Generate States, Choices and Shocks Grids and Get Functions (Borr + Save)
back to Fan's Dynamic Assets Repository Table of Content.
Contents
- FFS_ABZ_GET_FUNCGRID get funcs, params, states choices shocks grids
- Default
- Parse Parameters 1
- Parse Parameters 2
- Parse Parameters 3
- Get Shock: Income Shock (ar1)
- Get Shock: Interest Rate Shock (iid)
- Get Shock: Mesh Shocks Together
- Get Equations
- Get Asset and Choice Grid
- Store
- Graph: A, Shocks, COH, and Defaults
- Display
function [armt_map, func_map] = ffs_abz_get_funcgrid(varargin)
FFS_ABZ_GET_FUNCGRID get funcs, params, states choices shocks grids
centralized gateway for retrieving parameters, and solution grids and functions. Generate asset grid, and shock grid that accomandates two shocks jointly: one ar1 wage shock, one iid interest rate shock.
@param param_map container parameter container
@param support_map container support container
@param bl_input_override boolean if true varargin contained param_map and support_map fully overrides local default. Local default is not invoked. This could be important for speed if this function is getting invoked within certain loops. Default is 0.
@return armt_map container container with states, choices and shocks grids that are inputs for grid based solution algorithm
@return func_map container container with function handles for consumption cash-on-hand etc.
@example
it_param_set = 2; bl_input_override = true; [param_map, support_map] = ffs_abz_set_default_param(it_param_set); [armt_map, func_map] = ffs_abz_get_funcgrid(param_map, support_map, bl_input_override);
@include
@seealso
- initialize parameters: ffs_abz_set_default_param
- initialize functions: ffs_abz_set_functions
- set asset grid: ffs_abz_gen_borrsave_grid
- set shock borrow rate: fft_gen_discrete_var
- set shock wage: ffto_gen_tauchen_jhl
- gateway function processing grid, paramters, functions: ffs_abz_get_funcgrid
Default
if (~isempty(varargin)) % override when called from outside [param_map, support_map] = varargin{:}; else close all % default internal run it_param_set = 4; [param_map, support_map] = ffs_abz_set_default_param(it_param_set); support_map('bl_graph_funcgrids') = true; support_map('bl_display_funcgrids') = true; default_maps = {param_map, support_map}; % numvarargs is the number of varagin inputted [default_maps{1:length(varargin)}] = varargin{:}; param_map = [param_map; default_maps{1}]; support_map = [support_map; default_maps{2}]; end
Parse Parameters 1
% param_map asset grid params_group = values(param_map, {'fl_b_bd', 'bl_default', 'fl_a_min', 'fl_a_max', 'bl_loglin', 'fl_loglin_threshold', 'it_a_n'}); [fl_b_bd, bl_default, fl_a_min, fl_a_max, bl_loglin, fl_loglin_threshold, it_a_n] = params_group{:}; % param_map preference params_group = values(param_map, {'fl_crra', 'fl_c_min'}); [fl_crra, fl_c_min] = params_group{:}; % param_map borrowing and price params_group = values(param_map, {'bl_b_is_principle', 'fl_r_save', 'fl_w'}); [bl_b_is_principle, fl_r_save, fl_w] = params_group{:};
Parse Parameters 2
% param_map shock income params_group = values(param_map, {'it_z_wage_n', 'fl_z_wage_mu', 'fl_z_wage_rho', 'fl_z_wage_sig'}); [it_z_wage_n, fl_z_wage_mu, fl_z_wage_rho, fl_z_wage_sig] = params_group{:}; % param_map shock borrowing interest params_group = values(param_map, {'st_z_r_borr_drv_ele_type', 'st_z_r_borr_drv_prb_type', 'fl_z_r_borr_poiss_mean', ... 'fl_z_r_borr_max', 'fl_z_r_borr_min', 'fl_z_r_borr_n'}); [st_z_r_borr_drv_ele_type, st_z_r_borr_drv_prb_type, fl_z_r_borr_poiss_mean, ... fl_z_r_borr_max, fl_z_r_borr_min, fl_z_r_borr_n] = params_group{:};
Parse Parameters 3
% support_map controls params_group = values(support_map, {'bl_graph_funcgrids', 'bl_display_funcgrids'}); [bl_graph_funcgrids, bl_display_funcgrids] = params_group{:}; params_group = values(support_map, {'it_display_summmat_rowmax', 'it_display_summmat_colmax'}); [it_display_summmat_rowmax, it_display_summmat_colmax] = params_group{:};
Get Shock: Income Shock (ar1)
[~, mt_z_wage_trans, ~, ar_z_wage] = ffto_gen_tauchen_jhl(fl_z_wage_mu,fl_z_wage_rho,fl_z_wage_sig,it_z_wage_n);
Get Shock: Interest Rate Shock (iid)
% get borrowing grid and probabilities param_dsv_map = containers.Map('KeyType','char', 'ValueType','any'); param_dsv_map('st_drv_ele_type') = st_z_r_borr_drv_ele_type; param_dsv_map('st_drv_prb_type') = st_z_r_borr_drv_prb_type; param_dsv_map('fl_poiss_mean') = fl_z_r_borr_poiss_mean; param_dsv_map('fl_max') = fl_z_r_borr_max; param_dsv_map('fl_min') = fl_z_r_borr_min; param_dsv_map('fl_n') = fl_z_r_borr_n; [ar_z_r_borr, ar_z_r_borr_prob] = fft_gen_discrete_var(param_dsv_map, true); % iid transition matrix mt_z_r_borr_prob_trans = repmat(ar_z_r_borr_prob, [length(ar_z_r_borr_prob), 1]);
Get Shock: Mesh Shocks Together
% Kronecker product to get full transition matrix for the two shocks mt_z_trans = kron(mt_z_r_borr_prob_trans, mt_z_wage_trans); % mesh the shock vectors [mt_z_wage_mesh_r_borr, mt_z_r_borr_mesh_wage] = ndgrid(ar_z_wage, ar_z_r_borr); ar_z_r_borr_mesh_wage = mt_z_r_borr_mesh_wage(:)'; ar_z_wage_mesh_r_borr = mt_z_wage_mesh_r_borr(:)'; if (bl_display_funcgrids) disp('----------------------------------------'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp('Borrow R Shock: ar_z_r_borr_mesh_wage'); disp('Prod/Wage Shock: mt_z_wage_mesh_r_borr'); disp('show which shock is inner and which is outter'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); tb_two_shocks = array2table([ar_z_r_borr_mesh_wage;... ar_z_wage_mesh_r_borr]'); cl_col_names = ["Borrow R Shock (Meshed)", "Wage R Shock (Meshed)"]; cl_row_names = strcat('zi=', string((1:length(ar_z_r_borr_mesh_wage)))); tb_two_shocks.Properties.VariableNames = matlab.lang.makeValidName(cl_col_names); tb_two_shocks.Properties.RowNames = matlab.lang.makeValidName(cl_row_names); it_row_display = it_z_wage_n*2; disp(size(tb_two_shocks)); disp(head(tb_two_shocks, it_row_display)); disp(tail(tb_two_shocks, it_row_display)); disp('----------------------------------------'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp('Borrow Rate Transition Matrix: mt_z_r_borr_prob_trans'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); it_col_n_keep = 15; it_row_n_keep = 15; [it_row_n, it_col_n] = size(mt_z_r_borr_prob_trans); [ar_it_cols, ar_it_rows] = fft_row_col_subset(it_col_n, it_col_n_keep, it_row_n, it_row_n_keep); cl_st_full_rowscols = cellstr([num2str(ar_z_r_borr', 'r%3.2f')]); tb_z_r_borr_prob_trans = array2table(round(mt_z_r_borr_prob_trans(ar_it_rows, ar_it_cols), 6)); cl_col_names = strcat('zi=', num2str(ar_it_cols'), ':', cl_st_full_rowscols(ar_it_cols)); cl_row_names = strcat('zi=', num2str(ar_it_rows'), ':', cl_st_full_rowscols(ar_it_cols)); tb_z_r_borr_prob_trans.Properties.VariableNames = matlab.lang.makeValidName(cl_col_names); tb_z_r_borr_prob_trans.Properties.RowNames = matlab.lang.makeValidName(cl_row_names); disp(size(tb_z_r_borr_prob_trans)); disp(tb_z_r_borr_prob_trans); disp('----------------------------------------'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp('Wage Prod Shock Transition Matrix: mt_z_r_borr_prob_trans'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); it_col_n_keep = 15; it_row_n_keep = 15; [it_row_n, it_col_n] = size(mt_z_wage_trans); [ar_it_cols, ar_it_rows] = fft_row_col_subset(it_col_n, it_col_n_keep, it_row_n, it_row_n_keep); cl_st_full_rowscols = cellstr([num2str(ar_z_wage', 'w%3.2f')]); tb_z_wage_trans = array2table(round(mt_z_wage_trans(ar_it_rows, ar_it_cols),6)); cl_col_names = strcat('zi=', num2str(ar_it_cols'), ':', cl_st_full_rowscols(ar_it_cols)); cl_row_names = strcat('zi=', num2str(ar_it_rows'), ':', cl_st_full_rowscols(ar_it_cols)); tb_z_wage_trans.Properties.VariableNames = matlab.lang.makeValidName(cl_col_names); tb_z_wage_trans.Properties.RowNames = matlab.lang.makeValidName(cl_row_names); disp(size(tb_z_wage_trans)); disp(tb_z_wage_trans); disp('----------------------------------------'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp('Full Transition Matrix: mt_z_trans'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); it_col_n_keep = it_z_wage_n*3; it_row_n_keep = it_z_wage_n*3; [it_row_n, it_col_n] = size(mt_z_trans); [ar_it_cols, ar_it_rows] = fft_row_col_subset(it_col_n, it_col_n_keep, it_row_n, it_row_n_keep); cl_st_full_rowscols = cellstr([num2str(ar_z_r_borr_mesh_wage', 'r%3.2f;'), ... num2str(ar_z_wage_mesh_r_borr', 'w%3.2f')]); tb_mt_z_trans = array2table(round(mt_z_trans(ar_it_rows, ar_it_cols),6)); cl_col_names = strcat('i', num2str(ar_it_cols'), ':', cl_st_full_rowscols(ar_it_cols)); cl_row_names = strcat('i', num2str(ar_it_rows'), ':', cl_st_full_rowscols(ar_it_cols)); tb_mt_z_trans.Properties.VariableNames = matlab.lang.makeValidName(cl_col_names); tb_mt_z_trans.Properties.RowNames = matlab.lang.makeValidName(cl_row_names); disp(size(tb_mt_z_trans)); disp(tb_mt_z_trans); end
---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Borrow R Shock: ar_z_r_borr_mesh_wage Prod/Wage Shock: mt_z_wage_mesh_r_borr show which shock is inner and which is outter xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx 55 2 BorrowRShock_Meshed_ WageRShock_Meshed_ ____________________ __________________ zi_1 0.025 0.34664 zi_2 0.025 0.42338 zi_3 0.025 0.51712 zi_4 0.025 0.63162 zi_5 0.025 0.77146 zi_6 0.025 0.94226 zi_7 0.025 1.1509 zi_8 0.025 1.4057 zi_9 0.025 1.7169 zi_10 0.025 2.097 zi_11 0.025 2.5613 zi_12 0.0425 0.34664 zi_13 0.0425 0.42338 zi_14 0.0425 0.51712 zi_15 0.0425 0.63162 zi_16 0.0425 0.77146 zi_17 0.0425 0.94226 zi_18 0.0425 1.1509 zi_19 0.0425 1.4057 zi_20 0.0425 1.7169 zi_21 0.0425 2.097 zi_22 0.0425 2.5613 BorrowRShock_Meshed_ WageRShock_Meshed_ ____________________ __________________ zi_34 0.0775 0.34664 zi_35 0.0775 0.42338 zi_36 0.0775 0.51712 zi_37 0.0775 0.63162 zi_38 0.0775 0.77146 zi_39 0.0775 0.94226 zi_40 0.0775 1.1509 zi_41 0.0775 1.4057 zi_42 0.0775 1.7169 zi_43 0.0775 2.097 zi_44 0.0775 2.5613 zi_45 0.095 0.34664 zi_46 0.095 0.42338 zi_47 0.095 0.51712 zi_48 0.095 0.63162 zi_49 0.095 0.77146 zi_50 0.095 0.94226 zi_51 0.095 1.1509 zi_52 0.095 1.4057 zi_53 0.095 1.7169 zi_54 0.095 2.097 zi_55 0.095 2.5613 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Borrow Rate Transition Matrix: mt_z_r_borr_prob_trans xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx 5 5 zi_1_r0_03 zi_2_r0_04 zi_3_r0_06 zi_4_r0_08 zi_5_r0_10 __________ __________ __________ __________ __________ zi_1_r0_03 0.001552 0.01552 0.0776 0.25866 0.64666 zi_2_r0_04 0.001552 0.01552 0.0776 0.25866 0.64666 zi_3_r0_06 0.001552 0.01552 0.0776 0.25866 0.64666 zi_4_r0_08 0.001552 0.01552 0.0776 0.25866 0.64666 zi_5_r0_10 0.001552 0.01552 0.0776 0.25866 0.64666 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Wage Prod Shock Transition Matrix: mt_z_r_borr_prob_trans xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx 11 11 zi_1_w0_35 zi_2_w0_42 zi_3_w0_52 zi_4_w0_63 zi_5_w0_77 zi_6_w0_94 zi_7_w1_15 zi_8_w1_41 zi_9_w1_72 zi_10_w2_10 zi_11_w2_56 __________ __________ __________ __________ __________ __________ __________ __________ __________ ___________ ___________ zi_1_w0_35 0.30854 0.38293 0.24173 0.060598 0.005977 0.000229 3e-06 0 0 0 0 zi_2_w0_42 0.0968 0.28529 0.37595 0.1974 0.041098 0.003359 0.000106 1e-06 0 0 0 zi_3_w0_52 0.017864 0.1178 0.32451 0.35577 0.15534 0.026851 0.001818 4.8e-05 0 0 0 zi_4_w0_63 0.001866 0.026851 0.15534 0.35577 0.32451 0.1178 0.016897 0.000947 2e-05 0 0 zi_5_w0_77 0.000108 0.003359 0.041098 0.1974 0.37595 0.28529 0.086076 0.010241 0.000475 8e-06 0 zi_6_w0_94 3e-06 0.000229 0.005977 0.060598 0.24173 0.38293 0.24173 0.060598 0.005977 0.000229 3e-06 zi_7_w1_15 0 8e-06 0.000475 0.010241 0.086076 0.28529 0.37595 0.1974 0.041098 0.003359 0.000108 zi_8_w1_41 0 0 2e-05 0.000947 0.016897 0.1178 0.32451 0.35577 0.15534 0.026851 0.001866 zi_9_w1_72 0 0 0 4.8e-05 0.001818 0.026851 0.15534 0.35577 0.32451 0.1178 0.017864 zi_10_w2_10 0 0 0 1e-06 0.000106 0.003359 0.041098 0.1974 0.37595 0.28529 0.0968 zi_11_w2_56 0 0 0 0 3e-06 0.000229 0.005977 0.060598 0.24173 0.38293 0.30854 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Full Transition Matrix: mt_z_trans xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx 33 33 i1_r0_03_w0_35 i2_r0_03_w0_42 i3_r0_03_w0_52 i4_r0_03_w0_63 i5_r0_03_w0_77 i6_r0_03_w0_94 i7_r0_03_w1_15 i8_r0_03_w1_41 i9_r0_03_w1_72 i10_r0_03_w2_10 i11_r0_03_w2_56 i12_r0_04_w0_35 i13_r0_04_w0_42 i14_r0_04_w0_52 i15_r0_04_w0_63 i16_r0_04_w0_77 i28_r0_06_w0_94 i40_r0_08_w1_15 i41_r0_08_w1_41 i42_r0_08_w1_72 i43_r0_08_w2_10 i44_r0_08_w2_56 i45_r0_10_w0_35 i46_r0_10_w0_42 i47_r0_10_w0_52 i48_r0_10_w0_63 i49_r0_10_w0_77 i50_r0_10_w0_94 i51_r0_10_w1_15 i52_r0_10_w1_41 i53_r0_10_w1_72 i54_r0_10_w2_10 i55_r0_10_w2_56 ______________ ______________ ______________ ______________ ______________ ______________ ______________ ______________ ______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ i1_r0_03_w0_35 0.000479 0.000594 0.000375 9.4e-05 9e-06 0 0 0 0 0 0 0.004788 0.005943 0.003752 0.00094 9.3e-05 1.8e-05 1e-06 0 0 0 0 0.19952 0.24762 0.15632 0.039186 0.003865 0.000148 2e-06 0 0 0 0 i2_r0_03_w0_42 0.00015 0.000443 0.000583 0.000306 6.4e-05 5e-06 0 0 0 0 0 0.001502 0.004428 0.005835 0.003064 0.000638 0.000261 2.8e-05 0 0 0 0 0.062597 0.18449 0.24311 0.12765 0.026577 0.002172 6.9e-05 1e-06 0 0 0 i3_r0_03_w0_52 2.8e-05 0.000183 0.000504 0.000552 0.000241 4.2e-05 3e-06 0 0 0 0 0.000277 0.001828 0.005036 0.005521 0.002411 0.002084 0.00047 1.2e-05 0 0 0 0.011552 0.076178 0.20985 0.23006 0.10046 0.017363 0.001175 3.1e-05 0 0 0 i4_r0_03_w0_63 3e-06 4.2e-05 0.000241 0.000552 0.000504 0.000183 2.6e-05 1e-06 0 0 0 2.9e-05 0.000417 0.002411 0.005521 0.005036 0.009141 0.004371 0.000245 5e-06 0 0 0.001207 0.017363 0.10046 0.23006 0.20985 0.076178 0.010927 0.000612 1.3e-05 0 0 i5_r0_03_w0_77 0 5e-06 6.4e-05 0.000306 0.000583 0.000443 0.000134 1.6e-05 1e-06 0 0 2e-06 5.2e-05 0.000638 0.003064 0.005835 0.022138 0.022265 0.002649 0.000123 2e-06 0 7e-05 0.002172 0.026577 0.12765 0.24311 0.18449 0.055662 0.006622 0.000307 5e-06 0 i6_r0_03_w0_94 0 0 9e-06 9.4e-05 0.000375 0.000594 0.000375 9.4e-05 9e-06 0 0 0 4e-06 9.3e-05 0.00094 0.003752 0.029715 0.062527 0.015674 0.001546 5.9e-05 1e-06 2e-06 0.000148 0.003865 0.039186 0.15632 0.24762 0.15632 0.039186 0.003865 0.000148 2e-06 i7_r0_03_w1_15 0 0 1e-06 1.6e-05 0.000134 0.000443 0.000583 0.000306 6.4e-05 5e-06 0 0 0 7e-06 0.000159 0.001336 0.022138 0.097245 0.05106 0.010631 0.000869 2.8e-05 0 5e-06 0.000307 0.006622 0.055662 0.18449 0.24311 0.12765 0.026577 0.002172 7e-05 i8_r0_03_w1_41 0 0 0 1e-06 2.6e-05 0.000183 0.000504 0.000552 0.000241 4.2e-05 3e-06 0 0 0 1.5e-05 0.000262 0.009141 0.083938 0.092025 0.040182 0.006945 0.000483 0 0 1.3e-05 0.000612 0.010927 0.076178 0.20985 0.23006 0.10046 0.017363 0.001207 i9_r0_03_w1_72 0 0 0 0 3e-06 4.2e-05 0.000241 0.000552 0.000504 0.000183 2.8e-05 0 0 0 1e-06 2.8e-05 0.002084 0.040182 0.092025 0.083938 0.030471 0.004621 0 0 0 3.1e-05 0.001175 0.017363 0.10046 0.23006 0.20985 0.076178 0.011552 i10_r0_03_w2_10 0 0 0 0 0 5e-06 6.4e-05 0.000306 0.000583 0.000443 0.00015 0 0 0 0 2e-06 0.000261 0.010631 0.05106 0.097245 0.073794 0.025039 0 0 0 1e-06 6.9e-05 0.002172 0.026577 0.12765 0.24311 0.18449 0.062597 i11_r0_03_w2_56 0 0 0 0 0 0 9e-06 9.4e-05 0.000375 0.000594 0.000479 0 0 0 0 0 1.8e-05 0.001546 0.015674 0.062527 0.099049 0.079808 0 0 0 0 2e-06 0.000148 0.003865 0.039186 0.15632 0.24762 0.19952 i12_r0_04_w0_35 0.000479 0.000594 0.000375 9.4e-05 9e-06 0 0 0 0 0 0 0.004788 0.005943 0.003752 0.00094 9.3e-05 1.8e-05 1e-06 0 0 0 0 0.19952 0.24762 0.15632 0.039186 0.003865 0.000148 2e-06 0 0 0 0 i13_r0_04_w0_42 0.00015 0.000443 0.000583 0.000306 6.4e-05 5e-06 0 0 0 0 0 0.001502 0.004428 0.005835 0.003064 0.000638 0.000261 2.8e-05 0 0 0 0 0.062597 0.18449 0.24311 0.12765 0.026577 0.002172 6.9e-05 1e-06 0 0 0 i14_r0_04_w0_52 2.8e-05 0.000183 0.000504 0.000552 0.000241 4.2e-05 3e-06 0 0 0 0 0.000277 0.001828 0.005036 0.005521 0.002411 0.002084 0.00047 1.2e-05 0 0 0 0.011552 0.076178 0.20985 0.23006 0.10046 0.017363 0.001175 3.1e-05 0 0 0 i15_r0_04_w0_63 3e-06 4.2e-05 0.000241 0.000552 0.000504 0.000183 2.6e-05 1e-06 0 0 0 2.9e-05 0.000417 0.002411 0.005521 0.005036 0.009141 0.004371 0.000245 5e-06 0 0 0.001207 0.017363 0.10046 0.23006 0.20985 0.076178 0.010927 0.000612 1.3e-05 0 0 i16_r0_04_w0_77 0 5e-06 6.4e-05 0.000306 0.000583 0.000443 0.000134 1.6e-05 1e-06 0 0 2e-06 5.2e-05 0.000638 0.003064 0.005835 0.022138 0.022265 0.002649 0.000123 2e-06 0 7e-05 0.002172 0.026577 0.12765 0.24311 0.18449 0.055662 0.006622 0.000307 5e-06 0 i28_r0_06_w0_94 0 0 9e-06 9.4e-05 0.000375 0.000594 0.000375 9.4e-05 9e-06 0 0 0 4e-06 9.3e-05 0.00094 0.003752 0.029715 0.062527 0.015674 0.001546 5.9e-05 1e-06 2e-06 0.000148 0.003865 0.039186 0.15632 0.24762 0.15632 0.039186 0.003865 0.000148 2e-06 i40_r0_08_w1_15 0 0 1e-06 1.6e-05 0.000134 0.000443 0.000583 0.000306 6.4e-05 5e-06 0 0 0 7e-06 0.000159 0.001336 0.022138 0.097245 0.05106 0.010631 0.000869 2.8e-05 0 5e-06 0.000307 0.006622 0.055662 0.18449 0.24311 0.12765 0.026577 0.002172 7e-05 i41_r0_08_w1_41 0 0 0 1e-06 2.6e-05 0.000183 0.000504 0.000552 0.000241 4.2e-05 3e-06 0 0 0 1.5e-05 0.000262 0.009141 0.083938 0.092025 0.040182 0.006945 0.000483 0 0 1.3e-05 0.000612 0.010927 0.076178 0.20985 0.23006 0.10046 0.017363 0.001207 i42_r0_08_w1_72 0 0 0 0 3e-06 4.2e-05 0.000241 0.000552 0.000504 0.000183 2.8e-05 0 0 0 1e-06 2.8e-05 0.002084 0.040182 0.092025 0.083938 0.030471 0.004621 0 0 0 3.1e-05 0.001175 0.017363 0.10046 0.23006 0.20985 0.076178 0.011552 i43_r0_08_w2_10 0 0 0 0 0 5e-06 6.4e-05 0.000306 0.000583 0.000443 0.00015 0 0 0 0 2e-06 0.000261 0.010631 0.05106 0.097245 0.073794 0.025039 0 0 0 1e-06 6.9e-05 0.002172 0.026577 0.12765 0.24311 0.18449 0.062597 i44_r0_08_w2_56 0 0 0 0 0 0 9e-06 9.4e-05 0.000375 0.000594 0.000479 0 0 0 0 0 1.8e-05 0.001546 0.015674 0.062527 0.099049 0.079808 0 0 0 0 2e-06 0.000148 0.003865 0.039186 0.15632 0.24762 0.19952 i45_r0_10_w0_35 0.000479 0.000594 0.000375 9.4e-05 9e-06 0 0 0 0 0 0 0.004788 0.005943 0.003752 0.00094 9.3e-05 1.8e-05 1e-06 0 0 0 0 0.19952 0.24762 0.15632 0.039186 0.003865 0.000148 2e-06 0 0 0 0 i46_r0_10_w0_42 0.00015 0.000443 0.000583 0.000306 6.4e-05 5e-06 0 0 0 0 0 0.001502 0.004428 0.005835 0.003064 0.000638 0.000261 2.8e-05 0 0 0 0 0.062597 0.18449 0.24311 0.12765 0.026577 0.002172 6.9e-05 1e-06 0 0 0 i47_r0_10_w0_52 2.8e-05 0.000183 0.000504 0.000552 0.000241 4.2e-05 3e-06 0 0 0 0 0.000277 0.001828 0.005036 0.005521 0.002411 0.002084 0.00047 1.2e-05 0 0 0 0.011552 0.076178 0.20985 0.23006 0.10046 0.017363 0.001175 3.1e-05 0 0 0 i48_r0_10_w0_63 3e-06 4.2e-05 0.000241 0.000552 0.000504 0.000183 2.6e-05 1e-06 0 0 0 2.9e-05 0.000417 0.002411 0.005521 0.005036 0.009141 0.004371 0.000245 5e-06 0 0 0.001207 0.017363 0.10046 0.23006 0.20985 0.076178 0.010927 0.000612 1.3e-05 0 0 i49_r0_10_w0_77 0 5e-06 6.4e-05 0.000306 0.000583 0.000443 0.000134 1.6e-05 1e-06 0 0 2e-06 5.2e-05 0.000638 0.003064 0.005835 0.022138 0.022265 0.002649 0.000123 2e-06 0 7e-05 0.002172 0.026577 0.12765 0.24311 0.18449 0.055662 0.006622 0.000307 5e-06 0 i50_r0_10_w0_94 0 0 9e-06 9.4e-05 0.000375 0.000594 0.000375 9.4e-05 9e-06 0 0 0 4e-06 9.3e-05 0.00094 0.003752 0.029715 0.062527 0.015674 0.001546 5.9e-05 1e-06 2e-06 0.000148 0.003865 0.039186 0.15632 0.24762 0.15632 0.039186 0.003865 0.000148 2e-06 i51_r0_10_w1_15 0 0 1e-06 1.6e-05 0.000134 0.000443 0.000583 0.000306 6.4e-05 5e-06 0 0 0 7e-06 0.000159 0.001336 0.022138 0.097245 0.05106 0.010631 0.000869 2.8e-05 0 5e-06 0.000307 0.006622 0.055662 0.18449 0.24311 0.12765 0.026577 0.002172 7e-05 i52_r0_10_w1_41 0 0 0 1e-06 2.6e-05 0.000183 0.000504 0.000552 0.000241 4.2e-05 3e-06 0 0 0 1.5e-05 0.000262 0.009141 0.083938 0.092025 0.040182 0.006945 0.000483 0 0 1.3e-05 0.000612 0.010927 0.076178 0.20985 0.23006 0.10046 0.017363 0.001207 i53_r0_10_w1_72 0 0 0 0 3e-06 4.2e-05 0.000241 0.000552 0.000504 0.000183 2.8e-05 0 0 0 1e-06 2.8e-05 0.002084 0.040182 0.092025 0.083938 0.030471 0.004621 0 0 0 3.1e-05 0.001175 0.017363 0.10046 0.23006 0.20985 0.076178 0.011552 i54_r0_10_w2_10 0 0 0 0 0 5e-06 6.4e-05 0.000306 0.000583 0.000443 0.00015 0 0 0 0 2e-06 0.000261 0.010631 0.05106 0.097245 0.073794 0.025039 0 0 0 1e-06 6.9e-05 0.002172 0.026577 0.12765 0.24311 0.18449 0.062597 i55_r0_10_w2_56 0 0 0 0 0 0 9e-06 9.4e-05 0.000375 0.000594 0.000479 0 0 0 0 0 1.8e-05 0.001546 0.015674 0.062527 0.099049 0.079808 0 0 0 0 2e-06 0.000148 0.003865 0.039186 0.15632 0.24762 0.19952
Get Equations
[f_util_log, f_util_crra, f_util_standin, f_coh, f_cons_coh, f_cons_checkcmin] = ...
ffs_abz_set_functions(fl_crra, fl_c_min, fl_r_save, fl_w);
Get Asset and Choice Grid
note this requires ar_z
if (bl_loglin) % C:\Users\fan\M4Econ\asset\grid\ff_grid_loglin.m ar_a = fft_gen_grid_loglin(it_a_n, fl_a_max, fl_a_min, fl_loglin_threshold); else fl_r_borr_max = max(ar_z_r_borr); [ar_a, fl_borr_yminbd, fl_borr_ymaxbd] = ffs_abz_gen_borrsave_grid(... fl_b_bd, bl_default, ar_z_wage, fl_w, ... bl_b_is_principle, fl_r_borr_max, fl_a_min, fl_a_max, it_a_n); end
Store
armt_map = containers.Map('KeyType','char', 'ValueType','any'); armt_map('ar_a') = ar_a; armt_map('mt_z_trans') = mt_z_trans; armt_map('ar_z_r_borr_mesh_wage') = ar_z_r_borr_mesh_wage; armt_map('ar_z_wage_mesh_r_borr') = ar_z_wage_mesh_r_borr; armt_map('ar_z_r_borr') = ar_z_r_borr; armt_map('ar_z_r_borr_prob') = ar_z_r_borr_prob; % Exo. Prob func_map = containers.Map('KeyType','char', 'ValueType','any'); func_map('f_util_log') = f_util_log; func_map('f_util_crra') = f_util_crra; func_map('f_util_standin') = f_util_standin; func_map('f_coh') = f_coh; func_map('f_cons_coh') = f_cons_coh; func_map('f_cons_checkcmin') = f_cons_checkcmin;
Graph: A, Shocks, COH, and Defaults
- y-axis : coh(a,z)
- x-axis : a
- color: z
- overlay: coh points points where there is default.
if (bl_graph_funcgrids) % mesh a and and z [mt_a_mesh_z, mt_z_mesh_a] = ndgrid(ar_a, ar_z_wage); % cash-on-hand given a and z mt_coh = f_coh(mt_z_mesh_a, mt_a_mesh_z); % loop over level vs log graphs for sub_j=1:1:1 figure('PaperPosition', [0 0 7 4]); if (sub_j == 1) x_mat = mt_a_mesh_z; y_mat = mt_coh; st_title = 'coh(a,z)'; st_ylabel = 'Cash-on-hand(a, z)'; st_xlabel = 'Asset States (Choices)'; fl_b_bd_graph = fl_b_bd; fl_borr_yminbd_graph = fl_borr_yminbd; fl_borr_ymaxbd_graph = fl_borr_ymaxbd; else x_mat = log(mt_a_mesh_z - min(min(mt_a_mesh_z)) + 1); y_mat = log(mt_coh - min(min(mt_coh)) + 1); st_title = 'coh(a,z) log scale'; st_ylabel = 'log(Cash-on-hand(a, z) - min(coh) + 1)'; st_xlabel = 'log(a - min(a) + 1)'; fl_b_bd_graph = log(fl_b_bd - min(min(mt_a_mesh_z)) + 1); fl_borr_yminbd_graph = log(fl_borr_yminbd - min(min(mt_a_mesh_z)) + 1); fl_borr_ymaxbd_graph = log(fl_borr_ymaxbd - min(min(mt_a_mesh_z)) + 1); end % plot main x and y chart = plot(x_mat, y_mat, 'blue'); % add color based on z clr = jet(numel(chart)); for m = 1:numel(chart) set(chart(m), 'Color', clr(m,:)) end % if (length(ar_w_level_full) <= 100) % scatter(ar_a_meshk, ar_k_mesha, 3, 'filled', ... % 'MarkerEdgeColor', 'b', 'MarkerFaceColor', 'b'); % end % if (length(ar_w_level_full) <= 100) % gf_invalid_scatter = scatter(ar_a_meshk_full(ar_bl_wkb_invalid),... % ar_k_mesha_full(ar_bl_wkb_invalid),... % 20, 'O', 'MarkerEdgeColor', 'black', 'MarkerFaceColor', 'black'); % end % add various borrowing bound lines % add 0 lines xline(0); yline(0); % add 45 degrees line hline = refline([1 0]); hline.Color = 'k'; hline.LineStyle = ':'; hline.HandleVisibility = 'off'; hline.LineWidth = 2.5; title(st_title) ylabel(st_ylabel) grid on; grid minor; legend2plot = fliplr([1 round(numel(chart)/3) round((2*numel(chart))/4) numel(chart)]); legendCell = cellstr(num2str(ar_z_wage', 'z=%3.2f')); chart(length(chart)+1) = hline; legendCell{length(legendCell) + 1} = 'if coh(a,z) >= a'; legend2plot = [legend2plot length(legendCell)]; % if borrow plot additional borrowing bound lines if (fl_b_bd >= 0 ) ar_legend_ele = [legend2plot]; xlabel({st_xlabel}) else % add fl_b_bd exo borrow line if (fl_b_bd >= min(ar_a)) xline_borrbound = xline(fl_b_bd_graph); xline_borrbound.HandleVisibility = 'on'; xline_borrbound.LineStyle = '-'; xline_borrbound.Color = 'black'; xline_borrbound.LineWidth = 2.5; yline_borrbound = yline(fl_b_bd_graph); yline_borrbound.HandleVisibility = 'off'; yline_borrbound.LineStyle = '-'; yline_borrbound.Color = 'black'; yline_borrbound.LineWidth = 1; end xline_yminbd = xline(fl_borr_yminbd_graph); xline_yminbd.HandleVisibility = 'on'; xline_yminbd.LineStyle = '--'; xline_yminbd.Color = 'red'; xline_yminbd.LineWidth = 2.5; yline_yminbd = yline(fl_borr_yminbd_graph); yline_yminbd.HandleVisibility = 'off'; yline_yminbd.LineStyle = '--'; yline_yminbd.Color = 'red'; yline_yminbd.LineWidth = 1; if (bl_default) xline_ymaxbd = xline(fl_borr_ymaxbd_graph); xline_ymaxbd.HandleVisibility = 'on'; xline_ymaxbd.LineStyle = '--'; xline_ymaxbd.Color = 'blue'; xline_ymaxbd.LineWidth = 2.5; yline_ymaxbd = yline(fl_borr_ymaxbd_graph); yline_ymaxbd.HandleVisibility = 'on'; yline_ymaxbd.LineStyle = ':'; yline_ymaxbd.Color = 'blue'; yline_ymaxbd.LineWidth = 2.5; end % add bound line legends it_addlines_cn = 0; if (fl_b_bd >= min(ar_a)) it_addlines_cn = it_addlines_cn + 1; chart(length(chart)+1) = xline_borrbound; legendCell{length(legendCell) + 1} = 'exo. borrow bound fbbd'; end it_addlines_cn = it_addlines_cn + 1; chart(length(chart)+1) = xline_yminbd; legendCell{length(legendCell) + 1} = 'neg min inc: -zmin*w/r (no default)'; if (bl_default) it_addlines_cn = it_addlines_cn + 1; chart(length(chart)+1) = xline_ymaxbd; legendCell{length(legendCell) + 1} = 'neg max inc: -zmax*w/r (default)'; it_addlines_cn = it_addlines_cn + 1; chart(length(chart)+1) = yline_ymaxbd; legendCell{length(legendCell) + 1} = 'must default if coh(a,z)<dot-line'; end % draw legend ar_legend_ele = [legend2plot length(legendCell)-it_addlines_cn:1:length(legendCell)]; xlabel({st_xlabel 'if coh(a,z) < a, then a''(a,z)<a'}) end % draw legends legend(chart(unique(ar_legend_ele)), legendCell(unique(ar_legend_ele)), 'Location', 'northwest'); end end
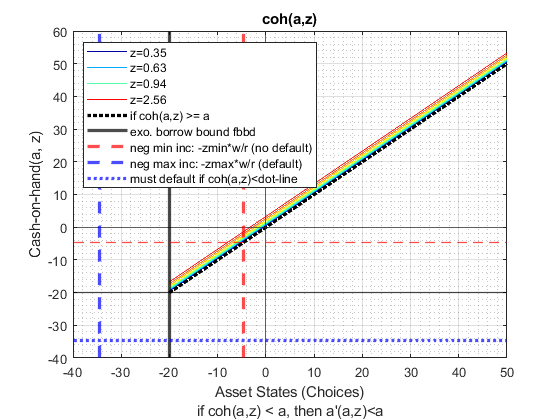
Display
if (bl_display_funcgrids) disp('ar_z_wage'); disp(size(ar_z_wage)); disp(ar_z_wage); disp('ar_z_r_borr'); disp(size(ar_z_r_borr)); disp(ar_z_r_borr); fft_container_map_display(armt_map, it_display_summmat_rowmax, it_display_summmat_colmax); fft_container_map_display(func_map, it_display_summmat_rowmax, it_display_summmat_colmax); end
ar_z_wage 1 11 Columns 1 through 7 0.3466 0.4234 0.5171 0.6316 0.7715 0.9423 1.1509 Columns 8 through 11 1.4057 1.7169 2.0970 2.5613 ar_z_r_borr 1 5 0.0250 0.0425 0.0600 0.0775 0.0950 ---------------------------------------- ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Begin: Show all key and value pairs from container CONTAINER NAME: ARMT_MAP ---------------------------------------- Map with properties: Count: 6 KeyType: char ValueType: any xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ---------------------------------------- ---------------------------------------- ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Matrix in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx rowN colN mean std min max _ ___ ____ ____ ________ ________ ________ _______ ar_a 1 1 1 750 15 20.248 -20 50 ar_z_r_borr 2 2 1 5 0.06 0.02767 0.025 0.095 ar_z_r_borr_mesh_wage 3 3 1 55 0.06 0.024977 0.025 0.095 ar_z_r_borr_prob 4 4 1 5 0.2 0.26988 0.001552 0.64666 ar_z_wage_mesh_r_borr 5 5 1 55 1.1422 0.70079 0.34664 2.5613 mt_z_trans 6 6 55 55 0.018182 0.046336 0 0.24762 ---------------------------------------- ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Begin: Show all key and value pairs from container CONTAINER NAME: FUNC_MAP ---------------------------------------- Map with properties: Count: 6 KeyType: char ValueType: any xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ---------------------------------------- ---------------------------------------- pos = 1 ; key = f_coh ; val = @(z,b)(z*fl_w+b) pos = 2 ; key = f_cons_checkcmin ; val = @(fl_r_borr,z,b,bprime)((f_coh_princ(fl_r_borr,z,b)-bprime).*((f_coh_princ(fl_r_borr,z,b)-bprime)>=fl_c_min)+fl_c_min.*((f_coh_princ(fl_r_borr,z,b)-bprime)<fl_c_min)) pos = 3 ; key = f_cons_coh ; val = @(coh,fl_r_borr,bprime)(coh-(bprime.*(1/(1+fl_r_save)).*(bprime>0)+bprime.*(1/(1+fl_r_borr)).*(bprime<=0))) pos = 4 ; key = f_util_crra ; val = @(c)(((c).^(1-fl_crra)-1)./(1-fl_crra)) pos = 5 ; key = f_util_log ; val = @(c)log(c) pos = 6 ; key = f_util_standin ; val = @(fl_r_borr,z,b)f_util_log(f_coh_princ(fl_r_borr,z,b).*(f_coh_princ(fl_r_borr,z,b)>0)+fl_c_min.*(f_coh_princ(fl_r_borr,z,b)<=0)) ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Scalars in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx xFunction _ ___ _________ f_coh 1 1 1 f_cons_checkcmin 2 2 2 f_cons_coh 3 3 3 f_util_crra 4 4 4 f_util_log 5 5 5 f_util_standin 6 6 6
end
ans = Map with properties: Count: 6 KeyType: char ValueType: any