Risky + Safe Asset (Saving Only) Interpolated-Percentage (Optimized-Vectorized)
back to Fan's Dynamic Assets Repository Table of Content.
Contents
- FF_IPWKZ_VF_VECSV solve infinite horizon exo shock + endo asset problem
- Default
- Parse Parameters 1
- Parse Parameters 2
- Initialize Output Matrixes
- Initialize Convergence Conditions
- Pre-calculate u(c)
- Iterate Value Function
- Interpolate (1) reacahble v(coh(k(w,z),b(w,z),z),z) given v(coh, z)
- Solve Second Stage Problem k*(w,z)
- Solve First Stage Problem w*(z) given k*(w,z)
- A. Interpolate FULL to get k*(w_perc, z), b*(k,w) based on k*(w_level, z)
- B. Calculate UPDATE u(c): u(c(coh_level, w_perc)) given k*_interp, b*_interp
- C. Interpolate FULL EV(k*(coh_level, w_perc, z), w - b*|z) based on EV(k*(w_level, z))
- D. Compute FULL U(coh_level, w_perc, z) over all w_perc
- E. Optimize Over Choices: max_{w_perc} U(coh_level, w_perc, z)
- F. Store Results
- Check Tolerance and Continuation
- Process Optimal Choices
- Display Various Containers
- Display 1 support_map
- Display 2 armt_map
- Display 3 param_map
- Display 4 func_map
- Display 5 result_map
function result_map = ff_ipwkz_vf_vecsv(varargin)
FF_IPWKZ_VF_VECSV solve infinite horizon exo shock + endo asset problem
This program solves the infinite horizon dynamic savings and risky capital asset problem with some ar1 shock. This is the two step solution with interpolation and with percentage asset grids version of ff_iwkz_vf_vecsv. See that file for more descriptions. This is the optimized-vectorized version of the program.
@param param_map container parameter container
@param support_map container support container
@param armt_map container container with states, choices and shocks grids that are inputs for grid based solution algorithm
@param func_map container container with function handles for consumption cash-on-hand etc.
@return result_map container contains policy function matrix, value function matrix, iteration results, and policy function, value function and iteration results tables.
keys included in result_map:
- mt_val matrix states_n by shock_n matrix of converged value function grid
- mt_pol_a matrix states_n by shock_n matrix of converged policy function grid
- ar_val_diff_norm array if bl_post = true it_iter_last by 1 val function difference between iteration
- ar_pol_diff_norm array if bl_post = true it_iter_last by 1 policy function difference between iterations
- mt_pol_perc_change matrix if bl_post = true it_iter_last by shock_n the proportion of grid points at which policy function changed between current and last iteration for each element of shock
@example
@include
Default
- it_param_set = 1: quick test
- it_param_set = 2: benchmark run
- it_param_set = 3: benchmark profile
- it_param_set = 4: press publish button
it_param_set = 4; [param_map, support_map] = ffs_ipwkz_set_default_param(it_param_set); % parameters can be set inside ffs_ipwkz_set_default_param or updated here % param_map('it_w_perc_n') = 50; % param_map('it_ak_perc_n') = param_map('it_w_perc_n'); % param_map('it_z_n') = 15; % param_map('fl_coh_interp_grid_gap') = 0.025; % param_map('it_c_interp_grid_gap') = 0.001; % param_map('fl_w_interp_grid_gap') = 0.25; % param_map('it_w_perc_n') = 100; % param_map('it_ak_perc_n') = param_map('it_w_perc_n'); % param_map('it_z_n') = 11; % param_map('fl_coh_interp_grid_gap') = 0.1; % param_map('it_c_interp_grid_gap') = 10^-4; % param_map('fl_w_interp_grid_gap') = 0.1; % get armt and func map [armt_map, func_map] = ffs_ipwkz_get_funcgrid(param_map, support_map); % 1 for override default_params = {param_map support_map armt_map func_map};
Parse Parameters 1
% if varargin only has param_map and support_map, params_len = length(varargin); [default_params{1:params_len}] = varargin{:}; param_map = [param_map; default_params{1}]; support_map = [support_map; default_params{2}]; if params_len >= 1 && params_len <= 2 % If override param_map, re-generate armt and func if they are not % provided [armt_map, func_map] = ffs_ipwkz_get_funcgrid(param_map, support_map); else % Override all armt_map = [armt_map; default_params{3}]; func_map = [func_map; default_params{4}]; end % append function name st_func_name = 'ff_ipwkz_vf_vecsv'; support_map('st_profile_name_main') = [st_func_name support_map('st_profile_name_main')]; support_map('st_mat_name_main') = [st_func_name support_map('st_mat_name_main')]; support_map('st_img_name_main') = [st_func_name support_map('st_img_name_main')];
Parse Parameters 2
% armt_map params_group = values(armt_map, {'ar_w_perc', 'ar_w_level', 'ar_z'}); [ar_w_perc, ar_w_level, ar_z] = params_group{:}; params_group = values(armt_map, {'ar_interp_c_grid', 'ar_interp_coh_grid', ... 'mt_interp_coh_grid_mesh_z', 'mt_z_mesh_coh_interp_grid',... 'mt_interp_coh_grid_mesh_w_perc', ... 'mt_w_by_interp_coh_interp_grid'}); [ar_interp_c_grid, ar_interp_coh_grid, ... mt_interp_coh_grid_mesh_z, mt_z_mesh_coh_interp_grid, ... mt_interp_coh_grid_mesh_w_perc,... mt_w_by_interp_coh_interp_grid] = params_group{:}; params_group = values(armt_map, {'mt_coh_wkb', 'mt_z_mesh_coh_wkb'}); [mt_coh_wkb, mt_z_mesh_coh_wkb] = params_group{:}; % func_map params_group = values(func_map, {'f_util_log', 'f_util_crra', 'f_cons'}); [f_util_log, f_util_crra, f_cons] = params_group{:}; % param_map params_group = values(param_map, {'it_z_n', 'fl_crra', 'fl_beta', 'fl_c_min'}); [it_z_n, fl_crra, fl_beta, fl_c_min] = params_group{:}; params_group = values(param_map, {'it_maxiter_val', 'fl_tol_val', 'fl_tol_pol', 'it_tol_pol_nochange'}); [it_maxiter_val, fl_tol_val, fl_tol_pol, it_tol_pol_nochange] = params_group{:}; % support_map params_group = values(support_map, {'bl_profile', 'st_profile_path', ... 'st_profile_prefix', 'st_profile_name_main', 'st_profile_suffix',... 'bl_time', 'bl_display_defparam', 'bl_graph_evf', 'bl_display', 'it_display_every', 'bl_post'}); [bl_profile, st_profile_path, ... st_profile_prefix, st_profile_name_main, st_profile_suffix, ... bl_time, bl_display_defparam, bl_graph_evf, bl_display, it_display_every, bl_post] = params_group{:}; params_group = values(support_map, {'it_display_summmat_rowmax', 'it_display_summmat_colmax'}); [it_display_summmat_rowmax, it_display_summmat_colmax] = params_group{:};
Initialize Output Matrixes
mt_val_cur = zeros(length(ar_interp_coh_grid),length(ar_z)); mt_val = mt_val_cur - 1; mt_pol_a = zeros(length(ar_interp_coh_grid),length(ar_z)); mt_pol_a_cur = mt_pol_a - 1; mt_pol_k = zeros(length(ar_interp_coh_grid),length(ar_z)); mt_pol_k_cur = mt_pol_k - 1; mt_pol_idx = zeros(length(ar_interp_coh_grid),length(ar_z)); % We did not need these in ff_oz_vf or ff_oz_vf_vec % see % <https://fanwangecon.github.io/M4Econ/support/speed/partupdate/fs_u_c_partrepeat_main.html % fs_u_c_partrepeat_main> for why store using cells. cl_u_c_store = cell([it_z_n, 1]); cl_w_kstar_interp_z = cell([it_z_n, 1]); for it_z_i = 1:length(ar_z) cl_w_kstar_interp_z{it_z_i} = zeros([length(ar_w_perc), length(ar_interp_coh_grid)]) - 1; end
Initialize Convergence Conditions
bl_vfi_continue = true; it_iter = 0; ar_val_diff_norm = zeros([it_maxiter_val, 1]); ar_pol_diff_norm = zeros([it_maxiter_val, 1]); mt_pol_perc_change = zeros([it_maxiter_val, it_z_n]);
Pre-calculate u(c)
Interpolation, see fs_u_c_partrepeat_main for why interpolate over u(c)
% Evaluate if (fl_crra == 1) ar_interp_u_of_c_grid = f_util_log(ar_interp_c_grid); fl_u_neg_c = f_util_log(fl_c_min); else ar_interp_u_of_c_grid = f_util_crra(ar_interp_c_grid); fl_u_neg_c = f_util_crra(fl_c_min); end ar_interp_u_of_c_grid(ar_interp_c_grid <= fl_c_min) = fl_u_neg_c; % Get Interpolant f_grid_interpolant_spln = griddedInterpolant(ar_interp_c_grid, ar_interp_u_of_c_grid, 'spline', 'nearest');
Iterate Value Function
Loop solution with 4 nested loops
- loop 1: over exogenous states
- loop 2: over endogenous states
- loop 3: over choices
- loop 4: add future utility, integration--loop over future shocks
% Start Profile if (bl_profile) close all; profile off; profile on; end % Start Timer if (bl_time) tic; end % Value Function Iteration while bl_vfi_continue
it_iter = it_iter + 1;
Interpolate (1) reacahble v(coh(k(w,z),b(w,z),z),z) given v(coh, z)
v(coh,z) solved on ar_interp_coh_grid, ar_z grids, see ffs_ipwkz_get_funcgrid.m. Generate interpolant based on that, Then interpolate for the coh reachable levels given the k(w,z) percentage choice grids in the second stage of the problem
% Generate Interpolant for v(coh,z) f_grid_interpolant_value = griddedInterpolant(... mt_z_mesh_coh_interp_grid', mt_interp_coh_grid_mesh_z', mt_val_cur', 'linear', 'nearest'); % Interpolate for v(coh(k(w,z),b(w,z),z),z) mt_val_wkb_interpolated = f_grid_interpolant_value(mt_z_mesh_coh_wkb, mt_coh_wkb);
Solve Second Stage Problem k*(w,z)
This is the key difference between this function and ffs_akz_set_functions which solves the two stages jointly Interpolation first, because solution coh grid is not the same as all points reachable by k and b choices given w.
support_map('bl_graph_evf') = false; if (it_iter == (it_maxiter_val + 1)) support_map('bl_graph_evf') = bl_graph_evf; end [mt_ev_condi_z_max, ~, mt_ev_condi_z_max_kp, ~] = ... ff_ipwkz_evf(mt_val_wkb_interpolated, param_map, support_map, armt_map);
Solve First Stage Problem w*(z) given k*(w,z)
% loop 1: over exogenous states for it_z_i = 1:length(ar_z)
A. Interpolate FULL to get k*(w_perc, z), b*(k,w) based on k*(w_level, z)
Generate interpolant for (2) k*(ar_w_perc) from k*(ar_w_level,z) There are two w=k'+b' arrays. ar_w_level is the level even grid based on which we solve the 2nd stage problem in ff_ipwkz_evf.m. Here for each coh level, we have a different vector of w levels, but the same vector of percentage ws. So we need to interpolate to get the optimal k* and b* choices at each percentage level of w.
f_interpolante_w_level_kstar_z = griddedInterpolant(ar_w_level, mt_ev_condi_z_max_kp(:, it_z_i)', 'linear', 'nearest'); % Interpolate (2), shift from w_level to w_perc mt_w_kstar_interp_z = f_interpolante_w_level_kstar_z(mt_w_by_interp_coh_interp_grid); mt_w_astar_interp_z = mt_w_by_interp_coh_interp_grid - mt_w_kstar_interp_z; % changes in w_perc kstar choices mt_w_kstar_diff_idx = (cl_w_kstar_interp_z{it_z_i} ~= mt_w_kstar_interp_z);
B. Calculate UPDATE u(c): u(c(coh_level, w_perc)) given k*_interp, b*_interp
Note that compared to ffs_akz_set_functions the mt_c here is much smaller the same number of columns (states) as in the ffs_akz_set_functions file, but the number of rows equal to ar_w length.
ar_c = f_cons(mt_interp_coh_grid_mesh_w_perc(mt_w_kstar_diff_idx), ... mt_w_astar_interp_z(mt_w_kstar_diff_idx), ... mt_w_kstar_interp_z(mt_w_kstar_diff_idx)); % EVAL current utility: N by N, f_util defined earlier ar_utility_update = f_grid_interpolant_spln(ar_c); % Update Storage if (it_iter == 1) cl_u_c_store{it_z_i} = reshape(ar_utility_update, [length(ar_w_perc), length(ar_interp_coh_grid)]); else cl_u_c_store{it_z_i}(mt_w_kstar_diff_idx) = ar_utility_update; end cl_w_kstar_interp_z{it_z_i} = mt_w_kstar_interp_z;
C. Interpolate FULL EV(k*(coh_level, w_perc, z), w - b*|z) based on EV(k*(w_level, z))
Generate Interpolant for (3) EV(k*(ar_w_perc),Z)
f_interpolante_ev_condi_z_max_z = griddedInterpolant(ar_w_level, mt_ev_condi_z_max(:, it_z_i)', 'linear', 'nearest'); % Interpolate (3), EVAL add on future utility, N by N + N by N mt_ev_condi_z_max_interp_z = f_interpolante_ev_condi_z_max_z(mt_w_by_interp_coh_interp_grid);
D. Compute FULL U(coh_level, w_perc, z) over all w_perc
mt_utility = cl_u_c_store{it_z_i} + fl_beta*mt_ev_condi_z_max_interp_z; % percentage algorithm does not have invalid (check to make sure % min percent is not 0 in ffs_ipwkz_get_funcgrid.m) % mt_utility = mt_utility.*(~mt_it_c_valid_idx) + fl_u_neg_c*(mt_it_c_valid_idx);
E. Optimize Over Choices: max_{w_perc} U(coh_level, w_perc, z)
Optimization: remember matlab is column major, rows must be choices, columns must be states COLUMN-MAJOR
[ar_opti_val1_z, ar_opti_idx_z] = max(mt_utility);
% Generate Linear Opti Index
[it_choies_n, it_states_n] = size(mt_utility);
ar_add_grid = linspace(0, it_choies_n*(it_states_n-1), it_states_n);
ar_opti_linear_idx_z = ar_opti_idx_z + ar_add_grid;
F. Store Results
mt_val(:,it_z_i) = ar_opti_val1_z; mt_pol_a(:,it_z_i) = mt_w_astar_interp_z(ar_opti_linear_idx_z); mt_pol_k(:,it_z_i) = mt_w_kstar_interp_z(ar_opti_linear_idx_z); if (it_iter == (it_maxiter_val + 1)) mt_pol_idx(:,it_z_i) = ar_opti_linear_idx_z; end
end
Check Tolerance and Continuation
% Difference across iterations ar_val_diff_norm(it_iter) = norm(mt_val - mt_val_cur); ar_pol_diff_norm(it_iter) = norm(mt_pol_a - mt_pol_a_cur) + norm(mt_pol_k - mt_pol_k_cur); ar_pol_a_perc_change = sum((mt_pol_a ~= mt_pol_a_cur))/(length(ar_interp_coh_grid)); ar_pol_k_perc_change = sum((mt_pol_k ~= mt_pol_k_cur))/(length(ar_interp_coh_grid)); mt_pol_perc_change(it_iter, :) = mean([ar_pol_a_perc_change;ar_pol_k_perc_change]); % Update mt_val_cur = mt_val; mt_pol_a_cur = mt_pol_a; mt_pol_k_cur = mt_pol_k; % Print Iteration Results if (bl_display && (rem(it_iter, it_display_every)==0)) fprintf('VAL it_iter:%d, fl_diff:%d, fl_diff_pol:%d\n', ... it_iter, ar_val_diff_norm(it_iter), ar_pol_diff_norm(it_iter)); tb_valpol_iter = array2table([mean(mt_val_cur,1);... mean(mt_pol_a_cur,1); ... mean(mt_pol_k_cur,1); ... mt_val_cur(length(ar_interp_coh_grid),:); ... mt_pol_a_cur(length(ar_interp_coh_grid),:); ... mt_pol_k_cur(length(ar_interp_coh_grid),:)]); tb_valpol_iter.Properties.VariableNames = strcat('z', string((1:size(mt_val_cur,2)))); tb_valpol_iter.Properties.RowNames = {'mval', 'map', 'mak', 'Hval', 'Hap', 'Hak'}; disp('mval = mean(mt_val_cur,1), average value over a') disp('map = mean(mt_pol_a_cur,1), average choice over a') disp('mkp = mean(mt_pol_k_cur,1), average choice over k') disp('Hval = mt_val_cur(it_ameshk_n,:), highest a state val') disp('Hap = mt_pol_a_cur(it_ameshk_n,:), highest a state choice') disp('mak = mt_pol_k_cur(it_ameshk_n,:), highest k state choice') disp(tb_valpol_iter); end % Continuation Conditions: % 1. if value function convergence criteria reached % 2. if policy function variation over iterations is less than % threshold if (it_iter == (it_maxiter_val + 1)) bl_vfi_continue = false; elseif ((it_iter == it_maxiter_val) || ... (ar_val_diff_norm(it_iter) < fl_tol_val) || ... (sum(ar_pol_diff_norm(max(1, it_iter-it_tol_pol_nochange):it_iter)) < fl_tol_pol)) % Fix to max, run again to save results if needed it_iter_last = it_iter; it_iter = it_maxiter_val; end
end % End Timer if (bl_time) toc; end % End Profile if (bl_profile) profile off profile viewer st_file_name = [st_profile_prefix st_profile_name_main st_profile_suffix]; profsave(profile('info'), strcat(st_profile_path, st_file_name)); end
Process Optimal Choices
result_map = containers.Map('KeyType','char', 'ValueType','any'); result_map('mt_val') = mt_val; result_map('mt_pol_idx') = mt_pol_idx; result_map('cl_mt_coh') = {mt_interp_coh_grid_mesh_z, zeros(1)}; result_map('cl_mt_pol_a') = {mt_pol_a, zeros(1)}; result_map('cl_mt_pol_k') = {mt_pol_k, zeros(1)}; result_map('cl_mt_pol_c') = {f_cons(mt_interp_coh_grid_mesh_z, mt_pol_a, mt_pol_k), zeros(1)}; result_map('cl_mt_val') = {mt_val, zeros(1)}; result_map('ar_st_pol_names') = ["cl_mt_val", "cl_mt_coh", "cl_mt_pol_a", "cl_mt_pol_k", "cl_mt_pol_c"]; if (bl_post) result_map('ar_val_diff_norm') = ar_val_diff_norm(1:it_iter_last); result_map('ar_pol_diff_norm') = ar_pol_diff_norm(1:it_iter_last); result_map('mt_pol_perc_change') = mt_pol_perc_change(1:it_iter_last, :); % graphing based on coh_wkb, but that does not match optimal choice % matrixes for graphs. armt_map('mt_coh_wkb') = mt_interp_coh_grid_mesh_z; armt_map('it_ameshk_n') = length(ar_interp_coh_grid); armt_map('ar_a_meshk') = mt_interp_coh_grid_mesh_z(:,1); armt_map('ar_k_mesha') = zeros(size(mt_interp_coh_grid_mesh_z(:,1)) + 0); result_map = ff_akz_vf_post(param_map, support_map, armt_map, func_map, result_map); end
valgap = norm(mt_val - mt_val_cur): value function difference across iterations polgap = norm(mt_pol_a - mt_pol_a_cur): policy function difference across iterations z1 = z1 perc change: (sum((mt_pol_a ~= mt_pol_a_cur))+sum((mt_pol_k ~= mt_pol_k_cur)))/(2*it_ameshk_n):percentage of state space points conditional on shock where the policy function is changing across iterations valgap polgap z1 z2 z3 z4 z5 z6 z7 z8 z9 z10 z11 z12 z13 z14 z15 ________ _______ _________ _________ _________ _________ _________ _________ _________ _________ _________ _________ _________ _________ _________ _________ _________ iter=1 142.93 187.28 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 iter=2 110.44 1633.1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 iter=3 92.187 549.07 0.99648 0.99648 0.99648 0.9956 0.99472 0.9956 0.99384 0.99384 0.9956 0.98856 0.98856 0.9868 0.99032 0.99472 0.99472 iter=4 79.058 269.06 0.99472 0.99472 0.99296 0.99472 0.99472 0.99384 0.99472 0.99296 0.99208 0.98856 0.98768 0.9868 0.98504 0.9868 0.9868 iter=5 68.865 156.72 0.99296 0.99472 0.99472 0.99472 0.99296 0.99032 0.99296 0.99296 0.98944 0.9868 0.99472 0.98856 0.98592 0.98151 0.98415 iter=6 60.626 111.18 0.98944 0.98239 0.98944 0.98592 0.98768 0.9868 0.98944 0.98592 0.98592 0.98944 0.98504 0.98151 0.98592 0.98415 0.98063 iter=7 53.789 75.6 0.94014 0.93838 0.9419 0.93662 0.94718 0.94102 0.94718 0.95599 0.95335 0.96127 0.96479 0.96831 0.96127 0.96567 0.97183 iter=8 48.015 57.429 0.72007 0.72359 0.69542 0.69366 0.67958 0.67782 0.6743 0.69718 0.77817 0.78433 0.78697 0.78169 0.77025 0.78081 0.81778 iter=9 43.078 43.803 0.41549 0.42254 0.45246 0.48592 0.51408 0.57042 0.62148 0.64261 0.62412 0.60035 0.58099 0.55634 0.58627 0.63908 0.68662 iter=10 38.799 42.944 0.53169 0.53521 0.51056 0.48768 0.47359 0.4419 0.41549 0.38908 0.3662 0.40581 0.45687 0.51761 0.53873 0.53081 0.49296 iter=11 35.069 30.095 0.25352 0.27289 0.29401 0.29754 0.31514 0.35035 0.37412 0.41725 0.44542 0.39437 0.36268 0.34507 0.3838 0.40669 0.46127 iter=12 31.784 26.42 0.29401 0.27993 0.25704 0.22183 0.19542 0.22535 0.22887 0.2588 0.28345 0.29577 0.34507 0.37148 0.35035 0.32658 0.3257 iter=13 28.889 28.085 0.24824 0.27641 0.27465 0.29401 0.30634 0.26937 0.23768 0.19542 0.19366 0.21479 0.23856 0.26585 0.29754 0.34067 0.34507 iter=14 26.317 20.627 0.16725 0.17254 0.18662 0.19366 0.19894 0.22535 0.22535 0.2588 0.26761 0.22183 0.17254 0.19366 0.23151 0.25352 0.27113 iter=15 24.02 15.758 0.10915 0.11268 0.13732 0.1162 0.14261 0.14965 0.16901 0.18926 0.20599 0.20775 0.25704 0.21127 0.16725 0.18662 0.22271 iter=16 21.959 15.824 0.073944 0.088028 0.089789 0.096831 0.11092 0.125 0.13028 0.13556 0.14965 0.17077 0.18134 0.21215 0.21831 0.18486 0.17165 iter=17 20.106 15.643 0.12852 0.12324 0.089789 0.068662 0.09331 0.077465 0.09507 0.091549 0.11268 0.12148 0.15317 0.15581 0.17958 0.19894 0.1919 iter=18 18.44 16.24 0.11972 0.12148 0.13028 0.11796 0.10387 0.065141 0.073944 0.088028 0.091549 0.096831 0.11972 0.12852 0.12852 0.17254 0.17077 iter=19 16.935 13.783 0.096831 0.089789 0.10035 0.10035 0.11796 0.10387 0.091549 0.06338 0.070423 0.079225 0.096831 0.12148 0.1338 0.13204 0.13028 iter=20 15.573 12.853 0.075704 0.077465 0.073944 0.079225 0.10035 0.10387 0.10035 0.10211 0.068662 0.075704 0.079225 0.084507 0.10035 0.10035 0.11972 iter=21 14.336 11.815 0.056338 0.051056 0.06162 0.058099 0.065141 0.077465 0.075704 0.091549 0.10563 0.065141 0.072183 0.084507 0.077465 0.09507 0.096831 iter=22 13.211 9.0758 0.038732 0.040493 0.047535 0.054577 0.059859 0.065141 0.06162 0.073944 0.079225 0.091549 0.073063 0.065141 0.053697 0.072183 0.075704 iter=23 12.187 9.5212 0.033451 0.042254 0.02993 0.044014 0.052817 0.047535 0.065141 0.06162 0.068662 0.077465 0.080106 0.065141 0.058099 0.073944 0.072183 iter=24 11.252 9.2509 0.024648 0.033451 0.03169 0.035211 0.033451 0.042254 0.044014 0.047535 0.056338 0.065141 0.06162 0.070423 0.054577 0.054577 0.070423 iter=25 10.399 9.5528 0.022887 0.026408 0.022887 0.022887 0.024648 0.02993 0.040493 0.045775 0.042254 0.047535 0.056338 0.066901 0.066901 0.042254 0.044894 iter=26 9.6192 8.091 0.017606 0.022887 0.024648 0.024648 0.022887 0.026408 0.024648 0.03169 0.050176 0.045775 0.052817 0.06338 0.058099 0.047535 0.036092 iter=27 8.9053 7.4769 0.015845 0.019366 0.015845 0.017606 0.022887 0.017606 0.02993 0.024648 0.038732 0.047535 0.036972 0.038732 0.054577 0.045775 0.047535 iter=28 8.2511 8.2732 0.012324 0.015845 0.015845 0.012324 0.024648 0.024648 0.017606 0.021127 0.021127 0.035211 0.033451 0.040493 0.054577 0.058099 0.045775 iter=29 7.651 6.7554 0.010563 0.0088028 0.010563 0.015845 0.012324 0.015845 0.019366 0.017606 0.024648 0.021127 0.028169 0.049296 0.042254 0.040493 0.047535 iter=30 7.0997 7.2493 0.012324 0.010563 0.0088028 0.012324 0.0088028 0.014085 0.021127 0.015845 0.014085 0.026408 0.036972 0.026408 0.042254 0.042254 0.045775 iter=31 6.5928 7.176 0.0052817 0.0070423 0.0070423 0.0088028 0.012324 0.010563 0.0088028 0.014085 0.017606 0.021127 0.015845 0.028169 0.022887 0.02993 0.045775 iter=32 6.1261 6.5528 0.0035211 0.0052817 0.0070423 0.0088028 0.0070423 0.014085 0.014085 0.024648 0.019366 0.021127 0.022887 0.028169 0.02993 0.035211 0.02993 iter=33 5.6961 5.1113 0.0052817 0.0035211 0.0070423 0.0070423 0.012324 0.0070423 0.0088028 0.0088028 0.014085 0.024648 0.017606 0.021127 0.026408 0.035211 0.035211 iter=34 5.2996 5.6949 0.0052817 0.0035211 0.0052817 0.0052817 0.014085 0.0052817 0.0035211 0.0070423 0.014085 0.0088028 0.014085 0.014085 0.021127 0.022887 0.028169 iter=35 4.9336 4.4316 0.010563 0.0035211 0.0017606 0.0035211 0.0035211 0.0070423 0.0070423 0.012324 0.014085 0.022887 0.0088028 0.019366 0.019366 0.015845 0.012324 iter=36 4.5955 4.7238 0.0017606 0.0035211 0.0017606 0.0052817 0.0052817 0.0035211 0.0070423 0.0052817 0.015845 0.0070423 0.012324 0.012324 0.019366 0.028169 0.028169 iter=37 4.2831 3.116 0.0052817 0.0070423 0.0052817 0.0017606 0.0035211 0.0035211 0.0017606 0.0052817 0.015845 0.0070423 0.014085 0.0070423 0.015845 0.028169 0.015845 iter=38 3.9939 3.4496 0.0052817 0.0035211 0.0017606 0.0052817 0.0035211 0.0088028 0.0035211 0.0035211 0.0052817 0.015845 0.0070423 0.0088028 0.012324 0.012324 0.012324 iter=39 3.7263 4.186 0.0017606 0.0017606 0.0035211 0.0017606 0.0017606 0.0070423 0.0035211 0.0035211 0.0017606 0.0070423 0.0070423 0.012324 0.019366 0.0070423 0.019366 iter=40 3.4783 3.1283 0.0035211 0.0017606 0.0052817 0.0017606 0.0052817 0.0017606 0.0052817 0.0035211 0.0088028 0.0070423 0.0052817 0.0070423 0.0088028 0.0088028 0.0088028 iter=41 3.2483 3.8575 0 0.0035211 0.0017606 0 0.0017606 0.0035211 0.0017606 0.0070423 0.0088028 0.0017606 0.0052817 0.0070423 0.014085 0.015845 0.010563 iter=42 3.0349 2.5945 0 0 0.0017606 0.0052817 0.0017606 0.0017606 0.0035211 0.0017606 0 0.0070423 0.0035211 0.0070423 0.0035211 0.0052817 0.010563 iter=43 2.8368 2.3457 0.0017606 0.0035211 0 0.0017606 0.0017606 0 0 0 0.0017606 0.0017606 0.0017606 0.012324 0.0052817 0.0088028 0.0035211 iter=44 2.6527 2.6964 0.0035211 0.0017606 0 0.0017606 0 0.0017606 0.0017606 0.0017606 0.0035211 0.0017606 0.0070423 0.0035211 0.0052817 0.0088028 0.012324 iter=45 2.4815 2.0694 0.0017606 0 0.0017606 0.0017606 0 0 0.0052817 0.0017606 0 0.0017606 0.0035211 0.0035211 0.0088028 0.0070423 0.0070423 iter=46 2.3222 2.0611 0.0035211 0 0 0 0.0017606 0.0017606 0.0017606 0.0035211 0.0017606 0.0017606 0.0017606 0 0.0070423 0.0052817 0.0035211 iter=47 2.1738 1.8933 0.0017606 0 0 0 0 0.0017606 0.0035211 0 0.0052817 0.0017606 0.0070423 0.0017606 0.0017606 0.0035211 0.0035211 iter=48 2.0356 1.9616 0.0035211 0 0 0.0017606 0 0.0035211 0 0 0 0 0.0017606 0.0035211 0.0017606 0.0052817 0.0088028 iter=49 1.9068 2.0207 0.0017606 0.0017606 0 0.0017606 0 0 0 0.0035211 0 0.0017606 0.0017606 0 0.0017606 0.0035211 0.0088028 iter=50 1.7866 2.0618 0 0 0 0 0 0 0.0052817 0 0.0017606 0 0.0017606 0.0017606 0.0035211 0.0035211 0.0035211 iter=55 1.2949 1.4372 0 0.0017606 0 0 0 0 0 0.0017606 0 0 0 0.0017606 0.0017606 0 0.0017606 iter=56 1.2149 1.9099 0.0017606 0 0.0017606 0 0.0017606 0 0 0 0.0017606 0 0 0.0017606 0 0.0017606 0.0070423 iter=57 1.14 2.6518 0.0017606 0 0 0 0 0 0 0.0035211 0.0017606 0.0017606 0.0017606 0 0 0.0017606 0.0088028 iter=58 1.0699 1.1249 0 0 0 0 0 0 0.0017606 0 0 0 0.0017606 0 0 0 0.0017606 iter=59 1.0043 1.1865 0 0.0017606 0 0 0 0 0.0017606 0 0 0 0 0 0 0 0 iter=60 0.94278 0.91343 0 0 0 0 0 0 0 0 0 0 0 0 0.0017606 0 0 iter=61 0.88516 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=62 0.83115 0.70928 0 0 0 0.0017606 0 0 0 0 0 0 0 0 0 0 0 iter=63 0.78051 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=64 0.73303 1.6575 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0.0017606 iter=65 0.68849 2.0023 0 0 0 0 0 0 0 0 0 0.0035211 0 0 0.0017606 0.0017606 0 iter=66 0.6467 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=67 0.6075 1.091 0.0017606 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=68 0.5707 1.8752 0 0 0 0 0 0 0 0 0 0 0 0.0017606 0 0 0 iter=69 0.53617 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=70 0.50375 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=71 0.47331 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=72 0.44473 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=73 0.4179 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=74 0.39269 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=75 0.36902 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=76 0.34679 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=77 0.3259 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=78 0.30628 0.96214 0 0 0 0 0 0 0 0 0.0035211 0 0 0 0 0 0 iter=79 0.28784 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=80 0.27052 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=81 0.25425 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=82 0.23896 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=83 0.22459 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=84 0.21109 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=85 0.19841 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=86 0.18648 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=87 0.17528 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=88 0.16475 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=89 0.15485 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=90 0.14555 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=91 0.13681 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=92 0.1286 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=93 0.12087 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=94 0.11362 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=95 0.1068 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=96 0.10038 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=97 0.094358 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=98 0.088694 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=99 0.08337 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=100 0.078366 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=101 0.073662 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=102 0.069241 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=103 0.065086 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=104 0.06118 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 tb_val: V(a,z) value at each state space point z1_0_34741 z2_0_40076 z3_0_4623 z4_0_5333 z5_0_61519 z6_0_70966 z10_1_2567 z11_1_4496 z12_1_6723 z13_1_9291 z14_2_2253 z15_2_567 __________ __________ _________ _________ __________ __________ __________ __________ __________ __________ __________ _________ coh1:k=0.443648,b=0 -0.27805 -0.0021088 0.3078 0.63974 0.98775 1.3492 2.9117 3.3277 3.7508 4.1766 4.5947 4.9837 coh2:k=0.543883,b=0 0.13838 0.41572 0.72707 1.0604 1.4098 1.7728 3.3415 3.7583 4.1819 4.608 5.0262 5.4152 coh3:k=0.644119,b=0 0.50582 0.78563 1.0993 1.4348 1.7862 2.1513 3.7256 4.1429 4.5667 4.9926 5.4104 5.7989 coh4:k=0.744354,b=0 0.80629 1.0864 1.4004 1.736 2.0874 2.4522 4.0237 4.44 4.8631 5.2882 5.7052 6.0928 coh5:k=0.84459,b=0 1.0824 1.3627 1.6768 2.0123 2.3633 2.7273 4.2944 4.7095 5.1308 5.5541 5.9691 6.3549 coh6:k=0.944825,b=0 1.3257 1.6053 1.9182 2.2523 2.6016 2.964 4.5241 4.9375 5.357 5.7786 6.1924 6.577 coh7:k=1.04506,b=0 1.5482 1.8271 2.1393 2.4725 2.8209 3.1821 4.7374 5.1489 5.5664 5.9857 6.3967 6.7787 coh8:k=1.1453,b=0 1.7561 2.0334 2.3437 2.6753 3.0222 3.3817 4.9255 5.3339 5.749 6.1659 6.5754 6.956 coh9:k=1.24553,b=0 1.9472 2.2232 2.5319 2.8612 3.2055 3.5628 5.0994 5.5068 5.9203 6.3356 6.7428 7.1213 coh10:k=1.34577,b=0 2.1257 2.4001 2.7075 3.0354 3.378 3.7334 5.2617 5.6658 6.0757 6.4881 6.8928 7.269 coh11:k=1.446,b=0 2.2952 2.5684 2.8738 3.1996 3.54 3.8926 5.4098 5.8118 6.22 6.6307 7.0338 7.4085 coh12:k=1.54624,b=0 2.4542 2.7255 3.029 3.3527 3.6908 4.0412 5.5504 5.95 6.3562 6.7647 7.1651 7.5374 coh13:k=1.64647,b=0 2.6047 2.8741 3.1754 3.4973 3.8339 4.1825 5.6826 6.0793 6.4826 6.8882 7.2862 7.6571 coh14:k=1.74671,b=0 2.7478 3.0159 3.3159 3.6358 3.9699 4.3164 5.8059 6.2009 6.6019 7.0058 7.402 7.7703 coh15:k=1.84694,b=0 2.8857 3.152 3.4497 3.7671 4.0994 4.4437 5.9239 6.317 6.7161 7.1171 7.5105 7.877 coh16:k=1.94718,b=0 3.0171 3.2813 3.5771 3.893 4.2229 4.5645 6.0366 6.4268 6.8229 7.2214 7.6132 7.9781 coh17:k=2.04742,b=0 3.1429 3.4058 3.6997 4.013 4.3412 4.6812 6.1432 6.5305 6.9249 7.3216 7.7118 8.0748 coh18:k=2.14765,b=0 3.2638 3.525 3.8175 4.1293 4.4548 4.7922 6.2452 6.6307 7.0228 7.4176 7.805 8.1654 coh19:k=2.24789,b=0 3.3808 3.6404 3.9304 4.2401 4.5641 4.8995 6.343 6.7268 7.1166 7.5084 7.8939 8.2529 coh20:k=2.34812,b=0 3.493 3.7513 4.0401 4.3477 4.6689 5.0022 6.4379 6.8185 7.2058 7.596 7.9796 8.3372 coh21:k=2.44836,b=0 3.6025 3.8585 4.1447 4.4508 4.7704 5.1013 6.5278 6.9068 7.292 7.6806 8.0621 8.4171 coh22:k=2.54859,b=0 3.707 3.9618 4.2466 4.5505 4.8685 5.1977 6.615 6.9922 7.3756 7.7612 8.1406 8.4943 coh23:k=2.64883,b=0 3.8084 4.0619 4.3453 4.6473 4.9625 5.2897 6.6998 7.0742 7.4551 7.8392 8.2168 8.5692 coh24:k=2.74906,b=0 3.9077 4.1589 4.4404 4.7409 5.0547 5.3795 6.7809 7.1536 7.5323 7.9148 8.2906 8.6403 coh25:k=2.8493,b=0 4.0038 4.2539 4.5333 4.8311 5.1433 5.4666 6.8597 7.2304 7.6075 7.9871 8.3613 8.7099 coh26:k=2.94953,b=0 4.096 4.3448 4.6229 4.9193 5.2293 5.5511 6.9363 7.3044 7.68 8.0581 8.4299 8.7772 coh27:k=3.04977,b=0 4.1872 4.4334 4.7102 5.0052 5.3132 5.6322 7.0108 7.3774 7.7501 8.1265 8.4966 8.8419 coh28:k=3.15001,b=0 4.2758 4.5205 4.7949 5.0886 5.3952 5.7127 7.0823 7.4474 7.8185 8.1931 8.5617 8.9051 coh29:k=3.25024,b=0 4.3621 4.6053 4.8783 5.1692 5.4743 5.7903 7.1528 7.5155 7.8851 8.2572 8.6241 8.9663 coh30:k=3.35048,b=0 4.4457 4.6873 4.959 5.2486 5.5513 5.8659 7.2211 7.5815 7.9495 8.3202 8.685 9.026 coh31:k=3.45071,b=0 4.5287 4.7671 5.0375 5.3258 5.6268 5.939 7.2876 7.6466 8.0119 8.3812 8.7445 9.0836 coh32:k=3.55095,b=0 4.6098 4.8464 5.1141 5.4011 5.7008 6.0112 7.3518 7.7095 8.0733 8.4407 8.8027 9.14 coh33:k=3.65118,b=0 4.6886 4.9236 5.1894 5.4744 5.7728 6.0818 7.4154 7.7709 8.1333 8.4984 8.8589 9.1951 coh34:k=3.75142,b=0 4.7655 4.999 5.2633 5.5463 5.8428 6.1504 7.4774 7.8307 8.1918 8.5555 8.9138 9.2488 coh35:k=3.85165,b=0 4.8409 5.0725 5.3351 5.6169 5.9112 6.2175 7.5378 7.8896 8.2484 8.6108 8.9673 9.301 coh36:k=3.95189,b=0 4.9158 5.1444 5.4051 5.6856 5.9785 6.2825 7.5967 7.9472 8.3038 8.6649 9.0202 9.3518 coh37:k=4.05212,b=0 4.9892 5.2163 5.4738 5.7529 6.0445 6.3466 7.6538 8.0029 8.3583 8.7173 9.0715 9.4018 coh38:k=4.15236,b=0 5.0606 5.2862 5.5417 5.8187 6.1091 6.4099 7.7102 8.0576 8.4117 8.7687 9.1216 9.4509 coh39:k=4.2526,b=0 5.1305 5.3546 5.6086 5.8829 6.1721 6.4717 7.7658 8.111 8.4639 8.8197 9.1706 9.4989 coh40:k=4.35283,b=0 5.1989 5.4217 5.674 5.9468 6.2339 6.5322 7.8202 8.1638 8.5148 8.8694 9.2185 9.5456 coh41:k=4.45307,b=0 5.2671 5.4872 5.738 6.0092 6.2945 6.5914 7.8736 8.2159 8.5648 8.9182 9.2661 9.5913 coh42:k=4.5533,b=0 5.3343 5.5521 5.8007 6.0703 6.3542 6.6491 7.9254 8.2665 8.6139 8.9656 9.3124 9.636 coh43:k=4.65354,b=0 5.4003 5.6166 5.8624 6.1302 6.4129 6.706 7.9762 8.3161 8.6622 9.0121 9.3577 9.6803 coh44:k=4.75377,b=0 5.4648 5.6798 5.9236 6.189 6.4703 6.7622 8.0262 8.3646 8.7096 9.0577 9.4022 9.7238 coh45:k=4.85401,b=0 5.528 5.7417 5.984 6.2468 6.5266 6.8173 8.0755 8.412 8.7558 9.1028 9.4455 9.7662 coh46:k=4.95424,b=0 5.5901 5.8024 6.0433 6.3041 6.582 6.8715 8.1241 8.4586 8.8012 9.1471 9.488 9.8078 coh47:k=5.05448,b=0 5.6514 5.8621 6.1015 6.3608 6.6365 6.9249 8.1719 8.5052 8.8458 9.1907 9.5305 9.8487 coh48:k=5.15471,b=0 5.7126 5.9206 6.1586 6.4164 6.6899 6.977 8.2186 8.5508 8.8895 9.2333 9.5721 9.8888 coh49:k=5.25495,b=0 5.7728 5.979 6.2148 6.471 6.743 7.0282 8.2646 8.5957 8.9332 9.2752 9.6131 9.9286 coh50:k=5.35519,b=0 5.8321 6.0369 6.2702 6.5249 6.7952 7.0786 8.3098 8.6397 8.9762 9.3164 9.6532 9.9679 coh519:k=52.3656,b=0 15.087 15.132 15.185 15.243 15.306 15.375 15.716 15.821 15.935 16.058 16.186 16.313 coh520:k=52.4658,b=0 15.097 15.142 15.194 15.252 15.315 15.384 15.724 15.829 15.943 16.066 16.194 16.32 coh521:k=52.5661,b=0 15.106 15.151 15.203 15.261 15.324 15.393 15.733 15.838 15.951 16.074 16.202 16.328 coh522:k=52.6663,b=0 15.115 15.16 15.212 15.27 15.333 15.401 15.741 15.846 15.959 16.082 16.209 16.335 coh523:k=52.7666,b=0 15.124 15.169 15.221 15.279 15.342 15.41 15.749 15.854 15.967 16.089 16.217 16.343 coh524:k=52.8668,b=0 15.133 15.178 15.23 15.288 15.351 15.419 15.758 15.862 15.975 16.097 16.225 16.35 coh525:k=52.967,b=0 15.143 15.187 15.239 15.297 15.36 15.428 15.766 15.87 15.983 16.105 16.232 16.357 coh526:k=53.0673,b=0 15.152 15.197 15.248 15.306 15.368 15.436 15.774 15.878 15.991 16.113 16.24 16.365 coh527:k=53.1675,b=0 15.161 15.206 15.257 15.315 15.377 15.445 15.783 15.887 15.999 16.121 16.247 16.372 coh528:k=53.2677,b=0 15.17 15.215 15.266 15.324 15.386 15.454 15.791 15.895 16.007 16.128 16.255 16.379 coh529:k=53.368,b=0 15.179 15.224 15.275 15.332 15.395 15.463 15.799 15.903 16.015 16.136 16.262 16.387 coh530:k=53.4682,b=0 15.188 15.233 15.284 15.341 15.404 15.471 15.807 15.911 16.023 16.144 16.27 16.394 coh531:k=53.5684,b=0 15.198 15.242 15.293 15.35 15.412 15.48 15.816 15.919 16.031 16.152 16.278 16.401 coh532:k=53.6687,b=0 15.207 15.251 15.302 15.359 15.421 15.489 15.824 15.927 16.039 16.159 16.285 16.409 coh533:k=53.7689,b=0 15.216 15.26 15.311 15.368 15.43 15.497 15.832 15.935 16.047 16.167 16.292 16.416 coh534:k=53.8691,b=0 15.225 15.269 15.32 15.377 15.439 15.506 15.84 15.943 16.055 16.175 16.3 16.423 coh535:k=53.9694,b=0 15.234 15.278 15.329 15.386 15.447 15.515 15.848 15.951 16.063 16.182 16.307 16.43 coh536:k=54.0696,b=0 15.243 15.287 15.338 15.394 15.456 15.523 15.856 15.959 16.07 16.19 16.315 16.437 coh537:k=54.1698,b=0 15.252 15.296 15.347 15.403 15.465 15.532 15.864 15.967 16.078 16.197 16.322 16.444 coh538:k=54.2701,b=0 15.261 15.305 15.355 15.412 15.473 15.54 15.873 15.975 16.086 16.205 16.329 16.451 coh539:k=54.3703,b=0 15.27 15.314 15.364 15.421 15.482 15.549 15.881 15.983 16.094 16.213 16.337 16.458 coh540:k=54.4706,b=0 15.279 15.323 15.373 15.429 15.491 15.557 15.889 15.991 16.102 16.22 16.344 16.465 coh541:k=54.5708,b=0 15.287 15.331 15.382 15.438 15.499 15.566 15.897 15.999 16.109 16.228 16.351 16.472 coh542:k=54.671,b=0 15.296 15.34 15.391 15.447 15.508 15.574 15.905 16.007 16.117 16.235 16.359 16.479 coh543:k=54.7713,b=0 15.305 15.349 15.399 15.455 15.517 15.583 15.913 16.015 16.125 16.243 16.366 16.486 coh544:k=54.8715,b=0 15.314 15.358 15.408 15.464 15.525 15.591 15.921 16.022 16.132 16.25 16.373 16.493 coh545:k=54.9717,b=0 15.323 15.367 15.417 15.473 15.534 15.6 15.929 16.03 16.14 16.258 16.38 16.5 coh546:k=55.072,b=0 15.332 15.375 15.425 15.481 15.542 15.608 15.937 16.038 16.148 16.265 16.388 16.507 coh547:k=55.1722,b=0 15.341 15.384 15.434 15.49 15.551 15.617 15.945 16.046 16.155 16.273 16.395 16.514 coh548:k=55.2724,b=0 15.349 15.393 15.443 15.499 15.559 15.625 15.953 16.054 16.163 16.28 16.402 16.521 coh549:k=55.3727,b=0 15.358 15.402 15.451 15.507 15.568 15.634 15.961 16.062 16.171 16.288 16.409 16.527 coh550:k=55.4729,b=0 15.367 15.41 15.46 15.516 15.576 15.642 15.969 16.069 16.178 16.295 16.415 16.533 coh551:k=55.5731,b=0 15.376 15.419 15.469 15.524 15.585 15.65 15.977 16.077 16.186 16.302 16.422 16.539 coh552:k=55.6734,b=0 15.385 15.428 15.477 15.533 15.593 15.659 15.985 16.085 16.193 16.309 16.429 16.545 coh553:k=55.7736,b=0 15.39 15.433 15.483 15.538 15.598 15.664 15.989 16.09 16.198 16.314 16.433 16.55 coh554:k=55.8739,b=0 15.391 15.434 15.483 15.539 15.599 15.665 15.99 16.09 16.199 16.315 16.436 16.556 coh555:k=55.9741,b=0 15.391 15.435 15.484 15.54 15.6 15.665 15.991 16.091 16.201 16.319 16.443 16.563 coh556:k=56.0743,b=0 15.392 15.435 15.485 15.54 15.601 15.667 15.997 16.098 16.209 16.327 16.45 16.57 coh557:k=56.1746,b=0 15.398 15.442 15.492 15.548 15.609 15.675 16.005 16.106 16.216 16.334 16.457 16.577 coh558:k=56.2748,b=0 15.407 15.45 15.5 15.556 15.617 15.683 16.012 16.114 16.224 16.341 16.464 16.584 coh559:k=56.375,b=0 15.415 15.459 15.509 15.565 15.626 15.692 16.02 16.121 16.231 16.348 16.471 16.59 coh560:k=56.4753,b=0 15.424 15.467 15.517 15.573 15.634 15.7 16.028 16.129 16.238 16.356 16.478 16.597 coh561:k=56.5755,b=0 15.432 15.476 15.526 15.581 15.642 15.708 16.036 16.136 16.246 16.363 16.484 16.603 coh562:k=56.6757,b=0 15.441 15.484 15.534 15.59 15.65 15.716 16.043 16.144 16.253 16.37 16.491 16.609 coh563:k=56.776,b=0 15.449 15.493 15.542 15.598 15.658 15.724 16.051 16.152 16.26 16.377 16.497 16.615 coh564:k=56.8762,b=0 15.458 15.501 15.551 15.606 15.667 15.732 16.059 16.159 16.268 16.384 16.504 16.621 coh565:k=56.9764,b=0 15.466 15.509 15.559 15.615 15.675 15.74 16.066 16.167 16.275 16.391 16.511 16.627 coh566:k=57.0767,b=0 15.471 15.514 15.564 15.619 15.679 15.745 16.07 16.171 16.279 16.395 16.514 16.63 coh567:k=57.1769,b=0 15.471 15.515 15.564 15.62 15.68 15.745 16.071 16.171 16.28 16.395 16.515 16.631 coh568:k=57.2771,b=0 15.472 15.515 15.565 15.62 15.681 15.746 16.072 16.172 16.28 16.396 16.516 16.631 tb_pol_a: optimal safe savings choice for each state space point z1_0_34741 z2_0_40076 z3_0_4623 z4_0_5333 z5_0_61519 z6_0_70966 z10_1_2567 z11_1_4496 z12_1_6723 z13_1_9291 z14_2_2253 z15_2_567 __________ __________ __________ __________ __________ __________ __________ __________ __________ __________ __________ __________ coh1:k=0.443648,b=0 9.9839e-05 9.9839e-05 9.9839e-05 9.9839e-05 9.9839e-05 9.9839e-05 9.9839e-05 9.9839e-05 9.9839e-05 9.9839e-05 9.9839e-05 9.9839e-05 coh2:k=0.543883,b=0 0.0001224 0.00013347 0.00013347 0.00013347 0.00013347 0.00014455 0.00014455 0.00014455 0.00014455 0.00014455 0.00014455 0.00014455 coh3:k=0.644119,b=0 0.00019743 0.00019743 0.00019743 0.00019743 0.00019743 0.00019743 0.00019743 0.00019743 0.00019743 0.00019743 0.00019743 0.00019743 coh4:k=0.744354,b=0 0.00024331 0.00024331 0.00024331 0.00024331 0.00024331 0.00024331 0.00024331 0.00022815 0.00022815 0.00022815 0.00022815 0.00022815 coh5:k=0.84459,b=0 0.00031048 0.00031048 0.00031048 0.00031048 0.00031048 0.00031048 0.00029328 0.00029328 0.00029328 0.00029328 0.00029328 0.00029328 coh6:k=0.944825,b=0 0.00038582 0.00038582 0.00038582 0.00038582 0.00038582 0.00036657 0.00034733 0.00034733 0.00034733 0.00032809 0.00032809 0.00032809 coh7:k=1.04506,b=0 0.00044803 0.00042675 0.00042675 0.00042675 0.00042675 0.00040546 0.00040546 0.00040546 0.00040546 0.00040546 0.00040546 0.00040546 coh8:k=1.1453,b=0 0.00051433 0.00051433 0.00051433 0.000491 0.000491 0.000491 0.000491 0.00046768 0.00046768 0.00046768 0.00044435 0.00044435 coh9:k=1.24553,b=0 0.00058471 0.00058471 0.00058471 0.00058471 0.00055935 0.00055935 0.00053398 0.00050861 0.00050861 0.00050861 0.00050861 0.00050861 coh10:k=1.34577,b=0 0.00065918 0.00065918 0.00063177 0.00063177 0.00063177 0.00060436 0.00060436 0.00060436 0.00060436 0.00057695 0.00057695 0.00057695 coh11:k=1.446,b=0 0.00070828 0.00070828 0.00070828 0.00070828 0.00070828 0.00070828 0.00067882 0.00064937 0.00064937 0.00061992 0.00061992 0.00061992 coh12:k=1.54624,b=0 0.00078887 0.00078887 0.00078887 0.00078887 0.00078887 0.00075737 0.00072588 0.00072588 0.00069439 0.00069439 0.00069439 0.00069439 coh13:k=1.64647,b=0 0.00087354 0.00087354 0.00087354 0.00084 0.00084 0.00084 0.00080647 0.00080647 0.00077294 0.00077294 0.0007394 0.0007394 coh14:k=1.74671,b=0 0.00096229 0.00092672 0.00092672 0.00092672 0.00092672 0.00089114 0.00085557 0.00085557 0.00081999 0.00081999 0.00081999 0.00081999 coh15:k=1.84694,b=0 0.0010175 0.0010175 0.0010175 0.0010175 0.0009799 0.0009799 0.00094228 0.00090466 0.00090466 0.00090466 0.00090466 0.00086705 coh16:k=1.94718,b=0 0.0011124 0.0011124 0.0010727 0.0010727 0.0010727 0.0010727 0.00099342 0.00099342 0.00099342 0.00095376 0.00095376 0.0009141 coh17:k=2.04742,b=0 0.0011697 0.0011697 0.0011697 0.0011697 0.001128 0.001128 0.0010863 0.0010446 0.0010446 0.0010029 0.0010029 0.0010029 coh18:k=2.14765,b=0 0.0012707 0.0012269 0.0012269 0.0012269 0.0012269 0.0011832 0.0011394 0.0011394 0.0010957 0.0010957 0.0010957 0.0010957 coh19:k=2.24789,b=0 0.00133 0.00133 0.00133 0.0012842 0.0012842 0.0012842 0.0011926 0.0011926 0.0011926 0.0011926 0.0011468 0.0011468 coh20:k=2.34812,b=0 0.0013893 0.0013893 0.0013893 0.0013893 0.0013893 0.0013414 0.0012936 0.0012936 0.0012458 0.0012458 0.001198 0.001198 coh21:k=2.44836,b=0 0.0014984 0.0014984 0.0014984 0.0014486 0.0014486 0.0014486 0.0013488 0.0013488 0.001299 0.001299 0.001299 0.001299 coh22:k=2.54859,b=0 0.0015598 0.0015598 0.0015598 0.0015079 0.0015079 0.0015079 0.001456 0.0014041 0.0014041 0.0014041 0.0013522 0.0013522 coh23:k=2.64883,b=0 0.0016751 0.0016211 0.0016211 0.0016211 0.0016211 0.0015672 0.0015132 0.0015132 0.0014593 0.0014593 0.0014053 0.0014053 coh24:k=2.74906,b=0 0.0017385 0.0017385 0.0016825 0.0016825 0.0016825 0.0016825 0.0015705 0.0015705 0.0015705 0.0015145 0.0015145 0.0015145 coh25:k=2.8493,b=0 0.0018019 0.0018019 0.0018019 0.0018019 0.0017438 0.0017438 0.0016858 0.0016278 0.0016278 0.0016278 0.0015697 0.0015697 coh26:k=2.94953,b=0 0.025719 0.0018652 0.0018652 0.0018652 0.0018052 0.0018052 0.0017451 0.0017451 0.001685 0.001685 0.001625 0.001625 coh27:k=3.04977,b=0 0.14879 0.0019908 0.0019286 0.0019286 0.0019286 0.0018665 0.0018044 0.0018044 0.0018044 0.0017423 0.0017423 0.0016802 coh28:k=3.15001,b=0 0.2142 0.0020562 0.001992 0.001992 0.001992 0.001992 0.0018637 0.0018637 0.0018637 0.0017996 0.0017996 0.0017996 coh29:k=3.25024,b=0 0.27699 0.0021216 0.0021216 0.0021216 0.0020554 0.0020554 0.0019892 0.001923 0.001923 0.001923 0.0018568 0.0018568 coh30:k=3.35048,b=0 0.34368 0.002187 0.002187 0.002187 0.0021188 0.0021188 0.0020506 0.0020506 0.0019823 0.0019823 0.0019141 0.0019141 coh31:k=3.45071,b=0 0.44583 0.069794 0.0022525 0.0022525 0.0022525 0.0021822 0.0021119 0.0021119 0.0020416 0.0020416 0.0020416 0.0019713 coh32:k=3.55095,b=0 0.52423 0.19029 0.0023179 0.0023179 0.0023179 0.0023179 0.0021733 0.0021733 0.0021733 0.0021009 0.0021009 0.0021009 coh33:k=3.65118,b=0 0.60694 0.25654 0.0024577 0.0023833 0.0023833 0.0023833 0.002309 0.0022346 0.0022346 0.0022346 0.0021602 0.0021602 coh34:k=3.75142,b=0 0.6818 0.33246 0.0025252 0.0025252 0.0024488 0.0024488 0.0023724 0.0022959 0.0022959 0.0022959 0.0022195 0.0022195 coh35:k=3.85165,b=0 0.80054 0.41223 0.0025926 0.0025926 0.0025926 0.0025142 0.0024357 0.0024357 0.0023573 0.0023573 0.0023573 0.0022788 coh36:k=3.95189,b=0 0.86008 0.54524 0.063035 0.0026601 0.0026601 0.0025796 0.0024991 0.0024991 0.0024186 0.0024186 0.0024186 0.0023381 coh37:k=4.05212,b=0 0.92387 0.63504 0.13912 0.0027276 0.0027276 0.0027276 0.0025625 0.0025625 0.0025625 0.00248 0.00248 0.00248 coh38:k=4.15236,b=0 1.0287 0.69174 0.28119 0.002795 0.002795 0.002795 0.0027105 0.0026259 0.0026259 0.0026259 0.0025413 0.0025413 coh39:k=4.2526,b=0 1.1106 0.75021 0.35622 0.0028625 0.0028625 0.0028625 0.0027759 0.0026893 0.0026893 0.0026893 0.0026027 0.0026027 coh40:k=4.35283,b=0 1.1791 0.81026 0.44137 0.0030186 0.00293 0.00293 0.0028413 0.0028413 0.0027527 0.0027527 0.0027527 0.002664 coh41:k=4.45307,b=0 1.3454 0.87262 0.49524 0.0030882 0.0030882 0.0029975 0.0029068 0.0029068 0.0028161 0.0028161 0.0028161 0.0027254 coh42:k=4.5533,b=0 1.3935 1.0921 0.5512 0.070231 0.0031577 0.0030649 0.0029722 0.0029722 0.0029722 0.0028795 0.0028795 0.0028795 coh43:k=4.65354,b=0 1.4348 1.1642 0.62271 0.14864 0.0032272 0.0032272 0.0030376 0.0030376 0.0030376 0.0029428 0.0029428 0.0029428 coh44:k=4.75377,b=0 1.515 1.2386 0.82386 0.19827 0.0032967 0.0032967 0.0031999 0.003103 0.003103 0.0030062 0.0030062 0.0030062 coh45:k=4.85401,b=0 1.5976 1.2738 0.89188 0.25031 0.0033662 0.0033662 0.0032673 0.0031685 0.0031685 0.0031685 0.0030696 0.0030696 coh46:k=4.95424,b=0 1.682 1.3218 0.96166 0.45744 0.0034357 0.0034357 0.0033348 0.0033348 0.0032339 0.0032339 0.003133 0.003133 coh47:k=5.05448,b=0 1.8206 1.4006 1.0332 0.51876 0.0035052 0.0035052 0.0034023 0.0034023 0.0032993 0.0032993 0.0032993 0.0031964 coh48:k=5.15471,b=0 1.9136 1.4823 1.1076 0.58295 0.0036797 0.0035747 0.0034698 0.0034698 0.0033648 0.0033648 0.0033648 0.0032598 coh49:k=5.25495,b=0 2.0087 1.6944 1.1838 0.64893 0.037704 0.0036443 0.0035372 0.0035372 0.0035372 0.0034302 0.0034302 0.0034302 coh50:k=5.35519,b=0 2.0861 1.7657 1.2496 0.71666 0.10587 0.0037138 0.0036047 0.0036047 0.0036047 0.0034956 0.0034956 0.0034956 coh519:k=52.3656,b=0 45.02 45.02 44.063 44.063 43.106 42.149 37.365 36.408 33.537 31.624 28.753 25.882 coh520:k=52.4658,b=0 45.106 45.106 44.147 44.147 43.189 42.23 37.436 36.478 33.602 31.684 28.808 25.932 coh521:k=52.5661,b=0 45.192 45.192 44.232 44.232 43.271 42.311 38.143 36.547 33.666 31.745 28.863 25.982 coh522:k=52.6663,b=0 45.278 45.278 44.316 44.316 43.354 42.391 38.542 36.617 34.267 31.805 28.918 26.031 coh523:k=52.7666,b=0 45.364 45.364 44.4 44.4 43.436 42.472 38.615 36.687 34.758 31.866 28.973 26.081 coh524:k=52.8668,b=0 45.451 45.451 44.485 44.485 43.519 42.553 38.688 36.756 34.824 31.926 29.028 26.13 coh525:k=52.967,b=0 45.537 45.537 44.569 44.569 43.601 42.633 38.762 36.826 34.89 31.987 29.083 26.18 coh526:k=53.0673,b=0 45.623 45.623 44.653 44.653 43.684 42.714 38.835 36.896 34.956 32.047 29.138 26.229 coh527:k=53.1675,b=0 45.709 45.709 44.738 44.738 43.766 42.795 38.909 36.965 35.022 32.108 29.193 26.279 coh528:k=53.2677,b=0 45.795 45.795 44.822 44.822 43.849 42.875 38.982 37.035 35.088 32.168 29.248 26.328 coh529:k=53.368,b=0 45.882 45.882 44.906 44.906 43.931 42.956 39.055 37.105 35.155 32.229 29.303 26.378 coh530:k=53.4682,b=0 45.968 45.968 44.991 44.991 44.014 43.037 39.129 37.175 35.221 32.289 29.358 26.427 coh531:k=53.5684,b=0 47.099 46.054 45.075 45.075 44.096 43.117 39.202 37.244 35.287 32.35 29.413 26.477 coh532:k=53.6687,b=0 47.188 46.14 45.159 45.159 44.179 43.198 39.275 37.314 35.353 32.411 29.469 26.526 coh533:k=53.7689,b=0 47.276 46.226 45.244 45.244 44.261 43.279 39.349 37.384 35.419 32.471 29.524 26.576 coh534:k=53.8691,b=0 47.364 46.312 45.328 45.328 44.344 43.359 39.422 37.453 35.485 32.532 29.579 26.626 coh535:k=53.9694,b=0 47.452 46.399 45.412 45.412 44.426 43.44 39.495 37.523 35.551 32.592 29.634 26.675 coh536:k=54.0696,b=0 47.54 46.485 45.497 45.497 44.509 43.521 39.569 37.593 35.617 32.653 29.81 26.846 coh537:k=54.1698,b=0 47.628 46.571 45.581 45.581 44.591 43.601 39.642 37.662 35.683 32.713 30.734 27.744 coh538:k=54.2701,b=0 47.716 46.657 45.665 45.665 44.674 43.682 39.715 37.732 35.749 32.774 30.79 26.905 coh539:k=54.3703,b=0 47.804 47.804 45.75 45.75 44.756 44.573 39.789 37.802 35.815 33.644 30.847 26.873 coh540:k=54.4706,b=0 47.893 47.893 45.834 45.834 44.839 44.839 39.862 37.871 35.881 33.181 30.904 26.923 coh541:k=54.5708,b=0 46.916 46.916 45.918 45.918 44.921 44.921 39.935 37.941 35.947 32.955 30.961 26.972 coh542:k=54.671,b=0 47.002 47.002 46.003 46.003 45.004 45.004 40.009 38.011 36.013 33.523 31.018 27.529 coh543:k=54.7713,b=0 47.088 47.088 46.087 46.087 45.086 45.086 40.082 38.081 36.079 34.077 31.075 28.072 coh544:k=54.8715,b=0 47.174 47.174 46.171 46.171 45.169 45.169 40.156 38.15 36.145 34.14 31.132 28.124 coh545:k=54.9717,b=0 47.26 47.26 46.256 46.256 45.251 45.251 40.229 38.22 36.211 34.202 31.188 28.175 coh546:k=55.072,b=0 47.346 47.346 46.34 46.34 45.334 45.334 40.302 38.29 36.277 34.264 31.245 28.226 coh547:k=55.1722,b=0 47.433 47.433 46.425 46.425 45.416 45.416 40.376 38.359 36.343 34.327 31.302 28.278 coh548:k=55.2724,b=0 47.519 47.519 46.509 46.509 45.499 45.499 40.449 38.429 36.409 34.389 31.359 28.329 coh549:k=55.3727,b=0 47.605 47.605 46.593 46.593 45.581 45.581 40.522 38.499 36.475 34.451 32.215 29.18 coh550:k=55.4729,b=0 47.691 47.691 46.678 46.678 45.664 45.664 40.596 38.568 36.541 34.514 31.789 28.749 coh551:k=55.5731,b=0 47.777 47.777 46.762 46.762 45.746 45.746 40.669 38.638 36.607 34.576 31.53 29.077 coh552:k=55.6734,b=0 47.864 47.864 46.846 46.846 45.829 45.829 40.742 38.708 36.673 34.639 32.078 30.043 coh553:k=55.7736,b=0 47.951 47.951 46.933 46.933 45.915 45.915 40.823 38.786 36.749 34.713 32.676 26.941 coh554:k=55.8739,b=0 48.041 48.041 47.023 47.023 46.004 46.004 40.913 38.876 36.839 34.802 30.981 26.989 coh555:k=55.9741,b=0 48.131 48.131 47.113 47.113 46.094 46.094 41.003 38.966 36.034 33.832 31.036 27.835 coh556:k=56.0743,b=0 48.221 48.221 47.203 47.203 45.111 45.111 40.104 38.101 36.099 34.096 31.092 28.088 coh557:k=56.1746,b=0 47.198 47.198 46.195 46.195 45.192 45.192 40.176 38.17 36.163 34.157 31.147 28.138 coh558:k=56.2748,b=0 47.282 47.282 46.277 46.277 45.272 45.272 40.248 38.238 36.228 34.218 31.203 28.188 coh559:k=56.375,b=0 47.366 47.366 46.36 46.36 45.353 45.353 40.319 38.306 36.292 34.279 31.259 28.238 coh560:k=56.4753,b=0 47.451 47.451 46.442 46.442 45.434 45.434 40.391 38.374 36.357 34.34 31.314 28.289 coh561:k=56.5755,b=0 47.535 47.535 46.525 46.525 45.514 45.514 40.463 38.442 36.421 34.401 31.43 28.399 coh562:k=56.6757,b=0 47.619 47.619 46.607 46.607 45.595 45.595 40.534 38.51 36.486 34.462 32.373 29.337 coh563:k=56.776,b=0 47.703 47.703 46.689 46.689 45.676 45.676 40.606 38.578 36.55 34.523 31.669 28.628 coh564:k=56.8762,b=0 47.788 47.788 46.772 46.772 45.756 45.756 40.678 38.646 36.615 34.584 31.536 29.192 coh565:k=56.9764,b=0 47.872 47.872 46.854 46.854 45.837 45.837 40.749 38.714 36.679 34.644 32.17 30.135 coh566:k=57.0767,b=0 47.958 47.958 46.939 46.939 45.921 45.921 40.829 38.793 36.756 34.719 32.682 30.646 coh567:k=57.1769,b=0 48.046 48.046 47.027 47.027 46.009 46.009 40.917 38.88 36.844 34.807 32.77 30.734 coh568:k=57.2771,b=0 48.134 48.134 47.115 47.115 46.097 46.097 41.005 38.968 36.932 34.895 32.858 30.821 tb_pol_k: optimal risky investment choice for each state space point z1_0_34741 z2_0_40076 z3_0_4623 z4_0_5333 z5_0_61519 z6_0_70966 z10_1_2567 z11_1_4496 z12_1_6723 z13_1_9291 z14_2_2253 z15_2_567 __________ __________ _________ _________ __________ __________ __________ __________ __________ __________ __________ _________ coh1:k=0.443648,b=0 0.099739 0.099739 0.099739 0.099739 0.099739 0.099739 0.099739 0.099739 0.099739 0.099739 0.099739 0.099739 coh2:k=0.543883,b=0 0.12227 0.13334 0.13334 0.13334 0.13334 0.14441 0.14441 0.14441 0.14441 0.14441 0.14441 0.14441 coh3:k=0.644119,b=0 0.19723 0.19723 0.19723 0.19723 0.19723 0.19723 0.19723 0.19723 0.19723 0.19723 0.19723 0.19723 coh4:k=0.744354,b=0 0.24307 0.24307 0.24307 0.24307 0.24307 0.24307 0.24307 0.22792 0.22792 0.22792 0.22792 0.22792 coh5:k=0.84459,b=0 0.31017 0.31017 0.31017 0.31017 0.31017 0.31017 0.29299 0.29299 0.29299 0.29299 0.29299 0.29299 coh6:k=0.944825,b=0 0.38543 0.38543 0.38543 0.38543 0.38543 0.36621 0.34698 0.34698 0.34698 0.32776 0.32776 0.32776 coh7:k=1.04506,b=0 0.44758 0.42632 0.42632 0.42632 0.42632 0.40506 0.40506 0.40506 0.40506 0.40506 0.40506 0.40506 coh8:k=1.1453,b=0 0.51382 0.51382 0.51382 0.49051 0.49051 0.49051 0.49051 0.46721 0.46721 0.46721 0.44391 0.44391 coh9:k=1.24553,b=0 0.58413 0.58413 0.58413 0.58413 0.55879 0.55879 0.53344 0.5081 0.5081 0.5081 0.5081 0.5081 coh10:k=1.34577,b=0 0.65852 0.65852 0.63114 0.63114 0.63114 0.60375 0.60375 0.60375 0.60375 0.57637 0.57637 0.57637 coh11:k=1.446,b=0 0.70757 0.70757 0.70757 0.70757 0.70757 0.70757 0.67815 0.64872 0.64872 0.6193 0.6193 0.6193 coh12:k=1.54624,b=0 0.78808 0.78808 0.78808 0.78808 0.78808 0.75662 0.72515 0.72515 0.69369 0.69369 0.69369 0.69369 coh13:k=1.64647,b=0 0.87266 0.87266 0.87266 0.83916 0.83916 0.83916 0.80566 0.80566 0.77216 0.77216 0.73866 0.73866 coh14:k=1.74671,b=0 0.96133 0.92579 0.92579 0.92579 0.92579 0.89025 0.85471 0.85471 0.81917 0.81917 0.81917 0.81917 coh15:k=1.84694,b=0 1.0165 1.0165 1.0165 1.0165 0.97892 0.97892 0.94134 0.90376 0.90376 0.90376 0.90376 0.86618 coh16:k=1.94718,b=0 1.1113 1.1113 1.0717 1.0717 1.0717 1.0717 0.99243 0.99243 0.99243 0.95281 0.95281 0.91319 coh17:k=2.04742,b=0 1.1685 1.1685 1.1685 1.1685 1.1268 1.1268 1.0852 1.0435 1.0435 1.0019 1.0019 1.0019 coh18:k=2.14765,b=0 1.2694 1.2257 1.2257 1.2257 1.2257 1.182 1.1383 1.1383 1.0946 1.0946 1.0946 1.0946 coh19:k=2.24789,b=0 1.3286 1.3286 1.3286 1.2829 1.2829 1.2829 1.1914 1.1914 1.1914 1.1914 1.1457 1.1457 coh20:k=2.34812,b=0 1.3879 1.3879 1.3879 1.3879 1.3879 1.3401 1.2923 1.2923 1.2446 1.2446 1.1968 1.1968 coh21:k=2.44836,b=0 1.4969 1.4969 1.4969 1.4471 1.4471 1.4471 1.3475 1.3475 1.2977 1.2977 1.2977 1.2977 coh22:k=2.54859,b=0 1.5582 1.5582 1.5582 1.5064 1.5064 1.5064 1.4545 1.4027 1.4027 1.4027 1.3508 1.3508 coh23:k=2.64883,b=0 1.6734 1.6195 1.6195 1.6195 1.6195 1.5656 1.5117 1.5117 1.4578 1.4578 1.4039 1.4039 coh24:k=2.74906,b=0 1.7367 1.7367 1.6808 1.6808 1.6808 1.6808 1.5689 1.5689 1.5689 1.513 1.513 1.513 coh25:k=2.8493,b=0 1.8001 1.8001 1.8001 1.8001 1.7421 1.7421 1.6841 1.6261 1.6261 1.6261 1.5682 1.5682 coh26:k=2.94953,b=0 1.8395 1.8634 1.8634 1.8634 1.8034 1.8034 1.7434 1.7434 1.6833 1.6833 1.6233 1.6233 coh27:k=3.04977,b=0 1.842 1.9888 1.9267 1.9267 1.9267 1.8647 1.8026 1.8026 1.8026 1.7405 1.7405 1.6785 coh28:k=3.15001,b=0 1.842 2.0541 1.99 1.99 1.99 1.99 1.8618 1.8618 1.8618 1.7978 1.7978 1.7978 coh29:k=3.25024,b=0 1.8446 2.1195 2.1195 2.1195 2.0534 2.0534 1.9872 1.9211 1.9211 1.9211 1.855 1.855 coh30:k=3.35048,b=0 1.8434 2.1849 2.1849 2.1849 2.1167 2.1167 2.0485 2.0485 1.9803 1.9803 1.9122 1.9122 coh31:k=3.45071,b=0 1.8769 2.1827 2.2502 2.2502 2.2502 2.18 2.1098 2.1098 2.0396 2.0396 2.0396 1.9694 coh32:k=3.55095,b=0 1.866 2.1999 2.3156 2.3156 2.3156 2.3156 2.1711 2.1711 2.1711 2.0988 2.0988 2.0988 coh33:k=3.65118,b=0 1.8508 2.2012 2.4552 2.3809 2.3809 2.3809 2.3067 2.2324 2.2324 2.2324 2.1581 2.1581 coh34:k=3.75142,b=0 1.8434 2.1927 2.5226 2.5226 2.4463 2.4463 2.37 2.2936 2.2936 2.2936 2.2173 2.2173 coh35:k=3.85165,b=0 1.8705 2.1804 2.59 2.59 2.59 2.5117 2.4333 2.4333 2.3549 2.3549 2.3549 2.2766 coh36:k=3.95189,b=0 1.8805 2.1954 2.5971 2.6574 2.6574 2.577 2.4966 2.4966 2.4162 2.4162 2.4162 2.3358 coh37:k=4.05212,b=0 1.8862 2.1751 2.5885 2.7248 2.7248 2.7248 2.56 2.56 2.56 2.4775 2.4775 2.4775 coh38:k=4.15236,b=0 1.8509 2.1879 2.5984 2.7923 2.7923 2.7923 2.7078 2.6233 2.6233 2.6233 2.5388 2.5388 coh39:k=4.2526,b=0 1.8385 2.1989 2.5929 2.8597 2.8597 2.8597 2.7731 2.6866 2.6866 2.6866 2.6001 2.6001 coh40:k=4.35283,b=0 1.8395 2.2084 2.5773 3.0156 2.9271 2.9271 2.8385 2.8385 2.7499 2.7499 2.7499 2.6614 coh41:k=4.45307,b=0 1.8334 2.2155 2.5929 3.0851 3.0851 2.9945 2.9039 2.9039 2.8132 2.8132 2.8132 2.7226 coh42:k=4.5533,b=0 1.8569 2.1584 2.6065 3.0874 3.1545 3.0619 2.9692 2.9692 2.9692 2.8766 2.8766 2.8766 coh43:k=4.65354,b=0 1.8872 2.1578 2.6045 3.0785 3.224 3.224 3.0346 3.0346 3.0346 2.9399 2.9399 2.9399 coh44:k=4.75377,b=0 1.8785 2.155 2.5697 3.0984 3.2934 3.2934 3.1967 3.0999 3.0999 3.0032 3.0032 3.0032 coh45:k=4.85401,b=0 1.8674 2.1913 2.5732 3.1159 3.3628 3.3628 3.2641 3.1653 3.1653 3.1653 3.0665 3.0665 coh46:k=4.95424,b=0 1.8546 2.2148 2.575 3.0792 3.4323 3.4323 3.3315 3.3315 3.2307 3.2307 3.1299 3.1299 coh47:k=5.05448,b=0 1.8905 2.2076 2.575 3.0894 3.5017 3.5017 3.3989 3.3989 3.296 3.296 3.296 3.1932 coh48:k=5.15471,b=0 1.8711 2.1974 2.5722 3.0968 3.676 3.5712 3.4663 3.4663 3.3614 3.3614 3.3614 3.2565 coh49:k=5.25495,b=0 1.8496 2.1639 2.5675 3.1024 3.7136 3.6406 3.5337 3.5337 3.5337 3.4268 3.4268 3.4268 coh50:k=5.35519,b=0 1.8458 2.1662 2.5732 3.1062 3.717 3.7101 3.6011 3.6011 3.6011 3.4921 3.4921 3.4921 coh519:k=52.3656,b=0 1.9607 1.9607 2.9176 2.9176 3.8745 4.8313 9.6157 10.573 13.443 15.357 18.227 21.098 coh520:k=52.4658,b=0 1.9645 1.9645 2.9232 2.9232 3.8819 4.8406 9.6341 10.593 13.469 15.386 18.262 21.138 coh521:k=52.5661,b=0 1.9682 1.9682 2.9288 2.9288 3.8893 4.8498 9.0177 10.613 13.495 15.416 18.297 21.179 coh522:k=52.6663,b=0 1.972 1.972 2.9343 2.9343 3.8967 4.8591 8.7085 10.633 12.983 15.445 18.332 21.219 coh523:k=52.7666,b=0 1.9757 1.9757 2.9399 2.9399 3.9041 4.8683 8.7251 10.653 12.582 15.474 18.367 21.26 coh524:k=52.8668,b=0 1.9795 1.9795 2.9455 2.9455 3.9115 4.8776 8.7417 10.674 12.606 15.504 18.402 21.3 coh525:k=52.967,b=0 1.9832 1.9832 2.9511 2.9511 3.919 4.8868 8.7582 10.694 12.63 15.533 18.437 21.34 coh526:k=53.0673,b=0 1.987 1.987 2.9567 2.9567 3.9264 4.8961 8.7748 10.714 12.654 15.563 18.472 21.381 coh527:k=53.1675,b=0 1.9907 1.9907 2.9623 2.9623 3.9338 4.9053 8.7914 10.734 12.677 15.592 18.507 21.421 coh528:k=53.2677,b=0 1.9945 1.9945 2.9678 2.9678 3.9412 4.9146 8.808 10.755 12.701 15.621 18.541 21.462 coh529:k=53.368,b=0 1.9982 1.9982 2.9734 2.9734 3.9486 4.9238 8.8245 10.775 12.725 15.651 18.576 21.502 coh530:k=53.4682,b=0 2.002 2.002 2.979 2.979 3.956 4.933 8.8411 10.795 12.749 15.68 18.611 21.542 coh531:k=53.5684,b=0 2.0513 2.0058 2.9846 2.9846 3.9634 4.9423 8.8577 10.815 12.773 15.71 18.646 21.583 coh532:k=53.6687,b=0 2.0551 2.0095 2.9902 2.9902 3.9709 4.9515 8.8743 10.836 12.797 15.739 18.681 21.623 coh533:k=53.7689,b=0 2.059 2.0133 2.9958 2.9958 3.9783 4.9608 8.8908 10.856 12.821 15.768 18.716 21.663 coh534:k=53.8691,b=0 2.0628 2.017 3.0014 3.0014 3.9857 4.97 8.9074 10.876 12.845 15.798 18.751 21.704 coh535:k=53.9694,b=0 2.0666 2.0208 3.0069 3.0069 3.9931 4.9793 8.924 10.896 12.869 15.827 18.786 21.744 coh536:k=54.0696,b=0 2.0705 2.0245 3.0125 3.0125 4.0005 4.9885 8.9406 10.917 12.893 15.857 18.699 21.663 coh537:k=54.1698,b=0 2.0743 2.0283 3.0181 3.0181 4.0079 4.9978 8.9571 10.937 12.916 15.886 17.866 20.855 coh538:k=54.2701,b=0 2.0782 2.032 3.0237 3.0237 4.0154 5.007 8.9737 10.957 12.94 15.915 17.899 21.784 coh539:k=54.3703,b=0 2.082 2.082 3.0293 3.0293 4.0228 4.2063 8.9903 10.977 12.964 15.135 17.932 21.906 coh540:k=54.4706,b=0 2.0858 2.0858 3.0349 3.0349 4.0302 4.0302 9.0069 10.998 12.988 15.688 17.965 21.946 coh541:k=54.5708,b=0 2.0433 2.0433 3.0404 3.0404 4.0376 4.0376 9.0234 11.018 13.012 16.004 17.998 21.987 coh542:k=54.671,b=0 2.047 2.047 3.046 3.046 4.045 4.045 9.04 11.038 13.036 15.526 18.031 21.52 coh543:k=54.7713,b=0 2.0508 2.0508 3.0516 3.0516 4.0524 4.0524 9.0566 11.058 13.06 15.062 18.064 21.066 coh544:k=54.8715,b=0 2.0545 2.0545 3.0572 3.0572 4.0599 4.0599 9.0731 11.078 13.084 15.089 18.097 21.105 coh545:k=54.9717,b=0 2.0583 2.0583 3.0628 3.0628 4.0673 4.0673 9.0897 11.099 13.108 15.117 18.13 21.144 coh546:k=55.072,b=0 2.0621 2.0621 3.0684 3.0684 4.0747 4.0747 9.1063 11.119 13.132 15.144 18.163 21.182 coh547:k=55.1722,b=0 2.0658 2.0658 3.074 3.074 4.0821 4.0821 9.1229 11.139 13.155 15.172 18.196 21.221 coh548:k=55.2724,b=0 2.0696 2.0696 3.0795 3.0795 4.0895 4.0895 9.1394 11.159 13.179 15.199 18.229 21.259 coh549:k=55.3727,b=0 2.0733 2.0733 3.0851 3.0851 4.0969 4.0969 9.156 11.18 13.203 15.227 17.463 20.498 coh550:k=55.4729,b=0 2.0771 2.0771 3.0907 3.0907 4.1044 4.1044 9.1726 11.2 13.227 15.254 17.979 21.02 coh551:k=55.5731,b=0 2.0808 2.0808 3.0963 3.0963 4.1118 4.1118 9.1892 11.22 13.251 15.282 18.328 20.781 coh552:k=55.6734,b=0 2.0846 2.0846 3.1019 3.1019 4.1192 4.1192 9.2057 11.24 13.275 15.31 17.871 19.905 coh553:k=55.7736,b=0 2.0867 2.0867 3.1051 3.1051 4.1235 4.1235 9.2153 11.252 13.289 15.326 17.362 21.961 coh554:k=55.8739,b=0 2.0867 2.0867 3.1051 3.1051 4.1235 4.1235 9.2153 11.252 13.289 15.326 18.009 22 coh555:k=55.9741,b=0 2.0867 2.0867 3.1051 3.1051 4.1235 4.1235 9.2153 11.252 13.044 15.245 18.042 21.243 coh556:k=56.0743,b=0 2.0867 2.0867 3.1051 3.1051 4.0547 4.0547 9.0615 11.064 13.067 15.07 18.074 21.078 coh557:k=56.1746,b=0 2.0556 2.0556 3.0588 3.0588 4.0619 4.0619 9.0777 11.084 13.09 15.097 18.106 21.116 coh558:k=56.2748,b=0 2.0593 2.0593 3.0642 3.0642 4.0692 4.0692 9.0939 11.104 13.114 15.124 18.139 21.153 coh559:k=56.375,b=0 2.0629 2.0629 3.0697 3.0697 4.0764 4.0764 9.1101 11.124 13.137 15.151 18.171 21.191 coh560:k=56.4753,b=0 2.0666 2.0666 3.0751 3.0751 4.0837 4.0837 9.1263 11.143 13.16 15.178 18.203 21.229 coh561:k=56.5755,b=0 2.0703 2.0703 3.0806 3.0806 4.0909 4.0909 9.1425 11.163 13.184 15.204 18.175 21.206 coh562:k=56.6757,b=0 2.0739 2.0739 3.086 3.086 4.0982 4.0982 9.1587 11.183 13.207 15.231 17.32 20.356 coh563:k=56.776,b=0 2.0776 2.0776 3.0915 3.0915 4.1054 4.1054 9.1749 11.203 13.231 15.258 18.112 21.153 coh564:k=56.8762,b=0 2.0813 2.0813 3.097 3.097 4.1127 4.1127 9.1911 11.223 13.254 15.285 18.332 20.677 coh565:k=56.9764,b=0 2.0849 2.0849 3.1024 3.1024 4.1199 4.1199 9.2073 11.242 13.277 15.312 17.786 19.821 coh566:k=57.0767,b=0 2.0867 2.0867 3.1051 3.1051 4.1235 4.1235 9.2153 11.252 13.289 15.326 17.362 19.399 coh567:k=57.1769,b=0 2.0867 2.0867 3.1051 3.1051 4.1235 4.1235 9.2153 11.252 13.289 15.326 17.362 19.399 coh568:k=57.2771,b=0 2.0867 2.0867 3.1051 3.1051 4.1235 4.1235 9.2153 11.252 13.289 15.326 17.362 19.399 tb_pol_w: risky + safe investment choices (first stage choice, choose within risky vs safe) z1_0_34741 z2_0_40076 z3_0_4623 z4_0_5333 z5_0_61519 z6_0_70966 z10_1_2567 z11_1_4496 z12_1_6723 z13_1_9291 z14_2_2253 z15_2_567 __________ __________ _________ _________ __________ __________ __________ __________ __________ __________ __________ _________ coh1:k=0.443648,b=0 0.099839 0.099839 0.099839 0.099839 0.099839 0.099839 0.099839 0.099839 0.099839 0.099839 0.099839 0.099839 coh2:k=0.543883,b=0 0.1224 0.13347 0.13347 0.13347 0.13347 0.14455 0.14455 0.14455 0.14455 0.14455 0.14455 0.14455 coh3:k=0.644119,b=0 0.19743 0.19743 0.19743 0.19743 0.19743 0.19743 0.19743 0.19743 0.19743 0.19743 0.19743 0.19743 coh4:k=0.744354,b=0 0.24331 0.24331 0.24331 0.24331 0.24331 0.24331 0.24331 0.22815 0.22815 0.22815 0.22815 0.22815 coh5:k=0.84459,b=0 0.31048 0.31048 0.31048 0.31048 0.31048 0.31048 0.29328 0.29328 0.29328 0.29328 0.29328 0.29328 coh6:k=0.944825,b=0 0.38582 0.38582 0.38582 0.38582 0.38582 0.36657 0.34733 0.34733 0.34733 0.32809 0.32809 0.32809 coh7:k=1.04506,b=0 0.44803 0.42675 0.42675 0.42675 0.42675 0.40546 0.40546 0.40546 0.40546 0.40546 0.40546 0.40546 coh8:k=1.1453,b=0 0.51433 0.51433 0.51433 0.491 0.491 0.491 0.491 0.46768 0.46768 0.46768 0.44435 0.44435 coh9:k=1.24553,b=0 0.58471 0.58471 0.58471 0.58471 0.55935 0.55935 0.53398 0.50861 0.50861 0.50861 0.50861 0.50861 coh10:k=1.34577,b=0 0.65918 0.65918 0.63177 0.63177 0.63177 0.60436 0.60436 0.60436 0.60436 0.57695 0.57695 0.57695 coh11:k=1.446,b=0 0.70828 0.70828 0.70828 0.70828 0.70828 0.70828 0.67882 0.64937 0.64937 0.61992 0.61992 0.61992 coh12:k=1.54624,b=0 0.78887 0.78887 0.78887 0.78887 0.78887 0.75737 0.72588 0.72588 0.69439 0.69439 0.69439 0.69439 coh13:k=1.64647,b=0 0.87354 0.87354 0.87354 0.84 0.84 0.84 0.80647 0.80647 0.77294 0.77294 0.7394 0.7394 coh14:k=1.74671,b=0 0.96229 0.92672 0.92672 0.92672 0.92672 0.89114 0.85557 0.85557 0.81999 0.81999 0.81999 0.81999 coh15:k=1.84694,b=0 1.0175 1.0175 1.0175 1.0175 0.9799 0.9799 0.94228 0.90466 0.90466 0.90466 0.90466 0.86705 coh16:k=1.94718,b=0 1.1124 1.1124 1.0727 1.0727 1.0727 1.0727 0.99342 0.99342 0.99342 0.95376 0.95376 0.9141 coh17:k=2.04742,b=0 1.1697 1.1697 1.1697 1.1697 1.128 1.128 1.0863 1.0446 1.0446 1.0029 1.0029 1.0029 coh18:k=2.14765,b=0 1.2707 1.2269 1.2269 1.2269 1.2269 1.1832 1.1394 1.1394 1.0957 1.0957 1.0957 1.0957 coh19:k=2.24789,b=0 1.33 1.33 1.33 1.2842 1.2842 1.2842 1.1926 1.1926 1.1926 1.1926 1.1468 1.1468 coh20:k=2.34812,b=0 1.3893 1.3893 1.3893 1.3893 1.3893 1.3414 1.2936 1.2936 1.2458 1.2458 1.198 1.198 coh21:k=2.44836,b=0 1.4984 1.4984 1.4984 1.4486 1.4486 1.4486 1.3488 1.3488 1.299 1.299 1.299 1.299 coh22:k=2.54859,b=0 1.5598 1.5598 1.5598 1.5079 1.5079 1.5079 1.456 1.4041 1.4041 1.4041 1.3522 1.3522 coh23:k=2.64883,b=0 1.6751 1.6211 1.6211 1.6211 1.6211 1.5672 1.5132 1.5132 1.4593 1.4593 1.4053 1.4053 coh24:k=2.74906,b=0 1.7385 1.7385 1.6825 1.6825 1.6825 1.6825 1.5705 1.5705 1.5705 1.5145 1.5145 1.5145 coh25:k=2.8493,b=0 1.8019 1.8019 1.8019 1.8019 1.7438 1.7438 1.6858 1.6278 1.6278 1.6278 1.5697 1.5697 coh26:k=2.94953,b=0 1.8652 1.8652 1.8652 1.8652 1.8052 1.8052 1.7451 1.7451 1.685 1.685 1.625 1.625 coh27:k=3.04977,b=0 1.9908 1.9908 1.9286 1.9286 1.9286 1.8665 1.8044 1.8044 1.8044 1.7423 1.7423 1.6802 coh28:k=3.15001,b=0 2.0562 2.0562 1.992 1.992 1.992 1.992 1.8637 1.8637 1.8637 1.7996 1.7996 1.7996 coh29:k=3.25024,b=0 2.1216 2.1216 2.1216 2.1216 2.0554 2.0554 1.9892 1.923 1.923 1.923 1.8568 1.8568 coh30:k=3.35048,b=0 2.187 2.187 2.187 2.187 2.1188 2.1188 2.0506 2.0506 1.9823 1.9823 1.9141 1.9141 coh31:k=3.45071,b=0 2.3228 2.2525 2.2525 2.2525 2.2525 2.1822 2.1119 2.1119 2.0416 2.0416 2.0416 1.9713 coh32:k=3.55095,b=0 2.3902 2.3902 2.3179 2.3179 2.3179 2.3179 2.1733 2.1733 2.1733 2.1009 2.1009 2.1009 coh33:k=3.65118,b=0 2.4577 2.4577 2.4577 2.3833 2.3833 2.3833 2.309 2.2346 2.2346 2.2346 2.1602 2.1602 coh34:k=3.75142,b=0 2.5252 2.5252 2.5252 2.5252 2.4488 2.4488 2.3724 2.2959 2.2959 2.2959 2.2195 2.2195 coh35:k=3.85165,b=0 2.6711 2.5926 2.5926 2.5926 2.5926 2.5142 2.4357 2.4357 2.3573 2.3573 2.3573 2.2788 coh36:k=3.95189,b=0 2.7406 2.7406 2.6601 2.6601 2.6601 2.5796 2.4991 2.4991 2.4186 2.4186 2.4186 2.3381 coh37:k=4.05212,b=0 2.8101 2.8101 2.7276 2.7276 2.7276 2.7276 2.5625 2.5625 2.5625 2.48 2.48 2.48 coh38:k=4.15236,b=0 2.8796 2.8796 2.8796 2.795 2.795 2.795 2.7105 2.6259 2.6259 2.6259 2.5413 2.5413 coh39:k=4.2526,b=0 2.9491 2.9491 2.9491 2.8625 2.8625 2.8625 2.7759 2.6893 2.6893 2.6893 2.6027 2.6027 coh40:k=4.35283,b=0 3.0186 3.0186 3.0186 3.0186 2.93 2.93 2.8413 2.8413 2.7527 2.7527 2.7527 2.664 coh41:k=4.45307,b=0 3.1789 3.0882 3.0882 3.0882 3.0882 2.9975 2.9068 2.9068 2.8161 2.8161 2.8161 2.7254 coh42:k=4.5533,b=0 3.2504 3.2504 3.1577 3.1577 3.1577 3.0649 2.9722 2.9722 2.9722 2.8795 2.8795 2.8795 coh43:k=4.65354,b=0 3.322 3.322 3.2272 3.2272 3.2272 3.2272 3.0376 3.0376 3.0376 2.9428 2.9428 2.9428 coh44:k=4.75377,b=0 3.3935 3.3935 3.3935 3.2967 3.2967 3.2967 3.1999 3.103 3.103 3.0062 3.0062 3.0062 coh45:k=4.85401,b=0 3.4651 3.4651 3.4651 3.3662 3.3662 3.3662 3.2673 3.1685 3.1685 3.1685 3.0696 3.0696 coh46:k=4.95424,b=0 3.5366 3.5366 3.5366 3.5366 3.4357 3.4357 3.3348 3.3348 3.2339 3.2339 3.133 3.133 coh47:k=5.05448,b=0 3.7111 3.6082 3.6082 3.6082 3.5052 3.5052 3.4023 3.4023 3.2993 3.2993 3.2993 3.1964 coh48:k=5.15471,b=0 3.7847 3.6797 3.6797 3.6797 3.6797 3.5747 3.4698 3.4698 3.3648 3.3648 3.3648 3.2598 coh49:k=5.25495,b=0 3.8583 3.8583 3.7513 3.7513 3.7513 3.6443 3.5372 3.5372 3.5372 3.4302 3.4302 3.4302 coh50:k=5.35519,b=0 3.9319 3.9319 3.8228 3.8228 3.8228 3.7138 3.6047 3.6047 3.6047 3.4956 3.4956 3.4956 coh519:k=52.3656,b=0 46.981 46.981 46.981 46.981 46.981 46.981 46.981 46.981 46.981 46.981 46.981 46.981 coh520:k=52.4658,b=0 47.07 47.07 47.07 47.07 47.07 47.07 47.07 47.07 47.07 47.07 47.07 47.07 coh521:k=52.5661,b=0 47.16 47.16 47.16 47.16 47.16 47.16 47.16 47.16 47.16 47.16 47.16 47.16 coh522:k=52.6663,b=0 47.25 47.25 47.25 47.25 47.25 47.25 47.25 47.25 47.25 47.25 47.25 47.25 coh523:k=52.7666,b=0 47.34 47.34 47.34 47.34 47.34 47.34 47.34 47.34 47.34 47.34 47.34 47.34 coh524:k=52.8668,b=0 47.43 47.43 47.43 47.43 47.43 47.43 47.43 47.43 47.43 47.43 47.43 47.43 coh525:k=52.967,b=0 47.52 47.52 47.52 47.52 47.52 47.52 47.52 47.52 47.52 47.52 47.52 47.52 coh526:k=53.0673,b=0 47.61 47.61 47.61 47.61 47.61 47.61 47.61 47.61 47.61 47.61 47.61 47.61 coh527:k=53.1675,b=0 47.7 47.7 47.7 47.7 47.7 47.7 47.7 47.7 47.7 47.7 47.7 47.7 coh528:k=53.2677,b=0 47.79 47.79 47.79 47.79 47.79 47.79 47.79 47.79 47.79 47.79 47.79 47.79 coh529:k=53.368,b=0 47.88 47.88 47.88 47.88 47.88 47.88 47.88 47.88 47.88 47.88 47.88 47.88 coh530:k=53.4682,b=0 47.97 47.97 47.97 47.97 47.97 47.97 47.97 47.97 47.97 47.97 47.97 47.97 coh531:k=53.5684,b=0 49.151 48.06 48.06 48.06 48.06 48.06 48.06 48.06 48.06 48.06 48.06 48.06 coh532:k=53.6687,b=0 49.243 48.15 48.15 48.15 48.15 48.15 48.15 48.15 48.15 48.15 48.15 48.15 coh533:k=53.7689,b=0 49.335 48.239 48.239 48.239 48.239 48.239 48.239 48.239 48.239 48.239 48.239 48.239 coh534:k=53.8691,b=0 49.427 48.329 48.329 48.329 48.329 48.329 48.329 48.329 48.329 48.329 48.329 48.329 coh535:k=53.9694,b=0 49.519 48.419 48.419 48.419 48.419 48.419 48.419 48.419 48.419 48.419 48.419 48.419 coh536:k=54.0696,b=0 49.611 48.509 48.509 48.509 48.509 48.509 48.509 48.509 48.509 48.509 48.509 48.509 coh537:k=54.1698,b=0 49.702 48.599 48.599 48.599 48.599 48.599 48.599 48.599 48.599 48.599 48.599 48.599 coh538:k=54.2701,b=0 49.794 48.689 48.689 48.689 48.689 48.689 48.689 48.689 48.689 48.689 48.689 48.689 coh539:k=54.3703,b=0 49.886 49.886 48.779 48.779 48.779 48.779 48.779 48.779 48.779 48.779 48.779 48.779 coh540:k=54.4706,b=0 49.978 49.978 48.869 48.869 48.869 48.869 48.869 48.869 48.869 48.869 48.869 48.869 coh541:k=54.5708,b=0 48.959 48.959 48.959 48.959 48.959 48.959 48.959 48.959 48.959 48.959 48.959 48.959 coh542:k=54.671,b=0 49.049 49.049 49.049 49.049 49.049 49.049 49.049 49.049 49.049 49.049 49.049 49.049 coh543:k=54.7713,b=0 49.139 49.139 49.139 49.139 49.139 49.139 49.139 49.139 49.139 49.139 49.139 49.139 coh544:k=54.8715,b=0 49.229 49.229 49.229 49.229 49.229 49.229 49.229 49.229 49.229 49.229 49.229 49.229 coh545:k=54.9717,b=0 49.319 49.319 49.319 49.319 49.319 49.319 49.319 49.319 49.319 49.319 49.319 49.319 coh546:k=55.072,b=0 49.409 49.409 49.409 49.409 49.409 49.409 49.409 49.409 49.409 49.409 49.409 49.409 coh547:k=55.1722,b=0 49.498 49.498 49.498 49.498 49.498 49.498 49.498 49.498 49.498 49.498 49.498 49.498 coh548:k=55.2724,b=0 49.588 49.588 49.588 49.588 49.588 49.588 49.588 49.588 49.588 49.588 49.588 49.588 coh549:k=55.3727,b=0 49.678 49.678 49.678 49.678 49.678 49.678 49.678 49.678 49.678 49.678 49.678 49.678 coh550:k=55.4729,b=0 49.768 49.768 49.768 49.768 49.768 49.768 49.768 49.768 49.768 49.768 49.768 49.768 coh551:k=55.5731,b=0 49.858 49.858 49.858 49.858 49.858 49.858 49.858 49.858 49.858 49.858 49.858 49.858 coh552:k=55.6734,b=0 49.948 49.948 49.948 49.948 49.948 49.948 49.948 49.948 49.948 49.948 49.948 49.948 coh553:k=55.7736,b=0 50.038 50.038 50.038 50.038 50.038 50.038 50.038 50.038 50.038 50.038 50.038 48.902 coh554:k=55.8739,b=0 50.128 50.128 50.128 50.128 50.128 50.128 50.128 50.128 50.128 50.128 48.99 48.99 coh555:k=55.9741,b=0 50.218 50.218 50.218 50.218 50.218 50.218 50.218 50.218 49.078 49.078 49.078 49.078 coh556:k=56.0743,b=0 50.308 50.308 50.308 50.308 49.166 49.166 49.166 49.166 49.166 49.166 49.166 49.166 coh557:k=56.1746,b=0 49.254 49.254 49.254 49.254 49.254 49.254 49.254 49.254 49.254 49.254 49.254 49.254 coh558:k=56.2748,b=0 49.342 49.342 49.342 49.342 49.342 49.342 49.342 49.342 49.342 49.342 49.342 49.342 coh559:k=56.375,b=0 49.429 49.429 49.429 49.429 49.429 49.429 49.429 49.429 49.429 49.429 49.429 49.429 coh560:k=56.4753,b=0 49.517 49.517 49.517 49.517 49.517 49.517 49.517 49.517 49.517 49.517 49.517 49.517 coh561:k=56.5755,b=0 49.605 49.605 49.605 49.605 49.605 49.605 49.605 49.605 49.605 49.605 49.605 49.605 coh562:k=56.6757,b=0 49.693 49.693 49.693 49.693 49.693 49.693 49.693 49.693 49.693 49.693 49.693 49.693 coh563:k=56.776,b=0 49.781 49.781 49.781 49.781 49.781 49.781 49.781 49.781 49.781 49.781 49.781 49.781 coh564:k=56.8762,b=0 49.869 49.869 49.869 49.869 49.869 49.869 49.869 49.869 49.869 49.869 49.869 49.869 coh565:k=56.9764,b=0 49.957 49.957 49.957 49.957 49.957 49.957 49.957 49.957 49.957 49.957 49.957 49.957 coh566:k=57.0767,b=0 50.045 50.045 50.045 50.045 50.045 50.045 50.045 50.045 50.045 50.045 50.045 50.045 coh567:k=57.1769,b=0 50.132 50.132 50.132 50.132 50.132 50.132 50.132 50.132 50.132 50.132 50.132 50.132 coh568:k=57.2771,b=0 50.22 50.22 50.22 50.22 50.22 50.22 50.22 50.22 50.22 50.22 50.22 50.22
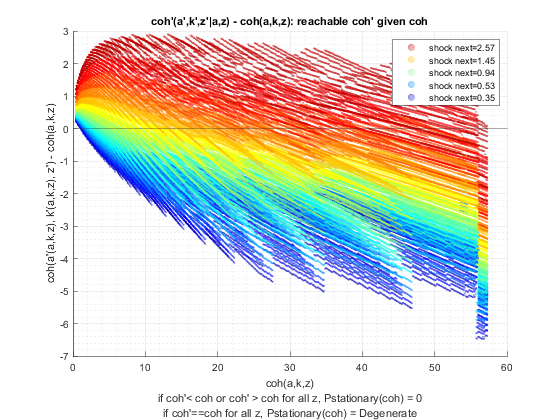
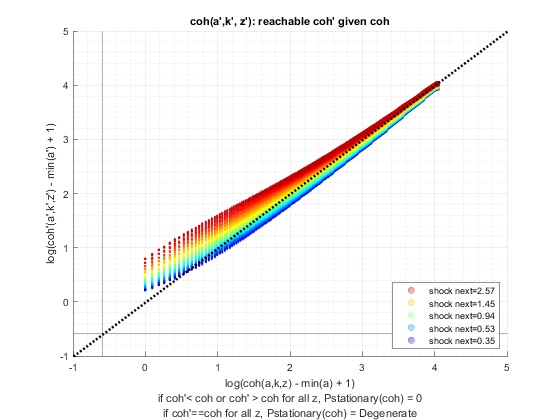
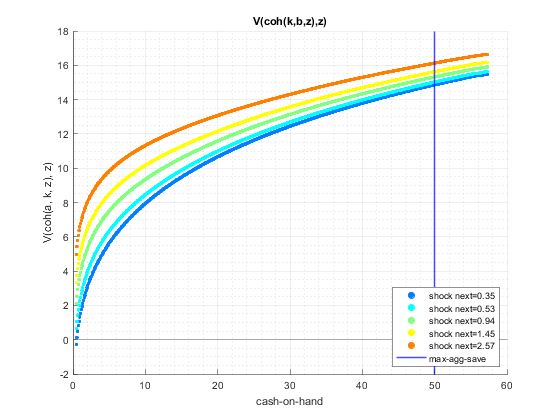
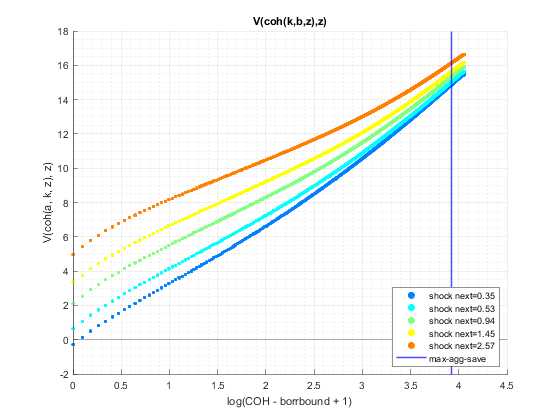
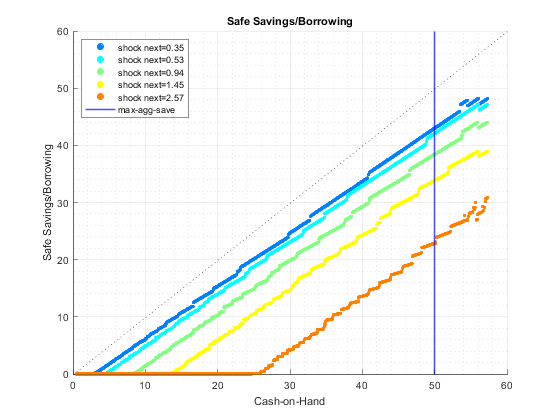
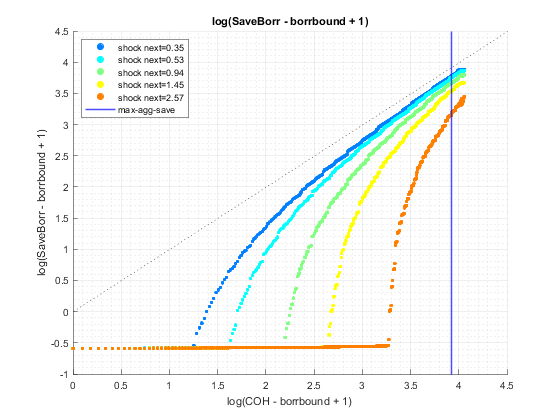
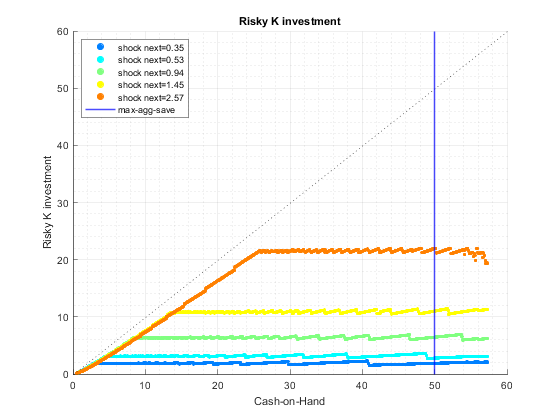
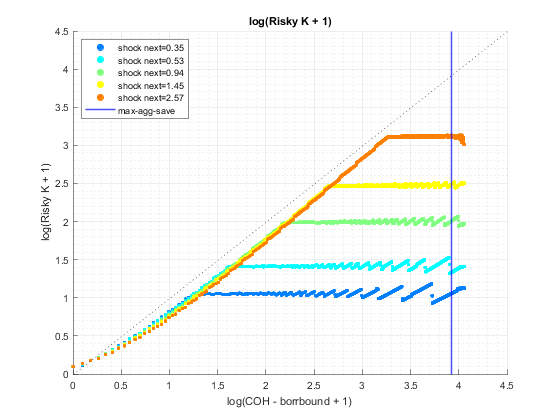
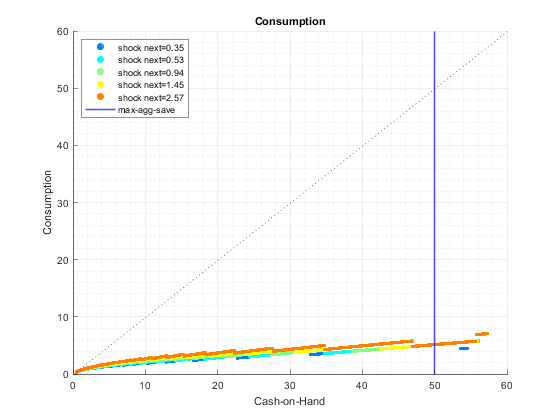
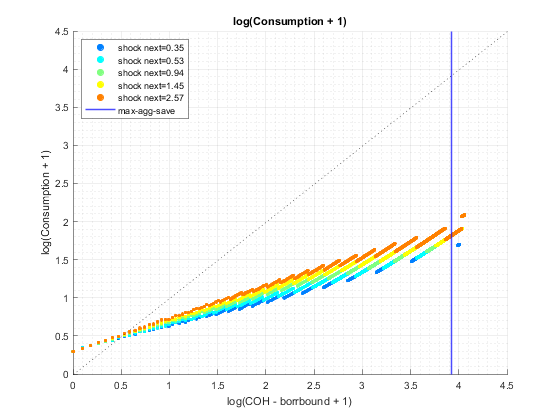
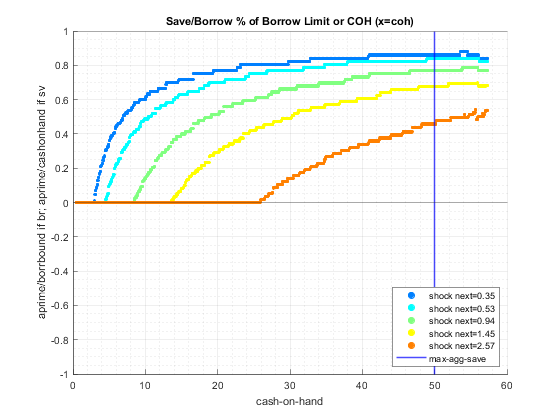
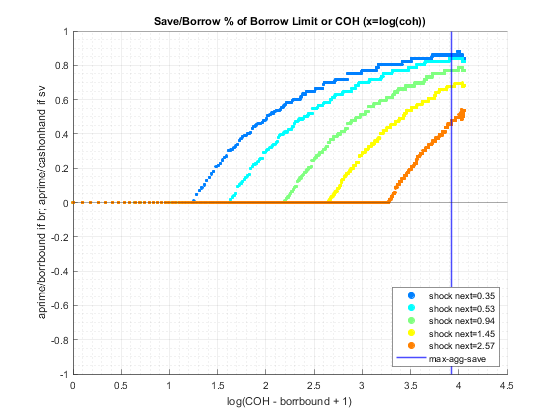
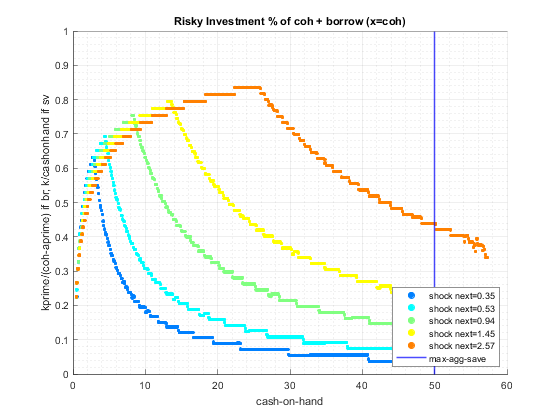
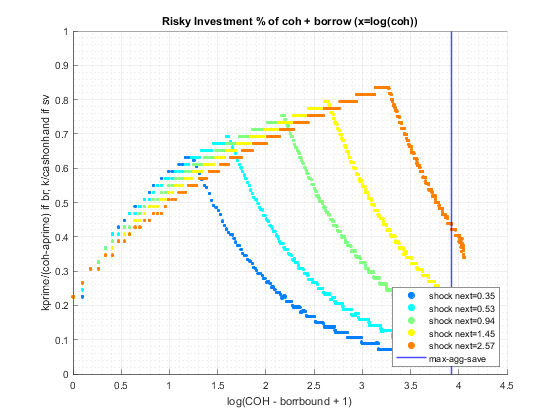
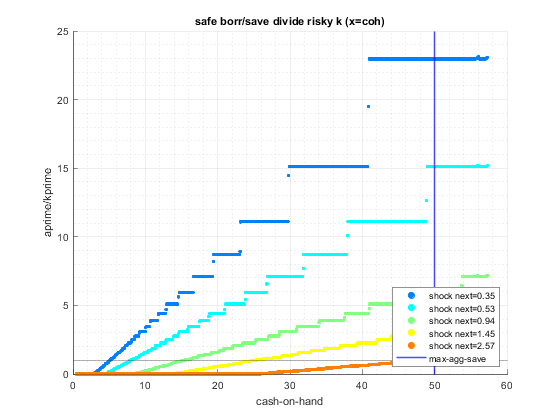
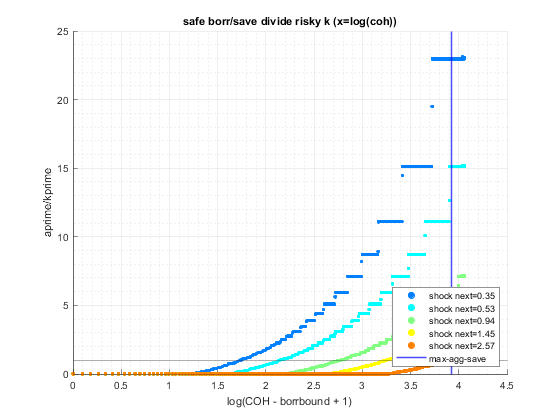
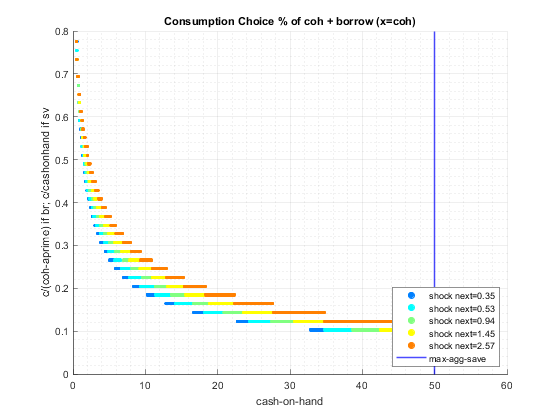
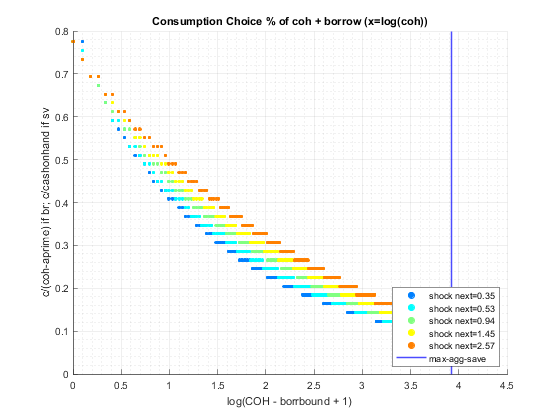
Display Various Containers
if (bl_display_defparam)
Display 1 support_map
fft_container_map_display(support_map, it_display_summmat_rowmax, it_display_summmat_colmax);
---------------------------------------- ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Begin: Show all key and value pairs from container CONTAINER NAME: SUPPORT_MAP ---------------------------------------- Map with properties: Count: 43 KeyType: char ValueType: any xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ---------------------------------------- ---------------------------------------- pos = 29 ; key = st_img_name_main ; val = ff_ipwkz_vf_vecsv_default pos = 30 ; key = st_img_path ; val = C:/Users/fan/CodeDynaAsset//m_ipwkz//solve/img/ pos = 31 ; key = st_img_prefix ; val = pos = 32 ; key = st_img_suffix ; val = _p4.png pos = 33 ; key = st_mat_name_main ; val = ff_ipwkz_vf_vecsv_default pos = 34 ; key = st_mat_path ; val = C:/Users/fan/CodeDynaAsset//m_ipwkz//solve/mat/ pos = 35 ; key = st_mat_prefix ; val = pos = 36 ; key = st_mat_suffix ; val = _p4 pos = 37 ; key = st_mat_test_path ; val = C:/Users/fan/CodeDynaAsset//m_ipwkz//test/ff_ipwkz_ds_vecsv/mat/ pos = 38 ; key = st_matimg_path_root ; val = C:/Users/fan/CodeDynaAsset//m_ipwkz/ pos = 39 ; key = st_profile_name_main ; val = ff_ipwkz_vf_vecsv_default pos = 40 ; key = st_profile_path ; val = C:/Users/fan/CodeDynaAsset//m_ipwkz//solve/profile/ pos = 41 ; key = st_profile_prefix ; val = pos = 42 ; key = st_profile_suffix ; val = _p4 pos = 43 ; key = st_title_prefix ; val = ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Scalars in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx value __ ___ _____ bl_display 1 1 0 bl_display_defparam 2 2 1 bl_display_dist 3 3 0 bl_display_evf 4 4 0 bl_display_final 5 5 1 bl_display_final_dist 6 6 0 bl_display_final_dist_detail 7 7 0 bl_display_funcgrids 8 8 0 bl_graph 9 9 1 bl_graph_coh_t_coh 10 10 1 bl_graph_evf 11 11 0 bl_graph_funcgrids 12 12 0 bl_graph_funcgrids_detail 13 13 0 bl_graph_onebyones 14 14 1 bl_graph_pol_lvl 15 15 1 bl_graph_pol_pct 16 16 1 bl_graph_val 17 17 1 bl_img_save 18 18 0 bl_mat 19 19 0 bl_post 20 20 1 bl_profile 21 21 0 bl_profile_dist 22 22 0 bl_time 23 23 0 it_display_every 24 24 5 it_display_final_colmax 25 25 12 it_display_final_rowmax 26 26 100 it_display_summmat_colmax 27 27 7 it_display_summmat_rowmax 28 28 7
Display 2 armt_map
fft_container_map_display(armt_map, it_display_summmat_rowmax, it_display_summmat_colmax);
---------------------------------------- ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Begin: Show all key and value pairs from container CONTAINER NAME: ARMT_MAP ---------------------------------------- Map with properties: Count: 18 KeyType: char ValueType: any xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ---------------------------------------- ---------------------------------------- ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Matrix in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx rowN colN mean std min max __ ___ _____ __________ ________ ________ __________ _______ ar_a_meshk 1 1 568 1 28.86 16.45 0.44365 57.277 ar_ak_perc 2 2 1 50 0.5 0.2969 0.001 0.999 ar_interp_c_grid 3 3 1 5.7257e+05 28.649 16.529 0.02 57.277 ar_interp_coh_grid 4 4 1 568 28.86 16.45 0.44365 57.277 ar_k_mesha 5 5 568 1 0 0 0 0 ar_stationary 6 6 1 15 0.066667 0.060897 0.0027089 0.16757 ar_w_level 7 7 1 500 25 14.477 0 50 ar_w_perc 8 8 1 50 0.5 0.2969 0.001 0.999 ar_z 9 9 1 15 1.1347 0.69878 0.34741 2.567 mt_coh_wkb 10 11 568 15 28.86 16.436 0.44365 57.277 mt_interp_coh_grid_mesh_w_perc 11 12 50 568 28.86 16.436 0.44365 57.277 mt_interp_coh_grid_mesh_z 12 13 568 15 28.86 16.436 0.44365 57.277 mt_k 13 14 50 500 12.5 11.152 0 49.95 mt_w_by_interp_coh_interp_grid 14 15 50 568 14.43 12.76 0.00044365 57.22 mt_z_mesh_coh_interp_grid 15 16 568 15 1.1347 0.67512 0.34741 2.567 mt_z_mesh_coh_wkb 16 17 25000 15 1.1347 0.67508 0.34741 2.567 mt_z_trans 17 18 15 15 0.066667 0.095337 0 0.27902 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Scalars in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx value _ ___ _____ it_ameshk_n 1 10 568
Display 3 param_map
fft_container_map_display(param_map, it_display_summmat_rowmax, it_display_summmat_colmax);
---------------------------------------- ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Begin: Show all key and value pairs from container CONTAINER NAME: PARAM_MAP ---------------------------------------- Map with properties: Count: 32 KeyType: char ValueType: any xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ---------------------------------------- ---------------------------------------- pos = 31 ; key = st_analytical_stationary_type ; val = eigenvector pos = 32 ; key = st_model ; val = ipwkz ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Scalars in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx value __ ___ _______ fl_Amean 1 1 1 fl_alpha 2 2 0.36 fl_b_bd 3 3 0 fl_beta 4 4 0.94 fl_c_min 5 5 0.02 fl_coh_interp_grid_gap 6 6 0.1 fl_crra 7 7 1.5 fl_delta 8 8 0.08 fl_k_max 9 9 50 fl_k_min 10 10 0 fl_nan_replace 11 11 -9999 fl_r_borr 12 12 0.095 fl_r_save 13 13 0.025 fl_tol_dist 14 14 1e-05 fl_tol_pol 15 15 1e-05 fl_tol_val 16 16 1e-05 fl_w 17 17 0.44365 fl_w_interp_grid_gap 18 18 0.1 fl_w_max 19 19 50 fl_w_min 20 20 0 fl_z_mu 21 21 0 fl_z_rho 22 22 0.8 fl_z_sig 23 23 0.2 it_ak_perc_n 24 24 50 it_c_interp_grid_gap 25 25 0.0001 it_maxiter_dist 26 26 1000 it_maxiter_val 27 27 250 it_tol_pol_nochange 28 28 25 it_w_perc_n 29 29 50 it_z_n 30 30 15
Display 4 func_map
fft_container_map_display(func_map, it_display_summmat_rowmax, it_display_summmat_colmax);
---------------------------------------- ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Begin: Show all key and value pairs from container CONTAINER NAME: FUNC_MAP ---------------------------------------- Map with properties: Count: 7 KeyType: char ValueType: any xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ---------------------------------------- ---------------------------------------- pos = 1 ; key = f_coh ; val = @(z,b,k)(f_prod(z,k)+k*(1-fl_delta)+fl_w+b.*(1+fl_r_save).*(b>0)+b.*(1+fl_r_borr).*(b<=0)) pos = 2 ; key = f_cons ; val = @(coh,bprime,kprime)(coh-kprime-bprime) pos = 3 ; key = f_inc ; val = @(z,b,k)(f_prod(z,k)-(fl_delta)*k+fl_w+b.*(fl_r_save).*(b>0)+b.*(fl_r_borr).*(b<=0)) pos = 4 ; key = f_prod ; val = @(z,k)((fl_Amean.*(z)).*(k.^(fl_alpha))) pos = 5 ; key = f_util_crra ; val = @(c)(((c).^(1-fl_crra)-1)./(1-fl_crra)) pos = 6 ; key = f_util_log ; val = @(c)log(c) pos = 7 ; key = f_util_standin ; val = @(z,b,k)f_util_log(f_coh(z,b,k).*(f_coh(z,b,k)>0)+fl_c_min.*(f_coh(z,b,k)<=0)) ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Scalars in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx xFunction _ ___ _________ f_coh 1 1 1 f_cons 2 2 2 f_inc 3 3 3 f_prod 4 4 4 f_util_crra 5 5 5 f_util_log 6 6 6 f_util_standin 7 7 7
Display 5 result_map
fft_container_map_display(result_map, it_display_summmat_rowmax, it_display_summmat_colmax);
---------------------------------------- ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Begin: Show all key and value pairs from container CONTAINER NAME: RESULT_MAP ---------------------------------------- Map with properties: Count: 15 KeyType: char ValueType: any xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ---------------------------------------- ---------------------------------------- pos = 2 ; key = ar_st_pol_names ; val = cl_mt_val cl_mt_coh cl_mt_pol_a cl_mt_pol_k cl_mt_pol_c ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Matrix in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx rowN colN mean std min max __ ___ ____ ____ _______ _______ __________ ______ ar_pol_diff_norm 1 1 104 1 33.694 171.03 0 1633.1 ar_val_diff_norm 2 3 104 1 11.341 23.836 0.06118 142.93 cl_mt_coh 3 4 568 15 28.86 16.436 0.44365 57.277 cl_mt_cons 4 5 568 15 3.6763 1.3777 0.34381 7.0568 cl_mt_pol_a 5 6 568 15 17.983 14.578 9.9839e-05 48.221 cl_mt_pol_c 6 7 568 15 3.6763 1.3777 0.34381 7.0568 cl_mt_pol_k 7 8 568 15 7.201 5.5098 0.099739 22 cl_mt_val 8 9 568 15 12.365 3.0296 -0.27805 16.631 mt_pol_idx 9 10 568 15 14217 8202.7 12 28394 mt_pol_perc_change 10 11 104 15 0.10707 0.26316 0 1 mt_val 11 12 568 15 12.365 3.0296 -0.27805 16.631 tb_pol_a 12 13 100 12 20.065 20.519 9.9839e-05 48.221 tb_val 13 14 100 12 10.877 5.1818 -0.27805 16.631 tb_valpol_alliter 14 15 100 17 2.8456 43.325 0 1633.1
end
end
ans = Map with properties: Count: 15 KeyType: char ValueType: any