Risky + Safe Asset (Save + Borr) Interpolated-Percentage (Optimized-Vectorized)
back to Fan's Dynamic Assets Repository Table of Content.
Contents
- FF_IPWKBZ_VF_VECSV solve infinite horizon exo shock + endo asset problem
- Default
- Parse Parameters 1
- Parse Parameters 2
- Initialize Output Matrixes
- Initialize Convergence Conditions
- Pre-calculate u(c)
- Iterate Value Function
- Interpolate (1) reacahble v(coh(k(w,z),b(w,z),z),z) given v(coh, z)
- Solve Second Stage Problem k*(w,z)
- Solve First Stage Problem w*(z) given k*(w,z)
- A. Interpolate FULL to get k*(w_perc, z), b*(k,w) based on k*(w_level, z)
- B. Calculate UPDATE u(c): u(c(coh_level, w_perc)) given k*_interp, b*_interp
- C. Interpolate FULL EV(k*(coh_level, w_perc, z), w - b*|z) based on EV(k*(w_level, z))
- D. Compute FULL U(coh_level, w_perc, z) over all w_perc
- E. Optimize Over Choices: max_{w_perc} U(coh_level, w_perc, z)
- F. Store Results
- Check Tolerance and Continuation
- Process Optimal Choices
- Display Various Containers
- Display 1 support_map
- Display 2 armt_map
- Display 3 param_map
- Display 4 func_map
- Display 5 result_map
function result_map = ff_ipwkbz_vf_vecsv(varargin)
FF_IPWKBZ_VF_VECSV solve infinite horizon exo shock + endo asset problem
This program solves the infinite horizon dynamic savings and risky capital asset problem with some ar1 shock. This is the two step solution with interpolation and with percentage asset grids version of ff_iwkz_vf_vecsv. See that file for more descriptions.
@param param_map container parameter container
@param support_map container support container
@param armt_map container container with states, choices and shocks grids that are inputs for grid based solution algorithm
@param func_map container container with function handles for consumption cash-on-hand etc.
@return result_map container contains policy function matrix, value function matrix, iteration results, and policy function, value function and iteration results tables.
keys included in result_map:
- mt_val matrix states_n by shock_n matrix of converged value function grid
- mt_pol_a matrix states_n by shock_n matrix of converged policy function grid
- ar_val_diff_norm array if bl_post = true it_iter_last by 1 val function difference between iteration
- ar_pol_diff_norm array if bl_post = true it_iter_last by 1 policy function difference between iterations
- mt_pol_perc_change matrix if bl_post = true it_iter_last by shock_n the proportion of grid points at which policy function changed between current and last iteration for each element of shock
@example
@include
Default
- it_param_set = 1: quick test
- it_param_set = 2: benchmark run
- it_param_set = 3: benchmark profile
- it_param_set = 4: press publish button
it_param_set = 4; bl_input_override = true; [param_map, support_map] = ffs_ipwkbz_set_default_param(it_param_set); % parameters can be set inside ffs_ipwkbz_set_default_param or updated here % param_map('it_w_perc_n') = 50; % param_map('it_ak_perc_n') = param_map('it_w_perc_n'); % param_map('it_z_n') = 15; % param_map('fl_coh_interp_grid_gap') = 0.025; % param_map('it_c_interp_grid_gap') = 0.001; % param_map('fl_w_interp_grid_gap') = 0.25; % param_map('it_w_perc_n') = 100; % param_map('it_ak_perc_n') = param_map('it_w_perc_n'); % param_map('it_z_n') = 11; % param_map('fl_coh_interp_grid_gap') = 0.1; % param_map('it_c_interp_grid_gap') = 10^-4; % param_map('fl_w_interp_grid_gap') = 0.1; % get armt and func map [armt_map, func_map] = ffs_ipwkbz_get_funcgrid(param_map, support_map, bl_input_override); % 1 for override default_params = {param_map support_map armt_map func_map};
Parse Parameters 1
% if varargin only has param_map and support_map, params_len = length(varargin); [default_params{1:params_len}] = varargin{:}; param_map = [param_map; default_params{1}]; support_map = [support_map; default_params{2}]; if params_len >= 1 && params_len <= 2 % If override param_map, re-generate armt and func if they are not % provided bl_input_override = true; [armt_map, func_map] = ffs_ipwkbz_get_funcgrid(param_map, support_map, bl_input_override); else % Override all armt_map = [armt_map; default_params{3}]; func_map = [func_map; default_params{4}]; end % append function name st_func_name = 'ff_ipwkbz_vf_vecsv'; support_map('st_profile_name_main') = [st_func_name support_map('st_profile_name_main')]; support_map('st_mat_name_main') = [st_func_name support_map('st_mat_name_main')]; support_map('st_img_name_main') = [st_func_name support_map('st_img_name_main')];
Parse Parameters 2
% armt_map params_group = values(armt_map, {'ar_w_perc', 'ar_w_level', 'ar_z'}); [ar_w_perc, ar_w_level, ar_z] = params_group{:}; params_group = values(armt_map, {'ar_interp_c_grid', 'ar_interp_coh_grid', ... 'ar_a_meshk', 'ar_k_mesha', ... 'mt_interp_coh_grid_mesh_z', 'mt_z_mesh_coh_interp_grid',... 'mt_interp_coh_grid_mesh_w_perc',... 'mt_w_by_interp_coh_interp_grid'}); [ar_interp_c_grid, ar_interp_coh_grid, ar_a_meshk, ar_k_mesha, ... mt_interp_coh_grid_mesh_z, mt_z_mesh_coh_interp_grid, ... mt_interp_coh_grid_mesh_w_perc,... mt_w_by_interp_coh_interp_grid] = params_group{:}; params_group = values(armt_map, {'mt_coh_wkb', 'mt_z_mesh_coh_wkb'}); [mt_coh_wkb, mt_z_mesh_coh_wkb] = params_group{:}; % func_map params_group = values(func_map, {'f_util_log', 'f_util_crra', 'f_cons'}); [f_util_log, f_util_crra, f_cons] = params_group{:}; % param_map params_group = values(param_map, {'it_z_n', 'fl_crra', 'fl_beta', ... 'fl_nan_replace', 'fl_c_min', 'bl_default', 'fl_default_wprime'}); [it_z_n, fl_crra, fl_beta, fl_nan_replace, fl_c_min, bl_default, fl_default_wprime] = params_group{:}; params_group = values(param_map, {'it_maxiter_val', 'fl_tol_val', 'fl_tol_pol', 'it_tol_pol_nochange'}); [it_maxiter_val, fl_tol_val, fl_tol_pol, it_tol_pol_nochange] = params_group{:}; % support_map params_group = values(support_map, {'bl_profile', 'st_profile_path', ... 'st_profile_prefix', 'st_profile_name_main', 'st_profile_suffix',... 'bl_time', 'bl_display_defparam', 'bl_graph_evf', 'bl_display', 'it_display_every', 'bl_post'}); [bl_profile, st_profile_path, ... st_profile_prefix, st_profile_name_main, st_profile_suffix, ... bl_time, bl_display_defparam, bl_graph_evf, bl_display, it_display_every, bl_post] = params_group{:}; params_group = values(support_map, {'it_display_summmat_rowmax', 'it_display_summmat_colmax'}); [it_display_summmat_rowmax, it_display_summmat_colmax] = params_group{:};
Initialize Output Matrixes
mt_val_cur = zeros(length(ar_interp_coh_grid),length(ar_z)); mt_val = mt_val_cur - 1; mt_pol_a = zeros(length(ar_interp_coh_grid),length(ar_z)); mt_pol_a_cur = mt_pol_a - 1; mt_pol_k = zeros(length(ar_interp_coh_grid),length(ar_z)); mt_pol_k_cur = mt_pol_k - 1; mt_pol_idx = zeros(length(ar_interp_coh_grid),length(ar_z)); % We did not need these in ff_oz_vf or ff_oz_vf_vec % see % <https://fanwangecon.github.io/M4Econ/support/speed/partupdate/fs_u_c_partrepeat_main.html % fs_u_c_partrepeat_main> for why store using cells. cl_u_c_store = cell([it_z_n, 1]); cl_c_valid_idx = cell([it_z_n, 1]); cl_w_kstar_interp_z = cell([it_z_n, 1]); for it_z_i = 1:length(ar_z) cl_w_kstar_interp_z{it_z_i} = zeros([length(ar_w_perc), length(ar_interp_coh_grid)]) - 1; end
Initialize Convergence Conditions
bl_vfi_continue = true; it_iter = 0; ar_val_diff_norm = zeros([it_maxiter_val, 1]); ar_pol_diff_norm = zeros([it_maxiter_val, 1]); mt_pol_perc_change = zeros([it_maxiter_val, it_z_n]);
Pre-calculate u(c)
Interpolation, see fs_u_c_partrepeat_main for why interpolate over u(c)
% Evaluate if (fl_crra == 1) ar_interp_u_of_c_grid = f_util_log(ar_interp_c_grid); fl_u_cmin = f_util_log(fl_c_min); else ar_interp_u_of_c_grid = f_util_crra(ar_interp_c_grid); fl_u_cmin = f_util_crra(fl_c_min); end ar_interp_u_of_c_grid(ar_interp_c_grid <= fl_c_min) = fl_u_cmin; % Get Interpolant f_grid_interpolant_spln = griddedInterpolant(ar_interp_c_grid, ar_interp_u_of_c_grid, 'spline', 'nearest');
Iterate Value Function
Loop solution with 4 nested loops
- loop 1: over exogenous states
- loop 2: over endogenous states
- loop 3: over choices
- loop 4: add future utility, integration--loop over future shocks
% Start Profile if (bl_profile) close all; profile off; profile on; end % Start Timer if (bl_time) tic; end % Value Function Iteration while bl_vfi_continue
it_iter = it_iter + 1;
Interpolate (1) reacahble v(coh(k(w,z),b(w,z),z),z) given v(coh, z)
v(coh,z) solved on ar_interp_coh_grid, ar_z grids, see ffs_ipwkbz_get_funcgrid.m. Generate interpolant based on that, Then interpolate for the coh reachable levels given the k(w,z) percentage choice grids in the second stage of the problem
% Generate Interpolant for v(coh,z) f_grid_interpolant_value = griddedInterpolant(... mt_z_mesh_coh_interp_grid', mt_interp_coh_grid_mesh_z', mt_val_cur', 'linear', 'nearest'); % Interpolate for v(coh(k(w,z),b(w,z),z),z) mt_val_wkb_interpolated = f_grid_interpolant_value(mt_z_mesh_coh_wkb, mt_coh_wkb); % ar_val_wkb_interpolated = f_grid_interpolant_value(mt_z_mesh_coh_wkb(:), mt_coh_wkb(:)); % mt_val_wkb_interpolated = reshape(ar_val_wkb_interpolated, size(mt_z_mesh_coh_wkb));
Solve Second Stage Problem k*(w,z)
This is the key difference between this function and ffs_akz_set_functions which solves the two stages jointly Interpolation first, because solution coh grid is not the same as all points reachable by k and b choices given w.
support_map('bl_graph_evf') = false; if (it_iter == (it_maxiter_val + 1)) support_map('bl_graph_evf') = bl_graph_evf; end bl_input_override = true; [mt_ev_condi_z_max, ~, mt_ev_condi_z_max_kp, ~] = ... ff_ipwkbz_evf(mt_val_wkb_interpolated, param_map, support_map, armt_map, bl_input_override);
Solve First Stage Problem w*(z) given k*(w,z)
% loop 1: over exogenous states for it_z_i = 1:length(ar_z)
A. Interpolate FULL to get k*(w_perc, z), b*(k,w) based on k*(w_level, z)
Generate interpolant for (2) k*(ar_w_perc) from k*(ar_w_level,z) There are two w=k'+b' arrays. ar_w_level is the level even grid based on which we solve the 2nd stage problem in ff_ipwkbz_evf.m. Here for each coh level, we have a different vector of w levels, but the same vector of percentage ws. So we need to interpolate to get the optimal k* and b* choices at each percentage level of w.
f_interpolante_w_level_kstar_z = griddedInterpolant(ar_w_level, mt_ev_condi_z_max_kp(:, it_z_i)', 'linear', 'nearest'); % Interpolate (2), shift from w_level to w_perc mt_w_kstar_interp_z = f_interpolante_w_level_kstar_z(mt_w_by_interp_coh_interp_grid); mt_w_astar_interp_z = mt_w_by_interp_coh_interp_grid - mt_w_kstar_interp_z; % changes in w_perc kstar choices mt_w_kstar_diff_idx = (cl_w_kstar_interp_z{it_z_i} ~= mt_w_kstar_interp_z);
B. Calculate UPDATE u(c): u(c(coh_level, w_perc)) given k*_interp, b*_interp
Note that compared to ffs_akz_set_functions the mt_c here is much smaller the same number of columns (states) as in the ffs_akz_set_functions file, but the number of rows equal to ar_w length.
ar_c = f_cons(mt_interp_coh_grid_mesh_w_perc(mt_w_kstar_diff_idx), ... mt_w_astar_interp_z(mt_w_kstar_diff_idx), ... mt_w_kstar_interp_z(mt_w_kstar_diff_idx)); ar_it_c_valid_idx = (ar_c <= fl_c_min); % EVAL current utility: N by N, f_util defined earlier ar_utility_update = f_grid_interpolant_spln(ar_c); % Update Storage if (it_iter == 1) cl_u_c_store{it_z_i} = reshape(ar_utility_update, [length(ar_w_perc), length(ar_interp_coh_grid)]); cl_c_valid_idx{it_z_i} = reshape(ar_it_c_valid_idx, [length(ar_w_perc), length(ar_interp_coh_grid)]); else cl_u_c_store{it_z_i}(mt_w_kstar_diff_idx) = ar_utility_update; cl_c_valid_idx{it_z_i}(mt_w_kstar_diff_idx) = ar_it_c_valid_idx; end cl_w_kstar_interp_z{it_z_i} = mt_w_kstar_interp_z;
C. Interpolate FULL EV(k*(coh_level, w_perc, z), w - b*|z) based on EV(k*(w_level, z))
Generate Interpolant for (3) EV(k*(ar_w_perc),Z)
f_interpolante_ev_condi_z_max_z = griddedInterpolant(ar_w_level, mt_ev_condi_z_max(:, it_z_i)', 'linear', 'nearest'); % Interpolate (3), EVAL add on future utility, N by N + N by N mt_ev_condi_z_max_interp_z = f_interpolante_ev_condi_z_max_z(mt_w_by_interp_coh_interp_grid);
D. Compute FULL U(coh_level, w_perc, z) over all w_perc
mt_utility = cl_u_c_store{it_z_i} + fl_beta*mt_ev_condi_z_max_interp_z; % Index update % using the method below is much faster than index replace % see <https://fanwangecon.github.io/M4Econ/support/speed/index/fs_subscript.html fs_subscript> mt_it_c_valid_idx = cl_c_valid_idx{it_z_i}; % Default or Not Utility Handling if (bl_default) % if default: only today u(cmin), transition out next period, debt wiped out fl_v_default = fl_u_cmin + fl_beta*f_interpolante_ev_condi_z_max_z(fl_default_wprime); mt_utility = mt_utility.*(~mt_it_c_valid_idx) + fl_v_default*(mt_it_c_valid_idx); else % if default is not allowed: v = u(cmin) mt_utility = mt_utility.*(~mt_it_c_valid_idx) + fl_nan_replace*(mt_it_c_valid_idx); end % percentage algorithm does not have invalid (check to make sure % min percent is not 0 in ffs_ipwkbz_get_funcgrid.m) % mt_utility = mt_utility.*(~mt_it_c_valid_idx) + fl_u_neg_c*(mt_it_c_valid_idx);
E. Optimize Over Choices: max_{w_perc} U(coh_level, w_perc, z)
Optimization: remember matlab is column major, rows must be choices, columns must be states COLUMN-MAJOR
[ar_opti_val_z, ar_opti_idx_z] = max(mt_utility); % Generate Linear Opti Index [it_choies_n, it_states_n] = size(mt_utility); ar_add_grid = linspace(0, it_choies_n*(it_states_n-1), it_states_n); ar_opti_linear_idx_z = ar_opti_idx_z + ar_add_grid; ar_opti_aprime_z = mt_w_astar_interp_z(ar_opti_linear_idx_z); ar_opti_kprime_z = mt_w_kstar_interp_z(ar_opti_linear_idx_z); ar_opti_c_z = f_cons(ar_interp_coh_grid, ar_opti_aprime_z, ar_opti_kprime_z); % Handle Default is optimal or not if (bl_default) % if defaulting is optimal choice, at these states, not required % to default, non-default possible, but default could be optimal fl_default_opti_kprime = f_interpolante_w_level_kstar_z(fl_default_wprime); ar_opti_aprime_z(ar_opti_c_z <= fl_c_min) = fl_default_wprime - fl_default_opti_kprime; ar_opti_kprime_z(ar_opti_c_z <= fl_c_min) = fl_default_opti_kprime; else % if default is not allowed, then next period same state as now % this is absorbing state, this is the limiting case, single % state space point, lowest a and lowest shock has this. ar_opti_aprime_z(ar_opti_c_z <= fl_c_min) = min(ar_a_meshk); ar_opti_kprime_z(ar_opti_c_z <= fl_c_min) = min(ar_k_mesha); end
F. Store Results
mt_val(:,it_z_i) = ar_opti_val_z; mt_pol_a(:,it_z_i) = ar_opti_aprime_z; mt_pol_k(:,it_z_i) = ar_opti_kprime_z; if (it_iter == (it_maxiter_val + 1)) mt_pol_idx(:,it_z_i) = ar_opti_linear_idx_z; end
end
Check Tolerance and Continuation
% Difference across iterations ar_val_diff_norm(it_iter) = norm(mt_val - mt_val_cur); ar_pol_diff_norm(it_iter) = norm(mt_pol_a - mt_pol_a_cur) + norm(mt_pol_k - mt_pol_k_cur); ar_pol_a_perc_change = sum((mt_pol_a ~= mt_pol_a_cur))/(length(ar_interp_coh_grid)); ar_pol_k_perc_change = sum((mt_pol_k ~= mt_pol_k_cur))/(length(ar_interp_coh_grid)); mt_pol_perc_change(it_iter, :) = mean([ar_pol_a_perc_change;ar_pol_k_perc_change]); % Update mt_val_cur = mt_val; mt_pol_a_cur = mt_pol_a; mt_pol_k_cur = mt_pol_k; % Print Iteration Results if (bl_display && (rem(it_iter, it_display_every)==0)) fprintf('VAL it_iter:%d, fl_diff:%d, fl_diff_pol:%d\n', ... it_iter, ar_val_diff_norm(it_iter), ar_pol_diff_norm(it_iter)); tb_valpol_iter = array2table([mean(mt_val_cur,1);... mean(mt_pol_a_cur,1); ... mean(mt_pol_k_cur,1); ... mt_val_cur(length(ar_interp_coh_grid),:); ... mt_pol_a_cur(length(ar_interp_coh_grid),:); ... mt_pol_k_cur(length(ar_interp_coh_grid),:)]); tb_valpol_iter.Properties.VariableNames = strcat('z', string((1:size(mt_val_cur,2)))); tb_valpol_iter.Properties.RowNames = {'mval', 'map', 'mak', 'Hval', 'Hap', 'Hak'}; disp('mval = mean(mt_val_cur,1), average value over a') disp('map = mean(mt_pol_a_cur,1), average choice over a') disp('mkp = mean(mt_pol_k_cur,1), average choice over k') disp('Hval = mt_val_cur(it_ameshk_n,:), highest a state val') disp('Hap = mt_pol_a_cur(it_ameshk_n,:), highest a state choice') disp('mak = mt_pol_k_cur(it_ameshk_n,:), highest k state choice') disp(tb_valpol_iter); end % Continuation Conditions: % 1. if value function convergence criteria reached % 2. if policy function variation over iterations is less than % threshold if (it_iter == (it_maxiter_val + 1)) bl_vfi_continue = false; elseif ((it_iter == it_maxiter_val) || ... (ar_val_diff_norm(it_iter) < fl_tol_val) || ... (sum(ar_pol_diff_norm(max(1, it_iter-it_tol_pol_nochange):it_iter)) < fl_tol_pol)) % Fix to max, run again to save results if needed it_iter_last = it_iter; it_iter = it_maxiter_val; end
end % End Timer if (bl_time) toc; end % End Profile if (bl_profile) profile off profile viewer st_file_name = [st_profile_prefix st_profile_name_main st_profile_suffix]; profsave(profile('info'), strcat(st_profile_path, st_file_name)); end
Process Optimal Choices
result_map = containers.Map('KeyType','char', 'ValueType','any'); result_map('mt_val') = mt_val; result_map('mt_pol_idx') = mt_pol_idx; result_map('cl_mt_coh') = {mt_interp_coh_grid_mesh_z, zeros(1)}; result_map('cl_mt_pol_a') = {mt_pol_a, zeros(1)}; result_map('cl_mt_pol_k') = {mt_pol_k, zeros(1)}; mt_pol_c = f_cons(mt_interp_coh_grid_mesh_z, mt_pol_a, mt_pol_k); mt_pol_c(mt_pol_c <= fl_c_min) = fl_c_min; result_map('cl_mt_pol_c') = {mt_pol_c, zeros(1)}; result_map('ar_st_pol_names') = ["cl_mt_coh", "cl_mt_pol_a", "cl_mt_pol_k", "cl_mt_pol_c"]; if (bl_post) bl_input_override = true; result_map('ar_val_diff_norm') = ar_val_diff_norm(1:it_iter_last); result_map('ar_pol_diff_norm') = ar_pol_diff_norm(1:it_iter_last); result_map('mt_pol_perc_change') = mt_pol_perc_change(1:it_iter_last, :); armt_map('mt_coh_wkb_ori') = mt_coh_wkb; armt_map('ar_a_meshk_ori') = ar_a_meshk; armt_map('ar_k_mesha_ori') = ar_k_mesha; % graphing based on coh_wkb, but that does not match optimal choice % matrixes for graphs. armt_map('mt_coh_wkb') = mt_interp_coh_grid_mesh_z; armt_map('it_ameshk_n') = length(ar_interp_coh_grid); armt_map('ar_a_meshk') = mt_interp_coh_grid_mesh_z(:,1); armt_map('ar_k_mesha') = zeros(size(mt_interp_coh_grid_mesh_z(:,1)) + 0); result_map = ff_akz_vf_post(param_map, support_map, armt_map, func_map, result_map, bl_input_override); end
valgap = norm(mt_val - mt_val_cur): value function difference across iterations polgap = norm(mt_pol_a - mt_pol_a_cur): policy function difference across iterations z1 = z1 perc change: (sum((mt_pol_a ~= mt_pol_a_cur))+sum((mt_pol_k ~= mt_pol_k_cur)))/(2*it_ameshk_n):percentage of state space points conditional on shock where the policy function is changing across iterations valgap polgap z1 z2 z3 z4 z5 z6 z7 z8 z9 z10 z11 z12 z13 z14 z15 __________ _______ _________ _________ _________ _________ _________ _________ _________ _________ _________ _________ __________ __________ __________ _________ _________ iter=1 179.7 2150.9 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 iter=2 173.52 2432 1 1 1 1 0.99352 0.98575 0.97992 0.9728 0.96308 0.95207 0.94106 0.92487 0.90933 0.88601 0.86334 iter=3 133.72 884.14 0.94948 0.9307 0.9171 0.97539 0.97863 0.94689 0.93523 0.96373 0.95855 0.93847 0.94819 0.93912 0.92617 0.90933 0.8899 iter=4 108.39 438.85 0.9715 0.9715 0.9715 0.97409 0.97668 0.96632 0.96049 0.96956 0.95984 0.9592 0.95272 0.94365 0.93199 0.92163 0.90479 iter=5 91.273 265.51 0.95466 0.95466 0.95725 0.95855 0.96114 0.96373 0.96697 0.96503 0.96503 0.9592 0.95466 0.94365 0.93394 0.92163 0.91904 iter=6 78.322 187.22 0.93912 0.94041 0.94171 0.9443 0.94689 0.95078 0.95466 0.95725 0.95855 0.95661 0.94624 0.9456 0.93394 0.92228 0.91062 iter=7 67.71 137.42 0.92617 0.92876 0.93653 0.9443 0.9456 0.9456 0.94689 0.94689 0.95078 0.95207 0.94754 0.94106 0.93199 0.91645 0.89119 iter=8 59.065 99.368 0.84197 0.84974 0.87953 0.6386 0.46503 0.40674 0.59585 0.82707 0.88277 0.90091 0.90026 0.89961 0.9294 0.64896 0.68653 iter=9 51.744 86.207 0.31347 0.29275 0.26166 0.49158 0.64443 0.70855 0.78951 0.82513 0.84391 0.81801 0.79145 0.47863 0.39832 0.5 0.71762 iter=10 45.5 87.746 0.64378 0.67228 0.71762 0.73834 0.76943 0.66969 0.33808 0.26878 0.23446 0.25777 0.28692 0.7351 0.66839 0.74611 0.64767 iter=11 40.159 61.276 0.17617 0.16321 0.16321 0.16192 0.15544 0.27526 0.63212 0.71956 0.77915 0.75972 0.40285 0.19106 0.3206 0.33355 0.30505 iter=12 35.509 48.088 0.31477 0.26166 0.24482 0.22733 0.20596 0.18264 0.17034 0.16516 0.16127 0.19171 0.56477 0.69754 0.50065 0.27073 0.38925 iter=13 31.489 72.386 0.42487 0.49741 0.55311 0.5978 0.64637 0.70596 0.25907 0.20596 0.17293 0.15674 0.14313 0.21373 0.38925 0.44495 0.36658 iter=14 28.001 51.591 0.088083 0.091969 0.095855 0.092617 0.1114 0.1101 0.61464 0.68653 0.63407 0.29858 0.13536 0.12306 0.16451 0.2636 0.21244 iter=15 24.925 39.373 0.099741 0.09456 0.097798 0.10751 0.11269 0.1114 0.10751 0.13148 0.22927 0.58484 0.51231 0.31801 0.13407 0.10492 0.26166 iter=16 22.211 36.274 0.1114 0.12435 0.11528 0.1114 0.10233 0.11399 0.11852 0.10427 0.11593 0.12111 0.34326 0.42358 0.34197 0.21762 0.087435 iter=17 19.821 28.891 0.17746 0.15155 0.12565 0.11269 0.12565 0.12241 0.10492 0.08614 0.09715 0.088731 0.10039 0.23964 0.32448 0.24935 0.24676 iter=18 17.727 48.03 0.42228 0.46503 0.51684 0.23575 0.13083 0.11658 0.10363 0.10492 0.087435 0.090026 0.088731 0.088731 0.23446 0.24935 0.19883 iter=19 15.886 43.695 0.049223 0.036269 0.038212 0.38083 0.5285 0.33808 0.14961 0.087435 0.087435 0.07772 0.067358 0.06671 0.076425 0.23381 0.19495 iter=20 14.254 35.548 0.046632 0.042746 0.047927 0.045337 0.036269 0.29404 0.54016 0.27073 0.13018 0.06671 0.057642 0.062176 0.066062 0.11464 0.16516 iter=21 12.799 25.968 0.03886 0.042746 0.037565 0.041451 0.03886 0.044041 0.041451 0.41062 0.28368 0.17293 0.071244 0.053756 0.043394 0.069301 0.1522 iter=22 11.505 25.434 0.044041 0.037565 0.03886 0.032383 0.036269 0.031736 0.029793 0.042746 0.33614 0.20984 0.17358 0.061528 0.064767 0.052461 0.071891 iter=23 10.356 24.849 0.032383 0.036269 0.028497 0.028497 0.027202 0.028497 0.034974 0.033679 0.072539 0.17746 0.16969 0.15933 0.069948 0.038212 0.046632 iter=24 9.3375 18.036 0.031088 0.025907 0.025907 0.01943 0.022021 0.024611 0.022021 0.031088 0.023964 0.23381 0.15155 0.12306 0.11788 0.067358 0.045337 iter=25 8.4312 21.636 0.027202 0.018135 0.020725 0.018135 0.01943 0.01943 0.014249 0.015544 0.01943 0.012953 0.1237 0.13018 0.10622 0.11658 0.032383 iter=26 7.6209 27.219 0.025907 0.022021 0.020725 0.01943 0.020725 0.022021 0.01943 0.0064767 0.012953 0.027202 0.096503 0.10233 0.090026 0.093912 0.10557 iter=27 6.8925 23.228 0.027202 0.01943 0.014249 0.014249 0.015544 0.012953 0.018135 0.014249 0.020725 0.020725 0.12824 0.080959 0.069948 0.084197 0.075777 iter=28 6.2336 23.123 0.022021 0.018135 0.015544 0.01943 0.015544 0.012953 0.024611 0.013601 0.012953 0.013601 0.01101 0.07513 0.054404 0.070596 0.071891 iter=29 5.6348 10.385 0.022021 0.018135 0.011658 0.011658 0.0064767 0.010363 0.007772 0.011658 0.016839 0.013601 0.017487 0.061528 0.072539 0.049223 0.072539 iter=30 5.0887 10.324 0.018782 0.014896 0.0064767 0.007772 0.015544 0.0064767 0.0064767 0.0025907 0.0090674 0.0025907 0.010363 0.04987 0.062824 0.040155 0.050518 iter=31 4.5904 24.941 0.012953 0.014249 0.0090674 0.011658 0.011658 0.010363 0.0090674 0.015544 0.007772 0.021373 0.0064767 0.072539 0.046632 0.044041 0.045337 iter=32 4.1359 7.8234 0.018135 0.014249 0.0090674 0.0051813 0.0025907 0.0051813 0.0025907 0.0051813 0.005829 0.001943 0.0032383 0.015544 0.058938 0.041451 0.038212 iter=33 3.7215 7.4554 0.014249 0.0090674 0.010363 0.007772 0.0051813 0.0051813 0.012953 0.0051813 0.007772 0.005829 0.0090674 0.017487 0.038212 0.031088 0.036269 iter=34 3.3442 7.6155 0.011658 0.0090674 0.0064767 0.003886 0.003886 0.0064767 0 0.0064767 0.013601 0.0032383 0.0071244 0.012306 0.038212 0.025259 0.027202 iter=35 3.0011 6.7574 0.007772 0.0064767 0.007772 0.0051813 0.0025907 0.0012953 0.0090674 0.007772 0.007772 0.0084197 0.010363 0.0064767 0.033031 0.022668 0.016192 iter=36 2.6899 13.307 0.053109 0.007772 0.0051813 0.007772 0.003886 0.0025907 0.0025907 0 0.001943 0.0090674 0.0071244 0.009715 0.061528 0.031736 0.018782 iter=37 2.4081 20.585 0.047927 0.028497 0.0025907 0.007772 0.0012953 0.003886 0.010363 0.0025907 0.0045337 0.0051813 0.0064767 0.0064767 0.017487 0.022021 0.018135 iter=38 2.1533 12.987 0.049223 0.044041 0.0090674 0.0064767 0.0064767 0.0012953 0.0025907 0.003886 0.0012953 0.0051813 0.018782 0.0064767 0.01943 0.013601 0.02785 iter=39 1.9232 10.997 0.062176 0.042746 0.041451 0.010363 0.0064767 0.0012953 0.0012953 0.003886 0.0051813 0.0051813 0.0084197 0.007772 0.001943 0.028497 0.017487 iter=40 1.7157 20.011 0.068653 0.044041 0.033679 0.014249 0.0012953 0.003886 0.0025907 0 0.0064767 0.0012953 0.0051813 0.007772 0.01101 0.023316 0.017487 iter=41 1.529 20.001 0.069948 0.041451 0.034974 0.037565 0.0012953 0.0051813 0 0.0090674 0.0032383 0.001943 0.0090674 0.003886 0.001943 0.018782 0.007772 iter=42 1.3609 10.589 0.069948 0.034974 0.036269 0.027202 0 0.0012953 0.003886 0.0012953 0.0064767 0.0071244 0.0090674 0.0064767 0.007772 0.04728 0.01943 iter=43 1.2104 10.608 0.079016 0.032383 0.028497 0.031088 0.022021 0.0064767 0.0012953 0.0012953 0.0051813 0.007772 0.00064767 0.0025907 0.0071244 0.03886 0.0090674 iter=44 1.0751 25.409 0.0012953 0.025907 0.024611 0.023316 0.023316 0 0.0012953 0.003886 0 0.0032383 0.0012953 0.001943 0.0071244 0.01101 0.007772 iter=45 0.95435 7.4954 0.0051813 0.023316 0.022021 0.016839 0.01943 0.003886 0.0012953 0.0051813 0.0051813 0.0090674 0.0051813 0.0071244 0.003886 0.014249 0.016192 iter=46 0.8469 7.5415 0.0025907 0.018135 0.014249 0.018135 0.018135 0.018135 0 0.003886 0.0051813 0.003886 0.0032383 0.0032383 0.00064767 0.013601 0.014249 iter=47 0.75126 6.167 0 0.015544 0.012953 0.014249 0.014249 0.018135 0.0012953 0.0025907 0 0 0.003886 0.0032383 0.0012953 0.014249 0.005829 iter=48 0.66659 5.9375 0.0012953 0.018135 0.011658 0.010363 0.012953 0.011658 0.0012953 0 0 0.0025907 0.0032383 0 0 0.0051813 0.012306 iter=49 0.5918 5.3293 0.0025907 0.014249 0.007772 0.011658 0.0090674 0.012953 0.0064767 0 0.0051813 0.0025907 0.0051813 0 0.005829 0.007772 0.003886 iter=50 0.52564 6.419 0 0.010363 0.007772 0.007772 0.015544 0.0090674 0 0 0.0051813 0 0.003886 0.0090674 0 0.007772 0.0064767 iter=84 0.020098 1.2944 0 0 0.0025907 0 0 0 0 0 0 0 0 0 0 0 0 iter=85 0.018741 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=86 0.017491 1.5074 0 0 0 0 0 0 0 0.0012953 0 0 0 0 0 0 0 iter=87 0.016339 1.4235 0 0 0 0.0012953 0 0 0.0012953 0 0 0 0 0 0 0 0 iter=88 0.015273 0.12289 0 0 0 0 0 0 0 0 0 0 0 0.00064767 0 0 0 iter=89 0.014287 0.64329 0 0 0 0 0 0 0 0 0 0 0 0 0.0012953 0 0 iter=90 0.013372 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=91 0.012522 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=92 0.011732 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=93 0.010997 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=94 0.010311 1.3034 0 0 0 0 0.0012953 0 0 0 0 0 0 0 0 0.0012953 0 iter=95 0.0096716 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=96 0.0090746 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=97 0.0085168 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=98 0.0079952 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=99 0.0075072 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=100 0.0070503 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=101 0.0066224 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=102 0.0062214 1.3456 0 0 0 0 0 0.0012953 0 0 0 0 0 0 0 0 0 iter=103 0.0058455 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=104 0.0054929 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=105 0.0051621 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=106 0.0048517 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=107 0.0045603 0.27236 0 0 0 0 0 0 0 0 0 0 0.0025907 0 0 0 0 iter=108 0.0042868 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=109 0.0040298 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=110 0.0037885 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=111 0.0035618 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=112 0.0033488 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=113 0.0031486 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=114 0.0029604 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=115 0.0027836 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=116 0.0026174 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=117 0.0024611 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=118 0.0023142 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=119 0.0021761 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=120 0.0020462 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=121 0.0019241 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=122 0.0018093 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=123 0.0017014 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=124 0.0015999 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=125 0.0015044 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=126 0.0014147 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=127 0.0013303 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=128 0.0012509 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=129 0.0011763 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=130 0.0011061 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=131 0.0010401 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=132 0.000978 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=133 0.00091964 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 tb_val: V(a,z) value at each state space point z1_0_34741 z2_0_40076 z3_0_4623 z4_0_5333 z5_0_61519 z6_0_70966 z10_1_2567 z11_1_4496 z12_1_6723 z13_1_9291 z14_2_2253 z15_2_567 __________ __________ _________ _________ __________ __________ __________ __________ __________ __________ __________ _________ coh1:k=-20,b=0 -9.9353 -9.6945 -9.4082 -9.09 -8.737 -8.3542 -6.5424 -6.0344 -5.5129 -4.9849 -4.4625 -3.9715 coh2:k=-19.8996,b=0 -9.9353 -9.6945 -9.4082 -9.09 -8.737 -8.3542 -6.5424 -6.0344 -5.5129 -4.9849 -4.4625 -3.9715 coh3:k=-19.7993,b=0 -9.9353 -9.6945 -9.4082 -9.09 -8.737 -8.3542 -6.5424 -6.0344 -5.5129 -4.9849 -4.4625 -3.9715 coh4:k=-19.6989,b=0 -9.9353 -9.6945 -9.4082 -9.09 -8.737 -8.3542 -6.5424 -6.0344 -5.5129 -4.9849 -4.4625 -3.9715 coh5:k=-19.5986,b=0 -9.9353 -9.6945 -9.4082 -9.09 -8.737 -8.3542 -6.5424 -6.0344 -5.5129 -4.9849 -4.4625 -3.9715 coh6:k=-19.4982,b=0 -9.7758 -9.5706 -9.3344 -9.0745 -8.737 -8.3542 -6.5424 -6.0344 -5.5129 -4.9849 -4.4625 -3.9715 coh7:k=-19.3978,b=0 -9.5298 -9.3246 -9.0884 -8.8285 -8.5486 -8.2503 -6.5424 -6.0344 -5.5129 -4.9849 -4.4625 -3.9715 coh8:k=-19.2975,b=0 -9.3386 -9.1334 -8.8972 -8.6373 -8.3574 -8.0591 -6.5424 -6.0344 -5.5129 -4.9849 -4.4625 -3.9715 coh9:k=-19.1971,b=0 -9.1845 -8.9793 -8.7431 -8.4832 -8.2033 -7.905 -6.5424 -6.0344 -5.5129 -4.9849 -4.4625 -3.9715 coh10:k=-19.0968,b=0 -9.0569 -8.8517 -8.6154 -8.3556 -8.0757 -7.7774 -6.4219 -6.0344 -5.5129 -4.9849 -4.4625 -3.9715 coh11:k=-18.9964,b=0 -8.9489 -8.7437 -8.5074 -8.2476 -7.9677 -7.6694 -6.3139 -5.9419 -5.5129 -4.9849 -4.4625 -3.9715 coh12:k=-18.896,b=0 -8.856 -8.6508 -8.4145 -8.1547 -7.8748 -7.5765 -6.221 -5.849 -5.4685 -4.9849 -4.4625 -3.9715 coh13:k=-18.7957,b=0 -8.7749 -8.5697 -8.3335 -8.0736 -7.7937 -7.4954 -6.14 -5.768 -5.3875 -4.9849 -4.4625 -3.9715 coh14:k=-18.6953,b=0 -8.7034 -8.4982 -8.262 -8.0021 -7.7222 -7.4239 -6.0685 -5.6965 -5.316 -4.9319 -4.4625 -3.9715 coh15:k=-18.595,b=0 -8.6398 -8.4345 -8.1983 -7.9384 -7.6585 -7.3602 -6.0048 -5.6328 -5.2523 -4.8682 -4.4625 -3.9715 coh16:k=-18.4946,b=0 -8.5825 -8.3773 -8.1411 -7.8812 -7.6013 -7.303 -5.9476 -5.5756 -5.1951 -4.811 -4.4338 -3.9715 coh17:k=-18.3942,b=0 -8.5308 -8.3256 -8.0893 -7.8295 -7.5496 -7.2513 -5.8958 -5.5238 -5.1433 -4.7593 -4.382 -3.9715 coh18:k=-18.2939,b=0 -8.4837 -8.2785 -8.0422 -7.7823 -7.5024 -7.2041 -5.8487 -5.4767 -5.0962 -4.7121 -4.3349 -3.9715 coh19:k=-18.1935,b=0 -8.4405 -8.2353 -7.9991 -7.7392 -7.4593 -7.161 -5.8056 -5.4336 -5.053 -4.669 -4.2918 -3.9415 coh20:k=-18.0932,b=0 -8.4008 -8.1956 -7.9594 -7.6995 -7.4196 -7.1213 -5.7659 -5.3939 -5.0134 -4.6293 -4.2521 -3.9018 coh21:k=-17.9928,b=0 -8.3642 -8.159 -7.9227 -7.6628 -7.3829 -7.0846 -5.7292 -5.3572 -4.9767 -4.5926 -4.2154 -3.8651 coh22:k=-17.8924,b=0 -8.3301 -8.1249 -7.8887 -7.6288 -7.3489 -7.0506 -5.6952 -5.3232 -4.9427 -4.5586 -4.1814 -3.8311 coh23:k=-17.7921,b=0 -8.2985 -8.0933 -7.857 -7.5971 -7.3172 -7.019 -5.6635 -5.2915 -4.911 -4.5269 -4.1497 -3.7994 coh24:k=-17.6917,b=0 -8.2689 -8.0637 -7.8274 -7.5676 -7.2877 -6.9894 -5.6339 -5.2619 -4.8814 -4.4973 -4.1201 -3.7698 coh25:k=-17.5914,b=0 -8.2412 -8.036 -7.7997 -7.5398 -7.2599 -6.9616 -5.6062 -5.2342 -4.8537 -4.4696 -4.0924 -3.7421 coh26:k=-17.491,b=0 -8.2151 -8.0099 -7.7737 -7.5138 -7.2339 -6.9356 -5.5802 -5.2082 -4.8277 -4.4436 -4.0664 -3.7161 coh27:k=-17.3906,b=0 -8.1906 -7.9854 -7.7492 -7.4893 -7.2094 -6.9111 -5.5557 -5.1837 -4.8031 -4.4191 -4.0419 -3.6915 coh28:k=-17.2903,b=0 -8.1675 -7.9623 -7.726 -7.4661 -7.1862 -6.8879 -5.5325 -5.1605 -4.78 -4.3959 -4.0187 -3.6684 coh29:k=-17.1899,b=0 -8.1456 -7.9404 -7.7041 -7.4442 -7.1644 -6.8661 -5.5106 -5.1386 -4.7581 -4.374 -3.9968 -3.6465 coh30:k=-17.0896,b=0 -8.1248 -7.9196 -7.6834 -7.4235 -7.1436 -6.8453 -5.4899 -5.1179 -4.7373 -4.3533 -3.9761 -3.6257 coh31:k=-16.9892,b=0 -8.0904 -7.8824 -7.6414 -7.3772 -7.091 -6.7893 -5.4504 -5.0926 -4.7176 -4.3336 -3.9564 -3.606 coh32:k=-16.8888,b=0 -7.9929 -7.7867 -7.5494 -7.2898 -7.0092 -6.7089 -5.3868 -5.0341 -4.6751 -4.3148 -3.9376 -3.5873 coh33:k=-16.7885,b=0 -7.9014 -7.6974 -7.4643 -7.2072 -6.9317 -6.6365 -5.3283 -4.9775 -4.6226 -4.2659 -3.9165 -3.5694 coh34:k=-16.6881,b=0 -7.8173 -7.6164 -7.3846 -7.1303 -6.858 -6.5672 -5.2723 -4.9255 -4.5726 -4.2185 -3.8725 -3.5512 coh35:k=-16.5878,b=0 -7.738 -7.5399 -7.3104 -7.0598 -6.7891 -6.5022 -5.2198 -4.875 -4.5255 -4.1741 -3.8296 -3.5111 coh36:k=-16.4874,b=0 -7.6644 -7.4666 -7.24 -6.9927 -6.7249 -6.4406 -5.1699 -4.8281 -4.4802 -4.1312 -3.7897 -3.4727 coh37:k=-16.387,b=0 -7.5947 -7.3987 -7.1747 -6.9279 -6.6636 -6.3823 -5.1216 -4.7826 -4.4375 -4.0905 -3.7509 -3.4359 coh38:k=-16.2867,b=0 -7.529 -7.3351 -7.1133 -6.8684 -6.6068 -6.3268 -5.0763 -4.7396 -4.3963 -4.0512 -3.7137 -3.401 coh39:k=-16.1863,b=0 -7.4665 -7.2744 -7.0539 -6.8116 -6.5522 -6.2751 -5.0327 -4.6985 -4.3573 -4.0137 -3.6774 -3.3527 coh40:k=-16.086,b=0 -7.4088 -7.2176 -6.9977 -6.7577 -6.4996 -6.2252 -4.9915 -4.6586 -4.3194 -3.9773 -3.6415 -3.2925 coh41:k=-15.9856,b=0 -7.3538 -7.1633 -6.9452 -6.7072 -6.4504 -6.1785 -4.9519 -4.621 -4.2831 -3.9424 -3.5966 -3.2268 coh42:k=-15.8852,b=0 -7.3006 -7.1111 -6.8947 -6.6583 -6.4033 -6.1332 -4.9141 -4.5844 -4.2477 -3.9069 -3.5396 -3.1616 coh43:k=-15.7849,b=0 -7.2505 -7.0623 -6.8473 -6.6113 -6.3583 -6.0894 -4.8778 -4.5496 -4.2137 -3.8653 -3.4815 -3.0974 coh44:k=-15.6845,b=0 -7.2026 -7.0156 -6.802 -6.567 -6.3157 -6.0483 -4.8427 -4.5157 -4.1795 -3.813 -3.4245 -3.0325 coh45:k=-15.5842,b=0 -7.1567 -6.9708 -6.7577 -6.5241 -6.2745 -6.0085 -4.8089 -4.4824 -4.1385 -3.7599 -3.3665 -2.9665 coh46:k=-15.4838,b=0 -7.1137 -6.9287 -6.716 -6.4838 -6.2355 -5.9706 -4.776 -4.4497 -4.0882 -3.7066 -3.3074 -2.8989 coh47:k=-15.3834,b=0 -7.0721 -6.8876 -6.6758 -6.4448 -6.1975 -5.9341 -4.744 -4.4054 -4.036 -3.6515 -3.2473 -2.832 coh48:k=-15.2831,b=0 -7.0326 -6.848 -6.6373 -6.4075 -6.1608 -5.899 -4.7107 -4.353 -3.9833 -3.5974 -3.1882 -2.7658 coh49:k=-15.1827,b=0 -6.9942 -6.8104 -6.6006 -6.3719 -6.126 -5.8655 -4.659 -4.3013 -3.9317 -3.5442 -3.1299 -2.6992 coh50:k=-15.0824,b=0 -6.9568 -6.7739 -6.565 -6.3371 -6.092 -5.8328 -4.6063 -4.2502 -3.8811 -3.4912 -3.0705 -2.6316 coh723:k=52.3596,b=0 15.043 15.087 15.139 15.196 15.258 15.327 15.674 15.782 15.899 16.024 16.154 16.283 coh724:k=52.46,b=0 15.052 15.096 15.148 15.205 15.267 15.336 15.683 15.79 15.907 16.032 16.162 16.29 coh725:k=52.5603,b=0 15.062 15.106 15.157 15.214 15.276 15.345 15.691 15.798 15.915 16.039 16.169 16.297 coh726:k=52.6607,b=0 15.071 15.115 15.167 15.223 15.285 15.353 15.699 15.806 15.923 16.047 16.177 16.305 coh727:k=52.7611,b=0 15.08 15.124 15.176 15.232 15.294 15.362 15.707 15.815 15.93 16.055 16.184 16.312 coh728:k=52.8614,b=0 15.09 15.134 15.185 15.241 15.303 15.371 15.715 15.823 15.938 16.062 16.192 16.319 coh729:k=52.9618,b=0 15.099 15.143 15.194 15.251 15.312 15.38 15.724 15.831 15.946 16.07 16.199 16.327 coh730:k=53.0621,b=0 15.108 15.152 15.203 15.26 15.321 15.389 15.732 15.839 15.954 16.078 16.207 16.334 coh731:k=53.1625,b=0 15.118 15.162 15.212 15.269 15.33 15.398 15.74 15.847 15.962 16.085 16.214 16.341 coh732:k=53.2629,b=0 15.127 15.171 15.222 15.278 15.339 15.406 15.748 15.855 15.97 16.093 16.222 16.348 coh733:k=53.3632,b=0 15.136 15.18 15.231 15.287 15.348 15.415 15.756 15.863 15.977 16.101 16.229 16.355 coh734:k=53.4636,b=0 15.146 15.189 15.24 15.296 15.357 15.424 15.764 15.87 15.985 16.108 16.236 16.363 coh735:k=53.5639,b=0 15.155 15.198 15.249 15.305 15.366 15.433 15.772 15.878 15.993 16.116 16.244 16.37 coh736:k=53.6643,b=0 15.164 15.207 15.258 15.314 15.375 15.441 15.781 15.886 16.001 16.123 16.251 16.377 coh737:k=53.7647,b=0 15.173 15.217 15.267 15.323 15.384 15.45 15.789 15.894 16.008 16.131 16.259 16.384 coh738:k=53.865,b=0 15.183 15.226 15.276 15.332 15.392 15.459 15.797 15.902 16.016 16.138 16.266 16.391 coh739:k=53.9654,b=0 15.192 15.235 15.285 15.341 15.401 15.468 15.805 15.91 16.024 16.146 16.273 16.399 coh740:k=54.0657,b=0 15.201 15.244 15.294 15.349 15.41 15.476 15.813 15.918 16.032 16.154 16.281 16.406 coh741:k=54.1661,b=0 15.21 15.253 15.303 15.358 15.419 15.485 15.821 15.926 16.039 16.161 16.288 16.413 coh742:k=54.2665,b=0 15.219 15.262 15.312 15.367 15.428 15.494 15.829 15.934 16.047 16.169 16.295 16.42 coh743:k=54.3668,b=0 15.228 15.271 15.321 15.376 15.436 15.502 15.837 15.941 16.055 16.176 16.303 16.426 coh744:k=54.4672,b=0 15.238 15.28 15.33 15.385 15.445 15.511 15.845 15.949 16.062 16.184 16.31 16.433 coh745:k=54.5675,b=0 15.247 15.289 15.339 15.394 15.454 15.519 15.853 15.957 16.07 16.191 16.317 16.44 coh746:k=54.6679,b=0 15.247 15.29 15.339 15.394 15.455 15.523 15.861 15.965 16.078 16.198 16.324 16.447 coh747:k=54.7683,b=0 15.252 15.295 15.346 15.402 15.464 15.532 15.869 15.973 16.085 16.206 16.331 16.454 coh748:k=54.8686,b=0 15.261 15.304 15.355 15.411 15.473 15.54 15.877 15.98 16.093 16.213 16.338 16.461 coh749:k=54.969,b=0 15.27 15.313 15.364 15.42 15.481 15.549 15.885 15.988 16.1 16.221 16.346 16.468 coh750:k=55.0693,b=0 15.279 15.322 15.373 15.429 15.49 15.557 15.892 15.996 16.108 16.228 16.353 16.475 coh751:k=55.1697,b=0 15.288 15.331 15.382 15.437 15.499 15.565 15.9 16.004 16.116 16.235 16.36 16.482 coh752:k=55.2701,b=0 15.296 15.34 15.39 15.446 15.507 15.574 15.908 16.011 16.123 16.243 16.367 16.488 coh753:k=55.3704,b=0 15.305 15.349 15.399 15.455 15.516 15.582 15.916 16.019 16.131 16.25 16.374 16.494 coh754:k=55.4708,b=0 15.314 15.358 15.408 15.463 15.524 15.591 15.924 16.027 16.138 16.257 16.381 16.5 coh755:k=55.5711,b=0 15.323 15.366 15.417 15.472 15.533 15.599 15.932 16.035 16.146 16.265 16.387 16.506 coh756:k=55.6715,b=0 15.332 15.375 15.425 15.481 15.541 15.608 15.94 16.042 16.153 16.272 16.394 16.512 coh757:k=55.7719,b=0 15.341 15.384 15.434 15.489 15.55 15.616 15.947 16.05 16.161 16.279 16.401 16.519 coh758:k=55.8722,b=0 15.35 15.393 15.443 15.498 15.558 15.624 15.955 16.058 16.168 16.286 16.408 16.526 coh759:k=55.9726,b=0 15.359 15.402 15.452 15.507 15.567 15.633 15.963 16.065 16.176 16.293 16.415 16.533 coh760:k=56.0729,b=0 15.368 15.41 15.46 15.515 15.575 15.641 15.971 16.073 16.183 16.301 16.422 16.54 coh761:k=56.1733,b=0 15.376 15.419 15.469 15.524 15.584 15.65 15.979 16.08 16.19 16.308 16.429 16.546 coh762:k=56.2737,b=0 15.381 15.424 15.473 15.528 15.588 15.654 15.983 16.084 16.194 16.312 16.433 16.55 coh763:k=56.374,b=0 15.382 15.424 15.474 15.529 15.589 15.654 15.983 16.085 16.195 16.312 16.433 16.55 coh764:k=56.4744,b=0 15.382 15.425 15.474 15.529 15.589 15.655 15.984 16.085 16.195 16.313 16.434 16.551 coh765:k=56.5747,b=0 15.383 15.425 15.475 15.53 15.59 15.655 15.984 16.086 16.196 16.313 16.434 16.555 coh766:k=56.6751,b=0 15.383 15.426 15.475 15.53 15.59 15.656 15.985 16.086 16.196 16.314 16.439 16.562 coh767:k=56.7755,b=0 15.384 15.426 15.476 15.531 15.591 15.656 15.986 16.089 16.201 16.321 16.446 16.569 coh768:k=56.8758,b=0 15.384 15.427 15.476 15.531 15.592 15.659 15.993 16.097 16.209 16.329 16.453 16.575 coh769:k=56.9762,b=0 15.389 15.433 15.483 15.539 15.6 15.667 16.001 16.104 16.216 16.336 16.46 16.581 coh770:k=57.0765,b=0 15.398 15.441 15.492 15.547 15.608 15.675 16.009 16.112 16.223 16.343 16.467 16.587 coh771:k=57.1769,b=0 15.407 15.45 15.5 15.556 15.617 15.683 16.016 16.119 16.231 16.35 16.473 16.592 coh772:k=57.2773,b=0 15.415 15.458 15.509 15.564 15.625 15.691 16.024 16.127 16.238 16.357 16.479 16.598 tb_pol_a: optimal safe savings choice for each state space point z1_0_34741 z2_0_40076 z3_0_4623 z4_0_5333 z5_0_61519 z6_0_70966 z10_1_2567 z11_1_4496 z12_1_6723 z13_1_9291 z14_2_2253 z15_2_567 __________ __________ _________ _________ __________ __________ __________ __________ __________ __________ __________ _________ coh1:k=-20,b=0 -0.81633 -0.81633 -1.2245 -1.6327 -1.6327 -2.0408 -4.0816 -4.898 -5.7143 -6.5306 -7.7551 -9.3878 coh2:k=-19.8996,b=0 -0.81633 -0.81633 -1.2245 -1.6327 -1.6327 -2.0408 -4.0816 -4.898 -5.7143 -6.5306 -7.7551 -9.3878 coh3:k=-19.7993,b=0 -0.81633 -0.81633 -1.2245 -1.6327 -1.6327 -2.0408 -4.0816 -4.898 -5.7143 -6.5306 -7.7551 -9.3878 coh4:k=-19.6989,b=0 -0.81633 -0.81633 -1.2245 -1.6327 -1.6327 -2.0408 -4.0816 -4.898 -5.7143 -6.5306 -7.7551 -9.3878 coh5:k=-19.5986,b=0 -0.81633 -0.81633 -1.2245 -1.6327 -1.6327 -2.0408 -4.0816 -4.898 -5.7143 -6.5306 -7.7551 -9.3878 coh6:k=-19.4982,b=0 -20 -20 -20 -20 -1.6327 -2.0408 -4.0816 -4.898 -5.7143 -6.5306 -7.7551 -9.3878 coh7:k=-19.3978,b=0 -20 -20 -20 -20 -20 -20 -4.0816 -4.898 -5.7143 -6.5306 -7.7551 -9.3878 coh8:k=-19.2975,b=0 -20 -20 -20 -20 -20 -20 -4.0816 -4.898 -5.7143 -6.5306 -7.7551 -9.3878 coh9:k=-19.1971,b=0 -20 -20 -20 -20 -20 -20 -4.0816 -4.898 -5.7143 -6.5306 -7.7551 -9.3878 coh10:k=-19.0968,b=0 -20 -20 -20 -20 -20 -20 -20 -4.898 -5.7143 -6.5306 -7.7551 -9.3878 coh11:k=-18.9964,b=0 -20 -20 -20 -20 -20 -20 -20 -20 -5.7143 -6.5306 -7.7551 -9.3878 coh12:k=-18.896,b=0 -20 -20 -20 -20 -20 -20 -20 -20 -20 -6.5306 -7.7551 -9.3878 coh13:k=-18.7957,b=0 -20 -20 -20 -20 -20 -20 -20 -20 -20 -6.5306 -7.7551 -9.3878 coh14:k=-18.6953,b=0 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 -7.7551 -9.3878 coh15:k=-18.595,b=0 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 -7.7551 -9.3878 coh16:k=-18.4946,b=0 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 -9.3878 coh17:k=-18.3942,b=0 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 -9.3878 coh18:k=-18.2939,b=0 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 -9.3878 coh19:k=-18.1935,b=0 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 coh20:k=-18.0932,b=0 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 coh21:k=-17.9928,b=0 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 coh22:k=-17.8924,b=0 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 coh23:k=-17.7921,b=0 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 coh24:k=-17.6917,b=0 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 coh25:k=-17.5914,b=0 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 coh26:k=-17.491,b=0 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 coh27:k=-17.3906,b=0 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 coh28:k=-17.2903,b=0 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 coh29:k=-17.1899,b=0 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 coh30:k=-17.0896,b=0 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 coh31:k=-16.9892,b=0 -18.791 -18.956 -19.173 -19.456 -19.689 -20 -20 -20 -20 -20 -20 -20 coh32:k=-16.8888,b=0 -18.722 -18.952 -19.118 -19.386 -19.627 -20 -20 -20 -20 -20 -20 -20 coh33:k=-16.7885,b=0 -18.719 -18.891 -19.062 -19.325 -19.563 -19.926 -20 -20 -20 -20 -20 -20 coh34:k=-16.6881,b=0 -18.65 -18.827 -19.064 -19.277 -19.567 -19.857 -20 -20 -20 -20 -20 -20 coh35:k=-16.5878,b=0 -18.59 -18.762 -18.983 -19.204 -19.502 -19.865 -20 -20 -20 -20 -20 -20 coh36:k=-16.4874,b=0 -18.549 -18.768 -18.904 -19.214 -19.453 -19.81 -20 -20 -20 -20 -20 -20 coh37:k=-16.387,b=0 -18.554 -18.703 -18.843 -19.14 -19.41 -19.767 -20 -20 -20 -20 -20 -20 coh38:k=-16.2867,b=0 -18.493 -18.637 -18.855 -19.087 -19.421 -19.735 -20 -20 -20 -20 -20 -20 coh39:k=-16.1863,b=0 -18.421 -18.569 -18.795 -19.033 -19.377 -19.676 -20 -20 -20 -20 -20 -20 coh40:k=-16.086,b=0 -18.347 -18.587 -18.733 -18.978 -19.328 -19.617 -20 -20 -20 -20 -20 -20 coh41:k=-15.9856,b=0 -18.29 -18.519 -18.696 -18.921 -19.253 -19.637 -20 -20 -20 -20 -20 -20 coh42:k=-15.8852,b=0 -18.303 -18.451 -18.657 -18.942 -19.19 -19.594 -20 -20 -20 -20 -20 -20 coh43:k=-15.7849,b=0 -18.262 -18.381 -18.592 -18.886 -19.141 -19.556 -20 -20 -20 -20 -20 -20 coh44:k=-15.6845,b=0 -18.201 -18.328 -18.525 -18.828 -19.089 -19.487 -20 -20 -20 -20 -20 -20 coh45:k=-15.5842,b=0 -18.126 -18.29 -18.557 -18.77 -19.037 -19.425 -20 -20 -20 -20 -20 -20 coh46:k=-15.4838,b=0 -18.081 -18.218 -18.491 -18.709 -19.066 -19.382 -20 -20 -20 -20 -20 -20 coh47:k=-15.3834,b=0 -18.028 -18.257 -18.425 -18.648 -19.014 -19.32 -20 -20 -20 -20 -20 -20 coh48:k=-15.2831,b=0 -17.949 -18.186 -18.357 -18.585 -18.961 -19.243 -20 -20 -20 -20 -20 -20 coh49:k=-15.1827,b=0 -17.996 -18.138 -18.313 -18.52 -18.907 -19.188 -20 -20 -20 -20 -20 -20 coh50:k=-15.0824,b=0 -17.921 -18.098 -18.276 -18.565 -18.852 -19.139 -20 -20 -20 -20 -20 -20 coh723:k=52.3596,b=0 46.543 45.157 45.157 45.157 43.77 43.77 36.959 35.603 32.891 31.535 27.466 24.754 coh724:k=52.46,b=0 46.635 45.247 45.247 45.247 43.859 43.859 37.038 35.68 32.964 31.606 27.532 24.816 coh725:k=52.5603,b=0 46.728 45.338 45.338 45.338 43.947 43.947 37.117 35.758 33.038 31.678 27.598 24.878 coh726:k=52.6607,b=0 46.82 45.428 45.428 45.428 44.036 44.036 37.196 35.835 33.111 31.749 27.664 24.94 coh727:k=52.7611,b=0 46.912 45.518 45.518 45.518 44.124 44.124 37.275 35.912 33.184 31.821 27.73 25.002 coh728:k=52.8614,b=0 47.005 45.609 45.609 45.609 44.213 44.213 37.354 35.989 33.258 31.892 28.101 25.064 coh729:k=52.9618,b=0 47.097 45.699 45.699 45.699 44.301 44.301 37.433 36.066 33.331 31.964 29.229 25.126 coh730:k=53.0621,b=0 47.189 45.789 45.789 45.789 44.39 44.39 37.512 36.143 33.404 32.035 29.296 25.188 coh731:k=53.1625,b=0 47.281 45.88 45.88 45.88 44.478 44.478 37.591 36.22 33.478 32.107 29.364 25.25 coh732:k=53.2629,b=0 47.374 45.97 45.97 45.97 44.567 44.567 37.67 36.297 33.551 32.178 29.432 25.313 coh733:k=53.3632,b=0 47.466 46.061 46.061 46.061 44.655 44.655 37.749 36.374 34.751 32.25 29.5 25.375 coh734:k=53.4636,b=0 47.558 46.151 46.151 46.151 44.743 44.743 37.828 36.452 35.075 32.321 29.567 25.437 coh735:k=53.5639,b=0 47.651 46.241 46.241 46.241 44.832 44.832 37.907 36.529 35.15 32.392 29.635 25.499 coh736:k=53.6643,b=0 47.743 46.332 46.332 46.332 44.92 44.92 37.986 36.606 35.225 32.464 29.703 25.561 coh737:k=53.7647,b=0 47.835 46.422 46.422 46.422 45.009 45.009 38.065 36.683 35.3 32.535 29.77 25.623 coh738:k=53.865,b=0 47.928 46.512 46.512 46.512 45.097 45.097 38.144 36.76 35.376 32.607 29.838 25.685 coh739:k=53.9654,b=0 48.02 46.603 46.603 46.603 45.186 45.186 38.223 36.837 35.451 32.678 29.906 25.747 coh740:k=54.0657,b=0 48.112 46.693 46.693 46.693 45.274 45.274 38.302 36.914 35.526 32.75 29.974 26.16 coh741:k=54.1661,b=0 48.204 46.783 46.783 46.783 45.363 45.363 38.381 36.991 35.601 32.821 30.041 27.261 coh742:k=54.2665,b=0 48.297 46.874 46.874 46.874 45.451 45.451 38.46 37.069 35.677 32.893 30.109 27.325 coh743:k=54.3668,b=0 48.389 46.964 46.964 46.964 45.539 45.539 38.539 37.146 35.752 32.964 30.177 27.389 coh744:k=54.4672,b=0 48.481 47.055 47.055 47.055 45.628 45.628 38.618 37.223 35.827 33.036 30.244 27.453 coh745:k=54.5675,b=0 48.574 47.145 47.145 47.145 45.716 45.716 38.697 37.3 35.902 33.107 30.312 27.517 coh746:k=54.6679,b=0 48.668 47.239 47.239 47.239 44.374 44.374 38.776 37.377 35.978 33.179 30.38 27.581 coh747:k=54.7683,b=0 47.263 45.862 45.862 45.862 44.461 44.461 38.855 37.454 36.053 33.25 30.448 27.645 coh748:k=54.8686,b=0 47.354 45.95 45.95 45.95 44.547 44.547 38.934 37.531 36.128 33.322 30.515 27.709 coh749:k=54.969,b=0 47.444 46.039 46.039 46.039 44.634 44.634 39.013 37.608 36.203 33.393 30.583 27.773 coh750:k=55.0693,b=0 47.534 46.127 46.127 46.127 44.72 44.72 39.092 37.686 36.279 33.465 30.651 27.837 coh751:k=55.1697,b=0 47.625 46.216 46.216 46.216 44.807 44.807 39.681 37.763 36.354 33.536 30.718 27.901 coh752:k=55.2701,b=0 47.715 46.304 46.304 46.304 44.893 44.893 40.661 37.84 36.429 33.608 30.786 28.361 coh753:k=55.3704,b=0 47.805 46.393 46.393 46.393 44.98 44.98 40.742 37.917 36.504 33.679 31.136 29.441 coh754:k=55.4708,b=0 47.895 46.481 46.481 46.481 45.066 45.066 40.823 37.994 36.58 33.751 32.336 29.339 coh755:k=55.5711,b=0 47.986 46.569 46.569 46.569 45.153 45.153 40.904 38.071 36.655 33.822 32.352 28.157 coh756:k=55.6715,b=0 48.076 46.658 46.658 46.658 45.239 45.239 40.985 38.148 36.73 33.893 31.118 28.22 coh757:k=55.7719,b=0 48.166 46.746 46.746 46.746 45.326 45.326 41.066 38.225 36.805 33.965 31.125 28.284 coh758:k=55.8722,b=0 48.257 46.835 46.835 46.835 45.413 45.413 41.147 38.302 36.88 34.036 31.192 28.348 coh759:k=55.9726,b=0 48.347 46.923 46.923 46.923 45.499 45.499 41.227 38.38 36.956 34.108 31.26 28.412 coh760:k=56.0729,b=0 48.437 47.011 47.011 47.011 45.586 45.586 41.308 38.457 37.031 34.179 31.328 28.476 coh761:k=56.1733,b=0 48.527 47.1 47.1 47.1 45.672 45.672 41.389 38.534 37.106 34.251 31.396 28.54 coh762:k=56.2737,b=0 48.619 47.19 47.19 47.19 45.762 45.762 41.476 38.619 37.19 34.333 31.476 28.619 coh763:k=56.374,b=0 48.711 47.282 47.282 47.282 45.854 45.854 41.568 38.711 37.282 34.425 31.568 28.711 coh764:k=56.4744,b=0 48.803 47.374 47.374 47.374 45.946 45.946 41.66 38.803 37.374 34.517 31.66 28.803 coh765:k=56.5747,b=0 48.895 47.467 47.467 47.467 46.038 46.038 41.752 38.895 37.467 34.609 31.752 27.712 coh766:k=56.6751,b=0 48.987 47.559 47.559 47.559 46.13 46.13 41.844 38.987 37.559 33.395 30.584 27.774 coh767:k=56.7755,b=0 49.08 47.651 47.651 47.651 46.222 46.222 39.092 37.686 36.279 33.465 30.651 27.837 coh768:k=56.8758,b=0 49.172 47.743 47.743 47.743 44.805 44.805 39.651 37.761 36.352 33.535 30.717 27.899 coh769:k=56.9762,b=0 47.711 46.3 46.3 46.3 44.89 44.89 40.658 37.836 36.426 33.604 30.783 28.3 coh770:k=57.0765,b=0 47.799 46.387 46.387 46.387 44.974 44.974 40.737 37.912 36.499 33.674 31.045 29.437 coh771:k=57.1769,b=0 47.887 46.473 46.473 46.473 45.059 45.059 40.816 37.987 36.573 33.744 32.33 29.449 coh772:k=57.2773,b=0 47.976 46.56 46.56 46.56 45.143 45.143 40.895 38.063 36.646 33.814 32.398 28.24 tb_pol_k: optimal risky investment choice for each state space point z1_0_34741 z2_0_40076 z3_0_4623 z4_0_5333 z5_0_61519 z6_0_70966 z10_1_2567 z11_1_4496 z12_1_6723 z13_1_9291 z14_2_2253 z15_2_567 __________ __________ _________ _________ __________ __________ __________ __________ __________ __________ __________ _________ coh1:k=-20,b=0 0.81633 0.81633 1.2245 1.6327 1.6327 2.0408 4.0816 4.898 5.7143 6.5306 7.7551 9.3878 coh2:k=-19.8996,b=0 0.81633 0.81633 1.2245 1.6327 1.6327 2.0408 4.0816 4.898 5.7143 6.5306 7.7551 9.3878 coh3:k=-19.7993,b=0 0.81633 0.81633 1.2245 1.6327 1.6327 2.0408 4.0816 4.898 5.7143 6.5306 7.7551 9.3878 coh4:k=-19.6989,b=0 0.81633 0.81633 1.2245 1.6327 1.6327 2.0408 4.0816 4.898 5.7143 6.5306 7.7551 9.3878 coh5:k=-19.5986,b=0 0.81633 0.81633 1.2245 1.6327 1.6327 2.0408 4.0816 4.898 5.7143 6.5306 7.7551 9.3878 coh6:k=-19.4982,b=0 0 0 0 0 1.6327 2.0408 4.0816 4.898 5.7143 6.5306 7.7551 9.3878 coh7:k=-19.3978,b=0 0 0 0 0 0 0 4.0816 4.898 5.7143 6.5306 7.7551 9.3878 coh8:k=-19.2975,b=0 0 0 0 0 0 0 4.0816 4.898 5.7143 6.5306 7.7551 9.3878 coh9:k=-19.1971,b=0 0 0 0 0 0 0 4.0816 4.898 5.7143 6.5306 7.7551 9.3878 coh10:k=-19.0968,b=0 0 0 0 0 0 0 0 4.898 5.7143 6.5306 7.7551 9.3878 coh11:k=-18.9964,b=0 0 0 0 0 0 0 0 0 5.7143 6.5306 7.7551 9.3878 coh12:k=-18.896,b=0 0 0 0 0 0 0 0 0 0 6.5306 7.7551 9.3878 coh13:k=-18.7957,b=0 0 0 0 0 0 0 0 0 0 6.5306 7.7551 9.3878 coh14:k=-18.6953,b=0 0 0 0 0 0 0 0 0 0 0 7.7551 9.3878 coh15:k=-18.595,b=0 0 0 0 0 0 0 0 0 0 0 7.7551 9.3878 coh16:k=-18.4946,b=0 0 0 0 0 0 0 0 0 0 0 0 9.3878 coh17:k=-18.3942,b=0 0 0 0 0 0 0 0 0 0 0 0 9.3878 coh18:k=-18.2939,b=0 0 0 0 0 0 0 0 0 0 0 0 9.3878 coh19:k=-18.1935,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh20:k=-18.0932,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh21:k=-17.9928,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh22:k=-17.8924,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh23:k=-17.7921,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh24:k=-17.6917,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh25:k=-17.5914,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh26:k=-17.491,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh27:k=-17.3906,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh28:k=-17.2903,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh29:k=-17.1899,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh30:k=-17.0896,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh31:k=-16.9892,b=0 0.81822 0.98374 1.1389 1.3608 1.5943 1.8433 1.659 1.5976 0 0 0 0 coh32:k=-16.8888,b=0 0.81684 0.98399 1.1499 1.3546 1.5956 1.9048 1.7143 1.6508 1.5873 0 0 0 coh33:k=-16.7885,b=0 0.8168 0.98801 1.1592 1.3572 1.5948 1.8918 1.7696 1.7041 1.6385 1.573 1.5074 0 coh34:k=-16.6881,b=0 0.81314 0.9897 1.1589 1.3727 1.5951 1.8847 1.8249 1.7573 1.6897 1.6221 1.5546 1.487 coh35:k=-16.5878,b=0 0.8186 0.99013 1.1422 1.3624 1.5913 1.8841 1.8802 1.8106 1.7409 1.6713 1.6017 1.532 coh36:k=-16.4874,b=0 0.84267 0.99026 1.1263 1.3644 1.6039 1.8889 1.8638 1.8638 1.7921 1.7205 1.6488 1.5771 coh37:k=-16.387,b=0 0.83966 0.98896 1.1289 1.3516 1.6225 1.9056 1.9171 1.9171 1.8433 1.7696 1.6959 1.6221 coh38:k=-16.2867,b=0 0.8421 0.98593 1.1284 1.3604 1.6183 1.9323 1.9703 1.8946 1.8946 1.8188 1.743 1.6672 coh39:k=-16.1863,b=0 0.83408 0.9818 1.1298 1.368 1.6344 1.933 2.0236 1.9458 1.9458 1.8679 1.8679 2.3349 coh40:k=-16.086,b=0 0.82372 0.98304 1.1298 1.3743 1.6449 1.9334 2.0768 1.997 1.997 1.9171 1.9171 2.5561 coh41:k=-15.9856,b=0 0.8293 0.9772 1.1537 1.3785 1.6292 1.9308 2.1301 2.0482 2.0482 1.9662 2.4578 2.7036 coh42:k=-15.8852,b=0 0.82261 0.97044 1.1761 1.3771 1.6256 1.9455 2.1833 2.0994 2.0994 2.0994 2.6872 2.7712 coh43:k=-15.7849,b=0 0.84267 0.96166 1.1723 1.3807 1.6353 1.9644 2.2366 2.1506 2.1506 2.6667 2.7527 2.8388 coh44:k=-15.6845,b=0 0.84363 0.97058 1.1672 1.3825 1.6431 1.9527 2.2898 2.2018 2.2898 2.8183 2.8183 2.9944 coh45:k=-15.5842,b=0 0.82983 0.99315 1.1701 1.3834 1.6501 1.9483 2.3431 2.3431 2.7937 2.8838 2.9739 3.0641 coh46:k=-15.4838,b=0 0.84644 0.98299 1.164 1.3822 1.6463 1.9623 2.3964 2.4885 2.9494 3.0415 3.1337 3.2259 coh47:k=-15.3834,b=0 0.85433 0.98968 1.157 1.38 1.6521 1.9583 2.4496 3.1091 3.1091 3.1091 3.2033 3.2975 coh48:k=-15.2831,b=0 0.83677 0.97755 1.1485 1.3764 1.6568 1.9384 3.0804 3.1767 3.1767 3.1767 3.273 3.3692 coh49:k=-15.1827,b=0 0.84731 0.98914 1.1637 1.371 1.6596 1.9405 3.2443 3.2443 3.2443 3.2443 3.3426 3.5392 coh50:k=-15.0824,b=0 0.83155 1.0084 1.1866 1.3751 1.6619 1.9486 3.4122 3.3119 3.3119 3.4122 3.5126 3.613 coh723:k=52.3596,b=0 1.3863 2.7726 2.7726 2.7726 4.1589 4.1589 9.4932 10.849 13.562 14.918 18.986 21.699 coh724:k=52.46,b=0 1.3882 2.7765 2.7765 2.7765 4.1647 4.1647 9.5064 10.864 13.581 14.939 19.013 21.729 coh725:k=52.5603,b=0 1.3902 2.7803 2.7803 2.7803 4.1705 4.1705 9.5196 10.88 13.599 14.959 19.039 21.759 coh726:k=52.6607,b=0 1.3921 2.7842 2.7842 2.7842 4.1763 4.1763 9.5327 10.895 13.618 14.98 19.065 21.789 coh727:k=52.7611,b=0 1.394 2.788 2.788 2.788 4.182 4.182 9.5459 10.91 13.637 15.001 19.092 21.819 coh728:k=52.8614,b=0 1.3959 2.7919 2.7919 2.7919 4.1878 4.1878 9.5591 10.925 13.656 15.021 18.812 21.849 coh729:k=52.9618,b=0 1.3979 2.7957 2.7957 2.7957 4.1936 4.1936 9.5722 10.94 13.675 15.042 17.777 21.879 coh730:k=53.0621,b=0 1.3998 2.7995 2.7995 2.7995 4.1993 4.1993 9.5854 10.955 13.693 15.063 17.801 21.91 coh731:k=53.1625,b=0 1.4017 2.8034 2.8034 2.8034 4.2051 4.2051 9.5986 10.97 13.712 15.083 17.826 21.94 coh732:k=53.2629,b=0 1.4036 2.8072 2.8072 2.8072 4.2109 4.2109 9.6117 10.985 13.731 15.104 17.85 21.97 coh733:k=53.3632,b=0 1.4055 2.8111 2.8111 2.8111 4.2166 4.2166 9.6249 11 12.624 15.125 17.875 22 coh734:k=53.4636,b=0 1.4075 2.8149 2.8149 2.8149 4.2224 4.2224 9.6381 11.015 12.392 15.146 17.899 22.03 coh735:k=53.5639,b=0 1.4094 2.8188 2.8188 2.8188 4.2282 4.2282 9.6512 11.03 12.409 15.166 17.924 22.06 coh736:k=53.6643,b=0 1.4113 2.8226 2.8226 2.8226 4.2339 4.2339 9.6644 11.045 12.426 15.187 17.948 22.09 coh737:k=53.7647,b=0 1.4132 2.8265 2.8265 2.8265 4.2397 4.2397 9.6776 11.06 12.443 15.208 17.973 22.12 coh738:k=53.865,b=0 1.4152 2.8303 2.8303 2.8303 4.2455 4.2455 9.6907 11.075 12.46 15.228 17.997 22.15 coh739:k=53.9654,b=0 1.4171 2.8342 2.8342 2.8342 4.2512 4.2512 9.7039 11.09 12.476 15.249 18.022 22.18 coh740:k=54.0657,b=0 1.419 2.838 2.838 2.838 4.257 4.257 9.7171 11.105 12.493 15.27 18.046 21.86 coh741:k=54.1661,b=0 1.4209 2.8418 2.8418 2.8418 4.2628 4.2628 9.7302 11.12 12.51 15.29 18.07 20.851 coh742:k=54.2665,b=0 1.4228 2.8457 2.8457 2.8457 4.2685 4.2685 9.7434 11.135 12.527 15.311 18.095 20.879 coh743:k=54.3668,b=0 1.4248 2.8495 2.8495 2.8495 4.2743 4.2743 9.7566 11.15 12.544 15.332 18.119 20.907 coh744:k=54.4672,b=0 1.4267 2.8534 2.8534 2.8534 4.2801 4.2801 9.7697 11.165 12.561 15.352 18.144 20.935 coh745:k=54.5675,b=0 1.4286 2.8571 2.8571 2.8571 4.2857 4.2857 9.7829 11.18 12.578 15.373 18.168 20.963 coh746:k=54.6679,b=0 1.4286 2.8571 2.8571 2.8571 4.1983 4.1983 9.7961 11.196 12.595 15.394 18.193 20.992 coh747:k=54.7683,b=0 1.4013 2.8026 2.8026 2.8026 4.204 4.204 9.8092 11.211 12.612 15.415 18.217 21.02 coh748:k=54.8686,b=0 1.4032 2.8064 2.8064 2.8064 4.2096 4.2096 9.8224 11.226 12.629 15.435 18.242 21.048 coh749:k=54.969,b=0 1.4051 2.8102 2.8102 2.8102 4.2152 4.2152 9.8356 11.241 12.646 15.456 18.266 21.076 coh750:k=55.0693,b=0 1.407 2.8139 2.8139 2.8139 4.2209 4.2209 9.8487 11.256 12.663 15.477 18.291 21.104 coh751:k=55.1697,b=0 1.4088 2.8177 2.8177 2.8177 4.2265 4.2265 9.352 11.271 12.68 15.497 18.315 21.133 coh752:k=55.2701,b=0 1.4107 2.8215 2.8215 2.8215 4.2322 4.2322 8.4644 11.286 12.697 15.518 18.339 20.765 coh753:k=55.3704,b=0 1.4126 2.8252 2.8252 2.8252 4.2378 4.2378 8.4756 11.301 12.713 15.539 18.081 19.776 coh754:k=55.4708,b=0 1.4145 2.829 2.829 2.829 4.2435 4.2435 8.4869 11.316 12.73 15.559 16.974 19.971 coh755:k=55.5711,b=0 1.4164 2.8327 2.8327 2.8327 4.2491 4.2491 8.4982 11.331 12.747 15.58 17.05 21.246 coh756:k=55.6715,b=0 1.4182 2.8365 2.8365 2.8365 4.2547 4.2547 8.5095 11.346 12.764 15.601 18.376 21.274 coh757:k=55.7719,b=0 1.4201 2.8403 2.8403 2.8403 4.2604 4.2604 8.5208 11.361 12.781 15.621 18.462 21.302 coh758:k=55.8722,b=0 1.422 2.844 2.844 2.844 4.266 4.266 8.5321 11.376 12.798 15.642 18.486 21.33 coh759:k=55.9726,b=0 1.4239 2.8478 2.8478 2.8478 4.2717 4.2717 8.5434 11.391 12.815 15.663 18.511 21.358 coh760:k=56.0729,b=0 1.4258 2.8515 2.8515 2.8515 4.2773 4.2773 8.5546 11.406 12.832 15.684 18.535 21.387 coh761:k=56.1733,b=0 1.4277 2.8553 2.8553 2.8553 4.283 4.283 8.5659 11.421 12.849 15.704 18.56 21.415 coh762:k=56.2737,b=0 1.4286 2.8571 2.8571 2.8571 4.2857 4.2857 8.5714 11.429 12.857 15.714 18.571 21.429 coh763:k=56.374,b=0 1.4286 2.8571 2.8571 2.8571 4.2857 4.2857 8.5714 11.429 12.857 15.714 18.571 21.429 coh764:k=56.4744,b=0 1.4286 2.8571 2.8571 2.8571 4.2857 4.2857 8.5714 11.429 12.857 15.714 18.571 21.429 coh765:k=56.5747,b=0 1.4286 2.8571 2.8571 2.8571 4.2857 4.2857 8.5714 11.429 12.857 15.714 18.571 21.049 coh766:k=56.6751,b=0 1.4286 2.8571 2.8571 2.8571 4.2857 4.2857 8.5714 11.429 12.857 15.456 18.267 21.077 coh767:k=56.7755,b=0 1.4286 2.8571 2.8571 2.8571 4.2857 4.2857 9.8487 11.256 12.663 15.477 18.291 21.104 coh768:k=56.8758,b=0 1.4286 2.8571 2.8571 2.8571 4.2264 4.2264 9.3805 11.27 12.679 15.497 18.314 21.132 coh769:k=56.9762,b=0 1.4106 2.8213 2.8213 2.8213 4.2319 4.2319 8.4639 11.285 12.696 15.517 18.338 20.821 coh770:k=57.0765,b=0 1.4125 2.825 2.825 2.825 4.2374 4.2374 8.4749 11.3 12.712 15.537 18.167 19.775 coh771:k=57.1769,b=0 1.4143 2.8286 2.8286 2.8286 4.243 4.243 8.4859 11.315 12.729 15.558 16.972 19.853 coh772:k=57.2773,b=0 1.4162 2.8323 2.8323 2.8323 4.2485 4.2485 8.497 11.329 12.745 15.578 16.994 21.151 tb_pol_w: risky + safe investment choices (first stage choice, choose within risky vs safe) z1_0_34741 z2_0_40076 z3_0_4623 z4_0_5333 z5_0_61519 z6_0_70966 z10_1_2567 z11_1_4496 z12_1_6723 z13_1_9291 z14_2_2253 z15_2_567 __________ __________ _________ _________ __________ __________ __________ __________ __________ __________ __________ _________ coh1:k=-20,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh2:k=-19.8996,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh3:k=-19.7993,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh4:k=-19.6989,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh5:k=-19.5986,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh6:k=-19.4982,b=0 -20 -20 -20 -20 0 0 0 0 0 0 0 0 coh7:k=-19.3978,b=0 -20 -20 -20 -20 -20 -20 0 0 0 0 0 0 coh8:k=-19.2975,b=0 -20 -20 -20 -20 -20 -20 0 0 0 0 0 0 coh9:k=-19.1971,b=0 -20 -20 -20 -20 -20 -20 0 0 0 0 0 0 coh10:k=-19.0968,b=0 -20 -20 -20 -20 -20 -20 -20 0 0 0 0 0 coh11:k=-18.9964,b=0 -20 -20 -20 -20 -20 -20 -20 -20 0 0 0 0 coh12:k=-18.896,b=0 -20 -20 -20 -20 -20 -20 -20 -20 -20 0 0 0 coh13:k=-18.7957,b=0 -20 -20 -20 -20 -20 -20 -20 -20 -20 0 0 0 coh14:k=-18.6953,b=0 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 0 0 coh15:k=-18.595,b=0 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 0 0 coh16:k=-18.4946,b=0 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 0 coh17:k=-18.3942,b=0 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 0 coh18:k=-18.2939,b=0 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 0 coh19:k=-18.1935,b=0 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 coh20:k=-18.0932,b=0 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 coh21:k=-17.9928,b=0 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 coh22:k=-17.8924,b=0 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 coh23:k=-17.7921,b=0 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 coh24:k=-17.6917,b=0 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 coh25:k=-17.5914,b=0 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 coh26:k=-17.491,b=0 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 coh27:k=-17.3906,b=0 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 coh28:k=-17.2903,b=0 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 coh29:k=-17.1899,b=0 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 coh30:k=-17.0896,b=0 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 -20 coh31:k=-16.9892,b=0 -17.972 -17.972 -18.034 -18.095 -18.095 -18.157 -18.341 -18.402 -20 -20 -20 -20 coh32:k=-16.8888,b=0 -17.905 -17.968 -17.968 -18.032 -18.032 -18.095 -18.286 -18.349 -18.413 -20 -20 -20 coh33:k=-16.7885,b=0 -17.903 -17.903 -17.903 -17.968 -17.968 -18.034 -18.23 -18.296 -18.361 -18.427 -18.493 -20 coh34:k=-16.6881,b=0 -17.837 -17.837 -17.905 -17.905 -17.972 -17.972 -18.175 -18.243 -18.31 -18.378 -18.445 -18.513 coh35:k=-16.5878,b=0 -17.772 -17.772 -17.841 -17.841 -17.911 -17.981 -18.12 -18.189 -18.259 -18.329 -18.398 -18.468 coh36:k=-16.4874,b=0 -17.706 -17.778 -17.778 -17.849 -17.849 -17.921 -18.136 -18.136 -18.208 -18.28 -18.351 -18.423 coh37:k=-16.387,b=0 -17.714 -17.714 -17.714 -17.788 -17.788 -17.862 -18.083 -18.083 -18.157 -18.23 -18.304 -18.378 coh38:k=-16.2867,b=0 -17.651 -17.651 -17.727 -17.727 -17.802 -17.802 -18.03 -18.105 -18.105 -18.181 -18.257 -18.333 coh39:k=-16.1863,b=0 -17.587 -17.587 -17.665 -17.665 -17.743 -17.743 -17.976 -18.054 -18.054 -18.132 -18.132 -17.665 coh40:k=-16.086,b=0 -17.524 -17.604 -17.604 -17.604 -17.684 -17.684 -17.923 -18.003 -18.003 -18.083 -18.083 -17.444 coh41:k=-15.9856,b=0 -17.46 -17.542 -17.542 -17.542 -17.624 -17.706 -17.87 -17.952 -17.952 -18.034 -17.542 -17.296 coh42:k=-15.8852,b=0 -17.481 -17.481 -17.481 -17.565 -17.565 -17.649 -17.817 -17.901 -17.901 -17.901 -17.313 -17.229 coh43:k=-15.7849,b=0 -17.419 -17.419 -17.419 -17.505 -17.505 -17.591 -17.763 -17.849 -17.849 -17.333 -17.247 -17.161 coh44:k=-15.6845,b=0 -17.358 -17.358 -17.358 -17.446 -17.446 -17.534 -17.71 -17.798 -17.71 -17.182 -17.182 -17.006 coh45:k=-15.5842,b=0 -17.296 -17.296 -17.387 -17.387 -17.387 -17.477 -17.657 -17.657 -17.206 -17.116 -17.026 -16.936 coh46:k=-15.4838,b=0 -17.235 -17.235 -17.327 -17.327 -17.419 -17.419 -17.604 -17.511 -17.051 -16.958 -16.866 -16.774 coh47:k=-15.3834,b=0 -17.174 -17.268 -17.268 -17.268 -17.362 -17.362 -17.55 -16.891 -16.891 -16.891 -16.797 -16.702 coh48:k=-15.2831,b=0 -17.112 -17.208 -17.208 -17.208 -17.305 -17.305 -16.92 -16.823 -16.823 -16.823 -16.727 -16.631 coh49:k=-15.1827,b=0 -17.149 -17.149 -17.149 -17.149 -17.247 -17.247 -16.756 -16.756 -16.756 -16.756 -16.657 -16.461 coh50:k=-15.0824,b=0 -17.09 -17.09 -17.09 -17.19 -17.19 -17.19 -16.588 -16.688 -16.688 -16.588 -16.487 -16.387 coh723:k=52.3596,b=0 47.929 47.929 47.929 47.929 47.929 47.929 46.453 46.453 46.453 46.453 46.453 46.453 coh724:k=52.46,b=0 48.024 48.024 48.024 48.024 48.024 48.024 46.545 46.545 46.545 46.545 46.545 46.545 coh725:k=52.5603,b=0 48.118 48.118 48.118 48.118 48.118 48.118 46.637 46.637 46.637 46.637 46.637 46.637 coh726:k=52.6607,b=0 48.212 48.212 48.212 48.212 48.212 48.212 46.729 46.729 46.729 46.729 46.729 46.729 coh727:k=52.7611,b=0 48.306 48.306 48.306 48.306 48.306 48.306 46.821 46.821 46.821 46.821 46.821 46.821 coh728:k=52.8614,b=0 48.401 48.401 48.401 48.401 48.401 48.401 46.914 46.914 46.914 46.914 46.914 46.914 coh729:k=52.9618,b=0 48.495 48.495 48.495 48.495 48.495 48.495 47.006 47.006 47.006 47.006 47.006 47.006 coh730:k=53.0621,b=0 48.589 48.589 48.589 48.589 48.589 48.589 47.098 47.098 47.098 47.098 47.098 47.098 coh731:k=53.1625,b=0 48.683 48.683 48.683 48.683 48.683 48.683 47.19 47.19 47.19 47.19 47.19 47.19 coh732:k=53.2629,b=0 48.777 48.777 48.777 48.777 48.777 48.777 47.282 47.282 47.282 47.282 47.282 47.282 coh733:k=53.3632,b=0 48.872 48.872 48.872 48.872 48.872 48.872 47.374 47.374 47.374 47.374 47.374 47.374 coh734:k=53.4636,b=0 48.966 48.966 48.966 48.966 48.966 48.966 47.467 47.467 47.467 47.467 47.467 47.467 coh735:k=53.5639,b=0 49.06 49.06 49.06 49.06 49.06 49.06 47.559 47.559 47.559 47.559 47.559 47.559 coh736:k=53.6643,b=0 49.154 49.154 49.154 49.154 49.154 49.154 47.651 47.651 47.651 47.651 47.651 47.651 coh737:k=53.7647,b=0 49.248 49.248 49.248 49.248 49.248 49.248 47.743 47.743 47.743 47.743 47.743 47.743 coh738:k=53.865,b=0 49.343 49.343 49.343 49.343 49.343 49.343 47.835 47.835 47.835 47.835 47.835 47.835 coh739:k=53.9654,b=0 49.437 49.437 49.437 49.437 49.437 49.437 47.927 47.927 47.927 47.927 47.927 47.927 coh740:k=54.0657,b=0 49.531 49.531 49.531 49.531 49.531 49.531 48.02 48.02 48.02 48.02 48.02 48.02 coh741:k=54.1661,b=0 49.625 49.625 49.625 49.625 49.625 49.625 48.112 48.112 48.112 48.112 48.112 48.112 coh742:k=54.2665,b=0 49.72 49.72 49.72 49.72 49.72 49.72 48.204 48.204 48.204 48.204 48.204 48.204 coh743:k=54.3668,b=0 49.814 49.814 49.814 49.814 49.814 49.814 48.296 48.296 48.296 48.296 48.296 48.296 coh744:k=54.4672,b=0 49.908 49.908 49.908 49.908 49.908 49.908 48.388 48.388 48.388 48.388 48.388 48.388 coh745:k=54.5675,b=0 50.002 50.002 50.002 50.002 50.002 50.002 48.48 48.48 48.48 48.48 48.48 48.48 coh746:k=54.6679,b=0 50.096 50.096 50.096 50.096 48.573 48.573 48.573 48.573 48.573 48.573 48.573 48.573 coh747:k=54.7683,b=0 48.665 48.665 48.665 48.665 48.665 48.665 48.665 48.665 48.665 48.665 48.665 48.665 coh748:k=54.8686,b=0 48.757 48.757 48.757 48.757 48.757 48.757 48.757 48.757 48.757 48.757 48.757 48.757 coh749:k=54.969,b=0 48.849 48.849 48.849 48.849 48.849 48.849 48.849 48.849 48.849 48.849 48.849 48.849 coh750:k=55.0693,b=0 48.941 48.941 48.941 48.941 48.941 48.941 48.941 48.941 48.941 48.941 48.941 48.941 coh751:k=55.1697,b=0 49.033 49.033 49.033 49.033 49.033 49.033 49.033 49.033 49.033 49.033 49.033 49.033 coh752:k=55.2701,b=0 49.126 49.126 49.126 49.126 49.126 49.126 49.126 49.126 49.126 49.126 49.126 49.126 coh753:k=55.3704,b=0 49.218 49.218 49.218 49.218 49.218 49.218 49.218 49.218 49.218 49.218 49.218 49.218 coh754:k=55.4708,b=0 49.31 49.31 49.31 49.31 49.31 49.31 49.31 49.31 49.31 49.31 49.31 49.31 coh755:k=55.5711,b=0 49.402 49.402 49.402 49.402 49.402 49.402 49.402 49.402 49.402 49.402 49.402 49.402 coh756:k=55.6715,b=0 49.494 49.494 49.494 49.494 49.494 49.494 49.494 49.494 49.494 49.494 49.494 49.494 coh757:k=55.7719,b=0 49.586 49.586 49.586 49.586 49.586 49.586 49.586 49.586 49.586 49.586 49.586 49.586 coh758:k=55.8722,b=0 49.679 49.679 49.679 49.679 49.679 49.679 49.679 49.679 49.679 49.679 49.679 49.679 coh759:k=55.9726,b=0 49.771 49.771 49.771 49.771 49.771 49.771 49.771 49.771 49.771 49.771 49.771 49.771 coh760:k=56.0729,b=0 49.863 49.863 49.863 49.863 49.863 49.863 49.863 49.863 49.863 49.863 49.863 49.863 coh761:k=56.1733,b=0 49.955 49.955 49.955 49.955 49.955 49.955 49.955 49.955 49.955 49.955 49.955 49.955 coh762:k=56.2737,b=0 50.047 50.047 50.047 50.047 50.047 50.047 50.047 50.047 50.047 50.047 50.047 50.047 coh763:k=56.374,b=0 50.139 50.139 50.139 50.139 50.139 50.139 50.139 50.139 50.139 50.139 50.139 50.139 coh764:k=56.4744,b=0 50.232 50.232 50.232 50.232 50.232 50.232 50.232 50.232 50.232 50.232 50.232 50.232 coh765:k=56.5747,b=0 50.324 50.324 50.324 50.324 50.324 50.324 50.324 50.324 50.324 50.324 50.324 48.761 coh766:k=56.6751,b=0 50.416 50.416 50.416 50.416 50.416 50.416 50.416 50.416 50.416 48.851 48.851 48.851 coh767:k=56.7755,b=0 50.508 50.508 50.508 50.508 50.508 50.508 48.941 48.941 48.941 48.941 48.941 48.941 coh768:k=56.8758,b=0 50.6 50.6 50.6 50.6 49.031 49.031 49.031 49.031 49.031 49.031 49.031 49.031 coh769:k=56.9762,b=0 49.121 49.121 49.121 49.121 49.121 49.121 49.121 49.121 49.121 49.121 49.121 49.121 coh770:k=57.0765,b=0 49.212 49.212 49.212 49.212 49.212 49.212 49.212 49.212 49.212 49.212 49.212 49.212 coh771:k=57.1769,b=0 49.302 49.302 49.302 49.302 49.302 49.302 49.302 49.302 49.302 49.302 49.302 49.302 coh772:k=57.2773,b=0 49.392 49.392 49.392 49.392 49.392 49.392 49.392 49.392 49.392 49.392 49.392 49.392
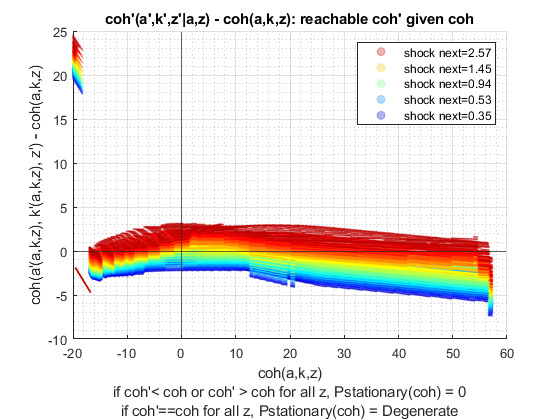
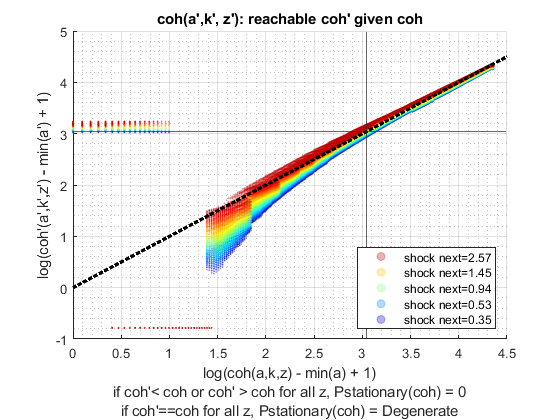
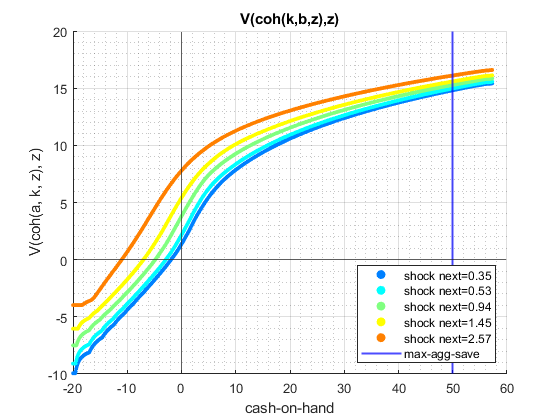
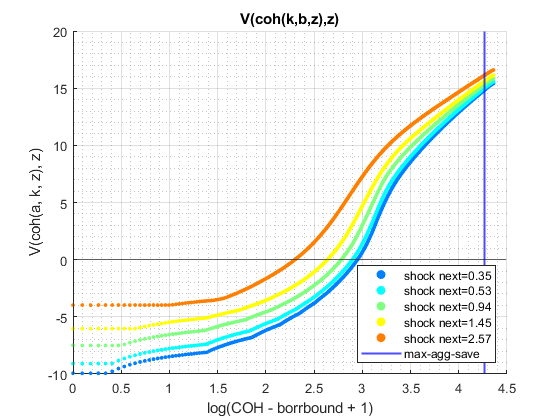
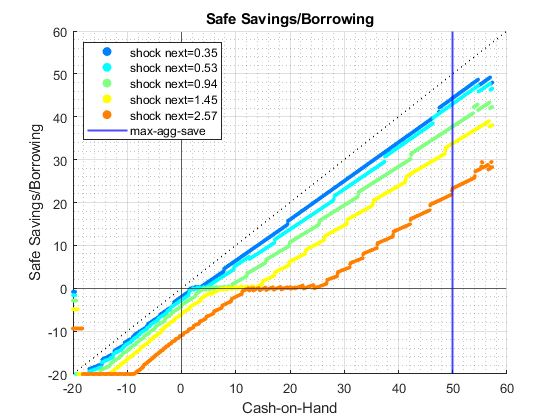
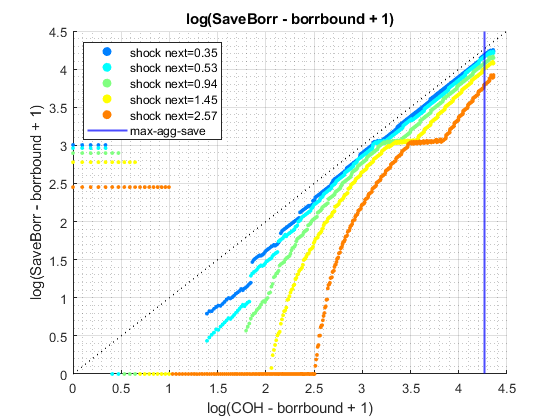
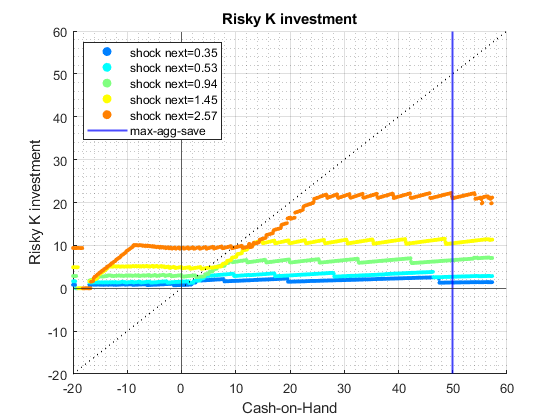
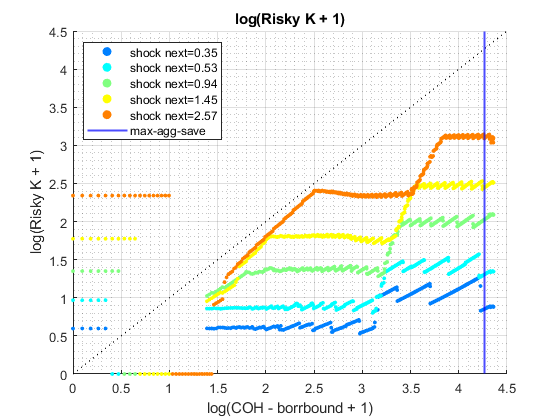
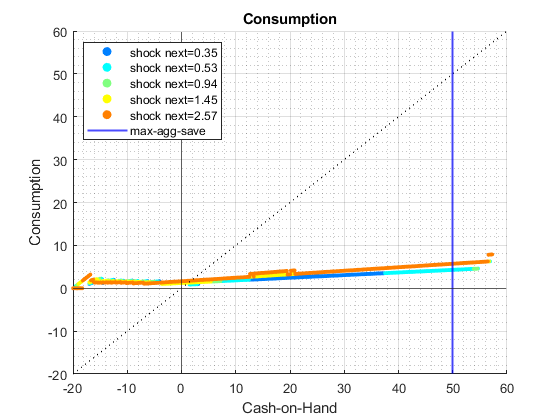
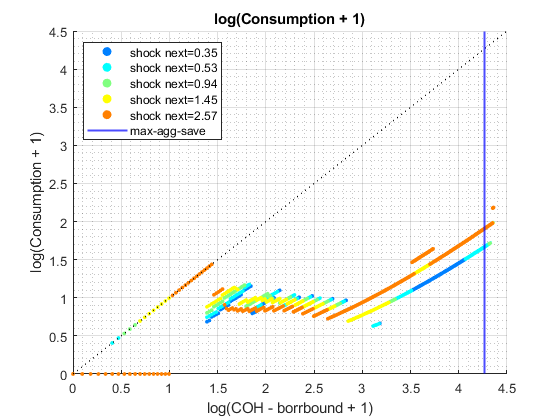
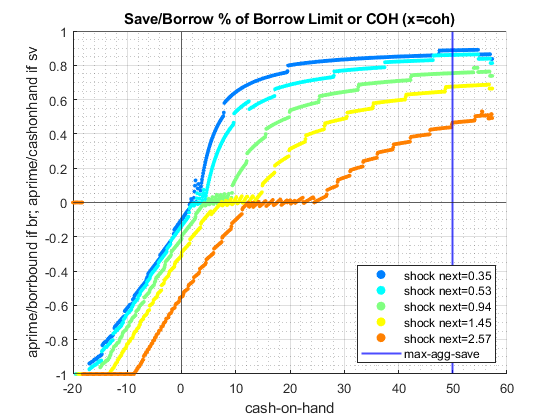
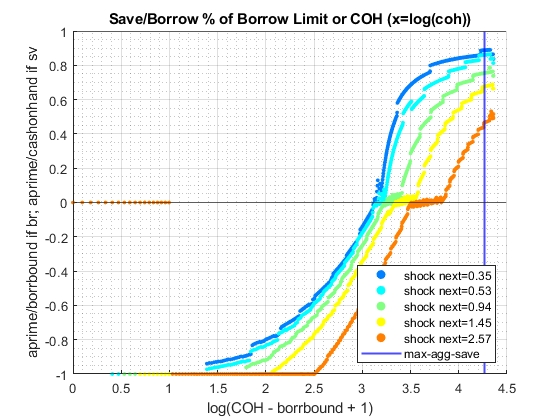
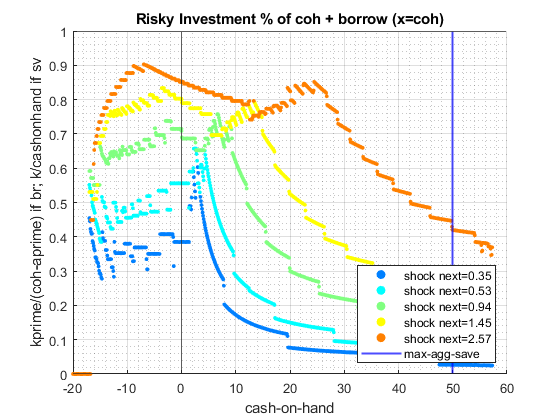
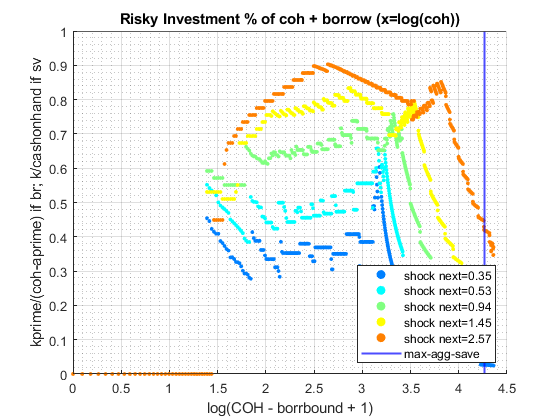
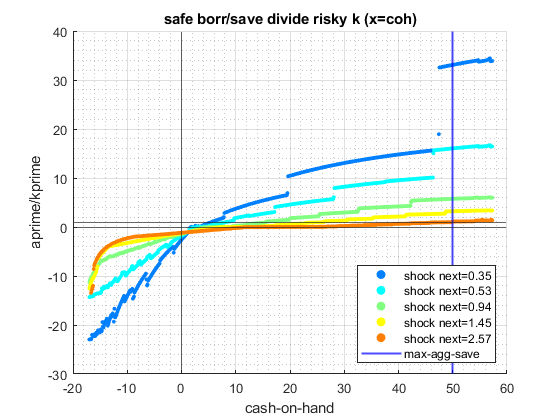
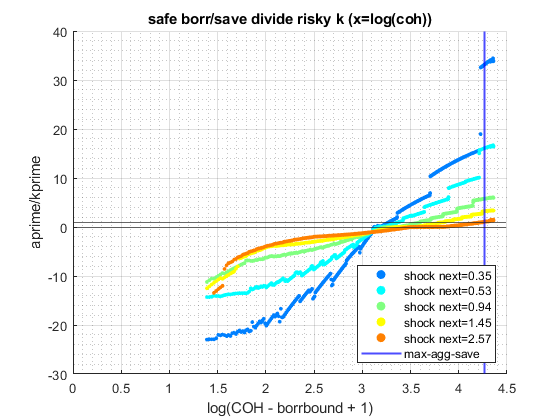
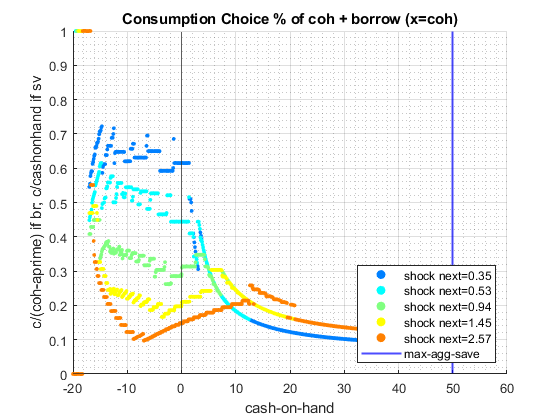
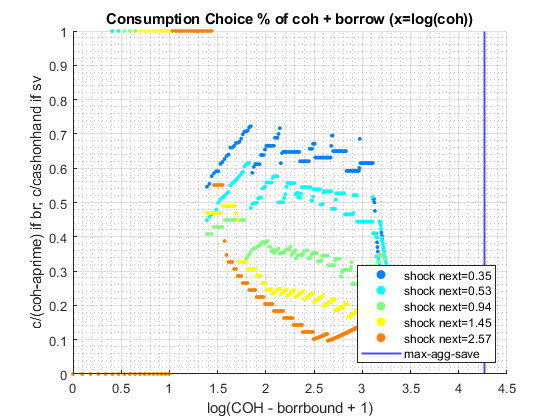
Display Various Containers
if (bl_display_defparam)
Display 1 support_map
fft_container_map_display(support_map, it_display_summmat_rowmax, it_display_summmat_colmax);
---------------------------------------- ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Begin: Show all key and value pairs from container CONTAINER NAME: SUPPORT_MAP ---------------------------------------- Map with properties: Count: 43 KeyType: char ValueType: any xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ---------------------------------------- ---------------------------------------- pos = 1 ; key = bl_display ; val = false pos = 2 ; key = bl_display_defparam ; val = true pos = 3 ; key = bl_display_dist ; val = false pos = 4 ; key = bl_display_evf ; val = false pos = 5 ; key = bl_display_final ; val = true pos = 6 ; key = bl_display_final_dist ; val = false pos = 7 ; key = bl_display_final_dist_detail ; val = false pos = 8 ; key = bl_display_funcgrids ; val = false pos = 9 ; key = bl_graph ; val = true pos = 10 ; key = bl_graph_coh_t_coh ; val = true pos = 11 ; key = bl_graph_evf ; val = false pos = 12 ; key = bl_graph_funcgrids ; val = false pos = 13 ; key = bl_graph_funcgrids_detail ; val = false pos = 14 ; key = bl_graph_onebyones ; val = true pos = 15 ; key = bl_graph_pol_lvl ; val = true pos = 16 ; key = bl_graph_pol_pct ; val = true pos = 17 ; key = bl_graph_val ; val = true pos = 18 ; key = bl_img_save ; val = false pos = 19 ; key = bl_mat ; val = false pos = 20 ; key = bl_post ; val = true pos = 21 ; key = bl_profile ; val = false pos = 22 ; key = bl_profile_dist ; val = false pos = 23 ; key = bl_time ; val = false pos = 24 ; key = it_display_every ; val = 5 pos = 25 ; key = it_display_final_colmax ; val = 12 pos = 26 ; key = it_display_final_rowmax ; val = 100 pos = 27 ; key = it_display_summmat_colmax ; val = 7 pos = 28 ; key = it_display_summmat_rowmax ; val = 7 pos = 29 ; key = st_img_name_main ; val = ff_ipwkbz_vf_vecsv_default pos = 30 ; key = st_img_path ; val = C:/Users/fan/CodeDynaAsset//m_ipwkbzr//solve/img/ pos = 31 ; key = st_img_prefix ; val = pos = 32 ; key = st_img_suffix ; val = _p4.png pos = 33 ; key = st_mat_name_main ; val = ff_ipwkbz_vf_vecsv_default pos = 34 ; key = st_mat_path ; val = C:/Users/fan/CodeDynaAsset//m_ipwkbzr//solve/mat/ pos = 35 ; key = st_mat_prefix ; val = pos = 36 ; key = st_mat_suffix ; val = _p4 pos = 37 ; key = st_mat_test_path ; val = C:/Users/fan/CodeDynaAsset//m_ipwkbzr//test/ff_ipwkbz_ds_vecsv/mat/ pos = 38 ; key = st_matimg_path_root ; val = C:/Users/fan/CodeDynaAsset//m_ipwkbzr/ pos = 39 ; key = st_profile_name_main ; val = ff_ipwkbz_vf_vecsv_default pos = 40 ; key = st_profile_path ; val = C:/Users/fan/CodeDynaAsset//m_ipwkbzr//solve/profile/ pos = 41 ; key = st_profile_prefix ; val = pos = 42 ; key = st_profile_suffix ; val = _p4 pos = 43 ; key = st_title_prefix ; val = ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Scalars in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx value __ ___ _____ bl_display 1 1 0 bl_display_defparam 2 2 1 bl_display_dist 3 3 0 bl_display_evf 4 4 0 bl_display_final 5 5 1 bl_display_final_dist 6 6 0 bl_display_final_dist_detail 7 7 0 bl_display_funcgrids 8 8 0 bl_graph 9 9 1 bl_graph_coh_t_coh 10 10 1 bl_graph_evf 11 11 0 bl_graph_funcgrids 12 12 0 bl_graph_funcgrids_detail 13 13 0 bl_graph_onebyones 14 14 1 bl_graph_pol_lvl 15 15 1 bl_graph_pol_pct 16 16 1 bl_graph_val 17 17 1 bl_img_save 18 18 0 bl_mat 19 19 0 bl_post 20 20 1 bl_profile 21 21 0 bl_profile_dist 22 22 0 bl_time 23 23 0 it_display_every 24 24 5 it_display_final_colmax 25 25 12 it_display_final_rowmax 26 26 100 it_display_summmat_colmax 27 27 7 it_display_summmat_rowmax 28 28 7 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Strings in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx __ ___ st_img_name_main 1 29 st_img_path 2 30 st_img_prefix 3 31 st_img_suffix 4 32 st_mat_name_main 5 33 st_mat_path 6 34 st_mat_prefix 7 35 st_mat_suffix 8 36 st_mat_test_path 9 37 st_matimg_path_root 10 38 st_profile_name_main 11 39 st_profile_path 12 40 st_profile_prefix 13 41 st_profile_suffix 14 42 st_title_prefix 15 43
Display 2 armt_map
fft_container_map_display(armt_map, it_display_summmat_rowmax, it_display_summmat_colmax);
---------------------------------------- ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Begin: Show all key and value pairs from container CONTAINER NAME: ARMT_MAP ---------------------------------------- Map with properties: Count: 21 KeyType: char ValueType: any xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ---------------------------------------- ---------------------------------------- pos = 1 ; key = ar_a_meshk ;rown= 772 ,coln= 1 ar_a_meshk :mu= 18.6145 ,sd= 22.347 ,min= -20 ,max= 57.2773 zi_1_c1 _______ zi_1_R1 -20 zi_2_R2 -19.9 zi_3_R3 -19.799 zi_386_r386 18.538 zi_770_r770 57.077 zi_771_r771 57.177 zi_772_r772 57.277 pos = 2 ; key = ar_a_meshk_ori ;rown= 35000 ,coln= 1 ar_a_meshk_ori :mu= -2.5107 ,sd= 15.6183 ,min= -20 ,max= 50 zi_1_c1 _______ zi_1_R1 -20 zi_2_R2 -20 zi_3_R3 -20 zi_17500_r17500 -20 zi_34998_r34998 -17.143 zi_34999_r34999 -18.571 zi_35000_r35000 -20 pos = 3 ; key = ar_ak_perc ;rown= 1 ,coln= 50 ar_ak_perc :mu= 0.5 ,sd= 0.2975 ,min= 0 ,max= 1 zi_1_C1 zi_2_C2 zi_3_C3 zi_25_c25 zi_48_c48 zi_49_c49 zi_50_c50 _______ ________ ________ _________ _________ _________ _________ zi_1_r1 0 0.020408 0.040816 0.4898 0.95918 0.97959 1 pos = 4 ; key = ar_interp_c_grid ;rown= 1 ,coln= 772572 ar_interp_c_grid :mu= 38.6486 ,sd= 22.3023 ,min= 0.02 ,max= 77.2773 zi_1_C1 zi_2_C2 zi_3_C3 zi_386286_c386286 zi_772570_c772570 zi_772571_c772571 zi_772572_c772572 _______ _______ _______ _________________ _________________ _________________ _________________ zi_1_r1 0.02 0.0201 0.0202 38.649 77.277 77.277 77.277 pos = 5 ; key = ar_interp_coh_grid ;rown= 1 ,coln= 772 ar_interp_coh_grid :mu= 18.6145 ,sd= 22.347 ,min= -20 ,max= 57.2773 zi_1_C1 zi_2_C2 zi_3_C3 zi_386_c386 zi_770_c770 zi_771_c771 zi_772_c772 _______ _______ _______ ___________ ___________ ___________ ___________ zi_1_r1 -20 -19.9 -19.799 18.538 57.077 57.177 57.277 pos = 6 ; key = ar_k_mesha ;rown= 772 ,coln= 1 ar_k_mesha :mu= 0 ,sd= 0 ,min= 0 ,max= 0 zi_1_c1 _______ zi_1_R1 0 zi_2_R2 0 zi_3_R3 0 zi_386_r386 0 zi_770_r770 0 zi_771_r771 0 zi_772_r772 0 pos = 7 ; key = ar_k_mesha_ori ;rown= 35000 ,coln= 1 ar_k_mesha_ori :mu= 17.4893 ,sd= 15.6183 ,min= 0 ,max= 70 zi_1_c1 _______ zi_1_R1 0 zi_2_R2 0 zi_3_R3 0 zi_17500_r17500 34.9 zi_34998_r34998 67.143 zi_34999_r34999 68.571 zi_35000_r35000 70 pos = 8 ; key = ar_stationary ;rown= 1 ,coln= 15 ar_stationary :mu= 0.066667 ,sd= 0.060897 ,min= 0.0027089 ,max= 0.16757 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 _________ _________ ________ _______ _________ _________ _________ zi_1_r1 0.0027089 0.0069499 0.018507 0.16757 0.018507 0.0069499 0.0027089 pos = 9 ; key = ar_w_level ;rown= 1 ,coln= 700 ar_w_level :mu= 14.9786 ,sd= 20.2441 ,min= -20 ,max= 50 zi_1_C1 zi_2_C2 zi_3_C3 zi_350_c350 zi_698_c698 zi_699_c699 zi_700_c700 _______ _______ _______ ___________ ___________ ___________ ___________ zi_1_r1 -20 -19.9 -19.799 14.9 49.799 49.9 50 pos = 10 ; key = ar_w_perc ;rown= 1 ,coln= 50 ar_w_perc :mu= 0.5 ,sd= 0.2975 ,min= 0 ,max= 1 zi_1_C1 zi_2_C2 zi_3_C3 zi_25_c25 zi_48_c48 zi_49_c49 zi_50_c50 _______ ________ ________ _________ _________ _________ _________ zi_1_r1 0 0.020408 0.040816 0.4898 0.95918 0.97959 1 pos = 11 ; key = ar_z ;rown= 1 ,coln= 15 ar_z :mu= 1.1347 ,sd= 0.69878 ,min= 0.34741 ,max= 2.567 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 _______ _______ _______ _______ _________ _________ _________ zi_1_r1 0.34741 0.40076 0.4623 0.94436 1.9291 2.2253 2.567 pos = 12 ; key = it_ameshk_n ; val = 772 pos = 13 ; key = mt_coh_wkb ;rown= 772 ,coln= 15 mt_coh_wkb :mu= 18.6145 ,sd= 22.3335 ,min= -20 ,max= 57.2773 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 _______ _______ _______ _______ _________ _________ _________ zi_1_R1 -20 -20 -20 -20 -20 -20 -20 zi_2_R2 -19.9 -19.9 -19.9 -19.9 -19.9 -19.9 -19.9 zi_3_R3 -19.799 -19.799 -19.799 -19.799 -19.799 -19.799 -19.799 zi_386_r386 18.538 18.538 18.538 18.538 18.538 18.538 18.538 zi_770_r770 57.077 57.077 57.077 57.077 57.077 57.077 57.077 zi_771_r771 57.177 57.177 57.177 57.177 57.177 57.177 57.177 zi_772_r772 57.277 57.277 57.277 57.277 57.277 57.277 57.277 pos = 14 ; key = mt_coh_wkb_ori ;rown= 35000 ,coln= 15 mt_coh_wkb_ori :mu= 16.2108 ,sd= 20.8413 ,min= -21.4564 ,max= 57.2773 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 _______ _______ _______ _______ _________ _________ _________ zi_1_R1 -21.456 -21.456 -21.456 -21.456 -21.456 -21.456 -21.456 zi_2_R2 -21.456 -21.456 -21.456 -21.456 -21.456 -21.456 -21.456 zi_3_R3 -21.456 -21.456 -21.456 -21.456 -21.456 -21.456 -21.456 zi_17500_r17500 11.9 12.091 12.312 14.044 17.582 18.646 19.874 zi_34998_r34998 45.023 45.266 45.546 47.738 52.215 53.562 55.116 zi_34999_r34999 44.785 45.03 45.312 47.52 52.032 53.389 54.955 zi_35000_r35000 44.547 44.793 45.077 47.302 51.848 53.215 54.792 pos = 15 ; key = mt_interp_coh_grid_mesh_w_perc ;rown= 50 ,coln= 772 mt_interp_coh_grid_mesh_w_perc :mu= 18.6145 ,sd= 22.3329 ,min= -20 ,max= 57.2773 zi_1_C1 zi_2_C2 zi_3_C3 zi_386_c386 zi_770_c770 zi_771_c771 zi_772_c772 _______ _______ _______ ___________ ___________ ___________ ___________ zi_1_R1 -20 -19.9 -19.799 18.538 57.077 57.177 57.277 zi_2_R2 -20 -19.9 -19.799 18.538 57.077 57.177 57.277 zi_3_R3 -20 -19.9 -19.799 18.538 57.077 57.177 57.277 zi_25_r25 -20 -19.9 -19.799 18.538 57.077 57.177 57.277 zi_48_r48 -20 -19.9 -19.799 18.538 57.077 57.177 57.277 zi_49_r49 -20 -19.9 -19.799 18.538 57.077 57.177 57.277 zi_50_r50 -20 -19.9 -19.799 18.538 57.077 57.177 57.277 pos = 16 ; key = mt_interp_coh_grid_mesh_z ;rown= 772 ,coln= 15 mt_interp_coh_grid_mesh_z :mu= 18.6145 ,sd= 22.3335 ,min= -20 ,max= 57.2773 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 _______ _______ _______ _______ _________ _________ _________ zi_1_R1 -20 -20 -20 -20 -20 -20 -20 zi_2_R2 -19.9 -19.9 -19.9 -19.9 -19.9 -19.9 -19.9 zi_3_R3 -19.799 -19.799 -19.799 -19.799 -19.799 -19.799 -19.799 zi_386_r386 18.538 18.538 18.538 18.538 18.538 18.538 18.538 zi_770_r770 57.077 57.077 57.077 57.077 57.077 57.077 57.077 zi_771_r771 57.177 57.177 57.177 57.177 57.177 57.177 57.177 zi_772_r772 57.277 57.277 57.277 57.277 57.277 57.277 57.277 pos = 17 ; key = mt_k ;rown= 50 ,coln= 700 mt_k :mu= 17.4893 ,sd= 15.6183 ,min= 0 ,max= 70 zi_1_C1 zi_2_C2 zi_3_C3 zi_350_c350 zi_698_c698 zi_699_c699 zi_700_c700 _______ _________ _________ ___________ ___________ ___________ ___________ zi_1_R1 0 0 0 0 0 0 0 zi_2_R2 0 0.0020467 0.0040933 0.71224 1.4245 1.4265 1.4286 zi_3_R3 0 0.0040933 0.0081867 1.4245 2.849 2.853 2.8571 zi_25_r25 0 0.04912 0.09824 17.094 34.187 34.237 34.286 zi_48_r48 0 0.096193 0.19239 33.475 66.95 67.047 67.143 zi_49_r49 0 0.09824 0.19648 34.187 68.375 68.473 68.571 zi_50_r50 0 0.10029 0.20057 34.9 69.799 69.9 70 pos = 18 ; key = mt_w_by_interp_coh_interp_grid ;rown= 50 ,coln= 772 mt_w_by_interp_coh_interp_grid :mu= -0.69276 ,sd= 17.2418 ,min= -20 ,max= 57.2773 zi_1_C1 zi_2_C2 zi_3_C3 zi_386_c386 zi_770_c770 zi_771_c771 zi_772_c772 _______ _______ _______ ___________ ___________ ___________ ___________ zi_1_R1 -20 -20 -20 -20 -20 -20 -20 zi_2_R2 -20 -19.998 -19.996 -19.214 -18.427 -18.425 -18.423 zi_3_R3 -20 -19.996 -19.992 -18.427 -16.854 -16.85 -16.846 zi_25_r25 -20 -19.951 -19.902 -1.1241 17.752 17.801 17.85 zi_48_r48 -20 -19.904 -19.807 16.965 53.931 54.027 54.123 zi_49_r49 -20 -19.902 -19.803 17.752 55.504 55.602 55.7 zi_50_r50 -20 -19.9 -19.799 18.538 57.077 57.177 57.277 pos = 19 ; key = mt_z_mesh_coh_interp_grid ;rown= 772 ,coln= 15 mt_z_mesh_coh_interp_grid :mu= 1.1347 ,sd= 0.67511 ,min= 0.34741 ,max= 2.567 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 _______ _______ _______ _______ _________ _________ _________ zi_1_R1 0.34741 0.40076 0.4623 0.94436 1.9291 2.2253 2.567 zi_2_R2 0.34741 0.40076 0.4623 0.94436 1.9291 2.2253 2.567 zi_3_R3 0.34741 0.40076 0.4623 0.94436 1.9291 2.2253 2.567 zi_386_r386 0.34741 0.40076 0.4623 0.94436 1.9291 2.2253 2.567 zi_770_r770 0.34741 0.40076 0.4623 0.94436 1.9291 2.2253 2.567 zi_771_r771 0.34741 0.40076 0.4623 0.94436 1.9291 2.2253 2.567 zi_772_r772 0.34741 0.40076 0.4623 0.94436 1.9291 2.2253 2.567 pos = 20 ; key = mt_z_mesh_coh_wkb ;rown= 35000 ,coln= 15 mt_z_mesh_coh_wkb :mu= 1.1347 ,sd= 0.67508 ,min= 0.34741 ,max= 2.567 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 _______ _______ _______ _______ _________ _________ _________ zi_1_R1 0.34741 0.40076 0.4623 0.94436 1.9291 2.2253 2.567 zi_2_R2 0.34741 0.40076 0.4623 0.94436 1.9291 2.2253 2.567 zi_3_R3 0.34741 0.40076 0.4623 0.94436 1.9291 2.2253 2.567 zi_17500_r17500 0.34741 0.40076 0.4623 0.94436 1.9291 2.2253 2.567 zi_34998_r34998 0.34741 0.40076 0.4623 0.94436 1.9291 2.2253 2.567 zi_34999_r34999 0.34741 0.40076 0.4623 0.94436 1.9291 2.2253 2.567 zi_35000_r35000 0.34741 0.40076 0.4623 0.94436 1.9291 2.2253 2.567 pos = 21 ; key = mt_z_trans ;rown= 15 ,coln= 15 mt_z_trans :mu= 0.066667 ,sd= 0.095337 ,min= 0 ,max= 0.27902 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 __________ __________ __________ __________ __________ __________ __________ zi_1_R1 0.26016 0.26831 0.25551 0.00012823 2.7001e-13 1.1102e-15 0 zi_2_R2 0.11232 0.19622 0.2763 0.00098855 1.5289e-11 9.3592e-14 3.3307e-16 zi_3_R3 0.037073 0.10492 0.2185 0.0055558 6.2811e-10 5.7438e-12 3.1863e-14 zi_8_R8 1.7181e-06 4.1008e-05 0.00061112 0.27902 0.00061112 4.1008e-05 1.7181e-06 zi_13_r13 3.1909e-14 5.7438e-12 6.2811e-10 0.0055558 0.2185 0.10492 0.037073 zi_14_r14 3.474e-16 9.3597e-14 1.5289e-11 0.00098855 0.2763 0.19622 0.11232 zi_15_r15 2.7412e-18 1.1057e-15 2.6998e-13 0.00012823 0.25551 0.26831 0.26016 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Matrix in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx rowN colN mean std min max __ ___ _____ __________ ________ ________ _________ _______ ar_a_meshk 1 1 772 1 18.614 22.347 -20 57.277 ar_a_meshk_ori 2 2 35000 1 -2.5107 15.618 -20 50 ar_ak_perc 3 3 1 50 0.5 0.2975 0 1 ar_interp_c_grid 4 4 1 7.7257e+05 38.649 22.302 0.02 77.277 ar_interp_coh_grid 5 5 1 772 18.614 22.347 -20 57.277 ar_k_mesha 6 6 772 1 0 0 0 0 ar_k_mesha_ori 7 7 35000 1 17.489 15.618 0 70 ar_stationary 8 8 1 15 0.066667 0.060897 0.0027089 0.16757 ar_w_level 9 9 1 700 14.979 20.244 -20 50 ar_w_perc 10 10 1 50 0.5 0.2975 0 1 ar_z 11 11 1 15 1.1347 0.69878 0.34741 2.567 mt_coh_wkb 12 13 772 15 18.614 22.334 -20 57.277 mt_coh_wkb_ori 13 14 35000 15 16.211 20.841 -21.456 57.277 mt_interp_coh_grid_mesh_w_perc 14 15 50 772 18.614 22.333 -20 57.277 mt_interp_coh_grid_mesh_z 15 16 772 15 18.614 22.334 -20 57.277 mt_k 16 17 50 700 17.489 15.618 0 70 mt_w_by_interp_coh_interp_grid 17 18 50 772 -0.69276 17.242 -20 57.277 mt_z_mesh_coh_interp_grid 18 19 772 15 1.1347 0.67511 0.34741 2.567 mt_z_mesh_coh_wkb 19 20 35000 15 1.1347 0.67508 0.34741 2.567 mt_z_trans 20 21 15 15 0.066667 0.095337 0 0.27902 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Scalars in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx value _ ___ _____ it_ameshk_n 1 12 772
Display 3 param_map
fft_container_map_display(param_map, it_display_summmat_rowmax, it_display_summmat_colmax);
---------------------------------------- ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Begin: Show all key and value pairs from container CONTAINER NAME: PARAM_MAP ---------------------------------------- Map with properties: Count: 35 KeyType: char ValueType: any xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ---------------------------------------- ---------------------------------------- pos = 1 ; key = bl_default ; val = true pos = 2 ; key = fl_Amean ; val = 1 pos = 3 ; key = fl_alpha ; val = 0.36 pos = 4 ; key = fl_b_bd ; val = -20 pos = 5 ; key = fl_beta ; val = 0.94 pos = 6 ; key = fl_c_min ; val = 0.02 pos = 7 ; key = fl_coh_interp_grid_gap ; val = 0.1 pos = 8 ; key = fl_crra ; val = 1.5 pos = 9 ; key = fl_default_wprime ; val = 0 pos = 10 ; key = fl_delta ; val = 0.08 pos = 11 ; key = fl_k_max ; val = 70 pos = 12 ; key = fl_k_min ; val = 0 pos = 13 ; key = fl_nan_replace ; val = -9999 pos = 14 ; key = fl_r_borr ; val = 0.095 pos = 15 ; key = fl_r_save ; val = 0.025 pos = 16 ; key = fl_tol_dist ; val = 1e-05 pos = 17 ; key = fl_tol_pol ; val = 1e-05 pos = 18 ; key = fl_tol_val ; val = 1e-05 pos = 19 ; key = fl_w ; val = 0.44365 pos = 20 ; key = fl_w_interp_grid_gap ; val = 0.1 pos = 21 ; key = fl_w_max ; val = 50 pos = 22 ; key = fl_w_min ; val = -20 pos = 23 ; key = fl_z_mu ; val = 0 pos = 24 ; key = fl_z_rho ; val = 0.8 pos = 25 ; key = fl_z_sig ; val = 0.2 pos = 26 ; key = it_ak_perc_n ; val = 50 pos = 27 ; key = it_c_interp_grid_gap ; val = 0.0001 pos = 28 ; key = it_maxiter_dist ; val = 1000 pos = 29 ; key = it_maxiter_val ; val = 250 pos = 30 ; key = it_tol_pol_nochange ; val = 25 pos = 31 ; key = it_w_perc_n ; val = 50 pos = 32 ; key = it_z_n ; val = 15 pos = 33 ; key = st_analytical_stationary_type ; val = eigenvector pos = 34 ; key = st_model ; val = ipwkbz pos = 35 ; key = st_v_coh_z_interp_method ; val = method_cell ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Scalars in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx value __ ___ _______ bl_default 1 1 1 fl_Amean 2 2 1 fl_alpha 3 3 0.36 fl_b_bd 4 4 -20 fl_beta 5 5 0.94 fl_c_min 6 6 0.02 fl_coh_interp_grid_gap 7 7 0.1 fl_crra 8 8 1.5 fl_default_wprime 9 9 0 fl_delta 10 10 0.08 fl_k_max 11 11 70 fl_k_min 12 12 0 fl_nan_replace 13 13 -9999 fl_r_borr 14 14 0.095 fl_r_save 15 15 0.025 fl_tol_dist 16 16 1e-05 fl_tol_pol 17 17 1e-05 fl_tol_val 18 18 1e-05 fl_w 19 19 0.44365 fl_w_interp_grid_gap 20 20 0.1 fl_w_max 21 21 50 fl_w_min 22 22 -20 fl_z_mu 23 23 0 fl_z_rho 24 24 0.8 fl_z_sig 25 25 0.2 it_ak_perc_n 26 26 50 it_c_interp_grid_gap 27 27 0.0001 it_maxiter_dist 28 28 1000 it_maxiter_val 29 29 250 it_tol_pol_nochange 30 30 25 it_w_perc_n 31 31 50 it_z_n 32 32 15 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Strings in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx _ ___ st_analytical_stationary_type 1 33 st_model 2 34 st_v_coh_z_interp_method 3 35
Display 4 func_map
fft_container_map_display(func_map, it_display_summmat_rowmax, it_display_summmat_colmax);
---------------------------------------- ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Begin: Show all key and value pairs from container CONTAINER NAME: FUNC_MAP ---------------------------------------- Map with properties: Count: 7 KeyType: char ValueType: any xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ---------------------------------------- ---------------------------------------- pos = 1 ; key = f_coh ; val = @(z,b,k)(f_prod(z,k)+k*(1-fl_delta)+fl_w+b.*(1+fl_r_save).*(b>0)+b.*(1+fl_r_borr).*(b<=0)) pos = 2 ; key = f_cons ; val = @(coh,bprime,kprime)(coh-kprime-bprime) pos = 3 ; key = f_inc ; val = @(z,b,k)(f_prod(z,k)-(fl_delta)*k+fl_w+b.*(fl_r_save).*(b>0)+b.*(fl_r_borr).*(b<=0)) pos = 4 ; key = f_prod ; val = @(z,k)((fl_Amean.*(z)).*(k.^(fl_alpha))) pos = 5 ; key = f_util_crra ; val = @(c)(((c).^(1-fl_crra)-1)./(1-fl_crra)) pos = 6 ; key = f_util_log ; val = @(c)log(c) pos = 7 ; key = f_util_standin ; val = @(z,b,k)f_util_log((f_coh(z,b,k)-fl_b_bd).*((f_coh(z,b,k)-fl_b_bd)>fl_c_min)+fl_c_min.*((f_coh(z,b,k)-fl_b_bd)<=fl_c_min)) ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Scalars in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx xFunction _ ___ _________ f_coh 1 1 1 f_cons 2 2 2 f_inc 3 3 3 f_prod 4 4 4 f_util_crra 5 5 5 f_util_log 6 6 6 f_util_standin 7 7 7
Display 5 result_map
fft_container_map_display(result_map, it_display_summmat_rowmax, it_display_summmat_colmax);
---------------------------------------- ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Begin: Show all key and value pairs from container CONTAINER NAME: RESULT_MAP ---------------------------------------- Map with properties: Count: 14 KeyType: char ValueType: any xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ---------------------------------------- ---------------------------------------- pos = 1 ; key = ar_pol_diff_norm ;rown= 133 ,coln= 1 ar_pol_diff_norm :mu= 58.4991 ,sd= 291.8239 ,min= 0 ,max= 2431.9588 zi_1_c1 _______ zi_1_R1 2150.9 zi_2_R2 2432 zi_3_R3 884.14 zi_67_R67 1.5197 zi_131_r131 0 zi_132_r132 0 zi_133_r133 0 pos = 2 ; key = ar_st_pol_names ; val = cl_mt_coh cl_mt_pol_a cl_mt_pol_k cl_mt_pol_c pos = 3 ; key = ar_val_diff_norm ;rown= 133 ,coln= 1 ar_val_diff_norm :mu= 10.2763 ,sd= 29.2676 ,min= 0.00091964 ,max= 179.6967 zi_1_c1 __________ zi_1_R1 179.7 zi_2_R2 173.52 zi_3_R3 133.72 zi_67_R67 0.082403 zi_131_r131 0.0010401 zi_132_r132 0.000978 zi_133_r133 0.00091964 pos = 4 ; key = cl_mt_coh ;rown= 772 ,coln= 15 cl_mt_coh :mu= 18.6145 ,sd= 22.3335 ,min= -20 ,max= 57.2773 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 _______ _______ _______ _______ _________ _________ _________ zi_1_R1 -20 -20 -20 -20 -20 -20 -20 zi_2_R2 -19.9 -19.9 -19.9 -19.9 -19.9 -19.9 -19.9 zi_3_R3 -19.799 -19.799 -19.799 -19.799 -19.799 -19.799 -19.799 zi_386_r386 18.538 18.538 18.538 18.538 18.538 18.538 18.538 zi_770_r770 57.077 57.077 57.077 57.077 57.077 57.077 57.077 zi_771_r771 57.177 57.177 57.177 57.177 57.177 57.177 57.177 zi_772_r772 57.277 57.277 57.277 57.277 57.277 57.277 57.277 pos = 5 ; key = cl_mt_cons ;rown= 772 ,coln= 15 cl_mt_cons :mu= 2.9005 ,sd= 2.8392 ,min= -20 ,max= 7.8854 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 _______ _______ _______ _______ _________ _________ _________ zi_1_R1 -20 -20 -20 -20 -20 -20 -20 zi_2_R2 -19.9 -19.9 -19.9 -19.9 -19.9 -19.9 -19.9 zi_3_R3 -19.799 -19.799 -19.799 -19.799 -19.799 -19.799 -19.799 zi_386_r386 2.3595 2.3595 3.146 3.146 3.146 3.146 3.9325 zi_770_r770 7.865 7.865 7.865 7.865 7.865 7.865 7.865 zi_771_r771 7.8752 7.8752 7.8752 7.8752 7.8752 7.8752 7.8752 zi_772_r772 7.8854 7.8854 7.8854 7.8854 7.8854 7.8854 7.8854 pos = 6 ; key = cl_mt_pol_a ;rown= 772 ,coln= 15 cl_mt_pol_a :mu= 9.3947 ,sd= 19.047 ,min= -20 ,max= 49.1717 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 ________ ________ _______ _______ _________ _________ _________ zi_1_R1 -0.81633 -0.81633 -1.2245 -2.8571 -6.5306 -7.7551 -9.3878 zi_2_R2 -0.81633 -0.81633 -1.2245 -2.8571 -6.5306 -7.7551 -9.3878 zi_3_R3 -0.81633 -0.81633 -1.2245 -2.8571 -6.5306 -7.7551 -9.3878 zi_386_r386 13.964 13.964 12.503 8.8917 0.22417 0.22417 -0.22526 zi_770_r770 47.799 46.387 46.387 42.149 33.674 31.045 29.437 zi_771_r771 47.887 46.473 46.473 42.23 33.744 32.33 29.449 zi_772_r772 47.976 46.56 46.56 42.311 33.814 32.398 28.24 pos = 7 ; key = cl_mt_pol_c ;rown= 772 ,coln= 15 cl_mt_pol_c :mu= 3.1183 ,sd= 1.581 ,min= 0.02 ,max= 7.8854 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 _______ _______ _______ _______ _________ _________ _________ zi_1_R1 0.02 0.02 0.02 0.02 0.02 0.02 0.02 zi_2_R2 0.02 0.02 0.02 0.02 0.02 0.02 0.02 zi_3_R3 0.02 0.02 0.02 0.02 0.02 0.02 0.02 zi_386_r386 2.3595 2.3595 3.146 3.146 3.146 3.146 3.9325 zi_770_r770 7.865 7.865 7.865 7.865 7.865 7.865 7.865 zi_771_r771 7.8752 7.8752 7.8752 7.8752 7.8752 7.8752 7.8752 zi_772_r772 7.8854 7.8854 7.8854 7.8854 7.8854 7.8854 7.8854 pos = 8 ; key = cl_mt_pol_k ;rown= 772 ,coln= 15 cl_mt_pol_k :mu= 6.3193 ,sd= 5.1683 ,min= 0 ,max= 22.2236 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 _______ _______ _______ _______ _________ _________ _________ zi_1_R1 0.81633 0.81633 1.2245 2.8571 6.5306 7.7551 9.3878 zi_2_R2 0.81633 0.81633 1.2245 2.8571 6.5306 7.7551 9.3878 zi_3_R3 0.81633 0.81633 1.2245 2.8571 6.5306 7.7551 9.3878 zi_386_r386 2.215 2.215 2.8892 6.5006 15.168 15.168 14.831 zi_770_r770 1.4125 2.825 2.825 7.0624 15.537 18.167 19.775 zi_771_r771 1.4143 2.8286 2.8286 7.0716 15.558 16.972 19.853 zi_772_r772 1.4162 2.8323 2.8323 7.0808 15.578 16.994 21.151 pos = 9 ; key = mt_pol_idx ;rown= 772 ,coln= 15 mt_pol_idx :mu= 19318.9589 ,sd= 11146.9408 ,min= 1 ,max= 38595 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 _______ _______ _______ _______ _________ _________ _________ zi_1_R1 1 1 1 1 1 1 1 zi_2_R2 91 91 91 91 91 91 91 zi_3_R3 146 146 146 146 146 146 146 zi_386_r386 19297 19297 19296 19296 19296 19296 19295 zi_770_r770 38495 38495 38495 38495 38495 38495 38495 zi_771_r771 38545 38545 38545 38545 38545 38545 38545 zi_772_r772 38595 38595 38595 38595 38595 38595 38595 pos = 10 ; key = mt_pol_perc_change ;rown= 133 ,coln= 15 mt_pol_perc_change :mu= 0.088391 ,sd= 0.23978 ,min= 0 ,max= 1 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 _______ _______ _________ _________ _________ _________ _________ zi_1_R1 1 1 1 1 1 1 1 zi_2_R2 1 1 1 0.9728 0.90933 0.88601 0.86334 zi_3_R3 0.94948 0.9307 0.9171 0.96373 0.92617 0.90933 0.8899 zi_67_R67 0 0 0.0012953 0.0012953 0 0.0012953 0 zi_131_r131 0 0 0 0 0 0 0 zi_132_r132 0 0 0 0 0 0 0 zi_133_r133 0 0 0 0 0 0 0 pos = 11 ; key = mt_val ;rown= 772 ,coln= 15 mt_val :mu= 8.527 ,sd= 7.1164 ,min= -9.9353 ,max= 16.5979 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 _______ _______ _______ _______ _________ _________ _________ zi_1_R1 -9.9353 -9.6945 -9.4082 -7.4998 -4.9849 -4.4625 -3.9715 zi_2_R2 -9.9353 -9.6945 -9.4082 -7.4998 -4.9849 -4.4625 -3.9715 zi_3_R3 -9.9353 -9.6945 -9.4082 -7.4998 -4.9849 -4.4625 -3.9715 zi_386_r386 10.255 10.358 10.48 11.263 12.344 12.589 12.824 zi_770_r770 15.398 15.441 15.492 15.827 16.343 16.467 16.587 zi_771_r771 15.407 15.45 15.5 15.835 16.35 16.473 16.592 zi_772_r772 15.415 15.458 15.509 15.843 16.357 16.479 16.598 pos = 12 ; key = tb_pol_a ;rown= 100 ,coln= 12 tb_pol_a :mu= 11.4475 ,sd= 29.2851 ,min= -20 ,max= 49.1717 zi_1_C1 zi_2_C2 zi_3_C3 zi_6_C6 zi_10_c10 zi_11_c11 zi_12_c12 ________ ________ _______ _______ _________ _________ _________ zi_1_R1 -0.81633 -0.81633 -1.2245 -2.0408 -6.5306 -7.7551 -9.3878 zi_2_R2 -0.81633 -0.81633 -1.2245 -2.0408 -6.5306 -7.7551 -9.3878 zi_3_R3 -0.81633 -0.81633 -1.2245 -2.0408 -6.5306 -7.7551 -9.3878 zi_50_R50 -17.921 -18.098 -18.276 -19.139 -20 -20 -20 zi_98_R98 47.799 46.387 46.387 44.974 33.674 31.045 29.437 zi_99_R99 47.887 46.473 46.473 45.059 33.744 32.33 29.449 zi_100_r100 47.976 46.56 46.56 45.143 33.814 32.398 28.24 pos = 13 ; key = tb_val ;rown= 100 ,coln= 12 tb_val :mu= 4.8055 ,sd= 11.0397 ,min= -9.9353 ,max= 16.5979 zi_1_C1 zi_2_C2 zi_3_C3 zi_6_C6 zi_10_c10 zi_11_c11 zi_12_c12 _______ _______ _______ _______ _________ _________ _________ zi_1_R1 -9.9353 -9.6945 -9.4082 -8.3542 -4.9849 -4.4625 -3.9715 zi_2_R2 -9.9353 -9.6945 -9.4082 -8.3542 -4.9849 -4.4625 -3.9715 zi_3_R3 -9.9353 -9.6945 -9.4082 -8.3542 -4.9849 -4.4625 -3.9715 zi_50_R50 -6.9568 -6.7739 -6.565 -5.8328 -3.4912 -3.0705 -2.6316 zi_98_R98 15.398 15.441 15.492 15.675 16.343 16.467 16.587 zi_99_R99 15.407 15.45 15.5 15.683 16.35 16.473 16.592 zi_100_r100 15.415 15.458 15.509 15.691 16.357 16.479 16.598 pos = 14 ; key = tb_valpol_alliter ;rown= 100 ,coln= 17 tb_valpol_alliter :mu= 5.4286 ,sd= 83.2435 ,min= 0 ,max= 2431.9588 zi_1_C1 zi_2_C2 zi_3_C3 zi_9_C9 zi_15_c15 zi_16_c16 zi_17_c17 __________ _______ _______ _______ _________ _________ _________ zi_1_R1 179.7 2150.9 1 1 1 1 1 zi_2_R2 173.52 2432 1 0.97992 0.90933 0.88601 0.86334 zi_3_R3 133.72 884.14 0.94948 0.93523 0.92617 0.90933 0.8899 zi_50_R50 0.52564 6.419 0 0 0 0.007772 0.0064767 zi_98_R98 0.0010401 0 0 0 0 0 0 zi_99_R99 0.000978 0 0 0 0 0 0 zi_100_r100 0.00091964 0 0 0 0 0 0 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Matrix in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx rowN colN mean std min max __ ___ ____ ____ ________ _______ __________ ______ ar_pol_diff_norm 1 1 133 1 58.499 291.82 0 2432 ar_val_diff_norm 2 3 133 1 10.276 29.268 0.00091964 179.7 cl_mt_coh 3 4 772 15 18.614 22.334 -20 57.277 cl_mt_cons 4 5 772 15 2.9005 2.8392 -20 7.8854 cl_mt_pol_a 5 6 772 15 9.3947 19.047 -20 49.172 cl_mt_pol_c 6 7 772 15 3.1183 1.581 0.02 7.8854 cl_mt_pol_k 7 8 772 15 6.3193 5.1683 0 22.224 mt_pol_idx 8 9 772 15 19319 11147 1 38595 mt_pol_perc_change 9 10 133 15 0.088391 0.23978 0 1 mt_val 10 11 772 15 8.527 7.1164 -9.9353 16.598 tb_pol_a 11 12 100 12 11.448 29.285 -20 49.172 tb_val 12 13 100 12 4.8055 11.04 -9.9353 16.598 tb_valpol_alliter 13 14 100 17 5.4286 83.244 0 2432 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Strings in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx _ ___ ar_st_pol_names 1 2
end
end
ans = Map with properties: Count: 14 KeyType: char ValueType: any