Generate States, Choices and Shocks Grids and Get Functions (ABZR FIBS)
back to Fan's Dynamic Assets Repository Table of Content.
Contents
- FFS_ABZ_FIBS_GET_FUNCGRID get funcs, params, states choices shocks grids
- Default
- Parse Parameters 1
- Parse Parameters 2
- Parse Parameters 3
- Get Shock: Income Shock (ar1)
- Get Shock: Interest Rate Shock (iid)
- Get Shock: Mesh Shocks Together
- Get Equations
- Get Formal Borrowing Blocks
- Get Asset and Choice Grid
- Store
- Graph: A, Shocks, COH, and Defaults
- Display
function [armt_map, func_map] = ffs_abzr_fibs_get_funcgrid(varargin)
FFS_ABZ_FIBS_GET_FUNCGRID get funcs, params, states choices shocks grids
centralized gateway for retrieving parameters, and solution grids and functions
@param param_map container parameter container
@param support_map container support container
@param bl_input_override boolean if true varargin contained param_map and support_map fully overrides local default. Local default is not invoked. This could be important for speed if this function is getting invoked within certain loops. Default is 0.
@return armt_map container container with states, choices and shocks grids that are inputs for grid based solution algorithm
@return func_map container container with function handles for consumption cash-on-hand etc.
@example
it_param_set = 2; bl_input_override = true; [param_map, support_map] = ffs_abzr_fibs_set_default_param(it_param_set); [armt_map, func_map] = ffs_abzr_fibs_get_funcgrid(param_map, support_map, bl_input_override);
@include
@seealso
Default
if (~isempty(varargin)) % override when called from outside [param_map, support_map] = varargin{:}; else close all % default internal run it_param_set = 4; [param_map, support_map] = ffs_abzr_fibs_set_default_param(it_param_set); support_map('bl_graph_funcgrids') = true; support_map('bl_display_funcgrids') = true; default_maps = {param_map, support_map}; % numvarargs is the number of varagin inputted [default_maps{1:length(varargin)}] = varargin{:}; param_map = [param_map; default_maps{1}]; support_map = [support_map; default_maps{2}]; end
Parse Parameters 1
% param_map asset grid params_group = values(param_map, {'fl_b_bd', 'bl_default', 'fl_a_min', 'fl_a_max', 'bl_loglin', 'fl_loglin_threshold', 'it_a_n'}); [fl_b_bd, bl_default, fl_a_min, fl_a_max, bl_loglin, fl_loglin_threshold, it_a_n] = params_group{:}; % param_map preference params_group = values(param_map, {'fl_crra', 'fl_c_min'}); [fl_crra, fl_c_min] = params_group{:}; % param_map borrowing and price params_group = values(param_map, {'bl_b_is_principle', 'fl_r_fbr', 'fl_r_fsv', 'fl_w'}); [bl_b_is_principle, fl_r_fbr, fl_r_fsv, fl_w] = params_group{:};
Parse Parameters 2
% param_map shock income params_group = values(param_map, {'it_z_wage_n', 'fl_z_wage_mu', 'fl_z_wage_rho', 'fl_z_wage_sig'}); [it_z_wage_n, fl_z_wage_mu, fl_z_wage_rho, fl_z_wage_sig] = params_group{:}; % param_map shock borrowing interest params_group = values(param_map, {'st_z_r_infbr_drv_ele_type', 'st_z_r_infbr_drv_prb_type', 'fl_z_r_infbr_poiss_mean', ... 'fl_z_r_infbr_max', 'fl_z_r_infbr_min', 'fl_z_r_infbr_n'}); [st_z_r_infbr_drv_ele_type, st_z_r_infbr_drv_prb_type, fl_z_r_infbr_poiss_mean, ... fl_z_r_infbr_max, fl_z_r_infbr_min, fl_z_r_infbr_n] = params_group{:}; % param_map formal menu params_group = values(param_map, {'st_forbrblk_type', 'fl_forbrblk_brmost', 'fl_forbrblk_brleast', 'fl_forbrblk_gap'}); [st_forbrblk_type, fl_forbrblk_brmost, fl_forbrblk_brleast, fl_forbrblk_gap] = params_group{:};
Parse Parameters 3
params_group = values(support_map, {'bl_graph_funcgrids', 'bl_display_funcgrids'}); [bl_graph_funcgrids, bl_display_funcgrids] = params_group{:};
Get Shock: Income Shock (ar1)
[~, mt_z_wage_trans, ar_wage_stationary, ar_z_wage] = ffto_gen_tauchen_jhl(fl_z_wage_mu,fl_z_wage_rho,fl_z_wage_sig,it_z_wage_n);
Get Shock: Interest Rate Shock (iid)
% get borrowing grid and probabilities param_dsv_map = containers.Map('KeyType','char', 'ValueType','any'); param_dsv_map('st_drv_ele_type') = st_z_r_infbr_drv_ele_type; param_dsv_map('st_drv_prb_type') = st_z_r_infbr_drv_prb_type; param_dsv_map('fl_poiss_mean') = fl_z_r_infbr_poiss_mean; param_dsv_map('fl_max') = fl_z_r_infbr_max; param_dsv_map('fl_min') = fl_z_r_infbr_min; param_dsv_map('fl_n') = fl_z_r_infbr_n; [ar_z_r_infbr, ar_z_r_inf_prob] = fft_gen_discrete_var(param_dsv_map, true); % iid transition matrix mt_z_r_infbr_prob_trans = repmat(ar_z_r_inf_prob, [length(ar_z_r_inf_prob), 1]);
Get Shock: Mesh Shocks Together
% Kronecker product to get full transition matrix for the two shocks mt_z_trans = kron(mt_z_r_infbr_prob_trans, mt_z_wage_trans); % mesh the shock vectors [mt_z_wage_mesh_r_infbr, mt_z_r_infbr_mesh_wage] = ndgrid(ar_z_wage, ar_z_r_infbr); ar_z_r_infbr_mesh_wage = mt_z_r_infbr_mesh_wage(:)'; ar_z_wage_mesh_r_infbr = mt_z_wage_mesh_r_infbr(:)'; if (bl_display_funcgrids) disp('----------------------------------------'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp('Borrow R Shock: ar_z_r_inf_mesh_wage'); disp('Prod/Wage Shock: mt_z_wage_mesh_r_infbr'); disp('show which shock is inner and which is outter'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); tb_two_shocks = array2table([ar_z_r_infbr_mesh_wage;... ar_z_wage_mesh_r_infbr]'); cl_col_names = ["Borrow R Shock (Meshed)", "Wage R Shock (Meshed)"]; cl_row_names = strcat('zi=', string((1:length(ar_z_r_infbr_mesh_wage)))); tb_two_shocks.Properties.VariableNames = matlab.lang.makeValidName(cl_col_names); tb_two_shocks.Properties.RowNames = matlab.lang.makeValidName(cl_row_names); it_row_display = it_z_wage_n*2; disp(size(tb_two_shocks)); disp(head(tb_two_shocks, it_row_display)); disp(tail(tb_two_shocks, it_row_display)); disp('----------------------------------------'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp('Borrow Rate Transition Matrix: mt_z_r_infbr_prob_trans'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); it_col_n_keep = 15; it_row_n_keep = 15; [it_row_n, it_col_n] = size(mt_z_r_infbr_prob_trans); [ar_it_cols, ar_it_rows] = fft_row_col_subset(it_col_n, it_col_n_keep, it_row_n, it_row_n_keep); cl_st_full_rowscols = cellstr([num2str(ar_z_r_infbr', 'r%3.2f')]); tb_z_r_infbr_prob_trans = array2table(round(mt_z_r_infbr_prob_trans(ar_it_rows, ar_it_cols), 6)); cl_col_names = strcat('zi=', num2str(ar_it_cols'), ':', cl_st_full_rowscols(ar_it_cols)); cl_row_names = strcat('zi=', num2str(ar_it_rows'), ':', cl_st_full_rowscols(ar_it_cols)); tb_z_r_infbr_prob_trans.Properties.VariableNames = matlab.lang.makeValidName(cl_col_names); tb_z_r_infbr_prob_trans.Properties.RowNames = matlab.lang.makeValidName(cl_row_names); disp(size(tb_z_r_infbr_prob_trans)); disp(tb_z_r_infbr_prob_trans); disp('----------------------------------------'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp('Wage Prod Shock Transition Matrix: mt_z_r_infbr_prob_trans'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); it_col_n_keep = 15; it_row_n_keep = 15; [it_row_n, it_col_n] = size(mt_z_wage_trans); [ar_it_cols, ar_it_rows] = fft_row_col_subset(it_col_n, it_col_n_keep, it_row_n, it_row_n_keep); cl_st_full_rowscols = cellstr([num2str(ar_z_wage', 'w%3.2f')]); tb_z_wage_trans = array2table(round(mt_z_wage_trans(ar_it_rows, ar_it_cols),6)); cl_col_names = strcat('zi=', num2str(ar_it_cols'), ':', cl_st_full_rowscols(ar_it_cols)); cl_row_names = strcat('zi=', num2str(ar_it_rows'), ':', cl_st_full_rowscols(ar_it_cols)); tb_z_wage_trans.Properties.VariableNames = matlab.lang.makeValidName(cl_col_names); tb_z_wage_trans.Properties.RowNames = matlab.lang.makeValidName(cl_row_names); disp(size(tb_z_wage_trans)); disp(tb_z_wage_trans); disp('----------------------------------------'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp('Full Transition Matrix: mt_z_trans'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); it_col_n_keep = it_z_wage_n*3; it_row_n_keep = it_z_wage_n*3; [it_row_n, it_col_n] = size(mt_z_trans); [ar_it_cols, ar_it_rows] = fft_row_col_subset(it_col_n, it_col_n_keep, it_row_n, it_row_n_keep); cl_st_full_rowscols = cellstr([num2str(ar_z_r_infbr_mesh_wage', 'r%3.2f;'), ... num2str(ar_z_wage_mesh_r_infbr', 'w%3.2f')]); tb_mt_z_trans = array2table(round(mt_z_trans(ar_it_rows, ar_it_cols),6)); cl_col_names = strcat('i', num2str(ar_it_cols'), ':', cl_st_full_rowscols(ar_it_cols)); cl_row_names = strcat('i', num2str(ar_it_rows'), ':', cl_st_full_rowscols(ar_it_cols)); tb_mt_z_trans.Properties.VariableNames = matlab.lang.makeValidName(cl_col_names); tb_mt_z_trans.Properties.RowNames = matlab.lang.makeValidName(cl_row_names); disp(size(tb_mt_z_trans)); disp(tb_mt_z_trans); end
---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Borrow R Shock: ar_z_r_inf_mesh_wage Prod/Wage Shock: mt_z_wage_mesh_r_infbr show which shock is inner and which is outter xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx 75 2 BorrowRShock_Meshed_ WageRShock_Meshed_ ____________________ __________________ zi_1 0.025 0.34741 zi_2 0.025 0.40076 zi_3 0.025 0.4623 zi_4 0.025 0.5333 zi_5 0.025 0.61519 zi_6 0.025 0.70966 zi_7 0.025 0.81864 zi_8 0.025 0.94436 zi_9 0.025 1.0894 zi_10 0.025 1.2567 zi_11 0.025 1.4496 zi_12 0.025 1.6723 zi_13 0.025 1.9291 zi_14 0.025 2.2253 zi_15 0.025 2.567 zi_16 0.0425 0.34741 zi_17 0.0425 0.40076 zi_18 0.0425 0.4623 zi_19 0.0425 0.5333 zi_20 0.0425 0.61519 zi_21 0.0425 0.70966 zi_22 0.0425 0.81864 zi_23 0.0425 0.94436 zi_24 0.0425 1.0894 zi_25 0.0425 1.2567 zi_26 0.0425 1.4496 zi_27 0.0425 1.6723 zi_28 0.0425 1.9291 zi_29 0.0425 2.2253 zi_30 0.0425 2.567 BorrowRShock_Meshed_ WageRShock_Meshed_ ____________________ __________________ zi_46 0.0775 0.34741 zi_47 0.0775 0.40076 zi_48 0.0775 0.4623 zi_49 0.0775 0.5333 zi_50 0.0775 0.61519 zi_51 0.0775 0.70966 zi_52 0.0775 0.81864 zi_53 0.0775 0.94436 zi_54 0.0775 1.0894 zi_55 0.0775 1.2567 zi_56 0.0775 1.4496 zi_57 0.0775 1.6723 zi_58 0.0775 1.9291 zi_59 0.0775 2.2253 zi_60 0.0775 2.567 zi_61 0.095 0.34741 zi_62 0.095 0.40076 zi_63 0.095 0.4623 zi_64 0.095 0.5333 zi_65 0.095 0.61519 zi_66 0.095 0.70966 zi_67 0.095 0.81864 zi_68 0.095 0.94436 zi_69 0.095 1.0894 zi_70 0.095 1.2567 zi_71 0.095 1.4496 zi_72 0.095 1.6723 zi_73 0.095 1.9291 zi_74 0.095 2.2253 zi_75 0.095 2.567 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Borrow Rate Transition Matrix: mt_z_r_infbr_prob_trans xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx 5 5 zi_1_r0_03 zi_2_r0_04 zi_3_r0_06 zi_4_r0_08 zi_5_r0_10 __________ __________ __________ __________ __________ zi_1_r0_03 0.000122 0.002433 0.024328 0.16219 0.81093 zi_2_r0_04 0.000122 0.002433 0.024328 0.16219 0.81093 zi_3_r0_06 0.000122 0.002433 0.024328 0.16219 0.81093 zi_4_r0_08 0.000122 0.002433 0.024328 0.16219 0.81093 zi_5_r0_10 0.000122 0.002433 0.024328 0.16219 0.81093 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Wage Prod Shock Transition Matrix: mt_z_r_infbr_prob_trans xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx 15 15 zi_1_w0_35 zi_2_w0_40 zi_3_w0_46 zi_4_w0_53 zi_5_w0_62 zi_6_w0_71 zi_7_w0_82 zi_8_w0_94 zi_9_w1_09 zi_10_w1_26 zi_11_w1_45 zi_12_w1_67 zi_13_w1_93 zi_14_w2_23 zi_15_w2_57 __________ __________ __________ __________ __________ __________ __________ __________ __________ ___________ ___________ ___________ ___________ ___________ ___________ zi_1_w0_35 0.26016 0.26831 0.25551 0.14921 0.053403 0.011702 0.001568 0.000128 6e-06 0 0 0 0 0 0 zi_2_w0_40 0.11232 0.19622 0.2763 0.23861 0.12635 0.040998 0.008143 0.000989 7.3e-05 3e-06 0 0 0 0 0 zi_3_w0_46 0.037073 0.10492 0.2185 0.27902 0.2185 0.10492 0.030863 0.005556 0.000611 4.1e-05 2e-06 0 0 0 0 zi_4_w0_53 0.009208 0.040998 0.12635 0.23861 0.2763 0.19622 0.085427 0.022782 0.003717 0.00037 2.3e-05 1e-06 0 0 0 zi_5_w0_62 0.001703 0.011702 0.053403 0.14921 0.25551 0.26831 0.17279 0.068209 0.016489 0.002438 0.00022 1.2e-05 0 0 0 zi_6_w0_71 0.000233 0.002438 0.016489 0.068209 0.17279 0.26831 0.25551 0.14921 0.053403 0.011702 0.001568 0.000128 6e-06 0 0 zi_7_w0_82 2.3e-05 0.00037 0.003717 0.022782 0.085427 0.19622 0.2763 0.23861 0.12635 0.040998 0.008143 0.000989 7.3e-05 3e-06 0 zi_8_w0_94 2e-06 4.1e-05 0.000611 0.005556 0.030863 0.10492 0.2185 0.27902 0.2185 0.10492 0.030863 0.005556 0.000611 4.1e-05 2e-06 zi_9_w1_09 0 3e-06 7.3e-05 0.000989 0.008143 0.040998 0.12635 0.23861 0.2763 0.19622 0.085427 0.022782 0.003717 0.00037 2.3e-05 zi_10_w1_26 0 0 6e-06 0.000128 0.001568 0.011702 0.053403 0.14921 0.25551 0.26831 0.17279 0.068209 0.016489 0.002438 0.000233 zi_11_w1_45 0 0 0 1.2e-05 0.00022 0.002438 0.016489 0.068209 0.17279 0.26831 0.25551 0.14921 0.053403 0.011702 0.001703 zi_12_w1_67 0 0 0 1e-06 2.3e-05 0.00037 0.003717 0.022782 0.085427 0.19622 0.2763 0.23861 0.12635 0.040998 0.009208 zi_13_w1_93 0 0 0 0 2e-06 4.1e-05 0.000611 0.005556 0.030863 0.10492 0.2185 0.27902 0.2185 0.10492 0.037073 zi_14_w2_23 0 0 0 0 0 3e-06 7.3e-05 0.000989 0.008143 0.040998 0.12635 0.23861 0.2763 0.19622 0.11232 zi_15_w2_57 0 0 0 0 0 0 6e-06 0.000128 0.001568 0.011702 0.053403 0.14921 0.25551 0.26831 0.26016 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Full Transition Matrix: mt_z_trans xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx 45 45 i1_r0_03_w0_35 i2_r0_03_w0_40 i3_r0_03_w0_46 i4_r0_03_w0_53 i5_r0_03_w0_62 i6_r0_03_w0_71 i7_r0_03_w0_82 i8_r0_03_w0_94 i9_r0_03_w1_09 i10_r0_03_w1_26 i11_r0_03_w1_45 i12_r0_03_w1_67 i13_r0_03_w1_93 i14_r0_03_w2_23 i15_r0_03_w2_57 i16_r0_04_w0_35 i17_r0_04_w0_40 i18_r0_04_w0_46 i19_r0_04_w0_53 i20_r0_04_w0_62 i21_r0_04_w0_71 i22_r0_04_w0_82 i38_r0_06_w0_94 i54_r0_08_w1_09 i55_r0_08_w1_26 i56_r0_08_w1_45 i57_r0_08_w1_67 i58_r0_08_w1_93 i59_r0_08_w2_23 i60_r0_08_w2_57 i61_r0_10_w0_35 i62_r0_10_w0_40 i63_r0_10_w0_46 i64_r0_10_w0_53 i65_r0_10_w0_62 i66_r0_10_w0_71 i67_r0_10_w0_82 i68_r0_10_w0_94 i69_r0_10_w1_09 i70_r0_10_w1_26 i71_r0_10_w1_45 i72_r0_10_w1_67 i73_r0_10_w1_93 i74_r0_10_w2_23 i75_r0_10_w2_57 ______________ ______________ ______________ ______________ ______________ ______________ ______________ ______________ ______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ i1_r0_03_w0_35 3.2e-05 3.3e-05 3.1e-05 1.8e-05 6e-06 1e-06 0 0 0 0 0 0 0 0 0 0.000633 0.000653 0.000622 0.000363 0.00013 2.8e-05 4e-06 3e-06 1e-06 0 0 0 0 0 0 0.21097 0.21758 0.2072 0.121 0.043306 0.009489 0.001271 0.000104 5e-06 0 0 0 0 0 0 i2_r0_03_w0_40 1.4e-05 2.4e-05 3.4e-05 2.9e-05 1.5e-05 5e-06 1e-06 0 0 0 0 0 0 0 0 0.000273 0.000477 0.000672 0.00058 0.000307 0.0001 2e-05 2.4e-05 1.2e-05 1e-06 0 0 0 0 0 0.091083 0.15912 0.22406 0.19349 0.10246 0.033247 0.006603 0.000802 5.9e-05 3e-06 0 0 0 0 0 i3_r0_03_w0_46 5e-06 1.3e-05 2.7e-05 3.4e-05 2.7e-05 1.3e-05 4e-06 1e-06 0 0 0 0 0 0 0 9e-05 0.000255 0.000532 0.000679 0.000532 0.000255 7.5e-05 0.000135 9.9e-05 7e-06 0 0 0 0 0 0.030063 0.085079 0.17719 0.22626 0.17719 0.085079 0.025028 0.004505 0.000496 3.3e-05 1e-06 0 0 0 0 i4_r0_03_w0_53 1e-06 5e-06 1.5e-05 2.9e-05 3.4e-05 2.4e-05 1e-05 3e-06 0 0 0 0 0 0 0 2.2e-05 0.0001 0.000307 0.00058 0.000672 0.000477 0.000208 0.000554 0.000603 6e-05 4e-06 0 0 0 0 0.007467 0.033247 0.10246 0.19349 0.22406 0.15912 0.069276 0.018474 0.003014 0.0003 1.8e-05 1e-06 0 0 0 i5_r0_03_w0_62 0 1e-06 6e-06 1.8e-05 3.1e-05 3.3e-05 2.1e-05 8e-06 2e-06 0 0 0 0 0 0 4e-06 2.8e-05 0.00013 0.000363 0.000622 0.000653 0.00042 0.001659 0.002674 0.000395 3.6e-05 2e-06 0 0 0 0.001381 0.009489 0.043306 0.121 0.2072 0.21758 0.14012 0.055312 0.013371 0.001977 0.000178 1e-05 0 0 0 i6_r0_03_w0_71 0 0 2e-06 8e-06 2.1e-05 3.3e-05 3.1e-05 1.8e-05 6e-06 1e-06 0 0 0 0 0 1e-06 6e-06 4e-05 0.000166 0.00042 0.000653 0.000622 0.00363 0.008661 0.001898 0.000254 2.1e-05 1e-06 0 0 0.000189 0.001977 0.013371 0.055312 0.14012 0.21758 0.2072 0.121 0.043306 0.009489 0.001271 0.000104 5e-06 0 0 i7_r0_03_w0_82 0 0 0 3e-06 1e-05 2.4e-05 3.4e-05 2.9e-05 1.5e-05 5e-06 1e-06 0 0 0 0 0 1e-06 9e-06 5.5e-05 0.000208 0.000477 0.000672 0.005805 0.020492 0.006649 0.001321 0.00016 1.2e-05 1e-06 0 1.9e-05 0.0003 0.003014 0.018474 0.069276 0.15912 0.22406 0.19349 0.10246 0.033247 0.006603 0.000802 5.9e-05 3e-06 0 i8_r0_03_w0_94 0 0 0 1e-06 4e-06 1.3e-05 2.7e-05 3.4e-05 2.7e-05 1.3e-05 4e-06 1e-06 0 0 0 0 0 1e-06 1.4e-05 7.5e-05 0.000255 0.000532 0.006788 0.035438 0.017016 0.005006 0.000901 9.9e-05 7e-06 0 1e-06 3.3e-05 0.000496 0.004505 0.025028 0.085079 0.17719 0.22626 0.17719 0.085079 0.025028 0.004505 0.000496 3.3e-05 1e-06 i9_r0_03_w1_09 0 0 0 0 1e-06 5e-06 1.5e-05 2.9e-05 3.4e-05 2.4e-05 1e-05 3e-06 0 0 0 0 0 0 2e-06 2e-05 0.0001 0.000307 0.005805 0.044812 0.031824 0.013855 0.003695 0.000603 6e-05 4e-06 0 3e-06 5.9e-05 0.000802 0.006603 0.033247 0.10246 0.19349 0.22406 0.15912 0.069276 0.018474 0.003014 0.0003 1.9e-05 i10_r0_03_w1_26 0 0 0 0 0 1e-06 6e-06 1.8e-05 3.1e-05 3.3e-05 2.1e-05 8e-06 2e-06 0 0 0 0 0 0 4e-06 2.8e-05 0.00013 0.00363 0.04144 0.043517 0.028024 0.011062 0.002674 0.000395 3.8e-05 0 0 5e-06 0.000104 0.001271 0.009489 0.043306 0.121 0.2072 0.21758 0.14012 0.055312 0.013371 0.001977 0.000189 i11_r0_03_w1_45 0 0 0 0 0 0 2e-06 8e-06 2.1e-05 3.3e-05 3.1e-05 1.8e-05 6e-06 1e-06 0 0 0 0 0 1e-06 6e-06 4e-05 0.001659 0.028024 0.043517 0.04144 0.0242 0.008661 0.001898 0.000276 0 0 0 1e-05 0.000178 0.001977 0.013371 0.055312 0.14012 0.21758 0.2072 0.121 0.043306 0.009489 0.001381 i12_r0_03_w1_67 0 0 0 0 0 0 0 3e-06 1e-05 2.4e-05 3.4e-05 2.9e-05 1.5e-05 5e-06 1e-06 0 0 0 0 0 1e-06 9e-06 0.000554 0.013855 0.031824 0.044812 0.038699 0.020492 0.006649 0.001493 0 0 0 1e-06 1.8e-05 0.0003 0.003014 0.018474 0.069276 0.15912 0.22406 0.19349 0.10246 0.033247 0.007467 i13_r0_03_w1_93 0 0 0 0 0 0 0 1e-06 4e-06 1.3e-05 2.7e-05 3.4e-05 2.7e-05 1.3e-05 5e-06 0 0 0 0 0 0 1e-06 0.000135 0.005006 0.017016 0.035438 0.045252 0.035438 0.017016 0.006013 0 0 0 0 1e-06 3.3e-05 0.000496 0.004505 0.025028 0.085079 0.17719 0.22626 0.17719 0.085079 0.030063 i14_r0_03_w2_23 0 0 0 0 0 0 0 0 1e-06 5e-06 1.5e-05 2.9e-05 3.4e-05 2.4e-05 1.4e-05 0 0 0 0 0 0 0 2.4e-05 0.001321 0.006649 0.020492 0.038699 0.044812 0.031824 0.018217 0 0 0 0 0 3e-06 5.9e-05 0.000802 0.006603 0.033247 0.10246 0.19349 0.22406 0.15912 0.091083 i15_r0_03_w2_57 0 0 0 0 0 0 0 0 0 1e-06 6e-06 1.8e-05 3.1e-05 3.3e-05 3.2e-05 0 0 0 0 0 0 0 3e-06 0.000254 0.001898 0.008661 0.0242 0.04144 0.043517 0.042194 0 0 0 0 0 0 5e-06 0.000104 0.001271 0.009489 0.043306 0.121 0.2072 0.21758 0.21097 i16_r0_04_w0_35 3.2e-05 3.3e-05 3.1e-05 1.8e-05 6e-06 1e-06 0 0 0 0 0 0 0 0 0 0.000633 0.000653 0.000622 0.000363 0.00013 2.8e-05 4e-06 3e-06 1e-06 0 0 0 0 0 0 0.21097 0.21758 0.2072 0.121 0.043306 0.009489 0.001271 0.000104 5e-06 0 0 0 0 0 0 i17_r0_04_w0_40 1.4e-05 2.4e-05 3.4e-05 2.9e-05 1.5e-05 5e-06 1e-06 0 0 0 0 0 0 0 0 0.000273 0.000477 0.000672 0.00058 0.000307 0.0001 2e-05 2.4e-05 1.2e-05 1e-06 0 0 0 0 0 0.091083 0.15912 0.22406 0.19349 0.10246 0.033247 0.006603 0.000802 5.9e-05 3e-06 0 0 0 0 0 i18_r0_04_w0_46 5e-06 1.3e-05 2.7e-05 3.4e-05 2.7e-05 1.3e-05 4e-06 1e-06 0 0 0 0 0 0 0 9e-05 0.000255 0.000532 0.000679 0.000532 0.000255 7.5e-05 0.000135 9.9e-05 7e-06 0 0 0 0 0 0.030063 0.085079 0.17719 0.22626 0.17719 0.085079 0.025028 0.004505 0.000496 3.3e-05 1e-06 0 0 0 0 i19_r0_04_w0_53 1e-06 5e-06 1.5e-05 2.9e-05 3.4e-05 2.4e-05 1e-05 3e-06 0 0 0 0 0 0 0 2.2e-05 0.0001 0.000307 0.00058 0.000672 0.000477 0.000208 0.000554 0.000603 6e-05 4e-06 0 0 0 0 0.007467 0.033247 0.10246 0.19349 0.22406 0.15912 0.069276 0.018474 0.003014 0.0003 1.8e-05 1e-06 0 0 0 i20_r0_04_w0_62 0 1e-06 6e-06 1.8e-05 3.1e-05 3.3e-05 2.1e-05 8e-06 2e-06 0 0 0 0 0 0 4e-06 2.8e-05 0.00013 0.000363 0.000622 0.000653 0.00042 0.001659 0.002674 0.000395 3.6e-05 2e-06 0 0 0 0.001381 0.009489 0.043306 0.121 0.2072 0.21758 0.14012 0.055312 0.013371 0.001977 0.000178 1e-05 0 0 0 i21_r0_04_w0_71 0 0 2e-06 8e-06 2.1e-05 3.3e-05 3.1e-05 1.8e-05 6e-06 1e-06 0 0 0 0 0 1e-06 6e-06 4e-05 0.000166 0.00042 0.000653 0.000622 0.00363 0.008661 0.001898 0.000254 2.1e-05 1e-06 0 0 0.000189 0.001977 0.013371 0.055312 0.14012 0.21758 0.2072 0.121 0.043306 0.009489 0.001271 0.000104 5e-06 0 0 i22_r0_04_w0_82 0 0 0 3e-06 1e-05 2.4e-05 3.4e-05 2.9e-05 1.5e-05 5e-06 1e-06 0 0 0 0 0 1e-06 9e-06 5.5e-05 0.000208 0.000477 0.000672 0.005805 0.020492 0.006649 0.001321 0.00016 1.2e-05 1e-06 0 1.9e-05 0.0003 0.003014 0.018474 0.069276 0.15912 0.22406 0.19349 0.10246 0.033247 0.006603 0.000802 5.9e-05 3e-06 0 i38_r0_06_w0_94 0 0 0 1e-06 4e-06 1.3e-05 2.7e-05 3.4e-05 2.7e-05 1.3e-05 4e-06 1e-06 0 0 0 0 0 1e-06 1.4e-05 7.5e-05 0.000255 0.000532 0.006788 0.035438 0.017016 0.005006 0.000901 9.9e-05 7e-06 0 1e-06 3.3e-05 0.000496 0.004505 0.025028 0.085079 0.17719 0.22626 0.17719 0.085079 0.025028 0.004505 0.000496 3.3e-05 1e-06 i54_r0_08_w1_09 0 0 0 0 1e-06 5e-06 1.5e-05 2.9e-05 3.4e-05 2.4e-05 1e-05 3e-06 0 0 0 0 0 0 2e-06 2e-05 0.0001 0.000307 0.005805 0.044812 0.031824 0.013855 0.003695 0.000603 6e-05 4e-06 0 3e-06 5.9e-05 0.000802 0.006603 0.033247 0.10246 0.19349 0.22406 0.15912 0.069276 0.018474 0.003014 0.0003 1.9e-05 i55_r0_08_w1_26 0 0 0 0 0 1e-06 6e-06 1.8e-05 3.1e-05 3.3e-05 2.1e-05 8e-06 2e-06 0 0 0 0 0 0 4e-06 2.8e-05 0.00013 0.00363 0.04144 0.043517 0.028024 0.011062 0.002674 0.000395 3.8e-05 0 0 5e-06 0.000104 0.001271 0.009489 0.043306 0.121 0.2072 0.21758 0.14012 0.055312 0.013371 0.001977 0.000189 i56_r0_08_w1_45 0 0 0 0 0 0 2e-06 8e-06 2.1e-05 3.3e-05 3.1e-05 1.8e-05 6e-06 1e-06 0 0 0 0 0 1e-06 6e-06 4e-05 0.001659 0.028024 0.043517 0.04144 0.0242 0.008661 0.001898 0.000276 0 0 0 1e-05 0.000178 0.001977 0.013371 0.055312 0.14012 0.21758 0.2072 0.121 0.043306 0.009489 0.001381 i57_r0_08_w1_67 0 0 0 0 0 0 0 3e-06 1e-05 2.4e-05 3.4e-05 2.9e-05 1.5e-05 5e-06 1e-06 0 0 0 0 0 1e-06 9e-06 0.000554 0.013855 0.031824 0.044812 0.038699 0.020492 0.006649 0.001493 0 0 0 1e-06 1.8e-05 0.0003 0.003014 0.018474 0.069276 0.15912 0.22406 0.19349 0.10246 0.033247 0.007467 i58_r0_08_w1_93 0 0 0 0 0 0 0 1e-06 4e-06 1.3e-05 2.7e-05 3.4e-05 2.7e-05 1.3e-05 5e-06 0 0 0 0 0 0 1e-06 0.000135 0.005006 0.017016 0.035438 0.045252 0.035438 0.017016 0.006013 0 0 0 0 1e-06 3.3e-05 0.000496 0.004505 0.025028 0.085079 0.17719 0.22626 0.17719 0.085079 0.030063 i59_r0_08_w2_23 0 0 0 0 0 0 0 0 1e-06 5e-06 1.5e-05 2.9e-05 3.4e-05 2.4e-05 1.4e-05 0 0 0 0 0 0 0 2.4e-05 0.001321 0.006649 0.020492 0.038699 0.044812 0.031824 0.018217 0 0 0 0 0 3e-06 5.9e-05 0.000802 0.006603 0.033247 0.10246 0.19349 0.22406 0.15912 0.091083 i60_r0_08_w2_57 0 0 0 0 0 0 0 0 0 1e-06 6e-06 1.8e-05 3.1e-05 3.3e-05 3.2e-05 0 0 0 0 0 0 0 3e-06 0.000254 0.001898 0.008661 0.0242 0.04144 0.043517 0.042194 0 0 0 0 0 0 5e-06 0.000104 0.001271 0.009489 0.043306 0.121 0.2072 0.21758 0.21097 i61_r0_10_w0_35 3.2e-05 3.3e-05 3.1e-05 1.8e-05 6e-06 1e-06 0 0 0 0 0 0 0 0 0 0.000633 0.000653 0.000622 0.000363 0.00013 2.8e-05 4e-06 3e-06 1e-06 0 0 0 0 0 0 0.21097 0.21758 0.2072 0.121 0.043306 0.009489 0.001271 0.000104 5e-06 0 0 0 0 0 0 i62_r0_10_w0_40 1.4e-05 2.4e-05 3.4e-05 2.9e-05 1.5e-05 5e-06 1e-06 0 0 0 0 0 0 0 0 0.000273 0.000477 0.000672 0.00058 0.000307 0.0001 2e-05 2.4e-05 1.2e-05 1e-06 0 0 0 0 0 0.091083 0.15912 0.22406 0.19349 0.10246 0.033247 0.006603 0.000802 5.9e-05 3e-06 0 0 0 0 0 i63_r0_10_w0_46 5e-06 1.3e-05 2.7e-05 3.4e-05 2.7e-05 1.3e-05 4e-06 1e-06 0 0 0 0 0 0 0 9e-05 0.000255 0.000532 0.000679 0.000532 0.000255 7.5e-05 0.000135 9.9e-05 7e-06 0 0 0 0 0 0.030063 0.085079 0.17719 0.22626 0.17719 0.085079 0.025028 0.004505 0.000496 3.3e-05 1e-06 0 0 0 0 i64_r0_10_w0_53 1e-06 5e-06 1.5e-05 2.9e-05 3.4e-05 2.4e-05 1e-05 3e-06 0 0 0 0 0 0 0 2.2e-05 0.0001 0.000307 0.00058 0.000672 0.000477 0.000208 0.000554 0.000603 6e-05 4e-06 0 0 0 0 0.007467 0.033247 0.10246 0.19349 0.22406 0.15912 0.069276 0.018474 0.003014 0.0003 1.8e-05 1e-06 0 0 0 i65_r0_10_w0_62 0 1e-06 6e-06 1.8e-05 3.1e-05 3.3e-05 2.1e-05 8e-06 2e-06 0 0 0 0 0 0 4e-06 2.8e-05 0.00013 0.000363 0.000622 0.000653 0.00042 0.001659 0.002674 0.000395 3.6e-05 2e-06 0 0 0 0.001381 0.009489 0.043306 0.121 0.2072 0.21758 0.14012 0.055312 0.013371 0.001977 0.000178 1e-05 0 0 0 i66_r0_10_w0_71 0 0 2e-06 8e-06 2.1e-05 3.3e-05 3.1e-05 1.8e-05 6e-06 1e-06 0 0 0 0 0 1e-06 6e-06 4e-05 0.000166 0.00042 0.000653 0.000622 0.00363 0.008661 0.001898 0.000254 2.1e-05 1e-06 0 0 0.000189 0.001977 0.013371 0.055312 0.14012 0.21758 0.2072 0.121 0.043306 0.009489 0.001271 0.000104 5e-06 0 0 i67_r0_10_w0_82 0 0 0 3e-06 1e-05 2.4e-05 3.4e-05 2.9e-05 1.5e-05 5e-06 1e-06 0 0 0 0 0 1e-06 9e-06 5.5e-05 0.000208 0.000477 0.000672 0.005805 0.020492 0.006649 0.001321 0.00016 1.2e-05 1e-06 0 1.9e-05 0.0003 0.003014 0.018474 0.069276 0.15912 0.22406 0.19349 0.10246 0.033247 0.006603 0.000802 5.9e-05 3e-06 0 i68_r0_10_w0_94 0 0 0 1e-06 4e-06 1.3e-05 2.7e-05 3.4e-05 2.7e-05 1.3e-05 4e-06 1e-06 0 0 0 0 0 1e-06 1.4e-05 7.5e-05 0.000255 0.000532 0.006788 0.035438 0.017016 0.005006 0.000901 9.9e-05 7e-06 0 1e-06 3.3e-05 0.000496 0.004505 0.025028 0.085079 0.17719 0.22626 0.17719 0.085079 0.025028 0.004505 0.000496 3.3e-05 1e-06 i69_r0_10_w1_09 0 0 0 0 1e-06 5e-06 1.5e-05 2.9e-05 3.4e-05 2.4e-05 1e-05 3e-06 0 0 0 0 0 0 2e-06 2e-05 0.0001 0.000307 0.005805 0.044812 0.031824 0.013855 0.003695 0.000603 6e-05 4e-06 0 3e-06 5.9e-05 0.000802 0.006603 0.033247 0.10246 0.19349 0.22406 0.15912 0.069276 0.018474 0.003014 0.0003 1.9e-05 i70_r0_10_w1_26 0 0 0 0 0 1e-06 6e-06 1.8e-05 3.1e-05 3.3e-05 2.1e-05 8e-06 2e-06 0 0 0 0 0 0 4e-06 2.8e-05 0.00013 0.00363 0.04144 0.043517 0.028024 0.011062 0.002674 0.000395 3.8e-05 0 0 5e-06 0.000104 0.001271 0.009489 0.043306 0.121 0.2072 0.21758 0.14012 0.055312 0.013371 0.001977 0.000189 i71_r0_10_w1_45 0 0 0 0 0 0 2e-06 8e-06 2.1e-05 3.3e-05 3.1e-05 1.8e-05 6e-06 1e-06 0 0 0 0 0 1e-06 6e-06 4e-05 0.001659 0.028024 0.043517 0.04144 0.0242 0.008661 0.001898 0.000276 0 0 0 1e-05 0.000178 0.001977 0.013371 0.055312 0.14012 0.21758 0.2072 0.121 0.043306 0.009489 0.001381 i72_r0_10_w1_67 0 0 0 0 0 0 0 3e-06 1e-05 2.4e-05 3.4e-05 2.9e-05 1.5e-05 5e-06 1e-06 0 0 0 0 0 1e-06 9e-06 0.000554 0.013855 0.031824 0.044812 0.038699 0.020492 0.006649 0.001493 0 0 0 1e-06 1.8e-05 0.0003 0.003014 0.018474 0.069276 0.15912 0.22406 0.19349 0.10246 0.033247 0.007467 i73_r0_10_w1_93 0 0 0 0 0 0 0 1e-06 4e-06 1.3e-05 2.7e-05 3.4e-05 2.7e-05 1.3e-05 5e-06 0 0 0 0 0 0 1e-06 0.000135 0.005006 0.017016 0.035438 0.045252 0.035438 0.017016 0.006013 0 0 0 0 1e-06 3.3e-05 0.000496 0.004505 0.025028 0.085079 0.17719 0.22626 0.17719 0.085079 0.030063 i74_r0_10_w2_23 0 0 0 0 0 0 0 0 1e-06 5e-06 1.5e-05 2.9e-05 3.4e-05 2.4e-05 1.4e-05 0 0 0 0 0 0 0 2.4e-05 0.001321 0.006649 0.020492 0.038699 0.044812 0.031824 0.018217 0 0 0 0 0 3e-06 5.9e-05 0.000802 0.006603 0.033247 0.10246 0.19349 0.22406 0.15912 0.091083 i75_r0_10_w2_57 0 0 0 0 0 0 0 0 0 1e-06 6e-06 1.8e-05 3.1e-05 3.3e-05 3.2e-05 0 0 0 0 0 0 0 3e-06 0.000254 0.001898 0.008661 0.0242 0.04144 0.043517 0.042194 0 0 0 0 0 0 5e-06 0.000104 0.001271 0.009489 0.043306 0.121 0.2072 0.21758 0.21097
Get Equations
[f_util_log, f_util_crra, f_util_standin, f_inc, f_coh, f_cons_coh_fbis, f_cons_coh_save, f_bprime] = ...
ffs_abzr_fibs_set_functions(fl_crra, fl_c_min, fl_r_fbr, fl_r_fsv, fl_w);
Get Formal Borrowing Blocks
[ar_forbrblk, ar_forbrblk_r] = ...
ffs_for_br_block_gen(fl_r_fbr, st_forbrblk_type, fl_forbrblk_brmost, fl_forbrblk_brleast, fl_forbrblk_gap);
Get Asset and Choice Grid
note this requires ar_z
if (bl_loglin) % C:\Users\fan\M4Econ\asset\grid\ff_grid_loglin.m ar_a = fft_gen_grid_loglin(it_a_n, fl_a_max, fl_a_min, fl_loglin_threshold); else fl_r_inf_max = max(ar_z_r_infbr); [ar_a_inf, fl_borr_yminbd_inf, fl_borr_ymaxbd_inf] = ffs_abz_gen_borrsave_grid(... fl_b_bd, bl_default, ar_z_wage, fl_w, ... bl_b_is_principle, fl_r_inf_max, fl_a_min, fl_a_max, it_a_n); [ar_a_for, fl_borr_yminbd_for, fl_borr_ymaxbd_for] = ffs_abz_gen_borrsave_grid(... fl_b_bd, bl_default, ar_z_wage, fl_w, ... bl_b_is_principle, fl_r_fbr, fl_a_min, fl_a_max, it_a_n); if (min(ar_a_for) <= min(ar_a_inf)) ar_a = ar_a_for; fl_borr_yminbd = fl_borr_yminbd_for; fl_borr_ymaxbd = fl_borr_ymaxbd_for; else ar_a = ar_a_inf; fl_borr_yminbd = fl_borr_yminbd_inf; fl_borr_ymaxbd = fl_borr_ymaxbd_inf; end end
Store
armt_map = containers.Map('KeyType','char', 'ValueType','any'); armt_map('ar_a') = ar_a; armt_map('mt_z_trans') = mt_z_trans; armt_map('ar_z_r_infbr_mesh_wage') = ar_z_r_infbr_mesh_wage; armt_map('ar_z_wage_mesh_r_infbr') = ar_z_wage_mesh_r_infbr; armt_map('ar_forbrblk') = ar_forbrblk; armt_map('ar_forbrblk_r') = ar_forbrblk_r; func_map = containers.Map('KeyType','char', 'ValueType','any'); func_map('f_util_log') = f_util_log; func_map('f_util_crra') = f_util_crra; func_map('f_util_standin') = f_util_standin; func_map('f_inc') = f_inc; func_map('f_coh') = f_coh; func_map('f_cons_coh_fbis') = f_cons_coh_fbis; func_map('f_cons_coh_save') = f_cons_coh_save; func_map('f_bprime') = f_bprime;
Graph: A, Shocks, COH, and Defaults
- y-axis : coh(a,z)
- x-axis : a
- color: z
- overlay: coh points points where there is default.
if (bl_graph_funcgrids) % mesh a and and z [mt_a_mesh_z, mt_z_mesh_a] = ndgrid(ar_a, ar_z_wage); % cash-on-hand given a and z mt_coh = f_coh(mt_z_mesh_a, mt_a_mesh_z); % loop over level vs log graphs for sub_j=1:1:1 figure('PaperPosition', [0 0 7 4]); if (sub_j == 1) x_mat = mt_a_mesh_z; y_mat = mt_coh; st_title = 'coh(a,z)'; st_ylabel = 'Cash-on-hand(a, z)'; st_xlabel = 'Asset States (Choices)'; fl_b_bd_graph = fl_b_bd; fl_borr_yminbd_graph = fl_borr_yminbd; fl_borr_ymaxbd_graph = fl_borr_ymaxbd; else x_mat = log(mt_a_mesh_z - min(min(mt_a_mesh_z)) + 1); y_mat = log(mt_coh - min(min(mt_coh)) + 1); st_title = 'coh(a,z) log scale'; st_ylabel = 'log(Cash-on-hand(a, z) - min(coh) + 1)'; st_xlabel = 'log(a - min(a) + 1)'; fl_b_bd_graph = log(fl_b_bd - min(min(mt_a_mesh_z)) + 1); fl_borr_yminbd_graph = log(fl_borr_yminbd - min(min(mt_a_mesh_z)) + 1); fl_borr_ymaxbd_graph = log(fl_borr_ymaxbd - min(min(mt_a_mesh_z)) + 1); end % plot main x and y chart = plot(x_mat, y_mat, 'blue'); % add color based on z clr = jet(numel(chart)); for m = 1:numel(chart) set(chart(m), 'Color', clr(m,:)) end % if (length(ar_w_level_full) <= 100) % scatter(ar_a_meshk, ar_k_mesha, 3, 'filled', ... % 'MarkerEdgeColor', 'b', 'MarkerFaceColor', 'b'); % end % if (length(ar_w_level_full) <= 100) % gf_invalid_scatter = scatter(ar_a_meshk_full(ar_bl_wkb_invalid),... % ar_k_mesha_full(ar_bl_wkb_invalid),... % 20, 'O', 'MarkerEdgeColor', 'black', 'MarkerFaceColor', 'black'); % end % add various borrowing bound lines % add 0 lines xline(0); yline(0); % add 45 degrees line hline = refline([1 0]); hline.Color = 'k'; hline.LineStyle = ':'; hline.HandleVisibility = 'off'; hline.LineWidth = 2.5; title(st_title) ylabel(st_ylabel) grid on; grid minor; legend2plot = fliplr([1 round(numel(chart)/3) round((2*numel(chart))/4) numel(chart)]); legendCell = cellstr(num2str(ar_z_wage', 'z=%3.2f')); chart(length(chart)+1) = hline; legendCell{length(legendCell) + 1} = 'if coh(a,z) >= a'; legend2plot = [legend2plot length(legendCell)]; % if borrow plot additional borrowing bound lines if (fl_b_bd >= 0 ) ar_legend_ele = [legend2plot]; xlabel({st_xlabel}) else % add fl_b_bd exo borrow line if (fl_b_bd >= min(ar_a)) xline_borrbound = xline(fl_b_bd_graph); xline_borrbound.HandleVisibility = 'on'; xline_borrbound.LineStyle = '-'; xline_borrbound.Color = 'black'; xline_borrbound.LineWidth = 2.5; yline_borrbound = yline(fl_b_bd_graph); yline_borrbound.HandleVisibility = 'off'; yline_borrbound.LineStyle = '-'; yline_borrbound.Color = 'black'; yline_borrbound.LineWidth = 1; end xline_yminbd = xline(fl_borr_yminbd_graph); xline_yminbd.HandleVisibility = 'on'; xline_yminbd.LineStyle = '--'; xline_yminbd.Color = 'red'; xline_yminbd.LineWidth = 2.5; yline_yminbd = yline(fl_borr_yminbd_graph); yline_yminbd.HandleVisibility = 'off'; yline_yminbd.LineStyle = '--'; yline_yminbd.Color = 'red'; yline_yminbd.LineWidth = 1; if (bl_default) xline_ymaxbd = xline(fl_borr_ymaxbd_graph); xline_ymaxbd.HandleVisibility = 'on'; xline_ymaxbd.LineStyle = '--'; xline_ymaxbd.Color = 'blue'; xline_ymaxbd.LineWidth = 2.5; yline_ymaxbd = yline(fl_borr_ymaxbd_graph); yline_ymaxbd.HandleVisibility = 'on'; yline_ymaxbd.LineStyle = ':'; yline_ymaxbd.Color = 'blue'; yline_ymaxbd.LineWidth = 2.5; end % add bound line legends it_addlines_cn = 0; if (fl_b_bd >= min(ar_a)) it_addlines_cn = it_addlines_cn + 1; chart(length(chart)+1) = xline_borrbound; legendCell{length(legendCell) + 1} = 'exo. borrow bound fbbd'; end it_addlines_cn = it_addlines_cn + 1; chart(length(chart)+1) = xline_yminbd; legendCell{length(legendCell) + 1} = 'neg min inc: -zmin*w/r (no default)'; if (bl_default) it_addlines_cn = it_addlines_cn + 1; chart(length(chart)+1) = xline_ymaxbd; legendCell{length(legendCell) + 1} = 'neg max inc: -zmax*w/r (default)'; it_addlines_cn = it_addlines_cn + 1; chart(length(chart)+1) = yline_ymaxbd; legendCell{length(legendCell) + 1} = 'must default if coh(a,z)<dot-line'; end % draw legend ar_legend_ele = [legend2plot length(legendCell)-it_addlines_cn:1:length(legendCell)]; xlabel({st_xlabel 'if coh(a,z) < a, then a''(a,z)<a'}) end % draw legends legend(chart(unique(ar_legend_ele)), legendCell(unique(ar_legend_ele)), 'Location', 'northwest'); end end
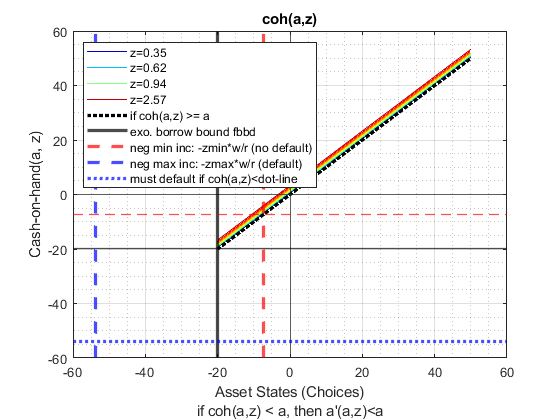
Display
if (bl_display_funcgrids) disp('ar_z_wage'); disp(size(ar_z_wage)); disp(ar_z_wage); disp('mt_z_trans'); disp(size(mt_z_trans)); disp(mt_z_trans); disp('ar_forbrblk, ar_forbrblk_r'); disp(size(ar_forbrblk)); disp([ar_forbrblk;ar_forbrblk_r]'); param_map_keys = keys(func_map); param_map_vals = values(func_map); for i = 1:length(func_map) st_display = strjoin(['pos =' num2str(i) '; key =' string(param_map_keys{i}) '; val =' func2str(param_map_vals{i})]); disp(st_display); end end
ar_z_wage 1 15 Columns 1 through 7 0.3474 0.4008 0.4623 0.5333 0.6152 0.7097 0.8186 Columns 8 through 14 0.9444 1.0894 1.2567 1.4496 1.6723 1.9291 2.2253 Column 15 2.5670 mt_z_trans 75 75 Columns 1 through 7 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 Columns 8 through 14 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 Columns 15 through 21 0 0.0006 0.0007 0.0006 0.0004 0.0001 0.0000 0.0000 0.0003 0.0005 0.0007 0.0006 0.0003 0.0001 0.0000 0.0001 0.0003 0.0005 0.0007 0.0005 0.0003 0.0000 0.0000 0.0001 0.0003 0.0006 0.0007 0.0005 0.0000 0.0000 0.0000 0.0001 0.0004 0.0006 0.0007 0.0000 0.0000 0.0000 0.0000 0.0002 0.0004 0.0007 0.0000 0.0000 0.0000 0.0000 0.0001 0.0002 0.0005 0.0000 0.0000 0.0000 0.0000 0.0000 0.0001 0.0003 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0001 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0 0.0006 0.0007 0.0006 0.0004 0.0001 0.0000 0.0000 0.0003 0.0005 0.0007 0.0006 0.0003 0.0001 0.0000 0.0001 0.0003 0.0005 0.0007 0.0005 0.0003 0.0000 0.0000 0.0001 0.0003 0.0006 0.0007 0.0005 0.0000 0.0000 0.0000 0.0001 0.0004 0.0006 0.0007 0.0000 0.0000 0.0000 0.0000 0.0002 0.0004 0.0007 0.0000 0.0000 0.0000 0.0000 0.0001 0.0002 0.0005 0.0000 0.0000 0.0000 0.0000 0.0000 0.0001 0.0003 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0001 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0 0.0006 0.0007 0.0006 0.0004 0.0001 0.0000 0.0000 0.0003 0.0005 0.0007 0.0006 0.0003 0.0001 0.0000 0.0001 0.0003 0.0005 0.0007 0.0005 0.0003 0.0000 0.0000 0.0001 0.0003 0.0006 0.0007 0.0005 0.0000 0.0000 0.0000 0.0001 0.0004 0.0006 0.0007 0.0000 0.0000 0.0000 0.0000 0.0002 0.0004 0.0007 0.0000 0.0000 0.0000 0.0000 0.0001 0.0002 0.0005 0.0000 0.0000 0.0000 0.0000 0.0000 0.0001 0.0003 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0001 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0 0.0006 0.0007 0.0006 0.0004 0.0001 0.0000 0.0000 0.0003 0.0005 0.0007 0.0006 0.0003 0.0001 0.0000 0.0001 0.0003 0.0005 0.0007 0.0005 0.0003 0.0000 0.0000 0.0001 0.0003 0.0006 0.0007 0.0005 0.0000 0.0000 0.0000 0.0001 0.0004 0.0006 0.0007 0.0000 0.0000 0.0000 0.0000 0.0002 0.0004 0.0007 0.0000 0.0000 0.0000 0.0000 0.0001 0.0002 0.0005 0.0000 0.0000 0.0000 0.0000 0.0000 0.0001 0.0003 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0001 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0 0.0006 0.0007 0.0006 0.0004 0.0001 0.0000 0.0000 0.0003 0.0005 0.0007 0.0006 0.0003 0.0001 0.0000 0.0001 0.0003 0.0005 0.0007 0.0005 0.0003 0.0000 0.0000 0.0001 0.0003 0.0006 0.0007 0.0005 0.0000 0.0000 0.0000 0.0001 0.0004 0.0006 0.0007 0.0000 0.0000 0.0000 0.0000 0.0002 0.0004 0.0007 0.0000 0.0000 0.0000 0.0000 0.0001 0.0002 0.0005 0.0000 0.0000 0.0000 0.0000 0.0000 0.0001 0.0003 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0001 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 Columns 22 through 28 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0001 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0002 0.0001 0.0000 0.0000 0.0000 0.0000 0.0000 0.0004 0.0002 0.0000 0.0000 0.0000 0.0000 0.0000 0.0006 0.0004 0.0001 0.0000 0.0000 0.0000 0.0000 0.0007 0.0006 0.0003 0.0001 0.0000 0.0000 0.0000 0.0005 0.0007 0.0005 0.0003 0.0001 0.0000 0.0000 0.0003 0.0006 0.0007 0.0005 0.0002 0.0001 0.0000 0.0001 0.0004 0.0006 0.0007 0.0004 0.0002 0.0000 0.0000 0.0002 0.0004 0.0007 0.0006 0.0004 0.0001 0.0000 0.0001 0.0002 0.0005 0.0007 0.0006 0.0003 0.0000 0.0000 0.0001 0.0003 0.0005 0.0007 0.0005 0.0000 0.0000 0.0000 0.0001 0.0003 0.0006 0.0007 0.0000 0.0000 0.0000 0.0000 0.0001 0.0004 0.0006 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0001 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0002 0.0001 0.0000 0.0000 0.0000 0.0000 0.0000 0.0004 0.0002 0.0000 0.0000 0.0000 0.0000 0.0000 0.0006 0.0004 0.0001 0.0000 0.0000 0.0000 0.0000 0.0007 0.0006 0.0003 0.0001 0.0000 0.0000 0.0000 0.0005 0.0007 0.0005 0.0003 0.0001 0.0000 0.0000 0.0003 0.0006 0.0007 0.0005 0.0002 0.0001 0.0000 0.0001 0.0004 0.0006 0.0007 0.0004 0.0002 0.0000 0.0000 0.0002 0.0004 0.0007 0.0006 0.0004 0.0001 0.0000 0.0001 0.0002 0.0005 0.0007 0.0006 0.0003 0.0000 0.0000 0.0001 0.0003 0.0005 0.0007 0.0005 0.0000 0.0000 0.0000 0.0001 0.0003 0.0006 0.0007 0.0000 0.0000 0.0000 0.0000 0.0001 0.0004 0.0006 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0001 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0002 0.0001 0.0000 0.0000 0.0000 0.0000 0.0000 0.0004 0.0002 0.0000 0.0000 0.0000 0.0000 0.0000 0.0006 0.0004 0.0001 0.0000 0.0000 0.0000 0.0000 0.0007 0.0006 0.0003 0.0001 0.0000 0.0000 0.0000 0.0005 0.0007 0.0005 0.0003 0.0001 0.0000 0.0000 0.0003 0.0006 0.0007 0.0005 0.0002 0.0001 0.0000 0.0001 0.0004 0.0006 0.0007 0.0004 0.0002 0.0000 0.0000 0.0002 0.0004 0.0007 0.0006 0.0004 0.0001 0.0000 0.0001 0.0002 0.0005 0.0007 0.0006 0.0003 0.0000 0.0000 0.0001 0.0003 0.0005 0.0007 0.0005 0.0000 0.0000 0.0000 0.0001 0.0003 0.0006 0.0007 0.0000 0.0000 0.0000 0.0000 0.0001 0.0004 0.0006 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0001 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0002 0.0001 0.0000 0.0000 0.0000 0.0000 0.0000 0.0004 0.0002 0.0000 0.0000 0.0000 0.0000 0.0000 0.0006 0.0004 0.0001 0.0000 0.0000 0.0000 0.0000 0.0007 0.0006 0.0003 0.0001 0.0000 0.0000 0.0000 0.0005 0.0007 0.0005 0.0003 0.0001 0.0000 0.0000 0.0003 0.0006 0.0007 0.0005 0.0002 0.0001 0.0000 0.0001 0.0004 0.0006 0.0007 0.0004 0.0002 0.0000 0.0000 0.0002 0.0004 0.0007 0.0006 0.0004 0.0001 0.0000 0.0001 0.0002 0.0005 0.0007 0.0006 0.0003 0.0000 0.0000 0.0001 0.0003 0.0005 0.0007 0.0005 0.0000 0.0000 0.0000 0.0001 0.0003 0.0006 0.0007 0.0000 0.0000 0.0000 0.0000 0.0001 0.0004 0.0006 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0001 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0002 0.0001 0.0000 0.0000 0.0000 0.0000 0.0000 0.0004 0.0002 0.0000 0.0000 0.0000 0.0000 0.0000 0.0006 0.0004 0.0001 0.0000 0.0000 0.0000 0.0000 0.0007 0.0006 0.0003 0.0001 0.0000 0.0000 0.0000 0.0005 0.0007 0.0005 0.0003 0.0001 0.0000 0.0000 0.0003 0.0006 0.0007 0.0005 0.0002 0.0001 0.0000 0.0001 0.0004 0.0006 0.0007 0.0004 0.0002 0.0000 0.0000 0.0002 0.0004 0.0007 0.0006 0.0004 0.0001 0.0000 0.0001 0.0002 0.0005 0.0007 0.0006 0.0003 0.0000 0.0000 0.0001 0.0003 0.0005 0.0007 0.0005 0.0000 0.0000 0.0000 0.0001 0.0003 0.0006 0.0007 0.0000 0.0000 0.0000 0.0000 0.0001 0.0004 0.0006 Columns 29 through 35 0.0000 0 0.0063 0.0065 0.0062 0.0036 0.0013 0.0000 0.0000 0.0027 0.0048 0.0067 0.0058 0.0031 0.0000 0.0000 0.0009 0.0026 0.0053 0.0068 0.0053 0.0000 0.0000 0.0002 0.0010 0.0031 0.0058 0.0067 0.0000 0.0000 0.0000 0.0003 0.0013 0.0036 0.0062 0.0000 0.0000 0.0000 0.0001 0.0004 0.0017 0.0042 0.0000 0.0000 0.0000 0.0000 0.0001 0.0006 0.0021 0.0000 0.0000 0.0000 0.0000 0.0000 0.0001 0.0008 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0002 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0001 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0003 0.0001 0.0000 0.0000 0.0000 0.0000 0.0000 0.0005 0.0003 0.0000 0.0000 0.0000 0.0000 0.0000 0.0007 0.0006 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0 0.0063 0.0065 0.0062 0.0036 0.0013 0.0000 0.0000 0.0027 0.0048 0.0067 0.0058 0.0031 0.0000 0.0000 0.0009 0.0026 0.0053 0.0068 0.0053 0.0000 0.0000 0.0002 0.0010 0.0031 0.0058 0.0067 0.0000 0.0000 0.0000 0.0003 0.0013 0.0036 0.0062 0.0000 0.0000 0.0000 0.0001 0.0004 0.0017 0.0042 0.0000 0.0000 0.0000 0.0000 0.0001 0.0006 0.0021 0.0000 0.0000 0.0000 0.0000 0.0000 0.0001 0.0008 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0002 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0001 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0003 0.0001 0.0000 0.0000 0.0000 0.0000 0.0000 0.0005 0.0003 0.0000 0.0000 0.0000 0.0000 0.0000 0.0007 0.0006 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0 0.0063 0.0065 0.0062 0.0036 0.0013 0.0000 0.0000 0.0027 0.0048 0.0067 0.0058 0.0031 0.0000 0.0000 0.0009 0.0026 0.0053 0.0068 0.0053 0.0000 0.0000 0.0002 0.0010 0.0031 0.0058 0.0067 0.0000 0.0000 0.0000 0.0003 0.0013 0.0036 0.0062 0.0000 0.0000 0.0000 0.0001 0.0004 0.0017 0.0042 0.0000 0.0000 0.0000 0.0000 0.0001 0.0006 0.0021 0.0000 0.0000 0.0000 0.0000 0.0000 0.0001 0.0008 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0002 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0001 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0003 0.0001 0.0000 0.0000 0.0000 0.0000 0.0000 0.0005 0.0003 0.0000 0.0000 0.0000 0.0000 0.0000 0.0007 0.0006 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0 0.0063 0.0065 0.0062 0.0036 0.0013 0.0000 0.0000 0.0027 0.0048 0.0067 0.0058 0.0031 0.0000 0.0000 0.0009 0.0026 0.0053 0.0068 0.0053 0.0000 0.0000 0.0002 0.0010 0.0031 0.0058 0.0067 0.0000 0.0000 0.0000 0.0003 0.0013 0.0036 0.0062 0.0000 0.0000 0.0000 0.0001 0.0004 0.0017 0.0042 0.0000 0.0000 0.0000 0.0000 0.0001 0.0006 0.0021 0.0000 0.0000 0.0000 0.0000 0.0000 0.0001 0.0008 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0002 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0001 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0003 0.0001 0.0000 0.0000 0.0000 0.0000 0.0000 0.0005 0.0003 0.0000 0.0000 0.0000 0.0000 0.0000 0.0007 0.0006 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0 0.0063 0.0065 0.0062 0.0036 0.0013 0.0000 0.0000 0.0027 0.0048 0.0067 0.0058 0.0031 0.0000 0.0000 0.0009 0.0026 0.0053 0.0068 0.0053 0.0000 0.0000 0.0002 0.0010 0.0031 0.0058 0.0067 0.0000 0.0000 0.0000 0.0003 0.0013 0.0036 0.0062 0.0000 0.0000 0.0000 0.0001 0.0004 0.0017 0.0042 0.0000 0.0000 0.0000 0.0000 0.0001 0.0006 0.0021 0.0000 0.0000 0.0000 0.0000 0.0000 0.0001 0.0008 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0002 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0001 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0003 0.0001 0.0000 0.0000 0.0000 0.0000 0.0000 0.0005 0.0003 0.0000 0.0000 0.0000 0.0000 0.0000 0.0007 0.0006 0.0000 0.0000 0.0000 0.0000 0.0000 Columns 36 through 42 0.0003 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0010 0.0002 0.0000 0.0000 0.0000 0.0000 0.0000 0.0026 0.0008 0.0001 0.0000 0.0000 0.0000 0.0000 0.0048 0.0021 0.0006 0.0001 0.0000 0.0000 0.0000 0.0065 0.0042 0.0017 0.0004 0.0001 0.0000 0.0000 0.0065 0.0062 0.0036 0.0013 0.0003 0.0000 0.0000 0.0048 0.0067 0.0058 0.0031 0.0010 0.0002 0.0000 0.0026 0.0053 0.0068 0.0053 0.0026 0.0008 0.0001 0.0010 0.0031 0.0058 0.0067 0.0048 0.0021 0.0006 0.0003 0.0013 0.0036 0.0062 0.0065 0.0042 0.0017 0.0001 0.0004 0.0017 0.0042 0.0065 0.0062 0.0036 0.0000 0.0001 0.0006 0.0021 0.0048 0.0067 0.0058 0.0000 0.0000 0.0001 0.0008 0.0026 0.0053 0.0068 0.0000 0.0000 0.0000 0.0002 0.0010 0.0031 0.0058 0.0000 0.0000 0.0000 0.0000 0.0003 0.0013 0.0036 0.0003 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0010 0.0002 0.0000 0.0000 0.0000 0.0000 0.0000 0.0026 0.0008 0.0001 0.0000 0.0000 0.0000 0.0000 0.0048 0.0021 0.0006 0.0001 0.0000 0.0000 0.0000 0.0065 0.0042 0.0017 0.0004 0.0001 0.0000 0.0000 0.0065 0.0062 0.0036 0.0013 0.0003 0.0000 0.0000 0.0048 0.0067 0.0058 0.0031 0.0010 0.0002 0.0000 0.0026 0.0053 0.0068 0.0053 0.0026 0.0008 0.0001 0.0010 0.0031 0.0058 0.0067 0.0048 0.0021 0.0006 0.0003 0.0013 0.0036 0.0062 0.0065 0.0042 0.0017 0.0001 0.0004 0.0017 0.0042 0.0065 0.0062 0.0036 0.0000 0.0001 0.0006 0.0021 0.0048 0.0067 0.0058 0.0000 0.0000 0.0001 0.0008 0.0026 0.0053 0.0068 0.0000 0.0000 0.0000 0.0002 0.0010 0.0031 0.0058 0.0000 0.0000 0.0000 0.0000 0.0003 0.0013 0.0036 0.0003 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0010 0.0002 0.0000 0.0000 0.0000 0.0000 0.0000 0.0026 0.0008 0.0001 0.0000 0.0000 0.0000 0.0000 0.0048 0.0021 0.0006 0.0001 0.0000 0.0000 0.0000 0.0065 0.0042 0.0017 0.0004 0.0001 0.0000 0.0000 0.0065 0.0062 0.0036 0.0013 0.0003 0.0000 0.0000 0.0048 0.0067 0.0058 0.0031 0.0010 0.0002 0.0000 0.0026 0.0053 0.0068 0.0053 0.0026 0.0008 0.0001 0.0010 0.0031 0.0058 0.0067 0.0048 0.0021 0.0006 0.0003 0.0013 0.0036 0.0062 0.0065 0.0042 0.0017 0.0001 0.0004 0.0017 0.0042 0.0065 0.0062 0.0036 0.0000 0.0001 0.0006 0.0021 0.0048 0.0067 0.0058 0.0000 0.0000 0.0001 0.0008 0.0026 0.0053 0.0068 0.0000 0.0000 0.0000 0.0002 0.0010 0.0031 0.0058 0.0000 0.0000 0.0000 0.0000 0.0003 0.0013 0.0036 0.0003 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0010 0.0002 0.0000 0.0000 0.0000 0.0000 0.0000 0.0026 0.0008 0.0001 0.0000 0.0000 0.0000 0.0000 0.0048 0.0021 0.0006 0.0001 0.0000 0.0000 0.0000 0.0065 0.0042 0.0017 0.0004 0.0001 0.0000 0.0000 0.0065 0.0062 0.0036 0.0013 0.0003 0.0000 0.0000 0.0048 0.0067 0.0058 0.0031 0.0010 0.0002 0.0000 0.0026 0.0053 0.0068 0.0053 0.0026 0.0008 0.0001 0.0010 0.0031 0.0058 0.0067 0.0048 0.0021 0.0006 0.0003 0.0013 0.0036 0.0062 0.0065 0.0042 0.0017 0.0001 0.0004 0.0017 0.0042 0.0065 0.0062 0.0036 0.0000 0.0001 0.0006 0.0021 0.0048 0.0067 0.0058 0.0000 0.0000 0.0001 0.0008 0.0026 0.0053 0.0068 0.0000 0.0000 0.0000 0.0002 0.0010 0.0031 0.0058 0.0000 0.0000 0.0000 0.0000 0.0003 0.0013 0.0036 0.0003 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0010 0.0002 0.0000 0.0000 0.0000 0.0000 0.0000 0.0026 0.0008 0.0001 0.0000 0.0000 0.0000 0.0000 0.0048 0.0021 0.0006 0.0001 0.0000 0.0000 0.0000 0.0065 0.0042 0.0017 0.0004 0.0001 0.0000 0.0000 0.0065 0.0062 0.0036 0.0013 0.0003 0.0000 0.0000 0.0048 0.0067 0.0058 0.0031 0.0010 0.0002 0.0000 0.0026 0.0053 0.0068 0.0053 0.0026 0.0008 0.0001 0.0010 0.0031 0.0058 0.0067 0.0048 0.0021 0.0006 0.0003 0.0013 0.0036 0.0062 0.0065 0.0042 0.0017 0.0001 0.0004 0.0017 0.0042 0.0065 0.0062 0.0036 0.0000 0.0001 0.0006 0.0021 0.0048 0.0067 0.0058 0.0000 0.0000 0.0001 0.0008 0.0026 0.0053 0.0068 0.0000 0.0000 0.0000 0.0002 0.0010 0.0031 0.0058 0.0000 0.0000 0.0000 0.0000 0.0003 0.0013 0.0036 Columns 43 through 49 0.0000 0.0000 0 0.0422 0.0435 0.0414 0.0242 0.0000 0.0000 0.0000 0.0182 0.0318 0.0448 0.0387 0.0000 0.0000 0.0000 0.0060 0.0170 0.0354 0.0453 0.0000 0.0000 0.0000 0.0015 0.0066 0.0205 0.0387 0.0000 0.0000 0.0000 0.0003 0.0019 0.0087 0.0242 0.0000 0.0000 0.0000 0.0000 0.0004 0.0027 0.0111 0.0000 0.0000 0.0000 0.0000 0.0001 0.0006 0.0037 0.0000 0.0000 0.0000 0.0000 0.0000 0.0001 0.0009 0.0001 0.0000 0.0000 0.0000 0.0000 0.0000 0.0002 0.0004 0.0001 0.0000 0.0000 0.0000 0.0000 0.0000 0.0013 0.0003 0.0000 0.0000 0.0000 0.0000 0.0000 0.0031 0.0010 0.0002 0.0000 0.0000 0.0000 0.0000 0.0053 0.0026 0.0009 0.0000 0.0000 0.0000 0.0000 0.0067 0.0048 0.0027 0.0000 0.0000 0.0000 0.0000 0.0062 0.0065 0.0063 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0 0.0422 0.0435 0.0414 0.0242 0.0000 0.0000 0.0000 0.0182 0.0318 0.0448 0.0387 0.0000 0.0000 0.0000 0.0060 0.0170 0.0354 0.0453 0.0000 0.0000 0.0000 0.0015 0.0066 0.0205 0.0387 0.0000 0.0000 0.0000 0.0003 0.0019 0.0087 0.0242 0.0000 0.0000 0.0000 0.0000 0.0004 0.0027 0.0111 0.0000 0.0000 0.0000 0.0000 0.0001 0.0006 0.0037 0.0000 0.0000 0.0000 0.0000 0.0000 0.0001 0.0009 0.0001 0.0000 0.0000 0.0000 0.0000 0.0000 0.0002 0.0004 0.0001 0.0000 0.0000 0.0000 0.0000 0.0000 0.0013 0.0003 0.0000 0.0000 0.0000 0.0000 0.0000 0.0031 0.0010 0.0002 0.0000 0.0000 0.0000 0.0000 0.0053 0.0026 0.0009 0.0000 0.0000 0.0000 0.0000 0.0067 0.0048 0.0027 0.0000 0.0000 0.0000 0.0000 0.0062 0.0065 0.0063 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0 0.0422 0.0435 0.0414 0.0242 0.0000 0.0000 0.0000 0.0182 0.0318 0.0448 0.0387 0.0000 0.0000 0.0000 0.0060 0.0170 0.0354 0.0453 0.0000 0.0000 0.0000 0.0015 0.0066 0.0205 0.0387 0.0000 0.0000 0.0000 0.0003 0.0019 0.0087 0.0242 0.0000 0.0000 0.0000 0.0000 0.0004 0.0027 0.0111 0.0000 0.0000 0.0000 0.0000 0.0001 0.0006 0.0037 0.0000 0.0000 0.0000 0.0000 0.0000 0.0001 0.0009 0.0001 0.0000 0.0000 0.0000 0.0000 0.0000 0.0002 0.0004 0.0001 0.0000 0.0000 0.0000 0.0000 0.0000 0.0013 0.0003 0.0000 0.0000 0.0000 0.0000 0.0000 0.0031 0.0010 0.0002 0.0000 0.0000 0.0000 0.0000 0.0053 0.0026 0.0009 0.0000 0.0000 0.0000 0.0000 0.0067 0.0048 0.0027 0.0000 0.0000 0.0000 0.0000 0.0062 0.0065 0.0063 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0 0.0422 0.0435 0.0414 0.0242 0.0000 0.0000 0.0000 0.0182 0.0318 0.0448 0.0387 0.0000 0.0000 0.0000 0.0060 0.0170 0.0354 0.0453 0.0000 0.0000 0.0000 0.0015 0.0066 0.0205 0.0387 0.0000 0.0000 0.0000 0.0003 0.0019 0.0087 0.0242 0.0000 0.0000 0.0000 0.0000 0.0004 0.0027 0.0111 0.0000 0.0000 0.0000 0.0000 0.0001 0.0006 0.0037 0.0000 0.0000 0.0000 0.0000 0.0000 0.0001 0.0009 0.0001 0.0000 0.0000 0.0000 0.0000 0.0000 0.0002 0.0004 0.0001 0.0000 0.0000 0.0000 0.0000 0.0000 0.0013 0.0003 0.0000 0.0000 0.0000 0.0000 0.0000 0.0031 0.0010 0.0002 0.0000 0.0000 0.0000 0.0000 0.0053 0.0026 0.0009 0.0000 0.0000 0.0000 0.0000 0.0067 0.0048 0.0027 0.0000 0.0000 0.0000 0.0000 0.0062 0.0065 0.0063 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0 0.0422 0.0435 0.0414 0.0242 0.0000 0.0000 0.0000 0.0182 0.0318 0.0448 0.0387 0.0000 0.0000 0.0000 0.0060 0.0170 0.0354 0.0453 0.0000 0.0000 0.0000 0.0015 0.0066 0.0205 0.0387 0.0000 0.0000 0.0000 0.0003 0.0019 0.0087 0.0242 0.0000 0.0000 0.0000 0.0000 0.0004 0.0027 0.0111 0.0000 0.0000 0.0000 0.0000 0.0001 0.0006 0.0037 0.0000 0.0000 0.0000 0.0000 0.0000 0.0001 0.0009 0.0001 0.0000 0.0000 0.0000 0.0000 0.0000 0.0002 0.0004 0.0001 0.0000 0.0000 0.0000 0.0000 0.0000 0.0013 0.0003 0.0000 0.0000 0.0000 0.0000 0.0000 0.0031 0.0010 0.0002 0.0000 0.0000 0.0000 0.0000 0.0053 0.0026 0.0009 0.0000 0.0000 0.0000 0.0000 0.0067 0.0048 0.0027 0.0000 0.0000 0.0000 0.0000 0.0062 0.0065 0.0063 0.0000 0.0000 0.0000 0.0000 Columns 50 through 56 0.0087 0.0019 0.0003 0.0000 0.0000 0.0000 0.0000 0.0205 0.0066 0.0013 0.0002 0.0000 0.0000 0.0000 0.0354 0.0170 0.0050 0.0009 0.0001 0.0000 0.0000 0.0448 0.0318 0.0139 0.0037 0.0006 0.0001 0.0000 0.0414 0.0435 0.0280 0.0111 0.0027 0.0004 0.0000 0.0280 0.0435 0.0414 0.0242 0.0087 0.0019 0.0003 0.0139 0.0318 0.0448 0.0387 0.0205 0.0066 0.0013 0.0050 0.0170 0.0354 0.0453 0.0354 0.0170 0.0050 0.0013 0.0066 0.0205 0.0387 0.0448 0.0318 0.0139 0.0003 0.0019 0.0087 0.0242 0.0414 0.0435 0.0280 0.0000 0.0004 0.0027 0.0111 0.0280 0.0435 0.0414 0.0000 0.0001 0.0006 0.0037 0.0139 0.0318 0.0448 0.0000 0.0000 0.0001 0.0009 0.0050 0.0170 0.0354 0.0000 0.0000 0.0000 0.0002 0.0013 0.0066 0.0205 0.0000 0.0000 0.0000 0.0000 0.0003 0.0019 0.0087 0.0087 0.0019 0.0003 0.0000 0.0000 0.0000 0.0000 0.0205 0.0066 0.0013 0.0002 0.0000 0.0000 0.0000 0.0354 0.0170 0.0050 0.0009 0.0001 0.0000 0.0000 0.0448 0.0318 0.0139 0.0037 0.0006 0.0001 0.0000 0.0414 0.0435 0.0280 0.0111 0.0027 0.0004 0.0000 0.0280 0.0435 0.0414 0.0242 0.0087 0.0019 0.0003 0.0139 0.0318 0.0448 0.0387 0.0205 0.0066 0.0013 0.0050 0.0170 0.0354 0.0453 0.0354 0.0170 0.0050 0.0013 0.0066 0.0205 0.0387 0.0448 0.0318 0.0139 0.0003 0.0019 0.0087 0.0242 0.0414 0.0435 0.0280 0.0000 0.0004 0.0027 0.0111 0.0280 0.0435 0.0414 0.0000 0.0001 0.0006 0.0037 0.0139 0.0318 0.0448 0.0000 0.0000 0.0001 0.0009 0.0050 0.0170 0.0354 0.0000 0.0000 0.0000 0.0002 0.0013 0.0066 0.0205 0.0000 0.0000 0.0000 0.0000 0.0003 0.0019 0.0087 0.0087 0.0019 0.0003 0.0000 0.0000 0.0000 0.0000 0.0205 0.0066 0.0013 0.0002 0.0000 0.0000 0.0000 0.0354 0.0170 0.0050 0.0009 0.0001 0.0000 0.0000 0.0448 0.0318 0.0139 0.0037 0.0006 0.0001 0.0000 0.0414 0.0435 0.0280 0.0111 0.0027 0.0004 0.0000 0.0280 0.0435 0.0414 0.0242 0.0087 0.0019 0.0003 0.0139 0.0318 0.0448 0.0387 0.0205 0.0066 0.0013 0.0050 0.0170 0.0354 0.0453 0.0354 0.0170 0.0050 0.0013 0.0066 0.0205 0.0387 0.0448 0.0318 0.0139 0.0003 0.0019 0.0087 0.0242 0.0414 0.0435 0.0280 0.0000 0.0004 0.0027 0.0111 0.0280 0.0435 0.0414 0.0000 0.0001 0.0006 0.0037 0.0139 0.0318 0.0448 0.0000 0.0000 0.0001 0.0009 0.0050 0.0170 0.0354 0.0000 0.0000 0.0000 0.0002 0.0013 0.0066 0.0205 0.0000 0.0000 0.0000 0.0000 0.0003 0.0019 0.0087 0.0087 0.0019 0.0003 0.0000 0.0000 0.0000 0.0000 0.0205 0.0066 0.0013 0.0002 0.0000 0.0000 0.0000 0.0354 0.0170 0.0050 0.0009 0.0001 0.0000 0.0000 0.0448 0.0318 0.0139 0.0037 0.0006 0.0001 0.0000 0.0414 0.0435 0.0280 0.0111 0.0027 0.0004 0.0000 0.0280 0.0435 0.0414 0.0242 0.0087 0.0019 0.0003 0.0139 0.0318 0.0448 0.0387 0.0205 0.0066 0.0013 0.0050 0.0170 0.0354 0.0453 0.0354 0.0170 0.0050 0.0013 0.0066 0.0205 0.0387 0.0448 0.0318 0.0139 0.0003 0.0019 0.0087 0.0242 0.0414 0.0435 0.0280 0.0000 0.0004 0.0027 0.0111 0.0280 0.0435 0.0414 0.0000 0.0001 0.0006 0.0037 0.0139 0.0318 0.0448 0.0000 0.0000 0.0001 0.0009 0.0050 0.0170 0.0354 0.0000 0.0000 0.0000 0.0002 0.0013 0.0066 0.0205 0.0000 0.0000 0.0000 0.0000 0.0003 0.0019 0.0087 0.0087 0.0019 0.0003 0.0000 0.0000 0.0000 0.0000 0.0205 0.0066 0.0013 0.0002 0.0000 0.0000 0.0000 0.0354 0.0170 0.0050 0.0009 0.0001 0.0000 0.0000 0.0448 0.0318 0.0139 0.0037 0.0006 0.0001 0.0000 0.0414 0.0435 0.0280 0.0111 0.0027 0.0004 0.0000 0.0280 0.0435 0.0414 0.0242 0.0087 0.0019 0.0003 0.0139 0.0318 0.0448 0.0387 0.0205 0.0066 0.0013 0.0050 0.0170 0.0354 0.0453 0.0354 0.0170 0.0050 0.0013 0.0066 0.0205 0.0387 0.0448 0.0318 0.0139 0.0003 0.0019 0.0087 0.0242 0.0414 0.0435 0.0280 0.0000 0.0004 0.0027 0.0111 0.0280 0.0435 0.0414 0.0000 0.0001 0.0006 0.0037 0.0139 0.0318 0.0448 0.0000 0.0000 0.0001 0.0009 0.0050 0.0170 0.0354 0.0000 0.0000 0.0000 0.0002 0.0013 0.0066 0.0205 0.0000 0.0000 0.0000 0.0000 0.0003 0.0019 0.0087 Columns 57 through 63 0.0000 0.0000 0.0000 0 0.2110 0.2176 0.2072 0.0000 0.0000 0.0000 0.0000 0.0911 0.1591 0.2241 0.0000 0.0000 0.0000 0.0000 0.0301 0.0851 0.1772 0.0000 0.0000 0.0000 0.0000 0.0075 0.0332 0.1025 0.0000 0.0000 0.0000 0.0000 0.0014 0.0095 0.0433 0.0000 0.0000 0.0000 0.0000 0.0002 0.0020 0.0134 0.0002 0.0000 0.0000 0.0000 0.0000 0.0003 0.0030 0.0009 0.0001 0.0000 0.0000 0.0000 0.0000 0.0005 0.0037 0.0006 0.0001 0.0000 0.0000 0.0000 0.0001 0.0111 0.0027 0.0004 0.0000 0.0000 0.0000 0.0000 0.0242 0.0087 0.0019 0.0003 0.0000 0.0000 0.0000 0.0387 0.0205 0.0066 0.0015 0.0000 0.0000 0.0000 0.0453 0.0354 0.0170 0.0060 0.0000 0.0000 0.0000 0.0387 0.0448 0.0318 0.0182 0.0000 0.0000 0.0000 0.0242 0.0414 0.0435 0.0422 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0 0.2110 0.2176 0.2072 0.0000 0.0000 0.0000 0.0000 0.0911 0.1591 0.2241 0.0000 0.0000 0.0000 0.0000 0.0301 0.0851 0.1772 0.0000 0.0000 0.0000 0.0000 0.0075 0.0332 0.1025 0.0000 0.0000 0.0000 0.0000 0.0014 0.0095 0.0433 0.0000 0.0000 0.0000 0.0000 0.0002 0.0020 0.0134 0.0002 0.0000 0.0000 0.0000 0.0000 0.0003 0.0030 0.0009 0.0001 0.0000 0.0000 0.0000 0.0000 0.0005 0.0037 0.0006 0.0001 0.0000 0.0000 0.0000 0.0001 0.0111 0.0027 0.0004 0.0000 0.0000 0.0000 0.0000 0.0242 0.0087 0.0019 0.0003 0.0000 0.0000 0.0000 0.0387 0.0205 0.0066 0.0015 0.0000 0.0000 0.0000 0.0453 0.0354 0.0170 0.0060 0.0000 0.0000 0.0000 0.0387 0.0448 0.0318 0.0182 0.0000 0.0000 0.0000 0.0242 0.0414 0.0435 0.0422 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0 0.2110 0.2176 0.2072 0.0000 0.0000 0.0000 0.0000 0.0911 0.1591 0.2241 0.0000 0.0000 0.0000 0.0000 0.0301 0.0851 0.1772 0.0000 0.0000 0.0000 0.0000 0.0075 0.0332 0.1025 0.0000 0.0000 0.0000 0.0000 0.0014 0.0095 0.0433 0.0000 0.0000 0.0000 0.0000 0.0002 0.0020 0.0134 0.0002 0.0000 0.0000 0.0000 0.0000 0.0003 0.0030 0.0009 0.0001 0.0000 0.0000 0.0000 0.0000 0.0005 0.0037 0.0006 0.0001 0.0000 0.0000 0.0000 0.0001 0.0111 0.0027 0.0004 0.0000 0.0000 0.0000 0.0000 0.0242 0.0087 0.0019 0.0003 0.0000 0.0000 0.0000 0.0387 0.0205 0.0066 0.0015 0.0000 0.0000 0.0000 0.0453 0.0354 0.0170 0.0060 0.0000 0.0000 0.0000 0.0387 0.0448 0.0318 0.0182 0.0000 0.0000 0.0000 0.0242 0.0414 0.0435 0.0422 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0 0.2110 0.2176 0.2072 0.0000 0.0000 0.0000 0.0000 0.0911 0.1591 0.2241 0.0000 0.0000 0.0000 0.0000 0.0301 0.0851 0.1772 0.0000 0.0000 0.0000 0.0000 0.0075 0.0332 0.1025 0.0000 0.0000 0.0000 0.0000 0.0014 0.0095 0.0433 0.0000 0.0000 0.0000 0.0000 0.0002 0.0020 0.0134 0.0002 0.0000 0.0000 0.0000 0.0000 0.0003 0.0030 0.0009 0.0001 0.0000 0.0000 0.0000 0.0000 0.0005 0.0037 0.0006 0.0001 0.0000 0.0000 0.0000 0.0001 0.0111 0.0027 0.0004 0.0000 0.0000 0.0000 0.0000 0.0242 0.0087 0.0019 0.0003 0.0000 0.0000 0.0000 0.0387 0.0205 0.0066 0.0015 0.0000 0.0000 0.0000 0.0453 0.0354 0.0170 0.0060 0.0000 0.0000 0.0000 0.0387 0.0448 0.0318 0.0182 0.0000 0.0000 0.0000 0.0242 0.0414 0.0435 0.0422 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0 0.2110 0.2176 0.2072 0.0000 0.0000 0.0000 0.0000 0.0911 0.1591 0.2241 0.0000 0.0000 0.0000 0.0000 0.0301 0.0851 0.1772 0.0000 0.0000 0.0000 0.0000 0.0075 0.0332 0.1025 0.0000 0.0000 0.0000 0.0000 0.0014 0.0095 0.0433 0.0000 0.0000 0.0000 0.0000 0.0002 0.0020 0.0134 0.0002 0.0000 0.0000 0.0000 0.0000 0.0003 0.0030 0.0009 0.0001 0.0000 0.0000 0.0000 0.0000 0.0005 0.0037 0.0006 0.0001 0.0000 0.0000 0.0000 0.0001 0.0111 0.0027 0.0004 0.0000 0.0000 0.0000 0.0000 0.0242 0.0087 0.0019 0.0003 0.0000 0.0000 0.0000 0.0387 0.0205 0.0066 0.0015 0.0000 0.0000 0.0000 0.0453 0.0354 0.0170 0.0060 0.0000 0.0000 0.0000 0.0387 0.0448 0.0318 0.0182 0.0000 0.0000 0.0000 0.0242 0.0414 0.0435 0.0422 0.0000 0.0000 0.0000 Columns 64 through 70 0.1210 0.0433 0.0095 0.0013 0.0001 0.0000 0.0000 0.1935 0.1025 0.0332 0.0066 0.0008 0.0001 0.0000 0.2263 0.1772 0.0851 0.0250 0.0045 0.0005 0.0000 0.1935 0.2241 0.1591 0.0693 0.0185 0.0030 0.0003 0.1210 0.2072 0.2176 0.1401 0.0553 0.0134 0.0020 0.0553 0.1401 0.2176 0.2072 0.1210 0.0433 0.0095 0.0185 0.0693 0.1591 0.2241 0.1935 0.1025 0.0332 0.0045 0.0250 0.0851 0.1772 0.2263 0.1772 0.0851 0.0008 0.0066 0.0332 0.1025 0.1935 0.2241 0.1591 0.0001 0.0013 0.0095 0.0433 0.1210 0.2072 0.2176 0.0000 0.0002 0.0020 0.0134 0.0553 0.1401 0.2176 0.0000 0.0000 0.0003 0.0030 0.0185 0.0693 0.1591 0.0000 0.0000 0.0000 0.0005 0.0045 0.0250 0.0851 0.0000 0.0000 0.0000 0.0001 0.0008 0.0066 0.0332 0.0000 0.0000 0.0000 0.0000 0.0001 0.0013 0.0095 0.1210 0.0433 0.0095 0.0013 0.0001 0.0000 0.0000 0.1935 0.1025 0.0332 0.0066 0.0008 0.0001 0.0000 0.2263 0.1772 0.0851 0.0250 0.0045 0.0005 0.0000 0.1935 0.2241 0.1591 0.0693 0.0185 0.0030 0.0003 0.1210 0.2072 0.2176 0.1401 0.0553 0.0134 0.0020 0.0553 0.1401 0.2176 0.2072 0.1210 0.0433 0.0095 0.0185 0.0693 0.1591 0.2241 0.1935 0.1025 0.0332 0.0045 0.0250 0.0851 0.1772 0.2263 0.1772 0.0851 0.0008 0.0066 0.0332 0.1025 0.1935 0.2241 0.1591 0.0001 0.0013 0.0095 0.0433 0.1210 0.2072 0.2176 0.0000 0.0002 0.0020 0.0134 0.0553 0.1401 0.2176 0.0000 0.0000 0.0003 0.0030 0.0185 0.0693 0.1591 0.0000 0.0000 0.0000 0.0005 0.0045 0.0250 0.0851 0.0000 0.0000 0.0000 0.0001 0.0008 0.0066 0.0332 0.0000 0.0000 0.0000 0.0000 0.0001 0.0013 0.0095 0.1210 0.0433 0.0095 0.0013 0.0001 0.0000 0.0000 0.1935 0.1025 0.0332 0.0066 0.0008 0.0001 0.0000 0.2263 0.1772 0.0851 0.0250 0.0045 0.0005 0.0000 0.1935 0.2241 0.1591 0.0693 0.0185 0.0030 0.0003 0.1210 0.2072 0.2176 0.1401 0.0553 0.0134 0.0020 0.0553 0.1401 0.2176 0.2072 0.1210 0.0433 0.0095 0.0185 0.0693 0.1591 0.2241 0.1935 0.1025 0.0332 0.0045 0.0250 0.0851 0.1772 0.2263 0.1772 0.0851 0.0008 0.0066 0.0332 0.1025 0.1935 0.2241 0.1591 0.0001 0.0013 0.0095 0.0433 0.1210 0.2072 0.2176 0.0000 0.0002 0.0020 0.0134 0.0553 0.1401 0.2176 0.0000 0.0000 0.0003 0.0030 0.0185 0.0693 0.1591 0.0000 0.0000 0.0000 0.0005 0.0045 0.0250 0.0851 0.0000 0.0000 0.0000 0.0001 0.0008 0.0066 0.0332 0.0000 0.0000 0.0000 0.0000 0.0001 0.0013 0.0095 0.1210 0.0433 0.0095 0.0013 0.0001 0.0000 0.0000 0.1935 0.1025 0.0332 0.0066 0.0008 0.0001 0.0000 0.2263 0.1772 0.0851 0.0250 0.0045 0.0005 0.0000 0.1935 0.2241 0.1591 0.0693 0.0185 0.0030 0.0003 0.1210 0.2072 0.2176 0.1401 0.0553 0.0134 0.0020 0.0553 0.1401 0.2176 0.2072 0.1210 0.0433 0.0095 0.0185 0.0693 0.1591 0.2241 0.1935 0.1025 0.0332 0.0045 0.0250 0.0851 0.1772 0.2263 0.1772 0.0851 0.0008 0.0066 0.0332 0.1025 0.1935 0.2241 0.1591 0.0001 0.0013 0.0095 0.0433 0.1210 0.2072 0.2176 0.0000 0.0002 0.0020 0.0134 0.0553 0.1401 0.2176 0.0000 0.0000 0.0003 0.0030 0.0185 0.0693 0.1591 0.0000 0.0000 0.0000 0.0005 0.0045 0.0250 0.0851 0.0000 0.0000 0.0000 0.0001 0.0008 0.0066 0.0332 0.0000 0.0000 0.0000 0.0000 0.0001 0.0013 0.0095 0.1210 0.0433 0.0095 0.0013 0.0001 0.0000 0.0000 0.1935 0.1025 0.0332 0.0066 0.0008 0.0001 0.0000 0.2263 0.1772 0.0851 0.0250 0.0045 0.0005 0.0000 0.1935 0.2241 0.1591 0.0693 0.0185 0.0030 0.0003 0.1210 0.2072 0.2176 0.1401 0.0553 0.0134 0.0020 0.0553 0.1401 0.2176 0.2072 0.1210 0.0433 0.0095 0.0185 0.0693 0.1591 0.2241 0.1935 0.1025 0.0332 0.0045 0.0250 0.0851 0.1772 0.2263 0.1772 0.0851 0.0008 0.0066 0.0332 0.1025 0.1935 0.2241 0.1591 0.0001 0.0013 0.0095 0.0433 0.1210 0.2072 0.2176 0.0000 0.0002 0.0020 0.0134 0.0553 0.1401 0.2176 0.0000 0.0000 0.0003 0.0030 0.0185 0.0693 0.1591 0.0000 0.0000 0.0000 0.0005 0.0045 0.0250 0.0851 0.0000 0.0000 0.0000 0.0001 0.0008 0.0066 0.0332 0.0000 0.0000 0.0000 0.0000 0.0001 0.0013 0.0095 Columns 71 through 75 0.0000 0.0000 0.0000 0.0000 0 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0002 0.0000 0.0000 0.0000 0.0000 0.0013 0.0001 0.0000 0.0000 0.0000 0.0066 0.0008 0.0001 0.0000 0.0000 0.0250 0.0045 0.0005 0.0000 0.0000 0.0693 0.0185 0.0030 0.0003 0.0000 0.1401 0.0553 0.0134 0.0020 0.0002 0.2072 0.1210 0.0433 0.0095 0.0014 0.2241 0.1935 0.1025 0.0332 0.0075 0.1772 0.2263 0.1772 0.0851 0.0301 0.1025 0.1935 0.2241 0.1591 0.0911 0.0433 0.1210 0.2072 0.2176 0.2110 0.0000 0.0000 0.0000 0.0000 0 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0002 0.0000 0.0000 0.0000 0.0000 0.0013 0.0001 0.0000 0.0000 0.0000 0.0066 0.0008 0.0001 0.0000 0.0000 0.0250 0.0045 0.0005 0.0000 0.0000 0.0693 0.0185 0.0030 0.0003 0.0000 0.1401 0.0553 0.0134 0.0020 0.0002 0.2072 0.1210 0.0433 0.0095 0.0014 0.2241 0.1935 0.1025 0.0332 0.0075 0.1772 0.2263 0.1772 0.0851 0.0301 0.1025 0.1935 0.2241 0.1591 0.0911 0.0433 0.1210 0.2072 0.2176 0.2110 0.0000 0.0000 0.0000 0.0000 0 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0002 0.0000 0.0000 0.0000 0.0000 0.0013 0.0001 0.0000 0.0000 0.0000 0.0066 0.0008 0.0001 0.0000 0.0000 0.0250 0.0045 0.0005 0.0000 0.0000 0.0693 0.0185 0.0030 0.0003 0.0000 0.1401 0.0553 0.0134 0.0020 0.0002 0.2072 0.1210 0.0433 0.0095 0.0014 0.2241 0.1935 0.1025 0.0332 0.0075 0.1772 0.2263 0.1772 0.0851 0.0301 0.1025 0.1935 0.2241 0.1591 0.0911 0.0433 0.1210 0.2072 0.2176 0.2110 0.0000 0.0000 0.0000 0.0000 0 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0002 0.0000 0.0000 0.0000 0.0000 0.0013 0.0001 0.0000 0.0000 0.0000 0.0066 0.0008 0.0001 0.0000 0.0000 0.0250 0.0045 0.0005 0.0000 0.0000 0.0693 0.0185 0.0030 0.0003 0.0000 0.1401 0.0553 0.0134 0.0020 0.0002 0.2072 0.1210 0.0433 0.0095 0.0014 0.2241 0.1935 0.1025 0.0332 0.0075 0.1772 0.2263 0.1772 0.0851 0.0301 0.1025 0.1935 0.2241 0.1591 0.0911 0.0433 0.1210 0.2072 0.2176 0.2110 0.0000 0.0000 0.0000 0.0000 0 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0000 0.0002 0.0000 0.0000 0.0000 0.0000 0.0013 0.0001 0.0000 0.0000 0.0000 0.0066 0.0008 0.0001 0.0000 0.0000 0.0250 0.0045 0.0005 0.0000 0.0000 0.0693 0.0185 0.0030 0.0003 0.0000 0.1401 0.0553 0.0134 0.0020 0.0002 0.2072 0.1210 0.0433 0.0095 0.0014 0.2241 0.1935 0.1025 0.0332 0.0075 0.1772 0.2263 0.1772 0.0851 0.0301 0.1025 0.1935 0.2241 0.1591 0.0911 0.0433 0.1210 0.2072 0.2176 0.2110 ar_forbrblk, ar_forbrblk_r 1 9 -19.0000 0.0650 -14.5000 0.0650 -10.0000 0.0650 -7.0000 0.0650 -5.5000 0.0650 -4.0000 0.0650 -2.5000 0.0650 -1.0000 0.0650 0 0.0650 pos = 1 ; key = f_bprime ; val = @(fl_r_inf,ar_for_borr,ar_inf_borr,ar_for_save)(ar_for_borr./(1+fl_r_fbr)+ar_inf_borr./(1+fl_r_inf)+ar_for_save./(1+fl_r_fsv)) pos = 2 ; key = f_coh ; val = @(ar_z,ar_b)(ar_z*fl_w+ar_b) pos = 3 ; key = f_cons_coh_fbis ; val = @(coh,ar_bprime_in_c)(coh+ar_bprime_in_c) pos = 4 ; key = f_cons_coh_save ; val = @(coh,ar_for_save)(coh-ar_for_save./(1+fl_r_fsv)) pos = 5 ; key = f_inc ; val = @(ar_z,fl_r_inf,ar_for_borr,ar_inf_borr,ar_for_save)(ar_z*fl_w+((ar_for_borr./(1+fl_r_fbr))*fl_r_fbr+(ar_inf_borr./(1+fl_r_inf))*fl_r_inf+(ar_for_save./(1+fl_r_fsv))*fl_r_fsv)) pos = 6 ; key = f_util_crra ; val = @(c)(((c).^(1-fl_crra)-1)./(1-fl_crra)) pos = 7 ; key = f_util_log ; val = @(c)log(c) pos = 8 ; key = f_util_standin ; val = @(z,b)f_util_log(f_coh_simple(z,b).*(f_coh_simple(z,b)>0)+fl_c_min.*(f_coh_simple(z,b)<=0))
end
ans = Map with properties: Count: 6 KeyType: char ValueType: any