Tabulate Value and Policy Iteration Results, Store to Mat, Graph Results
back to Fan's Dynamic Assets Repository Table of Content.
Contents
function [result_map] = ff_az_vf_post(varargin)
FF_AZ_VF_POST post ff_az_vf graphs, tables, mats.
Given the solution form ff_az_vf, graphs, tables, mats. Graphing code is separately in ff_az_vf_post_graph.m. Run this function directly with randomly generates matrixes for graph/table testings.
@param param_map container parameter container
@param support_map container support container
@param armt_map container container with states, choices and shocks grids that are inputs for grid based solution algorithm
@param func_map container container with function handles for consumption cash-on-hand etc.
@param result_map container contains policy function matrix, value function matrix, iteration results
@return result_map container add coh consumption and other matrixes to result_map also add table versions of val pol and iter matries
@example
bl_input_override = true; result_map = containers.Map('KeyType','char', 'ValueType','any'); result_map('mt_val') = mt_val; result_map('mt_pol_a') = mt_pol_a; result_map('ar_val_diff_norm') = ar_val_diff_norm(1:it_iter_last); result_map('ar_pol_diff_norm') = ar_pol_diff_norm(1:it_iter_last); result_map('mt_pol_perc_change') = mt_pol_perc_change(1:it_iter_last, :); result_map = ff_az_vf_post(param_map, support_map, armt_map, func_map, result_map, bl_input_override);
@include
Default
params_len = length(varargin); bl_input_override = 0; if (params_len == 6) bl_input_override = varargin{6}; end if (bl_input_override) % if invoked from outside overrid fully [param_map, support_map, armt_map, func_map, result_map, ~] = varargin{:}; params_group = values(result_map, {'mt_val', 'cl_mt_pol_a'}); [mt_val, cl_mt_pol_a] = params_group{:}; mt_pol_a = deal(cl_mt_pol_a{1}); params_group = values(result_map, {'ar_val_diff_norm', 'ar_pol_diff_norm', 'mt_pol_perc_change'}); [ar_val_diff_norm, ar_pol_diff_norm, mt_pol_perc_change] = params_group{:}; % Get Parameters params_group = values(param_map, {'it_z_n', 'it_a_n'}); [it_z_n, it_a_n] = params_group{:}; params_group = values(armt_map, {'ar_a'}); [ar_a] = params_group{:}; else clear all; close all; % internal invoke for testing it_param_set = 4; bl_input_override = true; % Get Parameters [param_map, support_map] = ffs_az_set_default_param(it_param_set); [armt_map, func_map] = ffs_az_get_funcgrid(param_map, support_map, bl_input_override); % 1 for override % Generate Default val and policy matrixes params_group = values(param_map, {'it_maxiter_val', 'it_z_n', 'it_a_n'}); [it_maxiter_val, it_z_n, it_a_n] = params_group{:}; params_group = values(armt_map, {'ar_a', 'ar_z'}); [ar_a, ar_z] = params_group{:}; f_util_standin = func_map('f_util_standin'); % Set Defaults mt_val = f_util_standin(ar_z, ar_a'); mt_pol_a = zeros(size(mt_val)) + ar_a'*(cumsum(sort(ar_z))/sum(ar_z)*0.4 + 0.4); it_iter_max = min(it_maxiter_val, 50); ar_val_diff_norm = rand([it_iter_max, 1]); ar_pol_diff_norm = rand([it_iter_max, 1]); mt_pol_perc_change = rand([it_iter_max, it_z_n]); % Set Results Map result_map = containers.Map('KeyType','char', 'ValueType','any'); result_map('mt_val') = mt_val; result_map('cl_mt_pol_a') = {mt_pol_a, zeros(1)}; end
Parse Parameter
% param_map params_group = values(param_map, {'st_model'}); [st_model] = params_group{:}; % armt_map if (strcmp(st_model, 'az')) params_group = values(armt_map, {'ar_z'}); [ar_z] = params_group{:}; elseif (ismember(st_model, ['abz', 'abz_fibs'])) params_group = values(armt_map, {'ar_z_r_borr_mesh_wage', 'ar_z_wage_mesh_r_borr'}); [ar_z_r_borr_mesh_wage, ar_z_wage_mesh_r_borr] = params_group{:}; params_group = values(param_map, {'fl_z_r_borr_n'}); [fl_z_r_borr_n] = params_group{:}; end % support_map params_group = values(support_map, {'bl_display_final', 'it_display_final_rowmax', 'it_display_final_colmax'}); [bl_display_final, it_display_final_rowmax, it_display_final_colmax] = params_group{:}; params_group = values(support_map, {'bl_graph', 'bl_graph_onebyones'}); [bl_graph] = params_group{:}; params_group = values(support_map, {'bl_mat', 'st_mat_path', 'st_mat_prefix', 'st_mat_name_main', 'st_mat_suffix'}); [bl_mat, st_mat_path, st_mat_prefix, st_mat_name_main, st_mat_suffix] = params_group{:};
Generate Consumption and Income Matrix
if (~isKey(result_map, 'cl_mt_pol_c')) f_cons = func_map('f_cons'); mt_cons = f_cons(ar_z, ar_a', mt_pol_a); result_map('cl_mt_pol_c') = {mt_cons, zeros(1)}; end if (~isKey(result_map, 'cl_mt_coh')) f_coh = func_map('f_coh'); mt_coh = f_coh(ar_z, ar_a'); result_map('cl_mt_coh') = {mt_coh, zeros(1)}; end
Save Mat
if (bl_mat) mkdir(support_map('st_mat_path')); st_file_name = [st_mat_prefix st_mat_name_main st_mat_suffix]; save(strcat(st_mat_path, st_file_name)); end
Generate and Save Graphs
if (bl_graph) bl_input_override = true; ff_az_vf_post_graph(param_map, support_map, armt_map, func_map, result_map, bl_input_override); end
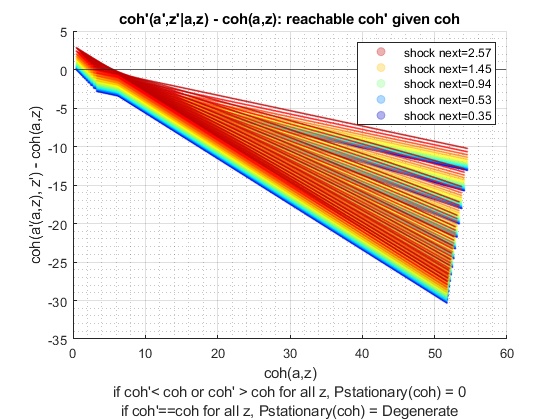
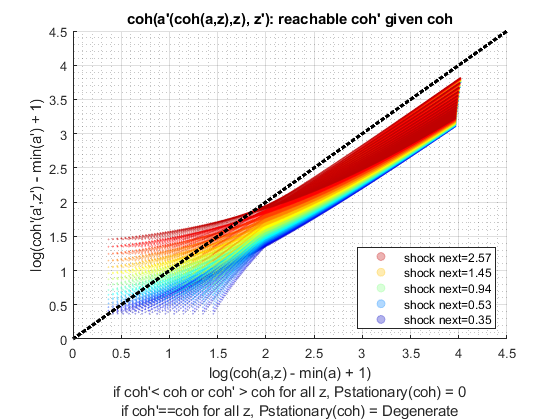
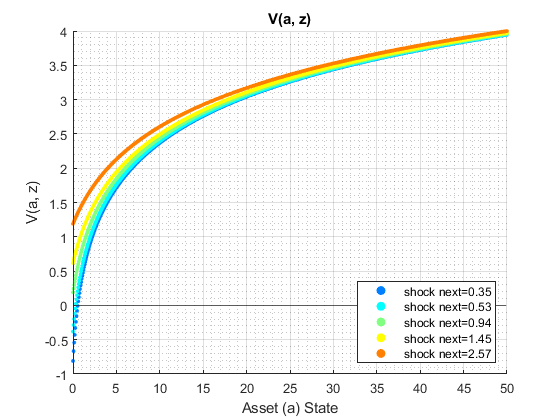
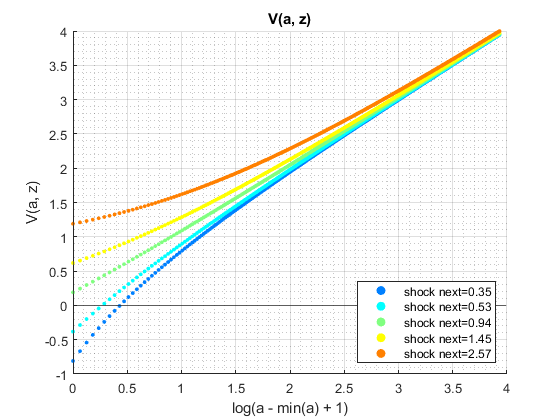
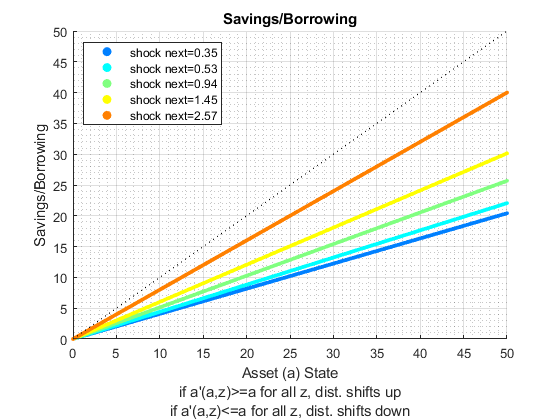
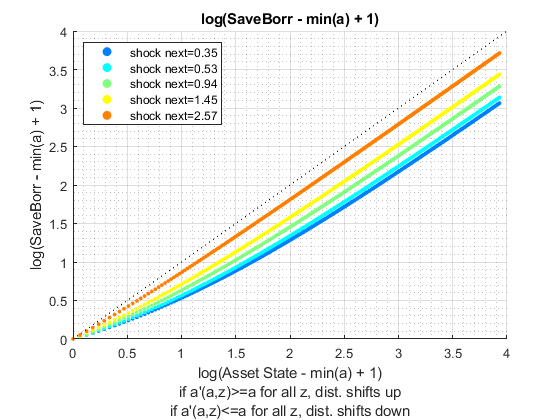
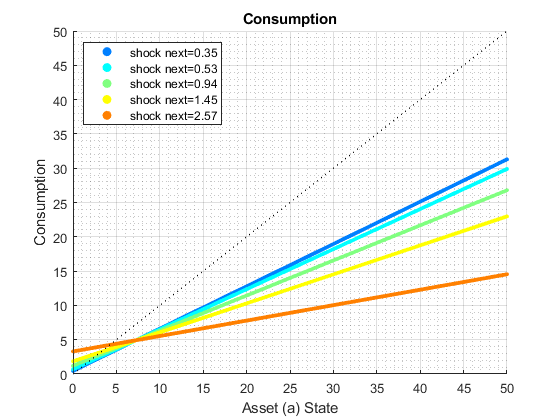
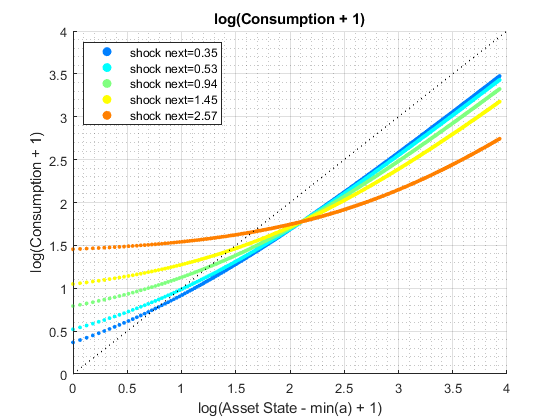
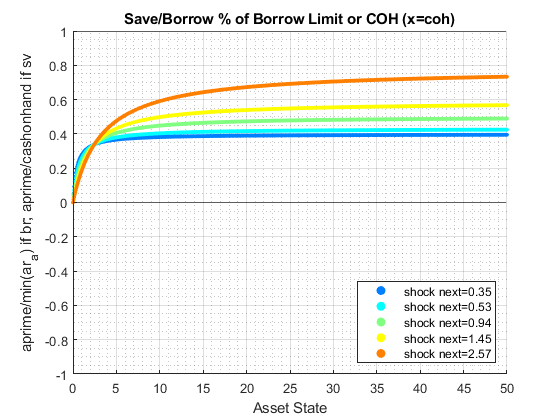
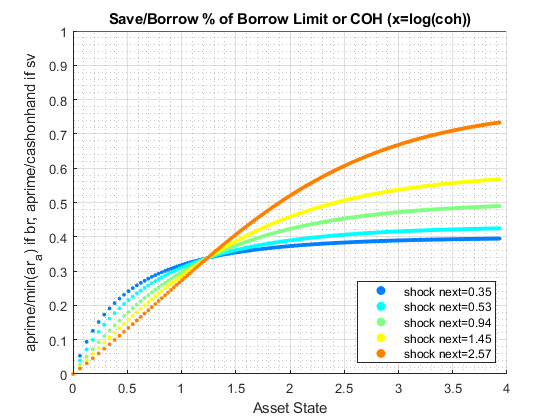
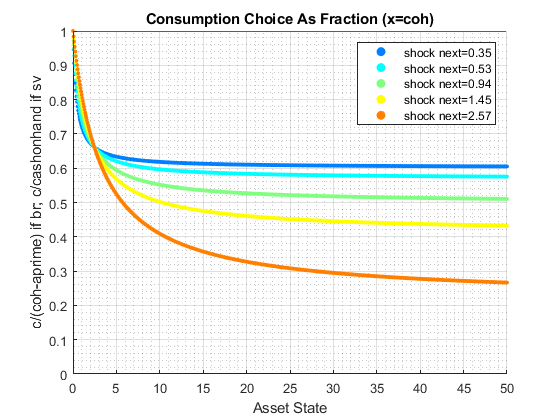
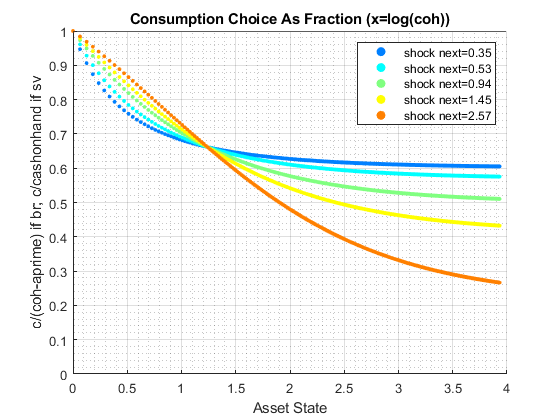
Display Val Pol Iter Table
if (bl_display_final) % Columns to Display if (it_z_n >= it_display_final_colmax) ar_it_cols = (1:1:round(it_display_final_colmax/2)); ar_it_cols = [ar_it_cols ((it_z_n)-round(it_display_final_colmax/2)+1):1:(it_z_n)]; else ar_it_cols = 1:1:it_z_n; end ar_it_cols = unique(ar_it_cols); % Column Z Names if (strcmp(st_model, 'az')) ar_st_col_zs = matlab.lang.makeValidName(strcat('z', string(ar_it_cols), '=', string(ar_z(ar_it_cols)))); elseif (ismember(st_model, ['abz', 'abz_fibs'])) if (fl_z_r_borr_n == 1) ar_st_col_zs = matlab.lang.makeValidName(strcat('z', string(ar_it_cols), '=', string(ar_z_wage_mesh_r_borr(ar_it_cols)))); else ar_st_col_zs = matlab.lang.makeValidName(strcat('zi', string(ar_it_cols), ... ':zr=', string(ar_z_r_borr_mesh_wage(ar_it_cols)), ... ';zw=', string(ar_z_wage_mesh_r_borr(ar_it_cols)))); end end % Display Value Function Iteration Step by Step REsults it_iter_max = length(ar_val_diff_norm); if (it_iter_max >= it_display_final_rowmax) ar_it_rows_iter = (1:1:round(it_display_final_rowmax/2)); ar_it_rows_iter = [ar_it_rows_iter ((it_iter_max)-round(it_display_final_rowmax/2)+1):1:(it_iter_max)]; else ar_it_rows_iter = 1:1:it_iter_max; end tb_valpol_alliter = array2table([ar_val_diff_norm(ar_it_rows_iter)';... ar_pol_diff_norm(ar_it_rows_iter)';... mt_pol_perc_change(ar_it_rows_iter, ar_it_cols)']'); cl_col_names = ['valgap', 'polgap', ar_st_col_zs]; cl_row_names = strcat('iter=', string(ar_it_rows_iter)); tb_valpol_alliter.Properties.VariableNames = cl_col_names; tb_valpol_alliter.Properties.RowNames = cl_row_names; tb_valpol_alliter.Properties.VariableDescriptions{'valgap'} = 'norm(mt_val - mt_val_cur)'; tb_valpol_alliter.Properties.VariableDescriptions{'polgap'} = 'norm(mt_pol_a - mt_pol_a_cur)'; disp('valgap = norm(mt_val - mt_val_cur): value function difference across iterations'); disp('polgap = norm(mt_pol_a - mt_pol_a_cur): policy function difference across iterations'); disp(['z1 = z1 perc change: sum((mt_pol_a ~= mt_pol_a_cur))/(it_a_n): percentage of state space'... ' points conditional on shock where the policy function is changing across iterations']); disp(tb_valpol_alliter); % Display Values by States % at most display 11 columns of shocks % at most display 50 rows for states % display first and last if (it_a_n >= it_display_final_rowmax) ar_it_rows = (1:1:round(it_display_final_rowmax/2)); ar_it_rows = [ar_it_rows ((it_a_n)-round(it_display_final_rowmax/2)+1):1:(it_a_n)]; else ar_it_rows = 1:1:it_a_n; end ar_it_rows = unique(ar_it_rows); % Subsetting mt_val_print = mt_val(ar_it_rows, ar_it_cols); mt_pol_a_print = mt_pol_a(ar_it_rows, ar_it_cols); % Display Optimal Values tb_val = array2table(mt_val_print); tb_val.Properties.RowNames = strcat('a', string(ar_it_rows), '=', string(ar_a(ar_it_rows))); tb_val.Properties.VariableNames = ar_st_col_zs; disp('tb_val: V(a,z) value at each state space point'); disp(tb_val); % Display Optimal Choices tb_pol_a = array2table(mt_pol_a_print); tb_pol_a.Properties.RowNames = strcat('a', string(ar_it_rows), '=', string(ar_a(ar_it_rows))); tb_pol_a.Properties.VariableNames = ar_st_col_zs; disp('tb_pol_a: optimal asset choice for each state space point'); disp(tb_pol_a); % Save to result map result_map('tb_valpol_alliter') = tb_valpol_alliter; result_map('tb_val') = tb_val; result_map('tb_pol_a') = tb_pol_a; end
valgap = norm(mt_val - mt_val_cur): value function difference across iterations polgap = norm(mt_pol_a - mt_pol_a_cur): policy function difference across iterations z1 = z1 perc change: sum((mt_pol_a ~= mt_pol_a_cur))/(it_a_n): percentage of state space points conditional on shock where the policy function is changing across iterations valgap polgap z1_0_34741 z2_0_40076 z3_0_4623 z4_0_5333 z5_0_61519 z6_0_70966 z10_1_2567 z11_1_4496 z12_1_6723 z13_1_9291 z14_2_2253 z15_2_567 ________ ________ __________ __________ _________ _________ __________ __________ __________ __________ __________ __________ __________ _________ iter=1 0.65521 0.15585 0.22883 0.64313 0.3851 0.68697 0.9084 0.39921 0.10671 0.83453 0.84542 0.94715 0.041196 0.97939 iter=2 0.70976 0.62071 0.37422 0.75669 0.53917 0.81793 0.49674 0.81434 0.20782 0.83622 0.24746 0.4698 0.92705 0.019326 iter=3 0.93663 0.3436 0.97924 0.40136 0.20491 0.46566 0.18316 0.93765 0.79958 0.76455 0.91028 0.74979 0.45441 0.029624 iter=4 0.87445 0.83174 0.31828 0.861 0.40271 0.58971 0.40966 0.67432 0.37291 0.87433 0.45078 0.67143 0.53493 0.28095 iter=5 0.57406 0.4488 0.55367 0.59993 0.60172 0.62351 0.46025 0.43162 0.31811 0.61092 0.13781 0.40253 0.86816 0.65637 iter=6 0.83806 0.63451 0.30709 0.56256 0.28575 0.98335 0.027015 0.53589 0.07099 0.0010682 0.36576 0.66222 0.39221 0.25747 iter=7 0.078833 0.59929 0.33202 0.46314 0.37315 0.21315 0.39492 0.78153 0.48377 0.89643 0.15491 0.089704 0.69175 0.053365 iter=8 0.080963 0.86037 0.12972 0.34177 0.91353 0.21968 0.10242 0.16101 0.07712 0.38079 0.63936 0.76418 0.25369 0.97772 iter=9 0.64568 0.8924 0.25498 0.54607 0.58975 0.67769 0.25611 0.59788 0.14263 0.41166 0.80073 0.04882 0.059971 0.70515 iter=10 0.85868 0.81771 0.74813 0.14479 0.54152 0.28472 0.61894 0.081868 0.15807 0.50336 0.58728 0.36127 0.16222 0.94328 iter=11 0.96825 0.16458 0.16478 0.74261 0.028002 0.25225 0.10017 0.01852 0.9345 0.55469 0.92675 0.30777 0.060783 0.56524 iter=12 0.1969 0.57233 0.40896 0.89774 0.73858 0.41014 0.087086 0.84457 0.5896 0.48261 0.046195 0.35677 0.61654 0.4003 iter=13 0.7696 0.7521 0.065643 0.23089 0.73688 0.26338 0.6102 0.71823 0.43715 0.49321 0.76788 0.80542 0.17448 0.4922 iter=14 0.87914 0.32933 0.29478 0.13038 0.76043 0.2589 0.98693 0.050377 0.35549 0.48429 0.59645 0.6638 0.17858 0.93874 iter=15 0.13906 0.080566 0.80056 0.63086 0.29385 0.91211 0.23488 0.62486 0.89472 0.33287 0.026973 0.60954 0.19947 0.61812 iter=16 0.61684 0.37843 0.80594 0.2139 0.24558 0.017367 0.7743 0.75744 0.86587 0.3458 0.93122 0.60821 0.39296 0.69167 iter=17 0.21929 0.062088 0.94646 0.06358 0.063255 0.80713 0.11057 0.51591 0.90181 0.46244 0.37784 0.61629 0.84053 0.21283 iter=18 0.084434 0.38868 0.2933 0.20612 0.47792 0.011002 0.2714 0.58585 0.13806 0.29136 0.26919 0.7366 0.25824 0.6023 iter=19 0.63113 0.073997 0.50118 0.10616 0.9169 0.21948 0.8723 0.49979 0.57061 0.61371 0.73601 0.9635 0.47096 0.91519 iter=20 0.5274 0.15303 0.61045 0.71262 0.069739 0.43425 0.28106 0.70083 0.03044 0.83774 0.67971 0.76083 0.57855 0.74045 iter=21 0.524 0.56519 0.80474 0.33874 0.98354 0.45657 0.93516 0.95553 0.67231 0.21628 0.99276 0.60306 0.69368 0.64938 iter=22 0.6644 0.24262 0.50776 0.22419 0.17099 0.97047 0.74029 0.041459 0.017391 0.39027 0.45606 0.20557 0.1121 0.72959 iter=23 0.47978 0.943 0.92961 0.19067 0.59085 0.66953 0.52257 0.035979 0.2742 0.60429 0.84621 0.028522 0.5481 0.41601 iter=24 0.030097 0.43874 0.81558 0.53407 0.64506 0.63165 0.018992 0.68209 0.90214 0.64731 0.60216 0.45157 0.32215 0.72784 iter=25 0.3496 0.30107 0.3688 0.066709 0.10605 0.16278 0.66116 0.98712 0.66721 0.13585 0.54165 0.85079 0.050832 0.40626 iter=26 0.44759 0.93037 0.076426 0.0052793 0.32739 0.0099438 0.32503 0.21856 0.66827 0.99372 0.38004 0.71858 0.64787 0.474 iter=27 0.62167 0.20725 0.61421 0.36504 0.43767 0.95666 0.70433 0.53865 0.70147 0.010843 0.06189 0.66506 0.88816 0.1605 iter=28 0.1938 0.07505 0.82739 0.98347 0.31973 0.42098 0.87266 0.39964 0.4976 0.71662 0.80563 0.47446 0.57152 0.53027 iter=29 0.050638 0.79752 0.47567 0.27882 0.89455 0.9742 0.37374 0.91875 0.57585 0.54215 0.63998 0.066988 0.19813 0.20001 iter=30 0.66765 0.31044 0.84112 0.42436 0.5999 0.78574 0.78843 0.89136 0.82625 0.62855 0.71104 0.84333 0.17631 0.27342 iter=31 0.45338 0.3528 0.3063 0.89196 0.12713 0.12718 0.80447 0.077428 0.20284 0.42681 0.24138 0.17642 0.8631 0.28619 iter=32 0.72847 0.13669 0.73949 0.26936 0.96391 0.044505 0.95236 0.63282 0.8177 0.1294 0.55092 0.94132 0.25006 0.56737 iter=33 0.8789 0.6787 0.92172 0.54815 0.61392 0.19968 0.14407 0.39912 0.46075 0.39333 0.65953 0.4917 0.020323 0.17514 iter=34 0.13874 0.35696 0.80629 0.94446 0.40271 0.04518 0.44324 0.27942 0.2078 0.41143 0.061147 0.36185 0.017039 0.11774 iter=35 0.11592 0.52555 0.2157 0.41042 0.12645 0.37627 0.21036 0.58805 0.39942 0.38708 0.98267 0.535 0.099954 0.80203 iter=36 0.38571 0.34928 0.8993 0.65987 0.38068 0.95344 0.085861 0.81377 0.49138 0.90749 0.17542 0.63173 0.66657 0.88681 iter=37 0.34773 0.1854 0.55341 0.26358 0.43175 0.61682 0.48622 0.33203 0.15906 0.60177 0.42664 0.94236 0.87129 0.58787 iter=38 0.224 0.34123 0.12302 0.83195 0.24057 0.65441 0.98976 0.80707 0.76826 0.33509 0.18021 0.25833 0.49582 0.672 iter=39 0.18655 0.55935 0.35713 0.94888 0.31439 0.67695 0.48702 0.62604 0.27473 0.83551 0.52188 0.8928 0.18652 0.78156 iter=40 0.21332 0.64916 0.12508 0.83649 0.75029 0.018384 0.90047 0.047986 0.95865 0.89407 0.49043 0.8998 0.89869 0.054707 iter=41 0.5012 0.79472 0.095606 0.95543 0.50733 0.02342 0.35842 0.26698 0.35266 0.37611 0.29825 0.11132 0.22696 0.35745 iter=42 0.077822 0.46655 0.31378 0.56781 0.71834 0.61832 0.77598 0.21008 0.4424 0.59956 0.20551 0.12701 0.70611 0.073841 iter=43 0.8907 0.21679 0.94108 0.042091 0.94354 0.20528 0.61792 0.032646 0.34099 0.50801 0.89066 0.76168 0.12194 0.202 iter=44 0.44676 0.9434 0.89726 0.13299 0.44359 0.46273 0.57954 0.58099 0.98357 0.20747 0.58012 0.48075 0.22757 0.4578 iter=45 0.85983 0.65321 0.68922 0.16515 0.72057 0.44862 0.48829 0.97481 0.91687 0.98086 0.87447 0.32556 0.25834 0.62886 iter=46 0.82015 0.30915 0.65747 0.49911 0.84642 0.86775 0.26196 0.11809 0.67989 0.90434 0.85251 0.47996 0.78906 0.85487 iter=47 0.47359 0.45008 0.30119 0.92037 0.22877 0.30362 0.75519 0.39567 0.69318 0.15213 0.26758 0.45685 0.19833 0.77006 iter=48 0.59055 0.18595 0.22508 0.5431 0.67806 0.43042 0.99561 0.88454 0.76692 0.70306 0.64252 0.79024 0.64472 0.76307 iter=49 0.77125 0.3372 0.45245 0.9408 0.87297 0.69834 0.20633 0.0060929 0.18169 0.78122 0.76316 0.096782 0.97625 0.22556 iter=50 0.060302 0.14395 0.82117 0.64693 0.99977 0.23528 0.83903 0.48095 0.68508 0.1889 0.14532 0.41386 0.50614 0.79691 tb_val: V(a,z) value at each state space point z1_0_34741 z2_0_40076 z3_0_4623 z4_0_5333 z5_0_61519 z6_0_70966 z10_1_2567 z11_1_4496 z12_1_6723 z13_1_9291 z14_2_2253 z15_2_567 __________ __________ _________ _________ __________ __________ __________ __________ __________ __________ __________ _________ a1=0 -0.81039 -0.66753 -0.52468 -0.38182 -0.23896 -0.096106 0.47532 0.61818 0.76104 0.90389 1.0468 1.1896 a2=0.0667557 -0.66727 -0.54232 -0.41526 -0.28629 -0.15564 -0.023481 0.51698 0.65439 0.7925 0.93123 1.0705 1.2102 a3=0.133511 -0.54209 -0.43106 -0.31664 -0.1991 -0.078726 0.044224 0.55697 0.68934 0.82301 0.95784 1.0937 1.2304 a4=0.200267 -0.43085 -0.33094 -0.22688 -0.11891 -0.007309 0.10764 0.59543 0.7231 0.85261 0.98375 1.1163 1.2502 a5=0.267023 -0.33075 -0.23995 -0.14451 -0.044667 0.059346 0.16726 0.63246 0.75577 0.88136 1.009 1.1385 1.2696 a6=0.333778 -0.23977 -0.15654 -0.068419 0.024439 0.12183 0.22354 0.66817 0.7874 0.90931 1.0337 1.1602 1.2887 a7=0.400534 -0.15638 -0.079564 0.002291 0.089077 0.18065 0.27681 0.70264 0.81806 0.9365 1.0577 1.1814 1.3073 a8=0.46729 -0.079416 -0.0080889 0.06833 0.14979 0.23619 0.32739 0.73597 0.84781 0.96297 1.0812 1.2022 1.3257 a9=0.534045 -0.0079511 0.058616 0.13028 0.20703 0.28881 0.37553 0.76822 0.87669 0.98876 1.1041 1.2225 1.3437 a10=0.600801 0.058745 0.12115 0.18861 0.26116 0.3388 0.42146 0.79947 0.90477 1.0139 1.1266 1.2425 1.3614 a11=0.667557 0.12127 0.18 0.24372 0.31252 0.38641 0.46538 0.82976 0.93208 1.0384 1.1485 1.262 1.3788 a12=0.734312 0.18011 0.23558 0.29596 0.36137 0.43186 0.50744 0.85917 0.95867 1.0624 1.17 1.2812 1.3959 a13=0.801068 0.23569 0.28823 0.3456 0.40794 0.47533 0.54781 0.88774 0.98456 1.0857 1.191 1.3 1.4127 a14=0.867824 0.28833 0.33825 0.3929 0.45244 0.51698 0.58661 0.91551 1.0098 1.1086 1.2116 1.3185 1.4292 a15=0.934579 0.33835 0.38589 0.43806 0.49504 0.55698 0.62396 0.94253 1.0344 1.1309 1.2317 1.3367 1.4454 a16=1.00134 0.38598 0.43135 0.48126 0.5359 0.59543 0.65997 0.96885 1.0585 1.1527 1.2515 1.3545 1.4614 a17=1.06809 0.43144 0.47484 0.52268 0.57516 0.63246 0.69473 0.99448 1.0819 1.1741 1.2709 1.372 1.4772 a18=1.13485 0.47493 0.51652 0.56245 0.61293 0.66817 0.72831 1.0195 1.1048 1.195 1.2899 1.3892 1.4927 a19=1.2016 0.5166 0.55653 0.6007 0.64933 0.70264 0.76081 1.0439 1.1273 1.2155 1.3086 1.4061 1.5079 a20=1.26836 0.55661 0.595 0.63754 0.68445 0.73597 0.79228 1.0677 1.1492 1.2356 1.3269 1.4227 1.523 a21=1.33511 0.59508 0.63205 0.67307 0.71838 0.76822 0.8228 1.0909 1.1706 1.2553 1.3449 1.4391 1.5378 a22=1.40187 0.63212 0.66777 0.70738 0.7512 0.79947 0.85241 1.1136 1.1916 1.2746 1.3625 1.4552 1.5524 a23=1.46862 0.66784 0.70226 0.74055 0.78297 0.82977 0.88116 1.1359 1.2122 1.2936 1.3799 1.471 1.5668 a24=1.53538 0.70233 0.7356 0.77266 0.81376 0.85917 0.90912 1.1576 1.2324 1.3122 1.397 1.4866 1.5809 a25=1.60214 0.73567 0.76787 0.80376 0.84364 0.88774 0.93631 1.1789 1.2521 1.3304 1.4138 1.502 1.5949 a26=1.66889 0.76793 0.79912 0.83393 0.87264 0.91551 0.96279 1.1997 1.2715 1.3484 1.4303 1.5171 1.6087 a27=1.73565 0.79918 0.82943 0.86322 0.90083 0.94254 0.98858 1.2201 1.2905 1.366 1.4465 1.532 1.6223 a28=1.8024 0.82949 0.85885 0.89167 0.92825 0.96885 1.0137 1.2401 1.3091 1.3833 1.4625 1.5467 1.6357 a29=1.86916 0.8589 0.88742 0.91934 0.95494 0.99449 1.0382 1.2597 1.3275 1.4003 1.4782 1.5611 1.649 a30=1.93591 0.88748 0.9152 0.94626 0.98093 1.0195 1.0622 1.2789 1.3454 1.417 1.4937 1.5754 1.6621 a31=2.00267 0.91526 0.94223 0.97248 1.0063 1.0439 1.0856 1.2978 1.3631 1.4335 1.5089 1.5895 1.675 a32=2.06943 0.94229 0.96855 0.99802 1.031 1.0677 1.1084 1.3163 1.3805 1.4497 1.524 1.6033 1.6877 a33=2.13618 0.96861 0.9942 1.0229 1.0551 1.0909 1.1307 1.3345 1.3975 1.4656 1.5388 1.617 1.7003 a34=2.20294 0.99425 1.0192 1.0472 1.0786 1.1137 1.1526 1.3524 1.4143 1.4813 1.5533 1.6305 1.7127 a35=2.26969 1.0193 1.0436 1.071 1.1016 1.1359 1.174 1.3699 1.4308 1.4967 1.5677 1.6438 1.7249 a36=2.33645 1.0436 1.0674 1.0941 1.1241 1.1576 1.1949 1.3871 1.447 1.5119 1.5819 1.6569 1.7371 a37=2.4032 1.0675 1.0907 1.1168 1.1461 1.1789 1.2154 1.4041 1.463 1.5269 1.5958 1.6699 1.749 a38=2.46996 1.0907 1.1134 1.1389 1.1676 1.1997 1.2355 1.4208 1.4787 1.5416 1.6096 1.6827 1.7609 a39=2.53672 1.1134 1.1356 1.1606 1.1887 1.2201 1.2552 1.4371 1.4942 1.5562 1.6232 1.6953 1.7726 a40=2.60347 1.1357 1.1574 1.1818 1.2093 1.2401 1.2745 1.4533 1.5094 1.5705 1.6366 1.7078 1.7841 a41=2.67023 1.1574 1.1786 1.2026 1.2295 1.2597 1.2935 1.4691 1.5244 1.5846 1.6498 1.7201 1.7955 a42=2.73698 1.1787 1.1995 1.2229 1.2493 1.2789 1.312 1.4848 1.5392 1.5986 1.6629 1.7323 1.8068 a43=2.80374 1.1995 1.2199 1.2429 1.2688 1.2978 1.3303 1.5002 1.5538 1.6123 1.6758 1.7444 1.818 a44=2.87049 1.2199 1.2399 1.2624 1.2878 1.3163 1.3482 1.5153 1.5682 1.6259 1.6885 1.7562 1.8291 a45=2.93725 1.2399 1.2595 1.2816 1.3065 1.3345 1.3658 1.5302 1.5823 1.6392 1.7011 1.768 1.84 a46=3.00401 1.2595 1.2787 1.3004 1.3249 1.3524 1.3832 1.5449 1.5963 1.6524 1.7135 1.7796 1.8508 a47=3.07076 1.2788 1.2976 1.3189 1.3429 1.3699 1.4002 1.5594 1.6101 1.6654 1.7257 1.7911 1.8615 a48=3.13752 1.2976 1.3161 1.337 1.3606 1.3871 1.4169 1.5737 1.6236 1.6783 1.7379 1.8024 1.8721 a49=3.20427 1.3162 1.3343 1.3548 1.378 1.4041 1.4334 1.5878 1.637 1.691 1.7498 1.8136 1.8825 a50=3.27103 1.3343 1.3522 1.3723 1.3951 1.4208 1.4495 1.6017 1.6503 1.7035 1.7616 1.8247 1.8929 a701=46.729 3.8783 3.8797 3.8813 3.8832 3.8854 3.8878 3.9021 3.9071 3.9128 3.9193 3.9268 3.9354 a702=46.7957 3.8797 3.8811 3.8827 3.8846 3.8868 3.8892 3.9035 3.9084 3.9141 3.9207 3.9282 3.9367 a703=46.8625 3.8811 3.8825 3.8842 3.886 3.8882 3.8906 3.9048 3.9098 3.9155 3.922 3.9295 3.9381 a704=46.9292 3.8825 3.8839 3.8856 3.8874 3.8896 3.892 3.9062 3.9112 3.9169 3.9234 3.9309 3.9394 a705=46.996 3.8839 3.8853 3.887 3.8888 3.891 3.8934 3.9076 3.9126 3.9182 3.9247 3.9322 3.9407 a706=47.0628 3.8854 3.8868 3.8884 3.8902 3.8924 3.8948 3.909 3.9139 3.9196 3.9261 3.9335 3.9421 a707=47.1295 3.8868 3.8882 3.8898 3.8916 3.8938 3.8962 3.9103 3.9153 3.9209 3.9274 3.9349 3.9434 a708=47.1963 3.8882 3.8896 3.8912 3.893 3.8952 3.8976 3.9117 3.9166 3.9223 3.9288 3.9362 3.9447 a709=47.263 3.8896 3.891 3.8926 3.8944 3.8965 3.899 3.9131 3.918 3.9237 3.9301 3.9376 3.946 a710=47.3298 3.891 3.8924 3.894 3.8958 3.8979 3.9004 3.9145 3.9194 3.925 3.9315 3.9389 3.9474 a711=47.3965 3.8924 3.8937 3.8953 3.8972 3.8993 3.9018 3.9158 3.9207 3.9264 3.9328 3.9402 3.9487 a712=47.4633 3.8937 3.8951 3.8967 3.8986 3.9007 3.9031 3.9172 3.9221 3.9277 3.9342 3.9415 3.95 a713=47.53 3.8951 3.8965 3.8981 3.9 3.9021 3.9045 3.9185 3.9234 3.9291 3.9355 3.9429 3.9513 a714=47.5968 3.8965 3.8979 3.8995 3.9014 3.9035 3.9059 3.9199 3.9248 3.9304 3.9368 3.9442 3.9526 a715=47.6636 3.8979 3.8993 3.9009 3.9027 3.9048 3.9073 3.9213 3.9261 3.9317 3.9382 3.9455 3.954 a716=47.7303 3.8993 3.9007 3.9023 3.9041 3.9062 3.9087 3.9226 3.9275 3.9331 3.9395 3.9468 3.9553 a717=47.7971 3.9007 3.9021 3.9037 3.9055 3.9076 3.91 3.924 3.9288 3.9344 3.9408 3.9482 3.9566 a718=47.8638 3.9021 3.9035 3.905 3.9069 3.909 3.9114 3.9253 3.9302 3.9358 3.9422 3.9495 3.9579 a719=47.9306 3.9035 3.9048 3.9064 3.9082 3.9103 3.9128 3.9267 3.9315 3.9371 3.9435 3.9508 3.9592 a720=47.9973 3.9048 3.9062 3.9078 3.9096 3.9117 3.9141 3.928 3.9329 3.9384 3.9448 3.9521 3.9605 a721=48.0641 3.9062 3.9076 3.9092 3.911 3.9131 3.9155 3.9294 3.9342 3.9398 3.9461 3.9534 3.9618 a722=48.1308 3.9076 3.909 3.9105 3.9124 3.9145 3.9169 3.9307 3.9355 3.9411 3.9475 3.9547 3.9631 a723=48.1976 3.909 3.9103 3.9119 3.9137 3.9158 3.9182 3.932 3.9369 3.9424 3.9488 3.9561 3.9644 a724=48.2644 3.9103 3.9117 3.9133 3.9151 3.9172 3.9196 3.9334 3.9382 3.9437 3.9501 3.9574 3.9657 a725=48.3311 3.9117 3.9131 3.9146 3.9165 3.9185 3.9209 3.9347 3.9395 3.9451 3.9514 3.9587 3.967 a726=48.3979 3.9131 3.9144 3.916 3.9178 3.9199 3.9223 3.9361 3.9409 3.9464 3.9527 3.96 3.9683 a727=48.4646 3.9144 3.9158 3.9174 3.9192 3.9213 3.9236 3.9374 3.9422 3.9477 3.954 3.9613 3.9696 a728=48.5314 3.9158 3.9172 3.9187 3.9205 3.9226 3.925 3.9387 3.9435 3.949 3.9553 3.9626 3.9709 a729=48.5981 3.9172 3.9185 3.9201 3.9219 3.924 3.9263 3.9401 3.9449 3.9504 3.9567 3.9639 3.9722 a730=48.6649 3.9185 3.9199 3.9214 3.9232 3.9253 3.9277 3.9414 3.9462 3.9517 3.958 3.9652 3.9734 a731=48.7316 3.9199 3.9212 3.9228 3.9246 3.9267 3.929 3.9427 3.9475 3.953 3.9593 3.9665 3.9747 a732=48.7984 3.9212 3.9226 3.9242 3.9259 3.928 3.9304 3.944 3.9488 3.9543 3.9606 3.9678 3.976 a733=48.8652 3.9226 3.9239 3.9255 3.9273 3.9294 3.9317 3.9454 3.9501 3.9556 3.9619 3.9691 3.9773 a734=48.9319 3.9239 3.9253 3.9269 3.9286 3.9307 3.9331 3.9467 3.9515 3.9569 3.9632 3.9704 3.9786 a735=48.9987 3.9253 3.9266 3.9282 3.93 3.932 3.9344 3.948 3.9528 3.9582 3.9645 3.9716 3.9799 a736=49.0654 3.9267 3.928 3.9295 3.9313 3.9334 3.9357 3.9493 3.9541 3.9595 3.9658 3.9729 3.9811 a737=49.1322 3.928 3.9293 3.9309 3.9327 3.9347 3.9371 3.9506 3.9554 3.9608 3.9671 3.9742 3.9824 a738=49.1989 3.9293 3.9307 3.9322 3.934 3.9361 3.9384 3.952 3.9567 3.9621 3.9684 3.9755 3.9837 a739=49.2657 3.9307 3.932 3.9336 3.9353 3.9374 3.9397 3.9533 3.958 3.9634 3.9697 3.9768 3.985 a740=49.3324 3.932 3.9334 3.9349 3.9367 3.9387 3.9411 3.9546 3.9593 3.9647 3.971 3.9781 3.9862 a741=49.3992 3.9334 3.9347 3.9362 3.938 3.9401 3.9424 3.9559 3.9606 3.966 3.9722 3.9794 3.9875 a742=49.466 3.9347 3.936 3.9376 3.9394 3.9414 3.9437 3.9572 3.9619 3.9673 3.9735 3.9806 3.9888 a743=49.5327 3.936 3.9374 3.9389 3.9407 3.9427 3.9451 3.9585 3.9632 3.9686 3.9748 3.9819 3.99 a744=49.5995 3.9374 3.9387 3.9402 3.942 3.944 3.9464 3.9598 3.9645 3.9699 3.9761 3.9832 3.9913 a745=49.6662 3.9387 3.94 3.9416 3.9433 3.9454 3.9477 3.9611 3.9658 3.9712 3.9774 3.9845 3.9926 a746=49.733 3.94 3.9414 3.9429 3.9447 3.9467 3.949 3.9624 3.9671 3.9725 3.9787 3.9857 3.9938 a747=49.7997 3.9414 3.9427 3.9442 3.946 3.948 3.9503 3.9637 3.9684 3.9738 3.9799 3.987 3.9951 a748=49.8665 3.9427 3.944 3.9456 3.9473 3.9493 3.9517 3.965 3.9697 3.9751 3.9812 3.9883 3.9963 a749=49.9332 3.944 3.9454 3.9469 3.9486 3.9506 3.953 3.9663 3.971 3.9764 3.9825 3.9895 3.9976 a750=50 3.9454 3.9467 3.9482 3.9499 3.952 3.9543 3.9676 3.9723 3.9776 3.9838 3.9908 3.9989 tb_pol_a: optimal asset choice for each state space point z1_0_34741 z2_0_40076 z3_0_4623 z4_0_5333 z5_0_61519 z6_0_70966 z10_1_2567 z11_1_4496 z12_1_6723 z13_1_9291 z14_2_2253 z15_2_567 __________ __________ _________ _________ __________ __________ __________ __________ __________ __________ __________ _________ a1=0 0 0 0 0 0 0 0 0 0 0 0 0 a2=0.0667557 0.027247 0.027876 0.028601 0.029438 0.030403 0.031516 0.037962 0.040237 0.04286 0.045886 0.049377 0.053405 a3=0.133511 0.054495 0.055752 0.057202 0.058876 0.060806 0.063033 0.075925 0.080473 0.08572 0.091773 0.098755 0.10681 a4=0.200267 0.081742 0.083628 0.085804 0.088314 0.091209 0.094549 0.11389 0.12071 0.12858 0.13766 0.14813 0.16021 a5=0.267023 0.10899 0.1115 0.1144 0.11775 0.12161 0.12607 0.15185 0.16095 0.17144 0.18355 0.19751 0.21362 a6=0.333778 0.13624 0.13938 0.14301 0.14719 0.15201 0.15758 0.18981 0.20118 0.2143 0.22943 0.24689 0.26702 a7=0.400534 0.16348 0.16726 0.17161 0.17663 0.18242 0.1891 0.22777 0.24142 0.25716 0.27532 0.29626 0.32043 a8=0.46729 0.19073 0.19513 0.20021 0.20607 0.21282 0.22061 0.26574 0.28166 0.30002 0.3212 0.34564 0.37383 a9=0.534045 0.21798 0.22301 0.22881 0.2355 0.24322 0.25213 0.3037 0.32189 0.34288 0.36709 0.39502 0.42724 a10=0.600801 0.24523 0.25088 0.25741 0.26494 0.27363 0.28365 0.34166 0.36213 0.38574 0.41298 0.4444 0.48064 a11=0.667557 0.27247 0.27876 0.28601 0.29438 0.30403 0.31516 0.37962 0.40237 0.4286 0.45886 0.49377 0.53405 a12=0.734312 0.29972 0.30664 0.31461 0.32382 0.33443 0.34668 0.41759 0.4426 0.47146 0.50475 0.54315 0.58745 a13=0.801068 0.32697 0.33451 0.34321 0.35325 0.36484 0.3782 0.45555 0.48284 0.51432 0.55064 0.59253 0.64085 a14=0.867824 0.35421 0.36239 0.37182 0.38269 0.39524 0.40971 0.49351 0.52308 0.55718 0.59652 0.64191 0.69426 a15=0.934579 0.38146 0.39026 0.40042 0.41213 0.42564 0.44123 0.53147 0.56331 0.60004 0.64241 0.69128 0.74766 a16=1.00134 0.40871 0.41814 0.42902 0.44157 0.45604 0.47274 0.56944 0.60355 0.6429 0.6883 0.74066 0.80107 a17=1.06809 0.43596 0.44602 0.45762 0.47101 0.48645 0.50426 0.6074 0.64379 0.68576 0.73418 0.79004 0.85447 a18=1.13485 0.4632 0.47389 0.48622 0.50044 0.51685 0.53578 0.64536 0.68402 0.72862 0.78007 0.83942 0.90788 a19=1.2016 0.49045 0.50177 0.51482 0.52988 0.54725 0.56729 0.68332 0.72426 0.77148 0.82596 0.88879 0.96128 a20=1.26836 0.5177 0.52964 0.54342 0.55932 0.57766 0.59881 0.72129 0.7645 0.81434 0.87184 0.93817 1.0147 a21=1.33511 0.54495 0.55752 0.57202 0.58876 0.60806 0.63033 0.75925 0.80473 0.8572 0.91773 0.98755 1.0681 a22=1.40187 0.57219 0.5854 0.60063 0.6182 0.63846 0.66184 0.79721 0.84497 0.90006 0.96361 1.0369 1.1215 a23=1.46862 0.59944 0.61327 0.62923 0.64763 0.66887 0.69336 0.83517 0.88521 0.94292 1.0095 1.0863 1.1749 a24=1.53538 0.62669 0.64115 0.65783 0.67707 0.69927 0.72487 0.87314 0.92544 0.98578 1.0554 1.1357 1.2283 a25=1.60214 0.65393 0.66902 0.68643 0.70651 0.72967 0.75639 0.9111 0.96568 1.0286 1.1013 1.1851 1.2817 a26=1.66889 0.68118 0.6969 0.71503 0.73595 0.76007 0.78791 0.94906 1.0059 1.0715 1.1472 1.2344 1.3351 a27=1.73565 0.70843 0.72478 0.74363 0.76538 0.79048 0.81942 0.98702 1.0462 1.1144 1.193 1.2838 1.3885 a28=1.8024 0.73568 0.75265 0.77223 0.79482 0.82088 0.85094 1.025 1.0864 1.1572 1.2389 1.3332 1.4419 a29=1.86916 0.76292 0.78053 0.80083 0.82426 0.85128 0.88246 1.0629 1.1266 1.2001 1.2848 1.3826 1.4953 a30=1.93591 0.79017 0.8084 0.82944 0.8537 0.88169 0.91397 1.1009 1.1669 1.2429 1.3307 1.4319 1.5487 a31=2.00267 0.81742 0.83628 0.85804 0.88314 0.91209 0.94549 1.1389 1.2071 1.2858 1.3766 1.4813 1.6021 a32=2.06943 0.84467 0.86416 0.88664 0.91257 0.94249 0.977 1.1768 1.2473 1.3287 1.4225 1.5307 1.6555 a33=2.13618 0.87191 0.89203 0.91524 0.94201 0.9729 1.0085 1.2148 1.2876 1.3715 1.4684 1.5801 1.7089 a34=2.20294 0.89916 0.91991 0.94384 0.97145 1.0033 1.04 1.2528 1.3278 1.4144 1.5143 1.6295 1.7623 a35=2.26969 0.92641 0.94778 0.97244 1.0009 1.0337 1.0716 1.2907 1.368 1.4572 1.5601 1.6788 1.8158 a36=2.33645 0.95365 0.97566 1.001 1.0303 1.0641 1.1031 1.3287 1.4083 1.5001 1.606 1.7282 1.8692 a37=2.4032 0.9809 1.0035 1.0296 1.0598 1.0945 1.1346 1.3666 1.4485 1.543 1.6519 1.7776 1.9226 a38=2.46996 1.0081 1.0314 1.0582 1.0892 1.1249 1.1661 1.4046 1.4888 1.5858 1.6978 1.827 1.976 a39=2.53672 1.0354 1.0593 1.0868 1.1186 1.1553 1.1976 1.4426 1.529 1.6287 1.7437 1.8763 2.0294 a40=2.60347 1.0626 1.0872 1.1154 1.1481 1.1857 1.2291 1.4805 1.5692 1.6715 1.7896 1.9257 2.0828 a41=2.67023 1.0899 1.115 1.144 1.1775 1.2161 1.2607 1.5185 1.6095 1.7144 1.8355 1.9751 2.1362 a42=2.73698 1.1171 1.1429 1.1727 1.207 1.2465 1.2922 1.5565 1.6497 1.7573 1.8813 2.0245 2.1896 a43=2.80374 1.1444 1.1708 1.2013 1.2364 1.2769 1.3237 1.5944 1.6899 1.8001 1.9272 2.0739 2.243 a44=2.87049 1.1716 1.1987 1.2299 1.2658 1.3073 1.3552 1.6324 1.7302 1.843 1.9731 2.1232 2.2964 a45=2.93725 1.1989 1.2265 1.2585 1.2953 1.3377 1.3867 1.6703 1.7704 1.8858 2.019 2.1726 2.3498 a46=3.00401 1.2261 1.2544 1.2871 1.3247 1.3681 1.4182 1.7083 1.8107 1.9287 2.0649 2.222 2.4032 a47=3.07076 1.2534 1.2823 1.3157 1.3541 1.3985 1.4497 1.7463 1.8509 1.9716 2.1108 2.2714 2.4566 a48=3.13752 1.2806 1.3102 1.3443 1.3836 1.4289 1.4813 1.7842 1.8911 2.0144 2.1567 2.3207 2.51 a49=3.20427 1.3079 1.338 1.3729 1.413 1.4593 1.5128 1.8222 1.9314 2.0573 2.2025 2.3701 2.5634 a50=3.27103 1.3351 1.3659 1.4015 1.4425 1.4897 1.5443 1.8602 1.9716 2.1001 2.2484 2.4195 2.6168 a701=46.729 19.073 19.513 20.021 20.607 21.282 22.061 26.574 28.166 30.002 32.12 34.564 37.383 a702=46.7957 19.1 19.541 20.049 20.636 21.312 22.093 26.612 28.206 30.045 32.166 34.614 37.437 a703=46.8625 19.128 19.569 20.078 20.665 21.343 22.124 26.65 28.246 30.088 32.212 34.663 37.49 a704=46.9292 19.155 19.597 20.107 20.695 21.373 22.156 26.688 28.286 30.131 32.258 34.712 37.543 a705=46.996 19.182 19.625 20.135 20.724 21.404 22.187 26.726 28.327 30.174 32.304 34.762 37.597 a706=47.0628 19.209 19.653 20.164 20.754 21.434 22.219 26.764 28.367 30.216 32.35 34.811 37.65 a707=47.1295 19.237 19.68 20.192 20.783 21.464 22.25 26.802 28.407 30.259 32.396 34.86 37.704 a708=47.1963 19.264 19.708 20.221 20.813 21.495 22.282 26.839 28.447 30.302 32.442 34.91 37.757 a709=47.263 19.291 19.736 20.25 20.842 21.525 22.314 26.877 28.488 30.345 32.488 34.959 37.81 a710=47.3298 19.318 19.764 20.278 20.871 21.556 22.345 26.915 28.528 30.388 32.533 35.009 37.864 a711=47.3965 19.346 19.792 20.307 20.901 21.586 22.377 26.953 28.568 30.431 32.579 35.058 37.917 a712=47.4633 19.373 19.82 20.335 20.93 21.617 22.408 26.991 28.608 30.474 32.625 35.107 37.971 a713=47.53 19.4 19.848 20.364 20.96 21.647 22.44 27.029 28.649 30.516 32.671 35.157 38.024 a714=47.5968 19.427 19.876 20.393 20.989 21.677 22.471 27.067 28.689 30.559 32.717 35.206 38.077 a715=47.6636 19.455 19.903 20.421 21.019 21.708 22.503 27.105 28.729 30.602 32.763 35.255 38.131 a716=47.7303 19.482 19.931 20.45 21.048 21.738 22.534 27.143 28.769 30.645 32.809 35.305 38.184 a717=47.7971 19.509 19.959 20.478 21.078 21.769 22.566 27.181 28.809 30.688 32.855 35.354 38.238 a718=47.8638 19.536 19.987 20.507 21.107 21.799 22.597 27.219 28.85 30.731 32.901 35.404 38.291 a719=47.9306 19.564 20.015 20.536 21.136 21.829 22.629 27.257 28.89 30.774 32.946 35.453 38.344 a720=47.9973 19.591 20.043 20.564 21.166 21.86 22.66 27.295 28.93 30.816 32.992 35.502 38.398 a721=48.0641 19.618 20.071 20.593 21.195 21.89 22.692 27.333 28.97 30.859 33.038 35.552 38.451 a722=48.1308 19.645 20.099 20.621 21.225 21.921 22.723 27.371 29.011 30.902 33.084 35.601 38.505 a723=48.1976 19.673 20.126 20.65 21.254 21.951 22.755 27.409 29.051 30.945 33.13 35.65 38.558 a724=48.2644 19.7 20.154 20.679 21.284 21.981 22.786 27.447 29.091 30.988 33.176 35.7 38.611 a725=48.3311 19.727 20.182 20.707 21.313 22.012 22.818 27.485 29.131 31.031 33.222 35.749 38.665 a726=48.3979 19.754 20.21 20.736 21.342 22.042 22.849 27.523 29.172 31.074 33.268 35.799 38.718 a727=48.4646 19.782 20.238 20.765 21.372 22.073 22.881 27.561 29.212 31.116 33.314 35.848 38.772 a728=48.5314 19.809 20.266 20.793 21.401 22.103 22.912 27.599 29.252 31.159 33.359 35.897 38.825 a729=48.5981 19.836 20.294 20.822 21.431 22.133 22.944 27.637 29.292 31.202 33.405 35.947 38.879 a730=48.6649 19.863 20.322 20.85 21.46 22.164 22.975 27.675 29.333 31.245 33.451 35.996 38.932 a731=48.7316 19.891 20.349 20.879 21.49 22.194 23.007 27.713 29.373 31.288 33.497 36.046 38.985 a732=48.7984 19.918 20.377 20.908 21.519 22.225 23.038 27.751 29.413 31.331 33.543 36.095 39.039 a733=48.8652 19.945 20.405 20.936 21.549 22.255 23.07 27.789 29.453 31.374 33.589 36.144 39.092 a734=48.9319 19.972 20.433 20.965 21.578 22.285 23.101 27.827 29.493 31.416 33.635 36.194 39.146 a735=48.9987 20 20.461 20.993 21.607 22.316 23.133 27.864 29.534 31.459 33.681 36.243 39.199 a736=49.0654 20.027 20.489 21.022 21.637 22.346 23.164 27.902 29.574 31.502 33.727 36.292 39.252 a737=49.1322 20.054 20.517 21.051 21.666 22.377 23.196 27.94 29.614 31.545 33.772 36.342 39.306 a738=49.1989 20.081 20.545 21.079 21.696 22.407 23.227 27.978 29.654 31.588 33.818 36.391 39.359 a739=49.2657 20.108 20.572 21.108 21.725 22.437 23.259 28.016 29.695 31.631 33.864 36.441 39.413 a740=49.3324 20.136 20.6 21.136 21.755 22.468 23.291 28.054 29.735 31.674 33.91 36.49 39.466 a741=49.3992 20.163 20.628 21.165 21.784 22.498 23.322 28.092 29.775 31.716 33.956 36.539 39.519 a742=49.466 20.19 20.656 21.194 21.813 22.529 23.354 28.13 29.815 31.759 34.002 36.589 39.573 a743=49.5327 20.217 20.684 21.222 21.843 22.559 23.385 28.168 29.856 31.802 34.048 36.638 39.626 a744=49.5995 20.245 20.712 21.251 21.872 22.589 23.417 28.206 29.896 31.845 34.094 36.687 39.68 a745=49.6662 20.272 20.74 21.279 21.902 22.62 23.448 28.244 29.936 31.888 34.139 36.737 39.733 a746=49.733 20.299 20.768 21.308 21.931 22.65 23.48 28.282 29.976 31.931 34.185 36.786 39.786 a747=49.7997 20.326 20.795 21.337 21.961 22.681 23.511 28.32 30.017 31.974 34.231 36.836 39.84 a748=49.8665 20.354 20.823 21.365 21.99 22.711 23.543 28.358 30.057 32.016 34.277 36.885 39.893 a749=49.9332 20.381 20.851 21.394 22.02 22.741 23.574 28.396 30.097 32.059 34.323 36.934 39.947 a750=50 20.408 20.879 21.422 22.049 22.772 23.606 28.434 30.137 32.102 34.369 36.984 40
end
ans = Map with properties: Count: 7 KeyType: char ValueType: any