.49 -10.347 -10.182 -9.9918 -9.7724 -9.5193 -9.2273 -8.8904 -8.5019 -11.064 -10.986 -10.896 -10.792 -10.673 -10.535 -10.376 -10.193 -9.9809 -9.7368 -9.4552 -9.1304 -8.7556 -8.3233 -7.8247 -11.134 -11.044 -10.94 -10.82 -10.682 -10.522 -10.338 -10.126 -9.8811 -9.5986 -9.2728 -8.8968 -8.4632 -7.963 -7.3859 -11.22 -11.12 -11.005 -10.872 -10.718 -10.542 -10.337 -10.102 -9.8304 -9.5171 -9.1556 -8.7387 -8.2578 -7.703 -7.0629 -11.316 -11.207 -11.082 -10.938 -10.772 -10.58 -10.359 -10.104 -9.8097 -9.4702 -9.0785 -8.6267 -8.1056 -7.5043 -6.8108 -11.418 -11.302 -11.169 -11.015 -10.837 -10.633 -10.396 -10.124 -9.8097 -9.4472 -9.029 -8.5465 -7.99 -7.348 -6.6074 -9.8064 -9.8064 -9.8064 -9.8064 -9.8064 -9.8064 -9.8064 -9.8064 -9.8064 -9.8064 -9.8064 -9.8064 -9.8064 -9.8064 -9.8064 -9.5613 -9.5007 -9.4307 -9.35 -9.2569 -9.1495 -9.0255 -8.8826 -8.7177 -8.5275 -8.3081 -8.055 -7.763 -7.4262 -7.0376 -9.5994 -9.5215 -9.4317 -9.3281 -9.2086 -9.0708 -8.9117 -8.7283 -8.5167 -8.2725 -7.9909 -7.6661 -7.2913 -6.859 -6.3604 -9.6697 -9.5796 -9.4757 -9.3558 -9.2175 -9.058 -8.874 -8.6617 -8.4168 -8.1343 -7.8085 -7.4326 -6.9989 -6.4987 -5.9217 -9.7557 -9.6558 -9.5405 -9.4076 -9.2542 -9.0773 -8.8731 -8.6377 -8.3661 -8.0528 -7.6914 -7.2744 -6.7935 -6.2387 -5.5987 -9.8513 -9.743 -9.6181 -9.474 -9.3078 -9.1161 -8.8949 -8.6398 -8.3454 -8.0059 -7.6143 -7.1625 -6.6413 -6.0401 -5.3465 -9.9534 -9.8378 -9.7045 -9.5506 -9.3731 -9.1684 -8.9322 -8.6597 -8.3455 -7.9829 -7.5647 -7.0822 -6.5257 -5.8837 -5.1431 -10.06 -9.9383 -9.7973 -9.6346 -9.447 -9.2306 -8.981 -8.693 -8.3607 -7.9775 -7.5354 -7.0254 -6.4371 -5.7585 -4.9756 -8.3421 -8.3421 -8.3421 -8.3421 -8.3421 -8.3421 -8.3421 -8.3421 -8.3421 -8.3421 -8.3421 -8.3421 -8.3421 -8.3421 -8.3421 -8.0971 -8.0364 -7.9664 -7.8857 -7.7926 -7.6852 -7.5613 -7.4183 -7.2534 -7.0632 -6.8438 -6.5907 -6.2987 -5.9619 -5.5733 -8.1351 -8.0573 -7.9674 -7.8638 -7.7443 -7.6065 -7.4474 -7.264 -7.0524 -6.8083 -6.5266 -6.2018 -5.827 -5.3948 -4.8961 -8.2054 -8.1153 -8.0114 -7.8915 -7.7533 -7.5937 -7.4097 -7.1974 -6.9525 -6.6701 -6.3442 -5.9683 -5.5346 -5.0344 -4.4574 -8.2914 -8.1915 -8.0762 -7.9433 -7.7899 -7.613 -7.4089 -7.1734 -6.9018 -6.5885 -6.2271 -5.8101 -5.3292 -4.7744 -4.1344 -8.387 -8.2787 -8.1538 -8.0097 -7.8435 -7.6518 -7.4306 -7.1755 -6.8812 -6.5416 -6.15 -5.6982 -5.177 -4.5758 -3.8822 -8.4892 -8.3735 -8.2402 -8.0863 -7.9088 -7.7041 -7.4679 -7.1955 -6.8812 -6.5186 -6.1004 -5.6179 -5.0614 -4.4194 -3.6788 -8.5962 -8.474 -8.333 -8.1704 -7.9827 -7.7663 -7.5167 -7.2287 -6.8964 -6.5132 -6.0711 -5.5611 -4.9728 -4.2942 -3.5113 -8.707 -8.5788 -8.4308 -8.2602 -8.0633 -7.8363 -7.5743 -7.2721 -6.9236 -6.5214 -6.0576 -5.5225 -4.9052 -4.1932 -3.3717 -6.8778 -6.8778 -6.8778 -6.8778 -6.8778 -6.8778 -6.8778 -6.8778 -6.8778 -6.8778 -6.8778 -6.8778 -6.8778 -6.8778 -6.8778 -6.6328 -6.5721 -6.5021 -6.4214 -6.3283 -6.2209 -6.097 -5.954 -5.7891 -5.5989 -5.3795 -5.1264 -4.8344 -4.4976 -4.109 -6.6708 -6.593 -6.5032 -6.3996 -6.2801 -6.1422 -5.9832 -5.7997 -5.5881 -5.344 -5.0624 -4.7375 -4.3628 -3.9305 -3.4318 -6.7411 -6.6511 -6.5471 -6.4273 -6.289 -6.1294 -5.9454 -5.7331 -5.4883 -5.2058 -4.8799 -4.504 -4.0704 -3.5701 -2.9931 -6.8271 -6.7272 -6.612 -6.479 -6.3256 -6.1487 -5.9446 -5.7091 -5.4375 -5.1242 -4.7628 -4.3459 -3.8649 -3.3101 -2.6701 -6.9227 -6.8144 -6.6895 -6.5454 -6.3792 -6.1875 -5.9663 -5.7112 -5.4169 -5.0773 -4.6857 -4.2339 -3.7127 -3.1115 -2.4179 -7.0249 -6.9093 -6.7759 -6.622 -6.4445 -6.2398 -6.0036 -5.7312 -5.4169 -5.0543 -4.6361 -4.1537 -3.5971 -2.9551 -2.2145 -7.1319 -7.0097 -6.8687 -6.7061 -6.5185 -6.302 -6.0524 -5.7644 -5.4322 -5.0489 -4.6068 -4.0969 -3.5086 -2.8299 -2.0471 -7.2427 -7.1145 -6.9666 -6.7959 -6.5991 -6.372 -6.11 -5.8078 -5.4593 -5.0572 -4.5933 -4.0582 -3.4409 -2.7289 -1.9075 -7.3565 -7.2228 -7.0684 -6.8904 -6.685 -6.4481 -6.1748 -5.8595 -5.4958 -5.0763 -4.5924 -4.0341 -3.3901 -2.6472 -1.7902 -5.4135 -5.4135 -5.4135 -5.4135 -5.4135 -5.4135 -5.4135 -5.4135 -5.4135 -5.4135 -5.4135 -5.4135 -5.4135 -5.4135 -5.4135 -5.1685 -5.1078 -5.0379 -4.9571 -4.864 -4.7566 -4.6327 -4.4898 -4.3249 -4.1347 -3.9152 -3.6621 -3.3701 -3.0333 -2.6448 -5.2065 -5.1287 -5.0389 -4.9353 -4.8158 -4.6779 -4.5189 -4.3354 -4.1238 -3.8797 -3.5981 -3.2732 -2.8985 -2.4662 -1.9675 -5.2769 -5.1868 -5.0828 -4.963 -4.8247 -4.6652 -4.4811 -4.2688 -4.024 -3.7415 -3.4156 -3.0397 -2.6061 -2.1058 -1.5288 -5.3628 -5.2629 -5.1477 -5.0147 -4.8613 -4.6844 -4.4803 -4.2448 -3.9732 -3.6599 -3.2985 -2.8816 -2.4006 -1.8458 -1.2058 -5.4584 -5.3501 -5.2252 -5.0812 -4.915 -4.7232 -4.502 -4.2469 -3.9526 -3.6131 -3.2214 -2.7696 -2.2484 -1.6472 -0.95366 -5.5606 -5.445 -5.3116 -5.1577 -4.9803 -4.7755 -4.5393 -4.2669 -3.9526 -3.59 -3.1718 -2.6894 -2.1328 -1.4908 -0.75023 -5.6676 -5.5454 -5.4044 -5.2418 -5.0542 -4.8378 -4.5881 -4.3001 -3.9679 -3.5846 -3.1425 -2.6326 -2.0443 -1.3656 -0.58278 -5.7784 -5.6502 -5.5023 -5.3316 -5.1348 -4.9077 -4.6457 -4.3436 -3.995 -3.5929 -3.129 -2.5939 -1.9766 -1.2646 -0.44318 -5.8923 -5.7585 -5.6041 -5.4261 -5.2207 -4.9838 -4.7105 -4.3952 -4.0316 -3.612 -3.1281 -2.5698 -1.9258 -1.1829 -0.32592 -6.0086 -5.8696 -5.7093 -5.5244 -5.3111 -5.065 -4.7811 -4.4537 -4.076 -3.6402 -3.1375 -2.5577 -1.8888 -1.1172 -0.22705 -3.9492 -3.9492 -3.9492 -3.9492 -3.9492 -3.9492 -3.9492 -3.9492 -3.9492 -3.9492 -3.9492 -3.9492 -3.9492 -3.9492 -3.9492 -3.7042 -3.6435 -3.5736 -3.4928 -3.3997 -3.2923 -3.1684 -3.0255 -2.8606 -2.6704 -2.4509 -2.1978 -1.9058 -1.569 -1.1805 -3.7422 -3.6644 -3.5746 -3.471 -3.3515 -3.2136 -3.0546 -2.8711 -2.6595 -2.4154 -2.1338 -1.8089 -1.4342 -1.0019 -0.50323 -3.8126 -3.7225 -3.6186 -3.4987 -3.3604 -3.2009 -3.0168 -2.8046 -2.5597 -2.2772 -1.9513 -1.5754 -1.1418 -0.64156 -0.064512 -3.8986 -3.7986 -3.6834 -3.5504 -3.397 -3.2201 -3.016 -2.7806 -2.509 -2.1956 -1.8342 -1.4173 -0.93634 -0.38153 0.25848 -3.9941 -3.8859 -3.761 -3.6169 -3.4507 -3.2589 -3.0378 -2.7826 -2.4883 -2.1488 -1.7571 -1.3053 -0.78413 -0.18292 0.51063 -4.0963 -3.9807 -3.8473 -3.6935 -3.516 -3.3112 -3.075 -2.8026 -2.4883 -2.1258 -1.7075 -1.2251 -0.66854 -0.02654 0.71405 -4.2033 -4.0811 -3.9401 -3.7775 -3.5899 -3.3735 -3.1238 -2.8358 -2.5036 -2.1204 -1.6783 -1.1683 -0.57998 0.098655 0.88151 -4.3141 -4.1859 -4.038 -3.8673 -3.6705 -3.4434 -3.1815 -2.8793 -2.5307 -2.1286 -1.6647 -1.1296 -0.51236 0.1997 1.0211 -4.428 -4.2942 -4.1399 -3.9618 -3.7564 -3.5195 -3.2462 -2.931 -2.5673 -2.1477 -1.6638 -1.1055 -0.46152 0.28138 1.1384 -4.5443 -4.4053 -4.245 -4.0601 -3.8468 -3.6007 -3.3169 -2.9894 -2.6117 -2.1759 -1.6733 -1.0934 -0.4245 0.34712 1.2372 -4.6627 -4.5189 -4.353 -4.1616 -3.9409 -3.6862 -3.3924 -3.0535 -2.6626 -2.2117 -1.6914 -1.0914 -0.3991 0.39945 1.3206 -2.4849 -2.4849 -2.4849 -2.4849 -2.4849 -2.4849 -2.4849 -2.4849 -2.4849 -2.4849 -2.4849 -2.4849 -2.4849 -2.4849 -2.4849 -2.2399 -2.1793 -2.1093 -2.0286 -1.9354 -1.828 -1.7041 -1.5612 -1.3963 -1.2061 -0.98666 -0.73354 -0.44156 -0.10473 0.28381 -2.278 -2.2001 -2.1103 -2.0067 -1.8872 -1.7493 -1.5903 -1.4069 -1.1952 -0.95111 -0.6695 -0.34464 0.030098 0.46239 0.96106 -2.3483 -2.2582 -2.1543 -2.0344 -1.8961 -1.7366 -1.5526 -1.3403 -1.0954 -0.81291 -0.48704 -0.11113 0.3225 0.82273 1.3998 -2.4343 -2.3344 -2.2191 -2.0861 -1.9328 -1.7558 -1.5517 -1.3163 -1.0447 -0.73136 -0.36993 0.046995 0.52795 1.0828 1.7228 -2.5298 -2.4216 -2.2967 -2.1526 -1.9864 -1.7946 -1.5735 -1.3183 -1.024 -0.68449 -0.29283 0.15897 0.68015 1.2814 1.9749 -2.632 -2.5164 -2.383 -2.2292 -2.0517 -1.8469 -1.6108 -1.3383 -1.024 -0.66147 -0.24325 0.2392 0.79574 1.4377 2.1783 -2.7391 -2.6168 -2.4759 -2.3132 -2.1256 -1.9092 -1.6595 -1.3715 -1.0393 -0.65607 -0.21398 0.29601 0.8843 1.5629 2.3458 -2.8499 -2.7216 -2.5737 -2.4031 -2.2062 -1.9791 -1.7172 -1.415 -1.0664 -0.6643 -0.20043 0.33466 0.95193 1.664 2.4854 -2.9637 -2.8299 -2.6756 -2.4975 -2.2922 -2.0552 -1.7819 -1.4667 -1.103 -0.68346 -0.19951 0.35876 1.0028 1.7457 2.6027 -3.08 -2.941 -2.7807 -2.5958 -2.3825 -2.1364 -1.8526 -1.5251 -1.1474 -0.71163 -0.20897 0.37089 1.0398 1.8114 2.7015 -3.1984 -3.0546 -2.8887 -2.6973 -2.4766 -2.2219 -1.9281 -1.5893 -1.1983 -0.74737 -0.22716 0.37293 1.0652 1.8637 2.7849 -3.3186 -3.1702 -2.9991 -2.8016 -2.5738 -2.311 -2.0079 -1.6583 -1.2549 -0.78958 -0.25282 0.36637 1.0807 1.9046 2.8551 -1.0206 -1.0206 -1.0206 -1.0206 -1.0206 -1.0206 -1.0206 -1.0206 -1.0206 -1.0206 -1.0206 -1.0206 -1.0206 -1.0206 -1.0206 -0.77563 -0.71497 -0.645 -0.56428 -0.47116 -0.36374 -0.23983 -0.096894 0.067995 0.25821 0.47763 0.73074 1.0227 1.3596 1.7481 -0.81367 -0.73582 -0.64601 -0.54242 -0.42291 -0.28505 -0.12602 0.057433 0.26906 0.51318 0.79479 1.1196 1.4944 1.9267 2.4253 -0.884 -0.79391 -0.68999 -0.57011 -0.43182 -0.2723 -0.088275 0.12401 0.36889 0.65138 0.97724 1.3532 1.7868 2.287 2.8641 -0.96999 -0.87007 -0.75481 -0.62185 -0.46847 -0.29154 -0.087433 0.14801 0.41962 0.73293 1.0944 1.5113 1.9922 2.547 3.1871 -1.0656 -0.95729 -0.83239 -0.6883 -0.52209 -0.33036 -0.10919 0.14595 0.44028 0.7798 1.1715 1.6233 2.1444 2.7457 3.4392 -1.1677 -1.0521 -0.91874 -0.76488 -0.5874 -0.38266 -0.14648 0.12597 0.44026 0.80281 1.221 1.7035 2.26 2.902 3.6426 -1.2748 -1.1526 -1.0116 -0.84893 -0.66132 -0.4449 -0.19524 0.092758 0.42498 0.80822 1.2503 1.7603 2.3486 3.0272 3.8101 -1.3856 -1.2573 -1.1094 -0.93877 -0.74191 -0.51483 -0.25288 0.049295 0.39788 0.79999 1.2639 1.7989 2.4162 3.1283 3.9497 mt_coh_wkb1 mt_coh_wkb2 mt_coh_wkb3 mt_coh_wkb4 mt_coh_wkb5 mt_coh_wkb6 mt_coh_wkb7 mt_coh_wkb8 mt_coh_wkb9 mt_coh_wkb10 mt_coh_wkb11 mt_coh_wkb12 mt_coh_wkb13 mt_coh_wkb14 mt_coh_wkb15 ___________ ___________ ___________ ___________ ___________ ___________ ___________ ___________ ___________ ____________ ____________ ____________ ____________ ____________ ____________ 43.295 43.537 43.817 44.14 44.512 44.942 45.437 46.009 46.668 47.429 48.307 49.319 50.486 51.833 53.387 50.229 50.229 50.229 50.229 50.229 50.229 50.229 50.229 50.229 50.229 50.229 50.229 50.229 50.229 50.229 50.474 50.535 50.605 50.686 50.779 50.886 51.01 51.153 51.318 51.508 51.728 51.981 52.273 52.61 52.998 50.436 50.514 50.604 50.708 50.827 50.965 51.124 51.307 51.519 51.763 52.045 52.37 52.744 53.177 53.675 50.366 50.456 50.56 50.68 50.818 50.978 51.162 51.374 51.619 51.901 52.227 52.603 53.037 53.537 54.114 50.28 50.38 50.495 50.628 50.782 50.958 51.163 51.398 51.67 51.983 52.344 52.761 53.242 53.797 54.437 50.184 50.293 50.418 50.562 50.728 50.92 51.141 51.396 51.69 52.03 52.421 52.873 53.394 53.996 54.689 50.082 50.198 50.331 50.485 50.663 50.867 51.104 51.376 51.69 52.053 52.471 52.953 53.51 54.152 54.893 49.975 50.097 50.238 50.401 50.589 50.805 51.055 51.343 51.675 52.058 52.5 53.01 53.599 54.277 55.06 49.864 49.993 50.141 50.311 50.508 50.735 50.997 51.299 51.648 52.05 52.514 53.049 53.666 54.378 55.2 49.751 49.884 50.039 50.217 50.422 50.659 50.932 51.248 51.611 52.031 52.515 53.073 53.717 54.46 55.317 49.634 49.773 49.934 50.118 50.332 50.578 50.862 51.189 51.567 52.003 52.505 53.085 53.754 54.526 55.416 49.516 49.66 49.826 50.017 50.238 50.492 50.786 51.125 51.516 51.967 52.487 53.087 53.779 54.578 55.499 49.396 49.544 49.715 49.913 50.14 50.403 50.706 51.056 51.459 51.925 52.461 53.081 53.795 54.619 55.569 49.274 49.427 49.603 49.806 50.041 50.311 50.623 50.983 51.398 51.877 52.429 53.067 53.802 54.65 55.628 49.151 49.308 49.489 49.697 49.938 50.216 50.536 50.906 51.332 51.824 52.392 53.046 53.801 54.672 55.677 49.026 49.187 49.373 49.587 49.834 50.118 50.447 50.826 51.263 51.767 52.349 53.02 53.794 54.687 55.717 48.901 49.066 49.256 49.475 49.727 50.019 50.355 50.743 51.19 51.706 52.301 52.988 53.78 54.694 55.749 48.775 48.943 49.137 49.361 49.619 49.917 50.261 50.657 51.114 51.642 52.25 52.952 53.762 54.696 55.773 48.648 48.819 49.017 49.246 49.509 49.813 50.164 50.569 51.036 51.574 52.195 52.912 53.738 54.692 55.792 48.52 48.695 48.897 49.13 49.398 49.708 50.066 50.479 50.955 51.504 52.137 52.867 53.71 54.682 55.804 48.391 48.569 48.775 49.012 49.286 49.602 49.966 50.386 50.871 51.43 52.076 52.82 53.678 54.669 55.811 48.261 48.443 48.652 48.894 49.172 49.494 49.865 50.292 50.786 51.355 52.011 52.769 53.642 54.65 55.813 48.131 48.316 48.529 48.774 49.058 49.385 49.762 50.197 50.698 51.277 51.945 52.715 53.603 54.628 55.81 48.001 48.188 48.405 48.654 48.942 49.274 49.657 50.099 50.609 51.197 51.876 52.658 53.561 54.602 55.804 47.87 48.06 48.28 48.533 48.825 49.163 49.552 50.001 50.518 51.115 51.804 52.599 53.516 54.573 55.793 47.738 47.931 48.154 48.411 48.708 49.05 49.445 49.9 50.426 51.032 51.731 52.537 53.468 54.541 55.779 47.606 47.802 48.028 48.289 48.59 48.937 49.337 49.799 50.332 50.947 51.656 52.473 53.417 54.505 55.761 47.473 47.672 47.901 48.166 48.471 48.822 49.228 49.697 50.237 50.86 51.578 52.408 53.364 54.467 55.74 47.34 47.542 47.774 48.042 48.351 48.707 49.118 49.593 50.14 50.771 51.5 52.34 53.309 54.426 55.716 47.207 47.411 47.646 47.917 48.23 48.591 49.008 49.488 50.042 50.682 51.419 52.27 53.251 54.383 55.689 47.073 47.28 47.518 47.792 48.109 48.475 48.896 49.382 49.943 50.591 51.337 52.198 53.192 54.338 55.66 46.939 47.148 47.389 47.667 47.987 48.357 48.784 49.276 49.844 50.498 51.254 52.125 53.13 54.29 55.627 46.805 47.016 47.26 47.541 47.865 48.239 48.671 49.168 49.743 50.405 51.169 52.05 53.067 54.24 55.593 46.67 46.884 47.13 47.414 47.742 48.12 48.557 49.06 49.641 50.31 51.083 51.974 53.002 54.188 55.556 46.535 46.751 47 47.287 47.619 48.001 48.442 48.951 49.538 50.215 50.996 51.896 52.936 54.134 55.517 46.4 46.618 46.87 47.16 47.495 47.881 48.327 48.841 49.434 50.118 50.907 51.817 52.868 54.079 55.476 46.264 46.485 46.739 47.032 47.371 47.761 48.211 48.73 49.329 50.02 50.818 51.737 52.798 54.022 55.433 46.129 46.351 46.608 46.904 47.246 47.64 48.095 48.619 49.224 49.922 50.727 51.656 52.727 53.963 55.388 45.993 46.217 46.477 46.776 47.121 47.518 47.977 48.507 49.118 49.822 50.635 51.573 52.655 53.902 55.342 45.856 46.083 46.345 46.647 46.995 47.397 47.86 48.394 49.011 49.722 50.543 51.489 52.581 53.84 55.293 45.72 45.949 46.213 46.517 46.869 47.274 47.742 48.281 48.903 49.621 50.449 51.404 52.506 53.777 55.243 45.583 45.814 46.081 46.388 46.742 47.151 47.623 48.167 48.795 49.519 50.355 51.318 52.43 53.712 55.191 45.446 45.679 45.948 46.258 46.616 47.028 47.504 48.053 48.686 49.417 50.259 51.231 52.353 53.646 55.138 45.309 45.544 45.815 46.128 46.488 46.904 47.384 47.938 48.577 49.313 50.163 51.143 52.274 53.579 55.084 45.172 45.409 45.682 45.997 46.361 46.78 47.264 47.823 48.466 49.209 50.066 51.055 52.195 53.51 55.028 45.034 45.273 45.549 45.867 46.233 46.656 47.144 47.707 48.356 49.105 49.968 50.965 52.114 53.441 54.97 44.897 45.138 45.415 45.736 46.105 46.531 47.023 47.59 48.244 48.999 49.87 50.874 52.033 53.37 54.911 44.759 45.002 45.281 45.604 45.977 46.406 46.902 47.473 48.133 48.893 49.771 50.783 51.951 53.298 54.851 44.621 44.865 45.147 45.473 45.848 46.281 46.78 47.356 48.02 48.787 49.671 50.691 51.867 53.225 54.79 51.694 51.694 51.694 51.694 51.694 51.694 51.694 51.694 51.694 51.694 51.694 51.694 51.694 51.694 51.694 51.939 51.999 52.069 52.15 52.243 52.351 52.474 52.617 52.782 52.972 53.192 53.445 53.737 54.074 54.462 51.901 51.978 52.068 52.172 52.291 52.429 52.588 52.772 52.983 53.227 53.509 53.834 54.209 54.641 55.14 51.83 51.92 52.024 52.144 52.282 52.442 52.626 52.838 53.083 53.366 53.692 54.067 54.501 55.001 55.578 51.744 51.844 51.959 52.092 52.246 52.423 52.627 52.862 53.134 53.447 53.809 54.226 54.707 55.261 55.901 51.649 51.757 51.882 52.026 52.192 52.384 52.605 52.86 53.155 53.494 53.886 54.338 54.859 55.46 56.153 51.547 51.662 51.796 51.949 52.127 52.332 52.568 52.84 53.155 53.517 53.935 54.418 54.974 55.616 56.357 51.44 51.562 51.703 51.865 52.053 52.269 52.519 52.807 53.139 53.523 53.965 54.475 55.063 55.742 56.524 51.329 51.457 51.605 51.776 51.972 52.199 52.461 52.764 53.112 53.514 53.978 54.513 55.131 55.843 56.664 51.215 51.349 51.503 51.681 51.886 52.123 52.397 52.712 53.076 53.495 53.979 54.537 55.181 55.924 56.781 51.099 51.238 51.398 51.583 51.796 52.042 52.326 52.653 53.031 53.467 53.97 54.549 55.218 55.99 56.88 50.98 51.124 51.29 51.481 51.702 51.957 52.25 52.589 52.98 53.431 53.951 54.552 55.244 56.042 56.963 50.86 51.008 51.18 51.377 51.605 51.868 52.171 52.52 52.924 53.389 53.926 54.545 55.259 56.083 57.034 50.738 50.891 51.067 51.27 51.505 51.775 52.087 52.447 52.862 53.341 53.894 54.531 55.266 56.114 57.092 50.615 50.772 50.953 51.162 51.402 51.68 52.001 52.37 52.797 53.288 53.856 54.51 55.265 56.136 57.141 50.491 50.652 50.837 51.051 51.298 51.583 51.911 52.29 52.727 53.231 53.813 54.484 55.258 56.151 57.181 50.365 50.53 50.72 50.939 51.191 51.483 51.819 52.207 52.654 53.17 53.766 54.452 55.245 56.159 57.213 50.239 50.407 50.601 50.825 51.083 51.381 51.725 52.121 52.578 53.106 53.714 54.416 55.226 56.16 57.238 50.112 50.284 50.482 50.71 50.974 51.278 51.629 52.033 52.5 53.038 53.659 54.376 55.202 56.156 57.256 49.984 50.159 50.361 50.594 50.863 51.173 51.53 51.943 52.419 52.968 53.601 54.332 55.175 56.147 57.268 49.855 50.033 50.239 50.476 50.75 51.066 51.43 51.851 52.335 52.895 53.54 54.284 55.142 56.133 57.275 49.726 49.907 50.117 50.358 50.637 50.958 51.329 51.757 52.25 52.819 53.476 54.233 55.107 56.115 57.277 49.596 49.78 49.993 50.239 50.522 50.849 51.226 51.661 52.163 52.741 53.409 54.179 55.068 56.092 57.275 49.465 49.653 49.869 50.118 50.406 50.738 51.122 51.564 52.073 52.661 53.34 54.122 55.025 56.067 57.268 49.334 49.524 49.744 49.997 50.29 50.627 51.016 51.465 51.982 52.58 53.269 54.063 54.98 56.037 57.257 49.202 49.395 49.618 49.876 50.172 50.514 50.909 51.365 51.89 52.496 53.195 54.002 54.932 56.005 57.243 49.07 49.266 49.492 49.753 50.054 50.401 50.801 51.263 51.796 52.411 53.12 53.938 54.881 55.97 57.225 48.938 49.136 49.365 49.63 49.935 50.287 50.693 51.161 51.701 52.324 53.043 53.872 54.828 55.932 57.204 48.805 49.006 49.238 49.506 49.815 50.172 50.583 51.057 51.604 52.236 52.964 53.804 54.773 55.891 57.18 48.671 48.875 49.11 49.382 49.695 50.056 50.472 50.952 51.507 52.146 52.883 53.734 54.715 55.848 57.153 48.538 48.744 48.982 49.257 49.573 49.939 50.36 50.847 51.408 52.055 52.801 53.663 54.656 55.802 57.124 48.404 48.612 48.853 49.131 49.452 49.821 50.248 50.74 51.308 51.963 52.718 53.589 54.595 55.754 57.092 48.269 48.48 48.724 49.005 49.329 49.703 50.135 50.633 51.207 51.869 52.633 53.515 54.531 55.704 57.057 48.134 48.348 48.594 48.879 49.207 49.585 50.021 50.524 51.105 51.775 52.547 53.438 54.466 55.652 57.02 47.999 48.215 48.464 48.752 49.083 49.465 49.906 50.415 51.002 51.679 52.46 53.361 54.4 55.599 56.982 47.864 48.082 48.334 48.624 48.959 49.346 49.791 50.305 50.898 51.582 52.371 53.282 54.332 55.543 56.941 47.729 47.949 48.203 48.497 48.835 49.225 49.675 50.195 50.794 51.485 52.282 53.201 54.262 55.486 56.898 47.593 47.816 48.072 48.368 48.71 49.104 49.559 50.083 50.688 51.386 52.191 53.12 54.191 55.427 56.853 47.457 47.682 47.941 48.24 48.585 48.983 49.442 49.971 50.582 51.287 52.1 53.037 54.119 55.367 56.806 47.321 47.547 47.809 48.111 48.459 48.861 49.324 49.859 50.475 51.186 52.007 52.953 54.045 55.305 56.758 47.184 47.413 47.677 47.982 48.333 48.738 49.206 49.745 50.368 51.085 51.913 52.868 53.97 55.241 56.707 47.048 47.278 47.545 47.852 48.207 48.616 49.087 49.632 50.259 50.983 51.819 52.783 53.894 55.177 56.656 46.911 47.144 47.412 47.722 48.08 48.492 48.968 49.517 50.15 50.881 51.724 52.696 53.817 55.11 56.603 46.774 47.008 47.279 47.592 47.953 48.369 48.849 49.402 50.041 50.778 51.627 52.608 53.739 55.043 56.548 46.636 46.873 47.146 47.462 47.825 48.245 48.729 49.287 49.931 50.674 51.53 52.519 53.659 54.975 56.492 46.499 46.738 47.013 47.331 47.697 48.12 48.608 49.171 49.82 50.569 51.433 52.429 53.579 54.905 56.434 46.361 46.602 46.879 47.2 47.569 47.996 48.487 49.054 49.709 50.464 51.334 52.339 53.497 54.834 56.376 46.223 46.466 46.746 47.069 47.441 47.87 48.366 48.938 49.597 50.358 51.235 52.247 53.415 54.762 56.316 46.085 46.33 46.612 46.937 47.312 47.745 48.244 48.82 49.485 50.251 51.135 52.155 53.332 54.689 56.255 45.947 46.193 46.477 46.805 47.183 47.619 48.122 48.702 49.372 50.144 51.035 52.062 53.248 54.615 56.192
IWKZ Interpolation Cash-on-hand Interpolation Grid
For the iwkz problems, we solve the problem along a grid of cash-on-hand values, the interpolate to find v(k',b',z) at (k',b') choices. Crucially, we have to coh matrxies
fl_max_mt_coh = max(max(mt_coh_wkb)); fl_min_mt_coh = min(min(mt_coh_wkb)); it_coh_interp_n = (fl_max_mt_coh-fl_min_mt_coh)/(fl_coh_interp_grid_gap); ar_interp_coh_grid = linspace(fl_min_mt_coh, fl_max_mt_coh, it_coh_interp_n); [mt_interp_coh_grid_mesh_z, mt_z_mesh_coh_interp_grid] = ndgrid(ar_interp_coh_grid, ar_z);
IWKZ Interpolation Consumption Grid
We also interpolate over consumption to speed the program up. We only solve for u(c) at this grid for the iwkz problmes, and then interpolate other c values.
fl_c_max = max(max(mt_coh_wkb)) - fl_b_bd; it_interp_c_grid_n = (fl_c_max-fl_c_min)/(it_c_interp_grid_gap); ar_interp_c_grid = linspace(fl_c_min, fl_c_max, it_interp_c_grid_n);
Store
armt_map = containers.Map('KeyType','char', 'ValueType','any'); armt_map('ar_w') = ar_w; armt_map('ar_k') = ar_k; armt_map('mt_z_trans') = mt_z_trans; armt_map('ar_stationary') = ar_stationary; armt_map('ar_z') = ar_z; armt_map('mt_k_wth_na') = mt_k_wth_na; armt_map('ar_a_mw_wth_na') = ar_a_mw_wth_na; armt_map('ar_k_mw_wth_na') = ar_k_mw_wth_na; armt_map('ar_a_meshk') = ar_a_meshk; armt_map('ar_k_mesha') = ar_k_mesha; armt_map('it_ameshk_n') = length(ar_a_meshk); armt_map('mt_coh_wkb') = mt_coh_wkb; armt_map('mt_z_mesh_coh_wkb') = mt_z_mesh_coh_wkb; armt_map('ar_interp_c_grid') = ar_interp_c_grid; armt_map('ar_interp_coh_grid') = ar_interp_coh_grid; armt_map('mt_interp_coh_grid_mesh_z') = mt_interp_coh_grid_mesh_z; armt_map('mt_z_mesh_coh_interp_grid') = mt_z_mesh_coh_interp_grid; func_map = containers.Map('KeyType','char', 'ValueType','any'); func_map('f_util_log') = f_util_log; func_map('f_util_crra') = f_util_crra; func_map('f_util_standin') = f_util_standin; func_map('f_prod') = f_prod; func_map('f_inc') = f_inc; func_map('f_coh') = f_coh; func_map('f_cons') = f_cons;
Graph
if (bl_graph_funcgrids)
Graph 1: a and k choice grid graphs
figure('PaperPosition', [0 0 7 4]); hold on; chart = plot(mt_a_wth_na, mt_k_wth_na, 'blue'); clr = jet(numel(chart)); for m = 1:numel(chart) set(chart(m),'Color',clr(m,:)) end if (length(ar_w) <= 100) scatter(ar_a_meshk, ar_k_mesha, 5, 'filled'); end xline(0); yline(0); title('Choice Grids Conditional on k+a=w') ylabel('Capital Choice') xlabel({'Borrowing or Saving'}) legend2plot = fliplr([1 round(numel(chart)/3) round((2*numel(chart))/4) numel(chart)]); legendCell = cellstr(num2str(ar_w', 'k+a=%3.2f')); legend(chart(legend2plot), legendCell(legend2plot), 'Location','northeast'); grid on;
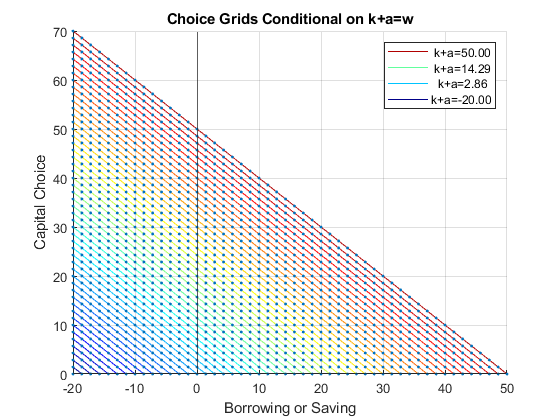
Graph 2: coh by shock
figure('PaperPosition', [0 0 7 4]); chart = plot(mt_coh_wkb); clr = jet(numel(chart)); for m = 1:numel(chart) set(chart(m),'Color',clr(m,:)) end title('Cash-on-Hand given w(k+b),k,z'); ylabel('Cash-on-Hand'); xlabel({'Index of Cash-on-Hand Discrete Point'... 'Each Segment is a w=k+b; within segment increasing k'... 'For each w and z, coh maximizing k is different'}); legend2plot = fliplr([1 round(numel(chart)/3) round((2*numel(chart))/4) numel(chart)]); legendCell = cellstr(num2str(ar_z', 'shock=%3.2f')); legend(chart(legend2plot), legendCell(legend2plot), 'Location','southeast'); grid on;
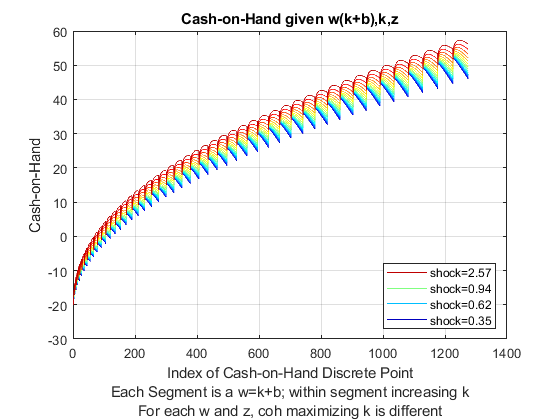
Graph 3: coh by index
figure('PaperPosition', [0 0 7 3.5]); ar_coh_kpzgrid_unique = unique(sort(mt_coh_wkb(:))); scatter(1:length(ar_coh_kpzgrid_unique), ar_coh_kpzgrid_unique); title('Cash-on-Hand given w(k+b),k,z'); ylabel('Cash-on-Hand'); xlabel({'Index of Cash-on-Hand Discrete Point'}); grid on;
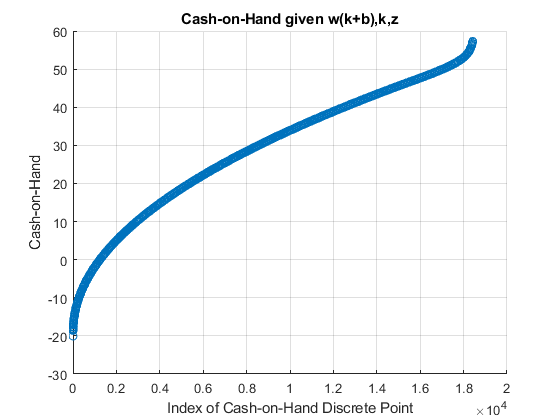
Graph 4: coh by coh
figure('PaperPosition', [0 0 7 3.5]); ar_coh_kpzgrid_unique = unique(sort(mt_coh_wkb(:))); scatter(ar_coh_kpzgrid_unique, ar_coh_kpzgrid_unique, '.'); xline(0); yline(0); title('Cash-on-Hand given w(k+b),k,z; See Clearly Sparsity Density of Grid across Z'); ylabel('Cash-on-Hand'); xlabel({'Cash-on-Hand'}); grid on;
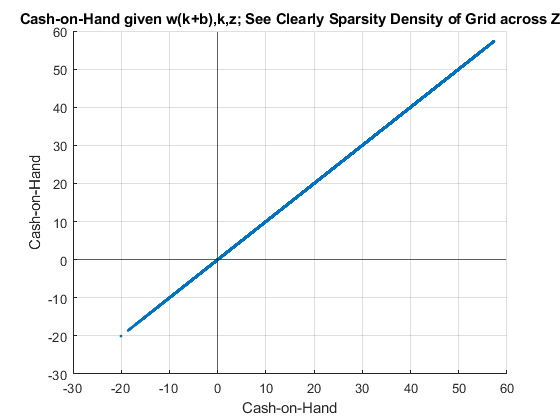
end
Display
if (bl_display_funcgrids) disp('----------------------------------------'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp('ar_z'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp(size(ar_z)); disp(ar_z); disp('----------------------------------------'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp('ar_w'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp(size(ar_w)); disp(ar_w); disp('----------------------------------------'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp('mt_z_trans'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp(size(mt_z_trans)); disp(head(array2table(mt_z_trans), 10)); disp(tail(array2table(mt_z_trans), 10)); disp('----------------------------------------'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp('ar_interp_coh_grid'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); summary(array2table(ar_interp_coh_grid')); disp('----------------------------------------'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp('ar_interp_c_grid'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); summary(array2table(ar_interp_c_grid')); disp('----------------------------------------'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp('mt_interp_coh_grid_mesh_z'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp(size(mt_interp_coh_grid_mesh_z)); disp(head(array2table(mt_interp_coh_grid_mesh_z), 10)); disp(tail(array2table(mt_interp_coh_grid_mesh_z), 10)); disp('----------------------------------------'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp('mt_a_wth_na'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp(size(mt_a_wth_na)); disp(head(array2table(mt_a_wth_na), 10)); disp(tail(array2table(mt_a_wth_na), 10)); disp('----------------------------------------'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp('mt_k_wth_na'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp(size(mt_k_wth_na)); disp(head(array2table(mt_k_wth_na), 10)); disp(tail(array2table(mt_k_wth_na), 10)); disp('----------------------------------------'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp('ar_a_meshk and ar_k_mesha'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); summary(array2table(ar_a_meshk)); summary(array2table(ar_k_mesha)); param_map_keys = keys(func_map); param_map_vals = values(func_map); for i = 1:length(func_map) st_display = strjoin(['pos =' num2str(i) '; key =' string(param_map_keys{i}) '; val =' func2str(param_map_vals{i})]); disp(st_display); end end
---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ar_z xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx 1 15 Columns 1 through 7 0.3474 0.4008 0.4623 0.5333 0.6152 0.7097 0.8186 Columns 8 through 14 0.9444 1.0894 1.2567 1.4496 1.6723 1.9291 2.2253 Column 15 2.5670 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ar_w xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx 1 50 Columns 1 through 7 -20.0000 -18.5714 -17.1429 -15.7143 -14.2857 -12.8571 -11.4286 Columns 8 through 14 -10.0000 -8.5714 -7.1429 -5.7143 -4.2857 -2.8571 -1.4286 Columns 15 through 21 0 1.4286 2.8571 4.2857 5.7143 7.1429 8.5714 Columns 22 through 28 10.0000 11.4286 12.8571 14.2857 15.7143 17.1429 18.5714 Columns 29 through 35 20.0000 21.4286 22.8571 24.2857 25.7143 27.1429 28.5714 Columns 36 through 42 30.0000 31.4286 32.8571 34.2857 35.7143 37.1429 38.5714 Columns 43 through 49 40.0000 41.4286 42.8571 44.2857 45.7143 47.1429 48.5714 Column 50 50.0000 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx mt_z_trans xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx 15 15 mt_z_trans1 mt_z_trans2 mt_z_trans3 mt_z_trans4 mt_z_trans5 mt_z_trans6 mt_z_trans7 mt_z_trans8 mt_z_trans9 mt_z_trans10 mt_z_trans11 mt_z_trans12 mt_z_trans13 mt_z_trans14 mt_z_trans15 ___________ ___________ ___________ ___________ ___________ ___________ ___________ ___________ ___________ ____________ ____________ ____________ ____________ ____________ ____________ 0.26016 0.26831 0.25551 0.14921 0.053403 0.011702 0.0015678 0.00012823 6.3911e-06 1.9381e-07 3.5701e-09 3.9889e-11 2.7001e-13 1.1102e-15 0 0.11232 0.19622 0.2763 0.23861 0.12635 0.040998 0.0081429 0.00098855 7.3236e-05 3.3054e-06 9.0736e-08 1.5125e-09 1.5289e-11 9.3592e-14 3.3307e-16 0.037073 0.10492 0.2185 0.27902 0.2185 0.10492 0.030863 0.0055558 0.00061112 4.1008e-05 1.6758e-06 4.164e-08 6.2811e-10 5.7438e-12 3.1863e-14 0.0092081 0.040998 0.12635 0.23861 0.2763 0.19622 0.085427 0.022782 0.0037167 0.0003704 2.2511e-05 8.3291e-07 1.8732e-08 2.5567e-10 2.1255e-12 0.0017026 0.011702 0.053403 0.14921 0.25551 0.26831 0.17279 0.068209 0.016489 0.0024379 0.0002201 1.2114e-05 4.058e-07 8.2598e-09 1.0277e-10 0.00023263 0.0024379 0.016489 0.068209 0.17279 0.26831 0.25551 0.14921 0.053403 0.011702 0.0015678 0.00012823 6.3911e-06 1.9381e-07 3.6102e-09 2.3363e-05 0.0003704 0.0037167 0.022782 0.085427 0.19622 0.2763 0.23861 0.12635 0.040998 0.0081429 0.00098855 7.3236e-05 3.3054e-06 9.2263e-08 1.7181e-06 4.1008e-05 0.00061112 0.0055558 0.030863 0.10492 0.2185 0.27902 0.2185 0.10492 0.030863 0.0055558 0.00061112 4.1008e-05 1.7181e-06 9.2263e-08 3.3054e-06 7.3236e-05 0.00098855 0.0081429 0.040998 0.12635 0.23861 0.2763 0.19622 0.085427 0.022782 0.0037167 0.0003704 2.3363e-05 3.6102e-09 1.9381e-07 6.3911e-06 0.00012823 0.0015678 0.011702 0.053403 0.14921 0.25551 0.26831 0.17279 0.068209 0.016489 0.0024379 0.00023263 mt_z_trans1 mt_z_trans2 mt_z_trans3 mt_z_trans4 mt_z_trans5 mt_z_trans6 mt_z_trans7 mt_z_trans8 mt_z_trans9 mt_z_trans10 mt_z_trans11 mt_z_trans12 mt_z_trans13 mt_z_trans14 mt_z_trans15 ___________ ___________ ___________ ___________ ___________ ___________ ___________ ___________ ___________ ____________ ____________ ____________ ____________ ____________ ____________ 0.00023263 0.0024379 0.016489 0.068209 0.17279 0.26831 0.25551 0.14921 0.053403 0.011702 0.0015678 0.00012823 6.3911e-06 1.9381e-07 3.6102e-09 2.3363e-05 0.0003704 0.0037167 0.022782 0.085427 0.19622 0.2763 0.23861 0.12635 0.040998 0.0081429 0.00098855 7.3236e-05 3.3054e-06 9.2263e-08 1.7181e-06 4.1008e-05 0.00061112 0.0055558 0.030863 0.10492 0.2185 0.27902 0.2185 0.10492 0.030863 0.0055558 0.00061112 4.1008e-05 1.7181e-06 9.2263e-08 3.3054e-06 7.3236e-05 0.00098855 0.0081429 0.040998 0.12635 0.23861 0.2763 0.19622 0.085427 0.022782 0.0037167 0.0003704 2.3363e-05 3.6102e-09 1.9381e-07 6.3911e-06 0.00012823 0.0015678 0.011702 0.053403 0.14921 0.25551 0.26831 0.17279 0.068209 0.016489 0.0024379 0.00023263 1.0277e-10 8.2598e-09 4.058e-07 1.2114e-05 0.0002201 0.0024379 0.016489 0.068209 0.17279 0.26831 0.25551 0.14921 0.053403 0.011702 0.0017026 2.1256e-12 2.5567e-10 1.8732e-08 8.3291e-07 2.2511e-05 0.0003704 0.0037167 0.022782 0.085427 0.19622 0.2763 0.23861 0.12635 0.040998 0.0092081 3.1909e-14 5.7438e-12 6.2811e-10 4.164e-08 1.6758e-06 4.1008e-05 0.00061112 0.0055558 0.030863 0.10492 0.2185 0.27902 0.2185 0.10492 0.037073 3.474e-16 9.3597e-14 1.5289e-11 1.5125e-09 9.0736e-08 3.3054e-06 7.3236e-05 0.00098855 0.0081429 0.040998 0.12635 0.23861 0.2763 0.19622 0.11232 2.7412e-18 1.1057e-15 2.6998e-13 3.9889e-11 3.5701e-09 1.9381e-07 6.3911e-06 0.00012823 0.0015678 0.011702 0.053403 0.14921 0.25551 0.26831 0.26016 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ar_interp_coh_grid xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Variables: Var1: 773×1 double Values: Min -20.056 Median 18.61 Max 57.277 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ar_interp_c_grid xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Variables: Var1: 772762×1 double Values: Min 0.001 Median 38.639 Max 77.277 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx mt_interp_coh_grid_mesh_z xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx 773 15 mt_interp_coh_grid_mesh_z1 mt_interp_coh_grid_mesh_z2 mt_interp_coh_grid_mesh_z3 mt_interp_coh_grid_mesh_z4 mt_interp_coh_grid_mesh_z5 mt_interp_coh_grid_mesh_z6 mt_interp_coh_grid_mesh_z7 mt_interp_coh_grid_mesh_z8 mt_interp_coh_grid_mesh_z9 mt_interp_coh_grid_mesh_z10 mt_interp_coh_grid_mesh_z11 mt_interp_coh_grid_mesh_z12 mt_interp_coh_grid_mesh_z13 mt_interp_coh_grid_mesh_z14 mt_interp_coh_grid_mesh_z15 __________________________ __________________________ __________________________ __________________________ __________________________ __________________________ __________________________ __________________________ __________________________ ___________________________ ___________________________ ___________________________ ___________________________ ___________________________ ___________________________ -20.056 -20.056 -20.056 -20.056 -20.056 -20.056 -20.056 -20.056 -20.056 -20.056 -20.056 -20.056 -20.056 -20.056 -20.056 -19.956 -19.956 -19.956 -19.956 -19.956 -19.956 -19.956 -19.956 -19.956 -19.956 -19.956 -19.956 -19.956 -19.956 -19.956 -19.856 -19.856 -19.856 -19.856 -19.856 -19.856 -19.856 -19.856 -19.856 -19.856 -19.856 -19.856 -19.856 -19.856 -19.856 -19.756 -19.756 -19.756 -19.756 -19.756 -19.756 -19.756 -19.756 -19.756 -19.756 -19.756 -19.756 -19.756 -19.756 -19.756 -19.656 -19.656 -19.656 -19.656 -19.656 -19.656 -19.656 -19.656 -19.656 -19.656 -19.656 -19.656 -19.656 -19.656 -19.656 -19.555 -19.555 -19.555 -19.555 -19.555 -19.555 -19.555 -19.555 -19.555 -19.555 -19.555 -19.555 -19.555 -19.555 -19.555 -19.455 -19.455 -19.455 -19.455 -19.455 -19.455 -19.455 -19.455 -19.455 -19.455 -19.455 -19.455 -19.455 -19.455 -19.455 -19.355 -19.355 -19.355 -19.355 -19.355 -19.355 -19.355 -19.355 -19.355 -19.355 -19.355 -19.355 -19.355 -19.355 -19.355 -19.255 -19.255 -19.255 -19.255 -19.255 -19.255 -19.255 -19.255 -19.255 -19.255 -19.255 -19.255 -19.255 -19.255 -19.255 -19.155 -19.155 -19.155 -19.155 -19.155 -19.155 -19.155 -19.155 -19.155 -19.155 -19.155 -19.155 -19.155 -19.155 -19.155 mt_interp_coh_grid_mesh_z1 mt_interp_coh_grid_mesh_z2 mt_interp_coh_grid_mesh_z3 mt_interp_coh_grid_mesh_z4 mt_interp_coh_grid_mesh_z5 mt_interp_coh_grid_mesh_z6 mt_interp_coh_grid_mesh_z7 mt_interp_coh_grid_mesh_z8 mt_interp_coh_grid_mesh_z9 mt_interp_coh_grid_mesh_z10 mt_interp_coh_grid_mesh_z11 mt_interp_coh_grid_mesh_z12 mt_interp_coh_grid_mesh_z13 mt_interp_coh_grid_mesh_z14 mt_interp_coh_grid_mesh_z15 __________________________ __________________________ __________________________ __________________________ __________________________ __________________________ __________________________ __________________________ __________________________ ___________________________ ___________________________ ___________________________ ___________________________ ___________________________ ___________________________ 56.376 56.376 56.376 56.376 56.376 56.376 56.376 56.376 56.376 56.376 56.376 56.376 56.376 56.376 56.376 56.476 56.476 56.476 56.476 56.476 56.476 56.476 56.476 56.476 56.476 56.476 56.476 56.476 56.476 56.476 56.576 56.576 56.576 56.576 56.576 56.576 56.576 56.576 56.576 56.576 56.576 56.576 56.576 56.576 56.576 56.676 56.676 56.676 56.676 56.676 56.676 56.676 56.676 56.676 56.676 56.676 56.676 56.676 56.676 56.676 56.776 56.776 56.776 56.776 56.776 56.776 56.776 56.776 56.776 56.776 56.776 56.776 56.776 56.776 56.776 56.877 56.877 56.877 56.877 56.877 56.877 56.877 56.877 56.877 56.877 56.877 56.877 56.877 56.877 56.877 56.977 56.977 56.977 56.977 56.977 56.977 56.977 56.977 56.977 56.977 56.977 56.977 56.977 56.977 56.977 57.077 57.077 57.077 57.077 57.077 57.077 57.077 57.077 57.077 57.077 57.077 57.077 57.077 57.077 57.077 57.177 57.177 57.177 57.177 57.177 57.177 57.177 57.177 57.177 57.177 57.177 57.177 57.177 57.177 57.177 57.277 57.277 57.277 57.277 57.277 57.277 57.277 57.277 57.277 57.277 57.277 57.277 57.277 57.277 57.277 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx mt_a_wth_na xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx 50 50 mt_a_wth_na1 mt_a_wth_na2 mt_a_wth_na3 mt_a_wth_na4 mt_a_wth_na5 mt_a_wth_na6 mt_a_wth_na7 mt_a_wth_na8 mt_a_wth_na9 mt_a_wth_na10 mt_a_wth_na11 mt_a_wth_na12 mt_a_wth_na13 mt_a_wth_na14 mt_a_wth_na15 mt_a_wth_na16 mt_a_wth_na17 mt_a_wth_na18 mt_a_wth_na19 mt_a_wth_na20 mt_a_wth_na21 mt_a_wth_na22 mt_a_wth_na23 mt_a_wth_na24 mt_a_wth_na25 mt_a_wth_na26 mt_a_wth_na27 mt_a_wth_na28 mt_a_wth_na29 mt_a_wth_na30 mt_a_wth_na31 mt_a_wth_na32 mt_a_wth_na33 mt_a_wth_na34 mt_a_wth_na35 mt_a_wth_na36 mt_a_wth_na37 mt_a_wth_na38 mt_a_wth_na39 mt_a_wth_na40 mt_a_wth_na41 mt_a_wth_na42 mt_a_wth_na43 mt_a_wth_na44 mt_a_wth_na45 mt_a_wth_na46 mt_a_wth_na47 mt_a_wth_na48 mt_a_wth_na49 mt_a_wth_na50 ____________ ____________ ____________ ____________ ____________ ____________ ____________ ____________ ____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ -20 -18.571 -17.143 -15.714 -14.286 -12.857 -11.429 -10 -8.5714 -7.1429 -5.7143 -4.2857 -2.8571 -1.4286 0 1.4286 2.8571 4.2857 5.7143 7.1429 8.5714 10 11.429 12.857 14.286 15.714 17.143 18.571 20 21.429 22.857 24.286 25.714 27.143 28.571 30 31.429 32.857 34.286 35.714 37.143 38.571 40 41.429 42.857 44.286 45.714 47.143 48.571 50 NaN -20 -18.571 -17.143 -15.714 -14.286 -12.857 -11.429 -10 -8.5714 -7.1429 -5.7143 -4.2857 -2.8571 -1.4286 -1.5543e-15 1.4286 2.8571 4.2857 5.7143 7.1429 8.5714 10 11.429 12.857 14.286 15.714 17.143 18.571 20 21.429 22.857 24.286 25.714 27.143 28.571 30 31.429 32.857 34.286 35.714 37.143 38.571 40 41.429 42.857 44.286 45.714 47.143 48.571 NaN NaN -20 -18.571 -17.143 -15.714 -14.286 -12.857 -11.429 -10 -8.5714 -7.1429 -5.7143 -4.2857 -2.8571 -1.4286 4.4409e-16 1.4286 2.8571 4.2857 5.7143 7.1429 8.5714 10 11.429 12.857 14.286 15.714 17.143 18.571 20 21.429 22.857 24.286 25.714 27.143 28.571 30 31.429 32.857 34.286 35.714 37.143 38.571 40 41.429 42.857 44.286 45.714 47.143 NaN NaN NaN -20 -18.571 -17.143 -15.714 -14.286 -12.857 -11.429 -10 -8.5714 -7.1429 -5.7143 -4.2857 -2.8571 -1.4286 -8.8818e-16 1.4286 2.8571 4.2857 5.7143 7.1429 8.5714 10 11.429 12.857 14.286 15.714 17.143 18.571 20 21.429 22.857 24.286 25.714 27.143 28.571 30 31.429 32.857 34.286 35.714 37.143 38.571 40 41.429 42.857 44.286 45.714 NaN NaN NaN NaN -20 -18.571 -17.143 -15.714 -14.286 -12.857 -11.429 -10 -8.5714 -7.1429 -5.7143 -4.2857 -2.8571 -1.4286 8.8818e-16 1.4286 2.8571 4.2857 5.7143 7.1429 8.5714 10 11.429 12.857 14.286 15.714 17.143 18.571 20 21.429 22.857 24.286 25.714 27.143 28.571 30 31.429 32.857 34.286 35.714 37.143 38.571 40 41.429 42.857 44.286 NaN NaN NaN NaN NaN -20 -18.571 -17.143 -15.714 -14.286 -12.857 -11.429 -10 -8.5714 -7.1429 -5.7143 -4.2857 -2.8571 -1.4286 -8.8818e-16 1.4286 2.8571 4.2857 5.7143 7.1429 8.5714 10 11.429 12.857 14.286 15.714 17.143 18.571 20 21.429 22.857 24.286 25.714 27.143 28.571 30 31.429 32.857 34.286 35.714 37.143 38.571 40 41.429 42.857 NaN NaN NaN NaN NaN NaN -20 -18.571 -17.143 -15.714 -14.286 -12.857 -11.429 -10 -8.5714 -7.1429 -5.7143 -4.2857 -2.8571 -1.4286 1.7764e-15 1.4286 2.8571 4.2857 5.7143 7.1429 8.5714 10 11.429 12.857 14.286 15.714 17.143 18.571 20 21.429 22.857 24.286 25.714 27.143 28.571 30 31.429 32.857 34.286 35.714 37.143 38.571 40 41.429 NaN NaN NaN NaN NaN NaN NaN -20 -18.571 -17.143 -15.714 -14.286 -12.857 -11.429 -10 -8.5714 -7.1429 -5.7143 -4.2857 -2.8571 -1.4286 0 1.4286 2.8571 4.2857 5.7143 7.1429 8.5714 10 11.429 12.857 14.286 15.714 17.143 18.571 20 21.429 22.857 24.286 25.714 27.143 28.571 30 31.429 32.857 34.286 35.714 37.143 38.571 40 NaN NaN NaN NaN NaN NaN NaN NaN -20 -18.571 -17.143 -15.714 -14.286 -12.857 -11.429 -10 -8.5714 -7.1429 -5.7143 -4.2857 -2.8571 -1.4286 -1.7764e-15 1.4286 2.8571 4.2857 5.7143 7.1429 8.5714 10 11.429 12.857 14.286 15.714 17.143 18.571 20 21.429 22.857 24.286 25.714 27.143 28.571 30 31.429 32.857 34.286 35.714 37.143 38.571 NaN NaN NaN NaN NaN NaN NaN NaN NaN -20 -18.571 -17.143 -15.714 -14.286 -12.857 -11.429 -10 -8.5714 -7.1429 -5.7143 -4.2857 -2.8571 -1.4286 -3.5527e-15 1.4286 2.8571 4.2857 5.7143 7.1429 8.5714 10 11.429 12.857 14.286 15.714 17.143 18.571 20 21.429 22.857 24.286 25.714 27.143 28.571 30 31.429 32.857 34.286 35.714 37.143 mt_a_wth_na1 mt_a_wth_na2 mt_a_wth_na3 mt_a_wth_na4 mt_a_wth_na5 mt_a_wth_na6 mt_a_wth_na7 mt_a_wth_na8 mt_a_wth_na9 mt_a_wth_na10 mt_a_wth_na11 mt_a_wth_na12 mt_a_wth_na13 mt_a_wth_na14 mt_a_wth_na15 mt_a_wth_na16 mt_a_wth_na17 mt_a_wth_na18 mt_a_wth_na19 mt_a_wth_na20 mt_a_wth_na21 mt_a_wth_na22 mt_a_wth_na23 mt_a_wth_na24 mt_a_wth_na25 mt_a_wth_na26 mt_a_wth_na27 mt_a_wth_na28 mt_a_wth_na29 mt_a_wth_na30 mt_a_wth_na31 mt_a_wth_na32 mt_a_wth_na33 mt_a_wth_na34 mt_a_wth_na35 mt_a_wth_na36 mt_a_wth_na37 mt_a_wth_na38 mt_a_wth_na39 mt_a_wth_na40 mt_a_wth_na41 mt_a_wth_na42 mt_a_wth_na43 mt_a_wth_na44 mt_a_wth_na45 mt_a_wth_na46 mt_a_wth_na47 mt_a_wth_na48 mt_a_wth_na49 mt_a_wth_na50 ____________ ____________ ____________ ____________ ____________ ____________ ____________ ____________ ____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN -20 -18.571 -17.143 -15.714 -14.286 -12.857 -11.429 -10 -8.5714 -7.1429 NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN -20 -18.571 -17.143 -15.714 -14.286 -12.857 -11.429 -10 -8.5714 NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN -20 -18.571 -17.143 -15.714 -14.286 -12.857 -11.429 -10 NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN -20 -18.571 -17.143 -15.714 -14.286 -12.857 -11.429 NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN -20 -18.571 -17.143 -15.714 -14.286 -12.857 NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN -20 -18.571 -17.143 -15.714 -14.286 NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN -20 -18.571 -17.143 -15.714 NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN -20 -18.571 -17.143 NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN -20 -18.571 NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN -20 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx mt_k_wth_na xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx 50 50 mt_k_wth_na1 mt_k_wth_na2 mt_k_wth_na3 mt_k_wth_na4 mt_k_wth_na5 mt_k_wth_na6 mt_k_wth_na7 mt_k_wth_na8 mt_k_wth_na9 mt_k_wth_na10 mt_k_wth_na11 mt_k_wth_na12 mt_k_wth_na13 mt_k_wth_na14 mt_k_wth_na15 mt_k_wth_na16 mt_k_wth_na17 mt_k_wth_na18 mt_k_wth_na19 mt_k_wth_na20 mt_k_wth_na21 mt_k_wth_na22 mt_k_wth_na23 mt_k_wth_na24 mt_k_wth_na25 mt_k_wth_na26 mt_k_wth_na27 mt_k_wth_na28 mt_k_wth_na29 mt_k_wth_na30 mt_k_wth_na31 mt_k_wth_na32 mt_k_wth_na33 mt_k_wth_na34 mt_k_wth_na35 mt_k_wth_na36 mt_k_wth_na37 mt_k_wth_na38 mt_k_wth_na39 mt_k_wth_na40 mt_k_wth_na41 mt_k_wth_na42 mt_k_wth_na43 mt_k_wth_na44 mt_k_wth_na45 mt_k_wth_na46 mt_k_wth_na47 mt_k_wth_na48 mt_k_wth_na49 mt_k_wth_na50 ____________ ____________ ____________ ____________ ____________ ____________ ____________ ____________ ____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 NaN 1.4286 1.4286 1.4286 1.4286 1.4286 1.4286 1.4286 1.4286 1.4286 1.4286 1.4286 1.4286 1.4286 1.4286 1.4286 1.4286 1.4286 1.4286 1.4286 1.4286 1.4286 1.4286 1.4286 1.4286 1.4286 1.4286 1.4286 1.4286 1.4286 1.4286 1.4286 1.4286 1.4286 1.4286 1.4286 1.4286 1.4286 1.4286 1.4286 1.4286 1.4286 1.4286 1.4286 1.4286 1.4286 1.4286 1.4286 1.4286 1.4286 NaN NaN 2.8571 2.8571 2.8571 2.8571 2.8571 2.8571 2.8571 2.8571 2.8571 2.8571 2.8571 2.8571 2.8571 2.8571 2.8571 2.8571 2.8571 2.8571 2.8571 2.8571 2.8571 2.8571 2.8571 2.8571 2.8571 2.8571 2.8571 2.8571 2.8571 2.8571 2.8571 2.8571 2.8571 2.8571 2.8571 2.8571 2.8571 2.8571 2.8571 2.8571 2.8571 2.8571 2.8571 2.8571 2.8571 2.8571 2.8571 2.8571 NaN NaN NaN 4.2857 4.2857 4.2857 4.2857 4.2857 4.2857 4.2857 4.2857 4.2857 4.2857 4.2857 4.2857 4.2857 4.2857 4.2857 4.2857 4.2857 4.2857 4.2857 4.2857 4.2857 4.2857 4.2857 4.2857 4.2857 4.2857 4.2857 4.2857 4.2857 4.2857 4.2857 4.2857 4.2857 4.2857 4.2857 4.2857 4.2857 4.2857 4.2857 4.2857 4.2857 4.2857 4.2857 4.2857 4.2857 4.2857 4.2857 NaN NaN NaN NaN 5.7143 5.7143 5.7143 5.7143 5.7143 5.7143 5.7143 5.7143 5.7143 5.7143 5.7143 5.7143 5.7143 5.7143 5.7143 5.7143 5.7143 5.7143 5.7143 5.7143 5.7143 5.7143 5.7143 5.7143 5.7143 5.7143 5.7143 5.7143 5.7143 5.7143 5.7143 5.7143 5.7143 5.7143 5.7143 5.7143 5.7143 5.7143 5.7143 5.7143 5.7143 5.7143 5.7143 5.7143 5.7143 5.7143 NaN NaN NaN NaN NaN 7.1429 7.1429 7.1429 7.1429 7.1429 7.1429 7.1429 7.1429 7.1429 7.1429 7.1429 7.1429 7.1429 7.1429 7.1429 7.1429 7.1429 7.1429 7.1429 7.1429 7.1429 7.1429 7.1429 7.1429 7.1429 7.1429 7.1429 7.1429 7.1429 7.1429 7.1429 7.1429 7.1429 7.1429 7.1429 7.1429 7.1429 7.1429 7.1429 7.1429 7.1429 7.1429 7.1429 7.1429 7.1429 NaN NaN NaN NaN NaN NaN 8.5714 8.5714 8.5714 8.5714 8.5714 8.5714 8.5714 8.5714 8.5714 8.5714 8.5714 8.5714 8.5714 8.5714 8.5714 8.5714 8.5714 8.5714 8.5714 8.5714 8.5714 8.5714 8.5714 8.5714 8.5714 8.5714 8.5714 8.5714 8.5714 8.5714 8.5714 8.5714 8.5714 8.5714 8.5714 8.5714 8.5714 8.5714 8.5714 8.5714 8.5714 8.5714 8.5714 8.5714 NaN NaN NaN NaN NaN NaN NaN 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 NaN NaN NaN NaN NaN NaN NaN NaN 11.429 11.429 11.429 11.429 11.429 11.429 11.429 11.429 11.429 11.429 11.429 11.429 11.429 11.429 11.429 11.429 11.429 11.429 11.429 11.429 11.429 11.429 11.429 11.429 11.429 11.429 11.429 11.429 11.429 11.429 11.429 11.429 11.429 11.429 11.429 11.429 11.429 11.429 11.429 11.429 11.429 11.429 NaN NaN NaN NaN NaN NaN NaN NaN NaN 12.857 12.857 12.857 12.857 12.857 12.857 12.857 12.857 12.857 12.857 12.857 12.857 12.857 12.857 12.857 12.857 12.857 12.857 12.857 12.857 12.857 12.857 12.857 12.857 12.857 12.857 12.857 12.857 12.857 12.857 12.857 12.857 12.857 12.857 12.857 12.857 12.857 12.857 12.857 12.857 12.857 mt_k_wth_na1 mt_k_wth_na2 mt_k_wth_na3 mt_k_wth_na4 mt_k_wth_na5 mt_k_wth_na6 mt_k_wth_na7 mt_k_wth_na8 mt_k_wth_na9 mt_k_wth_na10 mt_k_wth_na11 mt_k_wth_na12 mt_k_wth_na13 mt_k_wth_na14 mt_k_wth_na15 mt_k_wth_na16 mt_k_wth_na17 mt_k_wth_na18 mt_k_wth_na19 mt_k_wth_na20 mt_k_wth_na21 mt_k_wth_na22 mt_k_wth_na23 mt_k_wth_na24 mt_k_wth_na25 mt_k_wth_na26 mt_k_wth_na27 mt_k_wth_na28 mt_k_wth_na29 mt_k_wth_na30 mt_k_wth_na31 mt_k_wth_na32 mt_k_wth_na33 mt_k_wth_na34 mt_k_wth_na35 mt_k_wth_na36 mt_k_wth_na37 mt_k_wth_na38 mt_k_wth_na39 mt_k_wth_na40 mt_k_wth_na41 mt_k_wth_na42 mt_k_wth_na43 mt_k_wth_na44 mt_k_wth_na45 mt_k_wth_na46 mt_k_wth_na47 mt_k_wth_na48 mt_k_wth_na49 mt_k_wth_na50 ____________ ____________ ____________ ____________ ____________ ____________ ____________ ____________ ____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ _____________ NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN 57.143 57.143 57.143 57.143 57.143 57.143 57.143 57.143 57.143 57.143 NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN 58.571 58.571 58.571 58.571 58.571 58.571 58.571 58.571 58.571 NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN 60 60 60 60 60 60 60 60 NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN 61.429 61.429 61.429 61.429 61.429 61.429 61.429 NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN 62.857 62.857 62.857 62.857 62.857 62.857 NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN 64.286 64.286 64.286 64.286 64.286 NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN 65.714 65.714 65.714 65.714 NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN 67.143 67.143 67.143 NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN 68.571 68.571 NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN NaN 70 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ar_a_meshk and ar_k_mesha xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Variables: ar_a_meshk: 1275×1 double Values: Min -20 Median 8.8818e-16 Max 50 Variables: ar_k_mesha: 1275×1 double Values: Min 0 Median 20 Max 70 pos = 1 ; key = f_coh ; val = @(z,b,k)(f_prod(z,k)+k*(1-fl_delta)+fl_w+b.*(1+fl_r_save).*(b>0)+b.*(1+fl_r_borr).*(b<=0)) pos = 2 ; key = f_cons ; val = @(coh,bprime,kprime)(coh-kprime-bprime) pos = 3 ; key = f_inc ; val = @(z,b,k)(f_prod(z,k)-(fl_delta)*k+fl_w+b.*(fl_r_save).*(b>0)+b.*(fl_r_borr).*(b<=0)) pos = 4 ; key = f_prod ; val = @(z,k)((fl_Amean.*(z)).*(k.^(fl_alpha))) pos = 5 ; key = f_util_crra ; val = @(c)(((c).^(1-fl_crra)-1)./(1-fl_crra)) pos = 6 ; key = f_util_log ; val = @(c)log(c) pos = 7 ; key = f_util_standin ; val = @(z,b,k)f_util_log(f_coh(z,b,k).*(f_coh(z,b,k)>0)+fl_c_min.*(f_coh(z,b,k)<=0))
end
ans = Map with properties: Count: 17 KeyType: char ValueType: any