Generate States/Choices/Shocks Grids, get Functions (Interpolated + Percentage + Risky + Safe Asset + Save + Borrow)
back to Fan's Dynamic Assets Repository Table of Content.
Contents
- FFS_IPWKBZ_GET_FUNCGRID get funcs, params, states choices shocks grids
- Default
- Parse Parameters
- Generate Asset and Choice Grid for 2nd stage Problem
- Get Shock Grids
- Get Equations
- Generate Cash-on-Hand/State Matrix
- Check if COH is within Borrowing Bounds
- Update Valid 2nd stage choice matrix
- Select only Valid (k(w), a) choices
- Generate 1st Stage States: Interpolation Cash-on-hand Interpolation Grid
- Generate 1st Stage Choices: Interpolation Cash-on-hand Interpolation Grid
- Generate Interpolation Consumption Grid
- Initialize armt_map to store, state, choice, shock matrixes
- Store armt_map (1): 2nd Stage Problem Arrays and Matrixes
- Store armt_map (2): First Stage Aggregate Savings
- Store armt_map (3): First Stage Consumption and Cash-on-Hand Grids
- Store armt_map (4): Shock Grids
- Store Function Map
- Graph
- Graph 1: a and k choice grid graphs
- Graph 2: coh by shock
- Graph 3: 1st State Aggregate Savings Choices by COH interpolation grids
- Graph Details, Generally do Not Run
- Graph 1: 2nd stage coh reached by k' b' choices by index
- Graph 2: 2nd stage coh reached by k' b' choices by coh
- Display
- Display
function [armt_map, func_map] = ffs_ipwkbz_get_funcgrid(varargin)
FFS_IPWKBZ_GET_FUNCGRID get funcs, params, states choices shocks grids
centralized gateway for retrieving parameters, and solution grids and functions. Similar to ffs_akz_get_funcgrid function. This code deals with problems with savings and borrowing.
The graphs below show the difference between percentage choice grid and level choice grid. See comments by graphs below for explanations of differences between the choice grids here and choice grids in the ffs_akz_get_funcgrid function.
Note that for borrowing, we can not start at the min(coh(k,w-k,z)) reacheable given the w and k choice grids. That would be: min(coh(k,w-k,z)) < min(w). At min(coh), there is a single point, and also for all values between min(coh) and min(w), all percentage w_perc choices are below min(w), requiring extrapolation. It is in some sense safer to extrapolate by starting solution at min(coh) = min(w). Now, for the lowest levels of coh(k, w-k, z), which are < min(w), we now require extrapolation. But this extrapolation is straight forward, when coh < min(w), households have to default, the utility from default is the same regardless of the level of coh at the time of default. So nearest extrapolation is fully correct.
Note that the first stage w grid is based on cash-on-hand level reached by the coh(k,w-k,z) possible choice and shock combinations. This coh(k,w-k,z) > max(w), which also means that at max(coh) grid, the w_perc choices at higher points require extrapolation. Extrapolation is based on nearest extrapolation.
Note that even when w = 0, as long as interest rate is low, only the lowest level of borrowing is invalid.
@param param_map container parameter container
@param support_map container support container
@param bl_input_override boolean if true varargin contained param_map and support_map fully overrides local default. Local default is not invoked. This could be important for speed if this function is getting invoked within certain loops. Default is 0.
@return armt_map container container with states, choices and shocks grids that are inputs for grid based solution algorithm
@return func_map container container with function handles for consumption cash-on-hand etc.
@example
it_param_set = 2; bl_input_override = true; [param_map, support_map] = ffs_ipwkbz_set_default_param(it_param_set); [armt_map, func_map] = ffs_ipwkbz_get_funcgrid(param_map, support_map, bl_input_override);
@include
Default
if (~isempty(varargin)) % override when called from outside [param_map, support_map] = varargin{:}; else % default internal run [param_map, support_map] = ffs_ipwkbz_set_default_param(); support_map('bl_graph_funcgrids') = true; support_map('bl_graph_funcgrids_detail') = true; support_map('bl_display_funcgrids') = true; % to be able to visually see choice grid points param_map('fl_b_bd') = -20; % borrow bound, = 0 if save only param_map('fl_default_aprime') = 0; param_map('bl_default') = 0; % if borrowing is default allowed param_map('fl_w_min') = param_map('fl_b_bd'); param_map('it_w_perc_n') = 25; param_map('it_ak_perc_n') = 45; param_map('fl_w_interp_grid_gap') = 2; param_map('fl_coh_interp_grid_gap') = 2; default_maps = {param_map, support_map}; % numvarargs is the number of varagin inputted [default_maps{1:length(varargin)}] = varargin{:}; param_map = [param_map; default_maps{1}]; support_map = [support_map; default_maps{2}]; end
---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Display Parameters Specific to IPWKBZR xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ---------------------------------------- ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Begin: Show all key and value pairs from container CONTAINER NAME: PARAM_MAP ---------------------------------------- Map with properties: Count: 9 KeyType: char ValueType: any xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ---------------------------------------- ---------------------------------------- pos = 1 ; key = bl_default ; val = true pos = 2 ; key = fl_b_bd ; val = -20 pos = 3 ; key = fl_c_min ; val = 0.02 pos = 4 ; key = fl_default_wprime ; val = 0 pos = 5 ; key = fl_k_max ; val = 70 pos = 6 ; key = fl_w_max ; val = 50 pos = 7 ; key = fl_w_min ; val = -20 pos = 8 ; key = st_model ; val = ipwkbz pos = 9 ; key = st_v_coh_z_interp_method ; val = method_cell ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Scalars in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx value _ ___ _____ bl_default 1 1 1 fl_b_bd 2 2 -20 fl_c_min 3 3 0.02 fl_default_wprime 4 4 0 fl_k_max 5 5 70 fl_w_max 6 6 50 fl_w_min 7 7 -20 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Strings in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx _ ___ st_model 1 8 st_v_coh_z_interp_method 2 9 ---------------------------------------- ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Begin: Show all key and value pairs from container CONTAINER NAME: SUPPORT_MAP ---------------------------------------- Map with properties: Count: 5 KeyType: char ValueType: any xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ---------------------------------------- ---------------------------------------- pos = 1 ; key = st_img_path ; val = C:/Users/fan/CodeDynaAsset//m_ipwkbzr//solve/img/ pos = 2 ; key = st_mat_path ; val = C:/Users/fan/CodeDynaAsset//m_ipwkbzr//solve/mat/ pos = 3 ; key = st_mat_test_path ; val = C:/Users/fan/CodeDynaAsset//m_ipwkbzr//test/ff_ipwkbz_ds_vecsv/mat/ pos = 4 ; key = st_matimg_path_root ; val = C:/Users/fan/CodeDynaAsset//m_ipwkbzr/ pos = 5 ; key = st_profile_path ; val = C:/Users/fan/CodeDynaAsset//m_ipwkbzr//solve/profile/ ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Strings in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx _ ___ st_img_path 1 1 st_mat_path 2 2 st_mat_test_path 3 3 st_matimg_path_root 4 4 st_profile_path 5 5 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Display All Parameters with IPWKBZR overriding IPWKBZR xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ---------------------------------------- ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Begin: Show all key and value pairs from container CONTAINER NAME: PARAM_MAP ---------------------------------------- Map with properties: Count: 35 KeyType: char ValueType: any xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ---------------------------------------- ---------------------------------------- pos = 1 ; key = bl_default ; val = true pos = 2 ; key = fl_Amean ; val = 1 pos = 3 ; key = fl_alpha ; val = 0.36 pos = 4 ; key = fl_b_bd ; val = -20 pos = 5 ; key = fl_beta ; val = 0.94 pos = 6 ; key = fl_c_min ; val = 0.02 pos = 7 ; key = fl_coh_interp_grid_gap ; val = 0.1 pos = 8 ; key = fl_crra ; val = 1.5 pos = 9 ; key = fl_default_wprime ; val = 0 pos = 10 ; key = fl_delta ; val = 0.08 pos = 11 ; key = fl_k_max ; val = 70 pos = 12 ; key = fl_k_min ; val = 0 pos = 13 ; key = fl_nan_replace ; val = -9999 pos = 14 ; key = fl_r_borr ; val = 0.095 pos = 15 ; key = fl_r_save ; val = 0.025 pos = 16 ; key = fl_tol_dist ; val = 1e-05 pos = 17 ; key = fl_tol_pol ; val = 1e-05 pos = 18 ; key = fl_tol_val ; val = 1e-05 pos = 19 ; key = fl_w ; val = 0.44365 pos = 20 ; key = fl_w_interp_grid_gap ; val = 0.1 pos = 21 ; key = fl_w_max ; val = 50 pos = 22 ; key = fl_w_min ; val = -20 pos = 23 ; key = fl_z_mu ; val = 0 pos = 24 ; key = fl_z_rho ; val = 0.8 pos = 25 ; key = fl_z_sig ; val = 0.2 pos = 26 ; key = it_ak_perc_n ; val = 50 pos = 27 ; key = it_c_interp_grid_gap ; val = 0.0001 pos = 28 ; key = it_maxiter_dist ; val = 1000 pos = 29 ; key = it_maxiter_val ; val = 250 pos = 30 ; key = it_tol_pol_nochange ; val = 25 pos = 31 ; key = it_w_perc_n ; val = 50 pos = 32 ; key = it_z_n ; val = 15 pos = 33 ; key = st_analytical_stationary_type ; val = eigenvector pos = 34 ; key = st_model ; val = ipwkbz pos = 35 ; key = st_v_coh_z_interp_method ; val = method_cell ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Scalars in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx value __ ___ _______ bl_default 1 1 1 fl_Amean 2 2 1 fl_alpha 3 3 0.36 fl_b_bd 4 4 -20 fl_beta 5 5 0.94 fl_c_min 6 6 0.02 fl_coh_interp_grid_gap 7 7 0.1 fl_crra 8 8 1.5 fl_default_wprime 9 9 0 fl_delta 10 10 0.08 fl_k_max 11 11 70 fl_k_min 12 12 0 fl_nan_replace 13 13 -9999 fl_r_borr 14 14 0.095 fl_r_save 15 15 0.025 fl_tol_dist 16 16 1e-05 fl_tol_pol 17 17 1e-05 fl_tol_val 18 18 1e-05 fl_w 19 19 0.44365 fl_w_interp_grid_gap 20 20 0.1 fl_w_max 21 21 50 fl_w_min 22 22 -20 fl_z_mu 23 23 0 fl_z_rho 24 24 0.8 fl_z_sig 25 25 0.2 it_ak_perc_n 26 26 50 it_c_interp_grid_gap 27 27 0.0001 it_maxiter_dist 28 28 1000 it_maxiter_val 29 29 250 it_tol_pol_nochange 30 30 25 it_w_perc_n 31 31 50 it_z_n 32 32 15 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Strings in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx _ ___ st_analytical_stationary_type 1 33 st_model 2 34 st_v_coh_z_interp_method 3 35 ---------------------------------------- ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Begin: Show all key and value pairs from container CONTAINER NAME: SUPPORT_MAP ---------------------------------------- Map with properties: Count: 43 KeyType: char ValueType: any xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ---------------------------------------- ---------------------------------------- pos = 1 ; key = bl_display ; val = false pos = 2 ; key = bl_display_defparam ; val = true pos = 3 ; key = bl_display_dist ; val = false pos = 4 ; key = bl_display_evf ; val = false pos = 5 ; key = bl_display_final ; val = true pos = 6 ; key = bl_display_final_dist ; val = false pos = 7 ; key = bl_display_final_dist_detail ; val = false pos = 8 ; key = bl_display_funcgrids ; val = false pos = 9 ; key = bl_graph ; val = true pos = 10 ; key = bl_graph_coh_t_coh ; val = true pos = 11 ; key = bl_graph_evf ; val = false pos = 12 ; key = bl_graph_funcgrids ; val = false pos = 13 ; key = bl_graph_funcgrids_detail ; val = false pos = 14 ; key = bl_graph_onebyones ; val = true pos = 15 ; key = bl_graph_pol_lvl ; val = true pos = 16 ; key = bl_graph_pol_pct ; val = true pos = 17 ; key = bl_graph_val ; val = true pos = 18 ; key = bl_img_save ; val = false pos = 19 ; key = bl_mat ; val = false pos = 20 ; key = bl_post ; val = true pos = 21 ; key = bl_profile ; val = false pos = 22 ; key = bl_profile_dist ; val = false pos = 23 ; key = bl_time ; val = false pos = 24 ; key = it_display_every ; val = 5 pos = 25 ; key = it_display_final_colmax ; val = 12 pos = 26 ; key = it_display_final_rowmax ; val = 100 pos = 27 ; key = it_display_summmat_colmax ; val = 7 pos = 28 ; key = it_display_summmat_rowmax ; val = 7 pos = 29 ; key = st_img_name_main ; val = _default pos = 30 ; key = st_img_path ; val = C:/Users/fan/CodeDynaAsset//m_ipwkbzr//solve/img/ pos = 31 ; key = st_img_prefix ; val = pos = 32 ; key = st_img_suffix ; val = _p4.png pos = 33 ; key = st_mat_name_main ; val = _default pos = 34 ; key = st_mat_path ; val = C:/Users/fan/CodeDynaAsset//m_ipwkbzr//solve/mat/ pos = 35 ; key = st_mat_prefix ; val = pos = 36 ; key = st_mat_suffix ; val = _p4 pos = 37 ; key = st_mat_test_path ; val = C:/Users/fan/CodeDynaAsset//m_ipwkbzr//test/ff_ipwkbz_ds_vecsv/mat/ pos = 38 ; key = st_matimg_path_root ; val = C:/Users/fan/CodeDynaAsset//m_ipwkbzr/ pos = 39 ; key = st_profile_name_main ; val = _default pos = 40 ; key = st_profile_path ; val = C:/Users/fan/CodeDynaAsset//m_ipwkbzr//solve/profile/ pos = 41 ; key = st_profile_prefix ; val = pos = 42 ; key = st_profile_suffix ; val = _p4 pos = 43 ; key = st_title_prefix ; val = ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Scalars in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx value __ ___ _____ bl_display 1 1 0 bl_display_defparam 2 2 1 bl_display_dist 3 3 0 bl_display_evf 4 4 0 bl_display_final 5 5 1 bl_display_final_dist 6 6 0 bl_display_final_dist_detail 7 7 0 bl_display_funcgrids 8 8 0 bl_graph 9 9 1 bl_graph_coh_t_coh 10 10 1 bl_graph_evf 11 11 0 bl_graph_funcgrids 12 12 0 bl_graph_funcgrids_detail 13 13 0 bl_graph_onebyones 14 14 1 bl_graph_pol_lvl 15 15 1 bl_graph_pol_pct 16 16 1 bl_graph_val 17 17 1 bl_img_save 18 18 0 bl_mat 19 19 0 bl_post 20 20 1 bl_profile 21 21 0 bl_profile_dist 22 22 0 bl_time 23 23 0 it_display_every 24 24 5 it_display_final_colmax 25 25 12 it_display_final_rowmax 26 26 100 it_display_summmat_colmax 27 27 7 it_display_summmat_rowmax 28 28 7 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Strings in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx __ ___ st_img_name_main 1 29 st_img_path 2 30 st_img_prefix 3 31 st_img_suffix 4 32 st_mat_name_main 5 33 st_mat_path 6 34 st_mat_prefix 7 35 st_mat_suffix 8 36 st_mat_test_path 9 37 st_matimg_path_root 10 38 st_profile_name_main 11 39 st_profile_path 12 40 st_profile_prefix 13 41 st_profile_suffix 14 42 st_title_prefix 15 43
Parse Parameters
params_group = values(param_map, {'it_z_n', 'fl_z_mu', 'fl_z_rho', 'fl_z_sig'}); [it_z_n, fl_z_mu, fl_z_rho, fl_z_sig] = params_group{:}; params_group = values(param_map, {'bl_default', 'fl_b_bd', 'fl_w_min', 'fl_w_max', ... 'it_w_perc_n', 'fl_w_interp_grid_gap', 'fl_coh_interp_grid_gap'}); [bl_default, fl_b_bd, fl_w_min, fl_w_max, ... it_w_perc_n, fl_w_interp_grid_gap, fl_coh_interp_grid_gap] = params_group{:}; params_group = values(param_map, {'fl_k_min', 'fl_k_max', 'it_ak_perc_n'}); [fl_k_min, fl_k_max, it_ak_perc_n] = params_group{:}; params_group = values(param_map, {'fl_crra', 'fl_c_min', 'it_c_interp_grid_gap'}); [fl_crra, fl_c_min, it_c_interp_grid_gap] = params_group{:}; params_group = values(param_map, {'fl_Amean', 'fl_alpha', 'fl_delta'}); [fl_Amean, fl_alpha, fl_delta] = params_group{:}; params_group = values(param_map, {'fl_r_save', 'fl_r_borr', 'fl_w'}); [fl_r_save, fl_r_borr, fl_w] = params_group{:}; params_group = values(support_map, {'bl_graph_funcgrids', 'bl_graph_funcgrids_detail', 'bl_display_funcgrids'}); [bl_graph_funcgrids, bl_graph_funcgrids_detail, bl_display_funcgrids] = params_group{:}; params_group = values(support_map, {'it_display_summmat_rowmax', 'it_display_summmat_colmax'}); [it_display_summmat_rowmax, it_display_summmat_colmax] = params_group{:};
Generate Asset and Choice Grid for 2nd stage Problem
This generate triangular choice structure. Household choose total aggregate savings, and within that how much to put into risky capital and how much to put into safe assets, in percentages. See ffs_ipwkbz_set_default_param for details.
if (~bl_default) fl_w_min_use = max(fl_w_min, -(fl_w)/fl_r_borr); fl_b_bd = fl_w_min_use; else fl_w_min_use = fl_w_min; end % percentage grid for 1st stage choice problem, level grid for 2nd stage % solving optimal k given w and z. ar_w_perc = linspace(0, 1, it_w_perc_n); it_w_interp_n = (fl_w_max-fl_w_min_use)/(fl_w_interp_grid_gap); ar_w_level_full = fft_array_add_zero(linspace(fl_w_min_use, fl_w_max, it_w_interp_n), true); ar_w_level = ar_w_level_full; it_w_interp_n = length(ar_w_level_full); % max k given w, need to consider the possibility of borrowing. ar_k_max = ar_w_level_full - fl_b_bd; % k percentage choice grid ar_ak_perc = linspace(0, 1, it_ak_perc_n); % 2nd stage percentage choice matrixes % (ar_k_max') is it_w_interp_n by 1, and (ar_ak_perc) is 1 by it_ak_perc_n % mt_k is a it_w_interp_n by it_ak_perc_n matrix of choice points of k' % conditional on w, each column is a different w, each row for each col a % different k' value. mt_k = (ar_k_max'*ar_ak_perc)'; mt_a = (ar_w_level_full - mt_k); % can not have choice that are beyond feasible bound given the percentage % structure here. mt_bl_constrained = (mt_a < fl_b_bd); if (sum(mt_bl_constrained) > 0 ) error('at %s second stage choice points, percentage choice exceed bounds, can not happen',... num2str(sum(mt_bl_constrained))); end ar_a_meshk_full = mt_a(:); ar_k_mesha_full = mt_k(:); ar_a_meshk = ar_a_meshk_full; ar_k_mesha = ar_k_mesha_full;
Get Shock Grids
[~, mt_z_trans, ar_stationary, ar_z] = ffto_gen_tauchen_jhl(fl_z_mu,fl_z_rho,fl_z_sig,it_z_n);
Get Equations
[f_util_log, f_util_crra, f_util_standin, f_prod, f_inc, f_coh, f_cons] = ...
ffs_ipwkbz_set_functions(fl_crra, fl_c_min, fl_b_bd, fl_Amean, fl_alpha, fl_delta, fl_r_save, fl_r_borr, fl_w);
Generate Cash-on-Hand/State Matrix
The endogenous state variable is cash-on-hand, it has it_z_n*it_a_n number of points, covering all reachable points when ar_a is the choice vector and ar_z is the shock vector. requires inputs from get Asset and choice grids, get shock grids, and get equations above.
mt_coh_wkb_full = f_coh(ar_z, ar_a_meshk_full, ar_k_mesha_full); if (bl_display_funcgrids) % Generate Aggregate Variables ar_aplusk_mesh = ar_a_meshk_full + ar_k_mesha_full; % Genereate Table tab_ak_choices = array2table([ar_aplusk_mesh, ar_k_mesha_full, ar_a_meshk_full]); cl_col_names = {'ar_aplusk_mesh', 'ar_k_mesha_full', 'ar_a_meshk_full'}; tab_ak_choices.Properties.VariableNames = cl_col_names; % Label Table Variables tab_ak_choices.Properties.VariableDescriptions{'ar_aplusk_mesh'} = ... '*ar_aplusk_mesha*: ar_aplusk_mesha = ar_a_meshk_full + ar_k_mesha_full;'; tab_ak_choices.Properties.VariableDescriptions{'ar_a_meshk_full'} = ... '*ar_a_meshk_full*:'; tab_ak_choices.Properties.VariableDescriptions{'ar_k_mesha_full'} = ... '*ar_k_mesha_full*:'; cl_var_desc = tab_ak_choices.Properties.VariableDescriptions; for it_var_name = 1:length(cl_var_desc) disp(cl_var_desc{it_var_name}); end disp('----------------------------------------'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp('tab_ak_choices'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); it_rows_toshow = length(ar_w_level)*2; disp(size(tab_ak_choices)); disp(head(array2table(tab_ak_choices), it_rows_toshow)); disp(tail(array2table(tab_ak_choices), it_rows_toshow)); disp('----------------------------------------'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp('mt_coh_wkb_full'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp(size(mt_coh_wkb_full)); disp(head(array2table(mt_coh_wkb_full), it_rows_toshow)); disp(tail(array2table(mt_coh_wkb_full), it_rows_toshow)); end
*ar_aplusk_mesha*: ar_aplusk_mesha = ar_a_meshk_full + ar_k_mesha_full; *ar_k_mesha_full*: *ar_a_meshk_full*: ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx tab_ak_choices xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx 1215 3 tab_ak_choices1 tab_ak_choices2 tab_ak_choices3 ar_aplusk_mesh ar_k_mesha_full ar_a_meshk_full _______________ _______________ _______________ -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -2.4832 0 -2.4832 -2.4832 0.0497 -2.5329 -2.4832 0.0994 -2.5826 -2.4832 0.1491 -2.6323 -2.4832 0.1988 -2.682 -2.4832 0.2485 -2.7317 -2.4832 0.2982 -2.7814 -2.4832 0.3479 -2.8311 -2.4832 0.3976 -2.8808 tab_ak_choices1 tab_ak_choices2 tab_ak_choices3 ar_aplusk_mesh ar_k_mesha_full ar_a_meshk_full _______________ _______________ _______________ 47.813 42.941 4.8724 47.813 44.134 3.6796 47.813 45.326 2.4868 47.813 46.519 1.294 47.813 47.712 0.10122 47.813 48.905 -1.0916 47.813 50.098 -2.2844 47.813 51.29 -3.4772 47.813 52.483 -4.67 50 0 50 50 1.2425 48.758 50 2.485 47.515 50 3.7275 46.273 50 4.97 45.03 50 6.2125 43.788 50 7.455 42.545 50 8.6975 41.303 50 9.94 40.06 50 11.182 38.818 50 12.425 37.575 50 13.667 36.333 50 14.91 35.09 50 16.152 33.848 50 17.395 32.605 50 18.637 31.363 50 19.88 30.12 50 21.122 28.878 50 22.365 27.635 50 23.607 26.393 50 24.85 25.15 50 26.092 23.908 50 27.335 22.665 50 28.577 21.423 50 29.82 20.18 50 31.062 18.938 50 32.305 17.695 50 33.547 16.453 50 34.79 15.21 50 36.032 13.968 50 37.275 12.725 50 38.517 11.483 50 39.76 10.24 50 41.002 8.9975 50 42.245 7.755 50 43.487 6.5125 50 44.73 5.27 50 45.972 4.0275 50 47.215 2.785 50 48.457 1.5425 50 49.7 0.30002 50 50.942 -0.94248 50 52.185 -2.185 50 53.427 -3.4275 50 54.67 -4.67 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx mt_coh_wkb_full xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx 1215 15 mt_coh_wkb_full1 mt_coh_wkb_full2 mt_coh_wkb_full3 mt_coh_wkb_full4 mt_coh_wkb_full5 mt_coh_wkb_full6 mt_coh_wkb_full7 mt_coh_wkb_full8 mt_coh_wkb_full9 mt_coh_wkb_full10 mt_coh_wkb_full11 mt_coh_wkb_full12 mt_coh_wkb_full13 mt_coh_wkb_full14 mt_coh_wkb_full15 ________________ ________________ ________________ ________________ ________________ ________________ ________________ ________________ ________________ _________________ _________________ _________________ _________________ _________________ _________________ -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -2.2754 -2.2754 -2.2754 -2.2754 -2.2754 -2.2754 -2.2754 -2.2754 -2.2754 -2.2754 -2.2754 -2.2754 -2.2754 -2.2754 -2.2754 -2.1662 -2.1481 -2.1272 -2.1031 -2.0753 -2.0433 -2.0063 -1.9636 -1.9144 -1.8576 -1.7921 -1.7166 -1.6294 -1.5289 -1.4129 -2.1415 -2.1183 -2.0915 -2.0605 -2.0249 -1.9837 -1.9363 -1.8815 -1.8183 -1.7455 -1.6614 -1.5644 -1.4526 -1.3236 -1.1747 -2.1264 -2.0995 -2.0685 -2.0327 -1.9915 -1.9438 -1.8889 -1.8255 -1.7525 -1.6681 -1.5709 -1.4587 -1.3292 -1.1799 -1.0077 -2.116 -2.0862 -2.0518 -2.0121 -1.9663 -1.9135 -1.8526 -1.7823 -1.7012 -1.6077 -1.4998 -1.3754 -1.2318 -1.0662 -0.8752 -2.1085 -2.0761 -2.0389 -1.9959 -1.9462 -1.889 -1.823 -1.7468 -1.659 -1.5577 -1.4407 -1.3059 -1.1503 -0.97087 -0.76386 -2.1029 -2.0684 -2.0286 -1.9826 -1.9297 -1.8686 -1.7981 -1.7167 -1.6229 -1.5147 -1.3899 -1.2459 -1.0798 -0.88812 -0.66706 -2.0988 -2.0623 -2.0202 -1.9717 -1.9157 -1.8511 -1.7765 -1.6906 -1.5914 -1.477 -1.3451 -1.1928 -1.0172 -0.81468 -0.58101 -2.0958 -2.0575 -2.0133 -1.9624 -1.9036 -1.8359 -1.7577 -1.6675 -1.5634 -1.4434 -1.3049 -1.1452 -0.96098 -0.74844 -0.50326 mt_coh_wkb_full1 mt_coh_wkb_full2 mt_coh_wkb_full3 mt_coh_wkb_full4 mt_coh_wkb_full5 mt_coh_wkb_full6 mt_coh_wkb_full7 mt_coh_wkb_full8 mt_coh_wkb_full9 mt_coh_wkb_full10 mt_coh_wkb_full11 mt_coh_wkb_full12 mt_coh_wkb_full13 mt_coh_wkb_full14 mt_coh_wkb_full15 ________________ ________________ ________________ ________________ ________________ ________________ ________________ ________________ ________________ _________________ _________________ _________________ _________________ _________________ _________________ 46.288 46.495 46.733 47.008 47.325 47.691 48.112 48.599 49.16 49.808 50.555 51.417 52.411 53.558 54.881 46.176 46.385 46.626 46.903 47.223 47.593 48.019 48.51 49.077 49.731 50.485 51.356 52.36 53.518 54.854 46.064 46.275 46.518 46.798 47.121 47.494 47.924 48.42 48.993 49.653 50.415 51.294 52.307 53.477 54.825 45.952 46.164 46.41 46.692 47.019 47.395 47.829 48.33 48.908 49.575 50.343 51.23 52.254 53.434 54.795 45.839 46.054 46.301 46.587 46.916 47.296 47.734 48.239 48.823 49.495 50.271 51.166 52.199 53.39 54.764 45.65 45.867 46.116 46.404 46.736 47.12 47.562 48.072 48.66 49.339 50.121 51.025 52.066 53.268 54.654 45.454 45.672 45.924 46.214 46.549 46.936 47.382 47.896 48.49 49.174 49.964 50.875 51.926 53.138 54.536 45.257 45.477 45.731 46.024 46.362 46.752 47.202 47.72 48.319 49.009 49.806 50.724 51.784 53.007 54.417 45.06 45.282 45.538 45.834 46.174 46.568 47.021 47.544 48.148 48.844 49.647 50.573 51.642 52.874 54.296 51.694 51.694 51.694 51.694 51.694 51.694 51.694 51.694 51.694 51.694 51.694 51.694 51.694 51.694 51.694 51.939 51.997 52.063 52.14 52.228 52.331 52.448 52.584 52.741 52.922 53.131 53.371 53.649 53.969 54.339 51.915 51.989 52.074 52.173 52.286 52.418 52.569 52.743 52.945 53.177 53.444 53.753 54.11 54.521 54.995 51.86 51.946 52.045 52.159 52.29 52.442 52.617 52.819 53.052 53.32 53.63 53.988 54.4 54.876 55.425 51.791 51.886 51.995 52.122 52.268 52.436 52.63 52.854 53.112 53.41 53.754 54.15 54.608 55.135 55.744 51.712 51.815 51.934 52.071 52.229 52.411 52.621 52.864 53.144 53.467 53.839 54.269 54.765 55.336 55.996 51.627 51.737 51.864 52.01 52.179 52.373 52.598 52.857 53.156 53.501 53.899 54.357 54.887 55.497 56.202 51.537 51.654 51.788 51.942 52.121 52.326 52.564 52.838 53.154 53.518 53.939 54.424 54.983 55.628 56.373 51.444 51.566 51.707 51.869 52.056 52.272 52.521 52.809 53.14 53.523 53.964 54.473 55.06 55.737 56.518 51.348 51.475 51.622 51.791 51.987 52.212 52.472 52.772 53.118 53.517 53.977 54.508 55.12 55.827 56.642 51.25 51.382 51.534 51.71 51.913 52.147 52.417 52.728 53.088 53.502 53.98 54.531 55.168 55.901 56.748 51.149 51.286 51.444 51.626 51.836 52.078 52.357 52.68 53.051 53.48 53.975 54.546 55.204 55.963 56.839 51.047 51.188 51.351 51.539 51.755 52.005 52.294 52.626 53.01 53.452 53.963 54.551 55.231 56.014 56.918 50.943 51.089 51.256 51.45 51.672 51.93 52.226 52.569 52.963 53.419 53.944 54.55 55.249 56.056 56.986 50.839 50.988 51.16 51.358 51.587 51.851 52.156 52.508 52.913 53.381 53.921 54.543 55.261 56.089 57.045 50.733 50.885 51.062 51.265 51.5 51.771 52.083 52.444 52.859 53.339 53.892 54.53 55.266 56.115 57.095 50.625 50.782 50.963 51.171 51.411 51.688 52.008 52.377 52.802 53.293 53.859 54.512 55.266 56.135 57.137 50.518 50.677 50.862 51.075 51.32 51.604 51.931 52.307 52.742 53.244 53.823 54.49 55.26 56.148 57.173 50.409 50.572 50.76 50.978 51.228 51.518 51.851 52.236 52.68 53.192 53.783 54.464 55.25 56.157 57.203 50.299 50.466 50.658 50.879 51.135 51.43 51.77 52.162 52.615 53.137 53.739 54.434 55.236 56.16 57.227 50.189 50.358 50.554 50.78 51.04 51.341 51.687 52.087 52.548 53.08 53.693 54.401 55.217 56.159 57.245 50.078 50.251 50.45 50.679 50.944 51.25 51.603 52.009 52.479 53.02 53.644 54.365 55.195 56.154 57.26 49.967 50.142 50.345 50.578 50.848 51.158 51.517 51.931 52.408 52.958 53.593 54.326 55.17 56.145 57.269 49.854 50.033 50.239 50.476 50.75 51.066 51.43 51.85 52.335 52.894 53.54 54.284 55.142 56.133 57.275 49.742 49.923 50.132 50.373 50.651 50.972 51.342 51.769 52.261 52.829 53.484 54.24 55.111 56.117 57.277 49.629 49.813 50.025 50.269 50.551 50.877 51.252 51.686 52.185 52.761 53.426 54.193 55.078 56.099 57.276 49.516 49.702 49.917 50.165 50.451 50.781 51.162 51.601 52.108 52.693 53.367 54.145 55.042 56.077 57.271 49.402 49.591 49.809 50.06 50.35 50.685 51.071 51.516 52.03 52.622 53.306 54.094 55.004 56.053 57.263 49.287 49.479 49.7 49.954 50.248 50.587 50.978 51.43 51.95 52.55 53.243 54.042 54.963 56.026 57.253 49.173 49.367 49.59 49.848 50.146 50.489 50.885 51.342 51.869 52.477 53.179 53.988 54.921 55.997 57.239 49.058 49.254 49.481 49.742 50.043 50.391 50.791 51.254 51.787 52.403 53.113 53.932 54.876 55.966 57.223 48.943 49.141 49.37 49.635 49.939 50.291 50.697 51.165 51.705 52.327 53.046 53.874 54.83 55.933 57.205 48.827 49.028 49.26 49.527 49.835 50.191 50.601 51.075 51.621 52.251 52.977 53.815 54.782 55.898 57.184 48.711 48.914 49.148 49.419 49.731 50.09 50.505 50.984 51.536 52.173 52.908 53.755 54.733 55.861 57.162 48.595 48.8 49.037 49.31 49.625 49.989 50.408 50.892 51.45 52.094 52.837 53.693 54.682 55.822 57.137 48.478 48.686 48.925 49.201 49.52 49.887 50.311 50.8 51.364 52.014 52.765 53.63 54.629 55.781 57.11 48.362 48.571 48.813 49.092 49.414 49.785 50.213 50.707 51.277 51.934 52.692 53.566 54.575 55.739 57.081 48.245 48.456 48.701 48.982 49.307 49.682 50.114 50.613 51.188 51.852 52.618 53.501 54.52 55.695 57.051 48.128 48.341 48.588 48.872 49.2 49.579 50.015 50.519 51.1 51.77 52.543 53.435 54.463 55.65 57.019 48.01 48.226 48.475 48.762 49.093 49.475 49.916 50.424 51.01 51.687 52.467 53.367 54.405 55.603 56.985 47.893 48.11 48.361 48.651 48.985 49.371 49.815 50.328 50.92 51.603 52.39 53.298 54.346 55.555 56.949 47.709 47.929 48.182 48.474 48.811 49.2 49.649 50.166 50.763 51.452 52.246 53.163 54.22 55.44 56.846 47.504 47.725 47.981 48.276 48.616 49.008 49.461 49.983 50.585 51.28 52.081 53.005 54.072 55.302 56.721 47.299 47.522 47.78 48.077 48.42 48.816 49.272 49.799 50.406 51.107 51.915 52.847 53.923 55.163 56.594 47.093 47.319 47.579 47.878 48.224 48.623 49.083 49.614 50.227 50.933 51.748 52.688 53.772 55.023 56.466
Check if COH is within Borrowing Bounds
some coh levels are below borrowing bound, can not borrow enough to pay debt
mt_bl_coh_wkb_invalid = (mt_coh_wkb_full < fl_b_bd); % (k,a) invalid if coh(k,a,z) < bd for any z ar_bl_wkb_invalid = max(mt_bl_coh_wkb_invalid,[], 2); mt_bl_wkb_invalid = reshape(ar_bl_wkb_invalid, size(mt_a)); % find the first w_level choice where some k(w) percent choices are valid? ar_bl_w_level_invalid = min(mt_bl_wkb_invalid, [], 1); % w choices can not be lower than fl_w_level_min_valid. If w choices are % lower, given the current borrowing interest rate as well as the minimum % income level in the future, and the maximum borrowing level available % next period, and given the shock distribution, there exists some state in % the future when the household when making this choice will be unable to % borrow sufficiently to maintain positive consumption. fl_w_level_min_valid = min(ar_w_level_full(~ar_bl_w_level_invalid));
Update Valid 2nd stage choice matrix
ar_w_level = linspace(fl_w_level_min_valid, fl_w_max, it_w_interp_n); ar_k_max = ar_w_level - fl_b_bd; mt_k = (ar_k_max'*ar_ak_perc)'; mt_a = (ar_w_level - mt_k); ar_a_meshk = mt_a(:); ar_k_mesha = mt_k(:);
Select only Valid (k(w), a) choices
% mt_coh_wkb = mt_coh_wkb_full(~ar_bl_wkb_invalid, :);
mt_coh_wkb = mt_coh_wkb_full;
mt_z_mesh_coh_wkb = repmat(ar_z, [size(mt_coh_wkb,1),1]);
Generate 1st Stage States: Interpolation Cash-on-hand Interpolation Grid
For the iwkz problems, we solve the problem along a grid of cash-on-hand values, the interpolate to find v(k',b',z) at (k',b') choices. Crucially, we have to coh matrxies
fl_max_mt_coh = max(max(mt_coh_wkb)); % This is savings only condition % fl_min_mt_coh = min(min(mt_coh_wkb)); % This could be condition if no defaults are allowed % fl_min_mt_coh = fl_w_level_min_valid; % This is borrowing with default or not condition fl_min_mt_coh = fl_b_bd; it_coh_interp_n = (fl_max_mt_coh-fl_min_mt_coh)/(fl_coh_interp_grid_gap); ar_interp_coh_grid = fft_array_add_zero(linspace(fl_min_mt_coh, fl_max_mt_coh, it_coh_interp_n), true); [mt_interp_coh_grid_mesh_z, mt_z_mesh_coh_interp_grid] = ndgrid(ar_interp_coh_grid, ar_z); mt_interp_coh_grid_mesh_w_perc = repmat(ar_interp_coh_grid, [it_w_perc_n, 1]);
Generate 1st Stage Choices: Interpolation Cash-on-hand Interpolation Grid
previously, our ar_w was the first stage choice grid, the grid was the same for all coh levels. Now, for each coh level, there is a different ar_w. ar_interp_coh_grid is (1 by ar_interp_coh_grid) and ar_w_perc is ( 1 by it_w_perc_n). Conditional on z, each choice matrix is (it_w_perc_n by ar_interp_coh_grid). Here we are pre-computing the choice matrix. This could be a large matrix if the choice grid is large. This is the matrix of aggregate savings choices
if (fl_min_mt_coh < 0) % borrowing bound is below zero mt_w_by_interp_coh_interp_grid = ((ar_interp_coh_grid-fl_min_mt_coh)'*ar_w_perc)' + fl_min_mt_coh; else % savings only mt_w_by_interp_coh_interp_grid = ((ar_interp_coh_grid)'*ar_w_perc)'; end
Generate Interpolation Consumption Grid
We also interpolate over consumption to speed the program up. We only solve for u(c) at this grid for the iwkz problmes, and then interpolate other c values.
fl_c_max = max(max(mt_coh_wkb_full)) - fl_b_bd; it_interp_c_grid_n = (fl_c_max-fl_c_min)/(it_c_interp_grid_gap); ar_interp_c_grid = linspace(fl_c_min, fl_c_max, it_interp_c_grid_n);
Initialize armt_map to store, state, choice, shock matrixes
armt_map = containers.Map('KeyType','char', 'ValueType','any'); armtdesc_map = containers.Map('KeyType','char', 'ValueType','any');
Store armt_map (1): 2nd Stage Problem Arrays and Matrixes
armt_map('ar_ak_perc') = ar_ak_perc; armt_map('mt_k') = mt_k; armt_map('ar_a_meshk') = ar_a_meshk; armt_map('ar_k_mesha') = ar_k_mesha; armt_map('it_ameshk_n') = length(ar_a_meshk); armt_map('mt_coh_wkb') = mt_coh_wkb_full; armt_map('mt_z_mesh_coh_wkb') = mt_z_mesh_coh_wkb;
Store armt_map (2): First Stage Aggregate Savings
w = k' + b', w is aggregate Savings%
- ar_w_perc 1st stage, percentage w choice given coh, at each coh level the number of choice points is the same for this problem with percentage grid points.
- ar_w_level 2nd stage, level of w over which we solve the optimal percentage k' choices. Need to generate interpolant based on this so that we know optimal k* given ar_w_perc(coh) in the 1st stage
- mt_w_by_interp_coh_interp_grid 1st stage, generate w(coh, percent), meaning the level of w given coh and the percentage grid of ar_w_perc. Mesh this with the coh grid, Rows here correspond to percentage of w choices, columns correspond to cash-on-hand. The columns of cash-on-hand is determined by ar_interp_coh_grid, because we solve the 1st stage problem at that coh grid.
armt_map('ar_w_perc') = ar_w_perc; armt_map('ar_w_level') = ar_w_level; armt_map('mt_w_by_interp_coh_interp_grid') = mt_w_by_interp_coh_interp_grid; armt_map('mt_interp_coh_grid_mesh_w_perc') = mt_interp_coh_grid_mesh_w_perc;
Store armt_map (3): First Stage Consumption and Cash-on-Hand Grids
armt_map('ar_interp_c_grid') = ar_interp_c_grid; armt_map('ar_interp_coh_grid') = ar_interp_coh_grid; armt_map('mt_interp_coh_grid_mesh_z') = mt_interp_coh_grid_mesh_z; armt_map('mt_z_mesh_coh_interp_grid') = mt_z_mesh_coh_interp_grid;
Store armt_map (4): Shock Grids
armt_map('mt_z_trans') = mt_z_trans; armt_map('ar_stationary') = ar_stationary; armt_map('ar_z') = ar_z;
Store Function Map
func_map = containers.Map('KeyType','char', 'ValueType','any'); func_map('f_util_log') = f_util_log; func_map('f_util_crra') = f_util_crra; func_map('f_util_standin') = f_util_standin; func_map('f_prod') = f_prod; func_map('f_inc') = f_inc; func_map('f_coh') = f_coh; func_map('f_cons') = f_cons;
Graph
if (bl_graph_funcgrids)
Graph 1: a and k choice grid graphs
compare the figure here to the same figure in ffs_akz_get_funcgrid. there the grid points are on an even grid, half of the grid points have NA. for the grid here, the grid points get denser as we get closer to low w = k'+b' levels. This is what is different visually about percentage points based choice grid for the 2nd stage problem.
figure('PaperPosition', [0 0 7 4]); hold on; chart = plot(mt_a, mt_k, 'blue'); clr = jet(numel(chart)); for m = 1:numel(chart) set(chart(m),'Color',clr(m,:)) end % if (length(ar_w_level_full) <= 100) scatter(ar_a_meshk, ar_k_mesha, 3, 'filled', ... 'MarkerEdgeColor', 'b', 'MarkerFaceColor', 'b'); % end if (length(ar_w_level_full) <= 100) gf_invalid_scatter = scatter(ar_a_meshk_full(ar_bl_wkb_invalid),... ar_k_mesha_full(ar_bl_wkb_invalid),... 20, 'O', 'MarkerEdgeColor', 'black', 'MarkerFaceColor', 'black'); end xline(0); yline(0); title('Risky K Percentage Grids Given w=k+a (2nd Stage)') ylabel('Capital Choice (mt\_k)') xlabel({'Borrowing (<0) or Saving (>0) (mt\_a)'... 'Each Diagonal Line a Different w=k+a level'... 'Percentage for Risky K along Each Diagonal'}) legend2plot = fliplr([1 round(numel(chart)/3) round((2*numel(chart))/4) numel(chart)]); legendCell = cellstr(num2str(ar_w_level', 'k+a=%3.2f')); if (length(ar_w_level_full) <= 100) chart(length(chart)+1) = gf_invalid_scatter; legendCell{length(legendCell) + 1} = 'Invalid: COH(a,b,z)<bar(b) some z'; legend(chart([legend2plot length(legendCell)]), legendCell([legend2plot length(legendCell)]), 'Location', 'northeast'); else legend(chart([legend2plot]), legendCell([legend2plot]), 'Location', 'northeast'); end grid on;
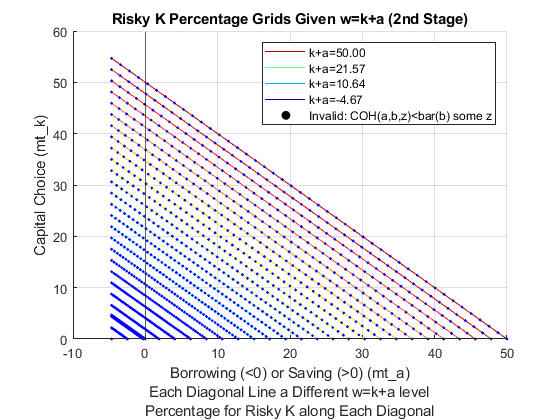
Graph 2: coh by shock
compare the figure here to the same figure in ffs_akz_get_funcgrid. there the grid points are on an even grid. Visually, one could see that the blue/red line segments here are always the same length, but in the ffs_akz_get_funcgrid figure, they are increasingly longer as we move towards the right. They are even because the number of percentage points available is constant regardless of w = k' + b' levels. But previously, the number of grid points available is increasing as w increases since choice grid is based on levels.
figure('PaperPosition', [0 0 7 4]); chart = plot(0:1:(size(mt_coh_wkb_full,1)-1), mt_coh_wkb_full); clr = jet(numel(chart)); for m = 1:numel(chart) set(chart(m),'Color',clr(m,:)) end % zero lines xline(0); yline(0); % invalid points separating lines yline_borrbound = yline(fl_b_bd); yline_borrbound.HandleVisibility = 'on'; yline_borrbound.LineStyle = '--'; yline_borrbound.Color = 'blue'; yline_borrbound.LineWidth = 2.5; title('Cash-on-Hand given w(k+b),k,z'); ylabel('Cash-on-Hand (mt\_coh\_wkb\_full)'); xlabel({'Index of Cash-on-Hand Discrete Point (0:1:(size(mt\_coh\_wkb\_full,1)-1))'... 'Each Segment is a w=k+b; within segment increasing k'... 'For each w and z, coh maximizing k is different'}); legend2plot = fliplr([1 round(numel(chart)/3) round((2*numel(chart))/4) numel(chart)]); legendCell = cellstr(num2str(ar_z', 'shock=%3.2f')); legendCell{length(legendCell) + 1} = 'borrow-constraint'; chart(length(chart)+1) = yline_borrbound; legend(chart([legend2plot length(legendCell)]), legendCell([legend2plot length(legendCell)]), 'Location', 'southeast'); grid on;
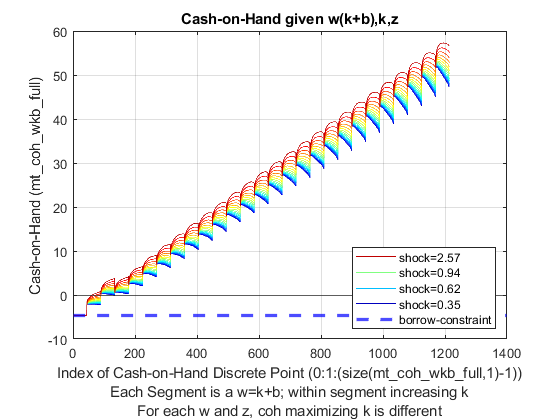
Graph 3: 1st State Aggregate Savings Choices by COH interpolation grids
figure('PaperPosition', [0 0 7 4]); hold on; chart = plot(ar_interp_coh_grid, mt_w_by_interp_coh_interp_grid'); clr = jet(numel(chart)); for m = 1:numel(chart) set(chart(m),'Color',clr(m,:)) end if (length(ar_interp_coh_grid) <= 100) [~, mt_interp_coh_grid_mesh_w_perc] = ndgrid(ar_w_perc, ar_interp_coh_grid); scatter(mt_interp_coh_grid_mesh_w_perc(:), mt_w_by_interp_coh_interp_grid(:), 3, 'filled', ... 'MarkerEdgeColor', 'b', 'MarkerFaceColor', 'b'); end % invalid points separating lines yline_borrbound = yline(fl_w_level_min_valid); yline_borrbound.HandleVisibility = 'on'; yline_borrbound.LineStyle = '--'; yline_borrbound.Color = 'red'; yline_borrbound.LineWidth = 2.5; xline0 = xline(0); xline0.HandleVisibility = 'off'; yline0 = yline(0); yline0.HandleVisibility = 'off'; title({'Aggregate Savings Percentage Grids (1st Stage)' ... 'y=mt\_w\_by\_interp\_coh\_interp\_grid, and, y=ar\_interp\_coh\_grid'}); ylabel('1st Stage Aggregate Savings Choices'); xlabel({'Cash-on-Hand Levels (Interpolation Points)'... 'w(coh)>min-agg-save, coh(k(w),w-k)>=bar(b)'}); legend2plot = fliplr([1 round(numel(chart)/3) round((2*numel(chart))/4) numel(chart)]); legendCell = cellstr(num2str(ar_w_perc', 'ar w perc=%3.2f')); legendCell{length(legendCell) + 1} = 'min-agg-save'; chart(length(chart)+1) = yline_borrbound; legend(chart([legend2plot length(legendCell)]), legendCell([legend2plot length(legendCell)]), 'Location', 'northwest'); grid on;
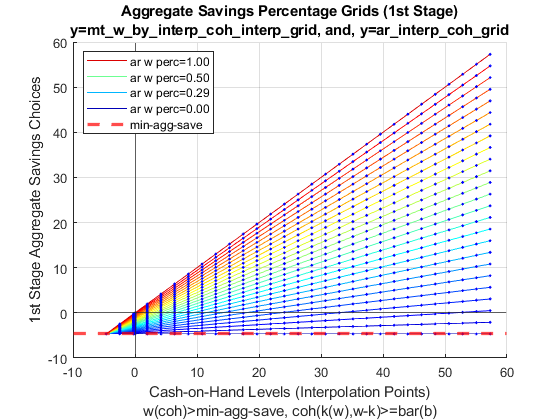
end
Graph Details, Generally do Not Run
if (bl_graph_funcgrids_detail)
Graph 1: 2nd stage coh reached by k' b' choices by index
figure('PaperPosition', [0 0 7 4]); ar_coh_kpzgrid_unique = unique(sort(mt_coh_wkb_full(:))); scatter(1:length(ar_coh_kpzgrid_unique), ar_coh_kpzgrid_unique); xline(0); yline(0); title('Cash-on-Hand given w(k+b),k,z'); ylabel('Cash-on-Hand (y=ar\_coh\_kpzgrid\_unique)'); xlabel({'Index of Cash-on-Hand Discrete Point' 'x = 1:length(ar\_coh\_kpzgrid\_unique)'}); grid on;
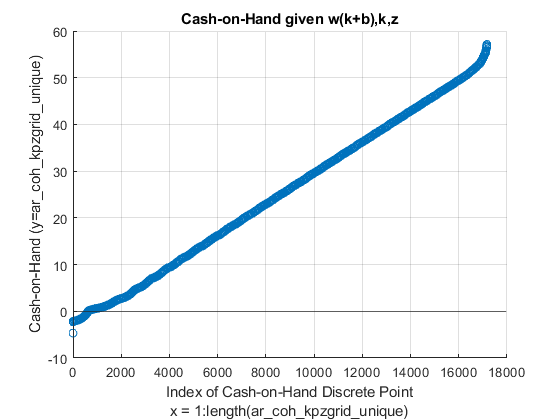
Graph 2: 2nd stage coh reached by k' b' choices by coh
figure('PaperPosition', [0 0 7 4]); ar_coh_kpzgrid_unique = unique(sort(mt_coh_wkb_full(:))); scatter(ar_coh_kpzgrid_unique, ar_coh_kpzgrid_unique, '.'); xline(0); yline(0); title('Cash-on-Hand given w(k+b),k,z; See Clearly Sparsity Density of Grid across Z'); ylabel('Cash-on-Hand (y = ar\_coh\_kpzgrid\_unique)'); xlabel({'Cash-on-Hand' 'x = ar\_coh\_kpzgrid\_unique'}); grid on;
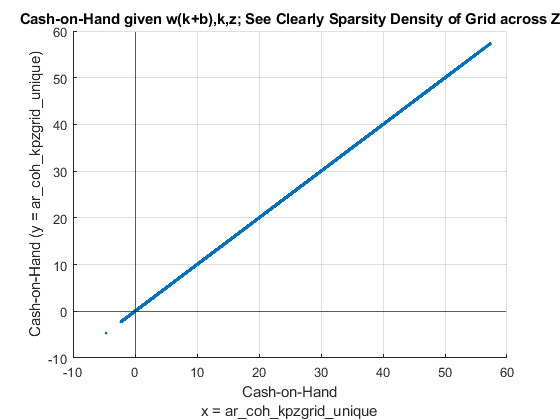
end
Display
if (bl_display_funcgrids) disp('----------------------------------------'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp('ar_z'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp(size(ar_z)); disp(ar_z); disp('----------------------------------------'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp('ar_w_level_full'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp(size(ar_w_level_full)); disp(ar_w_level_full); disp('----------------------------------------'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp('mt_w_by_interp_coh_interp_grid'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp(size(mt_w_by_interp_coh_interp_grid)); disp(head(array2table(mt_w_by_interp_coh_interp_grid), 10)); disp(tail(array2table(mt_w_by_interp_coh_interp_grid), 10)); disp('----------------------------------------'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp('mt_z_trans'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp(size(mt_z_trans)); disp(head(array2table(mt_z_trans), 10)); disp(tail(array2table(mt_z_trans), 10)); disp('----------------------------------------'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp('ar_interp_coh_grid'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); summary(array2table(ar_interp_coh_grid')); disp('----------------------------------------'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp('ar_interp_c_grid'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); summary(array2table(ar_interp_c_grid')); disp('----------------------------------------'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp('mt_interp_coh_grid_mesh_z'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp(size(mt_interp_coh_grid_mesh_z)); disp(head(array2table(mt_interp_coh_grid_mesh_z), 10)); disp(tail(array2table(mt_interp_coh_grid_mesh_z), 10)); disp('----------------------------------------'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp('mt_a'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp(size(mt_a)); disp(head(array2table(mt_a), 10)); disp(tail(array2table(mt_a), 10)); disp('----------------------------------------'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp('ar_a_meshk'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); summary(array2table(ar_a_meshk)); disp('----------------------------------------'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp('mt_k'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp(size(mt_k)); disp(head(array2table(mt_k), 10)); disp(tail(array2table(mt_k), 10)); disp('----------------------------------------'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp('ar_k_mesha'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); summary(array2table(ar_k_mesha)); param_map_keys = keys(func_map); param_map_vals = values(func_map); for i = 1:length(func_map) st_display = strjoin(['pos =' num2str(i) '; key =' string(param_map_keys{i}) '; val =' func2str(param_map_vals{i})]); disp(st_display); end end
---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ar_z xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx 1 15 Columns 1 through 7 0.3474 0.4008 0.4623 0.5333 0.6152 0.7097 0.8186 Columns 8 through 14 0.9444 1.0894 1.2567 1.4496 1.6723 1.9291 2.2253 Column 15 2.5670 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ar_w_level_full xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx 1 27 Columns 1 through 7 -4.6700 -2.4832 -0.2964 0 1.8904 4.0772 6.2640 Columns 8 through 14 8.4508 10.6376 12.8244 15.0112 17.1980 19.3848 21.5716 Columns 15 through 21 23.7584 25.9452 28.1320 30.3188 32.5056 34.6924 36.8792 Columns 22 through 27 39.0660 41.2528 43.4396 45.6264 47.8132 50.0000 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx mt_w_by_interp_coh_interp_grid xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx 25 30 mt_w_by_interp_coh_interp_grid1 mt_w_by_interp_coh_interp_grid2 mt_w_by_interp_coh_interp_grid3 mt_w_by_interp_coh_interp_grid4 mt_w_by_interp_coh_interp_grid5 mt_w_by_interp_coh_interp_grid6 mt_w_by_interp_coh_interp_grid7 mt_w_by_interp_coh_interp_grid8 mt_w_by_interp_coh_interp_grid9 mt_w_by_interp_coh_interp_grid10 mt_w_by_interp_coh_interp_grid11 mt_w_by_interp_coh_interp_grid12 mt_w_by_interp_coh_interp_grid13 mt_w_by_interp_coh_interp_grid14 mt_w_by_interp_coh_interp_grid15 mt_w_by_interp_coh_interp_grid16 mt_w_by_interp_coh_interp_grid17 mt_w_by_interp_coh_interp_grid18 mt_w_by_interp_coh_interp_grid19 mt_w_by_interp_coh_interp_grid20 mt_w_by_interp_coh_interp_grid21 mt_w_by_interp_coh_interp_grid22 mt_w_by_interp_coh_interp_grid23 mt_w_by_interp_coh_interp_grid24 mt_w_by_interp_coh_interp_grid25 mt_w_by_interp_coh_interp_grid26 mt_w_by_interp_coh_interp_grid27 mt_w_by_interp_coh_interp_grid28 mt_w_by_interp_coh_interp_grid29 mt_w_by_interp_coh_interp_grid30 _______________________________ _______________________________ _______________________________ _______________________________ _______________________________ _______________________________ _______________________________ _______________________________ _______________________________ ________________________________ ________________________________ ________________________________ ________________________________ ________________________________ ________________________________ ________________________________ ________________________________ ________________________________ ________________________________ ________________________________ ________________________________ ________________________________ ________________________________ ________________________________ ________________________________ ________________________________ ________________________________ ________________________________ ________________________________ ________________________________ -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.5778 -4.4856 -4.4754 -4.3934 -4.3012 -4.2091 -4.1169 -4.0247 -3.9325 -3.8403 -3.7481 -3.656 -3.5638 -3.4716 -3.3794 -3.2872 -3.195 -3.1029 -3.0107 -2.9185 -2.8263 -2.7341 -2.6419 -2.5498 -2.4576 -2.3654 -2.2732 -2.181 -2.0888 -4.67 -4.4856 -4.3012 -4.2808 -4.1169 -3.9325 -3.7481 -3.5638 -3.3794 -3.195 -3.0107 -2.8263 -2.6419 -2.4576 -2.2732 -2.0888 -1.9045 -1.7201 -1.5357 -1.3514 -1.167 -0.98264 -0.79828 -0.61391 -0.42954 -0.24518 -0.060809 0.12356 0.30792 0.49229 -4.67 -4.3934 -4.1169 -4.0862 -3.8403 -3.5638 -3.2872 -3.0107 -2.7341 -2.4576 -2.181 -1.9045 -1.6279 -1.3514 -1.0748 -0.79828 -0.52173 -0.24518 0.031374 0.30792 0.58447 0.86102 1.1376 1.4141 1.6907 1.9672 2.2438 2.5203 2.7969 3.0734 -4.67 -4.3012 -3.9325 -3.8916 -3.5638 -3.195 -2.8263 -2.4576 -2.0888 -1.7201 -1.3514 -0.98264 -0.61391 -0.24518 0.12356 0.49229 0.86102 1.2298 1.5985 1.9672 2.336 2.7047 3.0734 3.4422 3.8109 4.1796 4.5484 4.9171 5.2858 5.6546 -4.67 -4.2091 -3.7481 -3.6971 -3.2872 -2.8263 -2.3654 -1.9045 -1.4436 -0.98264 -0.52173 -0.060809 0.40011 0.86102 1.3219 1.7829 2.2438 2.7047 3.1656 3.6265 4.0874 4.5484 5.0093 5.4702 5.9311 6.392 6.8529 7.3139 7.7748 8.2357 -4.67 -4.1169 -3.5638 -3.5025 -3.0107 -2.4576 -1.9045 -1.3514 -0.79828 -0.24518 0.30792 0.86102 1.4141 1.9672 2.5203 3.0734 3.6265 4.1796 4.7327 5.2858 5.8389 6.392 6.9451 7.4982 8.0513 8.6044 9.1575 9.7106 10.264 10.817 -4.67 -4.0247 -3.3794 -3.3079 -2.7341 -2.0888 -1.4436 -0.79828 -0.15299 0.49229 1.1376 1.7829 2.4281 3.0734 3.7187 4.364 5.0093 5.6546 6.2998 6.9451 7.5904 8.2357 8.881 9.5263 10.172 10.817 11.462 12.107 12.753 13.398 -4.67 -3.9325 -3.195 -3.1133 -2.4576 -1.7201 -0.98264 -0.24518 0.49229 1.2298 1.9672 2.7047 3.4422 4.1796 4.9171 5.6546 6.392 7.1295 7.867 8.6044 9.3419 10.079 10.817 11.554 12.292 13.029 13.767 14.504 15.242 15.979 -4.67 -3.8403 -3.0107 -2.9187 -2.181 -1.3514 -0.52173 0.30792 1.1376 1.9672 2.7969 3.6265 4.4562 5.2858 6.1155 6.9451 7.7748 8.6044 9.4341 10.264 11.093 11.923 12.753 13.582 14.412 15.242 16.071 16.901 17.731 18.56 mt_w_by_interp_coh_interp_grid1 mt_w_by_interp_coh_interp_grid2 mt_w_by_interp_coh_interp_grid3 mt_w_by_interp_coh_interp_grid4 mt_w_by_interp_coh_interp_grid5 mt_w_by_interp_coh_interp_grid6 mt_w_by_interp_coh_interp_grid7 mt_w_by_interp_coh_interp_grid8 mt_w_by_interp_coh_interp_grid9 mt_w_by_interp_coh_interp_grid10 mt_w_by_interp_coh_interp_grid11 mt_w_by_interp_coh_interp_grid12 mt_w_by_interp_coh_interp_grid13 mt_w_by_interp_coh_interp_grid14 mt_w_by_interp_coh_interp_grid15 mt_w_by_interp_coh_interp_grid16 mt_w_by_interp_coh_interp_grid17 mt_w_by_interp_coh_interp_grid18 mt_w_by_interp_coh_interp_grid19 mt_w_by_interp_coh_interp_grid20 mt_w_by_interp_coh_interp_grid21 mt_w_by_interp_coh_interp_grid22 mt_w_by_interp_coh_interp_grid23 mt_w_by_interp_coh_interp_grid24 mt_w_by_interp_coh_interp_grid25 mt_w_by_interp_coh_interp_grid26 mt_w_by_interp_coh_interp_grid27 mt_w_by_interp_coh_interp_grid28 mt_w_by_interp_coh_interp_grid29 mt_w_by_interp_coh_interp_grid30 _______________________________ _______________________________ _______________________________ _______________________________ _______________________________ _______________________________ _______________________________ _______________________________ _______________________________ ________________________________ ________________________________ ________________________________ ________________________________ ________________________________ ________________________________ ________________________________ ________________________________ ________________________________ ________________________________ ________________________________ ________________________________ ________________________________ ________________________________ ________________________________ ________________________________ ________________________________ ________________________________ ________________________________ ________________________________ ________________________________ -4.67 -3.2872 -1.9045 -1.7512 -0.52173 0.86102 2.2438 3.6265 5.0093 6.392 7.7748 9.1575 10.54 11.923 13.306 14.689 16.071 17.454 18.837 20.22 21.602 22.985 24.368 25.751 27.133 28.516 29.899 31.282 32.664 34.047 -4.67 -3.195 -1.7201 -1.5567 -0.24518 1.2298 2.7047 4.1796 5.6546 7.1295 8.6044 10.079 11.554 13.029 14.504 15.979 17.454 18.929 20.404 21.879 23.354 24.829 26.304 27.779 29.254 30.728 32.203 33.678 35.153 36.628 -4.67 -3.1029 -1.5357 -1.3621 0.031374 1.5985 3.1656 4.7327 6.2998 7.867 9.4341 11.001 12.568 14.135 15.703 17.27 18.837 20.404 21.971 23.538 25.105 26.672 28.239 29.807 31.374 32.941 34.508 36.075 37.642 39.209 -4.67 -3.0107 -1.3514 -1.1675 0.30792 1.9672 3.6265 5.2858 6.9451 8.6044 10.264 11.923 13.582 15.242 16.901 18.56 20.22 21.879 23.538 25.197 26.857 28.516 30.175 31.835 33.494 35.153 36.813 38.472 40.131 41.79 -4.67 -2.9185 -1.167 -0.97291 0.58447 2.336 4.0874 5.8389 7.5904 9.3419 11.093 12.845 14.596 16.348 18.099 19.851 21.602 23.354 25.105 26.857 28.608 30.36 32.111 33.863 35.614 37.366 39.117 40.869 42.62 44.372 -4.67 -2.8263 -0.98264 -0.77833 0.86102 2.7047 4.5484 6.392 8.2357 10.079 11.923 13.767 15.61 17.454 19.298 21.141 22.985 24.829 26.672 28.516 30.36 32.203 34.047 35.891 37.734 39.578 41.422 43.265 45.109 46.953 -4.67 -2.7341 -0.79828 -0.58375 1.1376 3.0734 5.0093 6.9451 8.881 10.817 12.753 14.689 16.624 18.56 20.496 22.432 24.368 26.304 28.239 30.175 32.111 34.047 35.983 37.919 39.855 41.79 43.726 45.662 47.598 49.534 -4.67 -2.6419 -0.61391 -0.38916 1.4141 3.4422 5.4702 7.4982 9.5263 11.554 13.582 15.61 17.638 19.666 21.694 23.723 25.751 27.779 29.807 31.835 33.863 35.891 37.919 39.947 41.975 44.003 46.031 48.059 50.087 52.115 -4.67 -2.5498 -0.42954 -0.19458 1.6907 3.8109 5.9311 8.0513 10.172 12.292 14.412 16.532 18.652 20.773 22.893 25.013 27.133 29.254 31.374 33.494 35.614 37.734 39.855 41.975 44.095 46.215 48.335 50.456 52.576 54.696 -4.67 -2.4576 -0.24518 0 1.9672 4.1796 6.392 8.6044 10.817 13.029 15.242 17.454 19.666 21.879 24.091 26.304 28.516 30.728 32.941 35.153 37.366 39.578 41.79 44.003 46.215 48.428 50.64 52.852 55.065 57.277 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx mt_z_trans xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx 15 15 mt_z_trans1 mt_z_trans2 mt_z_trans3 mt_z_trans4 mt_z_trans5 mt_z_trans6 mt_z_trans7 mt_z_trans8 mt_z_trans9 mt_z_trans10 mt_z_trans11 mt_z_trans12 mt_z_trans13 mt_z_trans14 mt_z_trans15 ___________ ___________ ___________ ___________ ___________ ___________ ___________ ___________ ___________ ____________ ____________ ____________ ____________ ____________ ____________ 0.26016 0.26831 0.25551 0.14921 0.053403 0.011702 0.0015678 0.00012823 6.3911e-06 1.9381e-07 3.5701e-09 3.9889e-11 2.7001e-13 1.1102e-15 0 0.11232 0.19622 0.2763 0.23861 0.12635 0.040998 0.0081429 0.00098855 7.3236e-05 3.3054e-06 9.0736e-08 1.5125e-09 1.5289e-11 9.3592e-14 3.3307e-16 0.037073 0.10492 0.2185 0.27902 0.2185 0.10492 0.030863 0.0055558 0.00061112 4.1008e-05 1.6758e-06 4.164e-08 6.2811e-10 5.7438e-12 3.1863e-14 0.0092081 0.040998 0.12635 0.23861 0.2763 0.19622 0.085427 0.022782 0.0037167 0.0003704 2.2511e-05 8.3291e-07 1.8732e-08 2.5567e-10 2.1255e-12 0.0017026 0.011702 0.053403 0.14921 0.25551 0.26831 0.17279 0.068209 0.016489 0.0024379 0.0002201 1.2114e-05 4.058e-07 8.2598e-09 1.0277e-10 0.00023263 0.0024379 0.016489 0.068209 0.17279 0.26831 0.25551 0.14921 0.053403 0.011702 0.0015678 0.00012823 6.3911e-06 1.9381e-07 3.6102e-09 2.3363e-05 0.0003704 0.0037167 0.022782 0.085427 0.19622 0.2763 0.23861 0.12635 0.040998 0.0081429 0.00098855 7.3236e-05 3.3054e-06 9.2263e-08 1.7181e-06 4.1008e-05 0.00061112 0.0055558 0.030863 0.10492 0.2185 0.27902 0.2185 0.10492 0.030863 0.0055558 0.00061112 4.1008e-05 1.7181e-06 9.2263e-08 3.3054e-06 7.3236e-05 0.00098855 0.0081429 0.040998 0.12635 0.23861 0.2763 0.19622 0.085427 0.022782 0.0037167 0.0003704 2.3363e-05 3.6102e-09 1.9381e-07 6.3911e-06 0.00012823 0.0015678 0.011702 0.053403 0.14921 0.25551 0.26831 0.17279 0.068209 0.016489 0.0024379 0.00023263 mt_z_trans1 mt_z_trans2 mt_z_trans3 mt_z_trans4 mt_z_trans5 mt_z_trans6 mt_z_trans7 mt_z_trans8 mt_z_trans9 mt_z_trans10 mt_z_trans11 mt_z_trans12 mt_z_trans13 mt_z_trans14 mt_z_trans15 ___________ ___________ ___________ ___________ ___________ ___________ ___________ ___________ ___________ ____________ ____________ ____________ ____________ ____________ ____________ 0.00023263 0.0024379 0.016489 0.068209 0.17279 0.26831 0.25551 0.14921 0.053403 0.011702 0.0015678 0.00012823 6.3911e-06 1.9381e-07 3.6102e-09 2.3363e-05 0.0003704 0.0037167 0.022782 0.085427 0.19622 0.2763 0.23861 0.12635 0.040998 0.0081429 0.00098855 7.3236e-05 3.3054e-06 9.2263e-08 1.7181e-06 4.1008e-05 0.00061112 0.0055558 0.030863 0.10492 0.2185 0.27902 0.2185 0.10492 0.030863 0.0055558 0.00061112 4.1008e-05 1.7181e-06 9.2263e-08 3.3054e-06 7.3236e-05 0.00098855 0.0081429 0.040998 0.12635 0.23861 0.2763 0.19622 0.085427 0.022782 0.0037167 0.0003704 2.3363e-05 3.6102e-09 1.9381e-07 6.3911e-06 0.00012823 0.0015678 0.011702 0.053403 0.14921 0.25551 0.26831 0.17279 0.068209 0.016489 0.0024379 0.00023263 1.0277e-10 8.2598e-09 4.058e-07 1.2114e-05 0.0002201 0.0024379 0.016489 0.068209 0.17279 0.26831 0.25551 0.14921 0.053403 0.011702 0.0017026 2.1256e-12 2.5567e-10 1.8732e-08 8.3291e-07 2.2511e-05 0.0003704 0.0037167 0.022782 0.085427 0.19622 0.2763 0.23861 0.12635 0.040998 0.0092081 3.1909e-14 5.7438e-12 6.2811e-10 4.164e-08 1.6758e-06 4.1008e-05 0.00061112 0.0055558 0.030863 0.10492 0.2185 0.27902 0.2185 0.10492 0.037073 3.474e-16 9.3597e-14 1.5289e-11 1.5125e-09 9.0736e-08 3.3054e-06 7.3236e-05 0.00098855 0.0081429 0.040998 0.12635 0.23861 0.2763 0.19622 0.11232 2.7412e-18 1.1057e-15 2.6998e-13 3.9889e-11 3.5701e-09 1.9381e-07 6.3911e-06 0.00012823 0.0015678 0.011702 0.053403 0.14921 0.25551 0.26831 0.26016 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ar_interp_coh_grid xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Variables: Var1: 30×1 double Values: Min -4.67 Median 25.197 Max 57.277 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ar_interp_c_grid xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Variables: Var1: 619272×1 double Values: Min 0.02 Median 30.984 Max 61.947 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx mt_interp_coh_grid_mesh_z xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx 30 15 mt_interp_coh_grid_mesh_z1 mt_interp_coh_grid_mesh_z2 mt_interp_coh_grid_mesh_z3 mt_interp_coh_grid_mesh_z4 mt_interp_coh_grid_mesh_z5 mt_interp_coh_grid_mesh_z6 mt_interp_coh_grid_mesh_z7 mt_interp_coh_grid_mesh_z8 mt_interp_coh_grid_mesh_z9 mt_interp_coh_grid_mesh_z10 mt_interp_coh_grid_mesh_z11 mt_interp_coh_grid_mesh_z12 mt_interp_coh_grid_mesh_z13 mt_interp_coh_grid_mesh_z14 mt_interp_coh_grid_mesh_z15 __________________________ __________________________ __________________________ __________________________ __________________________ __________________________ __________________________ __________________________ __________________________ ___________________________ ___________________________ ___________________________ ___________________________ ___________________________ ___________________________ -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -2.4576 -2.4576 -2.4576 -2.4576 -2.4576 -2.4576 -2.4576 -2.4576 -2.4576 -2.4576 -2.4576 -2.4576 -2.4576 -2.4576 -2.4576 -0.24518 -0.24518 -0.24518 -0.24518 -0.24518 -0.24518 -0.24518 -0.24518 -0.24518 -0.24518 -0.24518 -0.24518 -0.24518 -0.24518 -0.24518 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 1.9672 1.9672 1.9672 1.9672 1.9672 1.9672 1.9672 1.9672 1.9672 1.9672 1.9672 1.9672 1.9672 1.9672 1.9672 4.1796 4.1796 4.1796 4.1796 4.1796 4.1796 4.1796 4.1796 4.1796 4.1796 4.1796 4.1796 4.1796 4.1796 4.1796 6.392 6.392 6.392 6.392 6.392 6.392 6.392 6.392 6.392 6.392 6.392 6.392 6.392 6.392 6.392 8.6044 8.6044 8.6044 8.6044 8.6044 8.6044 8.6044 8.6044 8.6044 8.6044 8.6044 8.6044 8.6044 8.6044 8.6044 10.817 10.817 10.817 10.817 10.817 10.817 10.817 10.817 10.817 10.817 10.817 10.817 10.817 10.817 10.817 13.029 13.029 13.029 13.029 13.029 13.029 13.029 13.029 13.029 13.029 13.029 13.029 13.029 13.029 13.029 mt_interp_coh_grid_mesh_z1 mt_interp_coh_grid_mesh_z2 mt_interp_coh_grid_mesh_z3 mt_interp_coh_grid_mesh_z4 mt_interp_coh_grid_mesh_z5 mt_interp_coh_grid_mesh_z6 mt_interp_coh_grid_mesh_z7 mt_interp_coh_grid_mesh_z8 mt_interp_coh_grid_mesh_z9 mt_interp_coh_grid_mesh_z10 mt_interp_coh_grid_mesh_z11 mt_interp_coh_grid_mesh_z12 mt_interp_coh_grid_mesh_z13 mt_interp_coh_grid_mesh_z14 mt_interp_coh_grid_mesh_z15 __________________________ __________________________ __________________________ __________________________ __________________________ __________________________ __________________________ __________________________ __________________________ ___________________________ ___________________________ ___________________________ ___________________________ ___________________________ ___________________________ 37.366 37.366 37.366 37.366 37.366 37.366 37.366 37.366 37.366 37.366 37.366 37.366 37.366 37.366 37.366 39.578 39.578 39.578 39.578 39.578 39.578 39.578 39.578 39.578 39.578 39.578 39.578 39.578 39.578 39.578 41.79 41.79 41.79 41.79 41.79 41.79 41.79 41.79 41.79 41.79 41.79 41.79 41.79 41.79 41.79 44.003 44.003 44.003 44.003 44.003 44.003 44.003 44.003 44.003 44.003 44.003 44.003 44.003 44.003 44.003 46.215 46.215 46.215 46.215 46.215 46.215 46.215 46.215 46.215 46.215 46.215 46.215 46.215 46.215 46.215 48.428 48.428 48.428 48.428 48.428 48.428 48.428 48.428 48.428 48.428 48.428 48.428 48.428 48.428 48.428 50.64 50.64 50.64 50.64 50.64 50.64 50.64 50.64 50.64 50.64 50.64 50.64 50.64 50.64 50.64 52.852 52.852 52.852 52.852 52.852 52.852 52.852 52.852 52.852 52.852 52.852 52.852 52.852 52.852 52.852 55.065 55.065 55.065 55.065 55.065 55.065 55.065 55.065 55.065 55.065 55.065 55.065 55.065 55.065 55.065 57.277 57.277 57.277 57.277 57.277 57.277 57.277 57.277 57.277 57.277 57.277 57.277 57.277 57.277 57.277 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx mt_a xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx 45 27 mt_a1 mt_a2 mt_a3 mt_a4 mt_a5 mt_a6 mt_a7 mt_a8 mt_a9 mt_a10 mt_a11 mt_a12 mt_a13 mt_a14 mt_a15 mt_a16 mt_a17 mt_a18 mt_a19 mt_a20 mt_a21 mt_a22 mt_a23 mt_a24 mt_a25 mt_a26 mt_a27 _____ _______ ________ ________ _______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ -4.67 -2.4832 -0.29638 0 1.8904 4.0772 6.264 8.4508 10.638 12.824 15.011 17.198 19.385 21.572 23.758 25.945 28.132 30.319 32.506 34.692 36.879 39.066 41.253 43.44 45.626 47.813 50 -4.67 -2.5329 -0.39578 -0.10614 1.7413 3.8784 6.0155 8.1526 10.29 12.427 14.564 16.701 18.838 20.975 23.112 25.249 27.387 29.524 31.661 33.798 35.935 38.072 40.209 42.346 44.483 46.62 48.758 -4.67 -2.5826 -0.49518 -0.21227 1.5922 3.6796 5.767 7.8544 9.9418 12.029 14.117 16.204 18.291 20.379 22.466 24.554 26.641 28.728 30.816 32.903 34.991 37.078 39.165 41.253 43.34 45.428 47.515 -4.67 -2.6323 -0.59458 -0.31841 1.4431 3.4808 5.5185 7.5562 9.5939 11.632 13.669 15.707 17.745 19.782 21.82 23.858 25.896 27.933 29.971 32.009 34.046 36.084 38.122 40.159 42.197 44.235 46.273 -4.67 -2.682 -0.69398 -0.42454 1.294 3.282 5.27 7.258 9.246 11.234 13.222 15.21 17.198 19.186 21.174 23.162 25.15 27.138 29.126 31.114 33.102 35.09 37.078 39.066 41.054 43.042 45.03 -4.67 -2.7317 -0.79338 -0.53068 1.1449 3.0832 5.0215 6.9598 8.8981 10.836 12.775 14.713 16.651 18.59 20.528 22.466 24.405 26.343 28.281 30.219 32.158 34.096 36.034 37.973 39.911 41.849 43.788 -4.67 -2.7814 -0.89278 -0.63682 0.99582 2.8844 4.773 6.6616 8.5502 10.439 12.327 14.216 16.105 17.993 19.882 21.77 23.659 25.548 27.436 29.325 31.213 33.102 34.991 36.879 38.768 40.656 42.545 -4.67 -2.8311 -0.99218 -0.74295 0.84672 2.6856 4.5245 6.3634 8.2023 10.041 11.88 13.719 15.558 17.397 19.236 21.075 22.914 24.752 26.591 28.43 30.269 32.108 33.947 35.786 37.625 39.464 41.303 -4.67 -2.8808 -1.0916 -0.84909 0.69762 2.4868 4.276 6.0652 7.8544 9.6436 11.433 13.222 15.011 16.8 18.59 20.379 22.168 23.957 25.746 27.536 29.325 31.114 32.903 34.692 36.482 38.271 40.06 -4.67 -2.9305 -1.191 -0.95522 0.54852 2.288 4.0275 5.767 7.5065 9.246 10.986 12.725 14.465 16.204 17.944 19.683 21.423 23.162 24.902 26.641 28.381 30.12 31.86 33.599 35.339 37.078 38.818 mt_a1 mt_a2 mt_a3 mt_a4 mt_a5 mt_a6 mt_a7 mt_a8 mt_a9 mt_a10 mt_a11 mt_a12 mt_a13 mt_a14 mt_a15 mt_a16 mt_a17 mt_a18 mt_a19 mt_a20 mt_a21 mt_a22 mt_a23 mt_a24 mt_a25 mt_a26 mt_a27 _____ _______ _______ _______ _______ _______ _______ _______ _______ _______ ________ ________ ________ ________ ________ ________ ________ ________ ________ ________ ________ ________ ________ ________ _________ _______ ________ -4.67 -4.2227 -3.7754 -3.7148 -3.3281 -2.8808 -2.4335 -1.9862 -1.5389 -1.0916 -0.64428 -0.19698 0.25032 0.69762 1.1449 1.5922 2.0395 2.4868 2.9341 3.3814 3.8287 4.276 4.7233 5.1706 5.6179 6.0652 6.5125 -4.67 -4.2724 -3.8748 -3.8209 -3.4772 -3.0796 -2.682 -2.2844 -1.8868 -1.4892 -1.0916 -0.69398 -0.29638 0.10122 0.49882 0.89642 1.294 1.6916 2.0892 2.4868 2.8844 3.282 3.6796 4.0772 4.4748 4.8724 5.27 -4.67 -4.3221 -3.9742 -3.927 -3.6263 -3.2784 -2.9305 -2.5826 -2.2347 -1.8868 -1.5389 -1.191 -0.84308 -0.49518 -0.14728 0.20062 0.54852 0.89642 1.2443 1.5922 1.9401 2.288 2.6359 2.9838 3.3317 3.6796 4.0275 -4.67 -4.3718 -4.0736 -4.0332 -3.7754 -3.4772 -3.179 -2.8808 -2.5826 -2.2844 -1.9862 -1.688 -1.3898 -1.0916 -0.79338 -0.49518 -0.19698 0.10122 0.39942 0.69762 0.99582 1.294 1.5922 1.8904 2.1886 2.4868 2.785 -4.67 -4.4215 -4.173 -4.1393 -3.9245 -3.676 -3.4275 -3.179 -2.9305 -2.682 -2.4335 -2.185 -1.9365 -1.688 -1.4395 -1.191 -0.94248 -0.69398 -0.44548 -0.19698 0.051519 0.30002 0.54852 0.79702 1.0455 1.294 1.5425 -4.67 -4.4712 -4.2724 -4.2454 -4.0736 -3.8748 -3.676 -3.4772 -3.2784 -3.0796 -2.8808 -2.682 -2.4832 -2.2844 -2.0856 -1.8868 -1.688 -1.4892 -1.2904 -1.0916 -0.89278 -0.69398 -0.49518 -0.29638 -0.097581 0.10122 0.30002 -4.67 -4.5209 -4.3718 -4.3516 -4.2227 -4.0736 -3.9245 -3.7754 -3.6263 -3.4772 -3.3281 -3.179 -3.0299 -2.8808 -2.7317 -2.5826 -2.4335 -2.2844 -2.1353 -1.9862 -1.8371 -1.688 -1.5389 -1.3898 -1.2407 -1.0916 -0.94248 -4.67 -4.5706 -4.4712 -4.4577 -4.3718 -4.2724 -4.173 -4.0736 -3.9742 -3.8748 -3.7754 -3.676 -3.5766 -3.4772 -3.3778 -3.2784 -3.179 -3.0796 -2.9802 -2.8808 -2.7814 -2.682 -2.5826 -2.4832 -2.3838 -2.2844 -2.185 -4.67 -4.6203 -4.5706 -4.5638 -4.5209 -4.4712 -4.4215 -4.3718 -4.3221 -4.2724 -4.2227 -4.173 -4.1233 -4.0736 -4.0239 -3.9742 -3.9245 -3.8748 -3.8251 -3.7754 -3.7257 -3.676 -3.6263 -3.5766 -3.5269 -3.4772 -3.4275 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ar_a_meshk xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Variables: ar_a_meshk: 1215×1 double Values: Min -4.67 Median 4.7233 Max 50 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx mt_k xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx 45 27 mt_k1 mt_k2 mt_k3 mt_k4 mt_k5 mt_k6 mt_k7 mt_k8 mt_k9 mt_k10 mt_k11 mt_k12 mt_k13 mt_k14 mt_k15 mt_k16 mt_k17 mt_k18 mt_k19 mt_k20 mt_k21 mt_k22 mt_k23 mt_k24 mt_k25 mt_k26 mt_k27 _____ ______ ______ _______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0.0497 0.0994 0.10614 0.1491 0.1988 0.2485 0.2982 0.3479 0.3976 0.4473 0.497 0.5467 0.5964 0.6461 0.6958 0.7455 0.7952 0.8449 0.8946 0.9443 0.994 1.0437 1.0934 1.1431 1.1928 1.2425 0 0.0994 0.1988 0.21227 0.2982 0.3976 0.497 0.5964 0.6958 0.7952 0.8946 0.994 1.0934 1.1928 1.2922 1.3916 1.491 1.5904 1.6898 1.7892 1.8886 1.988 2.0874 2.1868 2.2862 2.3856 2.485 0 0.1491 0.2982 0.31841 0.4473 0.5964 0.7455 0.8946 1.0437 1.1928 1.3419 1.491 1.6401 1.7892 1.9383 2.0874 2.2365 2.3856 2.5347 2.6838 2.8329 2.982 3.1311 3.2802 3.4293 3.5784 3.7275 0 0.1988 0.3976 0.42454 0.5964 0.7952 0.994 1.1928 1.3916 1.5904 1.7892 1.988 2.1868 2.3856 2.5844 2.7832 2.982 3.1808 3.3796 3.5784 3.7772 3.976 4.1748 4.3736 4.5724 4.7712 4.97 0 0.2485 0.497 0.53068 0.7455 0.994 1.2425 1.491 1.7395 1.988 2.2365 2.485 2.7335 2.982 3.2305 3.479 3.7275 3.976 4.2245 4.473 4.7215 4.97 5.2185 5.467 5.7155 5.964 6.2125 0 0.2982 0.5964 0.63682 0.8946 1.1928 1.491 1.7892 2.0874 2.3856 2.6838 2.982 3.2802 3.5784 3.8766 4.1748 4.473 4.7712 5.0694 5.3676 5.6658 5.964 6.2622 6.5604 6.8586 7.1568 7.455 0 0.3479 0.6958 0.74295 1.0437 1.3916 1.7395 2.0874 2.4353 2.7832 3.1311 3.479 3.8269 4.1748 4.5227 4.8706 5.2185 5.5664 5.9143 6.2622 6.6101 6.958 7.3059 7.6538 8.0017 8.3496 8.6975 0 0.3976 0.7952 0.84909 1.1928 1.5904 1.988 2.3856 2.7832 3.1808 3.5784 3.976 4.3736 4.7712 5.1688 5.5664 5.964 6.3616 6.7592 7.1568 7.5544 7.952 8.3496 8.7472 9.1448 9.5424 9.94 0 0.4473 0.8946 0.95522 1.3419 1.7892 2.2365 2.6838 3.1311 3.5784 4.0257 4.473 4.9203 5.3676 5.8149 6.2622 6.7095 7.1568 7.6041 8.0514 8.4987 8.946 9.3933 9.8406 10.288 10.735 11.182 mt_k1 mt_k2 mt_k3 mt_k4 mt_k5 mt_k6 mt_k7 mt_k8 mt_k9 mt_k10 mt_k11 mt_k12 mt_k13 mt_k14 mt_k15 mt_k16 mt_k17 mt_k18 mt_k19 mt_k20 mt_k21 mt_k22 mt_k23 mt_k24 mt_k25 mt_k26 mt_k27 _____ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ 0 1.7395 3.479 3.7148 5.2185 6.958 8.6975 10.437 12.176 13.916 15.655 17.395 19.134 20.874 22.613 24.353 26.092 27.832 29.571 31.311 33.05 34.79 36.529 38.269 40.008 41.748 43.487 0 1.7892 3.5784 3.8209 5.3676 7.1568 8.946 10.735 12.524 14.314 16.103 17.892 19.681 21.47 23.26 25.049 26.838 28.627 30.416 32.206 33.995 35.784 37.573 39.362 41.152 42.941 44.73 0 1.8389 3.6778 3.927 5.5167 7.3556 9.1945 11.033 12.872 14.711 16.55 18.389 20.228 22.067 23.906 25.745 27.583 29.422 31.261 33.1 34.939 36.778 38.617 40.456 42.295 44.134 45.972 0 1.8886 3.7772 4.0332 5.6658 7.5544 9.443 11.332 13.22 15.109 16.997 18.886 20.775 22.663 24.552 26.44 28.329 30.218 32.106 33.995 35.883 37.772 39.661 41.549 43.438 45.326 47.215 0 1.9383 3.8766 4.1393 5.8149 7.7532 9.6915 11.63 13.568 15.506 17.445 19.383 21.321 23.26 25.198 27.136 29.074 31.013 32.951 34.889 36.828 38.766 40.704 42.643 44.581 46.519 48.457 0 1.988 3.976 4.2454 5.964 7.952 9.94 11.928 13.916 15.904 17.892 19.88 21.868 23.856 25.844 27.832 29.82 31.808 33.796 35.784 37.772 39.76 41.748 43.736 45.724 47.712 49.7 0 2.0377 4.0754 4.3516 6.1131 8.1508 10.188 12.226 14.264 16.302 18.339 20.377 22.415 24.452 26.49 28.528 30.565 32.603 34.641 36.679 38.716 40.754 42.792 44.829 46.867 48.905 50.942 0 2.0874 4.1748 4.4577 6.2622 8.3496 10.437 12.524 14.612 16.699 18.787 20.874 22.961 25.049 27.136 29.224 31.311 33.398 35.486 37.573 39.661 41.748 43.835 45.923 48.01 50.098 52.185 0 2.1371 4.2742 4.5638 6.4113 8.5484 10.685 12.823 14.96 17.097 19.234 21.371 23.508 25.645 27.782 29.919 32.056 34.194 36.331 38.468 40.605 42.742 44.879 47.016 49.153 51.29 53.427 0 2.1868 4.3736 4.67 6.5604 8.7472 10.934 13.121 15.308 17.494 19.681 21.868 24.055 26.242 28.428 30.615 32.802 34.989 37.176 39.362 41.549 43.736 45.923 48.11 50.296 52.483 54.67 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ar_k_mesha xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Variables: ar_k_mesha: 1215×1 double Values: Min 0 Median 9.3933 Max 54.67 pos = 1 ; key = f_coh ; val = @(z,b,k)(f_prod(z,k)+k*(1-fl_delta)+fl_w+b.*(1+fl_r_save).*(b>0)+b.*(1+fl_r_borr).*(b<=0)) pos = 2 ; key = f_cons ; val = @(coh,bprime,kprime)(coh-kprime-bprime) pos = 3 ; key = f_inc ; val = @(z,b,k)(f_prod(z,k)-(fl_delta)*k+fl_w+b.*(fl_r_save).*(b>0)+b.*(fl_r_borr).*(b<=0)) pos = 4 ; key = f_prod ; val = @(z,k)((fl_Amean.*(z)).*(k.^(fl_alpha))) pos = 5 ; key = f_util_crra ; val = @(c)(((c).^(1-fl_crra)-1)./(1-fl_crra)) pos = 6 ; key = f_util_log ; val = @(c)log(c) pos = 7 ; key = f_util_standin ; val = @(z,b,k)f_util_log((f_coh(z,b,k)-fl_b_bd).*((f_coh(z,b,k)-fl_b_bd)>fl_c_min)+fl_c_min.*((f_coh(z,b,k)-fl_b_bd)<=fl_c_min))
Display
if (bl_display_funcgrids) fft_container_map_display(armt_map, it_display_summmat_rowmax, it_display_summmat_colmax); fft_container_map_display(func_map, it_display_summmat_rowmax, it_display_summmat_colmax); end
---------------------------------------- ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Begin: Show all key and value pairs from container CONTAINER NAME: ARMT_MAP ---------------------------------------- Map with properties: Count: 18 KeyType: char ValueType: any xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ---------------------------------------- ---------------------------------------- pos = 1 ; key = ar_a_meshk ;rown= 1215 ,coln= 1 ar_a_meshk :mu= 8.5778 ,sd= 12.4414 ,min= -4.67 ,max= 50 zi_1_c1 _______ zi_1_R1 -4.67 zi_2_R2 -4.67 zi_3_R3 -4.67 zi_608_R608 8.4508 zi_1213_r1213 -2.185 zi_1214_r1214 -3.4275 zi_1215_r1215 -4.67 pos = 2 ; key = ar_ak_perc ;rown= 1 ,coln= 45 ar_ak_perc :mu= 0.5 ,sd= 0.2985 ,min= 0 ,max= 1 zi_1_C1 zi_2_C2 zi_3_C3 zi_23_c23 zi_43_c43 zi_44_c44 zi_45_c45 _______ ________ ________ _________ _________ _________ _________ zi_1_r1 0 0.022727 0.045455 0.5 0.95455 0.97727 1 pos = 3 ; key = ar_interp_c_grid ;rown= 1 ,coln= 619272 ar_interp_c_grid :mu= 30.9836 ,sd= 17.8769 ,min= 0.02 ,max= 61.9472 zi_1_C1 zi_2_C2 zi_3_C3 zi_309636_c309636 zi_619270_c619270 zi_619271_c619271 zi_619272_c619272 _______ _______ _______ _________________ _________________ _________________ _________________ zi_1_r1 0.02 0.0201 0.0202 30.984 61.947 61.947 61.947 pos = 4 ; key = ar_interp_coh_grid ;rown= 1 ,coln= 30 ar_interp_coh_grid :mu= 25.4269 ,sd= 19.1231 ,min= -4.67 ,max= 57.2773 zi_1_C1 zi_2_C2 zi_3_C3 zi_15_c15 zi_28_c28 zi_29_c29 zi_30_c30 _______ _______ ________ _________ _________ _________ _________ zi_1_r1 -4.67 -2.4576 -0.24518 24.091 52.852 55.065 57.277 pos = 5 ; key = ar_k_mesha ;rown= 1215 ,coln= 1 ar_k_mesha :mu= 13.2478 ,sd= 12.4414 ,min= 0 ,max= 54.67 zi_1_c1 _______ zi_1_R1 0 zi_2_R2 0 zi_3_R3 0 zi_608_R608 13.121 zi_1213_r1213 52.185 zi_1214_r1214 53.427 zi_1215_r1215 54.67 pos = 6 ; key = ar_stationary ;rown= 1 ,coln= 15 ar_stationary :mu= 0.066667 ,sd= 0.060897 ,min= 0.0027089 ,max= 0.16757 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 _________ _________ ________ _______ _________ _________ _________ zi_1_r1 0.0027089 0.0069499 0.018507 0.16757 0.018507 0.0069499 0.0027089 pos = 7 ; key = ar_w_level ;rown= 1 ,coln= 27 ar_w_level :mu= 21.8256 ,sd= 16.9711 ,min= -4.67 ,max= 50 zi_1_C1 zi_2_C2 zi_3_C3 zi_14_c14 zi_25_c25 zi_26_c26 zi_27_c27 _______ _______ ________ _________ _________ _________ _________ zi_1_r1 -4.67 -2.4832 -0.29638 21.572 45.626 47.813 50 pos = 8 ; key = ar_w_perc ;rown= 1 ,coln= 25 ar_w_perc :mu= 0.5 ,sd= 0.30666 ,min= 0 ,max= 1 zi_1_C1 zi_2_C2 zi_3_C3 zi_13_c13 zi_23_c23 zi_24_c24 zi_25_c25 _______ ________ ________ _________ _________ _________ _________ zi_1_r1 0 0.041667 0.083333 0.5 0.91667 0.95833 1 pos = 9 ; key = ar_z ;rown= 1 ,coln= 15 ar_z :mu= 1.1347 ,sd= 0.69878 ,min= 0.34741 ,max= 2.567 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 _______ _______ _______ _______ _________ _________ _________ zi_1_r1 0.34741 0.40076 0.4623 0.94436 1.9291 2.2253 2.567 pos = 10 ; key = it_ameshk_n ; val = 1215 pos = 11 ; key = mt_coh_wkb ;rown= 1215 ,coln= 15 mt_coh_wkb :mu= 23.8417 ,sd= 17.1131 ,min= -4.67 ,max= 57.2773 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 _______ _______ _______ _______ _________ _________ _________ zi_1_R1 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 zi_2_R2 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 zi_3_R3 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 zi_608_R608 22.054 22.189 22.345 23.562 26.05 26.798 27.662 zi_1213_r1213 47.504 47.725 47.981 49.983 54.072 55.302 56.721 zi_1214_r1214 47.299 47.522 47.78 49.799 53.923 55.163 56.594 zi_1215_r1215 47.093 47.319 47.579 49.614 53.772 55.023 56.466 pos = 12 ; key = mt_interp_coh_grid_mesh_w_perc ;rown= 25 ,coln= 30 mt_interp_coh_grid_mesh_w_perc :mu= 25.4269 ,sd= 18.8142 ,min= -4.67 ,max= 57.2773 zi_1_C1 zi_2_C2 zi_3_C3 zi_15_c15 zi_28_c28 zi_29_c29 zi_30_c30 _______ _______ ________ _________ _________ _________ _________ zi_1_R1 -4.67 -2.4576 -0.24518 24.091 52.852 55.065 57.277 zi_2_R2 -4.67 -2.4576 -0.24518 24.091 52.852 55.065 57.277 zi_3_R3 -4.67 -2.4576 -0.24518 24.091 52.852 55.065 57.277 zi_13_r13 -4.67 -2.4576 -0.24518 24.091 52.852 55.065 57.277 zi_23_r23 -4.67 -2.4576 -0.24518 24.091 52.852 55.065 57.277 zi_24_r24 -4.67 -2.4576 -0.24518 24.091 52.852 55.065 57.277 zi_25_r25 -4.67 -2.4576 -0.24518 24.091 52.852 55.065 57.277 pos = 13 ; key = mt_interp_coh_grid_mesh_z ;rown= 30 ,coln= 15 mt_interp_coh_grid_mesh_z :mu= 25.4269 ,sd= 18.8226 ,min= -4.67 ,max= 57.2773 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 ________ ________ ________ ________ _________ _________ _________ zi_1_R1 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 zi_2_R2 -2.4576 -2.4576 -2.4576 -2.4576 -2.4576 -2.4576 -2.4576 zi_3_R3 -0.24518 -0.24518 -0.24518 -0.24518 -0.24518 -0.24518 -0.24518 zi_15_r15 24.091 24.091 24.091 24.091 24.091 24.091 24.091 zi_28_r28 52.852 52.852 52.852 52.852 52.852 52.852 52.852 zi_29_r29 55.065 55.065 55.065 55.065 55.065 55.065 55.065 zi_30_r30 57.277 57.277 57.277 57.277 57.277 57.277 57.277 pos = 14 ; key = mt_k ;rown= 45 ,coln= 27 mt_k :mu= 13.2478 ,sd= 12.4414 ,min= 0 ,max= 54.67 zi_1_C1 zi_2_C2 zi_3_C3 zi_14_c14 zi_25_c25 zi_26_c26 zi_27_c27 _______ _______ _______ _________ _________ _________ _________ zi_1_R1 0 0 0 0 0 0 0 zi_2_R2 0 0.0497 0.0994 0.5964 1.1431 1.1928 1.2425 zi_3_R3 0 0.0994 0.1988 1.1928 2.2862 2.3856 2.485 zi_23_r23 0 1.0934 2.1868 13.121 25.148 26.242 27.335 zi_43_r43 0 2.0874 4.1748 25.049 48.01 50.098 52.185 zi_44_r44 0 2.1371 4.2742 25.645 49.153 51.29 53.427 zi_45_r45 0 2.1868 4.3736 26.242 50.296 52.483 54.67 pos = 15 ; key = mt_w_by_interp_coh_interp_grid ;rown= 25 ,coln= 30 mt_w_by_interp_coh_interp_grid :mu= 10.3784 ,sd= 14.2244 ,min= -4.67 ,max= 57.2773 zi_1_C1 zi_2_C2 zi_3_C3 zi_15_c15 zi_28_c28 zi_29_c29 zi_30_c30 _______ _______ ________ _________ _________ _________ _________ zi_1_R1 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 zi_2_R2 -4.67 -4.5778 -4.4856 -3.4716 -2.2732 -2.181 -2.0888 zi_3_R3 -4.67 -4.4856 -4.3012 -2.2732 0.12356 0.30792 0.49229 zi_13_r13 -4.67 -3.5638 -2.4576 9.7106 24.091 25.197 26.304 zi_23_r23 -4.67 -2.6419 -0.61391 21.694 48.059 50.087 52.115 zi_24_r24 -4.67 -2.5498 -0.42954 22.893 50.456 52.576 54.696 zi_25_r25 -4.67 -2.4576 -0.24518 24.091 52.852 55.065 57.277 pos = 16 ; key = mt_z_mesh_coh_interp_grid ;rown= 30 ,coln= 15 mt_z_mesh_coh_interp_grid :mu= 1.1347 ,sd= 0.67583 ,min= 0.34741 ,max= 2.567 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 _______ _______ _______ _______ _________ _________ _________ zi_1_R1 0.34741 0.40076 0.4623 0.94436 1.9291 2.2253 2.567 zi_2_R2 0.34741 0.40076 0.4623 0.94436 1.9291 2.2253 2.567 zi_3_R3 0.34741 0.40076 0.4623 0.94436 1.9291 2.2253 2.567 zi_15_r15 0.34741 0.40076 0.4623 0.94436 1.9291 2.2253 2.567 zi_28_r28 0.34741 0.40076 0.4623 0.94436 1.9291 2.2253 2.567 zi_29_r29 0.34741 0.40076 0.4623 0.94436 1.9291 2.2253 2.567 zi_30_r30 0.34741 0.40076 0.4623 0.94436 1.9291 2.2253 2.567 pos = 17 ; key = mt_z_mesh_coh_wkb ;rown= 1215 ,coln= 15 mt_z_mesh_coh_wkb :mu= 1.1347 ,sd= 0.6751 ,min= 0.34741 ,max= 2.567 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 _______ _______ _______ _______ _________ _________ _________ zi_1_R1 0.34741 0.40076 0.4623 0.94436 1.9291 2.2253 2.567 zi_2_R2 0.34741 0.40076 0.4623 0.94436 1.9291 2.2253 2.567 zi_3_R3 0.34741 0.40076 0.4623 0.94436 1.9291 2.2253 2.567 zi_608_R608 0.34741 0.40076 0.4623 0.94436 1.9291 2.2253 2.567 zi_1213_r1213 0.34741 0.40076 0.4623 0.94436 1.9291 2.2253 2.567 zi_1214_r1214 0.34741 0.40076 0.4623 0.94436 1.9291 2.2253 2.567 zi_1215_r1215 0.34741 0.40076 0.4623 0.94436 1.9291 2.2253 2.567 pos = 18 ; key = mt_z_trans ;rown= 15 ,coln= 15 mt_z_trans :mu= 0.066667 ,sd= 0.095337 ,min= 0 ,max= 0.27902 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 __________ __________ __________ __________ __________ __________ __________ zi_1_R1 0.26016 0.26831 0.25551 0.00012823 2.7001e-13 1.1102e-15 0 zi_2_R2 0.11232 0.19622 0.2763 0.00098855 1.5289e-11 9.3592e-14 3.3307e-16 zi_3_R3 0.037073 0.10492 0.2185 0.0055558 6.2811e-10 5.7438e-12 3.1863e-14 zi_8_R8 1.7181e-06 4.1008e-05 0.00061112 0.27902 0.00061112 4.1008e-05 1.7181e-06 zi_13_r13 3.1909e-14 5.7438e-12 6.2811e-10 0.0055558 0.2185 0.10492 0.037073 zi_14_r14 3.474e-16 9.3597e-14 1.5289e-11 0.00098855 0.2763 0.19622 0.11232 zi_15_r15 2.7412e-18 1.1057e-15 2.6998e-13 0.00012823 0.25551 0.26831 0.26016 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Matrix in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx rowN colN mean std min max __ ___ ____ __________ ________ ________ _________ _______ ar_a_meshk 1 1 1215 1 8.5778 12.441 -4.67 50 ar_ak_perc 2 2 1 45 0.5 0.2985 0 1 ar_interp_c_grid 3 3 1 6.1927e+05 30.984 17.877 0.02 61.947 ar_interp_coh_grid 4 4 1 30 25.427 19.123 -4.67 57.277 ar_k_mesha 5 5 1215 1 13.248 12.441 0 54.67 ar_stationary 6 6 1 15 0.066667 0.060897 0.0027089 0.16757 ar_w_level 7 7 1 27 21.826 16.971 -4.67 50 ar_w_perc 8 8 1 25 0.5 0.30666 0 1 ar_z 9 9 1 15 1.1347 0.69878 0.34741 2.567 mt_coh_wkb 10 11 1215 15 23.842 17.113 -4.67 57.277 mt_interp_coh_grid_mesh_w_perc 11 12 25 30 25.427 18.814 -4.67 57.277 mt_interp_coh_grid_mesh_z 12 13 30 15 25.427 18.823 -4.67 57.277 mt_k 13 14 45 27 13.248 12.441 0 54.67 mt_w_by_interp_coh_interp_grid 14 15 25 30 10.378 14.224 -4.67 57.277 mt_z_mesh_coh_interp_grid 15 16 30 15 1.1347 0.67583 0.34741 2.567 mt_z_mesh_coh_wkb 16 17 1215 15 1.1347 0.6751 0.34741 2.567 mt_z_trans 17 18 15 15 0.066667 0.095337 0 0.27902 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Scalars in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx value _ ___ _____ it_ameshk_n 1 10 1215 ---------------------------------------- ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Begin: Show all key and value pairs from container CONTAINER NAME: FUNC_MAP ---------------------------------------- Map with properties: Count: 7 KeyType: char ValueType: any xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ---------------------------------------- ---------------------------------------- pos = 1 ; key = f_coh ; val = @(z,b,k)(f_prod(z,k)+k*(1-fl_delta)+fl_w+b.*(1+fl_r_save).*(b>0)+b.*(1+fl_r_borr).*(b<=0)) pos = 2 ; key = f_cons ; val = @(coh,bprime,kprime)(coh-kprime-bprime) pos = 3 ; key = f_inc ; val = @(z,b,k)(f_prod(z,k)-(fl_delta)*k+fl_w+b.*(fl_r_save).*(b>0)+b.*(fl_r_borr).*(b<=0)) pos = 4 ; key = f_prod ; val = @(z,k)((fl_Amean.*(z)).*(k.^(fl_alpha))) pos = 5 ; key = f_util_crra ; val = @(c)(((c).^(1-fl_crra)-1)./(1-fl_crra)) pos = 6 ; key = f_util_log ; val = @(c)log(c) pos = 7 ; key = f_util_standin ; val = @(z,b,k)f_util_log((f_coh(z,b,k)-fl_b_bd).*((f_coh(z,b,k)-fl_b_bd)>fl_c_min)+fl_c_min.*((f_coh(z,b,k)-fl_b_bd)<=fl_c_min)) ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Scalars in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx xFunction _ ___ _________ f_coh 1 1 1 f_cons 2 2 2 f_inc 3 3 3 f_prod 4 4 4 f_util_crra 5 5 5 f_util_log 6 6 6 f_util_standin 7 7 7
end
ans = Map with properties: Count: 18 KeyType: char ValueType: any