Generate States/Choices/Shocks Grids, get Functions (Interpolated + Percentage + Risky + Safe Asset)
back to Fan's Dynamic Assets Repository Table of Content.
Contents
- FFS_IPWKZ_GET_FUNCGRID get funcs, params, states choices shocks grids
- Default
- Parse Parameters 1a
- Parse Parameters 1b
- Parse Parameters 2
- Parse Parameters 3
- Generate Asset and Choice Grid for 2nd stage Problem
- Get Shock Grids
- Get Equations
- Generate Cash-on-Hand/State Matrix
- Generate 1st Stage States: Interpolation Cash-on-hand Interpolation Grid
- Generate 1st Stage Choices: Interpolation Cash-on-hand Interpolation Grid
- Generate Interpolation Consumption Grid
- Initialize armt_map to store, state, choice, shock matrixes
- Store armt_map (1): 2nd Stage Problem Arrays and Matrixes
- Store armt_map (2): First Stage Aggregate Savings
- Store armt_map (3): First Stage Consumption and Cash-on-Hand Grids
- Store armt_map (4): Shock Grids
- Store Function Map
- Graph
- Graph 1: a and k choice grid graphs
- Graph 2: coh by shock
- Graph 3: 2nd stage coh reached by k' b' choices by index
- Graph 4: 2nd stage coh reached by k' b' choices by coh
- Graph 5: 1st State Aggregate Savings Choices by COH interpolation grids
- Display
function [armt_map, func_map] = ffs_ipwkz_get_funcgrid(varargin)
FFS_IPWKZ_GET_FUNCGRID get funcs, params, states choices shocks grids
centralized gateway for retrieving parameters, and solution grids and functions. Similar to ffs_akz_get_funcgrid function. This code only deals with savings problems.
The graphs below show the difference between percentage choice grid and level choice grid. See comments by graphs below for explanations of differences between the choice grids here and choice grids in the ffs_akz_get_funcgrid function.
Note that the first stage w grid is based on cash-on-hand level reached by the coh(k,w-k,z) possible choice and shock combinations. This coh(k,w-k,z) > max(w), which also means that at max(coh) grid, the w_perc choices at higher points require extrapolation. Extrapolation is based on nearest extrapolation.
@param param_map container parameter container
@param support_map container support container
@param bl_input_override boolean if true varargin contained param_map and support_map fully overrides local default. Local default is not invoked. This could be important for speed if this function is getting invoked within certain loops. Default is 0.
@return armt_map container container with states, choices and shocks grids that are inputs for grid based solution algorithm
@return func_map container container with function handles for consumption cash-on-hand etc.
@example
it_param_set = 2; bl_input_override = true; [param_map, support_map] = ffs_ipwkz_set_default_param(it_param_set); [armt_map, func_map] = ffs_ipwkz_get_funcgrid(param_map, support_map, bl_input_override);
@include
Default
if (~isempty(varargin)) % override when called from outside [param_map, support_map] = varargin{:}; else % default internal run [param_map, support_map] = ffs_ipwkz_set_default_param(); support_map('bl_graph_funcgrids') = true; support_map('bl_display_funcgrids') = true; % to be able to visually see choice grid points param_map('fl_b_bd') = 0; param_map('fl_w_min') = param_map('fl_b_bd'); param_map('it_w_perc_n') = 25; param_map('it_ak_perc_n') = 45; param_map('fl_w_interp_grid_gap') = 2; param_map('fl_coh_interp_grid_gap') = 2; default_maps = {param_map, support_map}; % numvarargs is the number of varagin inputted [default_maps{1:length(varargin)}] = varargin{:}; param_map = [param_map; default_maps{1}]; support_map = [support_map; default_maps{2}]; end
---------------------------------------- ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Begin: Show all key and value pairs from container CONTAINER NAME: PARAM_MAP ---------------------------------------- Map with properties: Count: 32 KeyType: char ValueType: any xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ---------------------------------------- ---------------------------------------- pos = 1 ; key = fl_Amean ; val = 1 pos = 2 ; key = fl_alpha ; val = 0.36 pos = 3 ; key = fl_b_bd ; val = 0 pos = 4 ; key = fl_beta ; val = 0.94 pos = 5 ; key = fl_c_min ; val = 0.02 pos = 6 ; key = fl_coh_interp_grid_gap ; val = 0.1 pos = 7 ; key = fl_crra ; val = 1.5 pos = 8 ; key = fl_delta ; val = 0.08 pos = 9 ; key = fl_k_max ; val = 50 pos = 10 ; key = fl_k_min ; val = 0 pos = 11 ; key = fl_nan_replace ; val = -9999 pos = 12 ; key = fl_r_borr ; val = 0.095 pos = 13 ; key = fl_r_save ; val = 0.025 pos = 14 ; key = fl_tol_dist ; val = 1e-05 pos = 15 ; key = fl_tol_pol ; val = 1e-05 pos = 16 ; key = fl_tol_val ; val = 1e-05 pos = 17 ; key = fl_w ; val = 0.44365 pos = 18 ; key = fl_w_interp_grid_gap ; val = 0.1 pos = 19 ; key = fl_w_max ; val = 50 pos = 20 ; key = fl_w_min ; val = 0 pos = 21 ; key = fl_z_mu ; val = 0 pos = 22 ; key = fl_z_rho ; val = 0.8 pos = 23 ; key = fl_z_sig ; val = 0.2 pos = 24 ; key = it_ak_perc_n ; val = 50 pos = 25 ; key = it_c_interp_grid_gap ; val = 0.0001 pos = 26 ; key = it_maxiter_dist ; val = 1000 pos = 27 ; key = it_maxiter_val ; val = 250 pos = 28 ; key = it_tol_pol_nochange ; val = 25 pos = 29 ; key = it_w_perc_n ; val = 50 pos = 30 ; key = it_z_n ; val = 15 pos = 31 ; key = st_analytical_stationary_type ; val = eigenvector pos = 32 ; key = st_model ; val = ipwkz ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Scalars in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx value __ ___ _______ fl_Amean 1 1 1 fl_alpha 2 2 0.36 fl_b_bd 3 3 0 fl_beta 4 4 0.94 fl_c_min 5 5 0.02 fl_coh_interp_grid_gap 6 6 0.1 fl_crra 7 7 1.5 fl_delta 8 8 0.08 fl_k_max 9 9 50 fl_k_min 10 10 0 fl_nan_replace 11 11 -9999 fl_r_borr 12 12 0.095 fl_r_save 13 13 0.025 fl_tol_dist 14 14 1e-05 fl_tol_pol 15 15 1e-05 fl_tol_val 16 16 1e-05 fl_w 17 17 0.44365 fl_w_interp_grid_gap 18 18 0.1 fl_w_max 19 19 50 fl_w_min 20 20 0 fl_z_mu 21 21 0 fl_z_rho 22 22 0.8 fl_z_sig 23 23 0.2 it_ak_perc_n 24 24 50 it_c_interp_grid_gap 25 25 0.0001 it_maxiter_dist 26 26 1000 it_maxiter_val 27 27 250 it_tol_pol_nochange 28 28 25 it_w_perc_n 29 29 50 it_z_n 30 30 15 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Strings in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx _ ___ st_analytical_stationary_type 1 31 st_model 2 32 ---------------------------------------- ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Begin: Show all key and value pairs from container CONTAINER NAME: SUPPORT_MAP ---------------------------------------- Map with properties: Count: 43 KeyType: char ValueType: any xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ---------------------------------------- ---------------------------------------- pos = 1 ; key = bl_display ; val = false pos = 2 ; key = bl_display_defparam ; val = true pos = 3 ; key = bl_display_dist ; val = false pos = 4 ; key = bl_display_evf ; val = false pos = 5 ; key = bl_display_final ; val = true pos = 6 ; key = bl_display_final_dist ; val = false pos = 7 ; key = bl_display_final_dist_detail ; val = false pos = 8 ; key = bl_display_funcgrids ; val = false pos = 9 ; key = bl_graph ; val = true pos = 10 ; key = bl_graph_coh_t_coh ; val = true pos = 11 ; key = bl_graph_evf ; val = false pos = 12 ; key = bl_graph_funcgrids ; val = false pos = 13 ; key = bl_graph_funcgrids_detail ; val = false pos = 14 ; key = bl_graph_onebyones ; val = true pos = 15 ; key = bl_graph_pol_lvl ; val = true pos = 16 ; key = bl_graph_pol_pct ; val = true pos = 17 ; key = bl_graph_val ; val = true pos = 18 ; key = bl_img_save ; val = false pos = 19 ; key = bl_mat ; val = false pos = 20 ; key = bl_post ; val = true pos = 21 ; key = bl_profile ; val = false pos = 22 ; key = bl_profile_dist ; val = false pos = 23 ; key = bl_time ; val = false pos = 24 ; key = it_display_every ; val = 5 pos = 25 ; key = it_display_final_colmax ; val = 12 pos = 26 ; key = it_display_final_rowmax ; val = 100 pos = 27 ; key = it_display_summmat_colmax ; val = 7 pos = 28 ; key = it_display_summmat_rowmax ; val = 7 pos = 29 ; key = st_img_name_main ; val = _default pos = 30 ; key = st_img_path ; val = C:/Users/fan/CodeDynaAsset//m_ipwkz//solve/img/ pos = 31 ; key = st_img_prefix ; val = pos = 32 ; key = st_img_suffix ; val = _p4.png pos = 33 ; key = st_mat_name_main ; val = _default pos = 34 ; key = st_mat_path ; val = C:/Users/fan/CodeDynaAsset//m_ipwkz//solve/mat/ pos = 35 ; key = st_mat_prefix ; val = pos = 36 ; key = st_mat_suffix ; val = _p4 pos = 37 ; key = st_mat_test_path ; val = C:/Users/fan/CodeDynaAsset//m_ipwkz//test/ff_ipwkz_ds_vecsv/mat/ pos = 38 ; key = st_matimg_path_root ; val = C:/Users/fan/CodeDynaAsset//m_ipwkz/ pos = 39 ; key = st_profile_name_main ; val = _default pos = 40 ; key = st_profile_path ; val = C:/Users/fan/CodeDynaAsset//m_ipwkz//solve/profile/ pos = 41 ; key = st_profile_prefix ; val = pos = 42 ; key = st_profile_suffix ; val = _p4 pos = 43 ; key = st_title_prefix ; val = ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Scalars in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx value __ ___ _____ bl_display 1 1 0 bl_display_defparam 2 2 1 bl_display_dist 3 3 0 bl_display_evf 4 4 0 bl_display_final 5 5 1 bl_display_final_dist 6 6 0 bl_display_final_dist_detail 7 7 0 bl_display_funcgrids 8 8 0 bl_graph 9 9 1 bl_graph_coh_t_coh 10 10 1 bl_graph_evf 11 11 0 bl_graph_funcgrids 12 12 0 bl_graph_funcgrids_detail 13 13 0 bl_graph_onebyones 14 14 1 bl_graph_pol_lvl 15 15 1 bl_graph_pol_pct 16 16 1 bl_graph_val 17 17 1 bl_img_save 18 18 0 bl_mat 19 19 0 bl_post 20 20 1 bl_profile 21 21 0 bl_profile_dist 22 22 0 bl_time 23 23 0 it_display_every 24 24 5 it_display_final_colmax 25 25 12 it_display_final_rowmax 26 26 100 it_display_summmat_colmax 27 27 7 it_display_summmat_rowmax 28 28 7 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Strings in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx __ ___ st_img_name_main 1 29 st_img_path 2 30 st_img_prefix 3 31 st_img_suffix 4 32 st_mat_name_main 5 33 st_mat_path 6 34 st_mat_prefix 7 35 st_mat_suffix 8 36 st_mat_test_path 9 37 st_matimg_path_root 10 38 st_profile_name_main 11 39 st_profile_path 12 40 st_profile_prefix 13 41 st_profile_suffix 14 42 st_title_prefix 15 43
Parse Parameters 1a
params_group = values(param_map, {'fl_b_bd', 'fl_w_min', 'fl_w_max'}); [fl_b_bd, fl_w_min, fl_w_max] = params_group{:}; params_group = values(param_map, {'fl_crra', 'fl_c_min'}); [fl_crra, fl_c_min] = params_group{:}; params_group = values(param_map, {'fl_Amean', 'fl_alpha', 'fl_delta'}); [fl_Amean, fl_alpha, fl_delta] = params_group{:}; params_group = values(param_map, {'fl_r_save', 'fl_r_borr', 'fl_w'}); [fl_r_save, fl_r_borr, fl_w] = params_group{:};
Parse Parameters 1b
params_group = values(param_map, {... 'it_w_perc_n', 'it_ak_perc_n',... 'it_c_interp_grid_gap', 'fl_w_interp_grid_gap', 'fl_coh_interp_grid_gap'}); [it_w_perc_n, it_ak_perc_n,... it_c_interp_grid_gap, fl_w_interp_grid_gap, fl_coh_interp_grid_gap] = params_group{:};
Parse Parameters 2
params_group = values(param_map, {'it_z_n', 'fl_z_mu', 'fl_z_rho', 'fl_z_sig'}); [it_z_n, fl_z_mu, fl_z_rho, fl_z_sig] = params_group{:};
Parse Parameters 3
params_group = values(support_map, {'bl_graph_funcgrids', 'bl_display_funcgrids'}); [bl_graph_funcgrids, bl_display_funcgrids] = params_group{:};
Generate Asset and Choice Grid for 2nd stage Problem
This generate triangular choice structure. Household choose total aggregate savings, and within that how much to put into risky capital and how much to put into safe assets, in percentages. See ffs_ipwkz_set_default_param for details.
% percentage grid for 1st stage choice problem, level grid for 2nd stage % solving optimal k given w and z. ar_w_perc = linspace(0.001, 0.999, it_w_perc_n); it_w_interp_n = (fl_w_max-fl_w_min)/(fl_w_interp_grid_gap); ar_w_level = linspace(fl_w_min, fl_w_max, it_w_interp_n); % max k given w, need to consider the possibility of borrowing. ar_k_max = ar_w_level - fl_b_bd; % k percentage choice grid ar_ak_perc = linspace(0.001, 0.999, it_ak_perc_n); % 2nd stage percentage choice matrixes % (ar_k_max') is it_w_interp_n by 1, and (ar_ak_perc) is 1 by it_ak_perc_n % mt_k is a it_w_interp_n by it_ak_perc_n matrix of choice points of k' % conditional on w, each column is a different w, each row for each col a % different k' value. mt_k = (ar_k_max'*ar_ak_perc)'; mt_a = (ar_w_level - mt_k); % can not have choice that are beyond feasible bound given the percentage % structure here. mt_bl_constrained = (mt_a < fl_b_bd); if (sum(mt_bl_constrained) > 0 ) error('at %s second stage choice points, percentage choice exceed bounds, can not happen',... num2str(sum(mt_bl_constrained))); end ar_a_meshk = mt_a(:); ar_k_mesha = mt_k(:);
Get Shock Grids
[~, mt_z_trans, ar_stationary, ar_z] = ffto_gen_tauchen_jhl(fl_z_mu,fl_z_rho,fl_z_sig,it_z_n);
Get Equations
[f_util_log, f_util_crra, f_util_standin, f_prod, f_inc, f_coh, f_cons] = ...
ffs_ipwkz_set_functions(fl_crra, fl_c_min, fl_Amean, fl_alpha, fl_delta, fl_r_save, fl_r_borr, fl_w);
Generate Cash-on-Hand/State Matrix
The endogenous state variable is cash-on-hand, it has it_z_n*it_a_n number of points, covering all reachable points when ar_a is the choice vector and ar_z is the shock vector. requires inputs from get Asset and choice grids, get shock grids, and get equations above.
mt_coh_wkb = f_coh(ar_z, ar_a_meshk, ar_k_mesha); mt_z_mesh_coh_wkb = repmat(ar_z, [size(mt_coh_wkb,1),1]); if (bl_display_funcgrids) % Generate Aggregate Variables ar_aplusk_mesh = ar_a_meshk + ar_k_mesha; % Genereate Table tab_ak_choices = array2table([ar_aplusk_mesh, ar_k_mesha, ar_a_meshk]); cl_col_names = {'ar_aplusk_mesh', 'ar_k_mesha', 'ar_a_meshk'}; tab_ak_choices.Properties.VariableNames = cl_col_names; % Label Table Variables tab_ak_choices.Properties.VariableDescriptions{'ar_aplusk_mesh'} = ... '*ar_aplusk_mesh*: ar_aplusk_mesh = ar_a_meshk + ar_k_mesha;'; tab_ak_choices.Properties.VariableDescriptions{'ar_k_mesha'} = ... '*ar_k_mesha*:'; tab_ak_choices.Properties.VariableDescriptions{'ar_a_meshk'} = ... '*ar_a_meshk*:'; cl_var_desc = tab_ak_choices.Properties.VariableDescriptions; for it_var_name = 1:length(cl_var_desc) disp(cl_var_desc{it_var_name}); end disp('----------------------------------------'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp('tab_ak_choices'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); it_rows_toshow = length(ar_w_level)*2; disp(size(tab_ak_choices)); disp(head(array2table(tab_ak_choices), it_rows_toshow)); disp(tail(array2table(tab_ak_choices), it_rows_toshow)); disp('----------------------------------------'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp('mt_coh_wkb'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp(size(mt_coh_wkb)); disp(head(array2table(mt_coh_wkb), it_rows_toshow)); disp(tail(array2table(mt_coh_wkb), it_rows_toshow)); end
*ar_aplusk_mesh*: ar_aplusk_mesh = ar_a_meshk + ar_k_mesha; *ar_k_mesha*: *ar_a_meshk*: ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx tab_ak_choices xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx 1125 3 tab_ak_choices1 tab_ak_choices2 tab_ak_choices3 ar_aplusk_mesh ar_k_mesha ar_a_meshk _______________ _______________ _______________ 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 2.0833 0.0020833 2.0813 2.0833 0.049337 2.034 2.0833 0.096591 1.9867 2.0833 0.14384 1.9395 2.0833 0.1911 1.8922 tab_ak_choices1 tab_ak_choices2 tab_ak_choices3 ar_aplusk_mesh ar_k_mesha ar_a_meshk _______________ _______________ _______________ 47.917 43.521 4.3953 47.917 44.608 3.3084 47.917 45.695 2.2216 47.917 46.782 1.1348 47.917 47.869 0.047917 50 0.05 49.95 50 1.1841 48.816 50 2.3182 47.682 50 3.4523 46.548 50 4.5864 45.414 50 5.7205 44.28 50 6.8545 43.145 50 7.9886 42.011 50 9.1227 40.877 50 10.257 39.743 50 11.391 38.609 50 12.525 37.475 50 13.659 36.341 50 14.793 35.207 50 15.927 34.073 50 17.061 32.939 50 18.195 31.805 50 19.33 30.67 50 20.464 29.536 50 21.598 28.402 50 22.732 27.268 50 23.866 26.134 50 25 25 50 26.134 23.866 50 27.268 22.732 50 28.402 21.598 50 29.536 20.464 50 30.67 19.33 50 31.805 18.195 50 32.939 17.061 50 34.073 15.927 50 35.207 14.793 50 36.341 13.659 50 37.475 12.525 50 38.609 11.391 50 39.743 10.257 50 40.877 9.1227 50 42.011 7.9886 50 43.145 6.8545 50 44.28 5.7205 50 45.414 4.5864 50 46.548 3.4523 50 47.682 2.3182 50 48.816 1.1841 50 49.95 0.05 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx mt_coh_wkb xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx 1125 15 mt_coh_wkb1 mt_coh_wkb2 mt_coh_wkb3 mt_coh_wkb4 mt_coh_wkb5 mt_coh_wkb6 mt_coh_wkb7 mt_coh_wkb8 mt_coh_wkb9 mt_coh_wkb10 mt_coh_wkb11 mt_coh_wkb12 mt_coh_wkb13 mt_coh_wkb14 mt_coh_wkb15 ___________ ___________ ___________ ___________ ___________ ___________ ___________ ___________ ___________ ____________ ____________ ____________ ____________ ____________ ____________ 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 2.6165 2.6223 2.6289 2.6366 2.6455 2.6557 2.6675 2.6811 2.6969 2.715 2.7359 2.76 2.7878 2.8199 2.8569 2.6915 2.7095 2.7304 2.7544 2.7821 2.8141 2.851 2.8935 2.9426 2.9993 3.0646 3.1399 3.2268 3.3271 3.4428 2.7187 2.7417 2.7682 2.7988 2.8341 2.8749 2.9218 2.976 3.0386 3.1107 3.1939 3.2898 3.4005 3.5282 3.6756 2.7368 2.7634 2.794 2.8293 2.8701 2.9171 2.9713 3.0338 3.106 3.1892 3.2852 3.396 3.5238 3.6712 3.8412 2.7505 2.7799 2.8138 2.8529 2.898 2.9501 3.0102 3.0795 3.1594 3.2516 3.3579 3.4806 3.6222 3.7854 3.9738 mt_coh_wkb1 mt_coh_wkb2 mt_coh_wkb3 mt_coh_wkb4 mt_coh_wkb5 mt_coh_wkb6 mt_coh_wkb7 mt_coh_wkb8 mt_coh_wkb9 mt_coh_wkb10 mt_coh_wkb11 mt_coh_wkb12 mt_coh_wkb13 mt_coh_wkb14 mt_coh_wkb15 ___________ ___________ ___________ ___________ ___________ ___________ ___________ ___________ ___________ ____________ ____________ ____________ ____________ ____________ ____________ 46.34 46.547 46.787 47.063 47.381 47.749 48.173 48.662 49.226 49.877 50.627 51.493 52.492 53.645 54.974 46.238 46.447 46.689 46.967 47.289 47.659 48.087 48.581 49.15 49.806 50.564 51.437 52.445 53.608 54.949 46.136 46.347 46.59 46.871 47.196 47.57 48.001 48.499 49.073 49.735 50.499 51.38 52.397 53.57 54.922 46.033 46.246 46.492 46.775 47.102 47.479 47.914 48.416 48.995 49.663 50.434 51.322 52.348 53.53 54.895 45.931 46.145 46.393 46.679 47.008 47.389 47.827 48.334 48.917 49.591 50.368 51.264 52.297 53.49 54.866 51.807 51.825 51.846 51.87 51.898 51.93 51.967 52.01 52.059 52.116 52.181 52.257 52.345 52.445 52.561 51.939 51.995 52.061 52.136 52.223 52.323 52.439 52.573 52.727 52.905 53.11 53.346 53.619 53.934 54.297 51.92 51.993 52.076 52.172 52.283 52.411 52.558 52.728 52.925 53.151 53.412 53.714 54.061 54.462 54.925 51.874 51.957 52.053 52.164 52.292 52.44 52.61 52.806 53.033 53.294 53.596 53.943 54.345 54.807 55.341 51.813 51.906 52.012 52.135 52.277 52.44 52.629 52.846 53.097 53.387 53.72 54.106 54.55 55.063 55.654 51.744 51.844 51.959 52.092 52.246 52.423 52.627 52.862 53.134 53.447 53.809 54.226 54.707 55.262 55.903 51.669 51.775 51.898 52.04 52.204 52.393 52.611 52.862 53.152 53.487 53.873 54.318 54.831 55.424 56.107 51.589 51.702 51.832 51.982 52.155 52.354 52.585 52.85 53.157 53.51 53.918 54.388 54.931 55.557 56.279 51.506 51.624 51.76 51.918 52.099 52.309 52.55 52.829 53.15 53.521 53.949 54.442 55.011 55.668 56.425 51.42 51.543 51.685 51.85 52.039 52.257 52.509 52.8 53.135 53.522 53.968 54.483 55.076 55.761 56.551 51.332 51.46 51.608 51.778 51.975 52.201 52.463 52.765 53.113 53.515 53.978 54.512 55.129 55.84 56.661 51.242 51.374 51.527 51.703 51.907 52.142 52.412 52.725 53.085 53.5 53.98 54.533 55.171 55.907 56.756 51.15 51.287 51.444 51.626 51.836 52.078 52.358 52.68 53.052 53.48 53.975 54.545 55.204 55.963 56.839 51.057 51.197 51.36 51.547 51.763 52.012 52.3 52.631 53.014 53.455 53.964 54.551 55.229 56.01 56.911 50.962 51.107 51.274 51.466 51.688 51.944 52.239 52.579 52.972 53.425 53.948 54.551 55.247 56.049 56.975 50.867 51.015 51.186 51.383 51.61 51.873 52.175 52.524 52.927 53.392 53.927 54.546 55.259 56.081 57.03 50.77 50.922 51.097 51.299 51.531 51.8 52.11 52.467 52.879 53.354 53.903 54.535 55.265 56.107 57.078 50.673 50.828 51.007 51.213 51.451 51.725 52.042 52.407 52.828 53.314 53.874 54.521 55.267 56.127 57.119 50.575 50.733 50.915 51.126 51.369 51.649 51.972 52.345 52.774 53.27 53.842 54.502 55.264 56.142 57.155 50.476 50.637 50.823 51.038 51.285 51.571 51.9 52.28 52.719 53.224 53.808 54.481 55.257 56.152 57.185 50.376 50.541 50.73 50.949 51.201 51.492 51.827 52.214 52.661 53.176 53.77 54.455 55.246 56.158 57.21 50.276 50.443 50.636 50.859 51.115 51.411 51.753 52.147 52.601 53.125 53.73 54.427 55.232 56.16 57.231 50.176 50.346 50.542 50.768 51.029 51.33 51.677 52.077 52.54 53.073 53.687 54.397 55.215 56.159 57.247 50.074 50.247 50.446 50.676 50.941 51.247 51.6 52.007 52.476 53.018 53.643 54.363 55.195 56.154 57.26 49.973 50.148 50.35 50.584 50.853 51.163 51.522 51.935 52.412 52.961 53.596 54.328 55.172 56.146 57.269 49.87 50.048 50.254 50.49 50.764 51.079 51.442 51.862 52.345 52.903 53.547 54.29 55.146 56.135 57.275 49.768 49.948 50.156 50.397 50.674 50.993 51.362 51.787 52.278 52.844 53.497 54.25 55.119 56.121 57.277 49.665 49.848 50.059 50.302 50.583 50.907 51.281 51.712 52.209 52.783 53.445 54.208 55.089 56.105 57.277 49.561 49.747 49.96 50.207 50.492 50.82 51.199 51.635 52.139 52.72 53.391 54.164 55.057 56.086 57.273 49.457 49.645 49.862 50.112 50.4 50.732 51.116 51.558 52.068 52.657 53.336 54.119 55.023 56.065 57.268 49.353 49.543 49.763 50.015 50.307 50.644 51.032 51.48 51.996 52.592 53.279 54.072 54.987 56.042 57.259 49.249 49.441 49.663 49.919 50.214 50.555 50.947 51.4 51.923 52.526 53.221 54.024 54.949 56.017 57.249 49.144 49.339 49.563 49.822 50.12 50.465 50.862 51.32 51.849 52.459 53.162 53.974 54.91 55.99 57.236 49.039 49.236 49.463 49.724 50.026 50.375 50.776 51.24 51.774 52.391 53.102 53.923 54.869 55.961 57.221 48.934 49.133 49.362 49.627 49.932 50.284 50.69 51.158 51.698 52.322 53.041 53.87 54.827 55.93 57.204 48.829 49.029 49.261 49.528 49.837 50.192 50.603 51.076 51.622 52.252 52.978 53.816 54.783 55.898 57.185 48.723 48.926 49.16 49.43 49.741 50.1 50.515 50.993 51.545 52.181 52.915 53.761 54.738 55.864 57.164 48.617 48.822 49.058 49.331 49.645 50.008 50.427 50.909 51.466 52.109 52.85 53.705 54.691 55.829 57.142 48.511 48.717 48.956 49.231 49.549 49.915 50.338 50.825 51.388 52.036 52.785 53.648 54.644 55.792 57.118 48.404 48.613 48.854 49.132 49.452 49.822 50.249 50.741 51.308 51.963 52.718 53.59 54.595 55.754 57.092 48.297 48.508 48.751 49.032 49.355 49.728 50.159 50.655 51.228 51.889 52.651 53.53 54.545 55.715 57.065 48.191 48.403 48.648 48.931 49.258 49.634 50.069 50.57 51.147 51.814 52.583 53.47 54.494 55.674 57.036 48.084 48.298 48.545 48.831 49.16 49.54 49.978 50.483 51.066 51.739 52.514 53.409 54.442 55.632 57.006 47.976 48.193 48.442 48.73 49.062 49.445 49.887 50.396 50.984 51.662 52.445 53.347 54.388 55.589 56.975 47.869 48.087 48.339 48.629 48.964 49.35 49.795 50.309 50.902 51.586 52.375 53.285 54.334 55.545 56.942
Generate 1st Stage States: Interpolation Cash-on-hand Interpolation Grid
For the iwkz problems, we solve the problem along a grid of cash-on-hand values, the interpolate to find v(k',b',z) at (k',b') choices. Crucially, we have to coh matrxies
fl_max_mt_coh = max(max(mt_coh_wkb)); fl_min_mt_coh = min(min(mt_coh_wkb)); it_coh_interp_n = (fl_max_mt_coh-fl_min_mt_coh)/(fl_coh_interp_grid_gap); ar_interp_coh_grid = linspace(fl_min_mt_coh, fl_max_mt_coh, it_coh_interp_n); [mt_interp_coh_grid_mesh_z, mt_z_mesh_coh_interp_grid] = ndgrid(ar_interp_coh_grid, ar_z); mt_interp_coh_grid_mesh_w_perc = repmat(ar_interp_coh_grid, [it_w_perc_n, 1]);
Generate 1st Stage Choices: Interpolation Cash-on-hand Interpolation Grid
previously, our ar_w was the first stage choice grid, the grid was the same for all coh levels. Now, for each coh level, there is a different ar_w. ar_interp_coh_grid is (1 by ar_interp_coh_grid) and ar_w_perc is ( 1 by it_w_perc_n). Conditional on z, each choice matrix is (it_w_perc_n by ar_interp_coh_grid). Here we are pre-computing the choice matrix. This could be a large matrix if the choice grid is large. This is the matrix of aggregate savings choices
if (fl_min_mt_coh < 0 ) % borrowing bound is below zero mt_w_by_interp_coh_interp_grid = ((ar_interp_coh_grid-fl_min_mt_coh)'*ar_w_perc)' + fl_min_mt_coh; else % savings only mt_w_by_interp_coh_interp_grid = ((ar_interp_coh_grid)'*ar_w_perc)'; end
Generate Interpolation Consumption Grid
We also interpolate over consumption to speed the program up. We only solve for u(c) at this grid for the iwkz problmes, and then interpolate other c values.
fl_c_max = max(max(mt_coh_wkb)) - fl_b_bd; it_interp_c_grid_n = (fl_c_max-fl_c_min)/(it_c_interp_grid_gap); ar_interp_c_grid = linspace(fl_c_min, fl_c_max, it_interp_c_grid_n);
Initialize armt_map to store, state, choice, shock matrixes
armt_map = containers.Map('KeyType','char', 'ValueType','any'); armtdesc_map = containers.Map('KeyType','char', 'ValueType','any');
Store armt_map (1): 2nd Stage Problem Arrays and Matrixes
armt_map('ar_ak_perc') = ar_ak_perc; armt_map('mt_k') = mt_k; armt_map('ar_a_meshk') = ar_a_meshk; armt_map('ar_k_mesha') = ar_k_mesha; armt_map('it_ameshk_n') = length(ar_a_meshk); armt_map('mt_coh_wkb') = mt_coh_wkb; armt_map('mt_z_mesh_coh_wkb') = mt_z_mesh_coh_wkb;
Store armt_map (2): First Stage Aggregate Savings
w = k' + b', w is aggregate Savings%
- ar_w_perc 1st stage, percentage w choice given coh, at each coh level the number of choice points is the same for this problem with percentage grid points.
- ar_w_level 2nd stage, level of w over which we solve the optimal percentage k' choices. Need to generate interpolant based on this so that we know optimal k* given ar_w_perc(coh) in the 1st stage
- mt_w_by_interp_coh_interp_grid 1st stage, generate w(coh, percent), meaning the level of w given coh and the percentage grid of ar_w_perc. Mesh this with the coh grid, Rows here correspond to percentage of w choices, columns correspond to cash-on-hand. The columns of cash-on-hand is determined by ar_interp_coh_grid, because we solve the 1st stage problem at that coh grid.
armt_map('ar_w_perc') = ar_w_perc; armt_map('ar_w_level') = ar_w_level; armt_map('mt_w_by_interp_coh_interp_grid') = mt_w_by_interp_coh_interp_grid; armt_map('mt_interp_coh_grid_mesh_w_perc') = mt_interp_coh_grid_mesh_w_perc;
Store armt_map (3): First Stage Consumption and Cash-on-Hand Grids
armt_map('ar_interp_c_grid') = ar_interp_c_grid; armt_map('ar_interp_coh_grid') = ar_interp_coh_grid; armt_map('mt_interp_coh_grid_mesh_z') = mt_interp_coh_grid_mesh_z; armt_map('mt_z_mesh_coh_interp_grid') = mt_z_mesh_coh_interp_grid;
Store armt_map (4): Shock Grids
armt_map('mt_z_trans') = mt_z_trans; armt_map('ar_stationary') = ar_stationary; armt_map('ar_z') = ar_z;
Store Function Map
func_map = containers.Map('KeyType','char', 'ValueType','any'); func_map('f_util_log') = f_util_log; func_map('f_util_crra') = f_util_crra; func_map('f_util_standin') = f_util_standin; func_map('f_prod') = f_prod; func_map('f_inc') = f_inc; func_map('f_coh') = f_coh; func_map('f_cons') = f_cons;
Graph
if (bl_graph_funcgrids)
Graph 1: a and k choice grid graphs
compare the figure here to the same figure in ffs_akz_get_funcgrid. there the grid points are on an even grid, half of the grid points have NA. for the grid here, the grid points get denser as we get closer to low w = k'+b' levels. This is what is different visually about percentage points based choice grid for the 2nd stage problem.
figure('PaperPosition', [0 0 7 4]); hold on; chart = plot(mt_a, mt_k, 'blue'); clr = jet(numel(chart)); for m = 1:numel(chart) set(chart(m),'Color',clr(m,:)) end if (length(ar_w_level) <= 100) scatter(ar_a_meshk, ar_k_mesha, 3, 'filled', ... 'MarkerEdgeColor', 'b', 'MarkerFaceColor', 'b'); end xline(0); yline(0); title('Choice Grids Conditional on k+a=w') ylabel('Capital Choice') xlabel({'Saving'}) legend2plot = fliplr([1 round(numel(chart)/3) round((2*numel(chart))/4) numel(chart)]); legendCell = cellstr(num2str(ar_w_level', 'k+a=%3.2f')); legend(chart(legend2plot), legendCell(legend2plot), 'Location','northeast'); grid on;
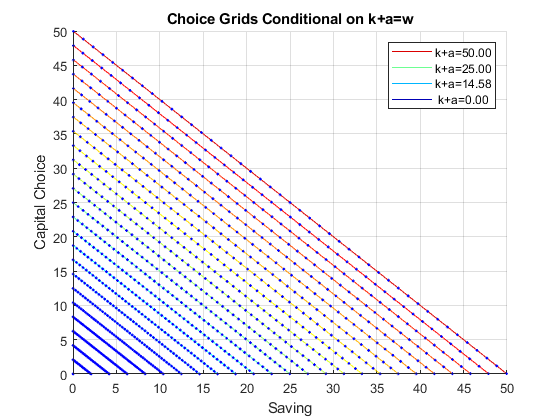
Graph 2: coh by shock
compare the figure here to the same figure in ffs_akz_get_funcgrid. there the grid points are on an even grid. Visually, one could see that the blue/red line segments here are always the same length, but in the ffs_akz_get_funcgrid figure, they are increasingly longer as we move towards the right. They are even because the number of percentage points available is constant regardless of w = k' + b' levels. But previously, the number of grid points available is increasing as w increases since choice grid is based on levels.
figure('PaperPosition', [0 0 7 4]); chart = plot(mt_coh_wkb); clr = jet(numel(chart)); for m = 1:numel(chart) set(chart(m),'Color',clr(m,:)) end xline(0); yline(0); title('Cash-on-Hand given w(k+b),k,z'); ylabel('Cash-on-Hand'); xlabel({'Index of Cash-on-Hand Discrete Point'... 'Each Segment is a w=k+b; within segment increasing k'... 'For each w and z, coh maximizing k is different'}); legend2plot = fliplr([1 round(numel(chart)/3) round((2*numel(chart))/4) numel(chart)]); legendCell = cellstr(num2str(ar_z', 'shock=%3.2f')); legend(chart(legend2plot), legendCell(legend2plot), 'Location','southeast'); grid on;
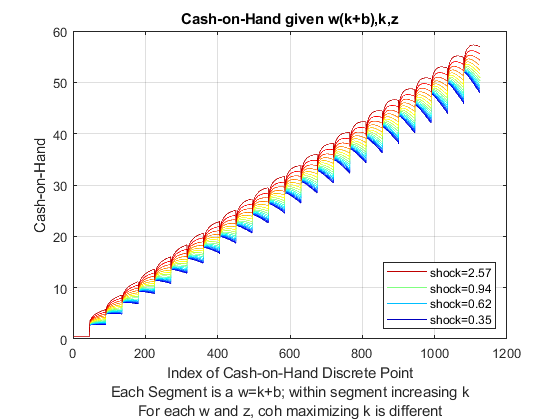
Graph 3: 2nd stage coh reached by k' b' choices by index
figure('PaperPosition', [0 0 7 4]); ar_coh_kpzgrid_unique = unique(sort(mt_coh_wkb(:))); scatter(1:length(ar_coh_kpzgrid_unique), ar_coh_kpzgrid_unique); xline(0); yline(0); title('Cash-on-Hand given w(k+b),k,z'); ylabel('Cash-on-Hand'); xlabel({'Index of Cash-on-Hand Discrete Point'}); grid on;
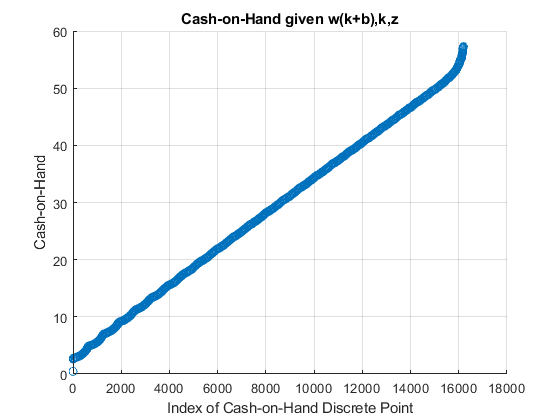
Graph 4: 2nd stage coh reached by k' b' choices by coh
figure('PaperPosition', [0 0 7 4]); ar_coh_kpzgrid_unique = unique(sort(mt_coh_wkb(:))); scatter(ar_coh_kpzgrid_unique, ar_coh_kpzgrid_unique, '.'); xline(0); yline(0); title('Cash-on-Hand given w(k+b),k,z; See Clearly Sparsity Density of Grid across Z'); ylabel('Cash-on-Hand'); xlabel({'Cash-on-Hand'}); grid on;
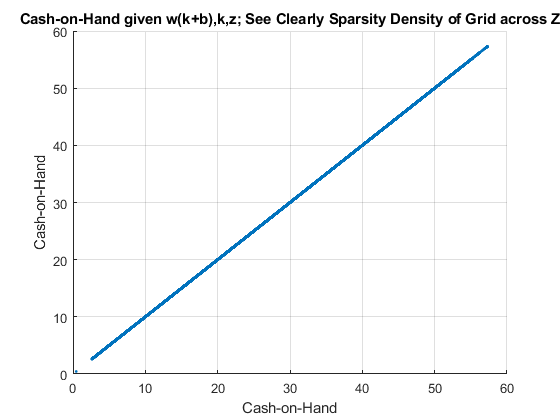
Graph 5: 1st State Aggregate Savings Choices by COH interpolation grids
figure('PaperPosition', [0 0 7 4]); hold on; chart = plot(ar_interp_coh_grid, mt_w_by_interp_coh_interp_grid'); clr = jet(numel(chart)); for m = 1:numel(chart) set(chart(m),'Color',clr(m,:)) end if (length(ar_interp_coh_grid) <= 100) [~, mt_interp_coh_grid_mesh_w_perc] = ndgrid(ar_w_perc, ar_interp_coh_grid); scatter(mt_interp_coh_grid_mesh_w_perc(:), mt_w_by_interp_coh_interp_grid(:), 3, 'filled', ... 'MarkerEdgeColor', 'b', 'MarkerFaceColor', 'b'); end xline0 = xline(0); xline0.HandleVisibility = 'off'; yline0 = yline(0); yline0.HandleVisibility = 'off'; title('Aggregate Savings Grid Given Cash-on-Hand'); ylabel('1st Stage Aggregate Savings Choices'); xlabel({'Cash-on-Hand Levels (Interpolation Points)'}); legend2plot = fliplr([1 round(numel(chart)/3) round((2*numel(chart))/4) numel(chart)]); legendCell = cellstr(num2str(ar_w_perc', 'ar w perc=%3.2f')); legend(chart(legend2plot), legendCell(legend2plot), 'Location','northwest'); grid on;
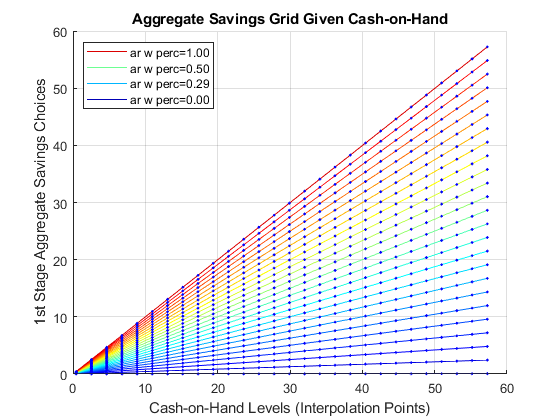
end
Display
if (bl_display_funcgrids) disp('----------------------------------------'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp('ar_z'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp(size(ar_z)); disp(ar_z); disp('----------------------------------------'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp('ar_w_level'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp(size(ar_w_level)); disp(ar_w_level); disp('----------------------------------------'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp('mt_w_by_interp_coh_interp_grid'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp(size(mt_w_by_interp_coh_interp_grid)); disp(head(array2table(mt_w_by_interp_coh_interp_grid), 10)); disp(tail(array2table(mt_w_by_interp_coh_interp_grid), 10)); disp('----------------------------------------'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp('mt_z_trans'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp(size(mt_z_trans)); disp(head(array2table(mt_z_trans), 10)); disp(tail(array2table(mt_z_trans), 10)); disp('----------------------------------------'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp('ar_interp_coh_grid'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); summary(array2table(ar_interp_coh_grid')); disp('----------------------------------------'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp('ar_interp_c_grid'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); summary(array2table(ar_interp_c_grid')); disp('----------------------------------------'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp('mt_interp_coh_grid_mesh_z'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp(size(mt_interp_coh_grid_mesh_z)); disp(head(array2table(mt_interp_coh_grid_mesh_z), 10)); disp(tail(array2table(mt_interp_coh_grid_mesh_z), 10)); disp('----------------------------------------'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp('mt_a'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp(size(mt_a)); disp(head(array2table(mt_a), 10)); disp(tail(array2table(mt_a), 10)); disp('----------------------------------------'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp('ar_a_meshk'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); summary(array2table(ar_a_meshk)); disp('----------------------------------------'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp('mt_k'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp(size(mt_k)); disp(head(array2table(mt_k), 10)); disp(tail(array2table(mt_k), 10)); disp('----------------------------------------'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp('ar_k_mesha'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); summary(array2table(ar_k_mesha)); param_map_keys = keys(func_map); param_map_vals = values(func_map); for i = 1:length(func_map) st_display = strjoin(['pos =' num2str(i) '; key =' string(param_map_keys{i}) '; val =' func2str(param_map_vals{i})]); disp(st_display); end end
---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ar_z xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx 1 15 Columns 1 through 7 0.3474 0.4008 0.4623 0.5333 0.6152 0.7097 0.8186 Columns 8 through 14 0.9444 1.0894 1.2567 1.4496 1.6723 1.9291 2.2253 Column 15 2.5670 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ar_w_level xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx 1 25 Columns 1 through 7 0 2.0833 4.1667 6.2500 8.3333 10.4167 12.5000 Columns 8 through 14 14.5833 16.6667 18.7500 20.8333 22.9167 25.0000 27.0833 Columns 15 through 21 29.1667 31.2500 33.3333 35.4167 37.5000 39.5833 41.6667 Columns 22 through 25 43.7500 45.8333 47.9167 50.0000 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx mt_w_by_interp_coh_interp_grid xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx 25 28 mt_w_by_interp_coh_interp_grid1 mt_w_by_interp_coh_interp_grid2 mt_w_by_interp_coh_interp_grid3 mt_w_by_interp_coh_interp_grid4 mt_w_by_interp_coh_interp_grid5 mt_w_by_interp_coh_interp_grid6 mt_w_by_interp_coh_interp_grid7 mt_w_by_interp_coh_interp_grid8 mt_w_by_interp_coh_interp_grid9 mt_w_by_interp_coh_interp_grid10 mt_w_by_interp_coh_interp_grid11 mt_w_by_interp_coh_interp_grid12 mt_w_by_interp_coh_interp_grid13 mt_w_by_interp_coh_interp_grid14 mt_w_by_interp_coh_interp_grid15 mt_w_by_interp_coh_interp_grid16 mt_w_by_interp_coh_interp_grid17 mt_w_by_interp_coh_interp_grid18 mt_w_by_interp_coh_interp_grid19 mt_w_by_interp_coh_interp_grid20 mt_w_by_interp_coh_interp_grid21 mt_w_by_interp_coh_interp_grid22 mt_w_by_interp_coh_interp_grid23 mt_w_by_interp_coh_interp_grid24 mt_w_by_interp_coh_interp_grid25 mt_w_by_interp_coh_interp_grid26 mt_w_by_interp_coh_interp_grid27 mt_w_by_interp_coh_interp_grid28 _______________________________ _______________________________ _______________________________ _______________________________ _______________________________ _______________________________ _______________________________ _______________________________ _______________________________ ________________________________ ________________________________ ________________________________ ________________________________ ________________________________ ________________________________ ________________________________ ________________________________ ________________________________ ________________________________ ________________________________ ________________________________ ________________________________ ________________________________ ________________________________ ________________________________ ________________________________ ________________________________ ________________________________ 0.00044365 0.0025486 0.0046535 0.0067585 0.0088634 0.010968 0.013073 0.015178 0.017283 0.019388 0.021493 0.023598 0.025703 0.027808 0.029913 0.032018 0.034123 0.036228 0.038333 0.040438 0.042543 0.044647 0.046752 0.048857 0.050962 0.053067 0.055172 0.057277 0.018892 0.10853 0.19816 0.2878 0.37743 0.46707 0.5567 0.64634 0.73598 0.82561 0.91525 1.0049 1.0945 1.1842 1.2738 1.3634 1.4531 1.5427 1.6323 1.722 1.8116 1.9012 1.9909 2.0805 2.1701 2.2598 2.3494 2.4391 0.03734 0.21451 0.39167 0.56884 0.746 0.92317 1.1003 1.2775 1.4547 1.6318 1.809 1.9862 2.1633 2.3405 2.5177 2.6948 2.872 3.0492 3.2263 3.4035 3.5807 3.7578 3.935 4.1122 4.2893 4.4665 4.6437 4.8208 0.055789 0.32049 0.58518 0.84988 1.1146 1.3793 1.644 1.9087 2.1734 2.4381 2.7028 2.9675 3.2321 3.4968 3.7615 4.0262 4.2909 4.5556 4.8203 5.085 5.3497 5.6144 5.8791 6.1438 6.4085 6.6732 6.9379 7.2026 0.074237 0.42646 0.77869 1.1309 1.4831 1.8354 2.1876 2.5398 2.8921 3.2443 3.5965 3.9487 4.301 4.6532 5.0054 5.3576 5.7099 6.0621 6.4143 6.7666 7.1188 7.471 7.8232 8.1755 8.5277 8.8799 9.2321 9.5844 0.092685 0.53244 0.9722 1.412 1.8517 2.2915 2.7312 3.171 3.6107 4.0505 4.4903 4.93 5.3698 5.8095 6.2493 6.6891 7.1288 7.5686 8.0083 8.4481 8.8878 9.3276 9.7674 10.207 10.647 11.087 11.526 11.966 0.11113 0.63842 1.1657 1.693 2.2203 2.7476 3.2749 3.8022 4.3294 4.8567 5.384 5.9113 6.4386 6.9659 7.4932 8.0205 8.5477 9.075 9.6023 10.13 10.657 11.184 11.711 12.239 12.766 13.293 13.821 14.348 0.12958 0.7444 1.3592 1.974 2.5889 3.2037 3.8185 4.4333 5.0481 5.663 6.2778 6.8926 7.5074 8.1222 8.737 9.3519 9.9667 10.582 11.196 11.811 12.426 13.041 13.656 14.27 14.885 15.5 16.115 16.73 0.14803 0.85038 1.5527 2.2551 2.9574 3.6598 4.3621 5.0645 5.7668 6.4692 7.1715 7.8739 8.5762 9.2786 9.9809 10.683 11.386 12.088 12.79 13.493 14.195 14.897 15.6 16.302 17.004 17.707 18.409 19.111 0.16648 0.95636 1.7462 2.5361 3.326 4.1159 4.9058 5.6956 6.4855 7.2754 8.0653 8.8552 9.645 10.435 11.225 12.015 12.805 13.594 14.384 15.174 15.964 16.754 17.544 18.334 19.124 19.913 20.703 21.493 mt_w_by_interp_coh_interp_grid1 mt_w_by_interp_coh_interp_grid2 mt_w_by_interp_coh_interp_grid3 mt_w_by_interp_coh_interp_grid4 mt_w_by_interp_coh_interp_grid5 mt_w_by_interp_coh_interp_grid6 mt_w_by_interp_coh_interp_grid7 mt_w_by_interp_coh_interp_grid8 mt_w_by_interp_coh_interp_grid9 mt_w_by_interp_coh_interp_grid10 mt_w_by_interp_coh_interp_grid11 mt_w_by_interp_coh_interp_grid12 mt_w_by_interp_coh_interp_grid13 mt_w_by_interp_coh_interp_grid14 mt_w_by_interp_coh_interp_grid15 mt_w_by_interp_coh_interp_grid16 mt_w_by_interp_coh_interp_grid17 mt_w_by_interp_coh_interp_grid18 mt_w_by_interp_coh_interp_grid19 mt_w_by_interp_coh_interp_grid20 mt_w_by_interp_coh_interp_grid21 mt_w_by_interp_coh_interp_grid22 mt_w_by_interp_coh_interp_grid23 mt_w_by_interp_coh_interp_grid24 mt_w_by_interp_coh_interp_grid25 mt_w_by_interp_coh_interp_grid26 mt_w_by_interp_coh_interp_grid27 mt_w_by_interp_coh_interp_grid28 _______________________________ _______________________________ _______________________________ _______________________________ _______________________________ _______________________________ _______________________________ _______________________________ _______________________________ ________________________________ ________________________________ ________________________________ ________________________________ ________________________________ ________________________________ ________________________________ ________________________________ ________________________________ ________________________________ ________________________________ ________________________________ ________________________________ ________________________________ ________________________________ ________________________________ ________________________________ ________________________________ ________________________________ 0.27717 1.5922 2.9073 4.2224 5.5374 6.8525 8.1675 9.4826 10.798 12.113 13.428 14.743 16.058 17.373 18.688 20.003 21.318 22.633 23.948 25.263 26.578 27.893 29.209 30.524 31.839 33.154 34.469 35.784 0.29562 1.6982 3.1008 4.5034 5.906 7.3086 8.7112 10.114 11.516 12.919 14.322 15.724 17.127 18.529 19.932 21.335 22.737 24.14 25.542 26.945 28.347 29.75 31.153 32.555 33.958 35.36 36.763 38.166 0.31407 1.8042 3.2943 4.7844 6.2746 7.7647 9.2548 10.745 12.235 13.725 15.215 16.705 18.196 19.686 21.176 22.666 24.156 25.646 27.136 28.626 30.117 31.607 33.097 34.587 36.077 37.567 39.057 40.547 0.33251 1.9102 3.4878 5.0655 6.6431 8.2208 9.7984 11.376 12.954 14.531 16.109 17.687 19.264 20.842 22.42 23.997 25.575 27.153 28.73 30.308 31.886 33.463 35.041 36.619 38.196 39.774 41.352 42.929 0.35096 2.0161 3.6813 5.3465 7.0117 8.6769 10.342 12.007 13.672 15.338 17.003 18.668 20.333 21.998 23.664 25.329 26.994 28.659 30.324 31.989 33.655 35.32 36.985 38.65 40.315 41.981 43.646 45.311 0.36941 2.1221 3.8748 5.6276 7.3803 9.133 10.886 12.638 14.391 16.144 17.897 19.649 21.402 23.155 24.907 26.66 28.413 30.166 31.918 33.671 35.424 37.176 38.929 40.682 42.435 44.187 45.94 47.693 0.38786 2.2281 4.0684 5.9086 7.7488 9.5891 11.429 13.27 15.11 16.95 18.79 20.631 22.471 24.311 26.151 27.992 29.832 31.672 33.512 35.353 37.193 39.033 40.873 42.714 44.554 46.394 48.234 50.075 0.40631 2.3341 4.2619 6.1896 8.1174 10.045 11.973 13.901 15.829 17.756 19.684 21.612 23.54 25.467 27.395 29.323 31.251 33.179 35.106 37.034 38.962 40.89 42.817 44.745 46.673 48.601 50.529 52.456 0.42476 2.4401 4.4554 6.4707 8.486 10.501 12.517 14.532 16.547 18.563 20.578 22.593 24.608 26.624 28.639 30.654 32.67 34.685 36.7 38.716 40.731 42.746 44.762 46.777 48.792 50.807 52.823 54.838 0.4432 2.546 4.6489 6.7517 8.8546 10.957 13.06 15.163 17.266 19.369 21.472 23.574 25.677 27.78 29.883 31.986 34.089 36.191 38.294 40.397 42.5 44.603 46.706 48.808 50.911 53.014 55.117 57.22 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx mt_z_trans xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx 15 15 mt_z_trans1 mt_z_trans2 mt_z_trans3 mt_z_trans4 mt_z_trans5 mt_z_trans6 mt_z_trans7 mt_z_trans8 mt_z_trans9 mt_z_trans10 mt_z_trans11 mt_z_trans12 mt_z_trans13 mt_z_trans14 mt_z_trans15 ___________ ___________ ___________ ___________ ___________ ___________ ___________ ___________ ___________ ____________ ____________ ____________ ____________ ____________ ____________ 0.26016 0.26831 0.25551 0.14921 0.053403 0.011702 0.0015678 0.00012823 6.3911e-06 1.9381e-07 3.5701e-09 3.9889e-11 2.7001e-13 1.1102e-15 0 0.11232 0.19622 0.2763 0.23861 0.12635 0.040998 0.0081429 0.00098855 7.3236e-05 3.3054e-06 9.0736e-08 1.5125e-09 1.5289e-11 9.3592e-14 3.3307e-16 0.037073 0.10492 0.2185 0.27902 0.2185 0.10492 0.030863 0.0055558 0.00061112 4.1008e-05 1.6758e-06 4.164e-08 6.2811e-10 5.7438e-12 3.1863e-14 0.0092081 0.040998 0.12635 0.23861 0.2763 0.19622 0.085427 0.022782 0.0037167 0.0003704 2.2511e-05 8.3291e-07 1.8732e-08 2.5567e-10 2.1255e-12 0.0017026 0.011702 0.053403 0.14921 0.25551 0.26831 0.17279 0.068209 0.016489 0.0024379 0.0002201 1.2114e-05 4.058e-07 8.2598e-09 1.0277e-10 0.00023263 0.0024379 0.016489 0.068209 0.17279 0.26831 0.25551 0.14921 0.053403 0.011702 0.0015678 0.00012823 6.3911e-06 1.9381e-07 3.6102e-09 2.3363e-05 0.0003704 0.0037167 0.022782 0.085427 0.19622 0.2763 0.23861 0.12635 0.040998 0.0081429 0.00098855 7.3236e-05 3.3054e-06 9.2263e-08 1.7181e-06 4.1008e-05 0.00061112 0.0055558 0.030863 0.10492 0.2185 0.27902 0.2185 0.10492 0.030863 0.0055558 0.00061112 4.1008e-05 1.7181e-06 9.2263e-08 3.3054e-06 7.3236e-05 0.00098855 0.0081429 0.040998 0.12635 0.23861 0.2763 0.19622 0.085427 0.022782 0.0037167 0.0003704 2.3363e-05 3.6102e-09 1.9381e-07 6.3911e-06 0.00012823 0.0015678 0.011702 0.053403 0.14921 0.25551 0.26831 0.17279 0.068209 0.016489 0.0024379 0.00023263 mt_z_trans1 mt_z_trans2 mt_z_trans3 mt_z_trans4 mt_z_trans5 mt_z_trans6 mt_z_trans7 mt_z_trans8 mt_z_trans9 mt_z_trans10 mt_z_trans11 mt_z_trans12 mt_z_trans13 mt_z_trans14 mt_z_trans15 ___________ ___________ ___________ ___________ ___________ ___________ ___________ ___________ ___________ ____________ ____________ ____________ ____________ ____________ ____________ 0.00023263 0.0024379 0.016489 0.068209 0.17279 0.26831 0.25551 0.14921 0.053403 0.011702 0.0015678 0.00012823 6.3911e-06 1.9381e-07 3.6102e-09 2.3363e-05 0.0003704 0.0037167 0.022782 0.085427 0.19622 0.2763 0.23861 0.12635 0.040998 0.0081429 0.00098855 7.3236e-05 3.3054e-06 9.2263e-08 1.7181e-06 4.1008e-05 0.00061112 0.0055558 0.030863 0.10492 0.2185 0.27902 0.2185 0.10492 0.030863 0.0055558 0.00061112 4.1008e-05 1.7181e-06 9.2263e-08 3.3054e-06 7.3236e-05 0.00098855 0.0081429 0.040998 0.12635 0.23861 0.2763 0.19622 0.085427 0.022782 0.0037167 0.0003704 2.3363e-05 3.6102e-09 1.9381e-07 6.3911e-06 0.00012823 0.0015678 0.011702 0.053403 0.14921 0.25551 0.26831 0.17279 0.068209 0.016489 0.0024379 0.00023263 1.0277e-10 8.2598e-09 4.058e-07 1.2114e-05 0.0002201 0.0024379 0.016489 0.068209 0.17279 0.26831 0.25551 0.14921 0.053403 0.011702 0.0017026 2.1256e-12 2.5567e-10 1.8732e-08 8.3291e-07 2.2511e-05 0.0003704 0.0037167 0.022782 0.085427 0.19622 0.2763 0.23861 0.12635 0.040998 0.0092081 3.1909e-14 5.7438e-12 6.2811e-10 4.164e-08 1.6758e-06 4.1008e-05 0.00061112 0.0055558 0.030863 0.10492 0.2185 0.27902 0.2185 0.10492 0.037073 3.474e-16 9.3597e-14 1.5289e-11 1.5125e-09 9.0736e-08 3.3054e-06 7.3236e-05 0.00098855 0.0081429 0.040998 0.12635 0.23861 0.2763 0.19622 0.11232 2.7412e-18 1.1057e-15 2.6998e-13 3.9889e-11 3.5701e-09 1.9381e-07 6.3911e-06 0.00012823 0.0015678 0.011702 0.053403 0.14921 0.25551 0.26831 0.26016 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ar_interp_coh_grid xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Variables: Var1: 28×1 double Values: Min 0.44365 Median 28.86 Max 57.277 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ar_interp_c_grid xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Variables: Var1: 572571×1 double Values: Min 0.02 Median 28.649 Max 57.277 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx mt_interp_coh_grid_mesh_z xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx 28 15 mt_interp_coh_grid_mesh_z1 mt_interp_coh_grid_mesh_z2 mt_interp_coh_grid_mesh_z3 mt_interp_coh_grid_mesh_z4 mt_interp_coh_grid_mesh_z5 mt_interp_coh_grid_mesh_z6 mt_interp_coh_grid_mesh_z7 mt_interp_coh_grid_mesh_z8 mt_interp_coh_grid_mesh_z9 mt_interp_coh_grid_mesh_z10 mt_interp_coh_grid_mesh_z11 mt_interp_coh_grid_mesh_z12 mt_interp_coh_grid_mesh_z13 mt_interp_coh_grid_mesh_z14 mt_interp_coh_grid_mesh_z15 __________________________ __________________________ __________________________ __________________________ __________________________ __________________________ __________________________ __________________________ __________________________ ___________________________ ___________________________ ___________________________ ___________________________ ___________________________ ___________________________ 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 2.5486 2.5486 2.5486 2.5486 2.5486 2.5486 2.5486 2.5486 2.5486 2.5486 2.5486 2.5486 2.5486 2.5486 2.5486 4.6535 4.6535 4.6535 4.6535 4.6535 4.6535 4.6535 4.6535 4.6535 4.6535 4.6535 4.6535 4.6535 4.6535 4.6535 6.7585 6.7585 6.7585 6.7585 6.7585 6.7585 6.7585 6.7585 6.7585 6.7585 6.7585 6.7585 6.7585 6.7585 6.7585 8.8634 8.8634 8.8634 8.8634 8.8634 8.8634 8.8634 8.8634 8.8634 8.8634 8.8634 8.8634 8.8634 8.8634 8.8634 10.968 10.968 10.968 10.968 10.968 10.968 10.968 10.968 10.968 10.968 10.968 10.968 10.968 10.968 10.968 13.073 13.073 13.073 13.073 13.073 13.073 13.073 13.073 13.073 13.073 13.073 13.073 13.073 13.073 13.073 15.178 15.178 15.178 15.178 15.178 15.178 15.178 15.178 15.178 15.178 15.178 15.178 15.178 15.178 15.178 17.283 17.283 17.283 17.283 17.283 17.283 17.283 17.283 17.283 17.283 17.283 17.283 17.283 17.283 17.283 19.388 19.388 19.388 19.388 19.388 19.388 19.388 19.388 19.388 19.388 19.388 19.388 19.388 19.388 19.388 mt_interp_coh_grid_mesh_z1 mt_interp_coh_grid_mesh_z2 mt_interp_coh_grid_mesh_z3 mt_interp_coh_grid_mesh_z4 mt_interp_coh_grid_mesh_z5 mt_interp_coh_grid_mesh_z6 mt_interp_coh_grid_mesh_z7 mt_interp_coh_grid_mesh_z8 mt_interp_coh_grid_mesh_z9 mt_interp_coh_grid_mesh_z10 mt_interp_coh_grid_mesh_z11 mt_interp_coh_grid_mesh_z12 mt_interp_coh_grid_mesh_z13 mt_interp_coh_grid_mesh_z14 mt_interp_coh_grid_mesh_z15 __________________________ __________________________ __________________________ __________________________ __________________________ __________________________ __________________________ __________________________ __________________________ ___________________________ ___________________________ ___________________________ ___________________________ ___________________________ ___________________________ 38.333 38.333 38.333 38.333 38.333 38.333 38.333 38.333 38.333 38.333 38.333 38.333 38.333 38.333 38.333 40.438 40.438 40.438 40.438 40.438 40.438 40.438 40.438 40.438 40.438 40.438 40.438 40.438 40.438 40.438 42.543 42.543 42.543 42.543 42.543 42.543 42.543 42.543 42.543 42.543 42.543 42.543 42.543 42.543 42.543 44.647 44.647 44.647 44.647 44.647 44.647 44.647 44.647 44.647 44.647 44.647 44.647 44.647 44.647 44.647 46.752 46.752 46.752 46.752 46.752 46.752 46.752 46.752 46.752 46.752 46.752 46.752 46.752 46.752 46.752 48.857 48.857 48.857 48.857 48.857 48.857 48.857 48.857 48.857 48.857 48.857 48.857 48.857 48.857 48.857 50.962 50.962 50.962 50.962 50.962 50.962 50.962 50.962 50.962 50.962 50.962 50.962 50.962 50.962 50.962 53.067 53.067 53.067 53.067 53.067 53.067 53.067 53.067 53.067 53.067 53.067 53.067 53.067 53.067 53.067 55.172 55.172 55.172 55.172 55.172 55.172 55.172 55.172 55.172 55.172 55.172 55.172 55.172 55.172 55.172 57.277 57.277 57.277 57.277 57.277 57.277 57.277 57.277 57.277 57.277 57.277 57.277 57.277 57.277 57.277 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx mt_a xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx 45 25 mt_a1 mt_a2 mt_a3 mt_a4 mt_a5 mt_a6 mt_a7 mt_a8 mt_a9 mt_a10 mt_a11 mt_a12 mt_a13 mt_a14 mt_a15 mt_a16 mt_a17 mt_a18 mt_a19 mt_a20 mt_a21 mt_a22 mt_a23 mt_a24 mt_a25 _____ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ 0 2.0813 4.1625 6.2438 8.325 10.406 12.488 14.569 16.65 18.731 20.813 22.894 24.975 27.056 29.138 31.219 33.3 35.381 37.462 39.544 41.625 43.706 45.788 47.869 49.95 0 2.034 4.068 6.102 8.136 10.17 12.204 14.238 16.272 18.306 20.34 22.374 24.408 26.442 28.476 30.51 32.544 34.578 36.612 38.646 40.68 42.714 44.748 46.782 48.816 0 1.9867 3.9735 5.9602 7.947 9.9337 11.92 13.907 15.894 17.881 19.867 21.854 23.841 25.828 27.814 29.801 31.788 33.775 35.761 37.748 39.735 41.722 43.708 45.695 47.682 0 1.9395 3.879 5.8185 7.758 9.6974 11.637 13.576 15.516 17.455 19.395 21.334 23.274 25.213 27.153 29.092 31.032 32.971 34.911 36.85 38.79 40.729 42.669 44.608 46.548 0 1.8922 3.7845 5.6767 7.5689 9.4612 11.353 13.246 15.138 17.03 18.922 20.815 22.707 24.599 26.491 28.384 30.276 32.168 34.06 35.952 37.845 39.737 41.629 43.521 45.414 0 1.845 3.69 5.5349 7.3799 9.2249 11.07 12.915 14.76 16.605 18.45 20.295 22.14 23.985 25.83 27.675 29.52 31.365 33.21 35.055 36.9 38.745 40.59 42.435 44.28 0 1.7977 3.5955 5.3932 7.1909 8.9886 10.786 12.584 14.382 16.18 17.977 19.775 21.573 23.37 25.168 26.966 28.764 30.561 32.359 34.157 35.955 37.752 39.55 41.348 43.145 0 1.7505 3.5009 5.2514 7.0019 8.7524 10.503 12.253 14.004 15.754 17.505 19.255 21.006 22.756 24.507 26.257 28.008 29.758 31.509 33.259 35.009 36.76 38.51 40.261 42.011 0 1.7032 3.4064 5.1097 6.8129 8.5161 10.219 11.923 13.626 15.329 17.032 18.735 20.439 22.142 23.845 25.548 27.252 28.955 30.658 32.361 34.064 35.768 37.471 39.174 40.877 0 1.656 3.3119 4.9679 6.6239 8.2798 9.9358 11.592 13.248 14.904 16.56 18.216 19.872 21.528 23.184 24.839 26.495 28.151 29.807 31.463 33.119 34.775 36.431 38.087 39.743 mt_a1 mt_a2 mt_a3 mt_a4 mt_a5 mt_a6 mt_a7 mt_a8 mt_a9 mt_a10 mt_a11 mt_a12 mt_a13 mt_a14 mt_a15 mt_a16 mt_a17 mt_a18 mt_a19 mt_a20 mt_a21 mt_a22 mt_a23 mt_a24 mt_a25 _____ _________ _________ _______ _________ ________ _______ ________ ________ _______ ________ ________ _______ ________ ________ _______ ________ ________ _______ ________ ________ _______ ________ ________ ______ 0 0.42737 0.85473 1.2821 1.7095 2.1368 2.5642 2.9916 3.4189 3.8463 4.2737 4.701 5.1284 5.5558 5.9831 6.4105 6.8379 7.2652 7.6926 8.12 8.5473 8.9747 9.4021 9.8295 10.257 0 0.38011 0.76023 1.1403 1.5205 1.9006 2.2807 2.6608 3.0409 3.421 3.8011 4.1813 4.5614 4.9415 5.3216 5.7017 6.0818 6.4619 6.842 7.2222 7.6023 7.9824 8.3625 8.7426 9.1227 0 0.33286 0.66572 0.99858 1.3314 1.6643 1.9972 2.33 2.6629 2.9957 3.3286 3.6615 3.9943 4.3272 4.66 4.9929 5.3258 5.6586 5.9915 6.3243 6.6572 6.9901 7.3229 7.6558 7.9886 0 0.28561 0.57121 0.85682 1.1424 1.428 1.7136 1.9992 2.2848 2.5705 2.8561 3.1417 3.4273 3.7129 3.9985 4.2841 4.5697 4.8553 5.1409 5.4265 5.7121 5.9977 6.2833 6.5689 6.8545 0 0.23835 0.4767 0.71506 0.95341 1.1918 1.4301 1.6685 1.9068 2.1452 2.3835 2.6219 2.8602 3.0986 3.3369 3.5753 3.8136 4.052 4.2903 4.5287 4.767 5.0054 5.2438 5.4821 5.7205 0 0.1911 0.3822 0.5733 0.76439 0.95549 1.1466 1.3377 1.5288 1.7199 1.911 2.1021 2.2932 2.4843 2.6754 2.8665 3.0576 3.2487 3.4398 3.6309 3.822 4.0131 4.2042 4.3953 4.5864 0 0.14384 0.28769 0.43153 0.57538 0.71922 0.86307 1.0069 1.1508 1.2946 1.4384 1.5823 1.7261 1.87 2.0138 2.1577 2.3015 2.4454 2.5892 2.733 2.8769 3.0207 3.1646 3.3084 3.4523 0 0.096591 0.19318 0.28977 0.38636 0.48295 0.57955 0.67614 0.77273 0.86932 0.96591 1.0625 1.1591 1.2557 1.3523 1.4489 1.5455 1.642 1.7386 1.8352 1.9318 2.0284 2.125 2.2216 2.3182 0 0.049337 0.098674 0.14801 0.19735 0.24669 0.29602 0.34536 0.3947 0.44403 0.49337 0.54271 0.59205 0.64138 0.69072 0.74006 0.78939 0.83873 0.88807 0.93741 0.98674 1.0361 1.0854 1.1348 1.1841 0 0.0020833 0.0041667 0.00625 0.0083333 0.010417 0.0125 0.014583 0.016667 0.01875 0.020833 0.022917 0.025 0.027083 0.029167 0.03125 0.033333 0.035417 0.0375 0.039583 0.041667 0.04375 0.045833 0.047917 0.05 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ar_a_meshk xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Variables: ar_a_meshk: 1125×1 double Values: Min 0 Median 9.1227 Max 49.95 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx mt_k xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx 45 25 mt_k1 mt_k2 mt_k3 mt_k4 mt_k5 mt_k6 mt_k7 mt_k8 mt_k9 mt_k10 mt_k11 mt_k12 mt_k13 mt_k14 mt_k15 mt_k16 mt_k17 mt_k18 mt_k19 mt_k20 mt_k21 mt_k22 mt_k23 mt_k24 mt_k25 _____ _________ _________ _______ _________ ________ _______ ________ ________ _______ ________ ________ _______ ________ ________ _______ ________ ________ _______ ________ ________ _______ ________ ________ ______ 0 0.0020833 0.0041667 0.00625 0.0083333 0.010417 0.0125 0.014583 0.016667 0.01875 0.020833 0.022917 0.025 0.027083 0.029167 0.03125 0.033333 0.035417 0.0375 0.039583 0.041667 0.04375 0.045833 0.047917 0.05 0 0.049337 0.098674 0.14801 0.19735 0.24669 0.29602 0.34536 0.3947 0.44403 0.49337 0.54271 0.59205 0.64138 0.69072 0.74006 0.78939 0.83873 0.88807 0.93741 0.98674 1.0361 1.0854 1.1348 1.1841 0 0.096591 0.19318 0.28977 0.38636 0.48295 0.57955 0.67614 0.77273 0.86932 0.96591 1.0625 1.1591 1.2557 1.3523 1.4489 1.5455 1.642 1.7386 1.8352 1.9318 2.0284 2.125 2.2216 2.3182 0 0.14384 0.28769 0.43153 0.57538 0.71922 0.86307 1.0069 1.1508 1.2946 1.4384 1.5823 1.7261 1.87 2.0138 2.1577 2.3015 2.4454 2.5892 2.733 2.8769 3.0207 3.1646 3.3084 3.4523 0 0.1911 0.3822 0.5733 0.76439 0.95549 1.1466 1.3377 1.5288 1.7199 1.911 2.1021 2.2932 2.4843 2.6754 2.8665 3.0576 3.2487 3.4398 3.6309 3.822 4.0131 4.2042 4.3953 4.5864 0 0.23835 0.4767 0.71506 0.95341 1.1918 1.4301 1.6685 1.9068 2.1452 2.3835 2.6219 2.8602 3.0986 3.3369 3.5753 3.8136 4.052 4.2903 4.5287 4.767 5.0054 5.2438 5.4821 5.7205 0 0.28561 0.57121 0.85682 1.1424 1.428 1.7136 1.9992 2.2848 2.5705 2.8561 3.1417 3.4273 3.7129 3.9985 4.2841 4.5697 4.8553 5.1409 5.4265 5.7121 5.9977 6.2833 6.5689 6.8545 0 0.33286 0.66572 0.99858 1.3314 1.6643 1.9972 2.33 2.6629 2.9957 3.3286 3.6615 3.9943 4.3272 4.66 4.9929 5.3258 5.6586 5.9915 6.3243 6.6572 6.9901 7.3229 7.6558 7.9886 0 0.38011 0.76023 1.1403 1.5205 1.9006 2.2807 2.6608 3.0409 3.421 3.8011 4.1813 4.5614 4.9415 5.3216 5.7017 6.0818 6.4619 6.842 7.2222 7.6023 7.9824 8.3625 8.7426 9.1227 0 0.42737 0.85473 1.2821 1.7095 2.1368 2.5642 2.9916 3.4189 3.8463 4.2737 4.701 5.1284 5.5558 5.9831 6.4105 6.8379 7.2652 7.6926 8.12 8.5473 8.9747 9.4021 9.8295 10.257 mt_k1 mt_k2 mt_k3 mt_k4 mt_k5 mt_k6 mt_k7 mt_k8 mt_k9 mt_k10 mt_k11 mt_k12 mt_k13 mt_k14 mt_k15 mt_k16 mt_k17 mt_k18 mt_k19 mt_k20 mt_k21 mt_k22 mt_k23 mt_k24 mt_k25 _____ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ 0 1.656 3.3119 4.9679 6.6239 8.2798 9.9358 11.592 13.248 14.904 16.56 18.216 19.872 21.528 23.184 24.839 26.495 28.151 29.807 31.463 33.119 34.775 36.431 38.087 39.743 0 1.7032 3.4064 5.1097 6.8129 8.5161 10.219 11.923 13.626 15.329 17.032 18.735 20.439 22.142 23.845 25.548 27.252 28.955 30.658 32.361 34.064 35.768 37.471 39.174 40.877 0 1.7505 3.5009 5.2514 7.0019 8.7524 10.503 12.253 14.004 15.754 17.505 19.255 21.006 22.756 24.507 26.257 28.008 29.758 31.509 33.259 35.009 36.76 38.51 40.261 42.011 0 1.7977 3.5955 5.3932 7.1909 8.9886 10.786 12.584 14.382 16.18 17.977 19.775 21.573 23.37 25.168 26.966 28.764 30.561 32.359 34.157 35.955 37.752 39.55 41.348 43.145 0 1.845 3.69 5.5349 7.3799 9.2249 11.07 12.915 14.76 16.605 18.45 20.295 22.14 23.985 25.83 27.675 29.52 31.365 33.21 35.055 36.9 38.745 40.59 42.435 44.28 0 1.8922 3.7845 5.6767 7.5689 9.4612 11.353 13.246 15.138 17.03 18.922 20.815 22.707 24.599 26.491 28.384 30.276 32.168 34.06 35.952 37.845 39.737 41.629 43.521 45.414 0 1.9395 3.879 5.8185 7.758 9.6974 11.637 13.576 15.516 17.455 19.395 21.334 23.274 25.213 27.153 29.092 31.032 32.971 34.911 36.85 38.79 40.729 42.669 44.608 46.548 0 1.9867 3.9735 5.9602 7.947 9.9337 11.92 13.907 15.894 17.881 19.867 21.854 23.841 25.828 27.814 29.801 31.788 33.775 35.761 37.748 39.735 41.722 43.708 45.695 47.682 0 2.034 4.068 6.102 8.136 10.17 12.204 14.238 16.272 18.306 20.34 22.374 24.408 26.442 28.476 30.51 32.544 34.578 36.612 38.646 40.68 42.714 44.748 46.782 48.816 0 2.0813 4.1625 6.2438 8.325 10.406 12.488 14.569 16.65 18.731 20.813 22.894 24.975 27.056 29.138 31.219 33.3 35.381 37.462 39.544 41.625 43.706 45.788 47.869 49.95 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ar_k_mesha xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Variables: ar_k_mesha: 1125×1 double Values: Min 0 Median 9.1227 Max 49.95 pos = 1 ; key = f_coh ; val = @(z,b,k)(f_prod(z,k)+k*(1-fl_delta)+fl_w+b.*(1+fl_r_save).*(b>0)+b.*(1+fl_r_borr).*(b<=0)) pos = 2 ; key = f_cons ; val = @(coh,bprime,kprime)(coh-kprime-bprime) pos = 3 ; key = f_inc ; val = @(z,b,k)(f_prod(z,k)-(fl_delta)*k+fl_w+b.*(fl_r_save).*(b>0)+b.*(fl_r_borr).*(b<=0)) pos = 4 ; key = f_prod ; val = @(z,k)((fl_Amean.*(z)).*(k.^(fl_alpha))) pos = 5 ; key = f_util_crra ; val = @(c)(((c).^(1-fl_crra)-1)./(1-fl_crra)) pos = 6 ; key = f_util_log ; val = @(c)log(c) pos = 7 ; key = f_util_standin ; val = @(z,b,k)f_util_log(f_coh(z,b,k).*(f_coh(z,b,k)>0)+fl_c_min.*(f_coh(z,b,k)<=0))
end
ans = Map with properties: Count: 18 KeyType: char ValueType: any