Tabulate Value and Policy Iteration Results, Store to Mat, Graph Results (Risky + Safe Asset)
back to Fan's Dynamic Assets Repository Table of Content.
Contents
function [result_map] = ff_akz_vf_post(varargin)
FF_AKZ_VF_POST post ff_akz_vf graphs, tables, mats.
Given the solution form ff_akz_vf, graphs, tables, mats. Graphing code is separately in ff_akz_vf_post_graph.m. Run this function directly with randomly generates matrixes for graph/table testings.
@param param_map container parameter container
@param support_map container support container
@param armt_map container container with states, choices and shocks grids that are inputs for grid based solution algorithm
@param func_map container container with function handles for consumption cash-on-hand etc.
@param result_map container contains policy function matrix, value function matrix, iteration results
@return result_map container add coh consumption and other matrixes to result_map also add table versions of val pol and iter matries
@example
bl_input_override = true; result_map = containers.Map('KeyType','char', 'ValueType','any'); result_map('mt_val') = mt_val; result_map('mt_pol_a') = mt_pol_a; result_map('mt_pol_k') = mt_pol_k; result_map('ar_val_diff_norm') = ar_val_diff_norm(1:it_iter_last); result_map('ar_pol_diff_norm') = ar_pol_diff_norm(1:it_iter_last); result_map('mt_pol_perc_change') = mt_pol_perc_change(1:it_iter_last, :); result_map = ff_akz_vf_post(param_map, support_map, armt_map, func_map, result_map, bl_input_override);
@include
Default
if (~isempty(varargin)) % if invoked from outside overrid fully [param_map, support_map, armt_map, func_map, result_map] = varargin{:}; params_group = values(result_map, {'mt_val', 'cl_mt_pol_a', 'cl_mt_pol_k'}); [mt_val, cl_mt_pol_a, cl_mt_pol_k] = params_group{:}; [mt_pol_a, mt_pol_k] = deal(cl_mt_pol_a{1}, cl_mt_pol_k{1}); params_group = values(result_map, {'ar_val_diff_norm', 'ar_pol_diff_norm', 'mt_pol_perc_change'}); [ar_val_diff_norm, ar_pol_diff_norm, mt_pol_perc_change] = params_group{:}; % Get Parameters params_group = values(param_map, {'it_z_n'}); [it_z_n] = params_group{:}; params_group = values(armt_map, {'ar_a_meshk', 'ar_k_mesha', 'mt_coh_wkb', 'it_ameshk_n'}); [ar_a_meshk, ar_k_mesha, mt_coh_wkb, it_ameshk_n] = params_group{:}; else clear all; close all; % internal invoke for testing it_param_set = 4; bl_input_override = true; % Get Parameters [param_map, support_map] = ffs_akz_set_default_param(it_param_set); [armt_map, func_map] = ffs_akz_get_funcgrid(param_map, support_map, bl_input_override); % 1 for override % Generate Default val and policy matrixes params_group = values(param_map, {'it_maxiter_val', 'it_z_n'}); [it_maxiter_val, it_z_n] = params_group{:}; params_group = values(armt_map, {'ar_a_meshk', 'ar_k_mesha', 'ar_z', 'mt_coh_wkb', 'it_ameshk_n'}); [ar_a_meshk, ar_k_mesha, ar_z, mt_coh_wkb, it_ameshk_n] = params_group{:}; params_group = values(func_map, {'f_util_standin', 'f_cons', 'f_coh'}); [f_util_standin, f_cons, f_coh] = params_group{:}; % Set Defaults mt_val = f_util_standin(ar_z, ar_a_meshk, ar_k_mesha); mt_pol_aksum = mt_coh_wkb.*(cumsum(sort(ar_z))/sum(ar_z)*0.4 + 0.4); mt_pol_a = mt_pol_aksum.*(0.7 - cumsum(sort(ar_z))/sum(ar_z)*0.3); mt_pol_k = mt_pol_aksum - mt_pol_a; it_iter_max = min(it_maxiter_val, 50); ar_val_diff_norm = rand([it_iter_max, 1]); ar_pol_diff_norm = rand([it_iter_max, 1]); mt_pol_perc_change = rand([it_iter_max, it_z_n]); % Set Results Map result_map = containers.Map('KeyType','char', 'ValueType','any'); result_map('mt_val') = mt_val; result_map('cl_mt_pol_a') = {mt_pol_a, zeros(1)}; result_map('cl_mt_pol_k') = {mt_pol_k, zeros(1)}; end
Parse Parameter
% Get Parameters params_group = values(param_map, {'st_model'}); [st_model] = params_group{:}; % armt_map if (ismember(st_model, ["ipwkbzr", "ipwkbzr_fibs"])) if (ismember(st_model, ["ipwkbzr"])) params_group = values(armt_map, {'ar_z_r_borr_mesh_wage_w1r2', 'ar_z_wage_mesh_r_borr_w1r2'}); [ar_z_r_borr_mesh_wage_w1r2, ar_z_wage_mesh_r_borr_w1r2] = params_group{:}; params_group = values(param_map, {'fl_z_r_borr_n'}); [fl_z_r_borr_n] = params_group{:}; elseif (ismember(st_model, ["ipwkbzr_fibs"])) params_group = values(armt_map, {'ar_z_r_infbr_mesh_wage_w1r2', 'ar_z_wage_mesh_r_infbr_w1r2'}); [ar_z_r_borr_mesh_wage_w1r2, ar_z_wage_mesh_r_borr_w1r2] = params_group{:}; params_group = values(param_map, {'fl_z_r_infbr_n'}); [fl_z_r_borr_n] = params_group{:}; end else params_group = values(armt_map, {'ar_z'}); [ar_z] = params_group{:}; end % support_map params_group = values(support_map, {'bl_display_final', 'it_display_final_rowmax', 'it_display_final_colmax'}); [bl_display_final, it_display_final_rowmax, it_display_final_colmax] = params_group{:}; params_group = values(support_map, {'bl_graph', 'bl_graph_onebyones'}); [bl_graph] = params_group{:}; params_group = values(support_map, {'bl_mat', 'st_mat_path', 'st_mat_prefix', 'st_mat_name_main', 'st_mat_suffix'}); [bl_mat, st_mat_path, st_mat_prefix, st_mat_name_main, st_mat_suffix] = params_group{:};
Generate Consumption and Income Matrix
if (~isKey(result_map, 'cl_mt_cons')) f_cons = func_map('f_cons'); mt_cons = f_cons(mt_coh_wkb, mt_pol_a, mt_pol_k); result_map('cl_mt_cons') = {mt_cons, zeros(1)}; end if (~isKey(result_map, 'cl_mt_coh')) f_coh = func_map('f_coh'); mt_coh = f_coh(ar_z, ar_a_meshk, ar_k_mesha); result_map('cl_mt_coh') = {mt_coh, zeros(1)}; end
Save Mat
if (bl_mat) mkdir(support_map('st_mat_path')); st_file_name = [st_mat_prefix st_mat_name_main st_mat_suffix]; save(strcat(st_mat_path, st_file_name)); end
Generate and Save Graphs
if (bl_graph) bl_input_override = true; ff_akz_vf_post_graph(param_map, support_map, armt_map, func_map, result_map, bl_input_override); end
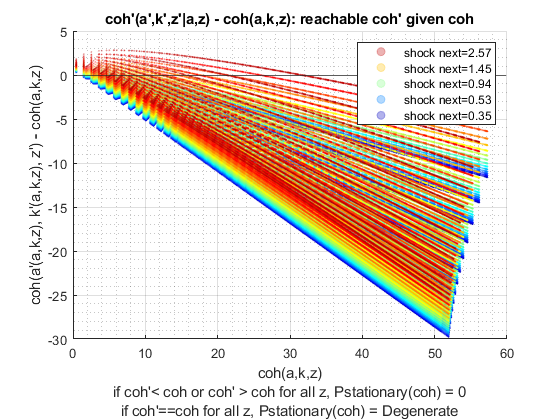
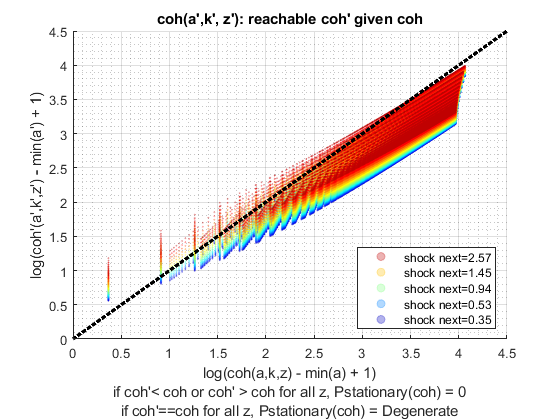
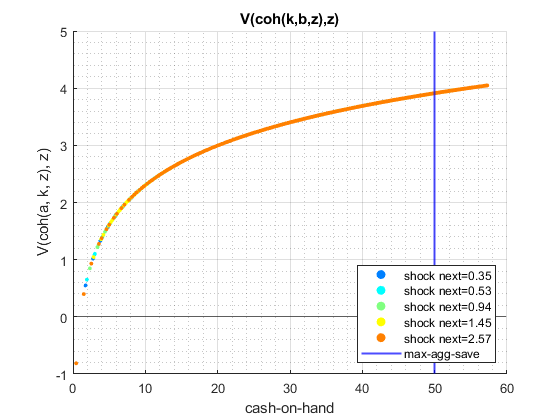
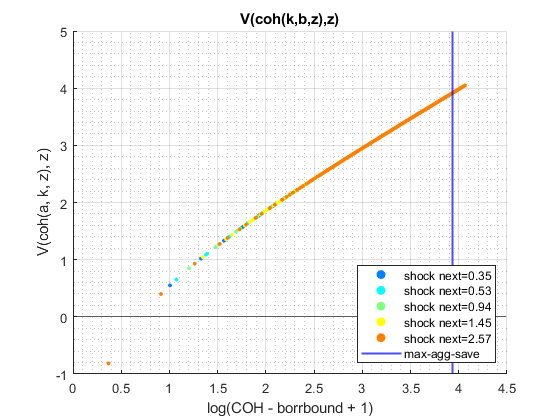
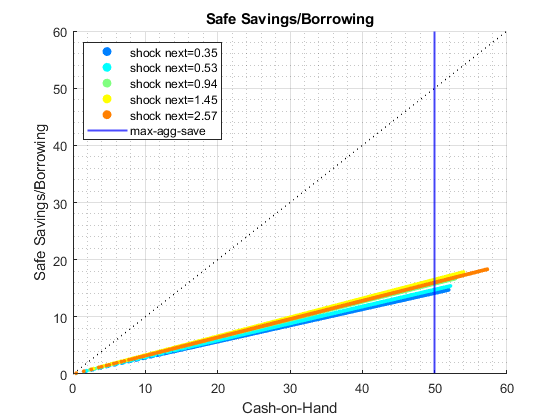
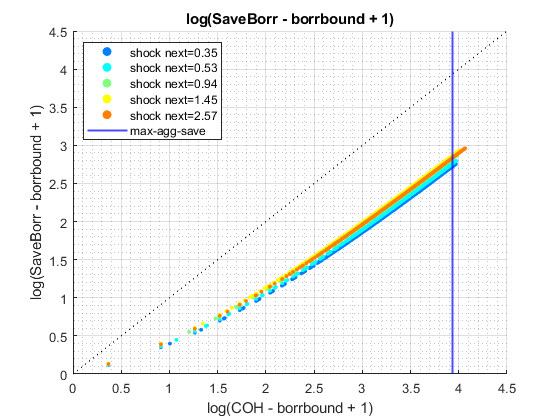
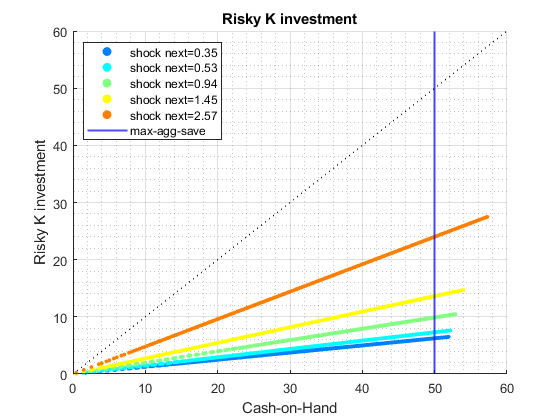
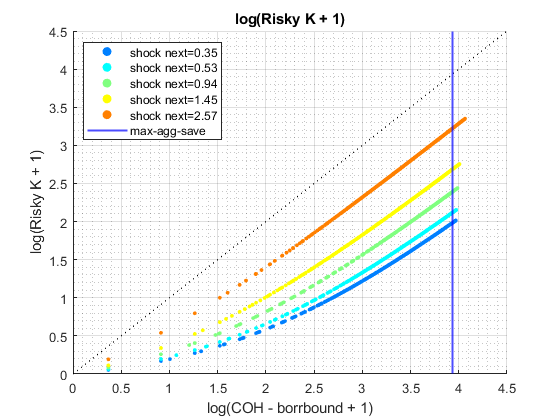
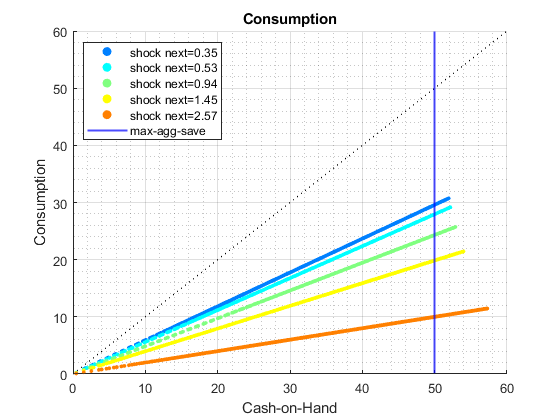
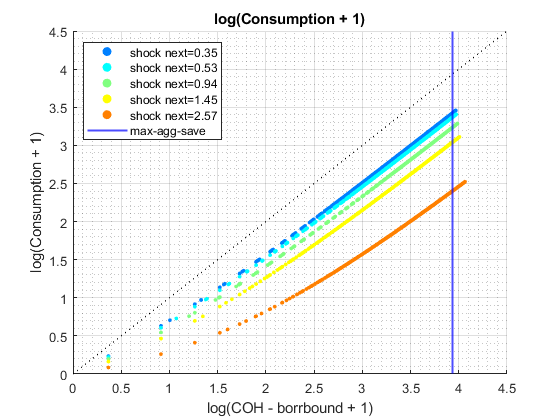
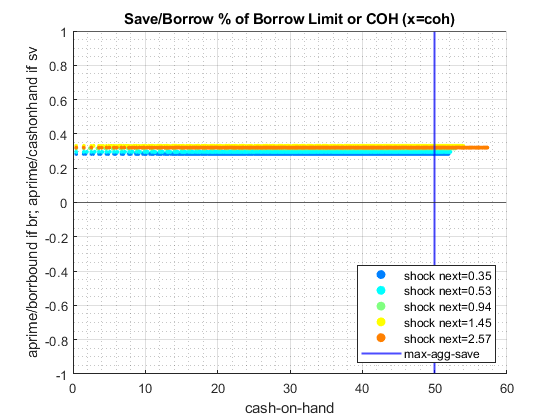
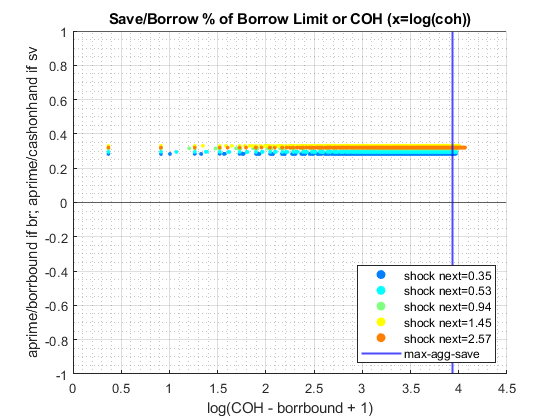
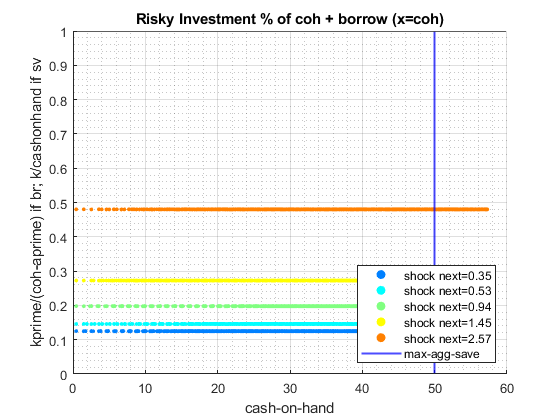
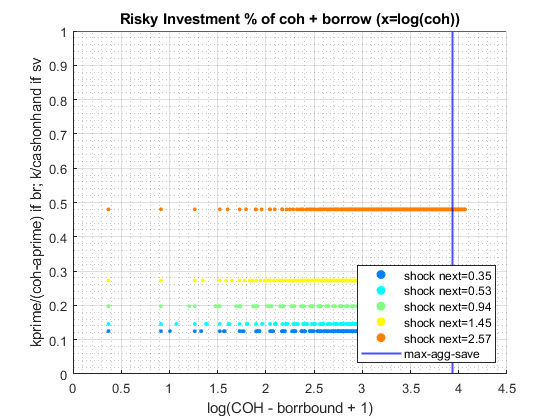
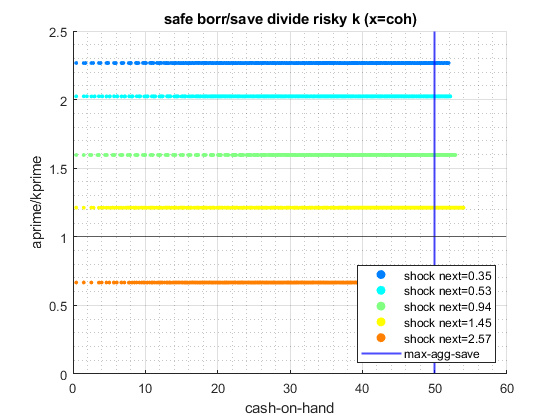
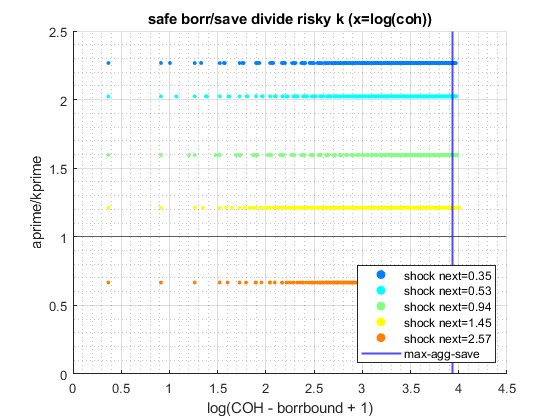
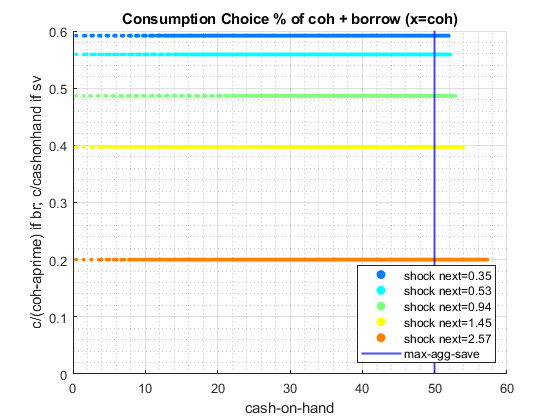
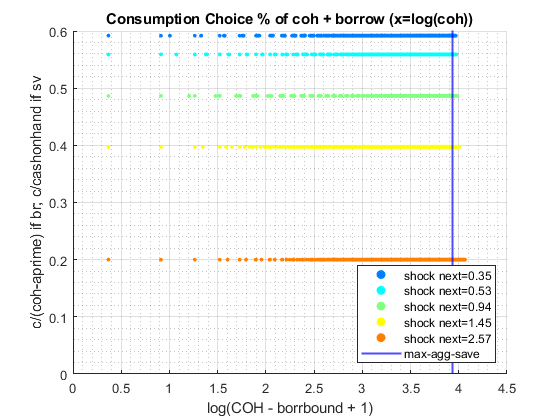
Display Val Pol Iter Table
if (bl_display_final) % Columns to Display if (it_z_n >= it_display_final_colmax) ar_it_cols = (1:1:round(it_display_final_colmax/2)); ar_it_cols = [ar_it_cols ((it_z_n)-round(it_display_final_colmax/2)+1):1:(it_z_n)]; else ar_it_cols = 1:1:it_z_n; end ar_it_cols = unique(ar_it_cols); % Column Z Names if (ismember(st_model, ["ipwkbzr", "ipwkbzr_fibs"])) if (fl_z_r_borr_n == 1) ar_st_col_zs = matlab.lang.makeValidName(strcat('z', string(ar_it_cols), '=', string(ar_z_wage_mesh_r_borr_w1r2(ar_it_cols)))); else ar_st_col_zs = matlab.lang.makeValidName(strcat('zi', string(ar_it_cols), ... ':zr=', string(ar_z_r_borr_mesh_wage_w1r2(ar_it_cols)), ... ';zw=', string(ar_z_wage_mesh_r_borr_w1r2(ar_it_cols)))); end else ar_st_col_zs = matlab.lang.makeValidName(strcat('z', string(ar_it_cols), '=', string(ar_z(ar_it_cols)))); end % Display Value Function Iteration Step by Step REsults it_iter_max = length(ar_val_diff_norm); if (it_iter_max >= it_display_final_rowmax) ar_it_rows_iter = (1:1:round(it_display_final_rowmax/2)); ar_it_rows_iter = [ar_it_rows_iter ((it_iter_max)-round(it_display_final_rowmax/2)+1):1:(it_iter_max)]; else ar_it_rows_iter = 1:1:it_iter_max; end tb_valpol_alliter = array2table([ar_val_diff_norm(ar_it_rows_iter)';... ar_pol_diff_norm(ar_it_rows_iter)';... mt_pol_perc_change(ar_it_rows_iter, :)']'); cl_col_names = ['valgap', 'polgap', strcat('z', string((1:it_z_n)))]; cl_row_names = strcat('iter=', string(ar_it_rows_iter)); tb_valpol_alliter.Properties.VariableNames = cl_col_names; tb_valpol_alliter.Properties.RowNames = cl_row_names; tb_valpol_alliter.Properties.VariableDescriptions{'valgap'} = 'norm(mt_val - mt_val_cur)'; tb_valpol_alliter.Properties.VariableDescriptions{'polgap'} = 'norm(mt_pol_a - mt_pol_a_cur)'; tb_valpol_alliter.Properties.VariableDescriptions{'z1'} = 'z1 perc change: sum((mt_pol_a ~= mt_pol_a_cur))/(it_ameshk_n)'; disp('valgap = norm(mt_val - mt_val_cur): value function difference across iterations'); disp('polgap = norm(mt_pol_a - mt_pol_a_cur): policy function difference across iterations'); disp(['z1 = z1 perc change: (sum((mt_pol_a ~= mt_pol_a_cur))+sum((mt_pol_k ~= mt_pol_k_cur)))/(2*it_ameshk_n):' ... 'percentage of state space points conditional on shock where the policy function is changing across iterations']); disp(tb_valpol_alliter); % Display Values by States % at most display 11 columns of shocks % at most display 50 rows for states % display first and last if (it_ameshk_n >= it_display_final_rowmax) ar_it_rows = (1:1:round(it_display_final_rowmax/2)); ar_it_rows = [ar_it_rows ((it_ameshk_n)-round(it_display_final_rowmax/2)+1):1:(it_ameshk_n)]; else ar_it_rows = 1:1:it_ameshk_n; end mt_val_print = mt_val(ar_it_rows, ar_it_cols); mt_pol_a_print = mt_pol_a(ar_it_rows, ar_it_cols); mt_pol_k_print = mt_pol_k(ar_it_rows, ar_it_cols); mt_pol_w_print = mt_pol_a_print + mt_pol_k_print; % Row Name Define ar_st_row_coh = strcat('coh', string(ar_it_rows), ... ':k=', string(ar_a_meshk(ar_it_rows)'), ... ',b=', string(ar_k_mesha(ar_it_rows)')); % Display Optimal Values tb_val = array2table(mt_val_print); tb_val.Properties.RowNames = ar_st_row_coh; tb_val.Properties.VariableNames = ar_st_col_zs; disp('tb_val: V(a,z) value at each state space point'); disp(tb_val); % Display Optimal Choices for a tb_pol_a = array2table(mt_pol_a_print); tb_pol_a.Properties.RowNames = ar_st_row_coh; tb_pol_a.Properties.VariableNames = ar_st_col_zs; disp('tb_pol_a: optimal safe savings choice for each state space point'); disp(tb_pol_a); % Display Optimal Choices for k tb_pol_k = array2table(mt_pol_k_print); tb_pol_k.Properties.RowNames = ar_st_row_coh; tb_pol_k.Properties.VariableNames = ar_st_col_zs; disp('tb_pol_k: optimal risky investment choice for each state space point'); disp(tb_pol_k); % Display Optimal Choices for k+a tb_pol_w = array2table(mt_pol_w_print); tb_pol_w.Properties.RowNames = ar_st_row_coh; tb_pol_w.Properties.VariableNames = ar_st_col_zs; disp('tb_pol_w: risky + safe investment choices (first stage choice, choose within risky vs safe)'); disp(tb_pol_w); % Save to result map result_map('tb_valpol_alliter') = tb_valpol_alliter; result_map('tb_val') = tb_val; result_map('tb_pol_a') = tb_pol_a; end
valgap = norm(mt_val - mt_val_cur): value function difference across iterations polgap = norm(mt_pol_a - mt_pol_a_cur): policy function difference across iterations z1 = z1 perc change: (sum((mt_pol_a ~= mt_pol_a_cur))+sum((mt_pol_k ~= mt_pol_k_cur)))/(2*it_ameshk_n):percentage of state space points conditional on shock where the policy function is changing across iterations valgap polgap z1 z2 z3 z4 z5 z6 z7 z8 z9 z10 z11 z12 z13 z14 z15 ________ ________ _________ ________ ________ ________ ________ ________ _________ _________ ________ ________ __________ ________ ________ ________ ________ iter=1 0.81472 0.27603 0.16218 0.41727 0.64432 0.96309 0.059619 0.30145 0.42289 0.91599 0.58225 0.73634 0.85071 0.14651 0.55903 0.45606 0.68372 iter=2 0.90579 0.6797 0.79428 0.049654 0.37861 0.54681 0.68197 0.7011 0.094229 0.0011511 0.54074 0.39471 0.56056 0.18907 0.8541 0.10167 0.13208 iter=3 0.12699 0.6551 0.31122 0.90272 0.81158 0.52114 0.042431 0.66634 0.59852 0.46245 0.86994 0.68342 0.92961 0.042652 0.34788 0.99539 0.72272 iter=4 0.91338 0.16261 0.52853 0.94479 0.53283 0.23159 0.071445 0.53913 0.47092 0.42435 0.26478 0.70405 0.69667 0.6352 0.44603 0.33209 0.11035 iter=5 0.63236 0.119 0.16565 0.49086 0.35073 0.4889 0.52165 0.69811 0.69595 0.46092 0.31807 0.44231 0.58279 0.28187 0.054239 0.29735 0.11749 iter=6 0.09754 0.49836 0.60198 0.48925 0.939 0.62406 0.09673 0.66653 0.69989 0.77016 0.11921 0.019578 0.8154 0.5386 0.17711 0.062045 0.64072 iter=7 0.2785 0.95974 0.26297 0.33772 0.87594 0.67914 0.81815 0.17813 0.63853 0.32247 0.93983 0.33086 0.87901 0.69516 0.66281 0.29824 0.32881 iter=8 0.54688 0.34039 0.65408 0.90005 0.55016 0.39552 0.81755 0.12801 0.033604 0.78474 0.64555 0.42431 0.98891 0.49912 0.33083 0.046351 0.65381 iter=9 0.95751 0.58527 0.68921 0.36925 0.62248 0.36744 0.72244 0.99908 0.068806 0.47136 0.47946 0.27027 0.00052238 0.5358 0.89849 0.50543 0.74913 iter=10 0.96489 0.22381 0.74815 0.1112 0.58704 0.98798 0.14987 0.17112 0.3196 0.035763 0.63932 0.19705 0.86544 0.44518 0.11816 0.76143 0.58319 iter=11 0.15761 0.75127 0.45054 0.78025 0.20774 0.037739 0.65961 0.032601 0.53086 0.17587 0.54472 0.82172 0.61257 0.12393 0.98842 0.63107 0.74003 iter=12 0.97059 0.2551 0.083821 0.38974 0.30125 0.88517 0.51859 0.5612 0.65445 0.72176 0.64731 0.42992 0.98995 0.49036 0.53998 0.089892 0.23483 iter=13 0.95717 0.50596 0.22898 0.24169 0.47092 0.91329 0.97297 0.88187 0.40762 0.47349 0.54389 0.88777 0.52768 0.853 0.70692 0.080862 0.73496 iter=14 0.48538 0.69908 0.91334 0.40391 0.23049 0.79618 0.64899 0.66918 0.81998 0.15272 0.72105 0.39118 0.47952 0.87393 0.99949 0.77724 0.9706 iter=15 0.80028 0.8909 0.15238 0.096455 0.84431 0.098712 0.80033 0.19043 0.71836 0.34112 0.5225 0.76911 0.80135 0.27029 0.28785 0.90513 0.86693 iter=16 0.14189 0.95929 0.82582 0.13197 0.19476 0.26187 0.4538 0.36892 0.96865 0.60739 0.9937 0.39679 0.22784 0.20846 0.41452 0.53377 0.086235 iter=17 0.42176 0.54722 0.53834 0.94205 0.22592 0.33536 0.43239 0.46073 0.53133 0.19175 0.21868 0.80851 0.49809 0.56498 0.46484 0.10915 0.36644 iter=18 0.91574 0.13862 0.99613 0.95613 0.17071 0.67973 0.82531 0.98164 0.32515 0.73843 0.1058 0.75508 0.90085 0.64031 0.76396 0.82581 0.3692 iter=19 0.79221 0.14929 0.078176 0.57521 0.22766 0.13655 0.08347 0.1564 0.10563 0.24285 0.1097 0.3774 0.57466 0.41703 0.8182 0.3381 0.68503 iter=20 0.95949 0.25751 0.44268 0.05978 0.4357 0.72123 0.13317 0.85552 0.61096 0.91742 0.063591 0.21602 0.84518 0.20598 0.10022 0.29397 0.59794 iter=21 0.65574 0.84072 0.10665 0.23478 0.3111 0.10676 0.17339 0.64476 0.7788 0.26906 0.40458 0.79041 0.73864 0.94793 0.17812 0.74631 0.78936 iter=22 0.035712 0.25428 0.9619 0.35316 0.92338 0.65376 0.39094 0.37627 0.42345 0.7655 0.44837 0.9493 0.58599 0.082071 0.35963 0.010337 0.36765 iter=23 0.84913 0.81428 0.0046342 0.82119 0.43021 0.49417 0.83138 0.19092 0.090823 0.18866 0.36582 0.32757 0.24673 0.10571 0.056705 0.048447 0.20603 iter=24 0.93399 0.24352 0.77491 0.015403 0.18482 0.77905 0.80336 0.42825 0.26647 0.2875 0.7635 0.67126 0.66642 0.14204 0.52189 0.66792 0.086667 iter=25 0.67874 0.92926 0.8173 0.043024 0.90488 0.71504 0.060471 0.48202 0.15366 0.091113 0.6279 0.43864 0.083483 0.16646 0.33585 0.60347 0.77193 iter=26 0.75774 0.34998 0.86869 0.16899 0.97975 0.90372 0.39926 0.12061 0.28101 0.57621 0.77198 0.8335 0.62596 0.62096 0.17567 0.5261 0.20567 iter=27 0.74313 0.1966 0.084436 0.64912 0.43887 0.89092 0.52688 0.58951 0.44009 0.68336 0.93285 0.76885 0.66094 0.57371 0.20895 0.72971 0.38827 iter=28 0.39223 0.25108 0.39978 0.73172 0.11112 0.33416 0.4168 0.22619 0.52714 0.54659 0.97274 0.16725 0.72975 0.052078 0.90515 0.70725 0.55178 iter=29 0.65548 0.61604 0.25987 0.64775 0.25806 0.69875 0.65686 0.38462 0.45742 0.42573 0.19203 0.86198 0.89075 0.9312 0.67539 0.78138 0.22895 iter=30 0.17119 0.47329 0.80007 0.45092 0.40872 0.19781 0.62797 0.58299 0.87537 0.64444 0.13887 0.98987 0.9823 0.72866 0.46847 0.28798 0.64194 iter=31 0.70605 0.35166 0.43141 0.54701 0.5949 0.030541 0.29198 0.25181 0.51805 0.64762 0.69627 0.51442 0.76903 0.73784 0.91213 0.69253 0.48448 iter=32 0.031833 0.83083 0.91065 0.29632 0.26221 0.74407 0.43165 0.29044 0.94362 0.67902 0.09382 0.88428 0.58145 0.063405 0.10401 0.55667 0.15185 iter=33 0.27692 0.58526 0.18185 0.74469 0.60284 0.50002 0.015487 0.61709 0.63771 0.63579 0.5254 0.58803 0.92831 0.86044 0.74555 0.39652 0.78193 iter=34 0.046171 0.54972 0.2638 0.18896 0.71122 0.47992 0.98406 0.26528 0.95769 0.94517 0.53034 0.15475 0.58009 0.93441 0.73627 0.061591 0.10061 iter=35 0.097132 0.91719 0.14554 0.68678 0.22175 0.90472 0.16717 0.82438 0.24071 0.20893 0.86114 0.19986 0.016983 0.9844 0.56186 0.78018 0.29407 iter=36 0.82346 0.28584 0.13607 0.18351 0.11742 0.60987 0.10622 0.98266 0.67612 0.70928 0.48485 0.40695 0.12086 0.85894 0.18419 0.33758 0.23737 iter=37 0.69483 0.7572 0.86929 0.36848 0.29668 0.61767 0.37241 0.73025 0.28906 0.23623 0.39346 0.74871 0.86271 0.78556 0.59721 0.60787 0.53087 iter=38 0.3171 0.75373 0.5797 0.62562 0.31878 0.85944 0.19812 0.34388 0.67181 0.1194 0.67143 0.82558 0.4843 0.51338 0.29994 0.74125 0.091499 iter=39 0.95022 0.38045 0.54986 0.78023 0.42417 0.80549 0.48969 0.58407 0.69514 0.6073 0.74126 0.78996 0.84486 0.1776 0.13412 0.10481 0.40532 iter=40 0.034446 0.56782 0.14495 0.081126 0.50786 0.57672 0.33949 0.10777 0.067993 0.45014 0.52005 0.31852 0.20941 0.39859 0.2126 0.12789 0.10485 iter=41 0.43874 0.075854 0.85303 0.92939 0.085516 0.18292 0.95163 0.90631 0.25479 0.45873 0.34771 0.53406 0.55229 0.13393 0.89494 0.54954 0.11228 iter=42 0.38156 0.05395 0.62206 0.77571 0.26248 0.23993 0.92033 0.87965 0.22404 0.66194 0.15 0.089951 0.62988 0.03089 0.071453 0.48523 0.78443 iter=43 0.76552 0.5308 0.35095 0.48679 0.80101 0.88651 0.052677 0.81776 0.66783 0.77029 0.58609 0.11171 0.031991 0.93914 0.24249 0.89048 0.29157 iter=44 0.7952 0.77917 0.51325 0.43586 0.02922 0.028674 0.73786 0.26073 0.84439 0.35022 0.26215 0.13629 0.61471 0.30131 0.053754 0.79896 0.60353 iter=45 0.18687 0.93401 0.40181 0.44678 0.92885 0.4899 0.26912 0.59436 0.34446 0.66201 0.044454 0.67865 0.36241 0.29553 0.44172 0.73434 0.96442 iter=46 0.48976 0.12991 0.075967 0.30635 0.73033 0.16793 0.42284 0.022513 0.78052 0.41616 0.75493 0.49518 0.049533 0.33294 0.013283 0.051332 0.43248 iter=47 0.44559 0.56882 0.23992 0.50851 0.48861 0.97868 0.54787 0.42526 0.67533 0.84193 0.24279 0.18971 0.48957 0.46707 0.89719 0.072885 0.69475 iter=48 0.64631 0.46939 0.12332 0.51077 0.57853 0.71269 0.94274 0.31272 0.0067153 0.83292 0.4424 0.49501 0.19251 0.6482 0.19666 0.088527 0.7581 iter=49 0.70936 0.011902 0.18391 0.81763 0.23728 0.50047 0.41774 0.16148 0.60217 0.25644 0.6878 0.14761 0.12308 0.025228 0.093371 0.79835 0.43264 iter=50 0.75469 0.33712 0.23995 0.79483 0.45885 0.47109 0.98305 0.17877 0.38677 0.61346 0.35923 0.054974 0.20549 0.84221 0.30737 0.94301 0.6555 tb_val: V(a,z) value at each state space point z1_0_34741 z2_0_40076 z3_0_4623 z4_0_5333 z5_0_61519 z6_0_70966 z10_1_2567 z11_1_4496 z12_1_6723 z13_1_9291 z14_2_2253 z15_2_567 __________ __________ _________ _________ __________ __________ __________ __________ __________ __________ __________ _________ coh1:k=0,b=0 -0.81272 -0.81272 -0.81272 -0.81272 -0.81272 -0.81272 -0.81272 -0.81272 -0.81272 -0.81272 -0.81272 -0.81272 coh2:k=1.02041,b=0 0.39849 0.39849 0.39849 0.39849 0.39849 0.39849 0.39849 0.39849 0.39849 0.39849 0.39849 0.39849 coh3:k=0,b=1.02041 0.54949 0.58004 0.61416 0.65212 0.6942 0.74063 0.9739 1.0447 1.1207 1.2016 1.2876 1.3783 coh4:k=2.04082,b=0 0.93038 0.93038 0.93038 0.93038 0.93038 0.93038 0.93038 0.93038 0.93038 0.93038 0.93038 0.93038 coh5:k=1.02041,b=1.02041 1.0218 1.041 1.0626 1.0871 1.1145 1.1452 1.3068 1.358 1.4141 1.4751 1.5411 1.6123 coh6:k=0,b=2.04082 1.019 1.0436 1.0712 1.1022 1.1367 1.1752 1.3727 1.434 1.5003 1.5718 1.6483 1.7299 coh7:k=3.06122,b=0 1.2758 1.2758 1.2758 1.2758 1.2758 1.2758 1.2758 1.2758 1.2758 1.2758 1.2758 1.2758 coh8:k=2.04082,b=1.02041 1.3414 1.3553 1.3712 1.3892 1.4095 1.4325 1.5561 1.5962 1.6407 1.6896 1.7432 1.8017 coh9:k=1.02041,b=2.04082 1.3393 1.3572 1.3774 1.4003 1.4261 1.455 1.6078 1.6566 1.71 1.7683 1.8316 1.9 coh10:k=0,b=3.06122 1.3296 1.3505 1.3741 1.4006 1.4304 1.4636 1.637 1.6917 1.7512 1.8158 1.8854 1.9601 coh11:k=4.08163,b=0 1.532 1.532 1.532 1.532 1.532 1.532 1.532 1.532 1.532 1.532 1.532 1.532 coh12:k=3.06122,b=1.02041 1.5831 1.5941 1.6066 1.6208 1.637 1.6554 1.7554 1.7885 1.8253 1.8661 1.9113 1.9609 coh13:k=2.04082,b=2.04082 1.5815 1.5956 1.6116 1.6297 1.6503 1.6734 1.798 1.8385 1.8833 1.9325 1.9865 2.0453 coh14:k=1.02041,b=3.06122 1.5739 1.5903 1.6089 1.63 1.6537 1.6804 1.8223 1.8679 1.918 1.9729 2.0327 2.0975 coh15:k=0,b=4.08163 1.5634 1.5818 1.6026 1.626 1.6524 1.682 1.838 1.8877 1.9421 2.0014 2.0657 2.1351 coh16:k=5.10204,b=0 1.7358 1.7358 1.7358 1.7358 1.7358 1.7358 1.7358 1.7358 1.7358 1.7358 1.7358 1.7358 coh17:k=4.08163,b=1.02041 1.7777 1.7867 1.797 1.8088 1.8223 1.8375 1.9216 1.9497 1.9811 2.0161 2.0551 2.0982 coh18:k=3.06122,b=2.04082 1.7763 1.7879 1.8012 1.8162 1.8333 1.8526 1.9578 1.9924 2.0309 2.0735 2.1206 2.1722 coh19:k=2.04082,b=3.06122 1.7701 1.7836 1.799 1.8164 1.8361 1.8584 1.9785 2.0176 2.061 2.1087 2.1611 2.2183 coh20:k=1.02041,b=4.08163 1.7615 1.7766 1.7937 1.8131 1.835 1.8598 1.9919 2.0347 2.0819 2.1336 2.1902 2.2517 coh21:k=0,b=5.10204 1.7513 1.7678 1.7865 1.8077 1.8316 1.8584 2.0011 2.0469 2.0973 2.1525 2.2125 2.2776 coh22:k=6.12245,b=0 1.905 1.905 1.905 1.905 1.905 1.905 1.905 1.905 1.905 1.905 1.905 1.905 coh23:k=5.10204,b=1.02041 1.9405 1.9482 1.9569 1.967 1.9785 1.9915 2.0641 2.0884 2.1158 2.1465 2.1808 2.219 coh24:k=4.08163,b=2.04082 1.9393 1.9492 1.9605 1.9733 1.9879 2.0045 2.0955 2.1257 2.1595 2.1971 2.2388 2.2848 coh25:k=3.06122,b=3.06122 1.934 1.9455 1.9586 1.9735 1.9903 2.0095 2.1136 2.1478 2.186 2.2282 2.2749 2.3261 coh26:k=2.04082,b=4.08163 1.9267 1.9395 1.9541 1.9707 1.9894 2.0106 2.1253 2.1628 2.2044 2.2504 2.3009 2.3561 coh27:k=1.02041,b=5.10204 1.9181 1.9321 1.948 1.966 1.9864 2.0095 2.1333 2.1736 2.2181 2.2672 2.3209 2.3794 coh28:k=0,b=6.12245 1.9086 1.9236 1.9407 1.9601 1.982 2.0067 2.1388 2.1815 2.2286 2.2803 2.3369 2.3983 coh29:k=7.14286,b=0 2.0496 2.0496 2.0496 2.0496 2.0496 2.0496 2.0496 2.0496 2.0496 2.0496 2.0496 2.0496 coh30:k=6.12245,b=1.02041 2.0804 2.0871 2.0948 2.1035 2.1136 2.125 2.1887 2.2103 2.2346 2.2619 2.2925 2.3267 coh31:k=5.10204,b=2.04082 2.0794 2.088 2.0978 2.109 2.1218 2.1363 2.2165 2.2434 2.2734 2.307 2.3445 2.386 coh32:k=4.08163,b=3.06122 2.0749 2.0848 2.0962 2.1092 2.1239 2.1407 2.2325 2.263 2.2971 2.335 2.377 2.4234 coh33:k=3.06122,b=4.08163 2.0685 2.0796 2.0923 2.1067 2.1231 2.1417 2.243 2.2764 2.3136 2.3549 2.4005 2.4506 coh34:k=2.04082,b=5.10204 2.061 2.0732 2.087 2.1027 2.1205 2.1407 2.2501 2.286 2.3259 2.37 2.4186 2.4719 coh35:k=1.02041,b=6.12245 2.0527 2.0658 2.0807 2.0975 2.1167 2.1383 2.255 2.2931 2.3353 2.3819 2.4331 2.4891 coh36:k=0,b=7.14286 2.0438 2.0578 2.0736 2.0915 2.1119 2.1348 2.2582 2.2983 2.3427 2.3915 2.4451 2.5035 coh37:k=8.16327,b=0 2.176 2.176 2.176 2.176 2.176 2.176 2.176 2.176 2.176 2.176 2.176 2.176 coh38:k=7.14286,b=1.02041 2.2032 2.2091 2.2159 2.2237 2.2325 2.2427 2.2995 2.3189 2.3407 2.3653 2.3929 2.4239 coh39:k=6.12245,b=2.04082 2.2023 2.2099 2.2186 2.2285 2.2399 2.2528 2.3245 2.3486 2.3757 2.4061 2.44 2.4778 coh40:k=5.10204,b=3.06122 2.1983 2.2071 2.2172 2.2287 2.2418 2.2567 2.3389 2.3663 2.3971 2.4315 2.4697 2.512 coh41:k=4.08163,b=4.08163 2.1926 2.2025 2.2137 2.2265 2.241 2.2576 2.3483 2.3784 2.4121 2.4496 2.4911 2.537 coh42:k=3.06122,b=5.10204 2.186 2.1968 2.209 2.2229 2.2387 2.2567 2.3547 2.3871 2.4232 2.4633 2.5077 2.5565 coh43:k=2.04082,b=6.12245 2.1787 2.1903 2.2034 2.2183 2.2353 2.2545 2.359 2.3935 2.4318 2.4742 2.521 2.5724 coh44:k=1.02041,b=7.14286 2.1709 2.1832 2.1971 2.213 2.231 2.2514 2.3619 2.3982 2.4384 2.4829 2.5319 2.5856 coh45:k=0,b=8.16327 2.1626 2.1756 2.1903 2.2071 2.2261 2.2476 2.3637 2.4016 2.4436 2.49 2.541 2.5968 coh46:k=9.18367,b=0 2.2882 2.2882 2.2882 2.2882 2.2882 2.2882 2.2882 2.2882 2.2882 2.2882 2.2882 2.2882 coh47:k=8.16327,b=1.02041 2.3125 2.3178 2.3239 2.3309 2.3389 2.348 2.3993 2.4168 2.4366 2.459 2.4842 2.5125 coh48:k=7.14286,b=2.04082 2.3117 2.3185 2.3263 2.3353 2.3454 2.3571 2.4219 2.4438 2.4685 2.4962 2.5273 2.562 coh49:k=6.12245,b=3.06122 2.3081 2.316 2.325 2.3354 2.3472 2.3606 2.435 2.4599 2.488 2.5194 2.5545 2.5934 coh50:k=5.10204,b=4.08163 2.3031 2.3119 2.3219 2.3334 2.3465 2.3614 2.4435 2.4709 2.5017 2.536 2.5742 2.6165 coh1226:k=50,b=0 3.9453 3.9453 3.9453 3.9453 3.9453 3.9453 3.9453 3.9453 3.9453 3.9453 3.9453 3.9453 coh1227:k=48.9796,b=1.02041 3.95 3.9511 3.9522 3.9536 3.9552 3.957 3.9675 3.9712 3.9754 3.9802 3.9858 3.9922 coh1228:k=47.9592,b=2.04082 3.9499 3.9512 3.9527 3.9545 3.9565 3.9588 3.9723 3.9769 3.9823 3.9885 3.9956 4.0037 coh1229:k=46.9388,b=3.06122 3.9492 3.9507 3.9525 3.9545 3.9569 3.9596 3.975 3.9804 3.9866 3.9938 4.0019 4.0112 coh1230:k=45.9184,b=4.08163 3.9482 3.9499 3.9519 3.9541 3.9567 3.9597 3.9769 3.9829 3.9897 3.9976 4.0066 4.0168 coh1231:k=44.898,b=5.10204 3.9471 3.9489 3.951 3.9535 3.9563 3.9596 3.9781 3.9846 3.992 4.0005 4.0102 4.0213 coh1232:k=43.8776,b=6.12245 3.9458 3.9478 3.9501 3.9527 3.9557 3.9592 3.979 3.9859 3.9938 4.0029 4.0132 4.025 coh1233:k=42.8571,b=7.14286 3.9445 3.9466 3.949 3.9517 3.9549 3.9586 3.9796 3.9869 3.9952 4.0048 4.0157 4.0281 coh1234:k=41.8367,b=8.16327 3.9431 3.9453 3.9478 3.9507 3.9541 3.9579 3.9799 3.9876 3.9963 4.0063 4.0177 4.0307 coh1235:k=40.8163,b=9.18367 3.9416 3.9439 3.9465 3.9496 3.9531 3.9571 3.9801 3.9881 3.9972 4.0076 4.0195 4.033 coh1236:k=39.7959,b=10.2041 3.9401 3.9425 3.9452 3.9484 3.952 3.9562 3.9801 3.9884 3.9979 4.0087 4.021 4.0351 coh1237:k=38.7755,b=11.2245 3.9386 3.941 3.9439 3.9472 3.9509 3.9553 3.98 3.9886 3.9984 4.0095 4.0223 4.0368 coh1238:k=37.7551,b=12.2449 3.937 3.9395 3.9425 3.9459 3.9498 3.9543 3.9798 3.9886 3.9987 4.0103 4.0234 4.0384 coh1239:k=36.7347,b=13.2653 3.9354 3.938 3.9411 3.9446 3.9486 3.9532 3.9795 3.9886 3.999 4.0108 4.0244 4.0397 coh1240:k=35.7143,b=14.2857 3.9338 3.9365 3.9396 3.9432 3.9473 3.9521 3.9791 3.9884 3.9991 4.0113 4.0252 4.0409 coh1241:k=34.6939,b=15.3061 3.9321 3.9349 3.9381 3.9418 3.946 3.9509 3.9786 3.9882 3.9992 4.0116 4.0259 4.042 coh1242:k=33.6735,b=16.3265 3.9304 3.9333 3.9366 3.9404 3.9447 3.9497 3.9781 3.9879 3.9991 4.0119 4.0264 4.043 coh1243:k=32.6531,b=17.3469 3.9287 3.9317 3.935 3.9389 3.9433 3.9484 3.9775 3.9875 3.999 4.0121 4.0269 4.0438 coh1244:k=31.6327,b=18.3673 3.927 3.93 3.9335 3.9374 3.942 3.9472 3.9768 3.9871 3.9988 4.0122 4.0273 4.0445 coh1245:k=30.6122,b=19.3878 3.9253 3.9284 3.9319 3.9359 3.9405 3.9459 3.9762 3.9866 3.9986 4.0122 4.0276 4.0452 coh1246:k=29.5918,b=20.4082 3.9236 3.9267 3.9303 3.9344 3.9391 3.9445 3.9754 3.9861 3.9983 4.0121 4.0279 4.0457 coh1247:k=28.5714,b=21.4286 3.9218 3.925 3.9286 3.9328 3.9376 3.9432 3.9746 3.9855 3.9979 4.012 4.028 4.0462 coh1248:k=27.551,b=22.449 3.92 3.9233 3.927 3.9313 3.9362 3.9418 3.9738 3.9849 3.9975 4.0119 4.0282 4.0466 coh1249:k=26.5306,b=23.4694 3.9182 3.9215 3.9253 3.9297 3.9347 3.9404 3.973 3.9842 3.9971 4.0116 4.0282 4.047 coh1250:k=25.5102,b=24.4898 3.9164 3.9198 3.9236 3.9281 3.9332 3.939 3.9721 3.9835 3.9966 4.0114 4.0282 4.0473 coh1251:k=24.4898,b=25.5102 3.9146 3.918 3.9219 3.9265 3.9316 3.9375 3.9712 3.9828 3.996 4.0111 4.0281 4.0475 coh1252:k=23.4694,b=26.5306 3.9128 3.9163 3.9202 3.9248 3.9301 3.9361 3.9703 3.982 3.9955 4.0107 4.028 4.0477 coh1253:k=22.449,b=27.551 3.911 3.9145 3.9185 3.9232 3.9285 3.9346 3.9693 3.9812 3.9949 4.0103 4.0279 4.0478 coh1254:k=21.4286,b=28.5714 3.9091 3.9127 3.9168 3.9215 3.9269 3.9331 3.9683 3.9804 3.9942 4.0099 4.0277 4.0479 coh1255:k=20.4082,b=29.5918 3.9073 3.9109 3.9151 3.9198 3.9253 3.9316 3.9673 3.9796 3.9936 4.0095 4.0275 4.0479 coh1256:k=19.3878,b=30.6122 3.9054 3.9091 3.9133 3.9181 3.9237 3.9301 3.9662 3.9787 3.9929 4.009 4.0272 4.0479 coh1257:k=18.3673,b=31.6327 3.9035 3.9073 3.9115 3.9164 3.9221 3.9285 3.9652 3.9778 3.9921 4.0085 4.0269 4.0478 coh1258:k=17.3469,b=32.6531 3.9016 3.9054 3.9098 3.9147 3.9204 3.927 3.9641 3.9769 3.9914 4.0079 4.0266 4.0478 coh1259:k=16.3265,b=33.6735 3.8997 3.9036 3.908 3.913 3.9188 3.9254 3.963 3.9759 3.9906 4.0073 4.0263 4.0476 coh1260:k=15.3061,b=34.6939 3.8978 3.9017 3.9062 3.9113 3.9171 3.9239 3.9619 3.975 3.9898 4.0067 4.0259 4.0475 coh1261:k=14.2857,b=35.7143 3.8959 3.8999 3.9044 3.9095 3.9155 3.9223 3.9607 3.974 3.989 4.0061 4.0254 4.0473 coh1262:k=13.2653,b=36.7347 3.894 3.898 3.9025 3.9078 3.9138 3.9207 3.9596 3.973 3.9882 4.0054 4.025 4.0471 coh1263:k=12.2449,b=37.7551 3.8921 3.8961 3.9007 3.906 3.9121 3.919 3.9584 3.9719 3.9873 4.0048 4.0245 4.0468 coh1264:k=11.2245,b=38.7755 3.8902 3.8942 3.8989 3.9042 3.9104 3.9174 3.9572 3.9709 3.9864 4.0041 4.024 4.0466 coh1265:k=10.2041,b=39.7959 3.8882 3.8923 3.897 3.9025 3.9087 3.9158 3.956 3.9698 3.9855 4.0033 4.0235 4.0463 coh1266:k=9.18367,b=40.8163 3.8863 3.8904 3.8952 3.9007 3.9069 3.9141 3.9548 3.9687 3.9846 4.0026 4.023 4.0459 coh1267:k=8.16327,b=41.8367 3.8843 3.8885 3.8933 3.8989 3.9052 3.9125 3.9535 3.9676 3.9837 4.0018 4.0224 4.0456 coh1268:k=7.14286,b=42.8571 3.8823 3.8866 3.8915 3.897 3.9035 3.9108 3.9523 3.9665 3.9827 4.0011 4.0218 4.0452 coh1269:k=6.12245,b=43.8776 3.8804 3.8847 3.8896 3.8952 3.9017 3.9091 3.951 3.9654 3.9817 4.0003 4.0212 4.0448 coh1270:k=5.10204,b=44.898 3.8784 3.8827 3.8877 3.8934 3.8999 3.9074 3.9498 3.9643 3.9808 3.9994 4.0206 4.0444 coh1271:k=4.08163,b=45.9184 3.8764 3.8808 3.8858 3.8916 3.8982 3.9057 3.9485 3.9631 3.9798 3.9986 4.0199 4.044 coh1272:k=3.06122,b=46.9388 3.8744 3.8788 3.8839 3.8897 3.8964 3.904 3.9472 3.9619 3.9787 3.9978 4.0193 4.0435 coh1273:k=2.04082,b=47.9592 3.8724 3.8769 3.882 3.8879 3.8946 3.9023 3.9458 3.9608 3.9777 3.9969 4.0186 4.043 coh1274:k=1.02041,b=48.9796 3.8704 3.8749 3.8801 3.886 3.8928 3.9006 3.9445 3.9596 3.9767 3.996 4.0179 4.0425 coh1275:k=0,b=50 3.8684 3.8729 3.8781 3.8841 3.891 3.8988 3.9432 3.9584 3.9756 3.9951 4.0172 4.042 tb_pol_a: optimal safe savings choice for each state space point z1_0_34741 z2_0_40076 z3_0_4623 z4_0_5333 z5_0_61519 z6_0_70966 z10_1_2567 z11_1_4496 z12_1_6723 z13_1_9291 z14_2_2253 z15_2_567 __________ __________ _________ _________ __________ __________ __________ __________ __________ __________ __________ _________ coh1:k=0,b=0 0.12565 0.12724 0.129 0.13093 0.13304 0.13529 0.14469 0.14652 0.14768 0.14774 0.14611 0.14197 coh2:k=1.02041,b=0 0.42187 0.42721 0.43312 0.43962 0.44668 0.45424 0.4858 0.49196 0.49584 0.49604 0.49057 0.47666 coh3:k=0,b=1.02041 0.49063 0.51226 0.53738 0.56654 0.60037 0.63955 0.86368 0.93884 1.0209 1.1075 1.1935 1.2698 coh4:k=2.04082,b=0 0.71809 0.72718 0.73725 0.7483 0.76032 0.77318 0.8269 0.83739 0.84401 0.84435 0.83503 0.81136 coh5:k=1.02041,b=1.02041 0.78685 0.81223 0.8415 0.87522 0.91401 0.9585 1.2048 1.2843 1.3691 1.4558 1.538 1.6045 coh6:k=0,b=2.04082 0.7846 0.81431 0.84872 0.88854 0.93455 0.98761 1.2869 1.3856 1.4923 1.6035 1.7119 1.8047 coh7:k=3.06122,b=0 1.0143 1.0272 1.0414 1.057 1.074 1.0921 1.168 1.1828 1.1922 1.1926 1.1795 1.146 coh8:k=2.04082,b=1.02041 1.0831 1.1122 1.1456 1.1839 1.2276 1.2774 1.5459 1.6297 1.7172 1.8041 1.8824 1.9392 coh9:k=1.02041,b=2.04082 1.0808 1.1143 1.1528 1.1972 1.2482 1.3066 1.628 1.731 1.8405 1.9518 2.0564 2.1394 coh10:k=0,b=3.06122 1.0705 1.1069 1.149 1.1976 1.2535 1.3179 1.6763 1.7929 1.9179 2.0466 2.17 2.2721 coh11:k=4.08163,b=0 1.3105 1.3271 1.3455 1.3657 1.3876 1.4111 1.5091 1.5283 1.5403 1.541 1.5239 1.4807 coh12:k=3.06122,b=1.02041 1.3793 1.4122 1.4497 1.4926 1.5413 1.5964 1.887 1.9751 2.0654 2.1524 2.2269 2.2739 coh13:k=2.04082,b=2.04082 1.377 1.4143 1.457 1.5059 1.5618 1.6255 1.9691 2.0764 2.1887 2.3001 2.4008 2.4741 coh14:k=1.02041,b=3.06122 1.3667 1.4069 1.4531 1.5063 1.5672 1.6368 2.0174 2.1383 2.2661 2.3949 2.5144 2.6067 coh15:k=0,b=4.08163 1.3524 1.3949 1.4439 1.5003 1.5652 1.6395 2.0494 2.1811 2.3213 2.4641 2.5988 2.7066 coh16:k=5.10204,b=0 1.6068 1.6271 1.6496 1.6744 1.7012 1.73 1.8502 1.8737 1.8885 1.8893 1.8684 1.8154 coh17:k=4.08163,b=1.02041 1.6755 1.7121 1.7539 1.8013 1.8549 1.9153 2.2281 2.3206 2.4136 2.5007 2.5713 2.6086 coh18:k=3.06122,b=2.04082 1.6733 1.7142 1.7611 1.8146 1.8755 1.9445 2.3102 2.4219 2.5368 2.6484 2.7453 2.8088 coh19:k=2.04082,b=3.06122 1.6629 1.7068 1.7572 1.8149 1.8808 1.9557 2.3585 2.4838 2.6142 2.7432 2.8589 2.9414 coh20:k=1.02041,b=4.08163 1.6486 1.6949 1.748 1.809 1.8788 1.9584 2.3905 2.5265 2.6695 2.8124 2.9432 3.0412 coh21:k=0,b=5.10204 1.6319 1.6801 1.7355 1.7992 1.8723 1.9558 2.4124 2.5576 2.711 2.8659 3.0097 3.121 coh22:k=6.12245,b=0 1.903 1.9271 1.9537 1.983 2.0149 2.049 2.1913 2.2191 2.2367 2.2376 2.2129 2.1501 coh23:k=5.10204,b=1.02041 1.9717 2.0121 2.058 2.11 2.1686 2.2343 2.5692 2.666 2.7617 2.849 2.9158 2.9433 coh24:k=4.08163,b=2.04082 1.9695 2.0142 2.0652 2.1233 2.1891 2.2634 2.6513 2.7673 2.885 2.9967 3.0897 3.1435 coh25:k=3.06122,b=3.06122 1.9591 2.0068 2.0614 2.1236 2.1945 2.2747 2.6996 2.8292 2.9624 3.0915 3.2034 3.2761 coh26:k=2.04082,b=4.08163 1.9448 1.9949 2.0522 2.1177 2.1924 2.2774 2.7316 2.872 3.0176 3.1607 3.2877 3.3759 coh27:k=1.02041,b=5.10204 1.9282 1.9801 2.0397 2.1079 2.1859 2.2747 2.7535 2.903 3.0592 3.2142 3.3541 3.4557 coh28:k=0,b=6.12245 1.9098 1.9634 2.0249 2.0955 2.1763 2.2684 2.7686 2.926 3.0914 3.2569 3.4082 3.5216 coh29:k=7.14286,b=0 2.1992 2.227 2.2579 2.2917 2.3285 2.3679 2.5324 2.5646 2.5848 2.5859 2.5573 2.4848 coh30:k=6.12245,b=1.02041 2.268 2.3121 2.3621 2.4186 2.4822 2.5532 2.9103 3.0114 3.1099 3.1973 3.2603 3.278 coh31:k=5.10204,b=2.04082 2.2657 2.3142 2.3693 2.432 2.5027 2.5823 2.9924 3.1127 3.2331 3.345 3.4342 3.4782 coh32:k=4.08163,b=3.06122 2.2553 2.3068 2.3655 2.4323 2.5081 2.5936 3.0407 3.1746 3.3106 3.4398 3.5478 3.6108 coh33:k=3.06122,b=4.08163 2.2411 2.2948 2.3563 2.4264 2.5061 2.5963 3.0727 3.2174 3.3658 3.509 3.6322 3.7106 coh34:k=2.04082,b=5.10204 2.2244 2.28 2.3438 2.4166 2.4996 2.5937 3.0946 3.2485 3.4074 3.5625 3.6986 3.7904 coh35:k=1.02041,b=6.12245 2.206 2.2633 2.329 2.4042 2.4899 2.5874 3.1097 3.2715 3.4396 3.6052 3.7527 3.8563 coh36:k=0,b=7.14286 2.1865 2.2452 2.3126 2.3898 2.478 2.5784 3.1196 3.2885 3.4649 3.6399 3.7977 3.912 coh37:k=8.16327,b=0 2.4954 2.527 2.562 2.6004 2.6422 2.6869 2.8735 2.91 2.933 2.9342 2.9018 2.8195 coh38:k=7.14286,b=1.02041 2.5642 2.6121 2.6662 2.7273 2.7958 2.8722 3.2514 3.3569 3.458 3.5456 3.6047 3.6127 coh39:k=6.12245,b=2.04082 2.5619 2.6141 2.6735 2.7406 2.8164 2.9013 3.3335 3.4582 3.5813 3.6933 3.7787 3.8129 coh40:k=5.10204,b=3.06122 2.5516 2.6068 2.6696 2.741 2.8217 2.9126 3.3818 3.5201 3.6587 3.7881 3.8923 3.9455 coh41:k=4.08163,b=4.08163 2.5373 2.5948 2.6604 2.7351 2.8197 2.9152 3.4138 3.5628 3.7139 3.8573 3.9766 4.0453 coh42:k=3.06122,b=5.10204 2.5206 2.58 2.6479 2.7253 2.8132 2.9126 3.4357 3.5939 3.7555 3.9108 4.043 4.125 coh43:k=2.04082,b=6.12245 2.5023 2.5633 2.6332 2.7129 2.8036 2.9063 3.4508 3.6169 3.7878 3.9535 4.0971 4.191 coh44:k=1.02041,b=7.14286 2.4827 2.5452 2.6167 2.6985 2.7916 2.8974 3.4607 3.634 3.8131 3.9882 4.1422 4.2467 coh45:k=0,b=8.16327 2.4622 2.5259 2.599 2.6826 2.778 2.8863 3.4667 3.6464 3.8331 4.0167 4.1801 4.2945 coh46:k=9.18367,b=0 2.7916 2.827 2.8661 2.9091 2.9558 3.0058 3.2147 3.2554 3.2812 3.2825 3.2462 3.1542 coh47:k=8.16327,b=1.02041 2.8604 2.912 2.9704 3.036 3.1095 3.1911 3.5925 3.7023 3.8062 3.8939 3.9492 3.9474 coh48:k=7.14286,b=2.04082 2.8581 2.9141 2.9776 3.0493 3.13 3.2202 3.6746 3.8036 3.9295 4.0416 4.1231 4.1476 coh49:k=6.12245,b=3.06122 2.8478 2.9067 2.9737 3.0497 3.1354 3.2315 3.7229 3.8655 4.0069 4.1364 4.2367 4.2802 coh50:k=5.10204,b=4.08163 2.8335 2.8948 2.9645 3.0438 3.1334 3.2342 3.7549 3.9083 4.0621 4.2056 4.3211 4.38 coh1226:k=50,b=0 14.64 14.826 15.031 15.256 15.501 15.764 16.859 17.073 17.208 17.215 17.025 16.542 coh1227:k=48.9796,b=1.02041 14.709 14.911 15.135 15.383 15.655 15.949 17.237 17.52 17.733 17.826 17.728 17.335 coh1228:k=47.9592,b=2.04082 14.707 14.913 15.143 15.397 15.676 15.978 17.319 17.621 17.856 17.974 17.901 17.535 coh1229:k=46.9388,b=3.06122 14.697 14.906 15.139 15.397 15.681 15.989 17.367 17.683 17.933 18.069 18.015 17.668 coh1230:k=45.9184,b=4.08163 14.682 14.894 15.129 15.391 15.679 15.992 17.399 17.726 17.989 18.138 18.099 17.768 coh1231:k=44.898,b=5.10204 14.666 14.879 15.117 15.381 15.672 15.989 17.421 17.757 18.03 18.191 18.166 17.847 coh1232:k=43.8776,b=6.12245 14.647 14.862 15.102 15.369 15.663 15.983 17.436 17.78 18.062 18.234 18.22 17.913 coh1233:k=42.8571,b=7.14286 14.628 14.844 15.086 15.355 15.651 15.974 17.446 17.797 18.088 18.269 18.265 17.969 coh1234:k=41.8367,b=8.16327 14.607 14.825 15.068 15.339 15.637 15.963 17.452 17.809 18.108 18.297 18.303 18.017 coh1235:k=40.8163,b=9.18367 14.586 14.805 15.049 15.322 15.622 15.95 17.455 17.818 18.123 18.321 18.335 18.058 coh1236:k=39.7959,b=10.2041 14.564 14.784 15.03 15.303 15.606 15.936 17.455 17.824 18.136 18.34 18.363 18.095 coh1237:k=38.7755,b=11.2245 14.542 14.762 15.009 15.285 15.588 15.921 17.453 17.827 18.145 18.356 18.387 18.127 coh1238:k=37.7551,b=12.2449 14.519 14.74 14.988 15.265 15.57 15.905 17.45 17.828 18.151 18.369 18.407 18.155 coh1239:k=36.7347,b=13.2653 14.496 14.718 14.967 15.245 15.552 15.888 17.444 17.827 18.156 18.38 18.425 18.18 coh1240:k=35.7143,b=14.2857 14.472 14.695 14.945 15.224 15.532 15.87 17.437 17.824 18.158 18.388 18.44 18.202 coh1241:k=34.6939,b=15.3061 14.448 14.672 14.923 15.202 15.512 15.852 17.429 17.82 18.159 18.395 18.452 18.221 coh1242:k=33.6735,b=16.3265 14.424 14.648 14.9 15.181 15.491 15.832 17.42 17.815 18.158 18.399 18.463 18.238 coh1243:k=32.6531,b=17.3469 14.399 14.624 14.877 15.159 15.47 15.813 17.41 17.809 18.156 18.402 18.472 18.254 coh1244:k=31.6327,b=18.3673 14.375 14.6 14.854 15.136 15.449 15.793 17.399 17.801 18.153 18.404 18.479 18.267 coh1245:k=30.6122,b=19.3878 14.35 14.576 14.83 15.113 15.427 15.772 17.387 17.792 18.149 18.404 18.485 18.279 coh1246:k=29.5918,b=20.4082 14.325 14.552 14.806 15.09 15.405 15.751 17.374 17.783 18.143 18.404 18.489 18.289 coh1247:k=28.5714,b=21.4286 14.3 14.527 14.782 15.067 15.383 15.73 17.36 17.773 18.137 18.402 18.493 18.298 coh1248:k=27.551,b=22.449 14.274 14.502 14.758 15.043 15.36 15.708 17.346 17.762 18.129 18.399 18.495 18.305 coh1249:k=26.5306,b=23.4694 14.249 14.477 14.733 15.019 15.337 15.686 17.332 17.75 18.121 18.395 18.495 18.312 coh1250:k=25.5102,b=24.4898 14.223 14.452 14.708 14.995 15.314 15.664 17.316 17.738 18.112 18.39 18.495 18.317 coh1251:k=24.4898,b=25.5102 14.198 14.426 14.684 14.971 15.29 15.641 17.301 17.725 18.103 18.384 18.494 18.321 coh1252:k=23.4694,b=26.5306 14.172 14.401 14.659 14.947 15.266 15.619 17.284 17.711 18.092 18.378 18.493 18.324 coh1253:k=22.449,b=27.551 14.146 14.375 14.633 14.922 15.243 15.596 17.268 17.697 18.081 18.371 18.49 18.327 coh1254:k=21.4286,b=28.5714 14.12 14.35 14.608 14.897 15.218 15.572 17.251 17.683 18.07 18.363 18.487 18.328 coh1255:k=20.4082,b=29.5918 14.094 14.324 14.583 14.872 15.194 15.549 17.233 17.667 18.058 18.355 18.482 18.329 coh1256:k=19.3878,b=30.6122 14.067 14.298 14.557 14.847 15.17 15.525 17.215 17.652 18.045 18.346 18.478 18.329 coh1257:k=18.3673,b=31.6327 14.041 14.272 14.531 14.822 15.145 15.501 17.197 17.636 18.032 18.336 18.472 18.328 coh1258:k=17.3469,b=32.6531 14.015 14.246 14.506 14.797 15.12 15.477 17.178 17.62 18.019 18.326 18.466 18.326 coh1259:k=16.3265,b=33.6735 13.988 14.219 14.48 14.771 15.095 15.453 17.159 17.603 18.005 18.316 18.459 18.324 coh1260:k=15.3061,b=34.6939 13.961 14.193 14.454 14.746 15.07 15.429 17.14 17.586 17.991 18.304 18.452 18.321 coh1261:k=14.2857,b=35.7143 13.935 14.167 14.428 14.72 15.045 15.404 17.121 17.569 17.976 18.293 18.444 18.318 coh1262:k=13.2653,b=36.7347 13.908 14.14 14.401 14.694 15.02 15.379 17.101 17.551 17.961 18.281 18.436 18.314 coh1263:k=12.2449,b=37.7551 13.881 14.114 14.375 14.668 14.994 15.355 17.081 17.533 17.945 18.269 18.428 18.309 coh1264:k=11.2245,b=38.7755 13.855 14.087 14.349 14.642 14.969 15.33 17.06 17.515 17.93 18.256 18.418 18.304 coh1265:k=10.2041,b=39.7959 13.828 14.06 14.322 14.616 14.943 15.305 17.04 17.496 17.913 18.243 18.409 18.299 coh1266:k=9.18367,b=40.8163 13.801 14.034 14.296 14.59 14.917 15.279 17.019 17.477 17.897 18.229 18.399 18.293 coh1267:k=8.16327,b=41.8367 13.774 14.007 14.269 14.564 14.892 15.254 16.998 17.458 17.88 18.215 18.388 18.286 coh1268:k=7.14286,b=42.8571 13.747 13.98 14.243 14.537 14.866 15.229 16.977 17.439 17.863 18.201 18.378 18.28 coh1269:k=6.12245,b=43.8776 13.719 13.953 14.216 14.511 14.84 15.203 16.955 17.419 17.846 18.187 18.366 18.272 coh1270:k=5.10204,b=44.898 13.692 13.926 14.189 14.484 14.813 15.177 16.934 17.399 17.828 18.172 18.355 18.265 coh1271:k=4.08163,b=45.9184 13.665 13.899 14.162 14.458 14.787 15.152 16.912 17.379 17.81 18.157 18.343 18.257 coh1272:k=3.06122,b=46.9388 13.638 13.872 14.135 14.431 14.761 15.126 16.89 17.359 17.792 18.141 18.331 18.248 coh1273:k=2.04082,b=47.9592 13.611 13.845 14.108 14.404 14.734 15.1 16.868 17.338 17.774 18.125 18.318 18.24 coh1274:k=1.02041,b=48.9796 13.583 13.817 14.081 14.377 14.708 15.074 16.845 17.318 17.755 18.109 18.305 18.23 coh1275:k=0,b=50 13.556 13.79 14.054 14.351 14.681 15.048 16.823 17.297 17.736 18.093 18.292 18.221 tb_pol_k: optimal risky investment choice for each state space point z1_0_34741 z2_0_40076 z3_0_4623 z4_0_5333 z5_0_61519 z6_0_70966 z10_1_2567 z11_1_4496 z12_1_6723 z13_1_9291 z14_2_2253 z15_2_567 __________ __________ _________ _________ __________ __________ __________ __________ __________ __________ __________ _________ coh1:k=0,b=0 0.055433 0.058021 0.061079 0.064705 0.069017 0.074164 0.10761 0.12088 0.13716 0.15721 0.18205 0.21295 coh2:k=1.02041,b=0 0.18612 0.19481 0.20508 0.21725 0.23173 0.24901 0.36129 0.40587 0.46052 0.52785 0.61123 0.71499 coh3:k=0,b=1.02041 0.21646 0.23359 0.25444 0.27997 0.31146 0.3506 0.64233 0.77456 0.94818 1.1785 1.4871 1.9047 coh4:k=2.04082,b=0 0.31681 0.33159 0.34907 0.36979 0.39444 0.42385 0.61497 0.69086 0.78389 0.89849 1.0404 1.217 coh5:k=1.02041,b=1.02041 0.34714 0.37038 0.39843 0.43251 0.47417 0.52544 0.89601 1.0595 1.2715 1.5491 1.9162 2.4068 coh6:k=0,b=2.04082 0.34615 0.37133 0.40185 0.43909 0.48483 0.5414 0.95704 1.1431 1.386 1.7063 2.133 2.7071 coh7:k=3.06122,b=0 0.44749 0.46838 0.49307 0.52234 0.55715 0.5987 0.86866 0.97585 1.1072 1.2691 1.4696 1.7191 coh8:k=2.04082,b=1.02041 0.47783 0.50716 0.54243 0.58506 0.63688 0.70029 1.1497 1.3445 1.5949 1.9197 2.3454 2.9088 coh9:k=1.02041,b=2.04082 0.47683 0.50811 0.54585 0.59164 0.64754 0.71624 1.2107 1.4281 1.7094 2.0769 2.5621 3.2092 coh10:k=0,b=3.06122 0.47227 0.50475 0.54403 0.59181 0.65031 0.72244 1.2467 1.4792 1.7813 2.1779 2.7037 3.4081 coh11:k=4.08163,b=0 0.57818 0.60517 0.63707 0.67488 0.71986 0.77354 1.1223 1.2608 1.4306 1.6398 1.8988 2.2211 coh12:k=3.06122,b=1.02041 0.60851 0.64395 0.68643 0.7376 0.79959 0.87513 1.4034 1.6295 1.9183 2.2904 2.7746 3.4109 coh13:k=2.04082,b=2.04082 0.60752 0.6449 0.68985 0.74418 0.81025 0.89109 1.4644 1.7131 2.0327 2.4476 2.9913 3.7112 coh14:k=1.02041,b=3.06122 0.60295 0.64154 0.68803 0.74436 0.81302 0.89728 1.5003 1.7642 2.1047 2.5485 3.1329 3.9101 coh15:k=0,b=4.08163 0.59665 0.63608 0.68367 0.74143 0.81198 0.89874 1.5241 1.7994 2.1559 2.6221 3.238 4.0598 coh16:k=5.10204,b=0 0.70886 0.74195 0.78106 0.82743 0.88257 0.94839 1.376 1.5458 1.754 2.0104 2.3279 2.7232 coh17:k=4.08163,b=1.02041 0.7392 0.78074 0.83042 0.89015 0.9623 1.05 1.6571 1.9145 2.2416 2.661 3.2038 3.9129 coh18:k=3.06122,b=2.04082 0.73821 0.78169 0.83384 0.89673 0.97296 1.0659 1.7181 1.9981 2.3561 2.8182 3.4205 4.2132 coh19:k=2.04082,b=3.06122 0.73364 0.77832 0.83202 0.8969 0.97573 1.0721 1.754 2.0491 2.428 2.9191 3.5621 4.4122 coh20:k=1.02041,b=4.08163 0.72734 0.77287 0.82766 0.89397 0.97469 1.0736 1.7778 2.0844 2.4793 2.9928 3.6671 4.5619 coh21:k=0,b=5.10204 0.71997 0.76613 0.82175 0.88914 0.9713 1.0721 1.7941 2.1101 2.5179 3.0497 3.7499 4.6814 coh22:k=6.12245,b=0 0.83955 0.87874 0.92506 0.97997 1.0453 1.1232 1.6297 1.8308 2.0773 2.381 2.7571 3.2252 coh23:k=5.10204,b=1.02041 0.86989 0.91752 0.97442 1.0427 1.125 1.2248 1.9107 2.1995 2.565 3.0317 3.633 4.4149 coh24:k=4.08163,b=2.04082 0.86889 0.91847 0.97784 1.0493 1.1357 1.2408 1.9718 2.2831 2.6795 3.1889 3.8497 4.7153 coh25:k=3.06122,b=3.06122 0.86432 0.91511 0.97602 1.0494 1.1384 1.247 2.0077 2.3341 2.7514 3.2898 3.9912 4.9142 coh26:k=2.04082,b=4.08163 0.85802 0.90965 0.97166 1.0465 1.1374 1.2484 2.0315 2.3694 2.8027 3.3634 4.0963 5.0639 coh27:k=1.02041,b=5.10204 0.85066 0.90291 0.96574 1.0417 1.134 1.247 2.0478 2.395 2.8413 3.4203 4.1791 5.1835 coh28:k=0,b=6.12245 0.84257 0.89529 0.95875 1.0355 1.129 1.2435 2.059 2.414 2.8712 3.4657 4.2465 5.2824 coh29:k=7.14286,b=0 0.97024 1.0155 1.0691 1.1325 1.208 1.2981 1.8834 2.1158 2.4007 2.7517 3.1863 3.7272 coh30:k=6.12245,b=1.02041 1.0006 1.0543 1.1184 1.1952 1.2877 1.3997 2.1644 2.4845 2.8884 3.4023 4.0621 4.917 coh31:k=5.10204,b=2.04082 0.99958 1.0553 1.1218 1.2018 1.2984 1.4156 2.2255 2.5681 3.0028 3.5595 4.2789 5.2173 coh32:k=4.08163,b=3.06122 0.99501 1.0519 1.12 1.202 1.3012 1.4218 2.2614 2.6191 3.0747 3.6604 4.4204 5.4162 coh33:k=3.06122,b=4.08163 0.98871 1.0464 1.1157 1.1991 1.3001 1.4233 2.2852 2.6544 3.126 3.734 4.5255 5.566 coh34:k=2.04082,b=5.10204 0.98135 1.0397 1.1097 1.1942 1.2967 1.4218 2.3015 2.68 3.1646 3.7909 4.6083 5.6855 coh35:k=1.02041,b=6.12245 0.97325 1.0321 1.1028 1.1881 1.2917 1.4184 2.3127 2.699 3.1946 3.8364 4.6757 5.7844 coh36:k=0,b=7.14286 0.96462 1.0238 1.095 1.181 1.2855 1.4135 2.3201 2.7131 3.2181 3.8733 4.7318 5.868 coh37:k=8.16327,b=0 1.1009 1.1523 1.2131 1.2851 1.3707 1.4729 2.1371 2.4008 2.7241 3.1223 3.6155 4.2293 coh38:k=7.14286,b=1.02041 1.1313 1.1911 1.2624 1.3478 1.4504 1.5745 2.4181 2.7695 3.2117 3.7729 4.4913 5.419 coh39:k=6.12245,b=2.04082 1.1303 1.192 1.2658 1.3544 1.4611 1.5905 2.4791 2.853 3.3262 3.9301 4.708 5.7194 coh40:k=5.10204,b=3.06122 1.1257 1.1887 1.264 1.3545 1.4639 1.5967 2.5151 2.9041 3.3981 4.0311 4.8496 5.9183 coh41:k=4.08163,b=4.08163 1.1194 1.1832 1.2597 1.3516 1.4628 1.5981 2.5388 2.9394 3.4494 4.1047 4.9547 6.068 coh42:k=3.06122,b=5.10204 1.112 1.1765 1.2537 1.3468 1.4594 1.5967 2.5552 2.965 3.488 4.1616 5.0375 6.1876 coh43:k=2.04082,b=6.12245 1.1039 1.1689 1.2467 1.3406 1.4544 1.5932 2.5664 2.984 3.5179 4.207 5.1049 6.2864 coh44:k=1.02041,b=7.14286 1.0953 1.1606 1.239 1.3335 1.4483 1.5883 2.5738 2.9981 3.5415 4.2439 5.1609 6.37 coh45:k=0,b=8.16327 1.0863 1.1518 1.2306 1.3257 1.4412 1.5823 2.5782 3.0084 3.56 4.2743 5.2082 6.4417 coh46:k=9.18367,b=0 1.2316 1.2891 1.357 1.4376 1.5334 1.6478 2.3908 2.6858 3.0474 3.493 4.0447 4.7313 coh47:k=8.16327,b=1.02041 1.2619 1.3279 1.4064 1.5003 1.6131 1.7494 2.6718 3.0545 3.5351 4.1436 4.9205 5.9211 coh48:k=7.14286,b=2.04082 1.261 1.3288 1.4098 1.5069 1.6238 1.7653 2.7328 3.138 3.6496 4.3008 5.1372 6.2214 coh49:k=6.12245,b=3.06122 1.2564 1.3255 1.408 1.5071 1.6266 1.7715 2.7688 3.1891 3.7215 4.4017 5.2788 6.4203 coh50:k=5.10204,b=4.08163 1.2501 1.32 1.4036 1.5041 1.6255 1.773 2.7925 3.2244 3.7727 4.4753 5.3839 6.57 coh1226:k=50,b=0 6.459 6.7606 7.1169 7.5394 8.0418 8.6416 12.538 14.085 15.982 18.319 21.212 24.813 coh1227:k=48.9796,b=1.02041 6.4894 6.7993 7.1663 7.6021 8.1216 8.7431 12.819 14.454 16.47 18.969 22.088 26.003 coh1228:k=47.9592,b=2.04082 6.4884 6.8003 7.1697 7.6087 8.1322 8.7591 12.88 14.538 16.584 19.126 22.304 26.303 coh1229:k=46.9388,b=3.06122 6.4838 6.7969 7.1679 7.6088 8.135 8.7653 12.916 14.589 16.656 19.227 22.446 26.502 coh1230:k=45.9184,b=4.08163 6.4775 6.7915 7.1635 7.6059 8.1339 8.7668 12.94 14.624 16.707 19.301 22.551 26.652 coh1231:k=44.898,b=5.10204 6.4702 6.7847 7.1576 7.6011 8.1306 8.7653 12.956 14.65 16.746 19.358 22.634 26.771 coh1232:k=43.8776,b=6.12245 6.4621 6.7771 7.1506 7.5949 8.1256 8.7619 12.967 14.669 16.776 19.403 22.701 26.87 coh1233:k=42.8571,b=7.14286 6.4534 6.7688 7.1428 7.5878 8.1194 8.7569 12.975 14.683 16.799 19.44 22.757 26.954 coh1234:k=41.8367,b=8.16327 6.4444 6.7601 7.1344 7.58 8.1123 8.7509 12.979 14.693 16.818 19.47 22.805 27.025 coh1235:k=40.8163,b=9.18367 6.435 6.7509 7.1256 7.5715 8.1044 8.7439 12.981 14.7 16.832 19.496 22.845 27.088 coh1236:k=39.7959,b=10.2041 6.4253 6.7414 7.1163 7.5626 8.096 8.7362 12.982 14.705 16.844 19.516 22.879 27.142 coh1237:k=38.7755,b=11.2245 6.4155 6.7316 7.1067 7.5532 8.087 8.7279 12.98 14.707 16.852 19.533 22.909 27.19 coh1238:k=37.7551,b=12.2449 6.4054 6.7216 7.0967 7.5435 8.0776 8.719 12.977 14.708 16.858 19.547 22.934 27.232 coh1239:k=36.7347,b=13.2653 6.3951 6.7113 7.0866 7.5335 8.0679 8.7096 12.973 14.708 16.862 19.559 22.956 27.269 coh1240:k=35.7143,b=14.2857 6.3847 6.7009 7.0762 7.5232 8.0577 8.6998 12.968 14.705 16.865 19.568 22.975 27.302 coh1241:k=34.6939,b=15.3061 6.3741 6.6903 7.0656 7.5127 8.0473 8.6897 12.962 14.702 16.866 19.574 22.991 27.332 coh1242:k=33.6735,b=16.3265 6.3635 6.6796 7.0549 7.5019 8.0367 8.6792 12.955 14.698 16.865 19.579 23.004 27.358 coh1243:k=32.6531,b=17.3469 6.3527 6.6688 7.0439 7.491 8.0258 8.6685 12.948 14.692 16.863 19.583 23.015 27.381 coh1244:k=31.6327,b=18.3673 6.3418 6.6578 7.0329 7.4799 8.0147 8.6574 12.939 14.686 16.86 19.584 23.024 27.401 coh1245:k=30.6122,b=19.3878 6.3309 6.6467 7.0217 7.4686 8.0034 8.6462 12.931 14.679 16.856 19.585 23.031 27.418 coh1246:k=29.5918,b=20.4082 6.3199 6.6355 7.0104 7.4572 7.9919 8.6347 12.921 14.671 16.851 19.584 23.037 27.434 coh1247:k=28.5714,b=21.4286 6.3087 6.6243 6.999 7.4456 7.9802 8.623 12.911 14.663 16.845 19.582 23.041 27.447 coh1248:k=27.551,b=22.449 6.2976 6.6129 6.9875 7.434 7.9685 8.6111 12.901 14.654 16.838 19.578 23.043 27.458 coh1249:k=26.5306,b=23.4694 6.2863 6.6015 6.9759 7.4222 7.9565 8.5991 12.89 14.644 16.83 19.574 23.045 27.468 coh1250:k=25.5102,b=24.4898 6.275 6.59 6.9642 7.4103 7.9445 8.5869 12.878 14.634 16.822 19.569 23.044 27.475 coh1251:k=24.4898,b=25.5102 6.2637 6.5785 6.9524 7.3983 7.9323 8.5745 12.867 14.623 16.813 19.563 23.043 27.482 coh1252:k=23.4694,b=26.5306 6.2523 6.5668 6.9406 7.3862 7.92 8.562 12.855 14.612 16.804 19.556 23.041 27.487 coh1253:k=22.449,b=27.551 6.2408 6.5552 6.9286 7.3741 7.9076 8.5494 12.842 14.6 16.793 19.549 23.038 27.49 coh1254:k=21.4286,b=28.5714 6.2293 6.5434 6.9167 7.3618 7.8951 8.5366 12.829 14.588 16.783 19.541 23.033 27.492 coh1255:k=20.4082,b=29.5918 6.2178 6.5316 6.9046 7.3495 7.8825 8.5238 12.816 14.576 16.772 19.532 23.028 27.493 coh1256:k=19.3878,b=30.6122 6.2062 6.5198 6.8925 7.3371 7.8698 8.5108 12.803 14.563 16.76 19.522 23.022 27.493 coh1257:k=18.3673,b=31.6327 6.1946 6.5079 6.8804 7.3247 7.857 8.4977 12.789 14.55 16.748 19.512 23.015 27.492 coh1258:k=17.3469,b=32.6531 6.1829 6.496 6.8681 7.3121 7.8441 8.4845 12.776 14.537 16.735 19.501 23.008 27.489 coh1259:k=16.3265,b=33.6735 6.1712 6.484 6.8559 7.2996 7.8312 8.4713 12.762 14.523 16.722 19.49 23 27.486 coh1260:k=15.3061,b=34.6939 6.1595 6.472 6.8436 7.2869 7.8182 8.4579 12.747 14.509 16.709 19.478 22.991 27.482 coh1261:k=14.2857,b=35.7143 6.1477 6.46 6.8312 7.2742 7.8051 8.4444 12.733 14.494 16.695 19.466 22.981 27.477 coh1262:k=13.2653,b=36.7347 6.136 6.4479 6.8188 7.2615 7.792 8.4309 12.718 14.48 16.681 19.453 22.971 27.471 coh1263:k=12.2449,b=37.7551 6.1241 6.4358 6.8064 7.2486 7.7788 8.4173 12.703 14.465 16.667 19.44 22.96 27.464 coh1264:k=11.2245,b=38.7755 6.1123 6.4237 6.7939 7.2358 7.7656 8.4036 12.688 14.45 16.652 19.427 22.949 27.456 coh1265:k=10.2041,b=39.7959 6.1004 6.4115 6.7814 7.2229 7.7522 8.3899 12.673 14.435 16.637 19.413 22.937 27.448 coh1266:k=9.18367,b=40.8163 6.0885 6.3993 6.7688 7.2099 7.7389 8.376 12.657 14.419 16.622 19.398 22.924 27.439 coh1267:k=8.16327,b=41.8367 6.0766 6.3871 6.7562 7.197 7.7255 8.3622 12.642 14.403 16.606 19.383 22.911 27.43 coh1268:k=7.14286,b=42.8571 6.0647 6.3748 6.7436 7.1839 7.712 8.3482 12.626 14.387 16.591 19.368 22.898 27.419 coh1269:k=6.12245,b=43.8776 6.0527 6.3625 6.7309 7.1709 7.6985 8.3342 12.61 14.371 16.575 19.353 22.884 27.409 coh1270:k=5.10204,b=44.898 6.0408 6.3502 6.7182 7.1578 7.6849 8.3201 12.594 14.355 16.558 19.337 22.869 27.397 coh1271:k=4.08163,b=45.9184 6.0288 6.3379 6.7055 7.1446 7.6713 8.306 12.577 14.338 16.542 19.321 22.855 27.385 coh1272:k=3.06122,b=46.9388 6.0167 6.3255 6.6928 7.1314 7.6576 8.2919 12.561 14.321 16.525 19.304 22.839 27.372 coh1273:k=2.04082,b=47.9592 6.0047 6.3131 6.68 7.1182 7.6439 8.2776 12.545 14.304 16.508 19.288 22.824 27.359 coh1274:k=1.02041,b=48.9796 5.9926 6.3007 6.6672 7.105 7.6302 8.2634 12.528 14.287 16.49 19.271 22.808 27.346 coh1275:k=0,b=50 5.9806 6.2883 6.6544 7.0917 7.6164 8.249 12.511 14.27 16.473 19.253 22.791 27.331 tb_pol_w: risky + safe investment choices (first stage choice, choose within risky vs safe) z1_0_34741 z2_0_40076 z3_0_4623 z4_0_5333 z5_0_61519 z6_0_70966 z10_1_2567 z11_1_4496 z12_1_6723 z13_1_9291 z14_2_2253 z15_2_567 __________ __________ _________ _________ __________ __________ __________ __________ __________ __________ __________ _________ coh1:k=0,b=0 0.18108 0.18526 0.19008 0.19564 0.20205 0.20945 0.25229 0.26741 0.28484 0.30495 0.32815 0.35492 coh2:k=1.02041,b=0 0.60799 0.62202 0.6382 0.65687 0.6784 0.70324 0.84708 0.89783 0.95637 1.0239 1.1018 1.1917 coh3:k=0,b=1.02041 0.70709 0.74585 0.79181 0.84651 0.91183 0.99015 1.506 1.7134 1.9691 2.2859 2.6806 3.1746 coh4:k=2.04082,b=0 1.0349 1.0588 1.0863 1.1181 1.1548 1.197 1.4419 1.5283 1.6279 1.7428 1.8754 2.0284 coh5:k=1.02041,b=1.02041 1.134 1.1826 1.2399 1.3077 1.3882 1.4839 2.1008 2.3438 2.6406 3.0049 3.4542 4.0113 coh6:k=0,b=2.04082 1.1307 1.1856 1.2506 1.3276 1.4194 1.529 2.2439 2.5287 2.8783 3.3098 3.8449 4.5119 coh7:k=3.06122,b=0 1.4618 1.4955 1.5344 1.5793 1.6311 1.6908 2.0367 2.1587 2.2994 2.4618 2.6491 2.8651 coh8:k=2.04082,b=1.02041 1.5609 1.6194 1.6881 1.769 1.8645 1.9777 2.6956 2.9742 3.3121 3.7238 4.2278 4.848 coh9:k=1.02041,b=2.04082 1.5577 1.6224 1.6987 1.7889 1.8957 2.0228 2.8387 3.1591 3.5499 4.0287 4.6185 5.3486 coh10:k=0,b=3.06122 1.5427 1.6117 1.693 1.7894 1.9039 2.0403 2.923 3.2721 3.6992 4.2245 4.8737 5.6801 coh11:k=4.08163,b=0 1.8887 1.9323 1.9826 2.0406 2.1075 2.1846 2.6315 2.7891 2.9709 3.1807 3.4227 3.7019 coh12:k=3.06122,b=1.02041 1.9878 2.0561 2.1362 2.2302 2.3409 2.4715 3.2904 3.6047 3.9837 4.4427 5.0015 5.6848 coh13:k=2.04082,b=2.04082 1.9846 2.0592 2.1468 2.2501 2.3721 2.5166 3.4335 3.7895 4.2214 4.7477 5.3921 6.1853 coh14:k=1.02041,b=3.06122 1.9696 2.0484 2.1412 2.2506 2.3802 2.5341 3.5177 3.9025 4.3707 4.9434 5.6473 6.5169 coh15:k=0,b=4.08163 1.9491 2.031 2.1276 2.2418 2.3771 2.5382 3.5735 3.9805 4.4772 5.0862 5.8367 6.7664 coh16:k=5.10204,b=0 2.3156 2.369 2.4307 2.5018 2.5838 2.6784 3.2262 3.4195 3.6425 3.8997 4.1963 4.5386 coh17:k=4.08163,b=1.02041 2.4147 2.4929 2.5843 2.6914 2.8172 2.9653 3.8852 4.2351 4.6552 5.1617 5.7751 6.5215 coh18:k=3.06122,b=2.04082 2.4115 2.4959 2.5949 2.7113 2.8484 3.0104 4.0283 4.42 4.8929 5.4666 6.1658 7.0221 coh19:k=2.04082,b=3.06122 2.3965 2.4852 2.5893 2.7118 2.8566 3.0279 4.1125 4.5329 5.0423 5.6624 6.4209 7.3536 coh20:k=1.02041,b=4.08163 2.376 2.4677 2.5757 2.703 2.8535 3.032 4.1683 4.611 5.1487 5.8052 6.6104 7.6031 coh21:k=0,b=5.10204 2.3519 2.4462 2.5573 2.6884 2.8436 3.0279 4.2065 4.6676 5.229 5.9156 6.7596 7.8024 coh22:k=6.12245,b=0 2.7425 2.8058 2.8788 2.963 3.0601 3.1722 3.821 4.0499 4.314 4.6186 4.97 5.3753 coh23:k=5.10204,b=1.02041 2.8416 2.9296 3.0324 3.1527 3.2936 3.4591 4.48 4.8655 5.3267 5.8806 6.5487 7.3582 coh24:k=4.08163,b=2.04082 2.8384 2.9327 3.0431 3.1725 3.3248 3.5042 4.6231 5.0504 5.5644 6.1855 6.9394 7.8588 coh25:k=3.06122,b=3.06122 2.8235 2.9219 3.0374 3.1731 3.3329 3.5217 4.7073 5.1633 5.7138 6.3813 7.1946 8.1903 coh26:k=2.04082,b=4.08163 2.8029 2.9045 3.0238 3.1642 3.3298 3.5258 4.7631 5.2414 5.8203 6.5241 7.384 8.4398 coh27:k=1.02041,b=5.10204 2.7788 2.883 3.0054 3.1496 3.3199 3.5217 4.8013 5.2981 5.9005 6.6345 7.5332 8.6392 coh28:k=0,b=6.12245 2.7524 2.8587 2.9837 3.131 3.3053 3.512 4.8275 5.3401 5.9627 6.7226 7.6547 8.8039 coh29:k=7.14286,b=0 3.1694 3.2426 3.3269 3.4242 3.5365 3.666 4.4158 4.6804 4.9855 5.3375 5.7436 6.2121 coh30:k=6.12245,b=1.02041 3.2685 3.3664 3.4805 3.6139 3.7699 3.9529 5.0748 5.4959 5.9982 6.5996 7.3224 8.195 coh31:k=5.10204,b=2.04082 3.2653 3.3694 3.4912 3.6338 3.8011 3.998 5.2179 5.6808 6.236 6.9045 7.7131 8.6955 coh32:k=4.08163,b=3.06122 3.2504 3.3587 3.4855 3.6343 3.8092 4.0155 5.3021 5.7938 6.3853 7.1002 7.9682 9.0271 coh33:k=3.06122,b=4.08163 3.2298 3.3413 3.4719 3.6254 3.8062 4.0196 5.3578 5.8718 6.4918 7.2431 8.1577 9.2766 coh34:k=2.04082,b=5.10204 3.2057 3.3197 3.4535 3.6108 3.7963 4.0155 5.3961 5.9285 6.572 7.3534 8.3069 9.4759 coh35:k=1.02041,b=6.12245 3.1793 3.2954 3.4318 3.5923 3.7817 4.0058 5.4223 5.9705 6.6342 7.4415 8.4284 9.6407 coh36:k=0,b=7.14286 3.1511 3.269 3.4076 3.5708 3.7636 3.9919 5.4397 6.0016 6.683 7.5131 8.5295 9.7799 coh37:k=8.16327,b=0 3.5963 3.6793 3.775 3.8855 4.0128 4.1598 5.0106 5.3108 5.657 6.0565 6.5173 7.0488 coh38:k=7.14286,b=1.02041 3.6954 3.8031 3.9287 4.0751 4.2463 4.4467 5.6695 6.1264 6.6698 7.3185 8.096 9.0317 coh39:k=6.12245,b=2.04082 3.6922 3.8062 3.9393 4.095 4.2775 4.4918 5.8126 6.3112 6.9075 7.6234 8.4867 9.5323 coh40:k=5.10204,b=3.06122 3.6773 3.7954 3.9336 4.0955 4.2856 4.5093 5.8969 6.4242 7.0568 7.8192 8.7419 9.8638 coh41:k=4.08163,b=4.08163 3.6567 3.778 3.9201 4.0867 4.2825 4.5134 5.9526 6.5022 7.1633 7.962 8.9313 10.113 coh42:k=3.06122,b=5.10204 3.6326 3.7565 3.9016 4.0721 4.2726 4.5093 5.9909 6.5589 7.2435 8.0724 9.0805 10.313 coh43:k=2.04082,b=6.12245 3.6062 3.7322 3.8799 4.0535 4.258 4.4996 6.0171 6.6009 7.3057 8.1605 9.202 10.477 coh44:k=1.02041,b=7.14286 3.578 3.7058 3.8557 4.032 4.2399 4.4857 6.0345 6.6321 7.3545 8.2321 9.3031 10.617 coh45:k=0,b=8.16327 3.5484 3.6778 3.8296 4.0083 4.2191 4.4686 6.045 6.6548 7.3931 8.291 9.3883 10.736 coh46:k=9.18367,b=0 4.0232 4.1161 4.2232 4.3467 4.4892 4.6536 5.6054 5.9412 6.3286 6.7754 7.2909 7.8855 coh47:k=8.16327,b=1.02041 4.1223 4.2399 4.3768 4.5363 4.7226 4.9405 6.2643 6.7568 7.3413 8.0375 8.8697 9.8684 coh48:k=7.14286,b=2.04082 4.1191 4.2429 4.3874 4.5562 4.7538 4.9856 6.4074 6.9417 7.579 8.3424 9.2603 10.369 coh49:k=6.12245,b=3.06122 4.1042 4.2322 4.3818 4.5568 4.7619 5.003 6.4917 7.0546 7.7284 8.5381 9.5155 10.701 coh50:k=5.10204,b=4.08163 4.0836 4.2148 4.3682 4.5479 4.7589 5.0072 6.5474 7.1327 7.8348 8.6809 9.7049 10.95 coh1226:k=50,b=0 21.1 21.586 22.148 22.796 23.543 24.405 29.397 31.158 33.19 35.533 38.236 41.355 coh1227:k=48.9796,b=1.02041 21.199 21.71 22.302 22.985 23.777 24.692 30.056 31.974 34.202 36.795 39.815 43.338 coh1228:k=47.9592,b=2.04082 21.195 21.713 22.312 23.005 23.808 24.737 30.199 32.159 34.44 37.1 40.206 43.838 coh1229:k=46.9388,b=3.06122 21.18 21.702 22.307 23.006 23.816 24.755 30.283 32.272 34.589 37.296 40.461 44.17 coh1230:k=45.9184,b=4.08163 21.16 21.685 22.293 22.997 23.813 24.759 30.339 32.35 34.696 37.439 40.65 44.419 coh1231:k=44.898,b=5.10204 21.136 21.664 22.275 22.982 23.803 24.755 30.377 32.406 34.776 37.549 40.8 44.619 coh1232:k=43.8776,b=6.12245 21.109 21.639 22.253 22.964 23.788 24.745 30.404 32.448 34.838 37.637 40.921 44.784 coh1233:k=42.8571,b=7.14286 21.081 21.613 22.229 22.942 23.77 24.731 30.421 32.479 34.887 37.709 41.022 44.923 coh1234:k=41.8367,b=8.16327 21.052 21.585 22.203 22.919 23.749 24.714 30.431 32.502 34.926 37.768 41.108 45.042 coh1235:k=40.8163,b=9.18367 21.021 21.555 22.175 22.893 23.726 24.694 30.436 32.518 34.956 37.816 41.18 45.146 coh1236:k=39.7959,b=10.2041 20.989 21.525 22.146 22.866 23.702 24.673 30.437 32.529 34.979 37.856 41.242 45.237 coh1237:k=38.7755,b=11.2245 20.957 21.494 22.116 22.838 23.676 24.649 30.433 32.534 34.997 37.89 41.296 45.316 coh1238:k=37.7551,b=12.2449 20.924 21.462 22.085 22.808 23.648 24.624 30.427 32.536 35.01 37.917 41.341 45.387 coh1239:k=36.7347,b=13.2653 20.891 21.429 22.054 22.778 23.619 24.597 30.417 32.535 35.018 37.939 41.381 45.449 coh1240:k=35.7143,b=14.2857 20.857 21.396 22.021 22.747 23.59 24.57 30.405 32.53 35.023 37.956 41.414 45.504 coh1241:k=34.6939,b=15.3061 20.822 21.362 21.988 22.715 23.559 24.541 30.391 32.523 35.025 37.969 41.443 45.553 coh1242:k=33.6735,b=16.3265 20.787 21.328 21.955 22.683 23.528 24.512 30.375 32.513 35.023 37.979 41.467 45.596 coh1243:k=32.6531,b=17.3469 20.752 21.293 21.921 22.65 23.496 24.481 30.357 32.501 35.019 37.985 41.487 45.634 coh1244:k=31.6327,b=18.3673 20.717 21.258 21.886 22.616 23.464 24.45 30.338 32.487 35.013 37.988 41.503 45.668 coh1245:k=30.6122,b=19.3878 20.681 21.223 21.852 22.582 23.431 24.418 30.317 32.472 35.004 37.989 41.516 45.697 coh1246:k=29.5918,b=20.4082 20.645 21.187 21.816 22.547 23.397 24.386 30.295 32.454 34.994 37.987 41.526 45.723 coh1247:k=28.5714,b=21.4286 20.609 21.151 21.781 22.512 23.363 24.353 30.272 32.436 34.981 37.983 41.533 45.745 coh1248:k=27.551,b=22.449 20.572 21.115 21.745 22.477 23.328 24.319 30.247 32.415 34.967 37.977 41.538 45.764 coh1249:k=26.5306,b=23.4694 20.535 21.079 21.709 22.442 23.293 24.285 30.221 32.394 34.951 37.969 41.54 45.779 coh1250:k=25.5102,b=24.4898 20.498 21.042 21.673 22.406 23.258 24.251 30.195 32.372 34.934 37.959 41.54 45.792 coh1251:k=24.4898,b=25.5102 20.461 21.005 21.636 22.369 23.222 24.216 30.167 32.348 34.916 37.947 41.538 45.803 coh1252:k=23.4694,b=26.5306 20.424 20.968 21.599 22.333 23.186 24.181 30.139 32.323 34.896 37.934 41.534 45.811 coh1253:k=22.449,b=27.551 20.387 20.931 21.562 22.296 23.15 24.145 30.11 32.297 34.875 37.92 41.528 45.817 coh1254:k=21.4286,b=28.5714 20.349 20.893 21.525 22.259 23.114 24.109 30.08 32.271 34.853 37.904 41.52 45.82 coh1255:k=20.4082,b=29.5918 20.311 20.855 21.487 22.222 23.077 24.073 30.049 32.243 34.83 37.886 41.511 45.822 coh1256:k=19.3878,b=30.6122 20.274 20.818 21.45 22.184 23.039 24.036 30.018 32.215 34.805 37.868 41.5 45.821 coh1257:k=18.3673,b=31.6327 20.236 20.78 21.412 22.147 23.002 23.999 29.986 32.186 34.78 37.848 41.488 45.819 coh1258:k=17.3469,b=32.6531 20.197 20.742 21.374 22.109 22.964 23.962 29.954 32.156 34.754 37.827 41.474 45.815 coh1259:k=16.3265,b=33.6735 20.159 20.703 21.336 22.071 22.927 23.924 29.921 32.126 34.727 37.806 41.459 45.81 coh1260:k=15.3061,b=34.6939 20.121 20.665 21.297 22.032 22.889 23.887 29.887 32.095 34.7 37.783 41.443 45.803 coh1261:k=14.2857,b=35.7143 20.083 20.627 21.259 21.994 22.85 23.849 29.853 32.063 34.671 37.759 41.425 45.794 coh1262:k=13.2653,b=36.7347 20.044 20.588 21.22 21.956 22.812 23.81 29.819 32.031 34.642 37.734 41.407 45.785 coh1263:k=12.2449,b=37.7551 20.005 20.549 21.182 21.917 22.773 23.772 29.784 31.998 34.612 37.709 41.387 45.773 coh1264:k=11.2245,b=38.7755 19.967 20.511 21.143 21.878 22.734 23.733 29.748 31.965 34.582 37.682 41.367 45.761 coh1265:k=10.2041,b=39.7959 19.928 20.472 21.104 21.839 22.695 23.694 29.712 31.931 34.551 37.655 41.345 45.747 coh1266:k=9.18367,b=40.8163 19.889 20.433 21.065 21.8 22.656 23.655 29.676 31.896 34.519 37.627 41.323 45.732 coh1267:k=8.16327,b=41.8367 19.85 20.394 21.025 21.761 22.617 23.616 29.64 31.861 34.487 37.599 41.299 45.716 coh1268:k=7.14286,b=42.8571 19.811 20.355 20.986 21.721 22.578 23.577 29.602 31.826 34.454 37.569 41.275 45.699 coh1269:k=6.12245,b=43.8776 19.772 20.315 20.947 21.682 22.538 23.537 29.565 31.79 34.42 37.539 41.25 45.681 coh1270:k=5.10204,b=44.898 19.733 20.276 20.907 21.642 22.498 23.498 29.527 31.754 34.386 37.509 41.224 45.662 coh1271:k=4.08163,b=45.9184 19.694 20.237 20.868 21.602 22.458 23.458 29.489 31.717 34.352 37.477 41.198 45.642 coh1272:k=3.06122,b=46.9388 19.655 20.197 20.828 21.562 22.418 23.418 29.451 31.68 34.317 37.446 41.17 45.621 coh1273:k=2.04082,b=47.9592 19.615 20.158 20.788 21.523 22.378 23.377 29.412 31.643 34.281 37.413 41.142 45.599 coh1274:k=1.02041,b=48.9796 19.576 20.118 20.748 21.482 22.338 23.337 29.373 31.605 34.246 37.38 41.113 45.576 coh1275:k=0,b=50 19.536 20.078 20.709 21.442 22.298 23.297 29.334 31.567 34.209 37.346 41.084 45.552
end
ans = Map with properties: Count: 8 KeyType: char ValueType: any