Risky + Safe Asset Dyna Prog Two-Step-Interpolated Solution (Loop)
back to Fan's Dynamic Assets Repository Table of Content.
Contents
- FF_IWKZ_VF solve infinite horizon exo shock + endo asset problem
- Default
- Parse Parameters 1
- Parse Parameters 2
- Initialize Output Matrixes
- Initialize Convergence Conditions
- Pre-calculate u(c)
- Iterate Value Function
- Interpolate (1) reacahble v(coh(k(w,z),b(w,z),z),z) given v(coh, z)
- Solve Second Stage Problem k*(w,z)
- Solve First Stage Problem w*(z) given k*(w,z)
- Check Tolerance and Continuation
- Process Optimal Choices
- Display Various Containers
- Display 1 support_map
- Display 2 armt_map
- Display 3 param_map
- Display 4 func_map
- Display 5 result_map
function result_map = ff_iwkz_vf(varargin)
FF_IWKZ_VF solve infinite horizon exo shock + endo asset problem
This program solves the infinite horizon dynamic savings and risky capital asset problem with some ar1 shock. This is the two step solution with interpolation version of ff_wkz_vf, which solves the problem in two steps without interpolation. See ff_wkz_evf for details about the second stage.
@param param_map container parameter container
@param support_map container support container
@param armt_map container container with states, choices and shocks grids that are inputs for grid based solution algorithm
@param func_map container container with function handles for consumption cash-on-hand etc.
@return result_map container contains policy function matrix, value function matrix, iteration results, and policy function, value function and iteration results tables.
keys included in result_map:
- mt_val matrix states_n by shock_n matrix of converged value function grid
- mt_pol_a matrix states_n by shock_n matrix of converged policy function grid
- ar_val_diff_norm array if bl_post = true it_iter_last by 1 val function difference between iteration
- ar_pol_diff_norm array if bl_post = true it_iter_last by 1 policy function difference between iterations
- mt_pol_perc_change matrix if bl_post = true it_iter_last by shock_n the proportion of grid points at which policy function changed between current and last iteration for each element of shock
@example
@include
@seealso
- concurrent (safe + risky) loop: ff_akz_vf
- concurrent (safe + risky) vectorized: ff_akz_vf_vec
- concurrent (safe + risky) optimized-vectorized: ff_akz_vf_vecsv
- two-stage (safe + risky) loop: ff_wkz_vf
- two-stage (safe + risky) vectorized: ff_wkz_vf_vec
- two-stage (safe + risky) optimized-vectorized: ff_wkz_vf_vecsv
- two-stage + interpolate (safe + risky) loop: ff_iwkz_vf
- two-stage + interpolate (safe + risky) vectorized: ff_iwkz_vf_vec
- two-stage + interpolate (safe + risky) optimized-vectorized: ff_iwkz_vf_vecsv
Default
- it_param_set = 1: quick test
- it_param_set = 2: benchmark run
- it_param_set = 3: benchmark profile
- it_param_set = 4: press publish button
it_param_set = 2; [param_map, support_map] = ffs_akz_set_default_param(it_param_set); % Note: param_map and support_map can be adjusted here or outside to override defaults % param_map('it_w_n') = 50; % param_map('it_ak_n') = param_map('it_w_n'); % param_map('it_z_n') = 15; % param_map('fl_coh_interp_grid_gap') = 0.1; % param_map('it_c_interp_grid_gap') = 10^-4; % get armt and func map [armt_map, func_map] = ffs_akz_get_funcgrid(param_map, support_map); % 1 for override default_params = {param_map support_map armt_map func_map};
Parse Parameters 1
% if varargin only has param_map and support_map, params_len = length(varargin); [default_params{1:params_len}] = varargin{:}; param_map = [param_map; default_params{1}]; support_map = [support_map; default_params{2}]; if params_len >= 1 && params_len <= 2 % If override param_map, re-generate armt and func if they are not % provided [armt_map, func_map] = ffs_akz_get_funcgrid(param_map, support_map); else % Override all armt_map = [armt_map; default_params{3}]; func_map = [func_map; default_params{4}]; end % append function name st_func_name = 'ff_iwkz_vf'; support_map('st_profile_name_main') = [st_func_name support_map('st_profile_name_main')]; support_map('st_mat_name_main') = [st_func_name support_map('st_mat_name_main')]; support_map('st_img_name_main') = [st_func_name support_map('st_img_name_main')];
Parse Parameters 2
% armt_map params_group = values(armt_map, {'ar_w', 'ar_z'}); [ar_w, ar_z] = params_group{:}; params_group = values(armt_map, {'ar_interp_c_grid', 'ar_interp_coh_grid', ... 'mt_interp_coh_grid_mesh_z', 'mt_z_mesh_coh_interp_grid'}); [ar_interp_c_grid, ar_interp_coh_grid, ... mt_interp_coh_grid_mesh_z, mt_z_mesh_coh_interp_grid] = params_group{:}; params_group = values(armt_map, {'mt_coh_wkb', 'mt_z_mesh_coh_wkb'}); [mt_coh_wkb, mt_z_mesh_coh_wkb] = params_group{:}; % func_map params_group = values(func_map, {'f_util_log', 'f_util_crra', 'f_cons'}); [f_util_log, f_util_crra, f_cons] = params_group{:}; % param_map params_group = values(param_map, {'fl_r_save', 'fl_r_borr', 'fl_w',... 'it_z_n', 'fl_crra', 'fl_beta', 'fl_c_min'}); [fl_r_save, fl_r_borr, fl_wage, it_z_n, fl_crra, fl_beta, fl_c_min] = params_group{:}; params_group = values(param_map, {'it_maxiter_val', 'fl_tol_val', 'fl_tol_pol', 'it_tol_pol_nochange'}); [it_maxiter_val, fl_tol_val, fl_tol_pol, it_tol_pol_nochange] = params_group{:}; % support_map params_group = values(support_map, {'bl_profile', 'st_profile_path', ... 'st_profile_prefix', 'st_profile_name_main', 'st_profile_suffix',... 'bl_time', 'bl_display_defparam', 'bl_graph_evf', 'bl_display', 'it_display_every', 'bl_post'}); [bl_profile, st_profile_path, ... st_profile_prefix, st_profile_name_main, st_profile_suffix, ... bl_time, bl_display_defparam, bl_graph_evf, bl_display, it_display_every, bl_post] = params_group{:}; params_group = values(support_map, {'it_display_summmat_rowmax', 'it_display_summmat_colmax'}); [it_display_summmat_rowmax, it_display_summmat_colmax] = params_group{:};
Initialize Output Matrixes
mt_val_cur = zeros(length(ar_interp_coh_grid),length(ar_z)); mt_val = mt_val_cur - 1; mt_pol_a = zeros(length(ar_interp_coh_grid),length(ar_z)); mt_pol_a_cur = mt_pol_a - 1; mt_pol_k = zeros(length(ar_interp_coh_grid),length(ar_z)); mt_pol_k_cur = mt_pol_k - 1;
Initialize Convergence Conditions
bl_vfi_continue = true; it_iter = 0; ar_val_diff_norm = zeros([it_maxiter_val, 1]); ar_pol_diff_norm = zeros([it_maxiter_val, 1]); mt_pol_perc_change = zeros([it_maxiter_val, it_z_n]);
Pre-calculate u(c)
Interpolation, see fs_u_c_partrepeat_main for why interpolate over u(c)
% Evaluate if (fl_crra == 1) ar_interp_u_of_c_grid = f_util_log(ar_interp_c_grid); fl_u_neg_c = f_util_log(fl_c_min); else ar_interp_u_of_c_grid = f_util_crra(ar_interp_c_grid); fl_u_neg_c = f_util_crra(fl_c_min); end ar_interp_u_of_c_grid(ar_interp_c_grid <= fl_c_min) = fl_u_neg_c; % Get Interpolant f_grid_interpolant_spln = griddedInterpolant(ar_interp_c_grid, ar_interp_u_of_c_grid, 'spline');
Iterate Value Function
Loop solution with 4 nested loops
- loop 1: over exogenous states
- loop 2: over endogenous states
- loop 3: over choices
- loop 4: add future utility, integration--loop over future shocks
% Start Profile if (bl_profile) close all; profile off; profile on; end % Start Timer if (bl_time) tic; end % Value Function Iteration while bl_vfi_continue
it_iter = it_iter + 1;
Interpolate (1) reacahble v(coh(k(w,z),b(w,z),z),z) given v(coh, z)
v(coh,z) solved on ar_interp_coh_grid, ar_z grids, see ffs_iwkz_get_funcgrid.m. Generate interpolant based on that, Then interpolate for the coh reachable levels given the k(w,z) percentage choice grids in the second stage of the problem
% Generate Interpolant for v(coh,z) f_grid_interpolant_value = griddedInterpolant(... mt_z_mesh_coh_interp_grid', mt_interp_coh_grid_mesh_z', mt_val_cur', 'linear'); % Interpoalte for v(coh(k(w,z),b(w,z),z),z) mt_val_wkb_interpolated = f_grid_interpolant_value(mt_z_mesh_coh_wkb, mt_coh_wkb);
Solve Second Stage Problem k*(w,z)
This is the key difference between this function and ffs_akz_set_functions which solves the two stages jointly Interpolation first, because solution coh grid is not the same as all points reachable by k and b choices given w.
[mt_ev_condi_z_max, ~, mt_ev_condi_z_max_kp, mt_ev_condi_z_max_bp] = ...
ff_wkz_evf(mt_val_wkb_interpolated, param_map, support_map, armt_map);
Solve First Stage Problem w*(z) given k*(w,z)
loop 1: over exogenous states
for it_z_i = 1:length(ar_z) % Get 2nd Stage Arrays ar_ev_condi_z_max_z = mt_ev_condi_z_max(:, it_z_i); ar_w_kstar_z = mt_ev_condi_z_max_kp(:, it_z_i); ar_w_astar_z = mt_ev_condi_z_max_bp(:, it_z_i); % loop 2: over endogenous states for it_coh_interp_j = 1:length(ar_interp_coh_grid) % Get cash-on-hand which include k,b,z fl_coh = mt_interp_coh_grid_mesh_z(it_coh_interp_j, it_z_i); % loop 3: over choices, only w vector % we choose w(z), know from ff_wkz_evf k*(w,z), b*=w-k* ar_val_cur = zeros(size(ar_w)); for it_cohp_k = 1:length(ar_w) fl_w_kstar_z = ar_w_kstar_z(it_cohp_k); fl_w_astar_z = ar_w_astar_z(it_cohp_k); % consumption fl_c = f_cons(fl_coh, fl_w_astar_z, fl_w_kstar_z); % loop 4: add future utility, integration already done in % ff_wkz_evf fl_ev_condi_z_max_z = ar_ev_condi_z_max_z(it_cohp_k); % Interpolate (2) ar_val_cur(it_cohp_k) = f_grid_interpolant_spln(fl_c) + fl_beta*fl_ev_condi_z_max_z; % Replace if negative consumption if fl_c <= 0 ar_val_cur(it_cohp_k) = fl_u_neg_c; end end % maximization over loop 3 choices for loop 1+2 states it_max_lin_idx = find(ar_val_cur == max(ar_val_cur)); mt_val(it_coh_interp_j,it_z_i) = ar_val_cur(it_max_lin_idx(1)); mt_pol_a(it_coh_interp_j,it_z_i) = ar_w_astar_z(it_max_lin_idx(1)); mt_pol_k(it_coh_interp_j,it_z_i) = ar_w_kstar_z(it_max_lin_idx(1)); end end
Check Tolerance and Continuation
% Difference across iterations ar_val_diff_norm(it_iter) = norm(mt_val - mt_val_cur); ar_pol_diff_norm(it_iter) = norm(mt_pol_a - mt_pol_a_cur) + norm(mt_pol_k - mt_pol_k_cur); ar_pol_a_perc_change = sum((mt_pol_a ~= mt_pol_a_cur))/length(ar_interp_coh_grid); ar_pol_k_perc_change = sum((mt_pol_k ~= mt_pol_k_cur))/length(ar_interp_coh_grid); mt_pol_perc_change(it_iter, :) = mean([ar_pol_a_perc_change;ar_pol_k_perc_change]); % Update mt_val_cur = mt_val; mt_pol_a_cur = mt_pol_a; mt_pol_k_cur = mt_pol_k; % Print Iteration Results if (bl_display && (rem(it_iter, it_display_every)==0)) fprintf('VAL it_iter:%d, fl_diff:%d, fl_diff_pol:%d\n', ... it_iter, ar_val_diff_norm(it_iter), ar_pol_diff_norm(it_iter)); tb_valpol_iter = array2table([mean(mt_val_cur,1);... mean(mt_pol_a_cur,1); ... mean(mt_pol_k_cur,1); ... mt_val_cur(length(ar_interp_coh_grid),:); ... mt_pol_a_cur(length(ar_interp_coh_grid),:); ... mt_pol_k_cur(length(ar_interp_coh_grid),:)]); tb_valpol_iter.Properties.VariableNames = strcat('z', string((1:size(mt_val_cur,2)))); tb_valpol_iter.Properties.RowNames = {'mval', 'map', 'mak', 'Hval', 'Hap', 'Hak'}; disp('mval = mean(mt_val_cur,1), average value over a') disp('map = mean(mt_pol_a_cur,1), average choice over a') disp('mkp = mean(mt_pol_k_cur,1), average choice over k') disp('Hval = mt_val_cur(ar_interp_coh_grid,:), highest a state val') disp('Hap = mt_pol_a_cur(ar_interp_coh_grid,:), highest a state choice') disp('mak = mt_pol_k_cur(ar_interp_coh_grid,:), highest k state choice') disp(tb_valpol_iter); end % Continuation Conditions: % 1. if value function convergence criteria reached % 2. if policy function variation over iterations is less than % threshold if (it_iter == (it_maxiter_val + 1)) bl_vfi_continue = false; elseif ((it_iter == it_maxiter_val) || ... (ar_val_diff_norm(it_iter) < fl_tol_val) || ... (sum(ar_pol_diff_norm(max(1, it_iter-it_tol_pol_nochange):it_iter)) < fl_tol_pol)) % Fix to max, run again to save results if needed it_iter_last = it_iter; it_iter = it_maxiter_val; end
VAL it_iter:5, fl_diff:6.912493e+01, fl_diff_pol:1.605054e+02 mval = mean(mt_val_cur,1), average value over a map = mean(mt_pol_a_cur,1), average choice over a mkp = mean(mt_pol_k_cur,1), average choice over k Hval = mt_val_cur(ar_interp_coh_grid,:), highest a state val Hap = mt_pol_a_cur(ar_interp_coh_grid,:), highest a state choice mak = mt_pol_k_cur(ar_interp_coh_grid,:), highest k state choice z1 z2 z3 z4 z5 z6 z7 z8 z9 z10 z11 z12 z13 z14 z15 ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ mval 4.8217 4.8457 4.8735 4.9049 4.9389 4.9757 5.0153 5.0577 5.103 5.1511 5.202 5.2557 5.3118 5.369 5.4241 map 19.765 19.722 18.712 18.648 17.651 17.552 16.585 15.619 14.178 12.931 11.309 9.7639 8.2944 6.3865 4.7176 mak 1.9815 1.9815 2.9373 2.9373 3.8643 3.8804 4.7535 5.6212 6.9542 8.0681 9.5412 10.932 12.225 13.95 15.426 Hval 6.3899 6.3947 6.4003 6.4069 6.4143 6.4227 6.4322 6.443 6.4555 6.4696 6.4853 6.5029 6.5223 6.5435 6.5647 Hap 41.837 41.837 40.816 40.816 39.796 39.796 38.776 36.735 34.694 33.673 31.633 29.592 26.531 23.469 20.408 Hak 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 5.102 6.1224 8.1633 9.1837 11.224 13.265 15.306 18.367 21.429
VAL it_iter:10, fl_diff:3.891496e+01, fl_diff_pol:5.107650e+01 mval = mean(mt_val_cur,1), average value over a map = mean(mt_pol_a_cur,1), average choice over a mkp = mean(mt_pol_k_cur,1), average choice over k Hval = mt_val_cur(ar_interp_coh_grid,:), highest a state val Hap = mt_pol_a_cur(ar_interp_coh_grid,:), highest a state choice mak = mt_pol_k_cur(ar_interp_coh_grid,:), highest k state choice z1 z2 z3 z4 z5 z6 z7 z8 z9 z10 z11 z12 z13 z14 z15 ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ mval 6.9451 7.0036 7.0704 7.1441 7.223 7.3073 7.397 7.4921 7.5928 7.6996 7.8125 7.9315 8.0554 8.1808 8.3003 map 22.26 22.226 21.22 21.166 20.171 20.022 19.122 18.152 16.792 15.435 13.855 12.229 10.682 8.6573 6.8446 mak 1.9851 1.9851 2.9409 2.9409 3.8732 3.9523 4.7805 5.6679 6.9291 8.1794 9.6508 11.151 12.557 14.438 16.102 Hval 9.7426 9.7591 9.7783 9.7999 9.8236 9.8497 9.8783 9.9097 9.9449 9.9838 10.026 10.072 10.122 10.175 10.226 Hap 46.939 46.939 45.918 45.918 44.898 44.898 43.878 42.857 39.796 38.776 36.735 34.694 32.653 29.592 26.531 Hak 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 5.102 6.1224 8.1633 9.1837 11.224 13.265 15.306 18.367 21.429
VAL it_iter:15, fl_diff:2.398535e+01, fl_diff_pol:2.715277e+01 mval = mean(mt_val_cur,1), average value over a map = mean(mt_pol_a_cur,1), average choice over a mkp = mean(mt_pol_k_cur,1), average choice over k Hval = mt_val_cur(ar_interp_coh_grid,:), highest a state val Hap = mt_pol_a_cur(ar_interp_coh_grid,:), highest a state choice mak = mt_pol_k_cur(ar_interp_coh_grid,:), highest k state choice z1 z2 z3 z4 z5 z6 z7 z8 z9 z10 z11 z12 z13 z14 z15 ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ mval 8.219 8.2971 8.3859 8.4834 8.5875 8.6989 8.8172 8.9426 9.0757 9.2171 9.3671 9.5253 9.69 9.8562 10.014 map 22.992 22.956 21.957 21.908 20.929 20.785 19.894 18.933 17.539 16.244 14.677 13.023 11.48 9.4406 7.592 mak 1.9869 1.9869 2.9445 2.9445 3.8786 3.9577 4.7895 5.6769 6.9937 8.2046 9.6759 11.226 12.649 14.573 16.298 Hval 11.742 11.769 11.799 11.833 11.87 11.91 11.954 12.002 12.054 12.111 12.173 12.241 12.313 12.389 12.464 Hap 47.959 47.959 46.939 46.939 45.918 45.918 44.898 43.878 41.837 40.816 38.776 36.735 34.694 31.633 27.551 Hak 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 5.102 6.1224 8.1633 9.1837 11.224 13.265 15.306 18.367 21.429
VAL it_iter:20, fl_diff:1.551092e+01, fl_diff_pol:1.773930e+01 mval = mean(mt_val_cur,1), average value over a map = mean(mt_pol_a_cur,1), average choice over a mkp = mean(mt_pol_k_cur,1), average choice over k Hval = mt_val_cur(ar_interp_coh_grid,:), highest a state val Hap = mt_pol_a_cur(ar_interp_coh_grid,:), highest a state choice mak = mt_pol_k_cur(ar_interp_coh_grid,:), highest k state choice z1 z2 z3 z4 z5 z6 z7 z8 z9 z10 z11 z12 z13 z14 z15 ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ mval 9.0537 9.1417 9.2419 9.3519 9.4693 9.5944 9.7274 9.8687 10.019 10.179 10.348 10.528 10.715 10.903 11.084 map 23.277 23.248 22.262 22.214 21.238 21.067 20.216 19.266 17.934 16.585 14.965 13.389 11.859 9.8017 7.9477 mak 1.9905 1.9905 2.9462 2.9462 3.8804 4.0008 4.7895 5.6787 6.9416 8.2118 9.7514 11.246 12.689 14.632 16.386 Hval 12.983 13.016 13.055 13.098 13.144 13.195 13.25 13.31 13.375 13.445 13.522 13.605 13.694 13.787 13.879 Hap 47.959 47.959 46.939 46.939 45.918 45.918 44.898 43.878 42.857 40.816 38.776 36.735 34.694 31.633 28.571 Hak 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 5.102 6.1224 7.1429 9.1837 11.224 13.265 15.306 18.367 21.429
VAL it_iter:25, fl_diff:1.032935e+01, fl_diff_pol:1.590158e+01 mval = mean(mt_val_cur,1), average value over a map = mean(mt_pol_a_cur,1), average choice over a mkp = mean(mt_pol_k_cur,1), average choice over k Hval = mt_val_cur(ar_interp_coh_grid,:), highest a state val Hap = mt_pol_a_cur(ar_interp_coh_grid,:), highest a state choice mak = mt_pol_k_cur(ar_interp_coh_grid,:), highest k state choice z1 z2 z3 z4 z5 z6 z7 z8 z9 z10 z11 z12 z13 z14 z15 ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ mval 9.6194 9.7122 9.8178 9.9338 10.058 10.19 10.33 10.48 10.639 10.809 10.989 11.18 11.379 11.581 11.773 map 23.424 23.396 22.399 22.368 21.382 21.182 20.379 19.424 18.06 16.768 15.171 13.578 12.045 9.9975 8.1112 mak 1.9905 1.9905 2.9462 2.948 3.8822 4.0421 4.7912 5.6787 6.9973 8.2154 9.7388 11.255 12.699 14.663 16.449 Hval 13.778 13.815 13.858 13.906 13.958 14.015 14.077 14.143 14.216 14.296 14.383 14.476 14.577 14.682 14.785 Hap 47.959 47.959 46.939 46.939 45.918 45.918 44.898 43.878 41.837 40.816 38.776 36.735 34.694 31.633 28.571 Hak 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 5.102 6.1224 8.1633 9.1837 11.224 13.265 15.306 18.367 21.429
VAL it_iter:30, fl_diff:7.030469e+00, fl_diff_pol:1.226954e+01 mval = mean(mt_val_cur,1), average value over a map = mean(mt_pol_a_cur,1), average choice over a mkp = mean(mt_pol_k_cur,1), average choice over k Hval = mt_val_cur(ar_interp_coh_grid,:), highest a state val Hap = mt_pol_a_cur(ar_interp_coh_grid,:), highest a state choice mak = mt_pol_k_cur(ar_interp_coh_grid,:), highest k state choice z1 z2 z3 z4 z5 z6 z7 z8 z9 z10 z11 z12 z13 z14 z15 ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ mval 10.01 10.105 10.213 10.332 10.46 10.595 10.74 10.894 11.058 11.233 11.419 11.616 11.822 12.03 12.23 map 23.493 23.469 22.476 22.438 21.466 21.276 20.462 19.517 18.161 16.867 15.258 13.705 12.15 10.096 8.2136 mak 1.9905 1.9923 2.948 2.948 3.884 4.0241 4.7948 5.6823 6.9794 8.2172 9.7621 11.239 12.707 14.672 16.465 Hval 14.298 14.338 14.384 14.435 14.49 14.551 14.617 14.689 14.767 14.853 14.945 15.046 15.153 15.265 15.375 Hap 47.959 47.959 46.939 46.939 45.918 45.918 44.898 43.878 41.837 40.816 38.776 36.735 34.694 31.633 28.571 Hak 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 5.102 6.1224 8.1633 9.1837 11.224 13.265 15.306 18.367 21.429
VAL it_iter:35, fl_diff:4.868514e+00, fl_diff_pol:7.346396e+00 mval = mean(mt_val_cur,1), average value over a map = mean(mt_pol_a_cur,1), average choice over a mkp = mean(mt_pol_k_cur,1), average choice over k Hval = mt_val_cur(ar_interp_coh_grid,:), highest a state val Hap = mt_pol_a_cur(ar_interp_coh_grid,:), highest a state choice mak = mt_pol_k_cur(ar_interp_coh_grid,:), highest k state choice z1 z2 z3 z4 z5 z6 z7 z8 z9 z10 z11 z12 z13 z14 z15 ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ mval 10.282 10.379 10.489 10.609 10.738 10.876 11.023 11.179 11.345 11.523 11.712 11.913 12.122 12.335 12.538 map 23.527 23.502 22.51 22.476 21.504 21.263 20.509 19.562 18.292 16.925 15.288 13.738 12.207 10.159 8.2585 mak 1.9923 1.9923 2.9498 2.9498 3.884 4.0798 4.7948 5.6823 6.9021 8.219 9.7819 11.259 12.708 14.676 16.497 Hval 14.64 14.682 14.73 14.783 14.841 14.905 14.974 15.049 15.131 15.22 15.317 15.421 15.533 15.65 15.764 Hap 47.959 47.959 46.939 46.939 45.918 45.918 44.898 43.878 42.857 40.816 38.776 36.735 34.694 31.633 28.571 Hak 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 5.102 6.1224 7.1429 9.1837 11.224 13.265 15.306 18.367 21.429
VAL it_iter:40, fl_diff:3.434801e+00, fl_diff_pol:6.905948e+00 mval = mean(mt_val_cur,1), average value over a map = mean(mt_pol_a_cur,1), average choice over a mkp = mean(mt_pol_k_cur,1), average choice over k Hval = mt_val_cur(ar_interp_coh_grid,:), highest a state val Hap = mt_pol_a_cur(ar_interp_coh_grid,:), highest a state choice mak = mt_pol_k_cur(ar_interp_coh_grid,:), highest k state choice z1 z2 z3 z4 z5 z6 z7 z8 z9 z10 z11 z12 z13 z14 z15 ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ mval 10.476 10.573 10.684 10.805 10.935 11.074 11.221 11.378 11.546 11.725 11.916 12.118 12.33 12.544 12.75 map 23.545 23.516 22.53 22.489 21.524 21.274 20.529 19.582 18.312 16.946 15.313 13.741 12.234 10.192 8.2729 mak 1.9923 1.9923 2.9498 2.9498 3.884 4.0906 4.7948 5.6823 6.9183 8.219 9.7819 11.278 12.71 14.679 16.519 Hval 14.869 14.912 14.96 15.015 15.075 15.14 15.211 15.289 15.373 15.464 15.563 15.67 15.785 15.904 16.021 Hap 47.959 47.959 46.939 46.939 45.918 44.898 44.898 43.878 42.857 40.816 38.776 36.735 34.694 31.633 28.571 Hak 2.0408 2.0408 3.0612 3.0612 4.0816 5.102 5.102 6.1224 7.1429 9.1837 11.224 13.265 15.306 18.367 21.429
VAL it_iter:45, fl_diff:2.456491e+00, fl_diff_pol:6.919109e+00 mval = mean(mt_val_cur,1), average value over a map = mean(mt_pol_a_cur,1), average choice over a mkp = mean(mt_pol_k_cur,1), average choice over k Hval = mt_val_cur(ar_interp_coh_grid,:), highest a state val Hap = mt_pol_a_cur(ar_interp_coh_grid,:), highest a state choice mak = mt_pol_k_cur(ar_interp_coh_grid,:), highest k state choice z1 z2 z3 z4 z5 z6 z7 z8 z9 z10 z11 z12 z13 z14 z15 ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ mval 10.616 10.713 10.824 10.946 11.076 11.215 11.363 11.521 11.689 11.869 12.06 12.263 12.476 12.691 12.898 map 23.552 23.523 22.537 22.501 21.533 21.303 20.538 19.596 18.321 16.955 15.328 13.758 12.25 10.204 8.2477 mak 1.9923 1.9923 2.9498 2.9498 3.884 4.0709 4.7948 5.6823 6.9183 8.219 9.7819 11.278 12.71 14.679 16.56 Hval 15.027 15.07 15.119 15.175 15.235 15.301 15.374 15.452 15.537 15.629 15.729 15.838 15.954 16.075 16.194 Hap 47.959 47.959 46.939 46.939 45.918 44.898 44.898 43.878 42.857 40.816 38.776 36.735 34.694 31.633 28.571 Hak 2.0408 2.0408 3.0612 3.0612 4.0816 5.102 5.102 6.1224 7.1429 9.1837 11.224 13.265 15.306 18.367 21.429
VAL it_iter:50, fl_diff:1.772770e+00, fl_diff_pol:1.020408e+00 mval = mean(mt_val_cur,1), average value over a map = mean(mt_pol_a_cur,1), average choice over a mkp = mean(mt_pol_k_cur,1), average choice over k Hval = mt_val_cur(ar_interp_coh_grid,:), highest a state val Hap = mt_pol_a_cur(ar_interp_coh_grid,:), highest a state choice mak = mt_pol_k_cur(ar_interp_coh_grid,:), highest k state choice z1 z2 z3 z4 z5 z6 z7 z8 z9 z10 z11 z12 z13 z14 z15 ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ mval 10.717 10.814 10.925 11.047 11.178 11.317 11.465 11.623 11.791 11.972 12.164 12.367 12.58 12.796 13.003 map 23.557 23.529 22.541 22.505 21.535 21.306 20.543 19.6 18.306 16.961 15.329 13.761 12.256 10.211 8.2549 mak 1.9923 1.9923 2.9498 2.9498 3.884 4.0709 4.7948 5.6823 6.9381 8.219 9.7819 11.278 12.71 14.679 16.56 Hval 15.138 15.181 15.231 15.287 15.348 15.414 15.487 15.566 15.651 15.744 15.845 15.954 16.071 16.193 16.312 Hap 47.959 47.959 46.939 46.939 45.918 44.898 44.898 43.878 42.857 40.816 38.776 36.735 34.694 31.633 28.571 Hak 2.0408 2.0408 3.0612 3.0612 4.0816 5.102 5.102 6.1224 7.1429 9.1837 11.224 13.265 15.306 18.367 21.429
VAL it_iter:55, fl_diff:1.286943e+00, fl_diff_pol:1.020408e+00 mval = mean(mt_val_cur,1), average value over a map = mean(mt_pol_a_cur,1), average choice over a mkp = mean(mt_pol_k_cur,1), average choice over k Hval = mt_val_cur(ar_interp_coh_grid,:), highest a state val Hap = mt_pol_a_cur(ar_interp_coh_grid,:), highest a state choice mak = mt_pol_k_cur(ar_interp_coh_grid,:), highest k state choice z1 z2 z3 z4 z5 z6 z7 z8 z9 z10 z11 z12 z13 z14 z15 ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ mval 10.79 10.888 10.999 11.121 11.251 11.391 11.539 11.697 11.866 12.046 12.238 12.442 12.655 12.871 13.079 map 23.557 23.53 22.546 22.506 21.538 21.312 20.543 19.602 18.288 16.964 15.331 13.765 12.259 10.217 8.2782 mak 1.9923 1.9923 2.9498 2.9498 3.884 4.0709 4.7948 5.6823 6.9578 8.219 9.7819 11.278 12.71 14.679 16.54 Hval 15.217 15.261 15.311 15.366 15.428 15.494 15.567 15.646 15.732 15.825 15.926 16.036 16.153 16.276 16.395 Hap 47.959 47.959 46.939 46.939 45.918 44.898 44.898 43.878 42.857 40.816 38.776 36.735 34.694 31.633 28.571 Hak 2.0408 2.0408 3.0612 3.0612 4.0816 5.102 5.102 6.1224 7.1429 9.1837 11.224 13.265 15.306 18.367 21.429
VAL it_iter:60, fl_diff:9.378474e-01, fl_diff_pol:0 mval = mean(mt_val_cur,1), average value over a map = mean(mt_pol_a_cur,1), average choice over a mkp = mean(mt_pol_k_cur,1), average choice over k Hval = mt_val_cur(ar_interp_coh_grid,:), highest a state val Hap = mt_pol_a_cur(ar_interp_coh_grid,:), highest a state choice mak = mt_pol_k_cur(ar_interp_coh_grid,:), highest k state choice z1 z2 z3 z4 z5 z6 z7 z8 z9 z10 z11 z12 z13 z14 z15 ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ mval 10.844 10.941 11.052 11.175 11.305 11.444 11.593 11.751 11.92 12.1 12.292 12.496 12.709 12.926 13.133 map 23.557 23.53 22.546 22.508 21.54 21.312 20.543 19.603 18.288 16.964 15.331 13.765 12.261 10.217 8.2782 mak 1.9923 1.9923 2.9498 2.9498 3.884 4.0709 4.7948 5.6823 6.9578 8.219 9.7819 11.278 12.71 14.679 16.54 Hval 15.274 15.318 15.368 15.424 15.485 15.552 15.625 15.704 15.789 15.883 15.984 16.094 16.212 16.334 16.454 Hap 47.959 47.959 46.939 46.939 45.918 44.898 44.898 43.878 42.857 40.816 38.776 36.735 34.694 31.633 28.571 Hak 2.0408 2.0408 3.0612 3.0612 4.0816 5.102 5.102 6.1224 7.1429 9.1837 11.224 13.265 15.306 18.367 21.429
VAL it_iter:65, fl_diff:6.851049e-01, fl_diff_pol:1.020408e+00 mval = mean(mt_val_cur,1), average value over a map = mean(mt_pol_a_cur,1), average choice over a mkp = mean(mt_pol_k_cur,1), average choice over k Hval = mt_val_cur(ar_interp_coh_grid,:), highest a state val Hap = mt_pol_a_cur(ar_interp_coh_grid,:), highest a state choice mak = mt_pol_k_cur(ar_interp_coh_grid,:), highest k state choice z1 z2 z3 z4 z5 z6 z7 z8 z9 z10 z11 z12 z13 z14 z15 ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ mval 10.883 10.98 11.092 11.214 11.344 11.484 11.632 11.79 11.959 12.139 12.332 12.536 12.749 12.965 13.173 map 23.557 23.53 22.546 22.508 21.54 21.312 20.543 19.603 18.288 16.966 15.331 13.765 12.261 10.218 8.2818 mak 1.9923 1.9923 2.9498 2.9498 3.884 4.0709 4.7948 5.6823 6.9578 8.219 9.7819 11.278 12.71 14.679 16.54 Hval 15.316 15.359 15.409 15.465 15.527 15.593 15.666 15.746 15.831 15.925 16.026 16.136 16.254 16.376 16.496 Hap 47.959 47.959 46.939 46.939 45.918 44.898 44.898 43.878 42.857 40.816 38.776 36.735 34.694 31.633 28.571 Hak 2.0408 2.0408 3.0612 3.0612 4.0816 5.102 5.102 6.1224 7.1429 9.1837 11.224 13.265 15.306 18.367 21.429
VAL it_iter:70, fl_diff:5.012569e-01, fl_diff_pol:0 mval = mean(mt_val_cur,1), average value over a map = mean(mt_pol_a_cur,1), average choice over a mkp = mean(mt_pol_k_cur,1), average choice over k Hval = mt_val_cur(ar_interp_coh_grid,:), highest a state val Hap = mt_pol_a_cur(ar_interp_coh_grid,:), highest a state choice mak = mt_pol_k_cur(ar_interp_coh_grid,:), highest k state choice z1 z2 z3 z4 z5 z6 z7 z8 z9 z10 z11 z12 z13 z14 z15 ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ mval 10.912 11.009 11.12 11.242 11.373 11.512 11.661 11.819 11.988 12.168 12.361 12.564 12.778 12.994 13.202 map 23.557 23.53 22.546 22.508 21.542 21.312 20.545 19.603 18.288 16.966 15.335 13.765 12.261 10.218 8.2818 mak 1.9923 1.9923 2.9498 2.9498 3.884 4.0709 4.7948 5.6823 6.9578 8.219 9.7819 11.278 12.71 14.679 16.54 Hval 15.346 15.389 15.44 15.496 15.557 15.624 15.697 15.776 15.862 15.955 16.056 16.166 16.284 16.407 16.527 Hap 47.959 47.959 46.939 46.939 45.918 44.898 44.898 43.878 42.857 40.816 38.776 36.735 34.694 31.633 28.571 Hak 2.0408 2.0408 3.0612 3.0612 4.0816 5.102 5.102 6.1224 7.1429 9.1837 11.224 13.265 15.306 18.367 21.429
VAL it_iter:75, fl_diff:3.671167e-01, fl_diff_pol:0 mval = mean(mt_val_cur,1), average value over a map = mean(mt_pol_a_cur,1), average choice over a mkp = mean(mt_pol_k_cur,1), average choice over k Hval = mt_val_cur(ar_interp_coh_grid,:), highest a state val Hap = mt_pol_a_cur(ar_interp_coh_grid,:), highest a state choice mak = mt_pol_k_cur(ar_interp_coh_grid,:), highest k state choice z1 z2 z3 z4 z5 z6 z7 z8 z9 z10 z11 z12 z13 z14 z15 ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ mval 10.933 11.03 11.141 11.264 11.394 11.533 11.682 11.84 12.009 12.189 12.382 12.586 12.799 13.015 13.223 map 23.557 23.53 22.546 22.508 21.542 21.312 20.545 19.603 18.288 16.966 15.337 13.765 12.261 10.218 8.2818 mak 1.9923 1.9923 2.9498 2.9498 3.884 4.0709 4.7948 5.6823 6.9578 8.219 9.7819 11.278 12.71 14.679 16.54 Hval 15.368 15.412 15.462 15.518 15.579 15.646 15.719 15.798 15.884 15.977 16.078 16.189 16.307 16.429 16.549 Hap 47.959 47.959 46.939 46.939 45.918 44.898 44.898 43.878 42.857 40.816 38.776 36.735 34.694 31.633 28.571 Hak 2.0408 2.0408 3.0612 3.0612 4.0816 5.102 5.102 6.1224 7.1429 9.1837 11.224 13.265 15.306 18.367 21.429
VAL it_iter:80, fl_diff:2.690540e-01, fl_diff_pol:0 mval = mean(mt_val_cur,1), average value over a map = mean(mt_pol_a_cur,1), average choice over a mkp = mean(mt_pol_k_cur,1), average choice over k Hval = mt_val_cur(ar_interp_coh_grid,:), highest a state val Hap = mt_pol_a_cur(ar_interp_coh_grid,:), highest a state choice mak = mt_pol_k_cur(ar_interp_coh_grid,:), highest k state choice z1 z2 z3 z4 z5 z6 z7 z8 z9 z10 z11 z12 z13 z14 z15 ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ mval 10.948 11.046 11.157 11.279 11.41 11.549 11.697 11.856 12.024 12.205 12.397 12.601 12.814 13.031 13.238 map 23.557 23.53 22.546 22.508 21.542 21.312 20.545 19.603 18.288 16.966 15.337 13.765 12.261 10.218 8.2818 mak 1.9923 1.9923 2.9498 2.9498 3.884 4.0709 4.7948 5.6823 6.9578 8.219 9.7819 11.278 12.71 14.679 16.54 Hval 15.384 15.428 15.478 15.534 15.595 15.662 15.735 15.814 15.9 15.993 16.095 16.205 16.323 16.445 16.565 Hap 47.959 47.959 46.939 46.939 45.918 44.898 44.898 43.878 42.857 40.816 38.776 36.735 34.694 31.633 28.571 Hak 2.0408 2.0408 3.0612 3.0612 4.0816 5.102 5.102 6.1224 7.1429 9.1837 11.224 13.265 15.306 18.367 21.429
VAL it_iter:85, fl_diff:1.972736e-01, fl_diff_pol:0 mval = mean(mt_val_cur,1), average value over a map = mean(mt_pol_a_cur,1), average choice over a mkp = mean(mt_pol_k_cur,1), average choice over k Hval = mt_val_cur(ar_interp_coh_grid,:), highest a state val Hap = mt_pol_a_cur(ar_interp_coh_grid,:), highest a state choice mak = mt_pol_k_cur(ar_interp_coh_grid,:), highest k state choice z1 z2 z3 z4 z5 z6 z7 z8 z9 z10 z11 z12 z13 z14 z15 ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ mval 10.959 11.057 11.168 11.29 11.421 11.56 11.709 11.867 12.036 12.216 12.408 12.612 12.825 13.042 13.25 map 23.557 23.53 22.546 22.51 21.542 21.312 20.545 19.603 18.288 16.966 15.337 13.765 12.261 10.218 8.2818 mak 1.9923 1.9923 2.9498 2.9498 3.884 4.0709 4.7948 5.6823 6.9578 8.219 9.7819 11.278 12.71 14.679 16.54 Hval 15.396 15.439 15.49 15.546 15.607 15.674 15.747 15.826 15.912 16.005 16.106 16.217 16.335 16.457 16.577 Hap 47.959 47.959 46.939 46.939 45.918 44.898 44.898 43.878 42.857 40.816 38.776 36.735 34.694 31.633 28.571 Hak 2.0408 2.0408 3.0612 3.0612 4.0816 5.102 5.102 6.1224 7.1429 9.1837 11.224 13.265 15.306 18.367 21.429
VAL it_iter:90, fl_diff:1.446869e-01, fl_diff_pol:0 mval = mean(mt_val_cur,1), average value over a map = mean(mt_pol_a_cur,1), average choice over a mkp = mean(mt_pol_k_cur,1), average choice over k Hval = mt_val_cur(ar_interp_coh_grid,:), highest a state val Hap = mt_pol_a_cur(ar_interp_coh_grid,:), highest a state choice mak = mt_pol_k_cur(ar_interp_coh_grid,:), highest k state choice z1 z2 z3 z4 z5 z6 z7 z8 z9 z10 z11 z12 z13 z14 z15 ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ mval 10.968 11.065 11.176 11.299 11.429 11.568 11.717 11.875 12.044 12.224 12.417 12.621 12.834 13.05 13.258 map 23.557 23.53 22.546 22.51 21.542 21.312 20.545 19.603 18.288 16.966 15.337 13.765 12.261 10.218 8.2818 mak 1.9923 1.9923 2.9498 2.9498 3.884 4.0709 4.7948 5.6823 6.9578 8.219 9.7819 11.278 12.71 14.679 16.54 Hval 15.405 15.448 15.498 15.554 15.616 15.682 15.755 15.835 15.92 16.014 16.115 16.225 16.343 16.466 16.586 Hap 47.959 47.959 46.939 46.939 45.918 44.898 44.898 43.878 42.857 40.816 38.776 36.735 34.694 31.633 28.571 Hak 2.0408 2.0408 3.0612 3.0612 4.0816 5.102 5.102 6.1224 7.1429 9.1837 11.224 13.265 15.306 18.367 21.429
VAL it_iter:95, fl_diff:1.061396e-01, fl_diff_pol:0 mval = mean(mt_val_cur,1), average value over a map = mean(mt_pol_a_cur,1), average choice over a mkp = mean(mt_pol_k_cur,1), average choice over k Hval = mt_val_cur(ar_interp_coh_grid,:), highest a state val Hap = mt_pol_a_cur(ar_interp_coh_grid,:), highest a state choice mak = mt_pol_k_cur(ar_interp_coh_grid,:), highest k state choice z1 z2 z3 z4 z5 z6 z7 z8 z9 z10 z11 z12 z13 z14 z15 ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ mval 10.974 11.071 11.182 11.305 11.435 11.575 11.723 11.881 12.05 12.23 12.423 12.627 12.84 13.057 13.264 map 23.557 23.53 22.546 22.51 21.542 21.312 20.545 19.603 18.288 16.966 15.337 13.765 12.261 10.218 8.2818 mak 1.9923 1.9923 2.9498 2.9498 3.884 4.0709 4.7948 5.6823 6.9578 8.219 9.7819 11.278 12.71 14.679 16.54 Hval 15.411 15.454 15.505 15.561 15.622 15.689 15.762 15.841 15.927 16.02 16.121 16.232 16.35 16.472 16.592 Hap 47.959 47.959 46.939 46.939 45.918 44.898 44.898 43.878 42.857 40.816 38.776 36.735 34.694 31.633 28.571 Hak 2.0408 2.0408 3.0612 3.0612 4.0816 5.102 5.102 6.1224 7.1429 9.1837 11.224 13.265 15.306 18.367 21.429
VAL it_iter:100, fl_diff:7.787268e-02, fl_diff_pol:0 mval = mean(mt_val_cur,1), average value over a map = mean(mt_pol_a_cur,1), average choice over a mkp = mean(mt_pol_k_cur,1), average choice over k Hval = mt_val_cur(ar_interp_coh_grid,:), highest a state val Hap = mt_pol_a_cur(ar_interp_coh_grid,:), highest a state choice mak = mt_pol_k_cur(ar_interp_coh_grid,:), highest k state choice z1 z2 z3 z4 z5 z6 z7 z8 z9 z10 z11 z12 z13 z14 z15 ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ mval 10.978 11.076 11.187 11.309 11.44 11.579 11.728 11.886 12.054 12.235 12.427 12.631 12.844 13.061 13.269 map 23.557 23.53 22.546 22.51 21.542 21.312 20.545 19.603 18.288 16.966 15.337 13.765 12.261 10.218 8.2818 mak 1.9923 1.9923 2.9498 2.9498 3.884 4.0709 4.7948 5.6823 6.9578 8.219 9.7819 11.278 12.71 14.679 16.54 Hval 15.416 15.459 15.509 15.565 15.627 15.693 15.766 15.846 15.931 16.025 16.126 16.236 16.354 16.477 16.597 Hap 47.959 47.959 46.939 46.939 45.918 44.898 44.898 43.878 42.857 40.816 38.776 36.735 34.694 31.633 28.571 Hak 2.0408 2.0408 3.0612 3.0612 4.0816 5.102 5.102 6.1224 7.1429 9.1837 11.224 13.265 15.306 18.367 21.429
VAL it_iter:105, fl_diff:5.713910e-02, fl_diff_pol:0 mval = mean(mt_val_cur,1), average value over a map = mean(mt_pol_a_cur,1), average choice over a mkp = mean(mt_pol_k_cur,1), average choice over k Hval = mt_val_cur(ar_interp_coh_grid,:), highest a state val Hap = mt_pol_a_cur(ar_interp_coh_grid,:), highest a state choice mak = mt_pol_k_cur(ar_interp_coh_grid,:), highest k state choice z1 z2 z3 z4 z5 z6 z7 z8 z9 z10 z11 z12 z13 z14 z15 ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ mval 10.981 11.079 11.19 11.312 11.443 11.582 11.731 11.889 12.058 12.238 12.43 12.634 12.848 13.064 13.272 map 23.557 23.53 22.546 22.51 21.542 21.312 20.545 19.603 18.288 16.966 15.337 13.765 12.261 10.218 8.2818 mak 1.9923 1.9923 2.9498 2.9498 3.884 4.0709 4.7948 5.6823 6.9578 8.219 9.7819 11.278 12.71 14.679 16.54 Hval 15.419 15.462 15.513 15.569 15.63 15.697 15.77 15.849 15.935 16.028 16.129 16.24 16.358 16.48 16.6 Hap 47.959 47.959 46.939 46.939 45.918 44.898 44.898 43.878 42.857 40.816 38.776 36.735 34.694 31.633 28.571 Hak 2.0408 2.0408 3.0612 3.0612 4.0816 5.102 5.102 6.1224 7.1429 9.1837 11.224 13.265 15.306 18.367 21.429
VAL it_iter:110, fl_diff:4.192851e-02, fl_diff_pol:0 mval = mean(mt_val_cur,1), average value over a map = mean(mt_pol_a_cur,1), average choice over a mkp = mean(mt_pol_k_cur,1), average choice over k Hval = mt_val_cur(ar_interp_coh_grid,:), highest a state val Hap = mt_pol_a_cur(ar_interp_coh_grid,:), highest a state choice mak = mt_pol_k_cur(ar_interp_coh_grid,:), highest k state choice z1 z2 z3 z4 z5 z6 z7 z8 z9 z10 z11 z12 z13 z14 z15 ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ ______ mval 10.984 11.081 11.193 11.315 11.445 11.585 11.733 11.891 12.06 12.241 12.433 12.637 12.85 13.067 13.274 map 23.557 23.53 22.546 22.51 21.542 21.312 20.545 19.603 18.288 16.966 15.337 13.765 12.261 10.218 8.2818 mak 1.9923 1.9923 2.9498 2.9498 3.884 4.0709 4.7948 5.6823 6.9578 8.219 9.7819 11.278 12.71 14.679 16.54 Hval 15.422 15.465 15.515 15.571 15.632 15.699 15.772 15.851 15.937 16.031 16.132 16.242 16.36 16.483 16.602 Hap 47.959 47.959 46.939 46.939 45.918 44.898 44.898 43.878 42.857 40.816 38.776 36.735 34.694 31.633 28.571 Hak 2.0408 2.0408 3.0612 3.0612 4.0816 5.102 5.102 6.1224 7.1429 9.1837 11.224 13.265 15.306 18.367 21.429
end % End Timer if (bl_time) toc; end % End Profile if (bl_profile) profile off profile viewer st_file_name = [st_profile_prefix st_profile_name_main st_profile_suffix]; profsave(profile('info'), strcat(st_profile_path, st_file_name)); end
Elapsed time is 388.127908 seconds.
Process Optimal Choices
result_map = containers.Map('KeyType','char', 'ValueType','any'); result_map('mt_val') = mt_val; result_map('cl_mt_coh') = {mt_interp_coh_grid_mesh_z, zeros(1)}; result_map('cl_mt_pol_a') = {mt_pol_a, zeros(1)}; result_map('cl_mt_pol_k') = {mt_pol_k, zeros(1)}; result_map('cl_mt_pol_c') = {f_cons(mt_interp_coh_grid_mesh_z, mt_pol_a, mt_pol_k), zeros(1)}; result_map('ar_st_pol_names') = ["cl_mt_coh", "cl_mt_pol_a", "cl_mt_pol_k", "cl_mt_pol_c"]; if (bl_post) bl_input_override = true; result_map('ar_val_diff_norm') = ar_val_diff_norm(1:it_iter_last); result_map('ar_pol_diff_norm') = ar_pol_diff_norm(1:it_iter_last); result_map('mt_pol_perc_change') = mt_pol_perc_change(1:it_iter_last, :); % graphing based on coh_wkb, but that does not match optimal choice % matrixes for graphs. armt_map('mt_coh_wkb') = mt_interp_coh_grid_mesh_z; armt_map('it_ameshk_n') = length(ar_interp_coh_grid); armt_map('ar_a_meshk') = mt_interp_coh_grid_mesh_z(:,1); armt_map('ar_k_mesha') = zeros(size(mt_interp_coh_grid_mesh_z(:,1)) + 0); result_map = ff_akz_vf_post(param_map, support_map, armt_map, func_map, result_map, bl_input_override); end
valgap = norm(mt_val - mt_val_cur): value function difference across iterations polgap = norm(mt_pol_a - mt_pol_a_cur): policy function difference across iterations z1 = z1 perc change: (sum((mt_pol_a ~= mt_pol_a_cur))+sum((mt_pol_k ~= mt_pol_k_cur)))/(2*it_ameshk_n):percentage of state space points conditional on shock where the policy function is changing across iterations valgap polgap z1 z2 z3 z4 z5 z6 z7 z8 z9 z10 z11 z12 z13 z14 z15 ________ ______ __________ __________ __________ __________ __________ __________ __________ __________ __________ __________ __________ __________ __________ __________ __________ iter=1 142.95 184.61 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 iter=2 111 1643.2 0.9419 0.94102 0.92165 0.92077 0.90141 0.90053 0.88028 0.86092 0.83979 0.80106 0.76144 0.72095 0.67958 0.6206 0.55986 iter=3 92.611 546.99 0.64789 0.64789 0.51408 0.5132 0.58099 0.59067 0.62764 0.57746 0.75088 0.4912 0.61444 0.5088 0.5713 0.53169 0.54577 iter=4 79.394 268.49 0.53081 0.52993 0.45951 0.45599 0.55018 0.56162 0.51849 0.47535 0.65669 0.43662 0.53609 0.44982 0.49472 0.45246 0.45775 iter=5 69.125 160.51 0.44366 0.44366 0.41285 0.41461 0.46655 0.50352 0.47447 0.42342 0.60915 0.40053 0.45335 0.41109 0.42254 0.40141 0.40493 iter=6 60.852 109.05 0.37236 0.37236 0.35035 0.34859 0.38116 0.41901 0.42958 0.36092 0.60827 0.35035 0.41549 0.38732 0.36708 0.35211 0.35387 iter=7 53.985 81.824 0.30722 0.31074 0.28697 0.28961 0.31866 0.35387 0.35387 0.29754 0.54754 0.29489 0.37324 0.33451 0.31162 0.3037 0.30546 iter=8 48.182 68.124 0.22535 0.22623 0.22711 0.22623 0.2368 0.3125 0.26496 0.23415 0.56074 0.22711 0.33451 0.30458 0.24824 0.23944 0.25 iter=9 43.211 58.243 0.1787 0.17518 0.16549 0.17077 0.18134 0.25 0.20951 0.17958 0.58099 0.1787 0.29137 0.26496 0.1919 0.1919 0.19454 iter=10 38.915 51.077 0.13116 0.13908 0.13468 0.13204 0.14085 0.22623 0.15669 0.13996 0.57394 0.13644 0.2632 0.21743 0.15405 0.15581 0.15317 iter=11 35.131 43.68 0.11004 0.10211 0.10299 0.10211 0.11092 0.18486 0.125 0.10827 0.48151 0.11884 0.17342 0.20951 0.12324 0.12148 0.12588 iter=12 31.821 37.193 0.089789 0.091549 0.083627 0.086268 0.089789 0.14085 0.10387 0.088028 0.38468 0.089789 0.23944 0.13996 0.10299 0.10123 0.10739 iter=13 28.888 34.388 0.066901 0.069542 0.070423 0.071303 0.078345 0.17254 0.088028 0.080106 0.3662 0.080106 0.14789 0.16901 0.088908 0.088028 0.091549 iter=14 26.295 28.888 0.057218 0.060739 0.057218 0.058979 0.065141 0.098592 0.071303 0.06338 0.28873 0.06338 0.18046 0.10035 0.070423 0.078345 0.074824 iter=15 23.985 27.153 0.051937 0.048415 0.049296 0.048415 0.057218 0.1294 0.0625 0.055458 0.28609 0.056338 0.15845 0.12324 0.060739 0.060739 0.0625 iter=16 21.911 25.382 0.040493 0.044014 0.037852 0.044014 0.042254 0.10211 0.050176 0.046655 0.24384 0.045775 0.15229 0.11444 0.055458 0.055458 0.058099 iter=17 20.057 22.86 0.034331 0.034331 0.03257 0.03081 0.036092 0.18662 0.039613 0.039613 0.14877 0.041373 0.084507 0.070423 0.046655 0.045775 0.048415 iter=18 18.39 24.561 0.023768 0.02993 0.034331 0.02993 0.026408 0.1963 0.035211 0.02993 0.19806 0.03169 0.055458 0.06338 0.044894 0.036972 0.043134 iter=19 16.881 20.709 0.028169 0.027289 0.019366 0.026408 0.03257 0.125 0.03257 0.02993 0.18134 0.03169 0.09507 0.080106 0.029049 0.034331 0.037852 iter=20 15.511 17.739 0.020246 0.017606 0.027289 0.019366 0.025528 0.13204 0.025528 0.022007 0.11532 0.020246 0.021127 0.044894 0.035211 0.033451 0.02993 iter=21 14.266 18.981 0.022887 0.022007 0.015845 0.020246 0.014965 0.040493 0.03081 0.024648 0.15669 0.02993 0.072183 0.068662 0.024648 0.028169 0.03081 iter=22 13.136 15.744 0.018486 0.0079225 0.019366 0.013204 0.025528 0.049296 0.019366 0.019366 0.10475 0.013204 0.033451 0.022007 0.023768 0.024648 0.021127 iter=23 12.111 14.682 0.010563 0.015845 0.012324 0.013204 0.013204 0.090669 0.010563 0.014965 0.084507 0.026408 0.086268 0.029049 0.021127 0.014085 0.042254 iter=24 11.179 11.435 0.014085 0.018486 0.0088028 0.019366 0.0096831 0.049296 0.026408 0.014085 0.006162 0.0044014 0.027289 0.012324 0.011444 0.022887 0.041373 iter=25 10.329 15.902 0.010563 0.010563 0.010563 0.011444 0.011444 0.10651 0.0088028 0.006162 0.09507 0.017606 0.022007 0.018486 0.018486 0.021127 0.03257 iter=26 9.5518 11.088 0.0044014 0.011444 0.0096831 0.0079225 0.010563 0.012324 0.0052817 0.015845 0.052817 0.011444 0.051937 0.015845 0.014085 0.006162 0.035211 iter=27 8.8393 11.008 0.006162 0.006162 0.012324 0.0035211 0.013204 0.040493 0.019366 0.0079225 0.050176 0.011444 0.010563 0.0096831 0.010563 0.021127 0.048415 iter=28 8.1848 14.714 0.015845 0.0052817 0.0052817 0.014085 0.0017606 0.052817 0.006162 0.013204 0.081866 0.0079225 0.029049 0.03257 0.0088028 0.0079225 0.034331 iter=29 7.5831 10.375 0.0044014 0.010563 0.0035211 0.0052817 0.0088028 0.039613 0.0079225 0.0052817 0.0088028 0.0088028 0.014085 0.012324 0.0079225 0.0079225 0.045775 iter=30 7.0305 12.27 0.0035211 0.0052817 0.0079225 0.0035211 0.010563 0.023768 0.0088028 0.0052817 0.066021 0.0096831 0.012324 0.014085 0.014085 0.0096831 0.006162 iter=31 6.5222 10.397 0.006162 0.0044014 0.0044014 0.0044014 0.0035211 0.044894 0.0070423 0.006162 0.0070423 0.0079225 0.028169 0.0079225 0.0079225 0.0079225 0.029049 iter=32 6.055 12.198 0.0026408 0.0035211 0.0044014 0.006162 0.006162 0.060739 0.0044014 0.0052817 0.027289 0.0079225 0.0035211 0.0052817 0.0088028 0.0070423 0.027289 iter=33 5.6257 12.33 0.0052817 0.0026408 0.0035211 0.0017606 0.0035211 0.0052817 0.0096831 0.0044014 0.064261 0.0052817 0.006162 0.026408 0.0052817 0.006162 0.006162 iter=34 5.2314 11.84 0.0026408 0.0026408 0.0026408 0.0035211 0.0044014 0.058979 0.0044014 0.0035211 0.044894 0.0044014 0.0052817 0.0052817 0.0052817 0.0088028 0.024648 iter=35 4.8685 7.3464 0.0017606 0.0026408 0.0026408 0.0035211 0.00088028 0.023768 0.0017606 0.0026408 0.006162 0.0035211 0.0035211 0.0044014 0.0026408 0.0026408 0.006162 iter=36 4.5344 10.117 0.0017606 0.0017606 0.0035211 0.0026408 0.0052817 0.021127 0.0026408 0.0044014 0.044014 0.00088028 0.0035211 0.0026408 0.0035211 0.0035211 0.0035211 iter=37 4.226 10.514 0.0026408 0.0035211 0.0017606 0.00088028 0.0017606 0.046655 0.0026408 0.00088028 0.022887 0.0044014 0.0035211 0.006162 0.0052817 0.006162 0.021127 iter=38 3.9414 7.4757 0 0.0017606 0.0026408 0.0017606 0.00088028 0.0017606 0.00088028 0.0026408 0.006162 0.0017606 0.0035211 0.0035211 0.0017606 0.0044014 0.023768 iter=39 3.6783 7.0696 0.0026408 0.00088028 0.0017606 0.00088028 0.00088028 0.0017606 0.0017606 0.00088028 0.0035211 0.0026408 0.00088028 0.020246 0.0026408 0.00088028 0.021127 iter=40 3.4348 6.9059 0.0017606 0 0 0 0.0017606 0.021127 0.0017606 0.00088028 0.0026408 0.00088028 0.0017606 0.00088028 0.00088028 0.0026408 0.0017606 iter=41 3.2092 2.0408 0 0.00088028 0.0017606 0.0026408 0 0.00088028 0.00088028 0.0035211 0.00088028 0.00088028 0.0017606 0.0035211 0.0026408 0.00088028 0.00088028 iter=42 2.9998 1.7674 0.00088028 0.0017606 0.00088028 0.00088028 0.0026408 0.00088028 0.00088028 0 0.00088028 0.00088028 0.00088028 0.0026408 0.0017606 0.00088028 0.0017606 iter=43 2.8053 7.3746 0.0017606 0 0 0.00088028 0.00088028 0.021127 0.0026408 0 0.0026408 0 0.00088028 0.0017606 0.0017606 0.0017606 0.020246 iter=44 2.6246 3.9426 0 0.00088028 0.00088028 0.00088028 0 0 0.00088028 0.0026408 0 0.0017606 0.0017606 0 0.0026408 0.0017606 0.0044014 iter=45 2.4565 6.9191 0.00088028 0 0 0.00088028 0.00088028 0.00088028 0 0.00088028 0.00088028 0.00088028 0.0017606 0.0026408 0 0.00088028 0.020246 iter=46 2.2999 6.7686 0 0 0.00088028 0.00088028 0 0 0 0 0.019366 0.00088028 0 0.00088028 0 0.00088028 0.00088028 iter=47 2.1541 1.7674 0 0.00088028 0.00088028 0 0 0.00088028 0 0.00088028 0 0.0017606 0 0 0.0026408 0.0017606 0.0017606 iter=48 2.0181 1.4431 0.0017606 0.00088028 0 0 0.00088028 0 0 0 0.00088028 0 0 0 0 0 0 iter=49 1.8912 1.4431 0 0.00088028 0 0 0 0 0.0017606 0.00088028 0.00088028 0 0.00088028 0 0 0 0 iter=50 1.7728 1.0204 0.00088028 0 0 0.00088028 0 0.00088028 0.00088028 0 0.00088028 0 0 0.0017606 0 0.00088028 0.00088028 iter=61 0.88062 1.0204 0 0 0 0 0 0 0 0 0 0.00088028 0 0 0 0 0.00088028 iter=62 0.82695 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=63 0.77662 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=64 0.7294 1.0204 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0.00088028 iter=65 0.6851 1.0204 0 0 0 0 0 0 0 0 0 0 0 0 0 0.00088028 0 iter=66 0.64354 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=67 0.60452 1.0204 0 0 0 0 0 0 0 0 0 0 0.0017606 0 0 0 0 iter=68 0.5679 1.0204 0 0 0 0 0.00088028 0 0 0 0 0 0.00088028 0 0 0 0 iter=69 0.53353 1.0204 0 0 0 0 0 0 0.00088028 0 0 0 0 0 0 0 0 iter=70 0.50126 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=71 0.47096 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=72 0.4425 1.0204 0 0 0 0 0 0 0 0 0 0 0.00088028 0 0 0 0 iter=73 0.41578 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=74 0.39069 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=75 0.36712 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=76 0.34498 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=77 0.32418 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=78 0.30465 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=79 0.2863 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=80 0.26905 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=81 0.25286 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=82 0.23764 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=83 0.22334 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=84 0.2099 1.0204 0 0 0 0.00088028 0 0 0 0 0 0 0 0 0 0 0 iter=85 0.19727 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=86 0.18541 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=87 0.17426 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=88 0.16378 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=89 0.15394 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=90 0.14469 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=91 0.13599 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=92 0.12782 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=93 0.12014 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=94 0.11292 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=95 0.10614 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=96 0.099764 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=97 0.093772 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=98 0.088141 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=99 0.082848 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=100 0.077873 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=101 0.073197 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=102 0.068802 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=103 0.064671 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=104 0.060788 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=105 0.057139 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=106 0.053709 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=107 0.050485 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=108 0.047454 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=109 0.044606 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=110 0.041929 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 tb_val: V(a,z) value at each state space point z1_0_34741 z2_0_40076 z3_0_4623 z4_0_5333 z5_0_61519 z6_0_70966 z10_1_2567 z11_1_4496 z12_1_6723 z13_1_9291 z14_2_2253 z15_2_567 __________ __________ _________ _________ __________ __________ __________ __________ __________ __________ __________ _________ coh1:k=0.443648,b=0 -16.694 -16.694 -16.694 -16.694 -16.694 -16.694 -16.694 -16.694 -16.694 -16.694 -16.694 -16.694 coh2:k=0.543883,b=0 -16.403 -16.403 -16.403 -16.403 -16.403 -16.403 -16.403 -16.403 -16.403 -16.403 -16.403 -16.403 coh3:k=0.644119,b=0 -16.183 -16.183 -16.183 -16.183 -16.183 -16.183 -16.183 -16.183 -16.183 -16.183 -16.183 -16.183 coh4:k=0.744354,b=0 -16.01 -16.01 -16.01 -16.01 -16.01 -16.01 -16.01 -16.01 -16.01 -16.01 -16.01 -16.01 coh5:k=0.84459,b=0 -15.868 -15.868 -15.868 -15.868 -15.868 -15.868 -15.868 -15.868 -15.868 -15.868 -15.868 -15.868 coh6:k=0.944825,b=0 -15.749 -15.749 -15.749 -15.749 -15.749 -15.749 -15.749 -15.749 -15.749 -15.749 -15.749 -15.749 coh7:k=1.04506,b=0 -8.132 -7.8785 -7.5987 -7.3037 -6.9978 -6.6804 -5.1835 -4.729 -4.2584 -3.792 -3.3506 -2.9567 coh8:k=1.1453,b=0 -1.0535 -0.80001 -0.52015 -0.22515 0.080782 0.3981 1.895 2.3495 2.8201 3.2865 3.7279 4.1219 coh9:k=1.24553,b=0 0.39069 0.64416 0.92402 1.219 1.525 1.8423 3.3392 3.7937 4.2643 4.7307 5.1721 5.566 coh10:k=1.34577,b=0 1.0996 1.3531 1.6329 1.9279 2.2339 2.5512 4.0481 4.5026 4.9732 5.4396 5.881 6.2749 coh11:k=1.446,b=0 1.5402 1.7937 2.0735 2.3685 2.6745 2.9918 4.4887 4.9432 5.4138 5.8802 6.3216 6.7155 coh12:k=1.54624,b=0 1.8478 2.1013 2.3812 2.6762 2.9821 3.2994 4.7963 5.2508 5.7214 6.1878 6.6292 7.0232 coh13:k=1.64647,b=0 2.0782 2.3317 2.6116 2.9066 3.2125 3.5298 5.0267 5.4812 5.9518 6.4182 6.8596 7.2536 coh14:k=1.74671,b=0 2.2591 2.5126 2.7925 3.0875 3.3934 3.7107 5.2076 5.6621 6.1327 6.5991 7.0405 7.4345 coh15:k=1.84694,b=0 2.406 2.6595 2.9394 3.2344 3.5403 3.8576 5.3545 5.809 6.2796 6.746 7.1874 7.5814 coh16:k=1.94718,b=0 2.5284 2.7819 3.0617 3.3567 3.6627 3.98 5.4769 5.9314 6.402 6.8684 7.3098 7.7037 coh17:k=2.04742,b=0 2.6324 2.8858 3.1657 3.4607 3.7666 4.084 5.5809 6.0353 6.506 6.9723 7.4138 7.8077 coh18:k=2.14765,b=0 2.7222 2.9756 3.2555 3.5505 3.8564 4.1737 5.6707 6.1251 6.5958 7.0621 7.5036 7.8975 coh19:k=2.24789,b=0 2.8007 3.0542 3.334 3.629 3.935 4.2523 5.7492 6.2037 6.6743 7.1407 7.5821 7.9761 coh20:k=2.34812,b=0 2.8702 3.1237 3.4035 3.6985 4.0045 4.3218 5.8187 6.2732 6.7438 7.2102 7.6516 8.0455 coh21:k=2.44836,b=0 2.9322 3.1857 3.4656 3.7605 4.0665 4.3838 5.8807 6.3352 6.8058 7.2722 7.7136 8.1076 coh22:k=2.54859,b=0 3.0218 3.2936 3.5894 3.8956 4.2058 4.5183 5.9365 6.391 6.8616 7.328 7.7694 8.1634 coh23:k=2.64883,b=0 3.2636 3.5354 3.8312 4.1373 4.4476 4.7601 6.0819 6.4545 6.9122 7.3786 7.82 8.214 coh24:k=2.74906,b=0 3.452 3.7238 4.0196 4.3258 4.636 4.9485 6.2704 6.6429 7.0323 7.4265 7.8661 8.2601 coh25:k=2.8493,b=0 3.6042 3.876 4.1718 4.478 4.7882 5.1007 6.4225 6.7951 7.1845 7.5787 7.9616 8.3122 coh26:k=2.94953,b=0 3.7305 4.0022 4.2981 4.6042 4.9144 5.227 6.5488 6.9214 7.3107 7.705 8.0879 8.4384 coh27:k=3.04977,b=0 3.8374 4.1092 4.405 4.7112 5.0214 5.3339 6.6557 7.0283 7.4177 7.8119 8.1948 8.5454 coh28:k=3.15001,b=0 3.9295 4.2013 4.4971 4.8033 5.1135 5.426 6.7478 7.1204 7.5098 7.904 8.2869 8.6374 coh29:k=3.25024,b=0 4.0099 4.2817 4.5775 4.8836 5.1939 5.5064 6.8282 7.2008 7.5902 7.9844 8.3673 8.7178 coh30:k=3.35048,b=0 4.0809 4.3527 4.6485 4.9546 5.2648 5.5774 6.8992 7.2718 7.6611 8.0554 8.4383 8.7888 coh31:k=3.45071,b=0 4.1442 4.4159 4.7118 5.0179 5.3281 5.6406 6.9625 7.3351 7.7244 8.1187 8.5016 8.8521 coh32:k=3.55095,b=0 4.201 4.4728 4.7686 5.0748 5.385 5.6975 7.0193 7.3919 7.7813 8.1755 8.5584 8.909 coh33:k=3.65118,b=0 4.2525 4.5243 4.8201 5.1262 5.4364 5.749 7.0708 7.4434 7.8327 8.227 8.6099 8.9604 coh34:k=3.75142,b=0 4.2994 4.5711 4.867 5.1731 5.4833 5.7958 7.1177 7.4903 7.8796 8.2739 8.6568 9.0073 coh35:k=3.85165,b=0 4.3423 4.614 4.9099 5.216 5.5262 5.8388 7.1606 7.5332 7.9225 8.3168 8.6997 9.0502 coh36:k=3.95189,b=0 4.4271 4.6767 4.9602 5.2675 5.5831 5.9011 7.2001 7.5727 7.962 8.3563 8.7392 9.0897 coh37:k=4.05212,b=0 4.5372 4.7868 5.0703 5.3776 5.6932 6.0111 7.298 7.6386 7.9985 8.3928 8.7757 9.1262 coh38:k=4.15236,b=0 4.6317 4.8813 5.1648 5.4721 5.7877 6.1056 7.3925 7.7331 8.086 8.4459 8.8096 9.1601 coh39:k=4.2526,b=0 4.714 4.9636 5.2471 5.5544 5.87 6.1879 7.4748 7.8154 8.1683 8.5282 8.8833 9.2141 coh40:k=4.35283,b=0 4.7865 5.0361 5.3196 5.6269 5.9425 6.2605 7.5473 7.8879 8.2409 8.6008 8.9558 9.2866 coh41:k=4.45307,b=0 4.8511 5.1007 5.3842 5.6915 6.0071 6.325 7.6119 7.9525 8.3054 8.6653 9.0204 9.3511 coh42:k=4.5533,b=0 4.909 5.1586 5.4421 5.7494 6.065 6.383 7.6698 8.0104 8.3633 8.7233 9.0783 9.4091 coh43:k=4.65354,b=0 4.9614 5.211 5.4945 5.8018 6.1174 6.4353 7.7222 8.0628 8.4157 8.7756 9.1307 9.4614 coh44:k=4.75377,b=0 5.009 5.2586 5.5422 5.8494 6.165 6.483 7.7698 8.1104 8.4634 8.8233 9.1783 9.5091 coh45:k=4.85401,b=0 5.0526 5.3022 5.5857 5.893 6.2086 6.5266 7.8134 8.154 8.507 8.8669 9.2219 9.5527 coh46:k=4.95424,b=0 5.0927 5.3423 5.6258 5.9331 6.2487 6.5667 7.8535 8.1941 8.547 8.907 9.262 9.5928 coh47:k=5.05448,b=0 5.1537 5.3793 5.6629 5.9701 6.2857 6.6037 7.8905 8.2311 8.5841 8.944 9.299 9.6298 coh48:k=5.15471,b=0 5.2507 5.4719 5.7249 6.0045 6.3201 6.638 7.9248 8.2655 8.6184 8.9783 9.3334 9.6641 coh49:k=5.25495,b=0 5.335 5.5562 5.8092 6.0845 6.3772 6.6821 7.9568 8.2974 8.6503 9.0103 9.3653 9.6961 coh50:k=5.35519,b=0 5.4091 5.6303 5.8833 6.1586 6.4514 6.7563 8.0133 8.339 8.6802 9.0401 9.3952 9.7259 coh519:k=52.3656,b=0 15.021 15.066 15.118 15.176 15.241 15.311 15.659 15.766 15.882 16.006 16.136 16.265 coh520:k=52.4659,b=0 15.031 15.076 15.129 15.187 15.251 15.32 15.667 15.774 15.889 16.014 16.144 16.273 coh521:k=52.5661,b=0 15.042 15.087 15.139 15.197 15.261 15.33 15.674 15.781 15.897 16.022 16.152 16.28 coh522:k=52.6663,b=0 15.051 15.097 15.149 15.207 15.271 15.34 15.682 15.789 15.904 16.029 16.159 16.288 coh523:k=52.7666,b=0 15.061 15.106 15.158 15.217 15.281 15.35 15.692 15.797 15.912 16.036 16.167 16.295 coh524:k=52.8668,b=0 15.071 15.116 15.168 15.226 15.29 15.359 15.701 15.806 15.92 16.043 16.174 16.302 coh525:k=52.967,b=0 15.08 15.125 15.177 15.235 15.299 15.368 15.71 15.815 15.929 16.052 16.18 16.309 coh526:k=53.0673,b=0 15.088 15.134 15.186 15.244 15.308 15.377 15.719 15.824 15.938 16.061 16.189 16.315 coh527:k=53.1675,b=0 15.097 15.142 15.194 15.253 15.316 15.386 15.728 15.833 15.947 16.069 16.197 16.323 coh528:k=53.2677,b=0 15.105 15.15 15.203 15.261 15.325 15.394 15.736 15.841 15.955 16.077 16.205 16.332 coh529:k=53.368,b=0 15.115 15.159 15.211 15.269 15.333 15.402 15.744 15.849 15.963 16.086 16.214 16.34 coh530:k=53.4682,b=0 15.125 15.17 15.221 15.278 15.341 15.41 15.752 15.857 15.971 16.093 16.221 16.348 coh531:k=53.5684,b=0 15.136 15.18 15.231 15.288 15.351 15.419 15.76 15.864 15.978 16.101 16.229 16.355 coh532:k=53.6687,b=0 15.146 15.19 15.241 15.298 15.361 15.429 15.767 15.872 15.986 16.108 16.237 16.363 coh533:k=53.7689,b=0 15.155 15.2 15.251 15.308 15.37 15.438 15.775 15.879 15.993 16.116 16.244 16.37 coh534:k=53.8691,b=0 15.165 15.209 15.26 15.317 15.38 15.448 15.784 15.888 16 16.123 16.251 16.377 coh535:k=53.9694,b=0 15.174 15.218 15.269 15.326 15.389 15.457 15.794 15.897 16.009 16.13 16.258 16.384 coh536:k=54.0696,b=0 15.183 15.227 15.278 15.335 15.398 15.466 15.802 15.906 16.018 16.138 16.264 16.391 coh537:k=54.1699,b=0 15.191 15.236 15.287 15.344 15.406 15.474 15.811 15.914 16.026 16.147 16.272 16.397 coh538:k=54.2701,b=0 15.2 15.244 15.295 15.352 15.415 15.483 15.819 15.923 16.035 16.155 16.281 16.404 coh539:k=54.3703,b=0 15.208 15.252 15.303 15.36 15.423 15.491 15.828 15.931 16.043 16.163 16.289 16.413 coh540:k=54.4706,b=0 15.218 15.261 15.311 15.368 15.431 15.499 15.835 15.939 16.051 16.171 16.297 16.42 coh541:k=54.5708,b=0 15.228 15.271 15.321 15.378 15.439 15.507 15.843 15.946 16.058 16.179 16.305 16.428 coh542:k=54.671,b=0 15.238 15.281 15.332 15.388 15.449 15.516 15.851 15.954 16.066 16.186 16.312 16.436 coh543:k=54.7713,b=0 15.248 15.291 15.341 15.397 15.459 15.526 15.858 15.961 16.073 16.194 16.319 16.443 coh544:k=54.8715,b=0 15.257 15.301 15.351 15.407 15.468 15.535 15.866 15.968 16.08 16.201 16.326 16.45 coh545:k=54.9717,b=0 15.266 15.31 15.36 15.416 15.477 15.544 15.875 15.977 16.087 16.208 16.333 16.457 coh546:k=55.072,b=0 15.275 15.319 15.369 15.425 15.486 15.553 15.884 15.986 16.096 16.214 16.34 16.464 coh547:k=55.1722,b=0 15.284 15.327 15.378 15.434 15.495 15.562 15.893 15.994 16.104 16.223 16.347 16.47 coh548:k=55.2724,b=0 15.292 15.336 15.386 15.442 15.503 15.57 15.901 16.003 16.113 16.231 16.354 16.477 coh549:k=55.3727,b=0 15.301 15.344 15.394 15.45 15.511 15.578 15.91 16.011 16.121 16.239 16.362 16.483 coh550:k=55.4729,b=0 15.309 15.352 15.402 15.458 15.519 15.586 15.917 16.019 16.129 16.247 16.37 16.489 coh551:k=55.5732,b=0 15.316 15.36 15.41 15.466 15.527 15.594 15.925 16.027 16.137 16.255 16.377 16.497 coh552:k=55.6734,b=0 15.324 15.367 15.417 15.473 15.535 15.601 15.933 16.034 16.144 16.262 16.385 16.505 coh553:k=55.7736,b=0 15.331 15.374 15.425 15.481 15.542 15.609 15.94 16.041 16.152 16.27 16.392 16.512 coh554:k=55.8739,b=0 15.338 15.382 15.432 15.488 15.549 15.616 15.947 16.049 16.159 16.277 16.399 16.519 coh555:k=55.9741,b=0 15.345 15.389 15.439 15.495 15.556 15.623 15.954 16.056 16.166 16.284 16.406 16.526 coh556:k=56.0743,b=0 15.352 15.395 15.445 15.501 15.563 15.63 15.961 16.062 16.172 16.29 16.413 16.533 coh557:k=56.1746,b=0 15.359 15.402 15.452 15.508 15.569 15.636 15.967 16.069 16.179 16.297 16.42 16.539 coh558:k=56.2748,b=0 15.365 15.408 15.459 15.515 15.576 15.643 15.974 16.075 16.185 16.304 16.426 16.546 coh559:k=56.375,b=0 15.371 15.415 15.465 15.521 15.582 15.649 15.98 16.082 16.192 16.31 16.432 16.552 coh560:k=56.4753,b=0 15.377 15.421 15.471 15.527 15.588 15.655 15.986 16.088 16.198 16.316 16.439 16.558 coh561:k=56.5755,b=0 15.383 15.427 15.477 15.533 15.594 15.661 15.992 16.094 16.204 16.322 16.445 16.564 coh562:k=56.6757,b=0 15.389 15.433 15.483 15.539 15.6 15.667 15.998 16.1 16.21 16.328 16.451 16.57 coh563:k=56.776,b=0 15.395 15.438 15.489 15.545 15.606 15.673 16.004 16.105 16.216 16.334 16.456 16.576 coh564:k=56.8762,b=0 15.401 15.444 15.494 15.55 15.612 15.678 16.01 16.111 16.221 16.339 16.462 16.582 coh565:k=56.9764,b=0 15.406 15.45 15.5 15.556 15.617 15.684 16.015 16.117 16.227 16.345 16.467 16.587 coh566:k=57.0767,b=0 15.412 15.455 15.505 15.561 15.622 15.689 16.021 16.122 16.232 16.35 16.473 16.592 coh567:k=57.1769,b=0 15.417 15.46 15.51 15.566 15.628 15.695 16.026 16.127 16.237 16.355 16.478 16.598 coh568:k=57.2772,b=0 15.422 15.465 15.516 15.572 15.633 15.7 16.031 16.132 16.243 16.361 16.483 16.603 tb_pol_a: optimal safe savings choice for each state space point z1_0_34741 z2_0_40076 z3_0_4623 z4_0_5333 z5_0_61519 z6_0_70966 z10_1_2567 z11_1_4496 z12_1_6723 z13_1_9291 z14_2_2253 z15_2_567 __________ __________ _________ _________ __________ __________ __________ __________ __________ __________ __________ _________ coh1:k=0.443648,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh2:k=0.543883,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh3:k=0.644119,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh4:k=0.744354,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh5:k=0.84459,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh6:k=0.944825,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh7:k=1.04506,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh8:k=1.1453,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh9:k=1.24553,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh10:k=1.34577,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh11:k=1.446,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh12:k=1.54624,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh13:k=1.64647,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh14:k=1.74671,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh15:k=1.84694,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh16:k=1.94718,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh17:k=2.04742,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh18:k=2.14765,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh19:k=2.24789,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh20:k=2.34812,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh21:k=2.44836,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh22:k=2.54859,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh23:k=2.64883,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh24:k=2.74906,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh25:k=2.8493,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh26:k=2.94953,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh27:k=3.04977,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh28:k=3.15001,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh29:k=3.25024,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh30:k=3.35048,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh31:k=3.45071,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh32:k=3.55095,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh33:k=3.65118,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh34:k=3.75142,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh35:k=3.85165,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh36:k=3.95189,b=0 1.0204 1.0204 0 0 0 0 0 0 0 0 0 0 coh37:k=4.05212,b=0 1.0204 1.0204 0 0 0 0 0 0 0 0 0 0 coh38:k=4.15236,b=0 1.0204 1.0204 0 0 0 0 0 0 0 0 0 0 coh39:k=4.2526,b=0 1.0204 1.0204 0 0 0 0 0 0 0 0 0 0 coh40:k=4.35283,b=0 1.0204 1.0204 0 0 0 0 0 0 0 0 0 0 coh41:k=4.45307,b=0 1.0204 1.0204 0 0 0 0 0 0 0 0 0 0 coh42:k=4.5533,b=0 1.0204 1.0204 0 0 0 0 0 0 0 0 0 0 coh43:k=4.65354,b=0 1.0204 1.0204 0 0 0 0 0 0 0 0 0 0 coh44:k=4.75377,b=0 1.0204 1.0204 0 0 0 0 0 0 0 0 0 0 coh45:k=4.85401,b=0 1.0204 1.0204 0 0 0 0 0 0 0 0 0 0 coh46:k=4.95424,b=0 1.0204 1.0204 0 0 0 0 0 0 0 0 0 0 coh47:k=5.05448,b=0 2.0408 1.0204 0 0 0 0 0 0 0 0 0 0 coh48:k=5.15471,b=0 2.0408 2.0408 1.0204 0 0 0 0 0 0 0 0 0 coh49:k=5.25495,b=0 2.0408 2.0408 1.0204 1.0204 0 0 0 0 0 0 0 0 coh50:k=5.35519,b=0 2.0408 2.0408 1.0204 1.0204 0 0 0 0 0 0 0 0 coh519:k=52.3656,b=0 45.918 45.918 44.898 44.898 42.857 42.857 37.755 35.714 33.673 31.633 28.571 25.51 coh520:k=52.4659,b=0 45.918 45.918 44.898 44.898 43.878 43.878 37.755 35.714 33.673 31.633 28.571 25.51 coh521:k=52.5661,b=0 45.918 45.918 44.898 44.898 43.878 43.878 37.755 35.714 33.673 31.633 28.571 25.51 coh522:k=52.6663,b=0 45.918 45.918 44.898 44.898 43.878 43.878 38.776 35.714 33.673 31.633 28.571 25.51 coh523:k=52.7666,b=0 45.918 45.918 44.898 44.898 43.878 43.878 38.776 36.735 33.673 31.633 28.571 25.51 coh524:k=52.8668,b=0 45.918 45.918 44.898 44.898 43.878 43.878 38.776 36.735 34.694 31.633 28.571 25.51 coh525:k=52.967,b=0 45.918 45.918 44.898 44.898 43.878 43.878 38.776 36.735 34.694 32.653 28.571 25.51 coh526:k=53.0673,b=0 45.918 45.918 44.898 44.898 43.878 43.878 38.776 36.735 34.694 32.653 29.592 25.51 coh527:k=53.1675,b=0 45.918 45.918 44.898 44.898 43.878 43.878 38.776 36.735 34.694 32.653 29.592 26.531 coh528:k=53.2677,b=0 45.918 45.918 44.898 44.898 43.878 43.878 38.776 36.735 34.694 32.653 29.592 26.531 coh529:k=53.368,b=0 46.939 46.939 44.898 44.898 43.878 43.878 38.776 36.735 34.694 32.653 29.592 26.531 coh530:k=53.4682,b=0 46.939 46.939 45.918 45.918 43.878 43.878 38.776 36.735 34.694 32.653 29.592 26.531 coh531:k=53.5684,b=0 46.939 46.939 45.918 45.918 44.898 44.898 38.776 36.735 34.694 32.653 29.592 26.531 coh532:k=53.6687,b=0 46.939 46.939 45.918 45.918 44.898 44.898 38.776 36.735 34.694 32.653 29.592 26.531 coh533:k=53.7689,b=0 46.939 46.939 45.918 45.918 44.898 44.898 39.796 36.735 34.694 32.653 29.592 26.531 coh534:k=53.8691,b=0 46.939 46.939 45.918 45.918 44.898 44.898 39.796 37.755 34.694 32.653 29.592 26.531 coh535:k=53.9694,b=0 46.939 46.939 45.918 45.918 44.898 44.898 39.796 37.755 35.714 32.653 29.592 26.531 coh536:k=54.0696,b=0 46.939 46.939 45.918 45.918 44.898 44.898 39.796 37.755 35.714 33.673 29.592 26.531 coh537:k=54.1699,b=0 46.939 46.939 45.918 45.918 44.898 44.898 39.796 37.755 35.714 33.673 30.612 26.531 coh538:k=54.2701,b=0 46.939 46.939 45.918 45.918 44.898 44.898 39.796 37.755 35.714 33.673 30.612 27.551 coh539:k=54.3703,b=0 46.939 46.939 45.918 45.918 44.898 44.898 39.796 37.755 35.714 33.673 30.612 27.551 coh540:k=54.4706,b=0 47.959 47.959 45.918 45.918 44.898 44.898 39.796 37.755 35.714 33.673 30.612 27.551 coh541:k=54.5708,b=0 47.959 47.959 46.939 46.939 45.918 44.898 39.796 37.755 35.714 33.673 30.612 27.551 coh542:k=54.671,b=0 47.959 47.959 46.939 46.939 45.918 44.898 39.796 37.755 35.714 33.673 30.612 27.551 coh543:k=54.7713,b=0 47.959 47.959 46.939 46.939 45.918 44.898 39.796 37.755 35.714 33.673 30.612 27.551 coh544:k=54.8715,b=0 47.959 47.959 46.939 46.939 45.918 44.898 40.816 37.755 35.714 33.673 30.612 27.551 coh545:k=54.9717,b=0 47.959 47.959 46.939 46.939 45.918 44.898 40.816 38.776 35.714 33.673 30.612 27.551 coh546:k=55.072,b=0 47.959 47.959 46.939 46.939 45.918 44.898 40.816 38.776 36.735 33.673 30.612 27.551 coh547:k=55.1722,b=0 47.959 47.959 46.939 46.939 45.918 44.898 40.816 38.776 36.735 34.694 30.612 27.551 coh548:k=55.2724,b=0 47.959 47.959 46.939 46.939 45.918 44.898 40.816 38.776 36.735 34.694 31.633 27.551 coh549:k=55.3727,b=0 47.959 47.959 46.939 46.939 45.918 44.898 40.816 38.776 36.735 34.694 31.633 27.551 coh550:k=55.4729,b=0 47.959 47.959 46.939 46.939 45.918 44.898 40.816 38.776 36.735 34.694 31.633 28.571 coh551:k=55.5732,b=0 47.959 47.959 46.939 46.939 45.918 44.898 40.816 38.776 36.735 34.694 31.633 28.571 coh552:k=55.6734,b=0 47.959 47.959 46.939 46.939 45.918 44.898 40.816 38.776 36.735 34.694 31.633 28.571 coh553:k=55.7736,b=0 47.959 47.959 46.939 46.939 45.918 44.898 40.816 38.776 36.735 34.694 31.633 28.571 coh554:k=55.8739,b=0 47.959 47.959 46.939 46.939 45.918 44.898 40.816 38.776 36.735 34.694 31.633 28.571 coh555:k=55.9741,b=0 47.959 47.959 46.939 46.939 45.918 44.898 40.816 38.776 36.735 34.694 31.633 28.571 coh556:k=56.0743,b=0 47.959 47.959 46.939 46.939 45.918 44.898 40.816 38.776 36.735 34.694 31.633 28.571 coh557:k=56.1746,b=0 47.959 47.959 46.939 46.939 45.918 44.898 40.816 38.776 36.735 34.694 31.633 28.571 coh558:k=56.2748,b=0 47.959 47.959 46.939 46.939 45.918 44.898 40.816 38.776 36.735 34.694 31.633 28.571 coh559:k=56.375,b=0 47.959 47.959 46.939 46.939 45.918 44.898 40.816 38.776 36.735 34.694 31.633 28.571 coh560:k=56.4753,b=0 47.959 47.959 46.939 46.939 45.918 44.898 40.816 38.776 36.735 34.694 31.633 28.571 coh561:k=56.5755,b=0 47.959 47.959 46.939 46.939 45.918 44.898 40.816 38.776 36.735 34.694 31.633 28.571 coh562:k=56.6757,b=0 47.959 47.959 46.939 46.939 45.918 44.898 40.816 38.776 36.735 34.694 31.633 28.571 coh563:k=56.776,b=0 47.959 47.959 46.939 46.939 45.918 44.898 40.816 38.776 36.735 34.694 31.633 28.571 coh564:k=56.8762,b=0 47.959 47.959 46.939 46.939 45.918 44.898 40.816 38.776 36.735 34.694 31.633 28.571 coh565:k=56.9764,b=0 47.959 47.959 46.939 46.939 45.918 44.898 40.816 38.776 36.735 34.694 31.633 28.571 coh566:k=57.0767,b=0 47.959 47.959 46.939 46.939 45.918 44.898 40.816 38.776 36.735 34.694 31.633 28.571 coh567:k=57.1769,b=0 47.959 47.959 46.939 46.939 45.918 44.898 40.816 38.776 36.735 34.694 31.633 28.571 coh568:k=57.2772,b=0 47.959 47.959 46.939 46.939 45.918 44.898 40.816 38.776 36.735 34.694 31.633 28.571 tb_pol_k: optimal risky investment choice for each state space point z1_0_34741 z2_0_40076 z3_0_4623 z4_0_5333 z5_0_61519 z6_0_70966 z10_1_2567 z11_1_4496 z12_1_6723 z13_1_9291 z14_2_2253 z15_2_567 __________ __________ _________ _________ __________ __________ __________ __________ __________ __________ __________ _________ coh1:k=0.443648,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh2:k=0.543883,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh3:k=0.644119,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh4:k=0.744354,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh5:k=0.84459,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh6:k=0.944825,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh7:k=1.04506,b=0 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 coh8:k=1.1453,b=0 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 coh9:k=1.24553,b=0 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 coh10:k=1.34577,b=0 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 coh11:k=1.446,b=0 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 coh12:k=1.54624,b=0 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 coh13:k=1.64647,b=0 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 coh14:k=1.74671,b=0 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 coh15:k=1.84694,b=0 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 coh16:k=1.94718,b=0 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 coh17:k=2.04742,b=0 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 coh18:k=2.14765,b=0 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 coh19:k=2.24789,b=0 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 coh20:k=2.34812,b=0 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 coh21:k=2.44836,b=0 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 coh22:k=2.54859,b=0 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 coh23:k=2.64883,b=0 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 1.0204 1.0204 1.0204 1.0204 coh24:k=2.74906,b=0 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 1.0204 1.0204 coh25:k=2.8493,b=0 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 coh26:k=2.94953,b=0 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 coh27:k=3.04977,b=0 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 coh28:k=3.15001,b=0 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 coh29:k=3.25024,b=0 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 coh30:k=3.35048,b=0 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 coh31:k=3.45071,b=0 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 coh32:k=3.55095,b=0 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 coh33:k=3.65118,b=0 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 coh34:k=3.75142,b=0 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 coh35:k=3.85165,b=0 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 coh36:k=3.95189,b=0 2.0408 2.0408 3.0612 3.0612 3.0612 3.0612 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 coh37:k=4.05212,b=0 2.0408 2.0408 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 2.0408 2.0408 2.0408 2.0408 coh38:k=4.15236,b=0 2.0408 2.0408 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 2.0408 2.0408 coh39:k=4.2526,b=0 2.0408 2.0408 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 coh40:k=4.35283,b=0 2.0408 2.0408 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 coh41:k=4.45307,b=0 2.0408 2.0408 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 coh42:k=4.5533,b=0 2.0408 2.0408 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 coh43:k=4.65354,b=0 2.0408 2.0408 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 coh44:k=4.75377,b=0 2.0408 2.0408 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 coh45:k=4.85401,b=0 2.0408 2.0408 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 coh46:k=4.95424,b=0 2.0408 2.0408 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 coh47:k=5.05448,b=0 2.0408 2.0408 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 coh48:k=5.15471,b=0 2.0408 2.0408 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 coh49:k=5.25495,b=0 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 coh50:k=5.35519,b=0 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 4.0816 4.0816 3.0612 3.0612 3.0612 3.0612 coh519:k=52.3656,b=0 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 21.429 coh520:k=52.4659,b=0 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 21.429 coh521:k=52.5661,b=0 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 21.429 coh522:k=52.6663,b=0 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 21.429 coh523:k=52.7666,b=0 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 21.429 coh524:k=52.8668,b=0 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 21.429 coh525:k=52.967,b=0 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 21.429 coh526:k=53.0673,b=0 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 21.429 coh527:k=53.1675,b=0 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 21.429 coh528:k=53.2677,b=0 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 21.429 coh529:k=53.368,b=0 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 21.429 coh530:k=53.4682,b=0 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 21.429 coh531:k=53.5684,b=0 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 21.429 coh532:k=53.6687,b=0 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 21.429 coh533:k=53.7689,b=0 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 21.429 coh534:k=53.8691,b=0 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 21.429 coh535:k=53.9694,b=0 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 21.429 coh536:k=54.0696,b=0 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 21.429 coh537:k=54.1699,b=0 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 21.429 coh538:k=54.2701,b=0 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 21.429 coh539:k=54.3703,b=0 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 21.429 coh540:k=54.4706,b=0 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 21.429 coh541:k=54.5708,b=0 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 21.429 coh542:k=54.671,b=0 2.0408 2.0408 3.0612 3.0612 4.0816 5.102 9.1837 11.224 13.265 15.306 18.367 21.429 coh543:k=54.7713,b=0 2.0408 2.0408 3.0612 3.0612 4.0816 5.102 9.1837 11.224 13.265 15.306 18.367 21.429 coh544:k=54.8715,b=0 2.0408 2.0408 3.0612 3.0612 4.0816 5.102 9.1837 11.224 13.265 15.306 18.367 21.429 coh545:k=54.9717,b=0 2.0408 2.0408 3.0612 3.0612 4.0816 5.102 9.1837 11.224 13.265 15.306 18.367 21.429 coh546:k=55.072,b=0 2.0408 2.0408 3.0612 3.0612 4.0816 5.102 9.1837 11.224 13.265 15.306 18.367 21.429 coh547:k=55.1722,b=0 2.0408 2.0408 3.0612 3.0612 4.0816 5.102 9.1837 11.224 13.265 15.306 18.367 21.429 coh548:k=55.2724,b=0 2.0408 2.0408 3.0612 3.0612 4.0816 5.102 9.1837 11.224 13.265 15.306 18.367 21.429 coh549:k=55.3727,b=0 2.0408 2.0408 3.0612 3.0612 4.0816 5.102 9.1837 11.224 13.265 15.306 18.367 21.429 coh550:k=55.4729,b=0 2.0408 2.0408 3.0612 3.0612 4.0816 5.102 9.1837 11.224 13.265 15.306 18.367 21.429 coh551:k=55.5732,b=0 2.0408 2.0408 3.0612 3.0612 4.0816 5.102 9.1837 11.224 13.265 15.306 18.367 21.429 coh552:k=55.6734,b=0 2.0408 2.0408 3.0612 3.0612 4.0816 5.102 9.1837 11.224 13.265 15.306 18.367 21.429 coh553:k=55.7736,b=0 2.0408 2.0408 3.0612 3.0612 4.0816 5.102 9.1837 11.224 13.265 15.306 18.367 21.429 coh554:k=55.8739,b=0 2.0408 2.0408 3.0612 3.0612 4.0816 5.102 9.1837 11.224 13.265 15.306 18.367 21.429 coh555:k=55.9741,b=0 2.0408 2.0408 3.0612 3.0612 4.0816 5.102 9.1837 11.224 13.265 15.306 18.367 21.429 coh556:k=56.0743,b=0 2.0408 2.0408 3.0612 3.0612 4.0816 5.102 9.1837 11.224 13.265 15.306 18.367 21.429 coh557:k=56.1746,b=0 2.0408 2.0408 3.0612 3.0612 4.0816 5.102 9.1837 11.224 13.265 15.306 18.367 21.429 coh558:k=56.2748,b=0 2.0408 2.0408 3.0612 3.0612 4.0816 5.102 9.1837 11.224 13.265 15.306 18.367 21.429 coh559:k=56.375,b=0 2.0408 2.0408 3.0612 3.0612 4.0816 5.102 9.1837 11.224 13.265 15.306 18.367 21.429 coh560:k=56.4753,b=0 2.0408 2.0408 3.0612 3.0612 4.0816 5.102 9.1837 11.224 13.265 15.306 18.367 21.429 coh561:k=56.5755,b=0 2.0408 2.0408 3.0612 3.0612 4.0816 5.102 9.1837 11.224 13.265 15.306 18.367 21.429 coh562:k=56.6757,b=0 2.0408 2.0408 3.0612 3.0612 4.0816 5.102 9.1837 11.224 13.265 15.306 18.367 21.429 coh563:k=56.776,b=0 2.0408 2.0408 3.0612 3.0612 4.0816 5.102 9.1837 11.224 13.265 15.306 18.367 21.429 coh564:k=56.8762,b=0 2.0408 2.0408 3.0612 3.0612 4.0816 5.102 9.1837 11.224 13.265 15.306 18.367 21.429 coh565:k=56.9764,b=0 2.0408 2.0408 3.0612 3.0612 4.0816 5.102 9.1837 11.224 13.265 15.306 18.367 21.429 coh566:k=57.0767,b=0 2.0408 2.0408 3.0612 3.0612 4.0816 5.102 9.1837 11.224 13.265 15.306 18.367 21.429 coh567:k=57.1769,b=0 2.0408 2.0408 3.0612 3.0612 4.0816 5.102 9.1837 11.224 13.265 15.306 18.367 21.429 coh568:k=57.2772,b=0 2.0408 2.0408 3.0612 3.0612 4.0816 5.102 9.1837 11.224 13.265 15.306 18.367 21.429 tb_pol_w: risky + safe investment choices (first stage choice, choose within risky vs safe) z1_0_34741 z2_0_40076 z3_0_4623 z4_0_5333 z5_0_61519 z6_0_70966 z10_1_2567 z11_1_4496 z12_1_6723 z13_1_9291 z14_2_2253 z15_2_567 __________ __________ _________ _________ __________ __________ __________ __________ __________ __________ __________ _________ coh1:k=0.443648,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh2:k=0.543883,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh3:k=0.644119,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh4:k=0.744354,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh5:k=0.84459,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh6:k=0.944825,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh7:k=1.04506,b=0 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 coh8:k=1.1453,b=0 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 coh9:k=1.24553,b=0 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 coh10:k=1.34577,b=0 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 coh11:k=1.446,b=0 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 coh12:k=1.54624,b=0 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 coh13:k=1.64647,b=0 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 coh14:k=1.74671,b=0 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 coh15:k=1.84694,b=0 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 coh16:k=1.94718,b=0 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 coh17:k=2.04742,b=0 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 coh18:k=2.14765,b=0 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 coh19:k=2.24789,b=0 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 coh20:k=2.34812,b=0 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 coh21:k=2.44836,b=0 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 coh22:k=2.54859,b=0 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 coh23:k=2.64883,b=0 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 1.0204 1.0204 1.0204 1.0204 coh24:k=2.74906,b=0 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 1.0204 1.0204 coh25:k=2.8493,b=0 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 coh26:k=2.94953,b=0 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 coh27:k=3.04977,b=0 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 coh28:k=3.15001,b=0 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 coh29:k=3.25024,b=0 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 coh30:k=3.35048,b=0 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 coh31:k=3.45071,b=0 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 coh32:k=3.55095,b=0 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 coh33:k=3.65118,b=0 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 coh34:k=3.75142,b=0 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 coh35:k=3.85165,b=0 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 coh36:k=3.95189,b=0 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 coh37:k=4.05212,b=0 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 2.0408 2.0408 2.0408 2.0408 coh38:k=4.15236,b=0 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 2.0408 2.0408 coh39:k=4.2526,b=0 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 coh40:k=4.35283,b=0 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 coh41:k=4.45307,b=0 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 coh42:k=4.5533,b=0 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 coh43:k=4.65354,b=0 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 coh44:k=4.75377,b=0 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 coh45:k=4.85401,b=0 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 coh46:k=4.95424,b=0 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 coh47:k=5.05448,b=0 4.0816 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 coh48:k=5.15471,b=0 4.0816 4.0816 4.0816 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 coh49:k=5.25495,b=0 4.0816 4.0816 4.0816 4.0816 4.0816 4.0816 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 coh50:k=5.35519,b=0 4.0816 4.0816 4.0816 4.0816 4.0816 4.0816 4.0816 4.0816 3.0612 3.0612 3.0612 3.0612 coh519:k=52.3656,b=0 47.959 47.959 47.959 47.959 46.939 46.939 46.939 46.939 46.939 46.939 46.939 46.939 coh520:k=52.4659,b=0 47.959 47.959 47.959 47.959 47.959 47.959 46.939 46.939 46.939 46.939 46.939 46.939 coh521:k=52.5661,b=0 47.959 47.959 47.959 47.959 47.959 47.959 46.939 46.939 46.939 46.939 46.939 46.939 coh522:k=52.6663,b=0 47.959 47.959 47.959 47.959 47.959 47.959 47.959 46.939 46.939 46.939 46.939 46.939 coh523:k=52.7666,b=0 47.959 47.959 47.959 47.959 47.959 47.959 47.959 47.959 46.939 46.939 46.939 46.939 coh524:k=52.8668,b=0 47.959 47.959 47.959 47.959 47.959 47.959 47.959 47.959 47.959 46.939 46.939 46.939 coh525:k=52.967,b=0 47.959 47.959 47.959 47.959 47.959 47.959 47.959 47.959 47.959 47.959 46.939 46.939 coh526:k=53.0673,b=0 47.959 47.959 47.959 47.959 47.959 47.959 47.959 47.959 47.959 47.959 47.959 46.939 coh527:k=53.1675,b=0 47.959 47.959 47.959 47.959 47.959 47.959 47.959 47.959 47.959 47.959 47.959 47.959 coh528:k=53.2677,b=0 47.959 47.959 47.959 47.959 47.959 47.959 47.959 47.959 47.959 47.959 47.959 47.959 coh529:k=53.368,b=0 48.98 48.98 47.959 47.959 47.959 47.959 47.959 47.959 47.959 47.959 47.959 47.959 coh530:k=53.4682,b=0 48.98 48.98 48.98 48.98 47.959 47.959 47.959 47.959 47.959 47.959 47.959 47.959 coh531:k=53.5684,b=0 48.98 48.98 48.98 48.98 48.98 48.98 47.959 47.959 47.959 47.959 47.959 47.959 coh532:k=53.6687,b=0 48.98 48.98 48.98 48.98 48.98 48.98 47.959 47.959 47.959 47.959 47.959 47.959 coh533:k=53.7689,b=0 48.98 48.98 48.98 48.98 48.98 48.98 48.98 47.959 47.959 47.959 47.959 47.959 coh534:k=53.8691,b=0 48.98 48.98 48.98 48.98 48.98 48.98 48.98 48.98 47.959 47.959 47.959 47.959 coh535:k=53.9694,b=0 48.98 48.98 48.98 48.98 48.98 48.98 48.98 48.98 48.98 47.959 47.959 47.959 coh536:k=54.0696,b=0 48.98 48.98 48.98 48.98 48.98 48.98 48.98 48.98 48.98 48.98 47.959 47.959 coh537:k=54.1699,b=0 48.98 48.98 48.98 48.98 48.98 48.98 48.98 48.98 48.98 48.98 48.98 47.959 coh538:k=54.2701,b=0 48.98 48.98 48.98 48.98 48.98 48.98 48.98 48.98 48.98 48.98 48.98 48.98 coh539:k=54.3703,b=0 48.98 48.98 48.98 48.98 48.98 48.98 48.98 48.98 48.98 48.98 48.98 48.98 coh540:k=54.4706,b=0 50 50 48.98 48.98 48.98 48.98 48.98 48.98 48.98 48.98 48.98 48.98 coh541:k=54.5708,b=0 50 50 50 50 50 48.98 48.98 48.98 48.98 48.98 48.98 48.98 coh542:k=54.671,b=0 50 50 50 50 50 50 48.98 48.98 48.98 48.98 48.98 48.98 coh543:k=54.7713,b=0 50 50 50 50 50 50 48.98 48.98 48.98 48.98 48.98 48.98 coh544:k=54.8715,b=0 50 50 50 50 50 50 50 48.98 48.98 48.98 48.98 48.98 coh545:k=54.9717,b=0 50 50 50 50 50 50 50 50 48.98 48.98 48.98 48.98 coh546:k=55.072,b=0 50 50 50 50 50 50 50 50 50 48.98 48.98 48.98 coh547:k=55.1722,b=0 50 50 50 50 50 50 50 50 50 50 48.98 48.98 coh548:k=55.2724,b=0 50 50 50 50 50 50 50 50 50 50 50 48.98 coh549:k=55.3727,b=0 50 50 50 50 50 50 50 50 50 50 50 48.98 coh550:k=55.4729,b=0 50 50 50 50 50 50 50 50 50 50 50 50 coh551:k=55.5732,b=0 50 50 50 50 50 50 50 50 50 50 50 50 coh552:k=55.6734,b=0 50 50 50 50 50 50 50 50 50 50 50 50 coh553:k=55.7736,b=0 50 50 50 50 50 50 50 50 50 50 50 50 coh554:k=55.8739,b=0 50 50 50 50 50 50 50 50 50 50 50 50 coh555:k=55.9741,b=0 50 50 50 50 50 50 50 50 50 50 50 50 coh556:k=56.0743,b=0 50 50 50 50 50 50 50 50 50 50 50 50 coh557:k=56.1746,b=0 50 50 50 50 50 50 50 50 50 50 50 50 coh558:k=56.2748,b=0 50 50 50 50 50 50 50 50 50 50 50 50 coh559:k=56.375,b=0 50 50 50 50 50 50 50 50 50 50 50 50 coh560:k=56.4753,b=0 50 50 50 50 50 50 50 50 50 50 50 50 coh561:k=56.5755,b=0 50 50 50 50 50 50 50 50 50 50 50 50 coh562:k=56.6757,b=0 50 50 50 50 50 50 50 50 50 50 50 50 coh563:k=56.776,b=0 50 50 50 50 50 50 50 50 50 50 50 50 coh564:k=56.8762,b=0 50 50 50 50 50 50 50 50 50 50 50 50 coh565:k=56.9764,b=0 50 50 50 50 50 50 50 50 50 50 50 50 coh566:k=57.0767,b=0 50 50 50 50 50 50 50 50 50 50 50 50 coh567:k=57.1769,b=0 50 50 50 50 50 50 50 50 50 50 50 50 coh568:k=57.2772,b=0 50 50 50 50 50 50 50 50 50 50 50 50
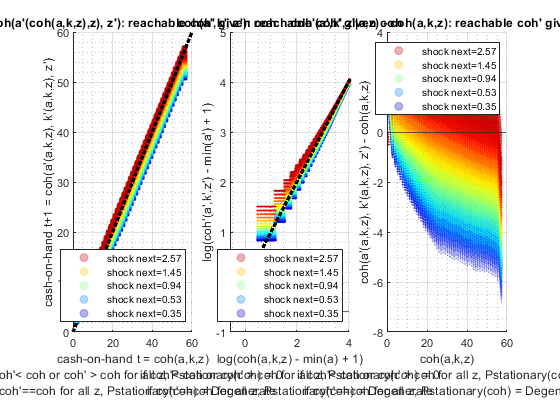
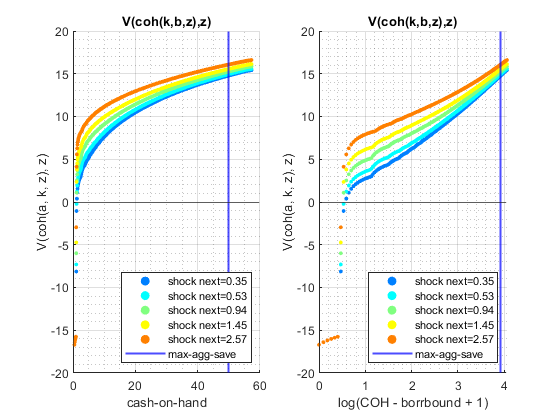
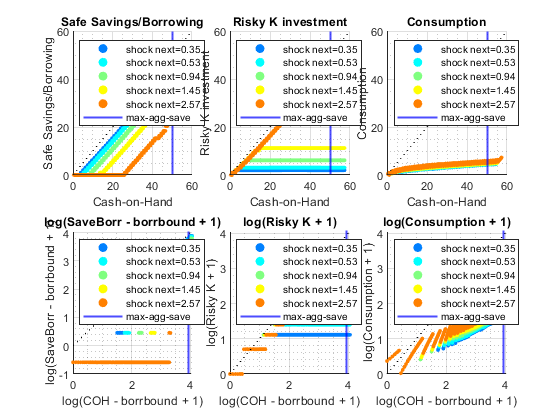
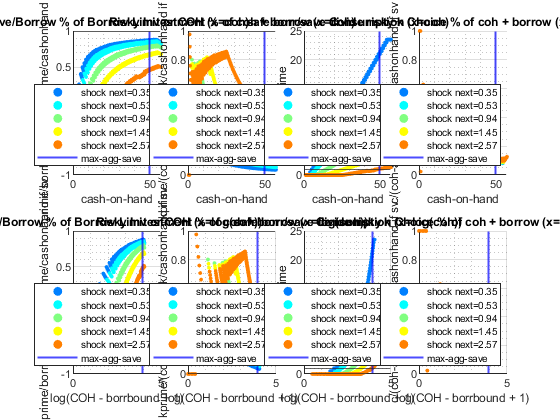
Display Various Containers
if (bl_display_defparam)
Display 1 support_map
fft_container_map_display(support_map, it_display_summmat_rowmax, it_display_summmat_colmax);
---------------------------------------- ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Begin: Show all key and value pairs from container CONTAINER NAME: SUPPORT_MAP ---------------------------------------- Map with properties: Count: 41 KeyType: char ValueType: any xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ---------------------------------------- ---------------------------------------- pos = 1 ; key = bl_display ; val = true pos = 2 ; key = bl_display_defparam ; val = true pos = 3 ; key = bl_display_dist ; val = false pos = 4 ; key = bl_display_evf ; val = false pos = 5 ; key = bl_display_final ; val = true pos = 6 ; key = bl_display_final_dist ; val = false pos = 7 ; key = bl_display_final_dist_detail ; val = false pos = 8 ; key = bl_display_funcgrids ; val = false pos = 9 ; key = bl_graph ; val = true pos = 10 ; key = bl_graph_coh_t_coh ; val = true pos = 11 ; key = bl_graph_evf ; val = false pos = 12 ; key = bl_graph_funcgrids ; val = false pos = 13 ; key = bl_graph_onebyones ; val = false pos = 14 ; key = bl_graph_pol_lvl ; val = true pos = 15 ; key = bl_graph_pol_pct ; val = true pos = 16 ; key = bl_graph_val ; val = true pos = 17 ; key = bl_img_save ; val = true pos = 18 ; key = bl_mat ; val = false pos = 19 ; key = bl_post ; val = true pos = 20 ; key = bl_profile ; val = false pos = 21 ; key = bl_profile_dist ; val = false pos = 22 ; key = bl_time ; val = true pos = 23 ; key = it_display_every ; val = 5 pos = 24 ; key = it_display_final_colmax ; val = 12 pos = 25 ; key = it_display_final_rowmax ; val = 100 pos = 26 ; key = it_display_summmat_colmax ; val = 7 pos = 27 ; key = it_display_summmat_rowmax ; val = 7 pos = 28 ; key = st_img_name_main ; val = ff_iwkz_vf_default pos = 29 ; key = st_img_path ; val = C:/Users/fan/CodeDynaAsset//m_akz//solve/img/ pos = 30 ; key = st_img_prefix ; val = pos = 31 ; key = st_img_suffix ; val = _p2.png pos = 32 ; key = st_mat_name_main ; val = ff_iwkz_vf_default pos = 33 ; key = st_mat_path ; val = C:/Users/fan/CodeDynaAsset//m_akz//solve/mat/ pos = 34 ; key = st_mat_prefix ; val = pos = 35 ; key = st_mat_suffix ; val = _p2 pos = 36 ; key = st_matimg_path_root ; val = C:/Users/fan/CodeDynaAsset//m_akz/ pos = 37 ; key = st_profile_name_main ; val = ff_iwkz_vf_default pos = 38 ; key = st_profile_path ; val = C:/Users/fan/CodeDynaAsset//m_akz//solve/profile/ pos = 39 ; key = st_profile_prefix ; val = pos = 40 ; key = st_profile_suffix ; val = _p2 pos = 41 ; key = st_title_prefix ; val = ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Scalars in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx value __ ___ _____ bl_display 1 1 1 bl_display_defparam 2 2 1 bl_display_dist 3 3 0 bl_display_evf 4 4 0 bl_display_final 5 5 1 bl_display_final_dist 6 6 0 bl_display_final_dist_detail 7 7 0 bl_display_funcgrids 8 8 0 bl_graph 9 9 1 bl_graph_coh_t_coh 10 10 1 bl_graph_evf 11 11 0 bl_graph_funcgrids 12 12 0 bl_graph_onebyones 13 13 0 bl_graph_pol_lvl 14 14 1 bl_graph_pol_pct 15 15 1 bl_graph_val 16 16 1 bl_img_save 17 17 1 bl_mat 18 18 0 bl_post 19 19 1 bl_profile 20 20 0 bl_profile_dist 21 21 0 bl_time 22 22 1 it_display_every 23 23 5 it_display_final_colmax 24 24 12 it_display_final_rowmax 25 25 100 it_display_summmat_colmax 26 26 7 it_display_summmat_rowmax 27 27 7 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Strings in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx __ ___ st_img_name_main 1 28 st_img_path 2 29 st_img_prefix 3 30 st_img_suffix 4 31 st_mat_name_main 5 32 st_mat_path 6 33 st_mat_prefix 7 34 st_mat_suffix 8 35 st_matimg_path_root 9 36 st_profile_name_main 10 37 st_profile_path 11 38 st_profile_prefix 12 39 st_profile_suffix 13 40 st_title_prefix 14 41
Display 2 armt_map
fft_container_map_display(armt_map, it_display_summmat_rowmax, it_display_summmat_colmax);
---------------------------------------- ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Begin: Show all key and value pairs from container CONTAINER NAME: ARMT_MAP ---------------------------------------- Map with properties: Count: 17 KeyType: char ValueType: any xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ---------------------------------------- ---------------------------------------- pos = 1 ; key = ar_a_meshk ;rown= 568 ,coln= 1 ar_a_meshk :mu= 28.8604 ,sd= 16.4498 ,min= 0.44365 ,max= 57.2772 zi_1_c1 _______ zi_1_R1 0.44365 zi_2_R2 0.54388 zi_3_R3 0.64412 zi_284_r284 28.81 zi_566_r566 57.077 zi_567_r567 57.177 zi_568_r568 57.277 pos = 2 ; key = ar_a_mw_wth_na ;rown= 2500 ,coln= 1 ar_a_mw_wth_na :mu= NaN ,sd= NaN ,min= 0 ,max= 50 zi_1_c1 _______ zi_1_R1 0 zi_2_R2 NaN zi_3_R3 NaN zi_1250_r1250 NaN zi_2498_r2498 2.0408 zi_2499_r2499 1.0204 zi_2500_r2500 0 pos = 3 ; key = ar_interp_c_grid ;rown= 1 ,coln= 572761 ar_interp_c_grid :mu= 28.6391 ,sd= 16.5342 ,min= 0.001 ,max= 57.2772 zi_1_C1 zi_2_C2 zi_3_C3 zi_286381_c286381 zi_572759_c572759 zi_572760_c572760 zi_572761_c572761 _______ _______ _______ _________________ _________________ _________________ _________________ zi_1_r1 0.001 0.0011 0.0012 28.639 57.277 57.277 57.277 pos = 4 ; key = ar_interp_coh_grid ;rown= 1 ,coln= 568 ar_interp_coh_grid :mu= 28.8604 ,sd= 16.4498 ,min= 0.44365 ,max= 57.2772 zi_1_C1 zi_2_C2 zi_3_C3 zi_284_c284 zi_566_c566 zi_567_c567 zi_568_c568 _______ _______ _______ ___________ ___________ ___________ ___________ zi_1_r1 0.44365 0.54388 0.64412 28.81 57.077 57.177 57.277 pos = 5 ; key = ar_k ;rown= 1 ,coln= 50 ar_k :mu= 25 ,sd= 14.8749 ,min= 0 ,max= 50 zi_1_C1 zi_2_C2 zi_3_C3 zi_25_c25 zi_48_c48 zi_49_c49 zi_50_c50 _______ _______ _______ _________ _________ _________ _________ zi_1_r1 0 1.0204 2.0408 24.49 47.959 48.98 50 pos = 6 ; key = ar_k_mesha ;rown= 568 ,coln= 1 ar_k_mesha :mu= 0 ,sd= 0 ,min= 0 ,max= 0 zi_1_c1 _______ zi_1_R1 0 zi_2_R2 0 zi_3_R3 0 zi_284_r284 0 zi_566_r566 0 zi_567_r567 0 zi_568_r568 0 pos = 7 ; key = ar_k_mw_wth_na ;rown= 2500 ,coln= 1 ar_k_mw_wth_na :mu= NaN ,sd= NaN ,min= 0 ,max= 50 zi_1_c1 _______ zi_1_R1 0 zi_2_R2 NaN zi_3_R3 NaN zi_1250_r1250 NaN zi_2498_r2498 47.959 zi_2499_r2499 48.98 zi_2500_r2500 50 pos = 8 ; key = ar_stationary ;rown= 1 ,coln= 15 ar_stationary :mu= 0.066667 ,sd= 0.060897 ,min= 0.0027089 ,max= 0.16757 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 _________ _________ ________ _______ _________ _________ _________ zi_1_r1 0.0027089 0.0069499 0.018507 0.16757 0.018507 0.0069499 0.0027089 pos = 9 ; key = ar_w ;rown= 1 ,coln= 50 ar_w :mu= 25 ,sd= 14.8749 ,min= 0 ,max= 50 zi_1_C1 zi_2_C2 zi_3_C3 zi_25_c25 zi_48_c48 zi_49_c49 zi_50_c50 _______ _______ _______ _________ _________ _________ _________ zi_1_r1 0 1.0204 2.0408 24.49 47.959 48.98 50 pos = 10 ; key = ar_z ;rown= 1 ,coln= 15 ar_z :mu= 1.1347 ,sd= 0.69878 ,min= 0.34741 ,max= 2.567 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 _______ _______ _______ _______ _________ _________ _________ zi_1_r1 0.34741 0.40076 0.4623 0.94436 1.9291 2.2253 2.567 pos = 11 ; key = it_ameshk_n ; val = 568 pos = 12 ; key = mt_coh_wkb ;rown= 568 ,coln= 15 mt_coh_wkb :mu= 28.8604 ,sd= 16.4363 ,min= 0.44365 ,max= 57.2772 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 _______ _______ _______ _______ _________ _________ _________ zi_1_R1 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 zi_2_R2 0.54388 0.54388 0.54388 0.54388 0.54388 0.54388 0.54388 zi_3_R3 0.64412 0.64412 0.64412 0.64412 0.64412 0.64412 0.64412 zi_284_r284 28.81 28.81 28.81 28.81 28.81 28.81 28.81 zi_566_r566 57.077 57.077 57.077 57.077 57.077 57.077 57.077 zi_567_r567 57.177 57.177 57.177 57.177 57.177 57.177 57.177 zi_568_r568 57.277 57.277 57.277 57.277 57.277 57.277 57.277 pos = 13 ; key = mt_interp_coh_grid_mesh_z ;rown= 568 ,coln= 15 mt_interp_coh_grid_mesh_z :mu= 28.8604 ,sd= 16.4363 ,min= 0.44365 ,max= 57.2772 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 _______ _______ _______ _______ _________ _________ _________ zi_1_R1 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 zi_2_R2 0.54388 0.54388 0.54388 0.54388 0.54388 0.54388 0.54388 zi_3_R3 0.64412 0.64412 0.64412 0.64412 0.64412 0.64412 0.64412 zi_284_r284 28.81 28.81 28.81 28.81 28.81 28.81 28.81 zi_566_r566 57.077 57.077 57.077 57.077 57.077 57.077 57.077 zi_567_r567 57.177 57.177 57.177 57.177 57.177 57.177 57.177 zi_568_r568 57.277 57.277 57.277 57.277 57.277 57.277 57.277 pos = 14 ; key = mt_k_wth_na ;rown= 50 ,coln= 50 mt_k_wth_na :mu= NaN ,sd= NaN ,min= 0 ,max= 50 zi_1_C1 zi_2_C2 zi_3_C3 zi_25_c25 zi_48_c48 zi_49_c49 zi_50_c50 _______ _______ _______ _________ _________ _________ _________ zi_1_R1 0 0 0 0 0 0 0 zi_2_R2 NaN 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 zi_3_R3 NaN NaN 2.0408 2.0408 2.0408 2.0408 2.0408 zi_25_r25 NaN NaN NaN 24.49 24.49 24.49 24.49 zi_48_r48 NaN NaN NaN NaN 47.959 47.959 47.959 zi_49_r49 NaN NaN NaN NaN NaN 48.98 48.98 zi_50_r50 NaN NaN NaN NaN NaN NaN 50 pos = 15 ; key = mt_z_mesh_coh_interp_grid ;rown= 568 ,coln= 15 mt_z_mesh_coh_interp_grid :mu= 1.1347 ,sd= 0.67512 ,min= 0.34741 ,max= 2.567 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 _______ _______ _______ _______ _________ _________ _________ zi_1_R1 0.34741 0.40076 0.4623 0.94436 1.9291 2.2253 2.567 zi_2_R2 0.34741 0.40076 0.4623 0.94436 1.9291 2.2253 2.567 zi_3_R3 0.34741 0.40076 0.4623 0.94436 1.9291 2.2253 2.567 zi_284_r284 0.34741 0.40076 0.4623 0.94436 1.9291 2.2253 2.567 zi_566_r566 0.34741 0.40076 0.4623 0.94436 1.9291 2.2253 2.567 zi_567_r567 0.34741 0.40076 0.4623 0.94436 1.9291 2.2253 2.567 zi_568_r568 0.34741 0.40076 0.4623 0.94436 1.9291 2.2253 2.567 pos = 16 ; key = mt_z_mesh_coh_wkb ;rown= 1275 ,coln= 15 mt_z_mesh_coh_wkb :mu= 1.1347 ,sd= 0.6751 ,min= 0.34741 ,max= 2.567 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 _______ _______ _______ _______ _________ _________ _________ zi_1_R1 0.34741 0.40076 0.4623 0.94436 1.9291 2.2253 2.567 zi_2_R2 0.34741 0.40076 0.4623 0.94436 1.9291 2.2253 2.567 zi_3_R3 0.34741 0.40076 0.4623 0.94436 1.9291 2.2253 2.567 zi_638_R638 0.34741 0.40076 0.4623 0.94436 1.9291 2.2253 2.567 zi_1273_r1273 0.34741 0.40076 0.4623 0.94436 1.9291 2.2253 2.567 zi_1274_r1274 0.34741 0.40076 0.4623 0.94436 1.9291 2.2253 2.567 zi_1275_r1275 0.34741 0.40076 0.4623 0.94436 1.9291 2.2253 2.567 pos = 17 ; key = mt_z_trans ;rown= 15 ,coln= 15 mt_z_trans :mu= 0.066667 ,sd= 0.095337 ,min= 0 ,max= 0.27902 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 __________ __________ __________ __________ __________ __________ __________ zi_1_R1 0.26016 0.26831 0.25551 0.00012823 2.7001e-13 1.1102e-15 0 zi_2_R2 0.11232 0.19622 0.2763 0.00098855 1.5289e-11 9.3592e-14 3.3307e-16 zi_3_R3 0.037073 0.10492 0.2185 0.0055558 6.2811e-10 5.7438e-12 3.1863e-14 zi_8_R8 1.7181e-06 4.1008e-05 0.00061112 0.27902 0.00061112 4.1008e-05 1.7181e-06 zi_13_r13 3.1909e-14 5.7438e-12 6.2811e-10 0.0055558 0.2185 0.10492 0.037073 zi_14_r14 3.474e-16 9.3597e-14 1.5289e-11 0.00098855 0.2763 0.19622 0.11232 zi_15_r15 2.7412e-18 1.1057e-15 2.6998e-13 0.00012823 0.25551 0.26831 0.26016 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Matrix in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx rowN colN mean std min max __ ___ ____ __________ ________ ________ _________ _______ ar_a_meshk 1 1 568 1 28.86 16.45 0.44365 57.277 ar_a_mw_wth_na 2 2 2500 1 NaN NaN 0 50 ar_interp_c_grid 3 3 1 5.7276e+05 28.639 16.534 0.001 57.277 ar_interp_coh_grid 4 4 1 568 28.86 16.45 0.44365 57.277 ar_k 5 5 1 50 25 14.875 0 50 ar_k_mesha 6 6 568 1 0 0 0 0 ar_k_mw_wth_na 7 7 2500 1 NaN NaN 0 50 ar_stationary 8 8 1 15 0.066667 0.060897 0.0027089 0.16757 ar_w 9 9 1 50 25 14.875 0 50 ar_z 10 10 1 15 1.1347 0.69878 0.34741 2.567 mt_coh_wkb 11 12 568 15 28.86 16.436 0.44365 57.277 mt_interp_coh_grid_mesh_z 12 13 568 15 28.86 16.436 0.44365 57.277 mt_k_wth_na 13 14 50 50 NaN NaN 0 50 mt_z_mesh_coh_interp_grid 14 15 568 15 1.1347 0.67512 0.34741 2.567 mt_z_mesh_coh_wkb 15 16 1275 15 1.1347 0.6751 0.34741 2.567 mt_z_trans 16 17 15 15 0.066667 0.095337 0 0.27902 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Scalars in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx value _ ___ _____ it_ameshk_n 1 11 568
Display 3 param_map
fft_container_map_display(param_map, it_display_summmat_rowmax, it_display_summmat_colmax);
---------------------------------------- ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Begin: Show all key and value pairs from container CONTAINER NAME: PARAM_MAP ---------------------------------------- Map with properties: Count: 32 KeyType: char ValueType: any xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ---------------------------------------- ---------------------------------------- pos = 1 ; key = bl_default ; val = 0 pos = 2 ; key = fl_Amean ; val = 1 pos = 3 ; key = fl_alpha ; val = 0.36 pos = 4 ; key = fl_b_bd ; val = 0 pos = 5 ; key = fl_beta ; val = 0.94 pos = 6 ; key = fl_c_min ; val = 0.001 pos = 7 ; key = fl_coh_interp_grid_gap ; val = 0.1 pos = 8 ; key = fl_crra ; val = 1.5 pos = 9 ; key = fl_default_aprime ; val = 0 pos = 10 ; key = fl_delta ; val = 0.08 pos = 11 ; key = fl_k_min ; val = 0 pos = 12 ; key = fl_nan_replace ; val = -9999 pos = 13 ; key = fl_r_borr ; val = 0.025 pos = 14 ; key = fl_r_save ; val = 0.025 pos = 15 ; key = fl_tol_dist ; val = 1e-05 pos = 16 ; key = fl_tol_pol ; val = 1e-05 pos = 17 ; key = fl_tol_val ; val = 1e-05 pos = 18 ; key = fl_w ; val = 0.44365 pos = 19 ; key = fl_w_max ; val = 50 pos = 20 ; key = fl_z_mu ; val = 0 pos = 21 ; key = fl_z_rho ; val = 0.8 pos = 22 ; key = fl_z_sig ; val = 0.2 pos = 23 ; key = it_ak_n ; val = 50 pos = 24 ; key = it_c_interp_grid_gap ; val = 0.0001 pos = 25 ; key = it_maxiter_dist ; val = 1000 pos = 26 ; key = it_maxiter_val ; val = 250 pos = 27 ; key = it_tol_pol_nochange ; val = 25 pos = 28 ; key = it_trans_power_dist ; val = 1000 pos = 29 ; key = it_w_n ; val = 50 pos = 30 ; key = it_z_n ; val = 15 pos = 31 ; key = st_analytical_stationary_type ; val = eigenvector pos = 32 ; key = st_model ; val = akz_wkz_iwkz ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Scalars in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx value __ ___ _______ bl_default 1 1 0 fl_Amean 2 2 1 fl_alpha 3 3 0.36 fl_b_bd 4 4 0 fl_beta 5 5 0.94 fl_c_min 6 6 0.001 fl_coh_interp_grid_gap 7 7 0.1 fl_crra 8 8 1.5 fl_default_aprime 9 9 0 fl_delta 10 10 0.08 fl_k_min 11 11 0 fl_nan_replace 12 12 -9999 fl_r_borr 13 13 0.025 fl_r_save 14 14 0.025 fl_tol_dist 15 15 1e-05 fl_tol_pol 16 16 1e-05 fl_tol_val 17 17 1e-05 fl_w 18 18 0.44365 fl_w_max 19 19 50 fl_z_mu 20 20 0 fl_z_rho 21 21 0.8 fl_z_sig 22 22 0.2 it_ak_n 23 23 50 it_c_interp_grid_gap 24 24 0.0001 it_maxiter_dist 25 25 1000 it_maxiter_val 26 26 250 it_tol_pol_nochange 27 27 25 it_trans_power_dist 28 28 1000 it_w_n 29 29 50 it_z_n 30 30 15 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Strings in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx _ ___ st_analytical_stationary_type 1 31 st_model 2 32
Display 4 func_map
fft_container_map_display(func_map, it_display_summmat_rowmax, it_display_summmat_colmax);
---------------------------------------- ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Begin: Show all key and value pairs from container CONTAINER NAME: FUNC_MAP ---------------------------------------- Map with properties: Count: 7 KeyType: char ValueType: any xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ---------------------------------------- ---------------------------------------- pos = 1 ; key = f_coh ; val = @(z,b,k)(f_prod(z,k)+k*(1-fl_delta)+fl_w+b.*(1+fl_r_save).*(b>0)+b.*(1+fl_r_borr).*(b<=0)) pos = 2 ; key = f_cons ; val = @(coh,bprime,kprime)(coh-kprime-bprime) pos = 3 ; key = f_inc ; val = @(z,b,k)(f_prod(z,k)-(fl_delta)*k+fl_w+b.*(fl_r_save).*(b>0)+b.*(fl_r_borr).*(b<=0)) pos = 4 ; key = f_prod ; val = @(z,k)((fl_Amean.*(z)).*(k.^(fl_alpha))) pos = 5 ; key = f_util_crra ; val = @(c)(((c).^(1-fl_crra)-1)./(1-fl_crra)) pos = 6 ; key = f_util_log ; val = @(c)log(c) pos = 7 ; key = f_util_standin ; val = @(z,b,k)f_util_log(f_coh(z,b,k).*(f_coh(z,b,k)>0)+fl_c_min.*(f_coh(z,b,k)<=0)) ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Scalars in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx xFunction _ ___ _________ f_coh 1 1 1 f_cons 2 2 2 f_inc 3 3 3 f_prod 4 4 4 f_util_crra 5 5 5 f_util_log 6 6 6 f_util_standin 7 7 7
Display 5 result_map
fft_container_map_display(result_map, it_display_summmat_rowmax, it_display_summmat_colmax);
---------------------------------------- ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Begin: Show all key and value pairs from container CONTAINER NAME: RESULT_MAP ---------------------------------------- Map with properties: Count: 13 KeyType: char ValueType: any xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ---------------------------------------- ---------------------------------------- pos = 1 ; key = ar_pol_diff_norm ;rown= 110 ,coln= 1 ar_pol_diff_norm :mu= 34.0952 ,sd= 167.118 ,min= 0 ,max= 1643.1596 zi_1_c1 _______ zi_1_R1 184.61 zi_2_R2 1643.2 zi_3_R3 546.99 zi_55_R55 1.0204 zi_108_r108 0 zi_109_r109 0 zi_110_r110 0 pos = 2 ; key = ar_st_pol_names ; val = cl_mt_coh cl_mt_pol_a cl_mt_pol_k cl_mt_pol_c pos = 3 ; key = ar_val_diff_norm ;rown= 110 ,coln= 1 ar_val_diff_norm :mu= 10.7296 ,sd= 23.3812 ,min= 0.041929 ,max= 142.9489 zi_1_c1 ________ zi_1_R1 142.95 zi_2_R2 111 zi_3_R3 92.611 zi_55_R55 1.2869 zi_108_r108 0.047454 zi_109_r109 0.044606 zi_110_r110 0.041929 pos = 4 ; key = cl_mt_coh ;rown= 568 ,coln= 15 cl_mt_coh :mu= 28.8604 ,sd= 16.4363 ,min= 0.44365 ,max= 57.2772 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 _______ _______ _______ _______ _________ _________ _________ zi_1_R1 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 zi_2_R2 0.54388 0.54388 0.54388 0.54388 0.54388 0.54388 0.54388 zi_3_R3 0.64412 0.64412 0.64412 0.64412 0.64412 0.64412 0.64412 zi_284_r284 28.81 28.81 28.81 28.81 28.81 28.81 28.81 zi_566_r566 57.077 57.077 57.077 57.077 57.077 57.077 57.077 zi_567_r567 57.177 57.177 57.177 57.177 57.177 57.177 57.177 zi_568_r568 57.277 57.277 57.277 57.277 57.277 57.277 57.277 pos = 5 ; key = cl_mt_cons ;rown= 568 ,coln= 15 cl_mt_cons :mu= 3.6107 ,sd= 1.3536 ,min= 0.024653 ,max= 7.2772 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 _______ _______ _______ _______ _________ _________ _________ zi_1_R1 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 zi_2_R2 0.54388 0.54388 0.54388 0.54388 0.54388 0.54388 0.54388 zi_3_R3 0.64412 0.64412 0.64412 0.64412 0.64412 0.64412 0.64412 zi_284_r284 3.3001 3.3001 3.3001 3.3001 4.3205 4.3205 4.3205 zi_566_r566 7.0767 7.0767 7.0767 7.0767 7.0767 7.0767 7.0767 zi_567_r567 7.1769 7.1769 7.1769 7.1769 7.1769 7.1769 7.1769 zi_568_r568 7.2772 7.2772 7.2772 7.2772 7.2772 7.2772 7.2772 pos = 6 ; key = cl_mt_pol_a ;rown= 568 ,coln= 15 cl_mt_pol_a :mu= 18.0175 ,sd= 14.6604 ,min= 0 ,max= 47.9592 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 _______ _______ _______ _______ _________ _________ _________ zi_1_R1 0 0 0 0 0 0 0 zi_2_R2 0 0 0 0 0 0 0 zi_3_R3 0 0 0 0 0 0 0 zi_284_r284 23.469 23.469 22.449 19.388 9.1837 6.1224 3.0612 zi_566_r566 47.959 47.959 46.939 43.878 34.694 31.633 28.571 zi_567_r567 47.959 47.959 46.939 43.878 34.694 31.633 28.571 zi_568_r568 47.959 47.959 46.939 43.878 34.694 31.633 28.571 pos = 7 ; key = cl_mt_pol_c ;rown= 568 ,coln= 15 cl_mt_pol_c :mu= 3.6107 ,sd= 1.3536 ,min= 0.024653 ,max= 7.2772 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 _______ _______ _______ _______ _________ _________ _________ zi_1_R1 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 zi_2_R2 0.54388 0.54388 0.54388 0.54388 0.54388 0.54388 0.54388 zi_3_R3 0.64412 0.64412 0.64412 0.64412 0.64412 0.64412 0.64412 zi_284_r284 3.3001 3.3001 3.3001 3.3001 4.3205 4.3205 4.3205 zi_566_r566 7.0767 7.0767 7.0767 7.0767 7.0767 7.0767 7.0767 zi_567_r567 7.1769 7.1769 7.1769 7.1769 7.1769 7.1769 7.1769 zi_568_r568 7.2772 7.2772 7.2772 7.2772 7.2772 7.2772 7.2772 pos = 8 ; key = cl_mt_pol_k ;rown= 568 ,coln= 15 cl_mt_pol_k :mu= 7.2322 ,sd= 5.5189 ,min= 0 ,max= 22.449 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 _______ _______ _______ _______ _________ _________ _________ zi_1_R1 0 0 0 0 0 0 0 zi_2_R2 0 0 0 0 0 0 0 zi_3_R3 0 0 0 0 0 0 0 zi_284_r284 2.0408 2.0408 3.0612 6.1224 15.306 18.367 21.429 zi_566_r566 2.0408 2.0408 3.0612 6.1224 15.306 18.367 21.429 zi_567_r567 2.0408 2.0408 3.0612 6.1224 15.306 18.367 21.429 zi_568_r568 2.0408 2.0408 3.0612 6.1224 15.306 18.367 21.429 pos = 9 ; key = mt_pol_perc_change ;rown= 110 ,coln= 15 mt_pol_perc_change :mu= 0.054135 ,sd= 0.15854 ,min= 0 ,max= 1 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 _______ _______ _______ __________ __________ __________ _________ zi_1_R1 1 1 1 1 1 1 1 zi_2_R2 0.9419 0.94102 0.92165 0.86092 0.67958 0.6206 0.55986 zi_3_R3 0.64789 0.64789 0.51408 0.57746 0.5713 0.53169 0.54577 zi_55_R55 0 0 0 0.00088028 0.00088028 0.00088028 0 zi_108_r108 0 0 0 0 0 0 0 zi_109_r109 0 0 0 0 0 0 0 zi_110_r110 0 0 0 0 0 0 0 pos = 10 ; key = mt_val ;rown= 568 ,coln= 15 mt_val :mu= 11.9863 ,sd= 4.2293 ,min= -16.6941 ,max= 16.6028 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 _______ _______ _______ _______ _________ _________ _________ zi_1_R1 -16.694 -16.694 -16.694 -16.694 -16.694 -16.694 -16.694 zi_2_R2 -16.403 -16.403 -16.403 -16.403 -16.403 -16.403 -16.403 zi_3_R3 -16.183 -16.183 -16.183 -16.183 -16.183 -16.183 -16.183 zi_284_r284 12.123 12.198 12.284 12.85 13.689 13.889 14.084 zi_566_r566 15.412 15.455 15.505 15.841 16.35 16.473 16.592 zi_567_r567 15.417 15.46 15.51 15.847 16.355 16.478 16.598 zi_568_r568 15.422 15.465 15.516 15.852 16.361 16.483 16.603 pos = 11 ; key = tb_pol_a ;rown= 100 ,coln= 12 tb_pol_a :mu= 20.1259 ,sd= 20.6339 ,min= 0 ,max= 47.9592 zi_1_C1 zi_2_C2 zi_3_C3 zi_6_C6 zi_10_c10 zi_11_c11 zi_12_c12 _______ _______ _______ _______ _________ _________ _________ zi_1_R1 0 0 0 0 0 0 0 zi_2_R2 0 0 0 0 0 0 0 zi_3_R3 0 0 0 0 0 0 0 zi_50_R50 2.0408 2.0408 1.0204 0 0 0 0 zi_98_R98 47.959 47.959 46.939 44.898 34.694 31.633 28.571 zi_99_R99 47.959 47.959 46.939 44.898 34.694 31.633 28.571 zi_100_r100 47.959 47.959 46.939 44.898 34.694 31.633 28.571 pos = 12 ; key = tb_val ;rown= 100 ,coln= 12 tb_val :mu= 9.3808 ,sd= 8.3155 ,min= -16.6941 ,max= 16.6028 zi_1_C1 zi_2_C2 zi_3_C3 zi_6_C6 zi_10_c10 zi_11_c11 zi_12_c12 _______ _______ _______ _______ _________ _________ _________ zi_1_R1 -16.694 -16.694 -16.694 -16.694 -16.694 -16.694 -16.694 zi_2_R2 -16.403 -16.403 -16.403 -16.403 -16.403 -16.403 -16.403 zi_3_R3 -16.183 -16.183 -16.183 -16.183 -16.183 -16.183 -16.183 zi_50_R50 5.4091 5.6303 5.8833 6.7563 9.0401 9.3952 9.7259 zi_98_R98 15.412 15.455 15.505 15.689 16.35 16.473 16.592 zi_99_R99 15.417 15.46 15.51 15.695 16.355 16.478 16.598 zi_100_r100 15.422 15.465 15.516 15.7 16.361 16.483 16.603 pos = 13 ; key = tb_valpol_alliter ;rown= 100 ,coln= 17 tb_valpol_alliter :mu= 2.9332 ,sd= 43.5993 ,min= 0 ,max= 1643.1596 zi_1_C1 zi_2_C2 zi_3_C3 zi_9_C9 zi_15_c15 zi_16_c16 zi_17_c17 ________ _______ __________ __________ _________ __________ __________ zi_1_R1 142.95 184.61 1 1 1 1 1 zi_2_R2 111 1643.2 0.9419 0.88028 0.67958 0.6206 0.55986 zi_3_R3 92.611 546.99 0.64789 0.62764 0.5713 0.53169 0.54577 zi_50_R50 1.7728 1.0204 0.00088028 0.00088028 0 0.00088028 0.00088028 zi_98_R98 0.047454 0 0 0 0 0 0 zi_99_R99 0.044606 0 0 0 0 0 0 zi_100_r100 0.041929 0 0 0 0 0 0 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Matrix in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx rowN colN mean std min max __ ___ ____ ____ ________ _______ ________ ______ ar_pol_diff_norm 1 1 110 1 34.095 167.12 0 1643.2 ar_val_diff_norm 2 3 110 1 10.73 23.381 0.041929 142.95 cl_mt_coh 3 4 568 15 28.86 16.436 0.44365 57.277 cl_mt_cons 4 5 568 15 3.6107 1.3536 0.024653 7.2772 cl_mt_pol_a 5 6 568 15 18.018 14.66 0 47.959 cl_mt_pol_c 6 7 568 15 3.6107 1.3536 0.024653 7.2772 cl_mt_pol_k 7 8 568 15 7.2322 5.5189 0 22.449 mt_pol_perc_change 8 9 110 15 0.054135 0.15854 0 1 mt_val 9 10 568 15 11.986 4.2293 -16.694 16.603 tb_pol_a 10 11 100 12 20.126 20.634 0 47.959 tb_val 11 12 100 12 9.3808 8.3155 -16.694 16.603 tb_valpol_alliter 12 13 100 17 2.9332 43.599 0 1643.2 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Strings in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx _ ___ ar_st_pol_names 1 2
end
end
ans = Map with properties: Count: 13 KeyType: char ValueType: any