Risky + Safe Asset Dyna Prog Concurrent Solution (Vectorized)
back to Fan's Dynamic Assets Repository Table of Content.
Contents
- FF_AKZ_VF_VEC solve infinite horizon exo shock + endo asset problem
- Default
- Parse Parameters 1
- Parse Parameters 2
- Initialize Output Matrixes
- Initialize Convergence Conditions
- Iterate Value Function
- Solve Optimization Problem Current Iteration
- Check Tolerance and Continuation
- Process Optimal Choices
- Display Various Containers
- Display 1 support_map
- Display 2 armt_map
- Display 3 param_map
- Display 4 func_map
- Display 5 result_map
function result_map = ff_akz_vf_vec(varargin)
FF_AKZ_VF_VEC solve infinite horizon exo shock + endo asset problem
This program solves the infinite horizon dynamic savings and risky capital asset problem with some ar1 shock. This is the vectorized version of ff_akz_vf. See that file for more descriptions.
@param param_map container parameter container
@param support_map container support container
@param armt_map container container with states, choices and shocks grids that are inputs for grid based solution algorithm
@param func_map container container with function handles for consumption cash-on-hand etc.
@return result_map container contains policy function matrix, value function matrix, iteration results, and policy function, value function and iteration results tables.
keys included in result_map:
- mt_val matrix states_n by shock_n matrix of converged value function grid
- mt_pol_a matrix states_n by shock_n matrix of converged policy function grid safe asset
- mt_pol_k matrix states_n by shock_n matrix of converged policy function grid risky asset
- ar_val_diff_norm array if bl_post = true it_iter_last by 1 val function difference between iteration
- ar_pol_diff_norm array if bl_post = true it_iter_last by 1 policy function difference between iterations
- mt_pol_perc_change matrix if bl_post = true it_iter_last by shock_n the proportion of grid points at which policy function changed between current and last iteration for each element of shock
@example
it_param_set = 2; [param_map, support_map] = ffs_akz_set_default_param(it_param_set); % Simulation Accuracy param_map('it_w_n') = 750; param_map('it_ak_n') = param_map('it_w_n'); param_map('it_z_n') = 11; % Display Parameters support_map('bl_display') = false; support_map('bl_display_final') = false; support_map('bl_time') = true; support_map('bl_profile') = false; % Call Program with external parameters that override defaults ff_akz_vf_vec(param_map, support_map);
@include
@seealso
- concurrent (safe + risky) loop: ff_akz_vf
- concurrent (safe + risky) vectorized: ff_akz_vf_vec
- concurrent (safe + risky) optimized-vectorized: ff_akz_vf_vecsv
- two-stage (safe + risky) loop: ff_wkz_vf
- two-stage (safe + risky) vectorized: ff_wkz_vf_vec
- two-stage (safe + risky) optimized-vectorized: ff_wkz_vf_vecsv
- two-stage + interpolate (safe + risky) loop: ff_iwkz_vf
- two-stage + interpolate (safe + risky) vectorized: ff_iwkz_vf_vec
- two-stage + interpolate (safe + risky) optimized-vectorized: ff_iwkz_vf_vecsv
Default
- it_param_set = 1: quick test
- it_param_set = 2: benchmark run
- it_param_set = 3: benchmark profile
- it_param_set = 4: press publish button
it_param_set = 4; [param_map, support_map] = ffs_akz_set_default_param(it_param_set); % Note: param_map and support_map can be adjusted here or outside to override defaults % param_map('it_w_n') = 50; % param_map('it_z_n') = 15; % get armt and func map [armt_map, func_map] = ffs_akz_get_funcgrid(param_map, support_map); % 1 for override default_params = {param_map support_map armt_map func_map};
Parse Parameters 1
% if varargin only has param_map and support_map, params_len = length(varargin); [default_params{1:params_len}] = varargin{:}; param_map = [param_map; default_params{1}]; support_map = [support_map; default_params{2}]; if params_len >= 1 && params_len <= 2 % If override param_map, re-generate armt and func if they are not % provided [armt_map, func_map] = ffs_akz_get_funcgrid(param_map, support_map); else % Override all armt_map = [armt_map; default_params{3}]; func_map = [func_map; default_params{4}]; end % append function name st_func_name = 'ff_akz_vf_vec'; support_map('st_profile_name_main') = [st_func_name support_map('st_profile_name_main')]; support_map('st_mat_name_main') = [st_func_name support_map('st_mat_name_main')]; support_map('st_img_name_main') = [st_func_name support_map('st_img_name_main')];
Parse Parameters 2
% armt_map params_group = values(armt_map, {'mt_z_trans', 'ar_z'}); [ mt_z_trans, ar_z] = params_group{:}; params_group = values(armt_map, {'ar_a_meshk', 'ar_k_mesha', 'mt_coh_wkb', 'it_ameshk_n'}); [ar_a_meshk, ar_k_mesha, mt_coh_wkb, it_ameshk_n] = params_group{:}; % func_map params_group = values(func_map, {'f_util_log', 'f_util_crra', 'f_cons', 'f_coh'}); [f_util_log, f_util_crra, f_cons, f_coh] = params_group{:}; % param_map params_group = values(param_map, {'it_z_n', 'fl_crra', 'fl_beta', 'fl_c_min'}); [it_z_n, fl_crra, fl_beta, fl_c_min] = params_group{:}; params_group = values(param_map, {'it_maxiter_val', 'fl_tol_val', 'fl_tol_pol', 'it_tol_pol_nochange'}); [it_maxiter_val, fl_tol_val, fl_tol_pol, it_tol_pol_nochange] = params_group{:}; % support_map params_group = values(support_map, {'bl_profile', 'st_profile_path', ... 'st_profile_prefix', 'st_profile_name_main', 'st_profile_suffix',... 'bl_time', 'bl_display_defparam', 'bl_display', 'it_display_every', 'bl_post'}); [bl_profile, st_profile_path, ... st_profile_prefix, st_profile_name_main, st_profile_suffix, ... bl_time, bl_display_defparam, bl_display, it_display_every, bl_post] = params_group{:}; params_group = values(support_map, {'it_display_summmat_rowmax', 'it_display_summmat_colmax'}); [it_display_summmat_rowmax, it_display_summmat_colmax] = params_group{:};
Initialize Output Matrixes
mt_val_cur = zeros(length(ar_a_meshk),length(ar_z)); mt_val = mt_val_cur - 1; mt_pol_a = zeros(length(ar_a_meshk),length(ar_z)); mt_pol_a_cur = mt_pol_a - 1; mt_pol_k = zeros(length(ar_a_meshk),length(ar_z)); mt_pol_k_cur = mt_pol_k - 1; mt_pol_idx = zeros(length(ar_a_meshk),length(ar_z));
Initialize Convergence Conditions
bl_vfi_continue = true; it_iter = 0; ar_val_diff_norm = zeros([it_maxiter_val, 1]); ar_pol_diff_norm = zeros([it_maxiter_val, 1]); mt_pol_perc_change = zeros([it_maxiter_val, it_z_n]);
Iterate Value Function
Loop solution with 4 nested loops
- loop 1: over exogenous states
- loop 2: over endogenous states
- loop 3: over choices
- loop 4: add future utility, integration--loop over future shocks
% Start Profile if (bl_profile) close all; profile off; profile on; end % Start Timer if (bl_time) tic; end % Value Function Iteration while bl_vfi_continue
it_iter = it_iter + 1;
Solve Optimization Problem Current Iteration
% loop 1: over exogenous states for it_z_i = 1:length(ar_z) % Consumption mt_c = f_cons(mt_coh_wkb(:, it_z_i)', ar_a_meshk, ar_k_mesha); % EVAL current utility: N by N, f_util defined earlier if (fl_crra == 1) mt_utility = f_util_log(mt_c); fl_u_neg_c = f_util_log(fl_c_min); else mt_utility = f_util_crra(mt_c); fl_u_neg_c = f_util_crra(fl_c_min); end % f(z'|z) ar_z_trans_condi = mt_z_trans(it_z_i,:); % EVAL EV((A',K'),Z'|Z) = V((A',K'),Z') x p(z'|z)', (N by Z) x (Z by 1) = N by 1 mt_evzp_condi_z = mt_val_cur * ar_z_trans_condi'; % EVAL add on future utility, N by N + N by 1 mt_utility = mt_utility + fl_beta*mt_evzp_condi_z; mt_utility(mt_c <= fl_c_min) = fl_u_neg_c; % Optimization: remember matlab is column major, rows must be % choices, columns must be states % <https://en.wikipedia.org/wiki/Row-_and_column-major_order COLUMN-MAJOR> [ar_opti_val1_z, ar_opti_idx_z] = max(mt_utility); mt_val(:,it_z_i) = ar_opti_val1_z; mt_pol_a(:,it_z_i) = ar_a_meshk(ar_opti_idx_z); mt_pol_k(:,it_z_i) = ar_k_mesha(ar_opti_idx_z); if (it_iter == (it_maxiter_val + 1)) mt_pol_idx(:,it_z_i) = ar_opti_idx_z; end end
Check Tolerance and Continuation
% Difference across iterations ar_val_diff_norm(it_iter) = norm(mt_val - mt_val_cur); ar_pol_diff_norm(it_iter) = norm(mt_pol_a - mt_pol_a_cur) + norm(mt_pol_k - mt_pol_k_cur); ar_pol_a_perc_change = sum((mt_pol_a ~= mt_pol_a_cur))/(it_ameshk_n); ar_pol_k_perc_change = sum((mt_pol_k ~= mt_pol_k_cur))/(it_ameshk_n); mt_pol_perc_change(it_iter, :) = mean([ar_pol_a_perc_change;ar_pol_k_perc_change]); % Update mt_val_cur = mt_val; mt_pol_a_cur = mt_pol_a; mt_pol_k_cur = mt_pol_k; % Print Iteration Results if (bl_display && (rem(it_iter, it_display_every)==0)) fprintf('VAL it_iter:%d, fl_diff:%d, fl_diff_pol:%d\n', ... it_iter, ar_val_diff_norm(it_iter), ar_pol_diff_norm(it_iter)); tb_valpol_iter = array2table([mean(mt_val_cur,1);... mean(mt_pol_a_cur,1); ... mean(mt_pol_k_cur,1); ... mt_val_cur(it_ameshk_n,:); ... mt_pol_a_cur(it_ameshk_n,:); ... mt_pol_k_cur(it_ameshk_n,:)]); tb_valpol_iter.Properties.VariableNames = strcat('z', string((1:size(mt_val_cur,2)))); tb_valpol_iter.Properties.RowNames = {'mval', 'map', 'mak', 'Hval', 'Hap', 'Hak'}; disp('mval = mean(mt_val_cur,1), average value over a') disp('map = mean(mt_pol_a_cur,1), average choice over a') disp('mkp = mean(mt_pol_k_cur,1), average choice over k') disp('Hval = mt_val_cur(it_ameshk_n,:), highest a state val') disp('Hap = mt_pol_a_cur(it_ameshk_n,:), highest a state choice') disp('mak = mt_pol_k_cur(it_ameshk_n,:), highest k state choice') disp(tb_valpol_iter); end % Continuation Conditions: % 1. if value function convergence criteria reached % 2. if policy function variation over iterations is less than % threshold if (it_iter == (it_maxiter_val + 1)) bl_vfi_continue = false; elseif ((it_iter == it_maxiter_val) || ... (ar_val_diff_norm(it_iter) < fl_tol_val) || ... (sum(ar_pol_diff_norm(max(1, it_iter-it_tol_pol_nochange):it_iter)) < fl_tol_pol)) % Fix to max, run again to save results if needed it_iter_last = it_iter; it_iter = it_maxiter_val; end
end % End Timer if (bl_time) toc; end % End Profile if (bl_profile) profile off profile viewer st_file_name = [st_profile_prefix st_profile_name_main st_profile_suffix]; profsave(profile('info'), strcat(st_profile_path, st_file_name)); end
Process Optimal Choices
result_map = containers.Map('KeyType','char', 'ValueType','any'); result_map('mt_val') = mt_val; mt_coh = f_coh(ar_z, ar_a_meshk, ar_k_mesha); result_map('cl_mt_coh') = {mt_coh, zeros(1)}; result_map('cl_mt_pol_a') = {mt_pol_a, zeros(1)}; result_map('cl_mt_pol_k') = {mt_pol_k, zeros(1)}; result_map('cl_mt_pol_c') = {f_cons(mt_coh, mt_pol_a, mt_pol_k), zeros(1)}; result_map('ar_st_pol_names') = ["cl_mt_coh", "cl_mt_pol_a", "cl_mt_pol_k", "cl_mt_pol_c"]; if (bl_post) bl_input_override = true; result_map('ar_val_diff_norm') = ar_val_diff_norm(1:it_iter_last); result_map('ar_pol_diff_norm') = ar_pol_diff_norm(1:it_iter_last); result_map('mt_pol_perc_change') = mt_pol_perc_change(1:it_iter_last, :); result_map = ff_akz_vf_post(param_map, support_map, armt_map, func_map, result_map, bl_input_override); end
valgap = norm(mt_val - mt_val_cur): value function difference across iterations polgap = norm(mt_pol_a - mt_pol_a_cur): policy function difference across iterations z1 = z1 perc change: (sum((mt_pol_a ~= mt_pol_a_cur))+sum((mt_pol_k ~= mt_pol_k_cur)))/(2*it_ameshk_n):percentage of state space points conditional on shock where the policy function is changing across iterations valgap polgap z1 z2 z3 z4 z5 z6 z7 z8 z9 z10 z11 z12 z13 z14 z15 _______ ______ __________ __________ __________ __________ __________ __________ __________ __________ __________ __________ __________ __________ __________ __________ __________ iter=1 227.04 276.59 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 iter=2 179.64 2790.4 0.99137 0.99137 0.98549 0.98549 0.97804 0.97804 0.96902 0.95804 0.94588 0.92039 0.88745 0.84824 0.80157 0.72 0.63529 iter=3 151.16 920.98 0.65176 0.65098 0.52314 0.52275 0.61882 0.62196 0.71686 0.62275 0.89216 0.51608 0.69725 0.54235 0.63294 0.5949 0.62627 iter=4 130.1 448.85 0.57725 0.57922 0.49608 0.49608 0.55608 0.57137 0.6251 0.52157 0.78431 0.48863 0.63765 0.50039 0.59569 0.50784 0.51647 iter=5 113.5 266.36 0.53608 0.53569 0.4749 0.47529 0.55882 0.59333 0.58627 0.49137 0.71569 0.47176 0.56196 0.48588 0.51529 0.47686 0.48863 iter=6 99.938 188.1 0.46471 0.46471 0.42588 0.42549 0.46627 0.5 0.54431 0.44627 0.73922 0.43647 0.54745 0.46471 0.47412 0.44902 0.45412 iter=7 88.609 140.12 0.37294 0.37569 0.35176 0.35412 0.39608 0.43098 0.46471 0.37333 0.62392 0.37647 0.50431 0.40941 0.41451 0.40314 0.40824 iter=8 79 120.94 0.27137 0.27412 0.26275 0.2651 0.28627 0.33098 0.32745 0.28588 0.73373 0.29059 0.42902 0.34549 0.33255 0.3298 0.33569 iter=9 70.745 98.728 0.2051 0.20667 0.20078 0.2051 0.22235 0.26471 0.26 0.21529 0.72157 0.21961 0.36275 0.3102 0.25176 0.25294 0.27059 iter=10 63.581 88.163 0.16118 0.16431 0.15412 0.15686 0.1698 0.21098 0.20275 0.17725 0.69804 0.17804 0.27686 0.32588 0.20471 0.21725 0.21922 iter=11 57.334 71.859 0.13059 0.12863 0.12431 0.12431 0.13255 0.16235 0.15529 0.13137 0.61412 0.14353 0.25412 0.26549 0.16431 0.16392 0.17216 iter=12 51.832 69.137 0.098431 0.10039 0.096863 0.096078 0.10902 0.12314 0.13569 0.12431 0.57569 0.11725 0.20902 0.21451 0.13451 0.14941 0.15333 iter=13 46.97 54.289 0.086275 0.086275 0.083529 0.086275 0.093725 0.12431 0.11059 0.092157 0.43373 0.094902 0.16745 0.18824 0.11882 0.10941 0.12196 iter=14 42.649 50.95 0.074118 0.074902 0.071765 0.072941 0.080784 0.13922 0.087059 0.07451 0.42902 0.082353 0.12784 0.11647 0.097255 0.099216 0.10039 iter=15 38.799 44.356 0.056471 0.055686 0.05451 0.055294 0.064314 0.20196 0.073725 0.071373 0.29569 0.073725 0.15098 0.083137 0.072549 0.090588 0.094118 iter=16 35.368 47.104 0.047451 0.049412 0.045882 0.047843 0.051765 0.23922 0.067451 0.065882 0.32314 0.060784 0.15451 0.094902 0.069804 0.072549 0.086275 iter=17 32.283 40.36 0.042353 0.043529 0.043137 0.042353 0.047059 0.25137 0.058039 0.052941 0.22431 0.049804 0.12667 0.088627 0.060784 0.061176 0.061569 iter=18 29.497 32.552 0.040784 0.040392 0.035686 0.041176 0.041569 0.13647 0.04902 0.042353 0.19529 0.042745 0.1302 0.088235 0.058039 0.051765 0.053333 iter=19 26.977 34.912 0.034118 0.033725 0.038431 0.035686 0.039216 0.11608 0.041961 0.035686 0.21686 0.039608 0.11961 0.057647 0.056471 0.045098 0.04902 iter=20 24.704 35.339 0.026275 0.031373 0.027451 0.030588 0.03098 0.19216 0.033333 0.027843 0.21529 0.034902 0.074902 0.040392 0.04 0.045098 0.063529 iter=21 22.656 25.724 0.026275 0.024706 0.024706 0.024706 0.023529 0.1102 0.026275 0.027059 0.12745 0.034902 0.049804 0.04902 0.032157 0.043529 0.081961 iter=22 20.807 24.188 0.019216 0.018431 0.020392 0.016863 0.021176 0.06902 0.025098 0.023922 0.1098 0.028627 0.083922 0.031765 0.027059 0.038431 0.08 iter=23 19.13 28.633 0.018824 0.017647 0.016863 0.019608 0.018824 0.10941 0.021569 0.021176 0.15098 0.021569 0.089804 0.038824 0.025098 0.02902 0.073333 iter=24 17.602 21.949 0.013725 0.016863 0.014902 0.014118 0.016863 0.087843 0.021961 0.018824 0.092549 0.02 0.087451 0.03451 0.021176 0.021961 0.065098 iter=25 16.207 24.666 0.014902 0.013333 0.013725 0.014118 0.013725 0.10863 0.01451 0.017255 0.11686 0.019216 0.043529 0.031373 0.022745 0.021569 0.043137 iter=26 14.932 25.232 0.011373 0.010588 0.012941 0.012549 0.016471 0.070588 0.016863 0.016078 0.12353 0.012941 0.036078 0.039216 0.016863 0.018431 0.061961 iter=27 13.764 21.592 0.01098 0.013333 0.0090196 0.011373 0.012157 0.089412 0.015294 0.014902 0.055686 0.012549 0.04 0.019216 0.016863 0.015294 0.061961 iter=28 12.694 21.639 0.0090196 0.010588 0.010196 0.010588 0.012941 0.087451 0.012941 0.01451 0.089412 0.011765 0.041176 0.014118 0.015686 0.01451 0.038039 iter=29 11.714 23.752 0.0086275 0.0082353 0.0082353 0.0070588 0.0098039 0.072157 0.012941 0.011765 0.10549 0.012549 0.033333 0.01451 0.016078 0.011765 0.062745 iter=30 10.819 19.707 0.0070588 0.0062745 0.0078431 0.007451 0.0070588 0.072941 0.010588 0.012549 0.013333 0.0098039 0.014118 0.011373 0.01451 0.01098 0.038431 iter=31 10.001 20.629 0.0078431 0.005098 0.0047059 0.0082353 0.0058824 0.065098 0.0078431 0.0094118 0.081569 0.0082353 0.011765 0.011373 0.01098 0.012549 0.037255 iter=32 9.2538 18.997 0.0039216 0.0058824 0.0058824 0.0035294 0.010196 0.027451 0.010588 0.0082353 0.070196 0.0094118 0.0094118 0.021569 0.013333 0.010196 0.037647 iter=33 8.5706 18.069 0.0043137 0.0047059 0.0023529 0.0054902 0.0043137 0.044314 0.0062745 0.0054902 0.033725 0.0078431 0.010196 0.0070588 0.011765 0.0090196 0.061569 iter=34 7.9453 10.089 0.0031373 0.0047059 0.007451 0.0062745 0.0035294 0.0070588 0.0062745 0.0047059 0.019608 0.0035294 0.0086275 0.0066667 0.010196 0.0098039 0.0094118 iter=35 7.3729 16.84 0.0027451 0.0031373 0.0023529 0.0027451 0.0058824 0.032157 0.0070588 0.0054902 0.05451 0.0066667 0.0062745 0.007451 0.0070588 0.0090196 0.037647 iter=36 6.8481 13.579 0.0039216 0.0027451 0.0019608 0.0015686 0.0043137 0.027451 0.0043137 0.0039216 0.0031373 0.005098 0.0094118 0.0039216 0.0090196 0.005098 0.034902 iter=37 6.3662 14.429 0.0015686 0.0027451 0.0035294 0.0039216 0.0019608 0.020392 0.0062745 0.0023529 0.039216 0.0031373 0.0043137 0.020392 0.0047059 0.007451 0.034118 iter=38 5.923 4.8384 0.0023529 0.0015686 0 0.0019608 0.0019608 0.0031373 0.0031373 0.0035294 0.0023529 0.0035294 0.0043137 0.0023529 0.0054902 0.0043137 0.0035294 iter=39 5.515 13.871 0.00078431 0.00078431 0.0031373 0.00039216 0.0035294 0.0031373 0.0023529 0.0019608 0.0027451 0.0019608 0.0047059 0.0039216 0.0070588 0.0043137 0.036471 iter=40 5.1387 4.6995 0.0015686 0.0011765 0.0023529 0.0019608 0.0031373 0.0015686 0.0011765 0.0019608 0.00039216 0.0031373 0.0027451 0.0039216 0.0027451 0.005098 0.0043137 iter=41 4.7913 14.522 0.00078431 0.0023529 0.0019608 0.0023529 0.0019608 0.0031373 0.0015686 0.00078431 0.04 0.0031373 0.0031373 0.0015686 0.0035294 0.0039216 0.035294 iter=42 4.4701 11.807 0.0023529 0.0011765 0.00078431 0.00078431 0.00078431 0.0011765 0.0031373 0.0023529 0.026667 0.0015686 0.0011765 0.0011765 0.0023529 0.0027451 0.0047059 iter=43 4.1728 5.4465 0.00039216 0.00039216 0.00078431 0.0011765 0.0011765 0.0023529 0.00078431 0.0015686 0.0023529 0.00078431 0.0031373 0.0043137 0.0035294 0.0043137 0.0054902 iter=44 3.8974 12.575 0.00039216 0.00078431 0.0011765 0.0015686 0.00078431 0.00078431 0.0015686 0.00039216 0.0019608 0.0019608 0.0011765 0.0031373 0.0015686 0.0023529 0.029804 iter=45 3.6419 13.302 0 0 0.00078431 0.00039216 0.00039216 0 0.00039216 0.0015686 0.00039216 0.0011765 0.00039216 0.00078431 0.00078431 0.0031373 0.033333 iter=46 3.4048 2.4995 0 0.0011765 0 0.0011765 0 0.0023529 0.00039216 0 0.0027451 0.00039216 0.00078431 0.0015686 0.0015686 0.0015686 0.0011765 iter=47 3.1844 3.2577 0.00039216 0.00039216 0 0 0.00078431 0.0019608 0.0011765 0.00039216 0 0.00039216 0.0015686 0.00078431 0.0011765 0.0019608 0.0039216 iter=48 2.9795 2.7878 0.0011765 0.00039216 0 0.00039216 0.00078431 0.00078431 0.0011765 0 0.00039216 0.0011765 0.00039216 0.00039216 0.00039216 0.0015686 0.0011765 iter=49 2.7887 2.2817 0.00039216 0 0.00039216 0.00078431 0.0015686 0.00039216 0.0019608 0.0011765 0 0.00078431 0.0011765 0.00078431 0.0019608 0.0011765 0.0019608 iter=50 2.611 14.289 0 0.00078431 0.00039216 0.00039216 0.00039216 0 0.00039216 0.00078431 0.038431 0.00078431 0.00039216 0.0011765 0.00078431 0.00078431 0.00078431 iter=51 2.4454 3.7202 0.00039216 0 0 0 0.00078431 0.00039216 0.00039216 0.00039216 0.00039216 0.00039216 0.00039216 0 0.0015686 0.0011765 0.0019608 iter=52 2.291 3.3021 0 0 0.00039216 0.00039216 0.00078431 0.0011765 0 0.00078431 0.00039216 0 0 0.00078431 0 0.0019608 0.0011765 iter=53 2.1469 4.5043 0.00039216 0 0.00039216 0.00039216 0 0.00078431 0 0 0.0019608 0.0015686 0.00078431 0.00039216 0.0011765 0.00039216 0 iter=54 2.0123 2.2817 0 0 0 0.00078431 0.00039216 0 0 0 0 0.00039216 0.00078431 0.0011765 0.00078431 0.00078431 0.0019608 iter=55 1.8866 13.458 0 0.00039216 0.00078431 0 0.0011765 0 0.00039216 0.00039216 0 0.00039216 0.00078431 0 0 0.00078431 0.034118 iter=56 1.7691 18.926 0.00039216 0 0 0 0 0 0 0 0.00078431 0.00078431 0.00039216 0 0 0.00078431 0.067451 iter=57 1.6593 1.0204 0 0.00039216 0 0 0 0.00039216 0 0 0.00039216 0 0 0.00039216 0 0 0 iter=58 1.5565 1.0204 0.00039216 0 0 0.00039216 0 0 0 0 0 0 0 0.00078431 0 0 0 iter=59 1.4603 1.4431 0 0 0 0 0 0.00039216 0.00039216 0 0.00078431 0 0 0.00039216 0.00039216 0 0 iter=60 1.3703 1.7674 0.00039216 0.00039216 0 0 0 0.00078431 0 0 0 0 0.0011765 0 0 0 0 iter=61 1.2861 1.4431 0 0 0 0 0 0.00039216 0.00039216 0 0 0.00039216 0.00078431 0 0 0 0 iter=62 1.2071 1.4431 0 0 0.00039216 0 0 0 0 0 0 0 0 0 0 0.00078431 0 iter=63 1.1332 1.0204 0 0 0 0 0 0.00039216 0 0 0 0 0 0 0 0 0 iter=64 1.0639 1.0204 0.00039216 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=65 0.99889 1.0204 0 0 0 0 0 0 0 0 0 0.00039216 0.00039216 0 0 0 0 iter=66 0.93798 1.0204 0 0 0 0.00039216 0 0 0 0 0 0.00039216 0 0 0 0.00039216 0 iter=67 0.88086 1.0204 0 0 0 0 0 0 0 0 0 0 0 0 0 0.00039216 0.00039216 iter=68 0.82728 1.0204 0 0 0 0 0 0 0 0 0 0 0 0.00039216 0 0 0.00039216 iter=69 0.77702 1.0204 0.00039216 0 0 0 0 0.00039216 0 0 0 0 0 0 0 0 0 iter=70 0.72986 1.0204 0 0 0 0 0 0 0 0 0 0 0 0 0 0.00039216 0.00039216 iter=71 0.6856 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=72 0.64407 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=73 0.60508 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=74 0.56848 1.0204 0 0 0 0 0 0 0 0 0 0.00039216 0 0 0 0 0 iter=75 0.53411 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=76 0.50184 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=77 0.47154 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=78 0.44308 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=79 0.41636 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=80 0.39125 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=81 0.36767 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=82 0.34552 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=83 0.32471 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=84 0.30516 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=85 0.28679 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=86 0.26953 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=87 0.25331 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=88 0.23808 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=89 0.22376 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=90 0.2103 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=91 0.19766 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=92 0.18578 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=93 0.17461 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=94 0.16412 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=95 0.15426 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=96 0.14499 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=97 0.13628 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=98 0.12809 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=99 0.1204 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=100 0.11317 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 tb_val: V(a,z) value at each state space point z1_0_34741 z2_0_40076 z3_0_4623 z4_0_5333 z5_0_61519 z6_0_70966 z10_1_2567 z11_1_4496 z12_1_6723 z13_1_9291 z14_2_2253 z15_2_567 __________ __________ _________ _________ __________ __________ __________ __________ __________ __________ __________ _________ coh1:k=0,b=0 -16.679 -16.679 -16.679 -16.679 -16.679 -16.679 -16.679 -16.679 -16.679 -16.679 -16.679 -16.679 coh2:k=1.02041,b=0 1.6951 1.9479 2.2271 2.5214 2.8265 3.1427 4.6343 5.0876 5.5573 6.0229 6.4636 6.8568 coh3:k=0,b=1.02041 2.2448 2.5822 2.9487 3.3322 3.7278 4.1353 6.0791 6.7832 7.4306 8.0338 8.5908 9.0897 coh4:k=2.04082,b=0 2.9914 3.2625 3.5574 3.8626 4.1718 4.4834 5.9294 6.3827 6.8524 7.3179 7.7586 8.1519 coh5:k=1.02041,b=1.02041 3.5061 3.8576 4.2358 4.6264 5.0226 5.4221 7.0898 7.5454 8.0444 8.6067 9.1318 9.6083 coh6:k=0,b=2.04082 3.4934 3.8679 4.2667 4.6754 5.0871 5.4996 7.1962 7.7648 8.3175 8.8438 9.3405 9.8339 coh7:k=3.06122,b=0 4.2237 4.4947 4.7897 5.0949 5.4041 5.7157 7.0339 7.4054 7.7937 8.1869 8.569 8.919 coh8:k=2.04082,b=1.02041 4.3374 4.6305 4.9498 5.3357 5.7345 6.1368 7.7599 8.1816 8.6138 9.051 9.5545 10.014 coh9:k=1.02041,b=2.04082 4.334 4.6334 4.9765 5.3827 5.7967 6.2118 7.864 8.2883 8.7762 9.281 9.7589 10.197 coh10:k=0,b=3.06122 4.3183 4.623 4.9609 5.3839 5.8119 6.2385 7.9162 8.3803 8.8955 9.3883 9.856 10.354 coh11:k=4.08163,b=0 4.9498 5.1987 5.4824 5.7892 6.1044 6.4219 7.7053 8.0447 8.3966 8.7558 9.1106 9.4413 coh12:k=3.06122,b=1.02041 5.0609 5.3315 5.6389 5.9718 6.3154 6.6635 8.246 8.6521 9.0659 9.4844 9.9108 10.356 coh13:k=2.04082,b=2.04082 5.0576 5.3343 5.6481 5.9875 6.3377 6.7307 8.348 8.7568 9.172 9.6484 10.111 10.536 coh14:k=1.02041,b=3.06122 5.0423 5.3242 5.6432 5.9879 6.3465 6.7567 8.3992 8.8106 9.2765 9.7536 10.207 10.653 coh15:k=0,b=4.08163 5.0203 5.3072 5.6313 5.981 6.3412 6.7626 8.4302 8.8525 9.346 9.8176 10.266 10.75 coh16:k=5.10204,b=0 5.5962 5.816 6.0667 6.3398 6.6306 6.9351 8.1927 8.5182 8.8533 9.1951 9.5337 9.8508 coh17:k=4.08163,b=1.02041 5.7049 5.9458 6.2199 6.5185 6.8372 7.1718 8.6475 9.0427 9.4435 9.848 10.247 10.655 coh18:k=3.06122,b=2.04082 5.7016 5.9486 6.2289 6.5339 6.8591 7.2002 8.7473 9.1454 9.5478 9.9687 10.418 10.832 coh19:k=2.04082,b=3.06122 5.6866 5.9387 6.2241 6.5343 6.8646 7.2107 8.7977 9.1984 9.6082 10.072 10.512 10.92 coh20:k=1.02041,b=4.08163 5.6651 5.9221 6.2124 6.5275 6.8625 7.2131 8.8281 9.2315 9.6762 10.135 10.571 11.016 coh21:k=0,b=5.10204 5.6387 5.9008 6.1961 6.5161 6.8557 7.2107 8.8479 9.254 9.7218 10.178 10.613 11.079 coh22:k=6.12245,b=0 6.1694 6.367 6.5911 6.8365 7.0995 7.3793 8.5954 8.9117 9.2353 9.5644 9.8905 10.205 coh23:k=5.10204,b=1.02041 6.2756 6.494 6.7409 7.0114 7.3017 7.6112 8.9965 9.3819 9.7719 10.165 10.552 10.922 coh24:k=4.08163,b=2.04082 6.2725 6.4967 6.7498 7.0266 7.3233 7.6391 9.0943 9.4828 9.8745 10.268 10.692 11.096 coh25:k=3.06122,b=3.06122 6.2577 6.487 6.7451 7.027 7.3287 7.6494 9.1437 9.5348 9.9281 10.355 10.785 11.181 coh26:k=2.04082,b=4.08163 6.2367 6.4707 6.7336 7.0203 7.3267 7.6518 9.1737 9.5674 9.9705 10.417 10.842 11.258 coh27:k=1.02041,b=5.10204 6.2109 6.4499 6.7176 7.009 7.32 7.6494 9.1931 9.5896 10.015 10.46 10.882 11.321 coh28:k=0,b=6.12245 6.181 6.4252 6.6981 6.9944 7.3099 7.6437 9.206 9.6053 10.047 10.491 10.918 11.366 coh29:k=7.14286,b=0 6.6824 6.8619 7.0655 7.289 7.5286 7.7849 8.9456 9.2589 9.5824 9.9115 10.238 10.543 coh30:k=6.12245,b=1.02041 6.7864 6.9862 7.2122 7.4603 7.7268 8.0122 9.3111 9.6849 10.064 10.447 10.824 11.182 coh31:k=5.10204,b=2.04082 6.7833 6.9889 7.2209 7.4752 7.7479 8.0396 9.4061 9.784 10.165 10.548 10.942 11.337 coh32:k=4.08163,b=3.06122 6.7689 6.9793 7.2162 7.4756 7.7533 8.0498 9.4546 9.8352 10.218 10.613 11.033 11.42 coh33:k=3.06122,b=4.08163 6.7482 6.9634 7.205 7.469 7.7512 8.0521 9.484 9.8673 10.252 10.674 11.09 11.482 coh34:k=2.04082,b=5.10204 6.723 6.943 7.1894 7.458 7.7447 8.0498 9.5031 9.8892 10.281 10.716 11.129 11.544 coh35:k=1.02041,b=6.12245 6.6938 6.9188 7.1702 7.4436 7.7348 8.0442 9.5158 9.9047 10.313 10.746 11.159 11.589 coh36:k=0,b=7.14286 6.6606 6.8912 7.1479 7.4263 7.7223 8.036 9.524 9.9158 10.336 10.77 11.19 11.623 coh37:k=8.16327,b=0 7.1458 7.3107 7.4979 7.7039 7.9242 8.1603 9.2863 9.5944 9.9086 10.227 10.543 10.839 coh38:k=7.14286,b=1.02041 7.2476 7.4324 7.6415 7.8717 8.1183 8.3831 9.6194 9.9597 10.33 10.703 11.072 11.422 coh39:k=6.12245,b=2.04082 7.2445 7.435 7.65 7.8863 8.1391 8.4101 9.6892 10.057 10.429 10.803 11.173 11.56 coh40:k=5.10204,b=3.06122 7.2304 7.4256 7.6455 7.8867 8.1443 8.4201 9.7368 10.107 10.481 10.856 11.263 11.642 coh41:k=4.08163,b=4.08163 7.2102 7.41 7.6345 7.8802 8.1424 8.4224 9.7657 10.139 10.515 10.912 11.319 11.694 coh42:k=3.06122,b=5.10204 7.1855 7.39 7.6191 7.8694 8.1359 8.4201 9.7846 10.161 10.538 10.953 11.358 11.753 coh43:k=2.04082,b=6.12245 7.1569 7.3663 7.6003 7.8553 8.1262 8.4146 9.797 10.176 10.56 10.983 11.387 11.797 coh44:k=1.02041,b=7.14286 7.1246 7.3393 7.5785 7.8384 8.1139 8.4065 9.8051 10.187 10.583 11.006 11.409 11.831 coh45:k=0,b=8.16327 7.0883 7.309 7.5538 7.8189 8.0994 8.3964 9.8098 10.195 10.6 11.024 11.436 11.858 coh46:k=9.18367,b=0 7.5679 7.7208 7.8945 8.086 8.2903 8.5093 9.5949 9.8939 10.199 10.508 10.815 11.103 coh47:k=8.16327,b=1.02041 7.6674 7.8399 8.0352 8.2504 8.4806 8.7277 9.8991 10.228 10.577 10.941 11.301 11.643 coh48:k=7.14286,b=2.04082 7.6645 7.8425 8.0436 8.2648 8.501 8.7542 9.9554 10.312 10.674 11.039 11.4 11.769 coh49:k=6.12245,b=3.06122 7.6506 7.8333 8.0392 8.2652 8.5062 8.7641 10.002 10.362 10.726 11.092 11.478 11.85 coh50:k=5.10204,b=4.08163 7.6309 7.818 8.0283 8.2588 8.5042 8.7664 10.031 10.393 10.759 11.134 11.533 11.901 coh1226:k=50,b=0 14.953 14.999 15.052 15.111 15.177 15.247 15.595 15.701 15.818 15.945 16.077 16.208 coh1227:k=48.9796,b=1.02041 14.975 15.026 15.085 15.15 15.222 15.301 15.694 15.817 15.95 16.092 16.242 16.392 coh1228:k=47.9592,b=2.04082 14.975 15.027 15.087 15.154 15.228 15.308 15.717 15.842 15.978 16.124 16.282 16.439 coh1229:k=46.9388,b=3.06122 14.971 15.024 15.086 15.154 15.229 15.311 15.729 15.857 15.995 16.149 16.309 16.467 coh1230:k=45.9184,b=4.08163 14.967 15.021 15.083 15.153 15.229 15.312 15.737 15.866 16.01 16.165 16.327 16.488 coh1231:k=44.898,b=5.10204 14.961 15.016 15.079 15.15 15.227 15.311 15.742 15.873 16.02 16.177 16.34 16.506 coh1232:k=43.8776,b=6.12245 14.955 15.011 15.075 15.146 15.224 15.31 15.746 15.879 16.028 16.187 16.352 16.521 coh1233:k=42.8571,b=7.14286 14.948 15.005 15.07 15.142 15.221 15.307 15.748 15.884 16.035 16.194 16.363 16.532 coh1234:k=41.8367,b=8.16327 14.941 14.998 15.064 15.137 15.217 15.304 15.75 15.887 16.039 16.2 16.372 16.542 coh1235:k=40.8163,b=9.18367 14.934 14.992 15.058 15.132 15.213 15.301 15.75 15.889 16.043 16.205 16.379 16.55 coh1236:k=39.7959,b=10.2041 14.926 14.984 15.052 15.126 15.208 15.297 15.75 15.891 16.046 16.209 16.386 16.557 coh1237:k=38.7755,b=11.2245 14.917 14.977 15.045 15.12 15.203 15.293 15.75 15.892 16.048 16.213 16.391 16.563 coh1238:k=37.7551,b=12.2449 14.908 14.969 15.037 15.114 15.198 15.289 15.749 15.892 16.05 16.216 16.395 16.568 coh1239:k=36.7347,b=13.2653 14.899 14.96 15.03 15.108 15.192 15.284 15.748 15.892 16.051 16.219 16.399 16.572 coh1240:k=35.7143,b=14.2857 14.892 14.951 15.022 15.101 15.186 15.279 15.746 15.891 16.051 16.221 16.402 16.576 coh1241:k=34.6939,b=15.3061 14.884 14.943 15.014 15.093 15.18 15.274 15.744 15.89 16.051 16.222 16.404 16.58 coh1242:k=33.6735,b=16.3265 14.877 14.937 15.005 15.086 15.173 15.268 15.742 15.889 16.051 16.224 16.407 16.582 coh1243:k=32.6531,b=17.3469 14.869 14.929 14.998 15.078 15.166 15.262 15.74 15.887 16.051 16.224 16.408 16.585 coh1244:k=31.6327,b=18.3673 14.861 14.922 14.992 15.069 15.159 15.256 15.737 15.885 16.05 16.225 16.41 16.587 coh1245:k=30.6122,b=19.3878 14.853 14.914 14.985 15.063 15.152 15.25 15.734 15.882 16.049 16.225 16.411 16.589 coh1246:k=29.5918,b=20.4082 14.844 14.906 14.977 15.056 15.144 15.243 15.731 15.88 16.048 16.225 16.412 16.591 coh1247:k=28.5714,b=21.4286 14.835 14.898 14.97 15.05 15.136 15.236 15.727 15.877 16.046 16.224 16.413 16.592 coh1248:k=27.551,b=22.449 14.826 14.889 14.962 15.043 15.13 15.229 15.724 15.874 16.044 16.223 16.413 16.594 coh1249:k=26.5306,b=23.4694 14.816 14.881 14.954 15.036 15.124 15.222 15.72 15.872 16.043 16.222 16.413 16.595 coh1250:k=25.5102,b=24.4898 14.806 14.871 14.946 15.028 15.117 15.214 15.716 15.869 16.04 16.221 16.413 16.595 coh1251:k=24.4898,b=25.5102 14.796 14.862 14.937 15.02 15.111 15.208 15.712 15.866 16.038 16.22 16.413 16.596 coh1252:k=23.4694,b=26.5306 14.787 14.852 14.928 15.012 15.104 15.202 15.707 15.863 16.036 16.218 16.413 16.597 coh1253:k=22.449,b=27.551 14.779 14.842 14.919 15.004 15.096 15.196 15.703 15.86 16.033 16.216 16.412 16.597 coh1254:k=21.4286,b=28.5714 14.771 14.834 14.91 14.996 15.089 15.189 15.698 15.857 16.03 16.214 16.411 16.597 coh1255:k=20.4082,b=29.5918 14.762 14.826 14.9 14.987 15.081 15.183 15.693 15.853 16.027 16.212 16.411 16.597 coh1256:k=19.3878,b=30.6122 14.753 14.818 14.892 14.978 15.073 15.176 15.688 15.85 16.024 16.21 16.41 16.597 coh1257:k=18.3673,b=31.6327 14.744 14.81 14.885 14.968 15.065 15.169 15.683 15.846 16.021 16.208 16.409 16.597 coh1258:k=17.3469,b=32.6531 14.735 14.801 14.877 14.961 15.056 15.161 15.677 15.842 16.017 16.206 16.407 16.597 coh1259:k=16.3265,b=33.6735 14.725 14.792 14.869 14.953 15.048 15.154 15.672 15.838 16.014 16.204 16.406 16.597 coh1260:k=15.3061,b=34.6939 14.715 14.783 14.86 14.946 15.038 15.146 15.668 15.834 16.01 16.202 16.404 16.596 coh1261:k=14.2857,b=35.7143 14.705 14.774 14.852 14.938 15.031 15.138 15.664 15.829 16.006 16.199 16.403 16.596 coh1262:k=13.2653,b=36.7347 14.694 14.764 14.843 14.93 15.024 15.13 15.659 15.825 16.002 16.197 16.401 16.595 coh1263:k=12.2449,b=37.7551 14.683 14.754 14.834 14.922 15.017 15.121 15.654 15.82 15.998 16.194 16.399 16.594 coh1264:k=11.2245,b=38.7755 14.675 14.743 14.824 14.913 15.009 15.112 15.649 15.815 15.994 16.191 16.397 16.593 coh1265:k=10.2041,b=39.7959 14.666 14.733 14.814 14.905 15.002 15.106 15.644 15.81 15.991 16.189 16.395 16.592 coh1266:k=9.18367,b=40.8163 14.657 14.725 14.804 14.895 14.994 15.099 15.639 15.805 15.987 16.186 16.393 16.591 coh1267:k=8.16327,b=41.8367 14.648 14.716 14.794 14.886 14.986 15.092 15.633 15.8 15.984 16.183 16.391 16.59 coh1268:k=7.14286,b=42.8571 14.639 14.708 14.786 14.876 14.977 15.084 15.628 15.794 15.98 16.18 16.389 16.589 coh1269:k=6.12245,b=43.8776 14.629 14.699 14.778 14.866 14.968 15.077 15.622 15.789 15.976 16.176 16.386 16.588 coh1270:k=5.10204,b=44.898 14.619 14.69 14.77 14.857 14.959 15.069 15.616 15.784 15.972 16.173 16.384 16.587 coh1271:k=4.08163,b=45.9184 14.609 14.68 14.761 14.85 14.95 15.061 15.61 15.78 15.968 16.17 16.381 16.586 coh1272:k=3.06122,b=46.9388 14.598 14.67 14.752 14.842 14.94 15.053 15.604 15.775 15.964 16.166 16.378 16.584 coh1273:k=2.04082,b=47.9592 14.587 14.66 14.743 14.834 14.931 15.045 15.597 15.77 15.959 16.162 16.376 16.583 coh1274:k=1.02041,b=48.9796 14.576 14.65 14.734 14.825 14.924 15.036 15.591 15.765 15.955 16.158 16.373 16.581 coh1275:k=0,b=50 14.566 14.639 14.724 14.817 14.916 15.027 15.584 15.761 15.95 16.155 16.37 16.579 tb_pol_a: optimal safe savings choice for each state space point z1_0_34741 z2_0_40076 z3_0_4623 z4_0_5333 z5_0_61519 z6_0_70966 z10_1_2567 z11_1_4496 z12_1_6723 z13_1_9291 z14_2_2253 z15_2_567 __________ __________ _________ _________ __________ __________ __________ __________ __________ __________ __________ _________ coh1:k=0,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh2:k=1.02041,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh3:k=0,b=1.02041 0 0 0 0 0 0 0 0 0 0 0 0 coh4:k=2.04082,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh5:k=1.02041,b=1.02041 0 0 0 0 0 0 0 0 0 0 0 0 coh6:k=0,b=2.04082 0 0 0 0 0 0 0 0 0 0 0 0 coh7:k=3.06122,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh8:k=2.04082,b=1.02041 0 0 0 0 0 0 0 0 0 0 0 0 coh9:k=1.02041,b=2.04082 0 0 0 0 0 0 0 0 0 0 0 0 coh10:k=0,b=3.06122 0 0 0 0 0 0 0 0 0 0 0 0 coh11:k=4.08163,b=0 1.0204 1.0204 0 0 0 0 0 0 0 0 0 0 coh12:k=3.06122,b=1.02041 1.0204 1.0204 0 0 0 0 0 0 0 0 0 0 coh13:k=2.04082,b=2.04082 1.0204 1.0204 0 0 0 0 0 0 0 0 0 0 coh14:k=1.02041,b=3.06122 1.0204 1.0204 0 0 0 0 0 0 0 0 0 0 coh15:k=0,b=4.08163 1.0204 1.0204 0 0 0 0 0 0 0 0 0 0 coh16:k=5.10204,b=0 2.0408 2.0408 1.0204 1.0204 0 0 0 0 0 0 0 0 coh17:k=4.08163,b=1.02041 2.0408 2.0408 1.0204 1.0204 0 0 0 0 0 0 0 0 coh18:k=3.06122,b=2.04082 2.0408 2.0408 1.0204 1.0204 0 0 0 0 0 0 0 0 coh19:k=2.04082,b=3.06122 2.0408 2.0408 1.0204 1.0204 0 0 0 0 0 0 0 0 coh20:k=1.02041,b=4.08163 2.0408 2.0408 1.0204 1.0204 0 0 0 0 0 0 0 0 coh21:k=0,b=5.10204 2.0408 2.0408 1.0204 1.0204 0 0 0 0 0 0 0 0 coh22:k=6.12245,b=0 3.0612 3.0612 2.0408 2.0408 1.0204 0 0 0 0 0 0 0 coh23:k=5.10204,b=1.02041 3.0612 3.0612 2.0408 2.0408 1.0204 0 0 0 0 0 0 0 coh24:k=4.08163,b=2.04082 3.0612 3.0612 2.0408 2.0408 1.0204 0 0 0 0 0 0 0 coh25:k=3.06122,b=3.06122 3.0612 3.0612 2.0408 2.0408 1.0204 0 0 0 0 0 0 0 coh26:k=2.04082,b=4.08163 3.0612 3.0612 2.0408 2.0408 1.0204 0 0 0 0 0 0 0 coh27:k=1.02041,b=5.10204 3.0612 3.0612 2.0408 2.0408 1.0204 0 0 0 0 0 0 0 coh28:k=0,b=6.12245 3.0612 3.0612 2.0408 2.0408 1.0204 0 0 0 0 0 0 0 coh29:k=7.14286,b=0 4.0816 4.0816 3.0612 3.0612 2.0408 1.0204 0 0 0 0 0 0 coh30:k=6.12245,b=1.02041 4.0816 4.0816 3.0612 3.0612 2.0408 1.0204 0 0 0 0 0 0 coh31:k=5.10204,b=2.04082 4.0816 4.0816 3.0612 3.0612 2.0408 1.0204 0 0 0 0 0 0 coh32:k=4.08163,b=3.06122 4.0816 4.0816 3.0612 3.0612 2.0408 1.0204 0 0 0 0 0 0 coh33:k=3.06122,b=4.08163 4.0816 4.0816 3.0612 3.0612 2.0408 1.0204 0 0 0 0 0 0 coh34:k=2.04082,b=5.10204 4.0816 4.0816 3.0612 3.0612 2.0408 1.0204 0 0 0 0 0 0 coh35:k=1.02041,b=6.12245 4.0816 4.0816 3.0612 3.0612 2.0408 1.0204 0 0 0 0 0 0 coh36:k=0,b=7.14286 4.0816 4.0816 3.0612 3.0612 2.0408 1.0204 0 0 0 0 0 0 coh37:k=8.16327,b=0 5.102 5.102 4.0816 4.0816 3.0612 2.0408 0 0 0 0 0 0 coh38:k=7.14286,b=1.02041 5.102 5.102 4.0816 4.0816 3.0612 2.0408 0 0 0 0 0 0 coh39:k=6.12245,b=2.04082 5.102 5.102 4.0816 4.0816 3.0612 2.0408 0 0 0 0 0 0 coh40:k=5.10204,b=3.06122 5.102 5.102 4.0816 4.0816 3.0612 2.0408 0 0 0 0 0 0 coh41:k=4.08163,b=4.08163 5.102 5.102 4.0816 4.0816 3.0612 2.0408 0 0 0 0 0 0 coh42:k=3.06122,b=5.10204 5.102 5.102 4.0816 4.0816 3.0612 2.0408 0 0 0 0 0 0 coh43:k=2.04082,b=6.12245 5.102 5.102 4.0816 4.0816 3.0612 2.0408 0 0 0 0 0 0 coh44:k=1.02041,b=7.14286 5.102 5.102 4.0816 4.0816 3.0612 2.0408 0 0 0 0 0 0 coh45:k=0,b=8.16327 5.102 5.102 4.0816 4.0816 3.0612 2.0408 0 0 0 0 0 0 coh46:k=9.18367,b=0 6.1224 6.1224 5.102 5.102 4.0816 4.0816 0 0 0 0 0 0 coh47:k=8.16327,b=1.02041 6.1224 6.1224 5.102 5.102 4.0816 4.0816 0 0 0 0 0 0 coh48:k=7.14286,b=2.04082 6.1224 6.1224 5.102 5.102 4.0816 4.0816 0 0 0 0 0 0 coh49:k=6.12245,b=3.06122 6.1224 6.1224 5.102 5.102 4.0816 4.0816 0 0 0 0 0 0 coh50:k=5.10204,b=4.08163 6.1224 6.1224 5.102 5.102 4.0816 4.0816 0 0 0 0 0 0 coh1226:k=50,b=0 44.898 44.898 43.878 43.878 42.857 42.857 37.755 35.714 32.653 30.612 27.551 24.49 coh1227:k=48.9796,b=1.02041 44.898 44.898 43.878 43.878 42.857 42.857 38.776 36.735 34.694 32.653 29.592 26.531 coh1228:k=47.9592,b=2.04082 44.898 44.898 43.878 43.878 42.857 42.857 38.776 36.735 34.694 32.653 30.612 27.551 coh1229:k=46.9388,b=3.06122 44.898 44.898 43.878 43.878 42.857 42.857 38.776 36.735 34.694 33.673 30.612 27.551 coh1230:k=45.9184,b=4.08163 44.898 44.898 43.878 43.878 42.857 42.857 38.776 36.735 35.714 33.673 30.612 29.592 coh1231:k=44.898,b=5.10204 44.898 44.898 43.878 43.878 42.857 42.857 38.776 36.735 35.714 33.673 30.612 29.592 coh1232:k=43.8776,b=6.12245 44.898 44.898 43.878 43.878 42.857 42.857 38.776 37.755 35.714 33.673 31.633 29.592 coh1233:k=42.8571,b=7.14286 44.898 44.898 43.878 43.878 42.857 42.857 38.776 37.755 35.714 33.673 31.633 29.592 coh1234:k=41.8367,b=8.16327 44.898 44.898 43.878 43.878 42.857 42.857 38.776 37.755 35.714 33.673 31.633 29.592 coh1235:k=40.8163,b=9.18367 44.898 44.898 43.878 43.878 42.857 42.857 38.776 37.755 35.714 33.673 31.633 29.592 coh1236:k=39.7959,b=10.2041 44.898 44.898 43.878 43.878 42.857 42.857 38.776 37.755 35.714 33.673 31.633 29.592 coh1237:k=38.7755,b=11.2245 44.898 44.898 43.878 43.878 42.857 42.857 38.776 37.755 35.714 34.694 31.633 29.592 coh1238:k=37.7551,b=12.2449 44.898 44.898 43.878 43.878 42.857 42.857 38.776 37.755 35.714 34.694 31.633 29.592 coh1239:k=36.7347,b=13.2653 44.898 44.898 43.878 43.878 42.857 42.857 38.776 37.755 35.714 34.694 31.633 29.592 coh1240:k=35.7143,b=14.2857 43.878 44.898 43.878 43.878 42.857 42.857 38.776 37.755 35.714 34.694 31.633 29.592 coh1241:k=34.6939,b=15.3061 43.878 43.878 43.878 43.878 42.857 42.857 38.776 37.755 35.714 34.694 31.633 29.592 coh1242:k=33.6735,b=16.3265 43.878 43.878 43.878 43.878 42.857 42.857 38.776 37.755 35.714 34.694 31.633 29.592 coh1243:k=32.6531,b=17.3469 43.878 43.878 42.857 43.878 42.857 42.857 38.776 37.755 35.714 34.694 31.633 29.592 coh1244:k=31.6327,b=18.3673 43.878 43.878 42.857 43.878 42.857 42.857 38.776 37.755 35.714 34.694 31.633 29.592 coh1245:k=30.6122,b=19.3878 43.878 43.878 42.857 42.857 42.857 42.857 38.776 37.755 35.714 34.694 31.633 29.592 coh1246:k=29.5918,b=20.4082 43.878 43.878 42.857 42.857 42.857 42.857 38.776 37.755 35.714 34.694 31.633 29.592 coh1247:k=28.5714,b=21.4286 43.878 43.878 42.857 42.857 41.837 42.857 38.776 37.755 35.714 34.694 31.633 29.592 coh1248:k=27.551,b=22.449 43.878 43.878 42.857 42.857 41.837 42.857 38.776 36.735 35.714 34.694 31.633 29.592 coh1249:k=26.5306,b=23.4694 43.878 43.878 42.857 42.857 41.837 42.857 38.776 36.735 35.714 34.694 31.633 29.592 coh1250:k=25.5102,b=24.4898 43.878 43.878 42.857 42.857 41.837 42.857 38.776 36.735 35.714 34.694 31.633 29.592 coh1251:k=24.4898,b=25.5102 43.878 43.878 42.857 42.857 41.837 41.837 38.776 36.735 35.714 34.694 31.633 29.592 coh1252:k=23.4694,b=26.5306 42.857 43.878 42.857 42.857 41.837 41.837 38.776 36.735 35.714 34.694 31.633 29.592 coh1253:k=22.449,b=27.551 42.857 43.878 42.857 42.857 41.837 41.837 38.776 36.735 35.714 34.694 31.633 29.592 coh1254:k=21.4286,b=28.5714 42.857 42.857 42.857 42.857 41.837 41.837 38.776 36.735 35.714 34.694 31.633 29.592 coh1255:k=20.4082,b=29.5918 42.857 42.857 41.837 42.857 41.837 41.837 38.776 36.735 35.714 34.694 31.633 29.592 coh1256:k=19.3878,b=30.6122 42.857 42.857 41.837 42.857 41.837 41.837 38.776 36.735 35.714 33.673 31.633 29.592 coh1257:k=18.3673,b=31.6327 42.857 42.857 41.837 42.857 41.837 41.837 38.776 36.735 35.714 33.673 31.633 29.592 coh1258:k=17.3469,b=32.6531 42.857 42.857 41.837 41.837 41.837 41.837 38.776 36.735 35.714 33.673 31.633 29.592 coh1259:k=16.3265,b=33.6735 42.857 42.857 41.837 41.837 41.837 41.837 37.755 36.735 35.714 33.673 31.633 29.592 coh1260:k=15.3061,b=34.6939 42.857 42.857 41.837 41.837 41.837 41.837 37.755 36.735 35.714 33.673 31.633 29.592 coh1261:k=14.2857,b=35.7143 42.857 42.857 41.837 41.837 40.816 41.837 37.755 36.735 35.714 33.673 31.633 29.592 coh1262:k=13.2653,b=36.7347 42.857 42.857 41.837 41.837 40.816 41.837 37.755 36.735 35.714 33.673 31.633 29.592 coh1263:k=12.2449,b=37.7551 42.857 42.857 41.837 41.837 40.816 41.837 37.755 36.735 35.714 33.673 31.633 29.592 coh1264:k=11.2245,b=38.7755 41.837 42.857 41.837 41.837 40.816 40.816 37.755 36.735 34.694 33.673 31.633 29.592 coh1265:k=10.2041,b=39.7959 41.837 41.837 41.837 41.837 40.816 40.816 37.755 36.735 34.694 33.673 31.633 29.592 coh1266:k=9.18367,b=40.8163 41.837 41.837 41.837 41.837 40.816 40.816 37.755 36.735 34.694 33.673 31.633 29.592 coh1267:k=8.16327,b=41.8367 41.837 41.837 40.816 41.837 40.816 40.816 37.755 36.735 34.694 33.673 31.633 29.592 coh1268:k=7.14286,b=42.8571 41.837 41.837 40.816 41.837 40.816 40.816 37.755 36.735 34.694 33.673 31.633 29.592 coh1269:k=6.12245,b=43.8776 41.837 41.837 40.816 41.837 40.816 40.816 37.755 36.735 34.694 33.673 31.633 29.592 coh1270:k=5.10204,b=44.898 41.837 41.837 40.816 40.816 40.816 40.816 37.755 35.714 34.694 33.673 31.633 29.592 coh1271:k=4.08163,b=45.9184 41.837 41.837 40.816 40.816 40.816 40.816 37.755 35.714 34.694 33.673 31.633 29.592 coh1272:k=3.06122,b=46.9388 41.837 41.837 40.816 40.816 40.816 40.816 37.755 35.714 34.694 33.673 31.633 29.592 coh1273:k=2.04082,b=47.9592 41.837 41.837 40.816 40.816 39.796 40.816 37.755 35.714 34.694 33.673 31.633 29.592 coh1274:k=1.02041,b=48.9796 41.837 41.837 40.816 40.816 39.796 40.816 37.755 35.714 34.694 33.673 31.633 29.592 coh1275:k=0,b=50 40.816 41.837 40.816 40.816 39.796 40.816 37.755 35.714 34.694 33.673 31.633 29.592 tb_pol_k: optimal risky investment choice for each state space point z1_0_34741 z2_0_40076 z3_0_4623 z4_0_5333 z5_0_61519 z6_0_70966 z10_1_2567 z11_1_4496 z12_1_6723 z13_1_9291 z14_2_2253 z15_2_567 __________ __________ _________ _________ __________ __________ __________ __________ __________ __________ __________ _________ coh1:k=0,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh2:k=1.02041,b=0 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 coh3:k=0,b=1.02041 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 coh4:k=2.04082,b=0 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 coh5:k=1.02041,b=1.02041 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 3.0612 3.0612 3.0612 3.0612 coh6:k=0,b=2.04082 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 3.0612 3.0612 3.0612 3.0612 4.0816 coh7:k=3.06122,b=0 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 coh8:k=2.04082,b=1.02041 2.0408 2.0408 2.0408 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 4.0816 4.0816 coh9:k=1.02041,b=2.04082 2.0408 2.0408 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 4.0816 4.0816 4.0816 4.0816 coh10:k=0,b=3.06122 2.0408 2.0408 3.0612 3.0612 3.0612 3.0612 3.0612 4.0816 4.0816 4.0816 4.0816 5.102 coh11:k=4.08163,b=0 2.0408 2.0408 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 coh12:k=3.06122,b=1.02041 2.0408 2.0408 3.0612 3.0612 3.0612 3.0612 4.0816 4.0816 4.0816 4.0816 5.102 5.102 coh13:k=2.04082,b=2.04082 2.0408 2.0408 3.0612 3.0612 3.0612 4.0816 4.0816 4.0816 4.0816 5.102 5.102 5.102 coh14:k=1.02041,b=3.06122 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 4.0816 4.0816 5.102 5.102 5.102 6.1224 coh15:k=0,b=4.08163 2.0408 2.0408 3.0612 3.0612 3.0612 4.0816 4.0816 5.102 5.102 5.102 5.102 6.1224 coh16:k=5.10204,b=0 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 4.0816 4.0816 4.0816 4.0816 4.0816 4.0816 coh17:k=4.08163,b=1.02041 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 5.102 5.102 5.102 5.102 5.102 6.1224 coh18:k=3.06122,b=2.04082 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 5.102 5.102 5.102 6.1224 6.1224 6.1224 coh19:k=2.04082,b=3.06122 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 5.102 5.102 6.1224 6.1224 6.1224 7.1429 coh20:k=1.02041,b=4.08163 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 5.102 5.102 6.1224 6.1224 6.1224 7.1429 coh21:k=0,b=5.10204 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 5.102 5.102 6.1224 6.1224 7.1429 7.1429 coh22:k=6.12245,b=0 2.0408 2.0408 3.0612 3.0612 4.0816 5.102 5.102 5.102 5.102 5.102 5.102 4.0816 coh23:k=5.10204,b=1.02041 2.0408 2.0408 3.0612 3.0612 4.0816 5.102 6.1224 6.1224 6.1224 6.1224 6.1224 7.1429 coh24:k=4.08163,b=2.04082 2.0408 2.0408 3.0612 3.0612 4.0816 5.102 6.1224 6.1224 6.1224 6.1224 7.1429 7.1429 coh25:k=3.06122,b=3.06122 2.0408 2.0408 3.0612 3.0612 4.0816 5.102 6.1224 6.1224 6.1224 7.1429 7.1429 7.1429 coh26:k=2.04082,b=4.08163 2.0408 2.0408 3.0612 3.0612 4.0816 5.102 6.1224 6.1224 7.1429 7.1429 7.1429 8.1633 coh27:k=1.02041,b=5.10204 2.0408 2.0408 3.0612 3.0612 4.0816 5.102 6.1224 6.1224 7.1429 7.1429 7.1429 8.1633 coh28:k=0,b=6.12245 2.0408 2.0408 3.0612 3.0612 4.0816 5.102 6.1224 6.1224 7.1429 7.1429 8.1633 8.1633 coh29:k=7.14286,b=0 2.0408 2.0408 3.0612 3.0612 4.0816 5.102 6.1224 5.102 5.102 5.102 5.102 5.102 coh30:k=6.12245,b=1.02041 2.0408 2.0408 3.0612 3.0612 4.0816 5.102 6.1224 7.1429 7.1429 7.1429 7.1429 7.1429 coh31:k=5.10204,b=2.04082 2.0408 2.0408 3.0612 3.0612 4.0816 5.102 7.1429 7.1429 7.1429 7.1429 8.1633 8.1633 coh32:k=4.08163,b=3.06122 2.0408 2.0408 3.0612 3.0612 4.0816 5.102 7.1429 7.1429 7.1429 8.1633 8.1633 8.1633 coh33:k=3.06122,b=4.08163 2.0408 2.0408 3.0612 3.0612 4.0816 5.102 7.1429 7.1429 7.1429 8.1633 8.1633 9.1837 coh34:k=2.04082,b=5.10204 2.0408 2.0408 3.0612 3.0612 4.0816 5.102 7.1429 7.1429 8.1633 8.1633 8.1633 9.1837 coh35:k=1.02041,b=6.12245 2.0408 2.0408 3.0612 3.0612 4.0816 5.102 7.1429 7.1429 8.1633 8.1633 8.1633 9.1837 coh36:k=0,b=7.14286 2.0408 2.0408 3.0612 3.0612 4.0816 5.102 7.1429 7.1429 8.1633 8.1633 9.1837 9.1837 coh37:k=8.16327,b=0 2.0408 2.0408 3.0612 3.0612 4.0816 5.102 6.1224 6.1224 6.1224 6.1224 6.1224 6.1224 coh38:k=7.14286,b=1.02041 2.0408 2.0408 3.0612 3.0612 4.0816 5.102 7.1429 8.1633 8.1633 8.1633 8.1633 8.1633 coh39:k=6.12245,b=2.04082 2.0408 2.0408 3.0612 3.0612 4.0816 5.102 8.1633 8.1633 8.1633 8.1633 9.1837 9.1837 coh40:k=5.10204,b=3.06122 2.0408 2.0408 3.0612 3.0612 4.0816 5.102 8.1633 8.1633 8.1633 8.1633 9.1837 9.1837 coh41:k=4.08163,b=4.08163 2.0408 2.0408 3.0612 3.0612 4.0816 5.102 8.1633 8.1633 8.1633 9.1837 9.1837 9.1837 coh42:k=3.06122,b=5.10204 2.0408 2.0408 3.0612 3.0612 4.0816 5.102 8.1633 8.1633 8.1633 9.1837 9.1837 10.204 coh43:k=2.04082,b=6.12245 2.0408 2.0408 3.0612 3.0612 4.0816 5.102 8.1633 8.1633 9.1837 9.1837 9.1837 10.204 coh44:k=1.02041,b=7.14286 2.0408 2.0408 3.0612 3.0612 4.0816 5.102 8.1633 8.1633 9.1837 9.1837 9.1837 10.204 coh45:k=0,b=8.16327 2.0408 2.0408 3.0612 3.0612 4.0816 5.102 8.1633 8.1633 9.1837 9.1837 10.204 10.204 coh46:k=9.18367,b=0 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 7.1429 7.1429 7.1429 7.1429 7.1429 7.1429 coh47:k=8.16327,b=1.02041 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 8.1633 8.1633 9.1837 9.1837 9.1837 9.1837 coh48:k=7.14286,b=2.04082 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 9.1837 9.1837 9.1837 9.1837 10.204 coh49:k=6.12245,b=3.06122 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 9.1837 9.1837 9.1837 10.204 10.204 coh50:k=5.10204,b=4.08163 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 9.1837 9.1837 10.204 10.204 10.204 coh1226:k=50,b=0 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 21.429 coh1227:k=48.9796,b=1.02041 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 21.429 coh1228:k=47.9592,b=2.04082 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 21.429 coh1229:k=46.9388,b=3.06122 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 21.429 coh1230:k=45.9184,b=4.08163 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 20.408 coh1231:k=44.898,b=5.10204 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 20.408 coh1232:k=43.8776,b=6.12245 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 20.408 coh1233:k=42.8571,b=7.14286 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 20.408 coh1234:k=41.8367,b=8.16327 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 20.408 coh1235:k=40.8163,b=9.18367 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 20.408 coh1236:k=39.7959,b=10.2041 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 20.408 coh1237:k=38.7755,b=11.2245 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 20.408 coh1238:k=37.7551,b=12.2449 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 20.408 coh1239:k=36.7347,b=13.2653 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 20.408 coh1240:k=35.7143,b=14.2857 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 20.408 coh1241:k=34.6939,b=15.3061 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 20.408 coh1242:k=33.6735,b=16.3265 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 20.408 coh1243:k=32.6531,b=17.3469 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 20.408 coh1244:k=31.6327,b=18.3673 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 20.408 coh1245:k=30.6122,b=19.3878 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 20.408 coh1246:k=29.5918,b=20.4082 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 20.408 coh1247:k=28.5714,b=21.4286 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 20.408 coh1248:k=27.551,b=22.449 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 20.408 coh1249:k=26.5306,b=23.4694 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 20.408 coh1250:k=25.5102,b=24.4898 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 20.408 coh1251:k=24.4898,b=25.5102 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 20.408 coh1252:k=23.4694,b=26.5306 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 20.408 coh1253:k=22.449,b=27.551 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 20.408 coh1254:k=21.4286,b=28.5714 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 20.408 coh1255:k=20.4082,b=29.5918 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 20.408 coh1256:k=19.3878,b=30.6122 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 20.408 coh1257:k=18.3673,b=31.6327 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 20.408 coh1258:k=17.3469,b=32.6531 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 20.408 coh1259:k=16.3265,b=33.6735 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 20.408 coh1260:k=15.3061,b=34.6939 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 20.408 coh1261:k=14.2857,b=35.7143 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 20.408 coh1262:k=13.2653,b=36.7347 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 20.408 coh1263:k=12.2449,b=37.7551 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 20.408 coh1264:k=11.2245,b=38.7755 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 20.408 coh1265:k=10.2041,b=39.7959 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 20.408 coh1266:k=9.18367,b=40.8163 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 20.408 coh1267:k=8.16327,b=41.8367 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 20.408 coh1268:k=7.14286,b=42.8571 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 20.408 coh1269:k=6.12245,b=43.8776 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 20.408 coh1270:k=5.10204,b=44.898 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 20.408 coh1271:k=4.08163,b=45.9184 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 20.408 coh1272:k=3.06122,b=46.9388 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 20.408 coh1273:k=2.04082,b=47.9592 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 20.408 coh1274:k=1.02041,b=48.9796 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 20.408 coh1275:k=0,b=50 2.0408 2.0408 3.0612 3.0612 4.0816 4.0816 9.1837 11.224 13.265 15.306 18.367 20.408 tb_pol_w: risky + safe investment choices (first stage choice, choose within risky vs safe) z1_0_34741 z2_0_40076 z3_0_4623 z4_0_5333 z5_0_61519 z6_0_70966 z10_1_2567 z11_1_4496 z12_1_6723 z13_1_9291 z14_2_2253 z15_2_567 __________ __________ _________ _________ __________ __________ __________ __________ __________ __________ __________ _________ coh1:k=0,b=0 0 0 0 0 0 0 0 0 0 0 0 0 coh2:k=1.02041,b=0 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 coh3:k=0,b=1.02041 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 coh4:k=2.04082,b=0 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 coh5:k=1.02041,b=1.02041 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 3.0612 3.0612 3.0612 3.0612 coh6:k=0,b=2.04082 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 3.0612 3.0612 3.0612 3.0612 4.0816 coh7:k=3.06122,b=0 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 2.0408 coh8:k=2.04082,b=1.02041 2.0408 2.0408 2.0408 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 4.0816 4.0816 coh9:k=1.02041,b=2.04082 2.0408 2.0408 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 4.0816 4.0816 4.0816 4.0816 coh10:k=0,b=3.06122 2.0408 2.0408 3.0612 3.0612 3.0612 3.0612 3.0612 4.0816 4.0816 4.0816 4.0816 5.102 coh11:k=4.08163,b=0 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 coh12:k=3.06122,b=1.02041 3.0612 3.0612 3.0612 3.0612 3.0612 3.0612 4.0816 4.0816 4.0816 4.0816 5.102 5.102 coh13:k=2.04082,b=2.04082 3.0612 3.0612 3.0612 3.0612 3.0612 4.0816 4.0816 4.0816 4.0816 5.102 5.102 5.102 coh14:k=1.02041,b=3.06122 3.0612 3.0612 3.0612 3.0612 4.0816 4.0816 4.0816 4.0816 5.102 5.102 5.102 6.1224 coh15:k=0,b=4.08163 3.0612 3.0612 3.0612 3.0612 3.0612 4.0816 4.0816 5.102 5.102 5.102 5.102 6.1224 coh16:k=5.10204,b=0 4.0816 4.0816 4.0816 4.0816 4.0816 4.0816 4.0816 4.0816 4.0816 4.0816 4.0816 4.0816 coh17:k=4.08163,b=1.02041 4.0816 4.0816 4.0816 4.0816 4.0816 4.0816 5.102 5.102 5.102 5.102 5.102 6.1224 coh18:k=3.06122,b=2.04082 4.0816 4.0816 4.0816 4.0816 4.0816 4.0816 5.102 5.102 5.102 6.1224 6.1224 6.1224 coh19:k=2.04082,b=3.06122 4.0816 4.0816 4.0816 4.0816 4.0816 4.0816 5.102 5.102 6.1224 6.1224 6.1224 7.1429 coh20:k=1.02041,b=4.08163 4.0816 4.0816 4.0816 4.0816 4.0816 4.0816 5.102 5.102 6.1224 6.1224 6.1224 7.1429 coh21:k=0,b=5.10204 4.0816 4.0816 4.0816 4.0816 4.0816 4.0816 5.102 5.102 6.1224 6.1224 7.1429 7.1429 coh22:k=6.12245,b=0 5.102 5.102 5.102 5.102 5.102 5.102 5.102 5.102 5.102 5.102 5.102 4.0816 coh23:k=5.10204,b=1.02041 5.102 5.102 5.102 5.102 5.102 5.102 6.1224 6.1224 6.1224 6.1224 6.1224 7.1429 coh24:k=4.08163,b=2.04082 5.102 5.102 5.102 5.102 5.102 5.102 6.1224 6.1224 6.1224 6.1224 7.1429 7.1429 coh25:k=3.06122,b=3.06122 5.102 5.102 5.102 5.102 5.102 5.102 6.1224 6.1224 6.1224 7.1429 7.1429 7.1429 coh26:k=2.04082,b=4.08163 5.102 5.102 5.102 5.102 5.102 5.102 6.1224 6.1224 7.1429 7.1429 7.1429 8.1633 coh27:k=1.02041,b=5.10204 5.102 5.102 5.102 5.102 5.102 5.102 6.1224 6.1224 7.1429 7.1429 7.1429 8.1633 coh28:k=0,b=6.12245 5.102 5.102 5.102 5.102 5.102 5.102 6.1224 6.1224 7.1429 7.1429 8.1633 8.1633 coh29:k=7.14286,b=0 6.1224 6.1224 6.1224 6.1224 6.1224 6.1224 6.1224 5.102 5.102 5.102 5.102 5.102 coh30:k=6.12245,b=1.02041 6.1224 6.1224 6.1224 6.1224 6.1224 6.1224 6.1224 7.1429 7.1429 7.1429 7.1429 7.1429 coh31:k=5.10204,b=2.04082 6.1224 6.1224 6.1224 6.1224 6.1224 6.1224 7.1429 7.1429 7.1429 7.1429 8.1633 8.1633 coh32:k=4.08163,b=3.06122 6.1224 6.1224 6.1224 6.1224 6.1224 6.1224 7.1429 7.1429 7.1429 8.1633 8.1633 8.1633 coh33:k=3.06122,b=4.08163 6.1224 6.1224 6.1224 6.1224 6.1224 6.1224 7.1429 7.1429 7.1429 8.1633 8.1633 9.1837 coh34:k=2.04082,b=5.10204 6.1224 6.1224 6.1224 6.1224 6.1224 6.1224 7.1429 7.1429 8.1633 8.1633 8.1633 9.1837 coh35:k=1.02041,b=6.12245 6.1224 6.1224 6.1224 6.1224 6.1224 6.1224 7.1429 7.1429 8.1633 8.1633 8.1633 9.1837 coh36:k=0,b=7.14286 6.1224 6.1224 6.1224 6.1224 6.1224 6.1224 7.1429 7.1429 8.1633 8.1633 9.1837 9.1837 coh37:k=8.16327,b=0 7.1429 7.1429 7.1429 7.1429 7.1429 7.1429 6.1224 6.1224 6.1224 6.1224 6.1224 6.1224 coh38:k=7.14286,b=1.02041 7.1429 7.1429 7.1429 7.1429 7.1429 7.1429 7.1429 8.1633 8.1633 8.1633 8.1633 8.1633 coh39:k=6.12245,b=2.04082 7.1429 7.1429 7.1429 7.1429 7.1429 7.1429 8.1633 8.1633 8.1633 8.1633 9.1837 9.1837 coh40:k=5.10204,b=3.06122 7.1429 7.1429 7.1429 7.1429 7.1429 7.1429 8.1633 8.1633 8.1633 8.1633 9.1837 9.1837 coh41:k=4.08163,b=4.08163 7.1429 7.1429 7.1429 7.1429 7.1429 7.1429 8.1633 8.1633 8.1633 9.1837 9.1837 9.1837 coh42:k=3.06122,b=5.10204 7.1429 7.1429 7.1429 7.1429 7.1429 7.1429 8.1633 8.1633 8.1633 9.1837 9.1837 10.204 coh43:k=2.04082,b=6.12245 7.1429 7.1429 7.1429 7.1429 7.1429 7.1429 8.1633 8.1633 9.1837 9.1837 9.1837 10.204 coh44:k=1.02041,b=7.14286 7.1429 7.1429 7.1429 7.1429 7.1429 7.1429 8.1633 8.1633 9.1837 9.1837 9.1837 10.204 coh45:k=0,b=8.16327 7.1429 7.1429 7.1429 7.1429 7.1429 7.1429 8.1633 8.1633 9.1837 9.1837 10.204 10.204 coh46:k=9.18367,b=0 8.1633 8.1633 8.1633 8.1633 8.1633 8.1633 7.1429 7.1429 7.1429 7.1429 7.1429 7.1429 coh47:k=8.16327,b=1.02041 8.1633 8.1633 8.1633 8.1633 8.1633 8.1633 8.1633 8.1633 9.1837 9.1837 9.1837 9.1837 coh48:k=7.14286,b=2.04082 8.1633 8.1633 8.1633 8.1633 8.1633 8.1633 9.1837 9.1837 9.1837 9.1837 9.1837 10.204 coh49:k=6.12245,b=3.06122 8.1633 8.1633 8.1633 8.1633 8.1633 8.1633 9.1837 9.1837 9.1837 9.1837 10.204 10.204 coh50:k=5.10204,b=4.08163 8.1633 8.1633 8.1633 8.1633 8.1633 8.1633 9.1837 9.1837 9.1837 10.204 10.204 10.204 coh1226:k=50,b=0 46.939 46.939 46.939 46.939 46.939 46.939 46.939 46.939 45.918 45.918 45.918 45.918 coh1227:k=48.9796,b=1.02041 46.939 46.939 46.939 46.939 46.939 46.939 47.959 47.959 47.959 47.959 47.959 47.959 coh1228:k=47.9592,b=2.04082 46.939 46.939 46.939 46.939 46.939 46.939 47.959 47.959 47.959 47.959 48.98 48.98 coh1229:k=46.9388,b=3.06122 46.939 46.939 46.939 46.939 46.939 46.939 47.959 47.959 47.959 48.98 48.98 48.98 coh1230:k=45.9184,b=4.08163 46.939 46.939 46.939 46.939 46.939 46.939 47.959 47.959 48.98 48.98 48.98 50 coh1231:k=44.898,b=5.10204 46.939 46.939 46.939 46.939 46.939 46.939 47.959 47.959 48.98 48.98 48.98 50 coh1232:k=43.8776,b=6.12245 46.939 46.939 46.939 46.939 46.939 46.939 47.959 48.98 48.98 48.98 50 50 coh1233:k=42.8571,b=7.14286 46.939 46.939 46.939 46.939 46.939 46.939 47.959 48.98 48.98 48.98 50 50 coh1234:k=41.8367,b=8.16327 46.939 46.939 46.939 46.939 46.939 46.939 47.959 48.98 48.98 48.98 50 50 coh1235:k=40.8163,b=9.18367 46.939 46.939 46.939 46.939 46.939 46.939 47.959 48.98 48.98 48.98 50 50 coh1236:k=39.7959,b=10.2041 46.939 46.939 46.939 46.939 46.939 46.939 47.959 48.98 48.98 48.98 50 50 coh1237:k=38.7755,b=11.2245 46.939 46.939 46.939 46.939 46.939 46.939 47.959 48.98 48.98 50 50 50 coh1238:k=37.7551,b=12.2449 46.939 46.939 46.939 46.939 46.939 46.939 47.959 48.98 48.98 50 50 50 coh1239:k=36.7347,b=13.2653 46.939 46.939 46.939 46.939 46.939 46.939 47.959 48.98 48.98 50 50 50 coh1240:k=35.7143,b=14.2857 45.918 46.939 46.939 46.939 46.939 46.939 47.959 48.98 48.98 50 50 50 coh1241:k=34.6939,b=15.3061 45.918 45.918 46.939 46.939 46.939 46.939 47.959 48.98 48.98 50 50 50 coh1242:k=33.6735,b=16.3265 45.918 45.918 46.939 46.939 46.939 46.939 47.959 48.98 48.98 50 50 50 coh1243:k=32.6531,b=17.3469 45.918 45.918 45.918 46.939 46.939 46.939 47.959 48.98 48.98 50 50 50 coh1244:k=31.6327,b=18.3673 45.918 45.918 45.918 46.939 46.939 46.939 47.959 48.98 48.98 50 50 50 coh1245:k=30.6122,b=19.3878 45.918 45.918 45.918 45.918 46.939 46.939 47.959 48.98 48.98 50 50 50 coh1246:k=29.5918,b=20.4082 45.918 45.918 45.918 45.918 46.939 46.939 47.959 48.98 48.98 50 50 50 coh1247:k=28.5714,b=21.4286 45.918 45.918 45.918 45.918 45.918 46.939 47.959 48.98 48.98 50 50 50 coh1248:k=27.551,b=22.449 45.918 45.918 45.918 45.918 45.918 46.939 47.959 47.959 48.98 50 50 50 coh1249:k=26.5306,b=23.4694 45.918 45.918 45.918 45.918 45.918 46.939 47.959 47.959 48.98 50 50 50 coh1250:k=25.5102,b=24.4898 45.918 45.918 45.918 45.918 45.918 46.939 47.959 47.959 48.98 50 50 50 coh1251:k=24.4898,b=25.5102 45.918 45.918 45.918 45.918 45.918 45.918 47.959 47.959 48.98 50 50 50 coh1252:k=23.4694,b=26.5306 44.898 45.918 45.918 45.918 45.918 45.918 47.959 47.959 48.98 50 50 50 coh1253:k=22.449,b=27.551 44.898 45.918 45.918 45.918 45.918 45.918 47.959 47.959 48.98 50 50 50 coh1254:k=21.4286,b=28.5714 44.898 44.898 45.918 45.918 45.918 45.918 47.959 47.959 48.98 50 50 50 coh1255:k=20.4082,b=29.5918 44.898 44.898 44.898 45.918 45.918 45.918 47.959 47.959 48.98 50 50 50 coh1256:k=19.3878,b=30.6122 44.898 44.898 44.898 45.918 45.918 45.918 47.959 47.959 48.98 48.98 50 50 coh1257:k=18.3673,b=31.6327 44.898 44.898 44.898 45.918 45.918 45.918 47.959 47.959 48.98 48.98 50 50 coh1258:k=17.3469,b=32.6531 44.898 44.898 44.898 44.898 45.918 45.918 47.959 47.959 48.98 48.98 50 50 coh1259:k=16.3265,b=33.6735 44.898 44.898 44.898 44.898 45.918 45.918 46.939 47.959 48.98 48.98 50 50 coh1260:k=15.3061,b=34.6939 44.898 44.898 44.898 44.898 45.918 45.918 46.939 47.959 48.98 48.98 50 50 coh1261:k=14.2857,b=35.7143 44.898 44.898 44.898 44.898 44.898 45.918 46.939 47.959 48.98 48.98 50 50 coh1262:k=13.2653,b=36.7347 44.898 44.898 44.898 44.898 44.898 45.918 46.939 47.959 48.98 48.98 50 50 coh1263:k=12.2449,b=37.7551 44.898 44.898 44.898 44.898 44.898 45.918 46.939 47.959 48.98 48.98 50 50 coh1264:k=11.2245,b=38.7755 43.878 44.898 44.898 44.898 44.898 44.898 46.939 47.959 47.959 48.98 50 50 coh1265:k=10.2041,b=39.7959 43.878 43.878 44.898 44.898 44.898 44.898 46.939 47.959 47.959 48.98 50 50 coh1266:k=9.18367,b=40.8163 43.878 43.878 44.898 44.898 44.898 44.898 46.939 47.959 47.959 48.98 50 50 coh1267:k=8.16327,b=41.8367 43.878 43.878 43.878 44.898 44.898 44.898 46.939 47.959 47.959 48.98 50 50 coh1268:k=7.14286,b=42.8571 43.878 43.878 43.878 44.898 44.898 44.898 46.939 47.959 47.959 48.98 50 50 coh1269:k=6.12245,b=43.8776 43.878 43.878 43.878 44.898 44.898 44.898 46.939 47.959 47.959 48.98 50 50 coh1270:k=5.10204,b=44.898 43.878 43.878 43.878 43.878 44.898 44.898 46.939 46.939 47.959 48.98 50 50 coh1271:k=4.08163,b=45.9184 43.878 43.878 43.878 43.878 44.898 44.898 46.939 46.939 47.959 48.98 50 50 coh1272:k=3.06122,b=46.9388 43.878 43.878 43.878 43.878 44.898 44.898 46.939 46.939 47.959 48.98 50 50 coh1273:k=2.04082,b=47.9592 43.878 43.878 43.878 43.878 43.878 44.898 46.939 46.939 47.959 48.98 50 50 coh1274:k=1.02041,b=48.9796 43.878 43.878 43.878 43.878 43.878 44.898 46.939 46.939 47.959 48.98 50 50 coh1275:k=0,b=50 42.857 43.878 43.878 43.878 43.878 44.898 46.939 46.939 47.959 48.98 50 50
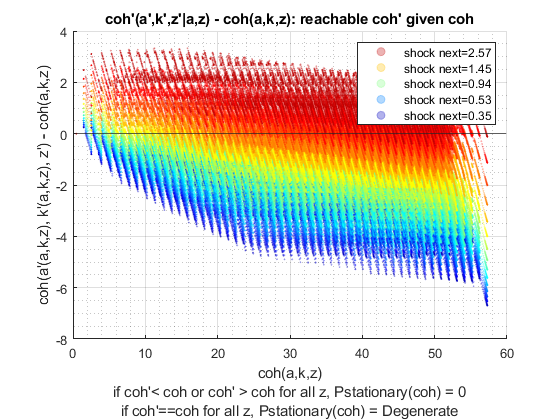
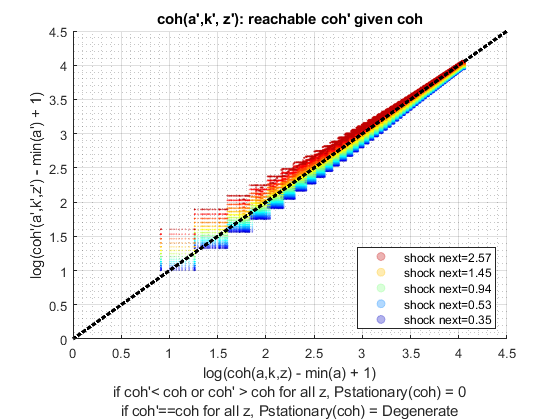
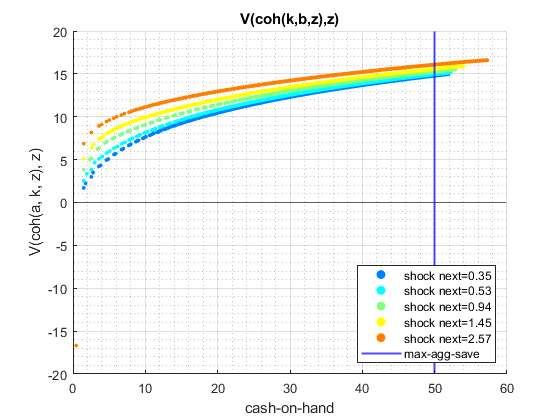
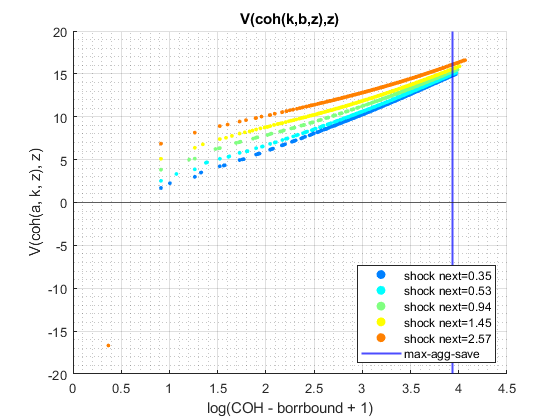
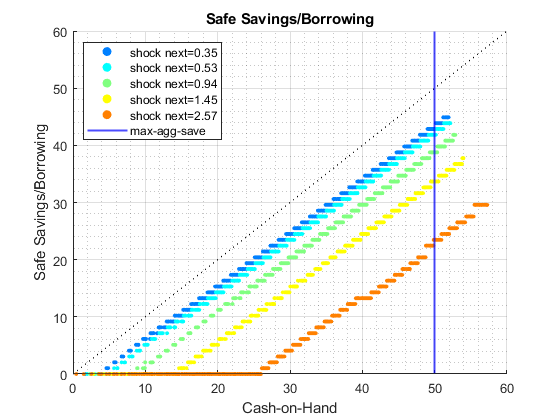
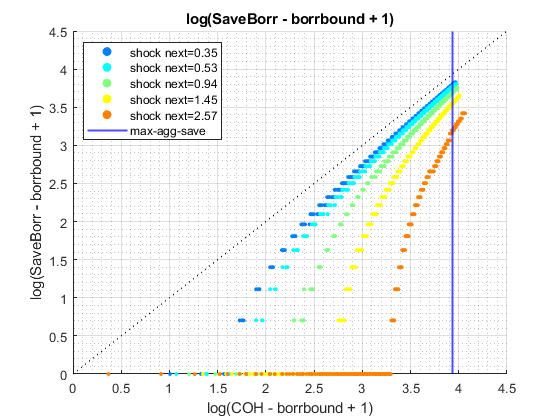
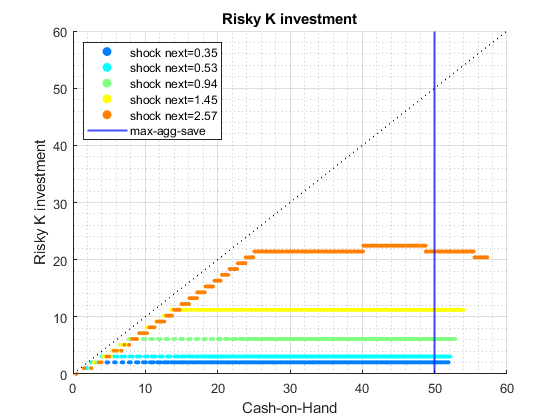
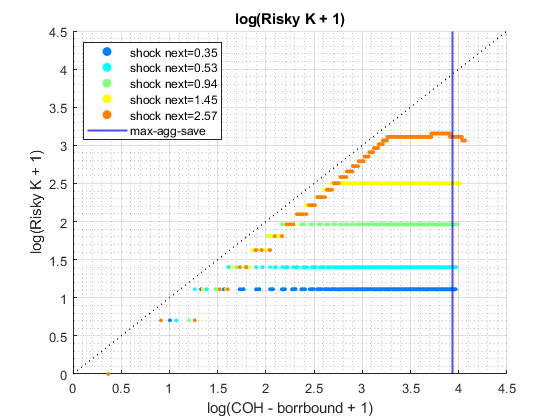
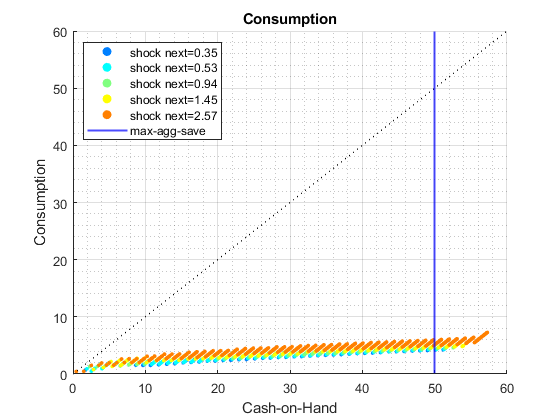
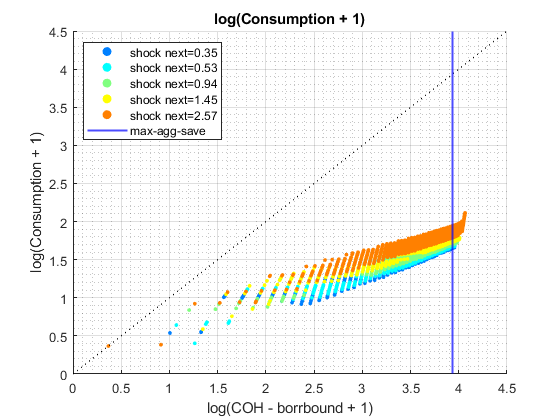
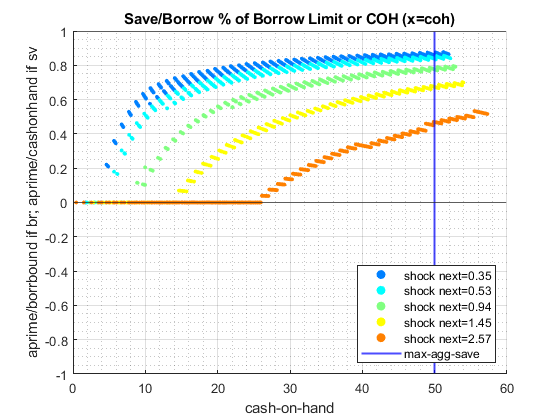
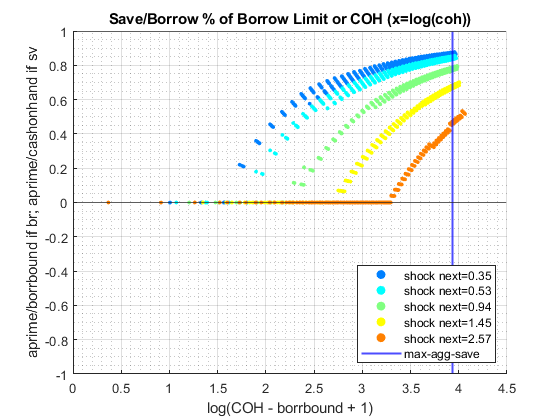
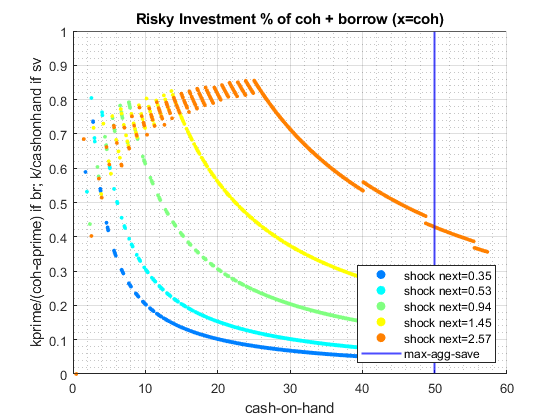
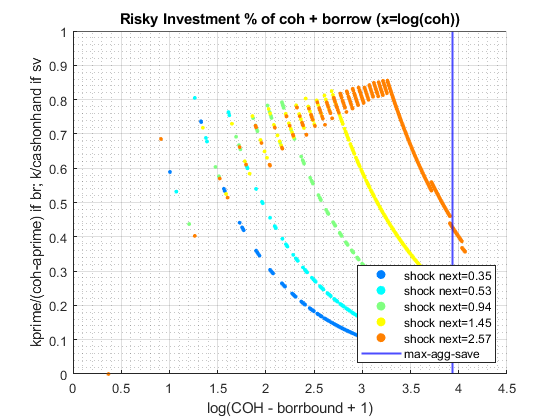
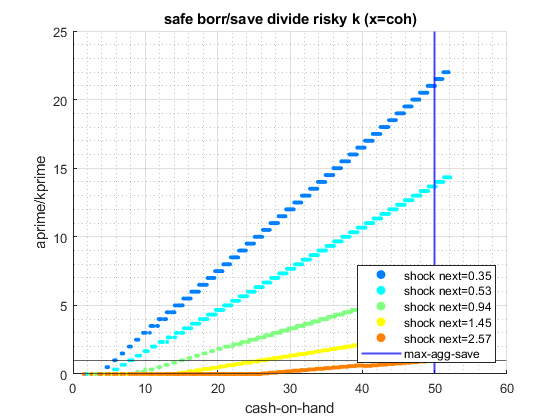
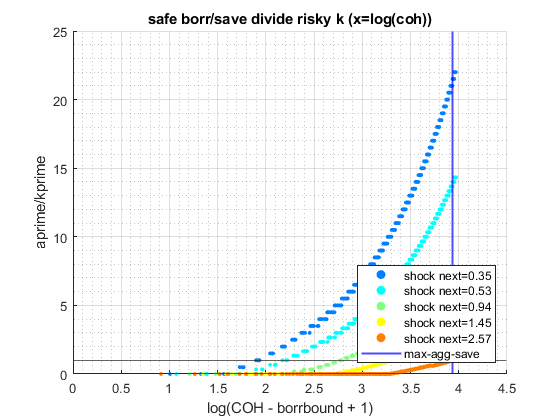
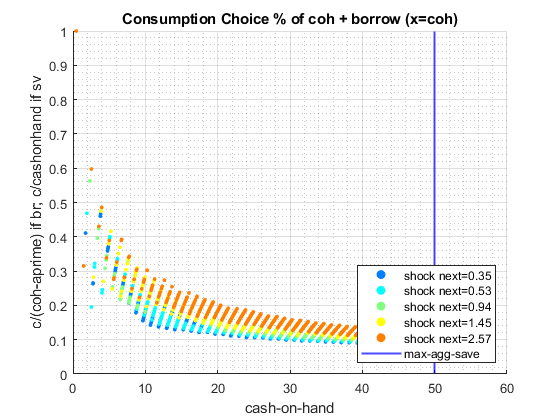
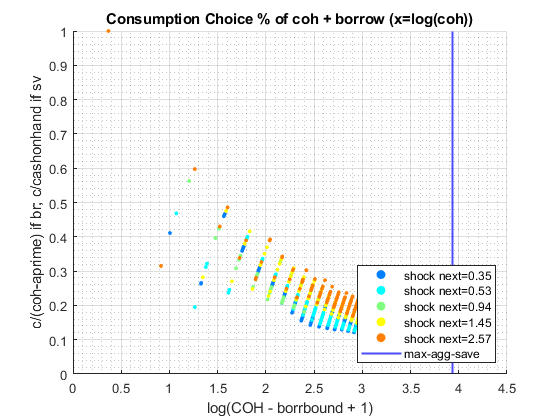
Display Various Containers
if (bl_display_defparam)
Display 1 support_map
fft_container_map_display(support_map, it_display_summmat_rowmax, it_display_summmat_colmax);
---------------------------------------- ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Begin: Show all key and value pairs from container CONTAINER NAME: SUPPORT_MAP ---------------------------------------- Map with properties: Count: 41 KeyType: char ValueType: any xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ---------------------------------------- ---------------------------------------- pos = 1 ; key = bl_display ; val = false pos = 2 ; key = bl_display_defparam ; val = true pos = 3 ; key = bl_display_dist ; val = false pos = 4 ; key = bl_display_evf ; val = false pos = 5 ; key = bl_display_final ; val = true pos = 6 ; key = bl_display_final_dist ; val = false pos = 7 ; key = bl_display_final_dist_detail ; val = false pos = 8 ; key = bl_display_funcgrids ; val = false pos = 9 ; key = bl_graph ; val = true pos = 10 ; key = bl_graph_coh_t_coh ; val = true pos = 11 ; key = bl_graph_evf ; val = false pos = 12 ; key = bl_graph_funcgrids ; val = false pos = 13 ; key = bl_graph_onebyones ; val = true pos = 14 ; key = bl_graph_pol_lvl ; val = true pos = 15 ; key = bl_graph_pol_pct ; val = true pos = 16 ; key = bl_graph_val ; val = true pos = 17 ; key = bl_img_save ; val = false pos = 18 ; key = bl_mat ; val = false pos = 19 ; key = bl_post ; val = true pos = 20 ; key = bl_profile ; val = false pos = 21 ; key = bl_profile_dist ; val = false pos = 22 ; key = bl_time ; val = false pos = 23 ; key = it_display_every ; val = 5 pos = 24 ; key = it_display_final_colmax ; val = 12 pos = 25 ; key = it_display_final_rowmax ; val = 100 pos = 26 ; key = it_display_summmat_colmax ; val = 7 pos = 27 ; key = it_display_summmat_rowmax ; val = 7 pos = 28 ; key = st_img_name_main ; val = ff_akz_vf_vec_default pos = 29 ; key = st_img_path ; val = C:/Users/fan/CodeDynaAsset//m_akz//solve/img/ pos = 30 ; key = st_img_prefix ; val = pos = 31 ; key = st_img_suffix ; val = _p4.png pos = 32 ; key = st_mat_name_main ; val = ff_akz_vf_vec_default pos = 33 ; key = st_mat_path ; val = C:/Users/fan/CodeDynaAsset//m_akz//solve/mat/ pos = 34 ; key = st_mat_prefix ; val = pos = 35 ; key = st_mat_suffix ; val = _p4 pos = 36 ; key = st_matimg_path_root ; val = C:/Users/fan/CodeDynaAsset//m_akz/ pos = 37 ; key = st_profile_name_main ; val = ff_akz_vf_vec_default pos = 38 ; key = st_profile_path ; val = C:/Users/fan/CodeDynaAsset//m_akz//solve/profile/ pos = 39 ; key = st_profile_prefix ; val = pos = 40 ; key = st_profile_suffix ; val = _p4 pos = 41 ; key = st_title_prefix ; val = ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Scalars in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx value __ ___ _____ bl_display 1 1 0 bl_display_defparam 2 2 1 bl_display_dist 3 3 0 bl_display_evf 4 4 0 bl_display_final 5 5 1 bl_display_final_dist 6 6 0 bl_display_final_dist_detail 7 7 0 bl_display_funcgrids 8 8 0 bl_graph 9 9 1 bl_graph_coh_t_coh 10 10 1 bl_graph_evf 11 11 0 bl_graph_funcgrids 12 12 0 bl_graph_onebyones 13 13 1 bl_graph_pol_lvl 14 14 1 bl_graph_pol_pct 15 15 1 bl_graph_val 16 16 1 bl_img_save 17 17 0 bl_mat 18 18 0 bl_post 19 19 1 bl_profile 20 20 0 bl_profile_dist 21 21 0 bl_time 22 22 0 it_display_every 23 23 5 it_display_final_colmax 24 24 12 it_display_final_rowmax 25 25 100 it_display_summmat_colmax 26 26 7 it_display_summmat_rowmax 27 27 7 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Strings in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx __ ___ st_img_name_main 1 28 st_img_path 2 29 st_img_prefix 3 30 st_img_suffix 4 31 st_mat_name_main 5 32 st_mat_path 6 33 st_mat_prefix 7 34 st_mat_suffix 8 35 st_matimg_path_root 9 36 st_profile_name_main 10 37 st_profile_path 11 38 st_profile_prefix 12 39 st_profile_suffix 13 40 st_title_prefix 14 41
Display 2 armt_map
fft_container_map_display(armt_map, it_display_summmat_rowmax, it_display_summmat_colmax);
---------------------------------------- ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Begin: Show all key and value pairs from container CONTAINER NAME: ARMT_MAP ---------------------------------------- Map with properties: Count: 17 KeyType: char ValueType: any xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ---------------------------------------- ---------------------------------------- pos = 1 ; key = ar_a_meshk ;rown= 1275 ,coln= 1 ar_a_meshk :mu= 16.6667 ,sd= 12.1453 ,min= 0 ,max= 50 zi_1_c1 _______ zi_1_R1 0 zi_2_R2 1.0204 zi_3_R3 0 zi_638_R638 28.571 zi_1273_r1273 2.0408 zi_1274_r1274 1.0204 zi_1275_r1275 0 pos = 2 ; key = ar_a_mw_wth_na ;rown= 2500 ,coln= 1 ar_a_mw_wth_na :mu= NaN ,sd= NaN ,min= 0 ,max= 50 zi_1_c1 _______ zi_1_R1 0 zi_2_R2 NaN zi_3_R3 NaN zi_1250_r1250 NaN zi_2498_r2498 2.0408 zi_2499_r2499 1.0204 zi_2500_r2500 0 pos = 3 ; key = ar_interp_c_grid ;rown= 1 ,coln= 572761 ar_interp_c_grid :mu= 28.6391 ,sd= 16.5342 ,min= 0.001 ,max= 57.2772 zi_1_C1 zi_2_C2 zi_3_C3 zi_286381_c286381 zi_572759_c572759 zi_572760_c572760 zi_572761_c572761 _______ _______ _______ _________________ _________________ _________________ _________________ zi_1_r1 0.001 0.0011 0.0012 28.639 57.277 57.277 57.277 pos = 4 ; key = ar_interp_coh_grid ;rown= 1 ,coln= 568 ar_interp_coh_grid :mu= 28.8604 ,sd= 16.4498 ,min= 0.44365 ,max= 57.2772 zi_1_C1 zi_2_C2 zi_3_C3 zi_284_c284 zi_566_c566 zi_567_c567 zi_568_c568 _______ _______ _______ ___________ ___________ ___________ ___________ zi_1_r1 0.44365 0.54388 0.64412 28.81 57.077 57.177 57.277 pos = 5 ; key = ar_k ;rown= 1 ,coln= 50 ar_k :mu= 25 ,sd= 14.8749 ,min= 0 ,max= 50 zi_1_C1 zi_2_C2 zi_3_C3 zi_25_c25 zi_48_c48 zi_49_c49 zi_50_c50 _______ _______ _______ _________ _________ _________ _________ zi_1_r1 0 1.0204 2.0408 24.49 47.959 48.98 50 pos = 6 ; key = ar_k_mesha ;rown= 1275 ,coln= 1 ar_k_mesha :mu= 16.6667 ,sd= 12.1453 ,min= 0 ,max= 50 zi_1_c1 _______ zi_1_R1 0 zi_2_R2 0 zi_3_R3 1.0204 zi_638_R638 7.1429 zi_1273_r1273 47.959 zi_1274_r1274 48.98 zi_1275_r1275 50 pos = 7 ; key = ar_k_mw_wth_na ;rown= 2500 ,coln= 1 ar_k_mw_wth_na :mu= NaN ,sd= NaN ,min= 0 ,max= 50 zi_1_c1 _______ zi_1_R1 0 zi_2_R2 NaN zi_3_R3 NaN zi_1250_r1250 NaN zi_2498_r2498 47.959 zi_2499_r2499 48.98 zi_2500_r2500 50 pos = 8 ; key = ar_stationary ;rown= 1 ,coln= 15 ar_stationary :mu= 0.066667 ,sd= 0.060897 ,min= 0.0027089 ,max= 0.16757 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 _________ _________ ________ _______ _________ _________ _________ zi_1_r1 0.0027089 0.0069499 0.018507 0.16757 0.018507 0.0069499 0.0027089 pos = 9 ; key = ar_w ;rown= 1 ,coln= 50 ar_w :mu= 25 ,sd= 14.8749 ,min= 0 ,max= 50 zi_1_C1 zi_2_C2 zi_3_C3 zi_25_c25 zi_48_c48 zi_49_c49 zi_50_c50 _______ _______ _______ _________ _________ _________ _________ zi_1_r1 0 1.0204 2.0408 24.49 47.959 48.98 50 pos = 10 ; key = ar_z ;rown= 1 ,coln= 15 ar_z :mu= 1.1347 ,sd= 0.69878 ,min= 0.34741 ,max= 2.567 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 _______ _______ _______ _______ _________ _________ _________ zi_1_r1 0.34741 0.40076 0.4623 0.94436 1.9291 2.2253 2.567 pos = 11 ; key = it_ameshk_n ; val = 1275 pos = 12 ; key = mt_coh_wkb ;rown= 1275 ,coln= 15 mt_coh_wkb :mu= 35.7035 ,sd= 12.4237 ,min= 0.44365 ,max= 57.2772 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 _______ _______ _______ _______ _________ _________ _________ zi_1_R1 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 zi_2_R2 1.4896 1.4896 1.4896 1.4896 1.4896 1.4896 1.4896 zi_3_R3 1.7324 1.7861 1.8481 2.3337 3.3256 3.624 3.9682 zi_638_R638 37.006 37.114 37.239 38.217 40.216 40.817 41.511 zi_1273_r1273 48.057 48.272 48.52 50.462 54.429 55.622 56.998 zi_1274_r1274 47.961 48.177 48.427 50.384 54.381 55.583 56.97 zi_1275_r1275 47.864 48.082 48.334 50.305 54.332 55.543 56.941 pos = 13 ; key = mt_interp_coh_grid_mesh_z ;rown= 568 ,coln= 15 mt_interp_coh_grid_mesh_z :mu= 28.8604 ,sd= 16.4363 ,min= 0.44365 ,max= 57.2772 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 _______ _______ _______ _______ _________ _________ _________ zi_1_R1 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 zi_2_R2 0.54388 0.54388 0.54388 0.54388 0.54388 0.54388 0.54388 zi_3_R3 0.64412 0.64412 0.64412 0.64412 0.64412 0.64412 0.64412 zi_284_r284 28.81 28.81 28.81 28.81 28.81 28.81 28.81 zi_566_r566 57.077 57.077 57.077 57.077 57.077 57.077 57.077 zi_567_r567 57.177 57.177 57.177 57.177 57.177 57.177 57.177 zi_568_r568 57.277 57.277 57.277 57.277 57.277 57.277 57.277 pos = 14 ; key = mt_k_wth_na ;rown= 50 ,coln= 50 mt_k_wth_na :mu= NaN ,sd= NaN ,min= 0 ,max= 50 zi_1_C1 zi_2_C2 zi_3_C3 zi_25_c25 zi_48_c48 zi_49_c49 zi_50_c50 _______ _______ _______ _________ _________ _________ _________ zi_1_R1 0 0 0 0 0 0 0 zi_2_R2 NaN 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 zi_3_R3 NaN NaN 2.0408 2.0408 2.0408 2.0408 2.0408 zi_25_r25 NaN NaN NaN 24.49 24.49 24.49 24.49 zi_48_r48 NaN NaN NaN NaN 47.959 47.959 47.959 zi_49_r49 NaN NaN NaN NaN NaN 48.98 48.98 zi_50_r50 NaN NaN NaN NaN NaN NaN 50 pos = 15 ; key = mt_z_mesh_coh_interp_grid ;rown= 568 ,coln= 15 mt_z_mesh_coh_interp_grid :mu= 1.1347 ,sd= 0.67512 ,min= 0.34741 ,max= 2.567 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 _______ _______ _______ _______ _________ _________ _________ zi_1_R1 0.34741 0.40076 0.4623 0.94436 1.9291 2.2253 2.567 zi_2_R2 0.34741 0.40076 0.4623 0.94436 1.9291 2.2253 2.567 zi_3_R3 0.34741 0.40076 0.4623 0.94436 1.9291 2.2253 2.567 zi_284_r284 0.34741 0.40076 0.4623 0.94436 1.9291 2.2253 2.567 zi_566_r566 0.34741 0.40076 0.4623 0.94436 1.9291 2.2253 2.567 zi_567_r567 0.34741 0.40076 0.4623 0.94436 1.9291 2.2253 2.567 zi_568_r568 0.34741 0.40076 0.4623 0.94436 1.9291 2.2253 2.567 pos = 16 ; key = mt_z_mesh_coh_wkb ;rown= 1275 ,coln= 15 mt_z_mesh_coh_wkb :mu= 1.1347 ,sd= 0.6751 ,min= 0.34741 ,max= 2.567 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 _______ _______ _______ _______ _________ _________ _________ zi_1_R1 0.34741 0.40076 0.4623 0.94436 1.9291 2.2253 2.567 zi_2_R2 0.34741 0.40076 0.4623 0.94436 1.9291 2.2253 2.567 zi_3_R3 0.34741 0.40076 0.4623 0.94436 1.9291 2.2253 2.567 zi_638_R638 0.34741 0.40076 0.4623 0.94436 1.9291 2.2253 2.567 zi_1273_r1273 0.34741 0.40076 0.4623 0.94436 1.9291 2.2253 2.567 zi_1274_r1274 0.34741 0.40076 0.4623 0.94436 1.9291 2.2253 2.567 zi_1275_r1275 0.34741 0.40076 0.4623 0.94436 1.9291 2.2253 2.567 pos = 17 ; key = mt_z_trans ;rown= 15 ,coln= 15 mt_z_trans :mu= 0.066667 ,sd= 0.095337 ,min= 0 ,max= 0.27902 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 __________ __________ __________ __________ __________ __________ __________ zi_1_R1 0.26016 0.26831 0.25551 0.00012823 2.7001e-13 1.1102e-15 0 zi_2_R2 0.11232 0.19622 0.2763 0.00098855 1.5289e-11 9.3592e-14 3.3307e-16 zi_3_R3 0.037073 0.10492 0.2185 0.0055558 6.2811e-10 5.7438e-12 3.1863e-14 zi_8_R8 1.7181e-06 4.1008e-05 0.00061112 0.27902 0.00061112 4.1008e-05 1.7181e-06 zi_13_r13 3.1909e-14 5.7438e-12 6.2811e-10 0.0055558 0.2185 0.10492 0.037073 zi_14_r14 3.474e-16 9.3597e-14 1.5289e-11 0.00098855 0.2763 0.19622 0.11232 zi_15_r15 2.7412e-18 1.1057e-15 2.6998e-13 0.00012823 0.25551 0.26831 0.26016 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Matrix in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx rowN colN mean std min max __ ___ ____ __________ ________ ________ _________ _______ ar_a_meshk 1 1 1275 1 16.667 12.145 0 50 ar_a_mw_wth_na 2 2 2500 1 NaN NaN 0 50 ar_interp_c_grid 3 3 1 5.7276e+05 28.639 16.534 0.001 57.277 ar_interp_coh_grid 4 4 1 568 28.86 16.45 0.44365 57.277 ar_k 5 5 1 50 25 14.875 0 50 ar_k_mesha 6 6 1275 1 16.667 12.145 0 50 ar_k_mw_wth_na 7 7 2500 1 NaN NaN 0 50 ar_stationary 8 8 1 15 0.066667 0.060897 0.0027089 0.16757 ar_w 9 9 1 50 25 14.875 0 50 ar_z 10 10 1 15 1.1347 0.69878 0.34741 2.567 mt_coh_wkb 11 12 1275 15 35.704 12.424 0.44365 57.277 mt_interp_coh_grid_mesh_z 12 13 568 15 28.86 16.436 0.44365 57.277 mt_k_wth_na 13 14 50 50 NaN NaN 0 50 mt_z_mesh_coh_interp_grid 14 15 568 15 1.1347 0.67512 0.34741 2.567 mt_z_mesh_coh_wkb 15 16 1275 15 1.1347 0.6751 0.34741 2.567 mt_z_trans 16 17 15 15 0.066667 0.095337 0 0.27902 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Scalars in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx value _ ___ _____ it_ameshk_n 1 11 1275
Display 3 param_map
fft_container_map_display(param_map, it_display_summmat_rowmax, it_display_summmat_colmax);
---------------------------------------- ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Begin: Show all key and value pairs from container CONTAINER NAME: PARAM_MAP ---------------------------------------- Map with properties: Count: 32 KeyType: char ValueType: any xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ---------------------------------------- ---------------------------------------- pos = 1 ; key = bl_default ; val = 0 pos = 2 ; key = fl_Amean ; val = 1 pos = 3 ; key = fl_alpha ; val = 0.36 pos = 4 ; key = fl_b_bd ; val = 0 pos = 5 ; key = fl_beta ; val = 0.94 pos = 6 ; key = fl_c_min ; val = 0.001 pos = 7 ; key = fl_coh_interp_grid_gap ; val = 0.1 pos = 8 ; key = fl_crra ; val = 1.5 pos = 9 ; key = fl_default_aprime ; val = 0 pos = 10 ; key = fl_delta ; val = 0.08 pos = 11 ; key = fl_k_min ; val = 0 pos = 12 ; key = fl_nan_replace ; val = -9999 pos = 13 ; key = fl_r_borr ; val = 0.025 pos = 14 ; key = fl_r_save ; val = 0.025 pos = 15 ; key = fl_tol_dist ; val = 1e-05 pos = 16 ; key = fl_tol_pol ; val = 1e-05 pos = 17 ; key = fl_tol_val ; val = 1e-05 pos = 18 ; key = fl_w ; val = 0.44365 pos = 19 ; key = fl_w_max ; val = 50 pos = 20 ; key = fl_z_mu ; val = 0 pos = 21 ; key = fl_z_rho ; val = 0.8 pos = 22 ; key = fl_z_sig ; val = 0.2 pos = 23 ; key = it_ak_n ; val = 50 pos = 24 ; key = it_c_interp_grid_gap ; val = 0.0001 pos = 25 ; key = it_maxiter_dist ; val = 1000 pos = 26 ; key = it_maxiter_val ; val = 250 pos = 27 ; key = it_tol_pol_nochange ; val = 25 pos = 28 ; key = it_trans_power_dist ; val = 1000 pos = 29 ; key = it_w_n ; val = 50 pos = 30 ; key = it_z_n ; val = 15 pos = 31 ; key = st_analytical_stationary_type ; val = eigenvector pos = 32 ; key = st_model ; val = akz_wkz_iwkz ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Scalars in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx value __ ___ _______ bl_default 1 1 0 fl_Amean 2 2 1 fl_alpha 3 3 0.36 fl_b_bd 4 4 0 fl_beta 5 5 0.94 fl_c_min 6 6 0.001 fl_coh_interp_grid_gap 7 7 0.1 fl_crra 8 8 1.5 fl_default_aprime 9 9 0 fl_delta 10 10 0.08 fl_k_min 11 11 0 fl_nan_replace 12 12 -9999 fl_r_borr 13 13 0.025 fl_r_save 14 14 0.025 fl_tol_dist 15 15 1e-05 fl_tol_pol 16 16 1e-05 fl_tol_val 17 17 1e-05 fl_w 18 18 0.44365 fl_w_max 19 19 50 fl_z_mu 20 20 0 fl_z_rho 21 21 0.8 fl_z_sig 22 22 0.2 it_ak_n 23 23 50 it_c_interp_grid_gap 24 24 0.0001 it_maxiter_dist 25 25 1000 it_maxiter_val 26 26 250 it_tol_pol_nochange 27 27 25 it_trans_power_dist 28 28 1000 it_w_n 29 29 50 it_z_n 30 30 15 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Strings in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx _ ___ st_analytical_stationary_type 1 31 st_model 2 32
Display 4 func_map
fft_container_map_display(func_map, it_display_summmat_rowmax, it_display_summmat_colmax);
---------------------------------------- ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Begin: Show all key and value pairs from container CONTAINER NAME: FUNC_MAP ---------------------------------------- Map with properties: Count: 7 KeyType: char ValueType: any xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ---------------------------------------- ---------------------------------------- pos = 1 ; key = f_coh ; val = @(z,b,k)(f_prod(z,k)+k*(1-fl_delta)+fl_w+b.*(1+fl_r_save).*(b>0)+b.*(1+fl_r_borr).*(b<=0)) pos = 2 ; key = f_cons ; val = @(coh,bprime,kprime)(coh-kprime-bprime) pos = 3 ; key = f_inc ; val = @(z,b,k)(f_prod(z,k)-(fl_delta)*k+fl_w+b.*(fl_r_save).*(b>0)+b.*(fl_r_borr).*(b<=0)) pos = 4 ; key = f_prod ; val = @(z,k)((fl_Amean.*(z)).*(k.^(fl_alpha))) pos = 5 ; key = f_util_crra ; val = @(c)(((c).^(1-fl_crra)-1)./(1-fl_crra)) pos = 6 ; key = f_util_log ; val = @(c)log(c) pos = 7 ; key = f_util_standin ; val = @(z,b,k)f_util_log(f_coh(z,b,k).*(f_coh(z,b,k)>0)+fl_c_min.*(f_coh(z,b,k)<=0)) ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Scalars in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx xFunction _ ___ _________ f_coh 1 1 1 f_cons 2 2 2 f_inc 3 3 3 f_prod 4 4 4 f_util_crra 5 5 5 f_util_log 6 6 6 f_util_standin 7 7 7
Display 5 result_map
fft_container_map_display(result_map, it_display_summmat_rowmax, it_display_summmat_colmax);
---------------------------------------- ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Begin: Show all key and value pairs from container CONTAINER NAME: RESULT_MAP ---------------------------------------- Map with properties: Count: 13 KeyType: char ValueType: any xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ---------------------------------------- ---------------------------------------- pos = 1 ; key = ar_pol_diff_norm ;rown= 100 ,coln= 1 ar_pol_diff_norm :mu= 63.3948 ,sd= 296.4925 ,min= 0 ,max= 2790.3913 zi_1_c1 _______ zi_1_R1 276.59 zi_2_R2 2790.4 zi_3_R3 920.98 zi_50_R50 14.289 zi_98_R98 0 zi_99_R99 0 zi_100_r100 0 pos = 2 ; key = ar_st_pol_names ; val = cl_mt_coh cl_mt_pol_a cl_mt_pol_k cl_mt_pol_c pos = 3 ; key = ar_val_diff_norm ;rown= 100 ,coln= 1 ar_val_diff_norm :mu= 18.9698 ,sd= 39.3221 ,min= 0.11317 ,max= 227.0448 zi_1_c1 _______ zi_1_R1 227.04 zi_2_R2 179.64 zi_3_R3 151.16 zi_50_R50 2.611 zi_98_R98 0.12809 zi_99_R99 0.1204 zi_100_r100 0.11317 pos = 4 ; key = cl_mt_coh ;rown= 1275 ,coln= 15 cl_mt_coh :mu= 35.7035 ,sd= 12.4237 ,min= 0.44365 ,max= 57.2772 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 _______ _______ _______ _______ _________ _________ _________ zi_1_R1 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 zi_2_R2 1.4896 1.4896 1.4896 1.4896 1.4896 1.4896 1.4896 zi_3_R3 1.7324 1.7861 1.8481 2.3337 3.3256 3.624 3.9682 zi_638_R638 37.006 37.114 37.239 38.217 40.216 40.817 41.511 zi_1273_r1273 48.057 48.272 48.52 50.462 54.429 55.622 56.998 zi_1274_r1274 47.961 48.177 48.427 50.384 54.381 55.583 56.97 zi_1275_r1275 47.864 48.082 48.334 50.305 54.332 55.543 56.941 pos = 5 ; key = cl_mt_cons ;rown= 1275 ,coln= 15 cl_mt_cons :mu= 4.1196 ,sd= 0.94111 ,min= 0.44365 ,max= 7.2772 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 _______ _______ _______ _______ _________ _________ _________ zi_1_R1 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 zi_2_R2 0.46916 0.46916 0.46916 0.46916 0.46916 0.46916 0.46916 zi_3_R3 0.71196 0.7657 0.82769 1.3133 1.2848 1.5831 1.9274 zi_638_R638 4.3528 3.4407 3.5656 4.5439 4.5016 5.1028 4.7759 zi_1273_r1273 4.1798 4.3947 4.6426 4.5436 5.449 5.6219 6.9985 zi_1274_r1274 4.0833 4.2999 4.5497 4.4654 5.401 5.583 6.97 zi_1275_r1275 5.0071 4.2048 4.4565 5.4073 5.3522 5.5431 6.9405 pos = 6 ; key = cl_mt_pol_a ;rown= 1275 ,coln= 15 cl_mt_pol_a :mu= 23.399 ,sd= 11.8889 ,min= 0 ,max= 44.898 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 _______ _______ _______ _______ _________ _________ _________ zi_1_R1 0 0 0 0 0 0 0 zi_2_R2 0 0 0 0 0 0 0 zi_3_R3 0 0 0 0 0 0 0 zi_638_R638 30.612 31.633 30.612 27.551 20.408 17.347 14.286 zi_1273_r1273 41.837 41.837 40.816 39.796 33.673 31.633 29.592 zi_1274_r1274 41.837 41.837 40.816 39.796 33.673 31.633 29.592 zi_1275_r1275 40.816 41.837 40.816 38.776 33.673 31.633 29.592 pos = 7 ; key = cl_mt_pol_c ;rown= 1275 ,coln= 15 cl_mt_pol_c :mu= 4.1196 ,sd= 0.94111 ,min= 0.44365 ,max= 7.2772 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 _______ _______ _______ _______ _________ _________ _________ zi_1_R1 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 0.44365 zi_2_R2 0.46916 0.46916 0.46916 0.46916 0.46916 0.46916 0.46916 zi_3_R3 0.71196 0.7657 0.82769 1.3133 1.2848 1.5831 1.9274 zi_638_R638 4.3528 3.4407 3.5656 4.5439 4.5016 5.1028 4.7759 zi_1273_r1273 4.1798 4.3947 4.6426 4.5436 5.449 5.6219 6.9985 zi_1274_r1274 4.0833 4.2999 4.5497 4.4654 5.401 5.583 6.97 zi_1275_r1275 5.0071 4.2048 4.4565 5.4073 5.3522 5.5431 6.9405 pos = 8 ; key = cl_mt_pol_k ;rown= 1275 ,coln= 15 cl_mt_pol_k :mu= 8.185 ,sd= 5.8646 ,min= 0 ,max= 22.449 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 _______ _______ _______ _______ _________ _________ _________ zi_1_R1 0 0 0 0 0 0 0 zi_2_R2 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 1.0204 zi_3_R3 1.0204 1.0204 1.0204 1.0204 2.0408 2.0408 2.0408 zi_638_R638 2.0408 2.0408 3.0612 6.1224 15.306 18.367 22.449 zi_1273_r1273 2.0408 2.0408 3.0612 6.1224 15.306 18.367 20.408 zi_1274_r1274 2.0408 2.0408 3.0612 6.1224 15.306 18.367 20.408 zi_1275_r1275 2.0408 2.0408 3.0612 6.1224 15.306 18.367 20.408 pos = 9 ; key = mt_pol_perc_change ;rown= 100 ,coln= 15 mt_pol_perc_change :mu= 0.069308 ,sd= 0.18182 ,min= 0 ,max= 1 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 _______ __________ __________ __________ __________ __________ __________ zi_1_R1 1 1 1 1 1 1 1 zi_2_R2 0.99137 0.99137 0.98549 0.95804 0.80157 0.72 0.63529 zi_3_R3 0.65176 0.65098 0.52314 0.62275 0.63294 0.5949 0.62627 zi_50_R50 0 0.00078431 0.00039216 0.00078431 0.00078431 0.00078431 0.00078431 zi_98_R98 0 0 0 0 0 0 0 zi_99_R99 0 0 0 0 0 0 0 zi_100_r100 0 0 0 0 0 0 0 pos = 10 ; key = mt_val ;rown= 1275 ,coln= 15 mt_val :mu= 13.5293 ,sd= 2.155 ,min= -16.6792 ,max= 16.5973 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 _______ _______ _______ _______ _________ _________ _________ zi_1_R1 -16.679 -16.679 -16.679 -16.679 -16.679 -16.679 -16.679 zi_2_R2 1.6951 1.9479 2.2271 3.8278 6.0229 6.4636 6.8568 zi_3_R3 2.2448 2.5822 2.9487 5.0025 8.0338 8.5908 9.0897 zi_638_R638 13.308 13.382 13.47 14.046 14.929 15.144 15.363 zi_1273_r1273 14.587 14.66 14.743 15.295 16.162 16.376 16.583 zi_1274_r1274 14.576 14.65 14.734 15.287 16.158 16.373 16.581 zi_1275_r1275 14.566 14.639 14.724 15.28 16.155 16.37 16.579 pos = 11 ; key = tb_pol_a ;rown= 100 ,coln= 12 tb_pol_a :mu= 19.7602 ,sd= 19.0738 ,min= 0 ,max= 44.898 zi_1_C1 zi_2_C2 zi_3_C3 zi_6_C6 zi_10_c10 zi_11_c11 zi_12_c12 _______ _______ _______ _______ _________ _________ _________ zi_1_R1 0 0 0 0 0 0 0 zi_2_R2 0 0 0 0 0 0 0 zi_3_R3 0 0 0 0 0 0 0 zi_50_R50 6.1224 6.1224 5.102 4.0816 0 0 0 zi_98_R98 41.837 41.837 40.816 40.816 33.673 31.633 29.592 zi_99_R99 41.837 41.837 40.816 40.816 33.673 31.633 29.592 zi_100_r100 40.816 41.837 40.816 40.816 33.673 31.633 29.592 pos = 12 ; key = tb_val ;rown= 100 ,coln= 12 tb_val :mu= 11.6227 ,sd= 4.8814 ,min= -16.6792 ,max= 16.5973 zi_1_C1 zi_2_C2 zi_3_C3 zi_6_C6 zi_10_c10 zi_11_c11 zi_12_c12 _______ _______ _______ _______ _________ _________ _________ zi_1_R1 -16.679 -16.679 -16.679 -16.679 -16.679 -16.679 -16.679 zi_2_R2 1.6951 1.9479 2.2271 3.1427 6.0229 6.4636 6.8568 zi_3_R3 2.2448 2.5822 2.9487 4.1353 8.0338 8.5908 9.0897 zi_50_R50 7.6309 7.818 8.0283 8.7664 11.134 11.533 11.901 zi_98_R98 14.587 14.66 14.743 15.045 16.162 16.376 16.583 zi_99_R99 14.576 14.65 14.734 15.036 16.158 16.373 16.581 zi_100_r100 14.566 14.639 14.724 15.027 16.155 16.37 16.579 pos = 13 ; key = tb_valpol_alliter ;rown= 100 ,coln= 17 tb_valpol_alliter :mu= 4.9061 ,sd= 73.7979 ,min= 0 ,max= 2790.3913 zi_1_C1 zi_2_C2 zi_3_C3 zi_9_C9 zi_15_c15 zi_16_c16 zi_17_c17 _______ _______ _______ __________ __________ __________ __________ zi_1_R1 227.04 276.59 1 1 1 1 1 zi_2_R2 179.64 2790.4 0.99137 0.96902 0.80157 0.72 0.63529 zi_3_R3 151.16 920.98 0.65176 0.71686 0.63294 0.5949 0.62627 zi_50_R50 2.611 14.289 0 0.00039216 0.00078431 0.00078431 0.00078431 zi_98_R98 0.12809 0 0 0 0 0 0 zi_99_R99 0.1204 0 0 0 0 0 0 zi_100_r100 0.11317 0 0 0 0 0 0 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Matrix in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx rowN colN mean std min max __ ___ ____ ____ ________ _______ _______ ______ ar_pol_diff_norm 1 1 100 1 63.395 296.49 0 2790.4 ar_val_diff_norm 2 3 100 1 18.97 39.322 0.11317 227.04 cl_mt_coh 3 4 1275 15 35.704 12.424 0.44365 57.277 cl_mt_cons 4 5 1275 15 4.1196 0.94111 0.44365 7.2772 cl_mt_pol_a 5 6 1275 15 23.399 11.889 0 44.898 cl_mt_pol_c 6 7 1275 15 4.1196 0.94111 0.44365 7.2772 cl_mt_pol_k 7 8 1275 15 8.185 5.8646 0 22.449 mt_pol_perc_change 8 9 100 15 0.069308 0.18182 0 1 mt_val 9 10 1275 15 13.529 2.155 -16.679 16.597 tb_pol_a 10 11 100 12 19.76 19.074 0 44.898 tb_val 11 12 100 12 11.623 4.8814 -16.679 16.597 tb_valpol_alliter 12 13 100 17 4.9061 73.798 0 2790.4 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Strings in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx _ ___ ar_st_pol_names 1 2
end
end
ans = Map with properties: Count: 13 KeyType: char ValueType: any