For Inf Tabulate Value and Policy Iteration Results, Store to Mat, Graph Results
back to Fan's Dynamic Assets Repository Table of Content.
Contents
function [result_map] = ff_az_fibs_vf_post(varargin)
FF_AZ_FIBS_VF_POST post formal informal results
In addition to regular post results
@param param_map container parameter container
@param support_map container support container
@param armt_map container container with states, choices and shocks grids that are inputs for grid based solution algorithm
@param func_map container container with function handles for consumption cash-on-hand etc.
@param result_map container contains policy function matrix, value function matrix, iteration results
@return result_map container add coh consumption and other matrixes to result_map also add table versions of val pol and iter matries
@example
bl_input_override = true; result_map = containers.Map('KeyType','char', 'ValueType','any'); result_map('mt_val') = mt_val; result_map('mt_pol_a') = mt_pol_a; result_map('ar_val_diff_norm') = ar_val_diff_norm(1:it_iter_last); result_map('ar_pol_diff_norm') = ar_pol_diff_norm(1:it_iter_last); result_map('mt_pol_perc_change') = mt_pol_perc_change(1:it_iter_last, :); result_map = ff_az_vf_post(param_map, support_map, armt_map, func_map, result_map, bl_input_override);
@include
Default
if (~isempty(varargin)) % if invoked from outside overrid fully [param_map, support_map, armt_map, func_map, result_map] = varargin{:}; else clear all; close all; % 1. internal invoke for testing it_param_set = 4; bl_input_override = true; % 2. Get Parameters [param_map, support_map] = ffs_abzr_fibs_set_default_param(it_param_set); [armt_map, func_map] = ffs_abzr_fibs_get_funcgrid(param_map, support_map, bl_input_override); % 1 for override % 3. Get Arrays and Functions params_group = values(param_map, {'it_a_n', 'it_z_n'}); [it_a_n, it_z_n] = params_group{:}; params_group = values(armt_map, {'ar_a'}); [ar_a] = params_group{:}; params_group = values(armt_map, {'ar_z_r_infbr_mesh_wage', 'ar_z_wage_mesh_r_infbr'}); [ar_z_r_inf_mesh_wage, ar_z_wage_mesh_r_inf] = params_group{:}; params_group = values(func_map, {'f_util_standin', 'f_cons_coh_fbis', 'f_cons_coh_save', 'f_coh'}); [f_util_standin, f_cons_coh_fbis, f_cons_coh_save, f_coh] = params_group{:}; % 4. Value Default mt_val = f_util_standin(ar_z_r_inf_mesh_wage, ar_a'); % 5. default optimal asset choices (overall, interesint + principle from % different formal and informal sources following how model is solved) mt_pol_a = zeros(size(mt_val)) + ... ar_a'*(cumsum(sort(ar_z_r_inf_mesh_wage))/sum(ar_z_r_inf_mesh_wage)*0.4 + 0.4); % 6. Default COH mt_coh = f_coh(ar_z_r_inf_mesh_wage, ar_a'); % 7. Set Default Consumption mt_pol_a_pos_idx = (mt_pol_a > 0); mt_pol_cons = zeros(size(mt_pol_a)); mt_pol_cons(mt_pol_a_pos_idx) = f_cons_coh_save(mt_coh(mt_pol_a_pos_idx), mt_pol_a(mt_pol_a_pos_idx)); mt_pol_cons(~mt_pol_a_pos_idx) = f_cons_coh_fbis(mt_coh(~mt_pol_a_pos_idx), mt_pol_a(~mt_pol_a_pos_idx)); % 8. Find Formal Informal Choices given Fake Data mt_pol_b_bridge = zeros(it_a_n,it_z_n); mt_pol_inf_borr_nobridge = zeros(it_a_n,it_z_n); mt_pol_for_borr = zeros(it_a_n,it_z_n); mt_pol_for_save = zeros(it_a_n,it_z_n); % 9. Solve for formal and informal combinations given the overall fake % choices. for it_z_i = 1:it_z_n for it_a_j = 1:it_a_n fl_z_r_borr = ar_z_r_inf_mesh_wage(it_z_i); fl_z_wage = ar_z_wage_mesh_r_inf(it_z_i); param_map('fl_r_inf') = fl_z_r_borr; fl_a = ar_a(it_a_j); fl_coh = f_coh(fl_z_wage, fl_a); fl_a_opti = mt_pol_a(it_a_j, it_z_i); % call formal and informal function. [~, fl_opti_b_bridge, fl_opti_inf_borr_nobridge, fl_opti_for_borr, fl_opti_for_save] = ... ffs_fibs_min_c_cost_bridge(fl_a_opti, fl_coh, ... param_map, support_map, armt_map, func_map, bl_input_override); % store savings and borrowing formal and inf optimal choices mt_pol_b_bridge(it_a_j,it_z_i) = fl_opti_b_bridge; mt_pol_inf_borr_nobridge(it_a_j,it_z_i) = fl_opti_inf_borr_nobridge; mt_pol_for_borr(it_a_j,it_z_i) = fl_opti_for_borr; mt_pol_for_save(it_a_j,it_z_i) = fl_opti_for_save; end end % 10. Set Results Map result_map = containers.Map('KeyType','char', 'ValueType','any'); result_map('mt_val') = mt_val; result_map('cl_mt_pol_a') = {mt_pol_a, zeros(1)}; result_map('cl_mt_coh') = {mt_coh, zeros(1)}; result_map('cl_mt_pol_c') = {mt_pol_cons, zeros(1)}; result_map('cl_mt_pol_b_bridge') = {mt_pol_b_bridge, zeros(1)}; result_map('cl_mt_pol_inf_borr_nobridge') = {mt_pol_inf_borr_nobridge, zeros(1)}; result_map('cl_mt_pol_for_borr') = {mt_pol_for_borr, zeros(1)}; result_map('cl_mt_pol_for_save') = {mt_pol_for_save, zeros(1)}; % Input over-ride bl_input_override = true; result_map = ffs_fibs_identify_discrete(result_map, bl_input_override); % Control which results to graph support_map('bl_graph_forinf_discrete') = true; support_map('bl_graph_forinf_pol_lvl') = true; support_map('bl_graph_forinf_pol_pct') = true; support_map('bl_graph') = true; end
Parse Parameter
% Model Name params_group = values(param_map, {'st_model'}); [st_model] = params_group{:}; % armt_map standards if (ismember(st_model, ["ipwkbzr_fibs", "abzr_fibs"])) params_group = values(param_map, {'fl_z_r_infbr_n'}); [fl_z_r_borr_n] = params_group{:}; if (ismember(st_model, ["ipwkbzr_fibs"])) params_group = values(armt_map, {'ar_z_r_infbr_mesh_wage_w1r2', 'ar_z_wage_mesh_r_infbr_w1r2'}); elseif (ismember(st_model, ["abzr_fibs"])) params_group = values(armt_map, {'ar_z_r_infbr_mesh_wage', 'ar_z_wage_mesh_r_infbr'}); end [ar_z_r_inf_mesh_wage, ar_z_wage_mesh_r_inf] = params_group{:}; else fl_z_r_borr_n = 1; params_group = values(armt_map, {'ar_z'}); [ar_z_wage_mesh_r_inf] = params_group{:}; end % result_map standards params_group = values(result_map, {'cl_mt_pol_a'}); [cl_mt_pol_a] = params_group{:}; [mt_pol_a] = deal(cl_mt_pol_a{1}); % result_map continuous formal informal choices params_group = values(result_map, {'cl_mt_pol_b_bridge', 'cl_mt_pol_inf_borr_nobridge', ... 'cl_mt_pol_for_borr', 'cl_mt_pol_for_save'}); [cl_mt_pol_b_bridge, cl_mt_pol_inf_borr_nobridge, cl_mt_pol_for_borr, cl_mt_pol_for_save] = params_group{:}; [mt_pol_b_bridge, mt_pol_inf_borr_nobridge, mt_pol_for_borr, mt_pol_for_save] = ... deal(cl_mt_pol_b_bridge{1}, cl_mt_pol_inf_borr_nobridge{1}, cl_mt_pol_for_borr{1}, cl_mt_pol_for_save{1}); % support_map params_group = values(support_map, {'bl_display_final', 'it_display_final_rowmax', 'it_display_final_colmax'}); [bl_display_final, it_display_final_rowmax, it_display_final_colmax] = params_group{:}; params_group = values(support_map, {'bl_graph', 'bl_graph_onebyones'}); [bl_graph] = params_group{:}; params_group = values(support_map, {'bl_mat', 'st_mat_path', 'st_mat_prefix', 'st_mat_name_main', 'st_mat_suffix'}); [bl_mat, st_mat_path, st_mat_prefix, st_mat_name_main, st_mat_suffix] = params_group{:};
Get Size of Endogenous and Exogenous State
it_endostates_n = size(mt_pol_a, 1); it_exostates_n = size(mt_pol_a, 2);
Generate Consumption and Income Matrix
if (~isKey(result_map, 'cl_mt_pol_c')) params_group = values(armt_map, {'ar_a', 'ar_z'}); [ar_a, ar_z_r_inf_mesh_wage] = params_group{:}; f_cons = func_map('f_cons'); mt_cons = f_cons(ar_z_r_inf_mesh_wage, ar_a', mt_pol_a); result_map('cl_mt_pol_c') = {mt_cons, zeros(1)}; end if (~isKey(result_map, 'cl_mt_coh')) params_group = values(armt_map, {'ar_a', 'ar_z'}); [ar_a, ar_z_r_inf_mesh_wage] = params_group{:}; f_coh = func_map('f_coh'); mt_coh = f_coh(ar_z_r_inf_mesh_wage, ar_a'); result_map('cl_mt_coh') = {mt_coh, zeros(1)}; else params_group = values(result_map, {'cl_mt_coh'}); [cl_mt_coh] = params_group{:}; [mt_coh] = deal(cl_mt_coh{1}); end
Save Mat
if (bl_mat) mkdir(support_map('st_mat_path')); st_file_name = [st_mat_prefix st_mat_name_main st_mat_suffix]; save(strcat(st_mat_path, st_file_name)); end
Generate and Save Graphs
if (bl_graph) bl_input_override = true; ff_az_fibs_vf_post_graph(param_map, support_map, armt_map, func_map, result_map, bl_input_override); end
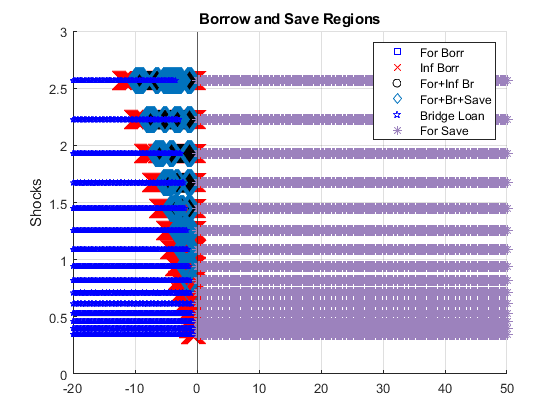
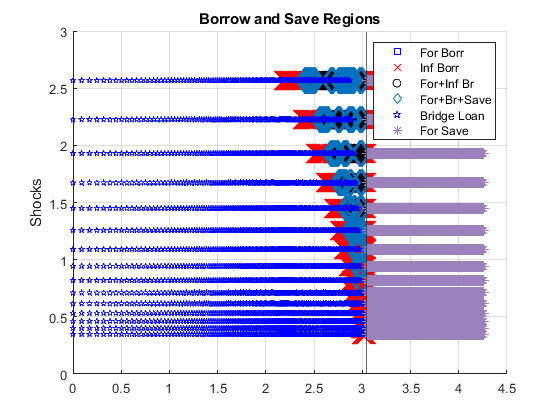
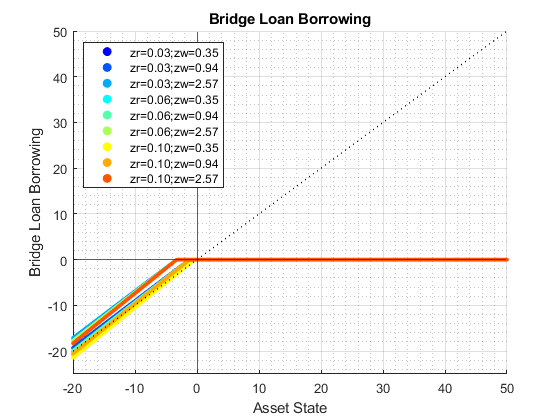
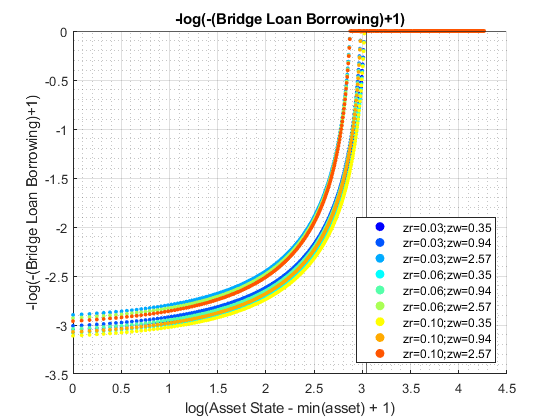
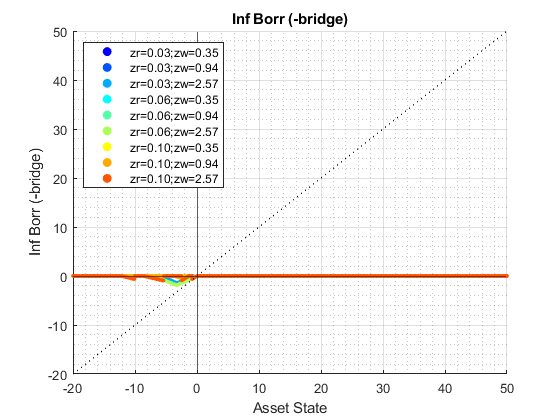
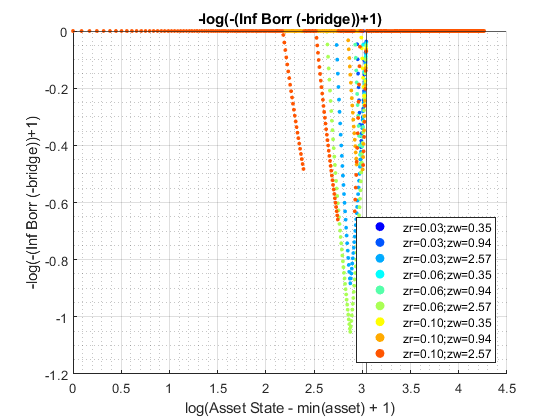
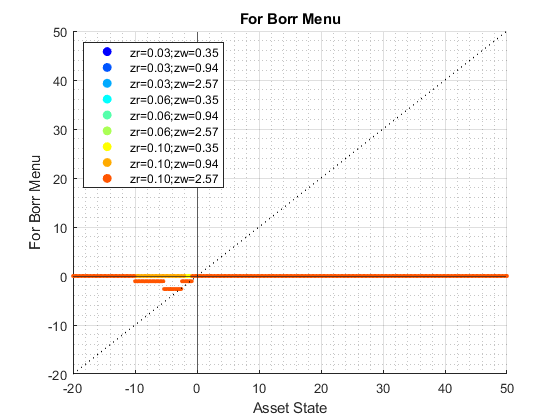
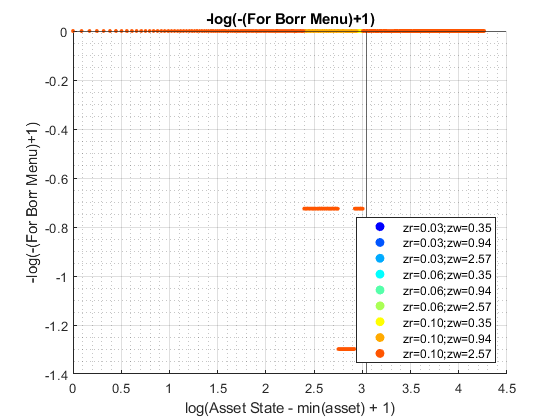
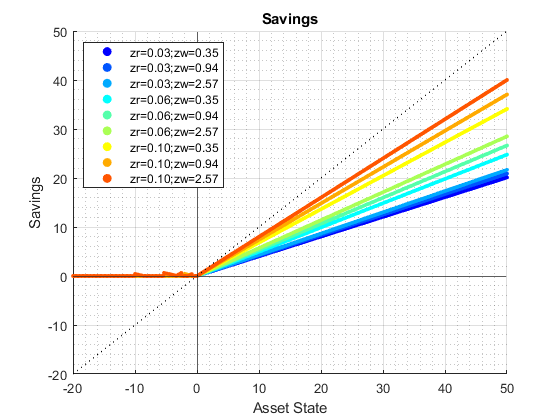
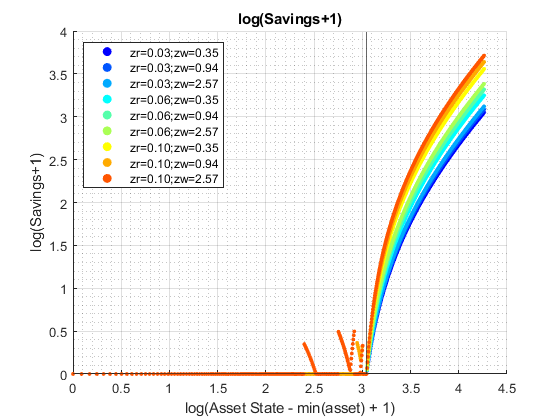
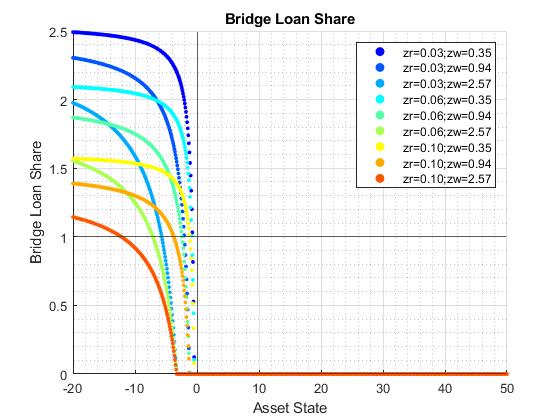
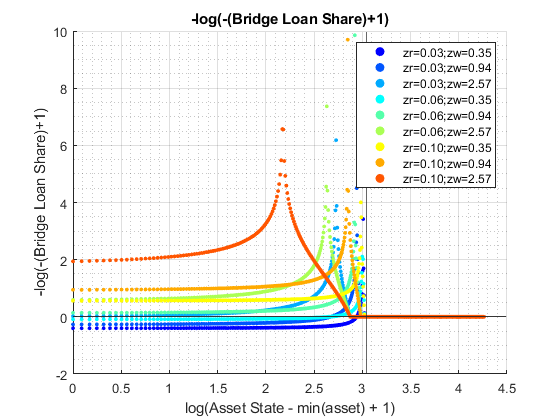
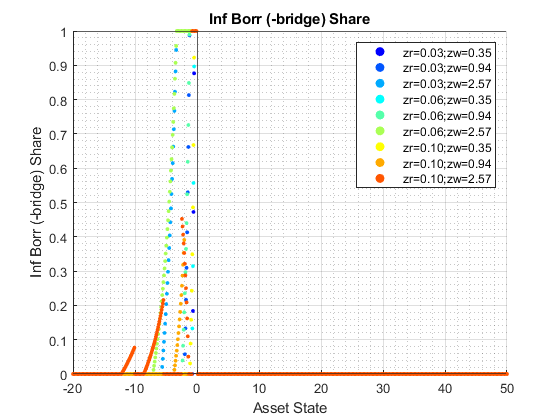
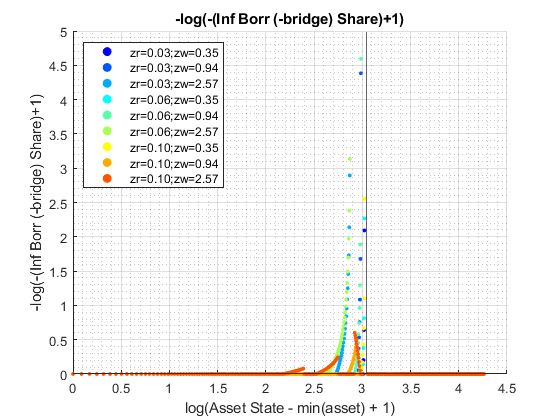
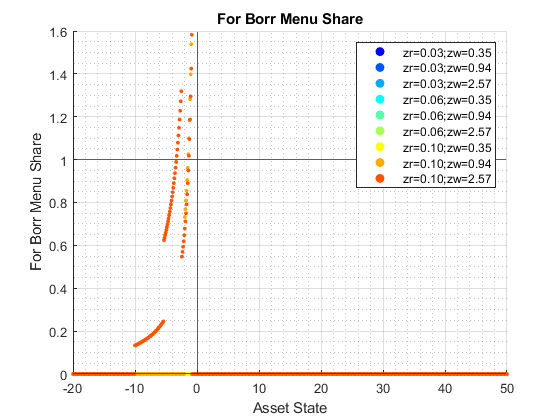
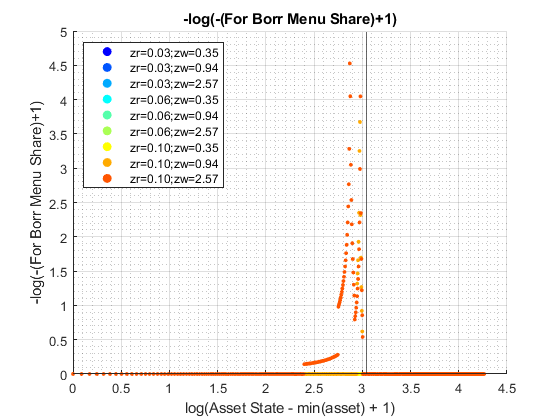
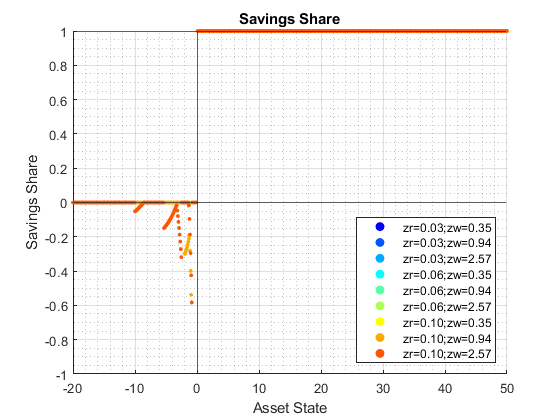
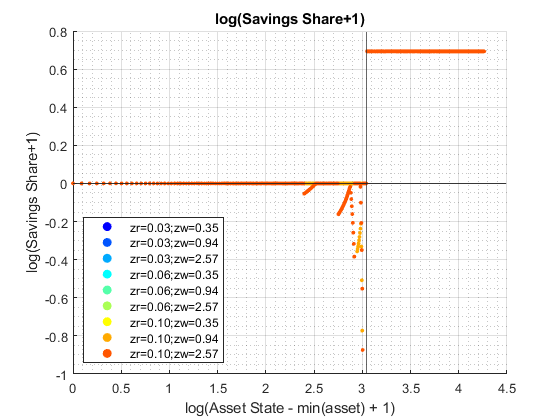
Display Val Pol Iter Table
if (bl_display_final) % Display Values by States % at most display 11 columns of shocks % at most display 50 rows for states % display first and last if (it_endostates_n >= it_display_final_rowmax) ar_it_rows = (1:1:round(it_display_final_rowmax/2)); ar_it_rows = [ar_it_rows ((it_endostates_n)-round(it_display_final_rowmax/2)+1):1:(it_endostates_n)]; else ar_it_rows = 1:1:it_endostates_n; end ar_it_rows = unique(ar_it_rows); if (it_exostates_n >= it_display_final_colmax) ar_it_cols = (1:1:round(it_display_final_colmax/2)); ar_it_cols = [ar_it_cols ((it_exostates_n)-round(it_display_final_colmax/2)+1):1:(it_exostates_n)]; else ar_it_cols = 1:1:it_exostates_n; end ar_it_cols = unique(ar_it_cols); mt_pol_b_bridge_print = mt_pol_b_bridge(ar_it_rows, ar_it_cols); mt_pol_inf_borr_nobridge_print = mt_pol_inf_borr_nobridge(ar_it_rows, ar_it_cols); mt_pol_for_borr_print = mt_pol_for_borr(ar_it_rows, ar_it_cols); mt_pol_for_save_print = mt_pol_for_save(ar_it_rows, ar_it_cols); % Column Z Names if (fl_z_r_borr_n == 1) ar_st_col_zs = matlab.lang.makeValidName(strcat('z', string(ar_it_cols), '=', string(ar_z_wage_mesh_r_inf(ar_it_cols)))); else ar_st_col_zs = matlab.lang.makeValidName(strcat('zi', string(ar_it_cols), ... ':zr=', string(ar_z_r_inf_mesh_wage(ar_it_cols)), ... ';zw=', string(ar_z_wage_mesh_r_inf(ar_it_cols)))); end % Display Optimal Values tb_mt_pol_b_bridge_print = array2table(mt_pol_b_bridge_print); tb_mt_pol_b_bridge_print.Properties.RowNames = strcat('coh', string(ar_it_rows), '=', string(mt_coh(ar_it_rows))); tb_mt_pol_b_bridge_print.Properties.VariableNames = ar_st_col_zs; disp('mt_pol_b_bridge_print: bridge loans'); disp(tb_mt_pol_b_bridge_print); % Display Optimal Values tb_mt_pol_inf_borr_nobridge_print = array2table(mt_pol_inf_borr_nobridge_print); tb_mt_pol_inf_borr_nobridge_print.Properties.RowNames = strcat('coh', string(ar_it_rows), '=', string(mt_coh(ar_it_rows))); tb_mt_pol_inf_borr_nobridge_print.Properties.VariableNames = ar_st_col_zs; disp('mt_pol_inf_borr_nobridge_print: Informal loans that is not bridge loan'); disp(tb_mt_pol_inf_borr_nobridge_print); % Display Optimal Values tb_mt_pol_for_borr_print = array2table(mt_pol_for_borr_print); tb_mt_pol_for_borr_print.Properties.RowNames = strcat('coh', string(ar_it_rows), '=', string(mt_coh(ar_it_rows))); tb_mt_pol_for_borr_print.Properties.VariableNames = ar_st_col_zs; disp('mt_pol_for_borr_print: formal borrowing'); disp(tb_mt_pol_for_borr_print); % Display Optimal Values tb_mt_pol_for_save_print = array2table(mt_pol_for_save_print); tb_mt_pol_for_save_print.Properties.RowNames = strcat('coh', string(ar_it_rows), '=', string(mt_coh(ar_it_rows))); tb_mt_pol_for_save_print.Properties.VariableNames = ar_st_col_zs; disp('mt_pol_for_save_print: formal savings'); disp(tb_mt_pol_for_save_print); end
mt_pol_b_bridge_print: bridge loans zi1_zr_0_025_zw_0_34741 zi2_zr_0_025_zw_0_40076 zi3_zr_0_025_zw_0_4623 zi4_zr_0_025_zw_0_5333 zi5_zr_0_025_zw_0_61519 zi6_zr_0_025_zw_0_70966 zi7_zr_0_025_zw_0_81864 zi8_zr_0_025_zw_0_94436 zi68_zr_0_095_zw_0_94436 zi69_zr_0_095_zw_1_0894 zi70_zr_0_095_zw_1_2567 zi71_zr_0_095_zw_1_4496 zi72_zr_0_095_zw_1_6723 zi73_zr_0_095_zw_1_9291 zi74_zr_0_095_zw_2_2253 zi75_zr_0_095_zw_2_567 _______________________ _______________________ ______________________ ______________________ _______________________ _______________________ _______________________ _______________________ ________________________ _______________________ _______________________ _______________________ _______________________ _______________________ _______________________ ______________________ coh1=-19.968 -20.044 -19.974 -19.893 -19.8 -19.693 -19.569 -19.426 -19.261 -20.576 -20.373 -20.139 -19.868 -19.556 -19.196 -18.781 -18.302 coh2=-19.8745 -19.948 -19.878 -19.798 -19.705 -19.597 -19.473 -19.33 -19.165 -20.474 -20.271 -20.036 -19.766 -19.454 -19.094 -18.679 -18.2 coh3=-19.7811 -19.853 -19.783 -19.702 -19.609 -19.501 -19.377 -19.234 -19.069 -20.372 -20.168 -19.934 -19.664 -19.351 -18.992 -18.576 -18.097 coh4=-19.6876 -19.757 -19.687 -19.606 -19.513 -19.405 -19.282 -19.139 -18.974 -20.269 -20.066 -19.832 -19.561 -19.249 -18.889 -18.474 -17.995 coh5=-19.5942 -19.661 -19.591 -19.51 -19.417 -19.31 -19.186 -19.043 -18.878 -20.167 -19.964 -19.729 -19.459 -19.147 -18.787 -18.372 -17.893 coh6=-19.5007 -19.565 -19.495 -19.414 -19.321 -19.214 -19.09 -18.947 -18.782 -20.065 -19.861 -19.627 -19.356 -19.044 -18.685 -18.269 -17.79 coh7=-19.4073 -19.469 -19.399 -19.319 -19.226 -19.118 -18.994 -18.851 -18.686 -19.962 -19.759 -19.525 -19.254 -18.942 -18.582 -18.167 -17.688 coh8=-19.3138 -19.374 -19.304 -19.223 -19.13 -19.022 -18.898 -18.755 -18.59 -19.86 -19.657 -19.422 -19.152 -18.84 -18.48 -18.065 -17.586 coh9=-19.2203 -19.278 -19.208 -19.127 -19.034 -18.927 -18.803 -18.66 -18.495 -19.758 -19.554 -19.32 -19.049 -18.737 -18.378 -17.962 -17.483 coh10=-19.1269 -19.182 -19.112 -19.031 -18.938 -18.831 -18.707 -18.564 -18.399 -19.655 -19.452 -19.218 -18.947 -18.635 -18.275 -17.86 -17.381 coh11=-19.0334 -19.086 -19.016 -18.936 -18.842 -18.735 -18.611 -18.468 -18.303 -19.553 -19.35 -19.115 -18.845 -18.533 -18.173 -17.758 -17.279 coh12=-18.94 -18.99 -18.92 -18.84 -18.747 -18.639 -18.515 -18.372 -18.207 -19.451 -19.247 -19.013 -18.742 -18.43 -18.071 -17.655 -17.176 coh13=-18.8465 -18.895 -18.825 -18.744 -18.651 -18.543 -18.419 -18.276 -18.111 -19.348 -19.145 -18.911 -18.64 -18.328 -17.968 -17.553 -17.074 coh14=-18.753 -18.799 -18.729 -18.648 -18.555 -18.448 -18.324 -18.181 -18.016 -19.246 -19.043 -18.808 -18.538 -18.226 -17.866 -17.451 -16.972 coh15=-18.6596 -18.703 -18.633 -18.552 -18.459 -18.352 -18.228 -18.085 -17.92 -19.144 -18.94 -18.706 -18.435 -18.123 -17.764 -17.348 -16.869 coh16=-18.5661 -18.607 -18.537 -18.457 -18.363 -18.256 -18.132 -17.989 -17.824 -19.041 -18.838 -18.604 -18.333 -18.021 -17.661 -17.246 -16.767 coh17=-18.4727 -18.511 -18.441 -18.361 -18.268 -18.16 -18.036 -17.893 -17.728 -18.939 -18.736 -18.501 -18.231 -17.919 -17.559 -17.144 -16.665 coh18=-18.3792 -18.416 -18.346 -18.265 -18.172 -18.064 -17.94 -17.797 -17.632 -18.837 -18.633 -18.399 -18.128 -17.816 -17.457 -17.041 -16.562 coh19=-18.2858 -18.32 -18.25 -18.169 -18.076 -17.969 -17.845 -17.702 -17.537 -18.734 -18.531 -18.297 -18.026 -17.714 -17.354 -16.939 -16.46 coh20=-18.1923 -18.224 -18.154 -18.073 -17.98 -17.873 -17.749 -17.606 -17.441 -18.632 -18.429 -18.194 -17.924 -17.612 -17.252 -16.837 -16.358 coh21=-18.0988 -18.128 -18.058 -17.978 -17.884 -17.777 -17.653 -17.51 -17.345 -18.53 -18.326 -18.092 -17.821 -17.509 -17.149 -16.734 -16.255 coh22=-18.0054 -18.033 -17.963 -17.882 -17.789 -17.681 -17.557 -17.414 -17.249 -18.427 -18.224 -17.99 -17.719 -17.407 -17.047 -16.632 -16.153 coh23=-17.9119 -17.937 -17.867 -17.786 -17.693 -17.585 -17.461 -17.318 -17.154 -18.325 -18.122 -17.887 -17.617 -17.305 -16.945 -16.53 -16.051 coh24=-17.8185 -17.841 -17.771 -17.69 -17.597 -17.49 -17.366 -17.223 -17.058 -18.223 -18.019 -17.785 -17.514 -17.202 -16.842 -16.427 -15.948 coh25=-17.725 -17.745 -17.675 -17.594 -17.501 -17.394 -17.27 -17.127 -16.962 -18.12 -17.917 -17.683 -17.412 -17.1 -16.74 -16.325 -15.846 coh26=-17.6316 -17.649 -17.579 -17.499 -17.405 -17.298 -17.174 -17.031 -16.866 -18.018 -17.815 -17.58 -17.31 -16.998 -16.638 -16.223 -15.744 coh27=-17.5381 -17.554 -17.484 -17.403 -17.31 -17.202 -17.078 -16.935 -16.77 -17.916 -17.712 -17.478 -17.207 -16.895 -16.535 -16.12 -15.641 coh28=-17.4446 -17.458 -17.388 -17.307 -17.214 -17.106 -16.982 -16.839 -16.675 -17.813 -17.61 -17.376 -17.105 -16.793 -16.433 -16.018 -15.539 coh29=-17.3512 -17.362 -17.292 -17.211 -17.118 -17.011 -16.887 -16.744 -16.579 -17.711 -17.508 -17.273 -17.003 -16.691 -16.331 -15.916 -15.437 coh30=-17.2577 -17.266 -17.196 -17.115 -17.022 -16.915 -16.791 -16.648 -16.483 -17.609 -17.405 -17.171 -16.9 -16.588 -16.228 -15.813 -15.334 coh31=-17.1643 -17.17 -17.1 -17.02 -16.926 -16.819 -16.695 -16.552 -16.387 -17.506 -17.303 -17.069 -16.798 -16.486 -16.126 -15.711 -15.232 coh32=-17.0708 -17.075 -17.005 -16.924 -16.831 -16.723 -16.599 -16.456 -16.291 -17.404 -17.201 -16.966 -16.696 -16.384 -16.024 -15.609 -15.13 coh33=-16.9773 -16.979 -16.909 -16.828 -16.735 -16.627 -16.504 -16.361 -16.196 -17.302 -17.098 -16.864 -16.593 -16.281 -15.921 -15.506 -15.027 coh34=-16.8839 -16.883 -16.813 -16.732 -16.639 -16.532 -16.408 -16.265 -16.1 -17.199 -16.996 -16.762 -16.491 -16.179 -15.819 -15.404 -14.925 coh35=-16.7904 -16.787 -16.717 -16.636 -16.543 -16.436 -16.312 -16.169 -16.004 -17.097 -16.894 -16.659 -16.389 -16.077 -15.717 -15.302 -14.823 coh36=-16.697 -16.691 -16.621 -16.541 -16.448 -16.34 -16.216 -16.073 -15.908 -16.995 -16.791 -16.557 -16.286 -15.974 -15.614 -15.199 -14.72 coh37=-16.6035 -16.596 -16.526 -16.445 -16.352 -16.244 -16.12 -15.977 -15.812 -16.892 -16.689 -16.455 -16.184 -15.872 -15.512 -15.097 -14.618 coh38=-16.5101 -16.5 -16.43 -16.349 -16.256 -16.148 -16.025 -15.882 -15.717 -16.79 -16.587 -16.352 -16.082 -15.77 -15.41 -14.995 -14.516 coh39=-16.4166 -16.404 -16.334 -16.253 -16.16 -16.053 -15.929 -15.786 -15.621 -16.688 -16.484 -16.25 -15.979 -15.667 -15.307 -14.892 -14.413 coh40=-16.3231 -16.308 -16.238 -16.157 -16.064 -15.957 -15.833 -15.69 -15.525 -16.585 -16.382 -16.148 -15.877 -15.565 -15.205 -14.79 -14.311 coh41=-16.2297 -16.212 -16.142 -16.062 -15.969 -15.861 -15.737 -15.594 -15.429 -16.483 -16.28 -16.045 -15.775 -15.463 -15.103 -14.688 -14.209 coh42=-16.1362 -16.117 -16.047 -15.966 -15.873 -15.765 -15.641 -15.498 -15.333 -16.381 -16.177 -15.943 -15.672 -15.36 -15 -14.585 -14.106 coh43=-16.0428 -16.021 -15.951 -15.87 -15.777 -15.67 -15.546 -15.403 -15.238 -16.278 -16.075 -15.841 -15.57 -15.258 -14.898 -14.483 -14.004 coh44=-15.9493 -15.925 -15.855 -15.774 -15.681 -15.574 -15.45 -15.307 -15.142 -16.176 -15.973 -15.738 -15.468 -15.156 -14.796 -14.381 -13.902 coh45=-15.8559 -15.829 -15.759 -15.679 -15.585 -15.478 -15.354 -15.211 -15.046 -16.074 -15.87 -15.636 -15.365 -15.053 -14.693 -14.278 -13.799 coh46=-15.7624 -15.733 -15.663 -15.583 -15.49 -15.382 -15.258 -15.115 -14.95 -15.971 -15.768 -15.534 -15.263 -14.951 -14.591 -14.176 -13.697 coh47=-15.6689 -15.638 -15.568 -15.487 -15.394 -15.286 -15.162 -15.019 -14.854 -15.869 -15.666 -15.431 -15.161 -14.849 -14.489 -14.074 -13.595 coh48=-15.5755 -15.542 -15.472 -15.391 -15.298 -15.191 -15.067 -14.924 -14.759 -15.767 -15.563 -15.329 -15.058 -14.746 -14.386 -13.971 -13.492 coh49=-15.482 -15.446 -15.376 -15.295 -15.202 -15.095 -14.971 -14.828 -14.663 -15.664 -15.461 -15.227 -14.956 -14.644 -14.284 -13.869 -13.39 coh50=-15.3886 -15.35 -15.28 -15.2 -15.106 -14.999 -14.875 -14.732 -14.567 -15.562 -15.359 -15.124 -14.854 -14.542 -14.182 -13.767 -13.288 coh701=45.4526 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh702=45.546 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh703=45.6395 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh704=45.7329 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh705=45.8264 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh706=45.9199 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh707=46.0133 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh708=46.1068 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh709=46.2002 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh710=46.2937 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh711=46.3871 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh712=46.4806 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh713=46.5741 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh714=46.6675 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh715=46.761 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh716=46.8544 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh717=46.9479 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh718=47.0413 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh719=47.1348 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh720=47.2283 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh721=47.3217 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh722=47.4152 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh723=47.5086 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh724=47.6021 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh725=47.6956 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh726=47.789 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh727=47.8825 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh728=47.9759 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh729=48.0694 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh730=48.1628 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh731=48.2563 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh732=48.3498 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh733=48.4432 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh734=48.5367 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh735=48.6301 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh736=48.7236 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh737=48.817 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh738=48.9105 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh739=49.004 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh740=49.0974 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh741=49.1909 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh742=49.2843 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh743=49.3778 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh744=49.4713 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh745=49.5647 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh746=49.6582 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh747=49.7516 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh748=49.8451 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh749=49.9385 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh750=50.032 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 mt_pol_inf_borr_nobridge_print: Informal loans that is not bridge loan zi1_zr_0_025_zw_0_34741 zi2_zr_0_025_zw_0_40076 zi3_zr_0_025_zw_0_4623 zi4_zr_0_025_zw_0_5333 zi5_zr_0_025_zw_0_61519 zi6_zr_0_025_zw_0_70966 zi7_zr_0_025_zw_0_81864 zi8_zr_0_025_zw_0_94436 zi68_zr_0_095_zw_0_94436 zi69_zr_0_095_zw_1_0894 zi70_zr_0_095_zw_1_2567 zi71_zr_0_095_zw_1_4496 zi72_zr_0_095_zw_1_6723 zi73_zr_0_095_zw_1_9291 zi74_zr_0_095_zw_2_2253 zi75_zr_0_095_zw_2_567 _______________________ _______________________ ______________________ ______________________ _______________________ _______________________ _______________________ _______________________ ________________________ _______________________ _______________________ _______________________ _______________________ _______________________ _______________________ ______________________ coh1=-19.968 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh2=-19.8745 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh3=-19.7811 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh4=-19.6876 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh5=-19.5942 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh6=-19.5007 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh7=-19.4073 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh8=-19.3138 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh9=-19.2203 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh10=-19.1269 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh11=-19.0334 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh12=-18.94 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh13=-18.8465 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh14=-18.753 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh15=-18.6596 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh16=-18.5661 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh17=-18.4727 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh18=-18.3792 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh19=-18.2858 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh20=-18.1923 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh21=-18.0988 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh22=-18.0054 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh23=-17.9119 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh24=-17.8185 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh25=-17.725 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh26=-17.6316 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh27=-17.5381 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh28=-17.4446 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh29=-17.3512 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh30=-17.2577 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh31=-17.1643 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh32=-17.0708 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh33=-16.9773 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh34=-16.8839 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh35=-16.7904 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh36=-16.697 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh37=-16.6035 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh38=-16.5101 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh39=-16.4166 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh40=-16.3231 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh41=-16.2297 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh42=-16.1362 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh43=-16.0428 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh44=-15.9493 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh45=-15.8559 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh46=-15.7624 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh47=-15.6689 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh48=-15.5755 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh49=-15.482 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh50=-15.3886 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh701=45.4526 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh702=45.546 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh703=45.6395 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh704=45.7329 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh705=45.8264 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh706=45.9199 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh707=46.0133 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh708=46.1068 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh709=46.2002 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh710=46.2937 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh711=46.3871 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh712=46.4806 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh713=46.5741 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh714=46.6675 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh715=46.761 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh716=46.8544 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh717=46.9479 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh718=47.0413 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh719=47.1348 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh720=47.2283 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh721=47.3217 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh722=47.4152 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh723=47.5086 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh724=47.6021 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh725=47.6956 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh726=47.789 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh727=47.8825 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh728=47.9759 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh729=48.0694 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh730=48.1628 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh731=48.2563 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh732=48.3498 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh733=48.4432 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh734=48.5367 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh735=48.6301 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh736=48.7236 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh737=48.817 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh738=48.9105 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh739=49.004 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh740=49.0974 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh741=49.1909 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh742=49.2843 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh743=49.3778 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh744=49.4713 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh745=49.5647 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh746=49.6582 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh747=49.7516 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh748=49.8451 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh749=49.9385 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh750=50.032 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 mt_pol_for_borr_print: formal borrowing zi1_zr_0_025_zw_0_34741 zi2_zr_0_025_zw_0_40076 zi3_zr_0_025_zw_0_4623 zi4_zr_0_025_zw_0_5333 zi5_zr_0_025_zw_0_61519 zi6_zr_0_025_zw_0_70966 zi7_zr_0_025_zw_0_81864 zi8_zr_0_025_zw_0_94436 zi68_zr_0_095_zw_0_94436 zi69_zr_0_095_zw_1_0894 zi70_zr_0_095_zw_1_2567 zi71_zr_0_095_zw_1_4496 zi72_zr_0_095_zw_1_6723 zi73_zr_0_095_zw_1_9291 zi74_zr_0_095_zw_2_2253 zi75_zr_0_095_zw_2_567 _______________________ _______________________ ______________________ ______________________ _______________________ _______________________ _______________________ _______________________ ________________________ _______________________ _______________________ _______________________ _______________________ _______________________ _______________________ ______________________ coh1=-19.968 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh2=-19.8745 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh3=-19.7811 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh4=-19.6876 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh5=-19.5942 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh6=-19.5007 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh7=-19.4073 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh8=-19.3138 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh9=-19.2203 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh10=-19.1269 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh11=-19.0334 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh12=-18.94 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh13=-18.8465 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh14=-18.753 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh15=-18.6596 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh16=-18.5661 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh17=-18.4727 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh18=-18.3792 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh19=-18.2858 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh20=-18.1923 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh21=-18.0988 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh22=-18.0054 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh23=-17.9119 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh24=-17.8185 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh25=-17.725 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh26=-17.6316 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh27=-17.5381 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh28=-17.4446 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh29=-17.3512 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh30=-17.2577 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh31=-17.1643 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh32=-17.0708 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh33=-16.9773 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh34=-16.8839 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh35=-16.7904 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh36=-16.697 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh37=-16.6035 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh38=-16.5101 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh39=-16.4166 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh40=-16.3231 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh41=-16.2297 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh42=-16.1362 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh43=-16.0428 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh44=-15.9493 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh45=-15.8559 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh46=-15.7624 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh47=-15.6689 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh48=-15.5755 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh49=-15.482 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh50=-15.3886 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh701=45.4526 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh702=45.546 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh703=45.6395 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh704=45.7329 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh705=45.8264 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh706=45.9199 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh707=46.0133 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh708=46.1068 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh709=46.2002 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh710=46.2937 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh711=46.3871 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh712=46.4806 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh713=46.5741 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh714=46.6675 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh715=46.761 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh716=46.8544 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh717=46.9479 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh718=47.0413 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh719=47.1348 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh720=47.2283 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh721=47.3217 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh722=47.4152 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh723=47.5086 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh724=47.6021 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh725=47.6956 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh726=47.789 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh727=47.8825 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh728=47.9759 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh729=48.0694 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh730=48.1628 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh731=48.2563 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh732=48.3498 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh733=48.4432 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh734=48.5367 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh735=48.6301 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh736=48.7236 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh737=48.817 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh738=48.9105 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh739=49.004 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh740=49.0974 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh741=49.1909 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh742=49.2843 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh743=49.3778 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh744=49.4713 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh745=49.5647 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh746=49.6582 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh747=49.7516 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh748=49.8451 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh749=49.9385 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh750=50.032 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 mt_pol_for_save_print: formal savings zi1_zr_0_025_zw_0_34741 zi2_zr_0_025_zw_0_40076 zi3_zr_0_025_zw_0_4623 zi4_zr_0_025_zw_0_5333 zi5_zr_0_025_zw_0_61519 zi6_zr_0_025_zw_0_70966 zi7_zr_0_025_zw_0_81864 zi8_zr_0_025_zw_0_94436 zi68_zr_0_095_zw_0_94436 zi69_zr_0_095_zw_1_0894 zi70_zr_0_095_zw_1_2567 zi71_zr_0_095_zw_1_4496 zi72_zr_0_095_zw_1_6723 zi73_zr_0_095_zw_1_9291 zi74_zr_0_095_zw_2_2253 zi75_zr_0_095_zw_2_567 _______________________ _______________________ ______________________ ______________________ _______________________ _______________________ _______________________ _______________________ ________________________ _______________________ _______________________ _______________________ _______________________ _______________________ _______________________ ______________________ coh1=-19.968 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh2=-19.8745 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh3=-19.7811 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh4=-19.6876 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh5=-19.5942 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh6=-19.5007 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh7=-19.4073 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh8=-19.3138 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh9=-19.2203 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh10=-19.1269 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh11=-19.0334 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh12=-18.94 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh13=-18.8465 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh14=-18.753 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh15=-18.6596 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh16=-18.5661 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh17=-18.4727 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh18=-18.3792 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh19=-18.2858 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh20=-18.1923 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh21=-18.0988 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh22=-18.0054 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh23=-17.9119 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh24=-17.8185 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh25=-17.725 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh26=-17.6316 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh27=-17.5381 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh28=-17.4446 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh29=-17.3512 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh30=-17.2577 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh31=-17.1643 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh32=-17.0708 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh33=-16.9773 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh34=-16.8839 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh35=-16.7904 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh36=-16.697 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh37=-16.6035 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh38=-16.5101 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh39=-16.4166 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh40=-16.3231 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh41=-16.2297 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh42=-16.1362 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh43=-16.0428 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh44=-15.9493 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh45=-15.8559 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh46=-15.7624 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh47=-15.6689 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh48=-15.5755 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh49=-15.482 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh50=-15.3886 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 coh701=45.4526 18.269 18.37 18.471 18.572 18.673 18.774 18.875 18.976 33.652 34.035 34.419 34.802 35.186 35.569 35.953 36.336 coh702=45.546 18.307 18.408 18.509 18.61 18.711 18.812 18.914 19.015 33.721 34.105 34.49 34.874 35.258 35.643 36.027 36.411 coh703=45.6395 18.344 18.446 18.547 18.648 18.75 18.851 18.952 19.054 33.79 34.175 34.56 34.945 35.331 35.716 36.101 36.486 coh704=45.7329 18.382 18.483 18.585 18.687 18.788 18.89 18.991 19.093 33.859 34.245 34.631 35.017 35.403 35.789 36.175 36.561 coh705=45.8264 18.42 18.521 18.623 18.725 18.827 18.928 19.03 19.132 33.929 34.315 34.702 35.089 35.475 35.862 36.249 36.636 coh706=45.9199 18.457 18.559 18.661 18.763 18.865 18.967 19.069 19.171 33.998 34.385 34.773 35.16 35.548 35.935 36.323 36.71 coh707=46.0133 18.495 18.597 18.699 18.801 18.903 19.006 19.108 19.21 34.067 34.455 34.844 35.232 35.62 36.008 36.397 36.785 coh708=46.1068 18.532 18.635 18.737 18.839 18.942 19.044 19.147 19.249 34.136 34.525 34.914 35.304 35.693 36.082 36.471 36.86 coh709=46.2002 18.57 18.672 18.775 18.878 18.98 19.083 19.185 19.288 34.206 34.595 34.985 35.375 35.765 36.155 36.545 36.935 coh710=46.2937 18.607 18.71 18.813 18.916 19.019 19.121 19.224 19.327 34.275 34.665 35.056 35.447 35.837 36.228 36.619 37.009 coh711=46.3871 18.645 18.748 18.851 18.954 19.057 19.16 19.263 19.366 34.344 34.735 35.127 35.518 35.91 36.301 36.693 37.084 coh712=46.4806 18.683 18.786 18.889 18.992 19.096 19.199 19.302 19.405 34.413 34.805 35.198 35.59 35.982 36.374 36.767 37.159 coh713=46.5741 18.72 18.824 18.927 19.031 19.134 19.237 19.341 19.444 34.482 34.876 35.269 35.662 36.055 36.448 36.841 37.234 coh714=46.6675 18.758 18.861 18.965 19.069 19.172 19.276 19.38 19.483 34.552 34.946 35.339 35.733 36.127 36.521 36.915 37.308 coh715=46.761 18.795 18.899 19.003 19.107 19.211 19.315 19.418 19.522 34.621 35.016 35.41 35.805 36.199 36.594 36.989 37.383 coh716=46.8544 18.833 18.937 19.041 19.145 19.249 19.353 19.457 19.561 34.69 35.086 35.481 35.876 36.272 36.667 37.063 37.458 coh717=46.9479 18.871 18.975 19.079 19.183 19.288 19.392 19.496 19.6 34.759 35.156 35.552 35.948 36.344 36.74 37.137 37.533 coh718=47.0413 18.908 19.013 19.117 19.222 19.326 19.431 19.535 19.639 34.829 35.226 35.623 36.02 36.417 36.814 37.211 37.607 coh719=47.1348 18.946 19.05 19.155 19.26 19.364 19.469 19.574 19.679 34.898 35.296 35.693 36.091 36.489 36.887 37.284 37.682 coh720=47.2283 18.983 19.088 19.193 19.298 19.403 19.508 19.613 19.718 34.967 35.366 35.764 36.163 36.561 36.96 37.358 37.757 coh721=47.3217 19.021 19.126 19.231 19.336 19.441 19.546 19.652 19.757 35.036 35.436 35.835 36.234 36.634 37.033 37.432 37.832 coh722=47.4152 19.059 19.164 19.269 19.374 19.48 19.585 19.69 19.796 35.106 35.506 35.906 36.306 36.706 37.106 37.506 37.907 coh723=47.5086 19.096 19.202 19.307 19.413 19.518 19.624 19.729 19.835 35.175 35.576 35.977 36.378 36.779 37.179 37.58 37.981 coh724=47.6021 19.134 19.239 19.345 19.451 19.557 19.662 19.768 19.874 35.244 35.646 36.048 36.449 36.851 37.253 37.654 38.056 coh725=47.6956 19.171 19.277 19.383 19.489 19.595 19.701 19.807 19.913 35.313 35.716 36.118 36.521 36.923 37.326 37.728 38.131 coh726=47.789 19.209 19.315 19.421 19.527 19.633 19.74 19.846 19.952 35.383 35.786 36.189 36.592 36.996 37.399 37.802 38.206 coh727=47.8825 19.247 19.353 19.459 19.566 19.672 19.778 19.885 19.991 35.452 35.856 36.26 36.664 37.068 37.472 37.876 38.28 coh728=47.9759 19.284 19.391 19.497 19.604 19.71 19.817 19.923 20.03 35.521 35.926 36.331 36.736 37.141 37.545 37.95 38.355 coh729=48.0694 19.322 19.428 19.535 19.642 19.749 19.855 19.962 20.069 35.59 35.996 36.402 36.807 37.213 37.619 38.024 38.43 coh730=48.1628 19.359 19.466 19.573 19.68 19.787 19.894 20.001 20.108 35.66 36.066 36.472 36.879 37.285 37.692 38.098 38.505 coh731=48.2563 19.397 19.504 19.611 19.718 19.826 19.933 20.04 20.147 35.729 36.136 36.543 36.951 37.358 37.765 38.172 38.579 coh732=48.3498 19.434 19.542 19.649 19.757 19.864 19.971 20.079 20.186 35.798 36.206 36.614 37.022 37.43 37.838 38.246 38.654 coh733=48.4432 19.472 19.58 19.687 19.795 19.902 20.01 20.118 20.225 35.867 36.276 36.685 37.094 37.503 37.911 38.32 38.729 coh734=48.5367 19.51 19.617 19.725 19.833 19.941 20.049 20.156 20.264 35.937 36.346 36.756 37.165 37.575 37.985 38.394 38.804 coh735=48.6301 19.547 19.655 19.763 19.871 19.979 20.087 20.195 20.303 36.006 36.416 36.827 37.237 37.647 38.058 38.468 38.879 coh736=48.7236 19.585 19.693 19.801 19.909 20.018 20.126 20.234 20.342 36.075 36.486 36.897 37.309 37.72 38.131 38.542 38.953 coh737=48.817 19.622 19.731 19.839 19.948 20.056 20.164 20.273 20.381 36.144 36.556 36.968 37.38 37.792 38.204 38.616 39.028 coh738=48.9105 19.66 19.769 19.877 19.986 20.094 20.203 20.312 20.42 36.214 36.626 37.039 37.452 37.865 38.277 38.69 39.103 coh739=49.004 19.698 19.806 19.915 20.024 20.133 20.242 20.351 20.459 36.283 36.696 37.11 37.523 37.937 38.35 38.764 39.178 coh740=49.0974 19.735 19.844 19.953 20.062 20.171 20.28 20.389 20.498 36.352 36.766 37.181 37.595 38.009 38.424 38.838 39.252 coh741=49.1909 19.773 19.882 19.991 20.101 20.21 20.319 20.428 20.537 36.421 36.836 37.252 37.667 38.082 38.497 38.912 39.327 coh742=49.2843 19.81 19.92 20.029 20.139 20.248 20.358 20.467 20.577 36.491 36.906 37.322 37.738 38.154 38.57 38.986 39.402 coh743=49.3778 19.848 19.958 20.067 20.177 20.287 20.396 20.506 20.616 36.56 36.976 37.393 37.81 38.227 38.643 39.06 39.477 coh744=49.4713 19.886 19.995 20.105 20.215 20.325 20.435 20.545 20.655 36.629 37.046 37.464 37.881 38.299 38.716 39.134 39.551 coh745=49.5647 19.923 20.033 20.143 20.253 20.363 20.474 20.584 20.694 36.698 37.117 37.535 37.953 38.371 38.79 39.208 39.626 coh746=49.6582 19.961 20.071 20.181 20.292 20.402 20.512 20.622 20.733 36.767 37.187 37.606 38.025 38.444 38.863 39.282 39.701 coh747=49.7516 19.998 20.109 20.219 20.33 20.44 20.551 20.661 20.772 36.837 37.257 37.676 38.096 38.516 38.936 39.356 39.776 coh748=49.8451 20.036 20.147 20.257 20.368 20.479 20.589 20.7 20.811 36.906 37.327 37.747 38.168 38.589 39.009 39.43 39.85 coh749=49.9385 20.074 20.184 20.295 20.406 20.517 20.628 20.739 20.85 36.975 37.397 37.818 38.24 38.661 39.082 39.504 39.925 coh750=50.032 20.111 20.222 20.333 20.444 20.556 20.667 20.778 20.889 37.044 37.467 37.889 38.311 38.733 39.156 39.578 40
end
ans = Map with properties: Count: 14 KeyType: char ValueType: any