Generate States/Choices/Shocks Grids, get Functions (Interpolated + Percentage + Risky + Safe Asset + Save + Borrow + R Shock)
back to Fan's Dynamic Assets Repository Table of Content.
Contents
- FFS_IPWKBZR_GET_FUNCGRID get funcs, params, states choices shocks grids
- Default
- Parse Parameters 1a
- Parse Parameters 1b
- Parse Parameters 2
- Parse Parameters 3
- F1: Get Shock: Income Shock (ar1)
- F2: Get Shock: Interest Rate Shock (iid)
- G: Generate Asset and Choice Grid for 2nd stage Problem
- F3: Get Shock: Mesh Shocks Together
- Get Equations
- Generate Cash-on-Hand/State Matrix
- Check if COH is within Borrowing Bounds
- Update Valid 2nd stage choice matrix
- Generate 2nd Stage Cash-on-Hand Points for Interpolation
- Generate 1st Stage States: Interpolation Cash-on-hand Interpolation Grid
- Generate 1st Stage Choices: Interpolation Cash-on-hand Interpolation Grid
- Generate Interpolation Consumption Grid
- Initialize armt_map to store, state, choice, shock matrixes
- Store armt_map (1): base arrays
- Store armt_map (2): 1st stage level coh on hand related arrays
- Store armt_map (3): 2nd stage reachable coh(k(w), a(w,k), z', r)
- Store armt_map (4): 2nd stage additional arrays
- Store armt_map (5): Shock Grids Arrays and Mesh
- Store Function Map
- Graph
- Generate Limited Legends
- Graph 1: a and k choice grid graphs
- Graph 2: coh by shock
- Graph 3: 1st State Aggregate Savings Choices by COH interpolation grids
- Graph Details, Generally do Not Run
- Graph 1: 2nd stage coh reached by k' b' choices by index
- Graph 2: 2nd stage coh reached by k' b' choices by coh
- Display
- Display
function [armt_map, func_map] = ffs_ipwkbzr_get_funcgrid(varargin)
FFS_IPWKBZR_GET_FUNCGRID get funcs, params, states choices shocks grids
centralized gateway for retrieving parameters, and solution grids and functions. Similar to ffs_akz_get_funcgrid function. This code deals with problems with savings and borrowing.
This file is a continuation of ffs_ipwkz_get_funcgrid. A significant change here is the inclusion of borrowing shocks, which changes significantly the implementation of the two-stage solution structure. Specifically, for the coh matrix here, there is another dimension which is the borrowing interest rate shock states, which expands the number of cash-on-hand points given the same productivity shock z. So compared to ffs_ipwkz_get_funcgrid, the same number of columns for the mt_coh_wkb_full matirx, but more rows.
Note that for borrowing, we can not start at the min(coh(k,w-k,z)) reacheable given the w and k choice grids. That would be: min(coh(k,w-k,z)) < min(w). At min(coh), there is a single point, and also for all values between min(coh) and min(w), all percentage w_perc choices are below min(w), requiring extrapolation. It is in some sense safer to extrapolate by starting solution at min(coh) = min(w). Now, for the lowest levels of coh(k, w-k, z), which are < min(w), we now require extrapolation. But this extrapolation is straight forward, when coh < min(w), households have to default, the utility from default is the same regardless of the level of coh at the time of default. So nearest extrapolation is fully correct.
Note that the first stage w grid is based on cash-on-hand level reached by the coh(k,w-k,z) possible choice and shock combinations. This coh(k,w-k,z) > max(w), which also means that at max(coh) grid, the w_perc choices at higher points require extrapolation. Extrapolation is based on nearest extrapolation.
Note that even when w = 0, as long as interest rate is low, only the lowest level of borrowing is invalid.
All discussion of of the word wage refers to productivity shock.
@param param_map container parameter container
@param support_map container support container
@param bl_input_override boolean if true varargin contained param_map and support_map fully overrides local default. Local default is not invoked. This could be important for speed if this function is getting invoked within certain loops. Default is 0.
@return armt_map container container with states, choices and shocks grids that are inputs for grid based solution algorithm. Contains these:
armt_map base arrays:
- ar_interp_c_grid: 1 by I^c
- ar_interp_coh_grid: 1 by I^{coh}
- ar_w_level: 1 by I^{W=k+b}
- ar_w_perc: 1 by P^{W=k+b}
- ar_ak_perc: 1 by P^{k and b}
armt_map 1st stage level coh on hand related arrays:
- mt_interp_coh_grid_mesh_z_wage: I^{coh} by M^w
- mt_z_wage_mesh_interp_coh_grid: I^{coh} by M^w
- mt_interp_coh_grid_mesh_w_perc: I^{coh} by P^{LAM=k+b}
- mt_w_perc_mesh_interp_coh_grid: I^{coh} by P^{LAM=k+b}
armt_map 2nd stage reachable coh(k(w), a(w,k), z', r)
- mt_coh_wkb: (I^k x I^w x M^r) by (M^z)
- mt_z_wage_mesh_coh_wkb: (I^k x I^w x M^r) by (M^z)
armt_map 2nd stage additional arrays
- mt_k: (I^w) by (P^{k and b})
- ar_a_meshk: 1 by (I^w x P^{k and b})
- ar_k_mesha: 1 by (I^w x P^{k and b})
- ar_aplusk_mesh: 1 by (I^w x P^{k and b})
- it_ameshk_n: scalar
armt_map Shock Grids Arrays and Mesh
- ar_z_r_borr: 1 by (M^r)
- ar_z_r_borr_prob: 1 by (M^r)
- ar_z_wage: 1 by (M^z)
- ar_z_wage_prob: 1 by (M^z)
- ar_z_r_borr_mesh_wage_w1r2: 1 by (M^z x M^r)
- ar_z_wage_mesh_r_borr_w1r2: 1 by (M^z x M^r)
- ar_z_r_borr_mesh_wage_r1w2: 1 by (M^r x M^z)
- ar_z_wage_mesh_r_borr_r1w2: 1 by (M^r x M^z)
@return func_map container container with function handles for consumption cash-on-hand etc.
@example
it_param_set = 2; bl_input_override = true; [param_map, support_map] = ffs_ipwkbzr_set_default_param(it_param_set); [armt_map, func_map] = ffs_ipwkbzr_get_funcgrid(param_map, support_map, bl_input_override);
@include
Default
if (~isempty(varargin)) % override when called from outside [param_map, support_map] = varargin{:}; else close all; % default internal run [param_map, support_map] = ffs_ipwkbzr_set_default_param(); support_map('bl_graph_funcgrids') = true; support_map('bl_graph_funcgrids_detail') = true; bl_display_funcgrids = true; support_map('bl_display_funcgrids') = bl_display_funcgrids; st_param_which = 'medium'; if (ismember(st_param_which, ['default'])) param_map('it_ak_perc_n') = 250; elseif (ismember(st_param_which, ['medium'])) % to be able to visually see choice grid points param_map('fl_b_bd') = -20; % borrow bound, = 0 if save only param_map('fl_default_aprime') = 0; param_map('bl_default') = false; % if borrowing is default allowed param_map('fl_w_min') = param_map('fl_b_bd'); param_map('it_w_perc_n') = 25; param_map('it_ak_perc_n') = 45; param_map('fl_w_interp_grid_gap') = 2; param_map('fl_coh_interp_grid_gap') = 2; % param_map('fl_z_r_borr_min') = 0.025; % param_map('fl_z_r_borr_max') = 0.95; % param_map('fl_z_r_borr_n') = 3; param_map('fl_z_r_borr_min') = 0.025; param_map('fl_z_r_borr_max') = 0.095; param_map('fl_z_r_borr_n') = 3; elseif (strcmp(st_param_which, 'small')) param_map('fl_z_r_borr_n') = 2; param_map('it_z_wage_n') = 3; param_map('fl_b_bd') = -20; % borrow bound, = 0 if save only param_map('fl_default_aprime') = 0; param_map('bl_default') = 0; % if borrowing is default allowed param_map('fl_w_min') = param_map('fl_b_bd'); param_map('it_w_perc_n') = 5; param_map('it_ak_perc_n') = 6; param_map('fl_w_interp_grid_gap') = 3; param_map('fl_coh_interp_grid_gap') = 3; param_map('fl_z_r_borr_min') = 0.025; param_map('fl_z_r_borr_max') = 0.95; param_map('fl_z_r_borr_n') = 3; end param_map('it_z_n') = param_map('it_z_wage_n') * param_map('fl_z_r_borr_n'); default_maps = {param_map, support_map}; % numvarargs is the number of varagin inputted [default_maps{1:length(varargin)}] = varargin{:}; param_map = [param_map; default_maps{1}]; support_map = [support_map; default_maps{2}]; % Display Parameters if (bl_display_funcgrids) fft_container_map_display(param_map); fft_container_map_display(support_map); end end
---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Display Parameters Specific to IPWKBZR xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ---------------------------------------- ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Begin: Show all key and value pairs from container CONTAINER NAME: PARAM_MAP ---------------------------------------- Map with properties: Count: 20 KeyType: char ValueType: any xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ---------------------------------------- ---------------------------------------- pos = 1 ; key = bl_default ; val = true pos = 2 ; key = fl_b_bd ; val = -20 pos = 3 ; key = fl_c_min ; val = 0.02 pos = 4 ; key = fl_default_wprime ; val = 0 pos = 5 ; key = fl_k_max ; val = 70 pos = 6 ; key = fl_w_max ; val = 50 pos = 7 ; key = fl_w_min ; val = -20 pos = 8 ; key = fl_z_r_borr_max ; val = 0.095 pos = 9 ; key = fl_z_r_borr_min ; val = 0.025 pos = 10 ; key = fl_z_r_borr_n ; val = 5 pos = 11 ; key = fl_z_r_borr_poiss_mean ; val = 20 pos = 12 ; key = fl_z_wage_mu ; val = 0 pos = 13 ; key = fl_z_wage_rho ; val = 0.8 pos = 14 ; key = fl_z_wage_sig ; val = 0.2 pos = 15 ; key = it_z_n ; val = 55 pos = 16 ; key = it_z_wage_n ; val = 11 pos = 17 ; key = st_model ; val = ipwkbzr pos = 18 ; key = st_v_coh_z_interp_method ; val = method_cell pos = 19 ; key = st_z_r_borr_drv_ele_type ; val = unif pos = 20 ; key = st_z_r_borr_drv_prb_type ; val = poiss ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Scalars in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx value __ ___ _____ bl_default 1 1 1 fl_b_bd 2 2 -20 fl_c_min 3 3 0.02 fl_default_wprime 4 4 0 fl_k_max 5 5 70 fl_w_max 6 6 50 fl_w_min 7 7 -20 fl_z_r_borr_max 8 8 0.095 fl_z_r_borr_min 9 9 0.025 fl_z_r_borr_n 10 10 5 fl_z_r_borr_poiss_mean 11 11 20 fl_z_wage_mu 12 12 0 fl_z_wage_rho 13 13 0.8 fl_z_wage_sig 14 14 0.2 it_z_n 15 15 55 it_z_wage_n 16 16 11 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Strings in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx _ ___ st_model 1 17 st_v_coh_z_interp_method 2 18 st_z_r_borr_drv_ele_type 3 19 st_z_r_borr_drv_prb_type 4 20 ---------------------------------------- ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Begin: Show all key and value pairs from container CONTAINER NAME: SUPPORT_MAP ---------------------------------------- Map with properties: Count: 5 KeyType: char ValueType: any xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ---------------------------------------- ---------------------------------------- pos = 1 ; key = st_img_path ; val = C:/Users/fan/CodeDynaAsset//m_ipwkbzr//solve/img/ pos = 2 ; key = st_mat_path ; val = C:/Users/fan/CodeDynaAsset//m_ipwkbzr//solve/mat/ pos = 3 ; key = st_mat_test_path ; val = C:/Users/fan/CodeDynaAsset//m_ipwkbzr//test/ff_ipwkbzr_ds_vecsv/mat/ pos = 4 ; key = st_matimg_path_root ; val = C:/Users/fan/CodeDynaAsset//m_ipwkbzr/ pos = 5 ; key = st_profile_path ; val = C:/Users/fan/CodeDynaAsset//m_ipwkbzr//solve/profile/ ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Strings in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx _ ___ st_img_path 1 1 st_mat_path 2 2 st_mat_test_path 3 3 st_matimg_path_root 4 4 st_profile_path 5 5 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Display All Parameters with IPWKBZR overriding IPWKBZR xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ---------------------------------------- ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Begin: Show all key and value pairs from container CONTAINER NAME: PARAM_MAP ---------------------------------------- Map with properties: Count: 41 KeyType: char ValueType: any xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ---------------------------------------- ---------------------------------------- pos = 1 ; key = bl_default ; val = true pos = 2 ; key = fl_Amean ; val = 1 pos = 3 ; key = fl_alpha ; val = 0.36 pos = 4 ; key = fl_b_bd ; val = -20 pos = 5 ; key = fl_beta ; val = 0.94 pos = 6 ; key = fl_c_min ; val = 0.02 pos = 7 ; key = fl_coh_interp_grid_gap ; val = 0.1 pos = 8 ; key = fl_crra ; val = 1.5 pos = 9 ; key = fl_default_wprime ; val = 0 pos = 10 ; key = fl_delta ; val = 0.08 pos = 11 ; key = fl_k_max ; val = 70 pos = 12 ; key = fl_k_min ; val = 0 pos = 13 ; key = fl_nan_replace ; val = -9999 pos = 14 ; key = fl_r_save ; val = 0.025 pos = 15 ; key = fl_tol_dist ; val = 1e-05 pos = 16 ; key = fl_tol_pol ; val = 1e-05 pos = 17 ; key = fl_tol_val ; val = 1e-05 pos = 18 ; key = fl_w ; val = 0.44365 pos = 19 ; key = fl_w_interp_grid_gap ; val = 0.1 pos = 20 ; key = fl_w_max ; val = 50 pos = 21 ; key = fl_w_min ; val = -20 pos = 22 ; key = fl_z_r_borr_max ; val = 0.095 pos = 23 ; key = fl_z_r_borr_min ; val = 0.025 pos = 24 ; key = fl_z_r_borr_n ; val = 5 pos = 25 ; key = fl_z_r_borr_poiss_mean ; val = 20 pos = 26 ; key = fl_z_wage_mu ; val = 0 pos = 27 ; key = fl_z_wage_rho ; val = 0.8 pos = 28 ; key = fl_z_wage_sig ; val = 0.2 pos = 29 ; key = it_ak_perc_n ; val = 50 pos = 30 ; key = it_c_interp_grid_gap ; val = 0.0001 pos = 31 ; key = it_maxiter_dist ; val = 1000 pos = 32 ; key = it_maxiter_val ; val = 250 pos = 33 ; key = it_tol_pol_nochange ; val = 25 pos = 34 ; key = it_w_perc_n ; val = 50 pos = 35 ; key = it_z_n ; val = 55 pos = 36 ; key = it_z_wage_n ; val = 11 pos = 37 ; key = st_analytical_stationary_type ; val = eigenvector pos = 38 ; key = st_model ; val = ipwkbzr pos = 39 ; key = st_v_coh_z_interp_method ; val = method_cell pos = 40 ; key = st_z_r_borr_drv_ele_type ; val = unif pos = 41 ; key = st_z_r_borr_drv_prb_type ; val = poiss ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Scalars in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx value __ ___ _______ bl_default 1 1 1 fl_Amean 2 2 1 fl_alpha 3 3 0.36 fl_b_bd 4 4 -20 fl_beta 5 5 0.94 fl_c_min 6 6 0.02 fl_coh_interp_grid_gap 7 7 0.1 fl_crra 8 8 1.5 fl_default_wprime 9 9 0 fl_delta 10 10 0.08 fl_k_max 11 11 70 fl_k_min 12 12 0 fl_nan_replace 13 13 -9999 fl_r_save 14 14 0.025 fl_tol_dist 15 15 1e-05 fl_tol_pol 16 16 1e-05 fl_tol_val 17 17 1e-05 fl_w 18 18 0.44365 fl_w_interp_grid_gap 19 19 0.1 fl_w_max 20 20 50 fl_w_min 21 21 -20 fl_z_r_borr_max 22 22 0.095 fl_z_r_borr_min 23 23 0.025 fl_z_r_borr_n 24 24 5 fl_z_r_borr_poiss_mean 25 25 20 fl_z_wage_mu 26 26 0 fl_z_wage_rho 27 27 0.8 fl_z_wage_sig 28 28 0.2 it_ak_perc_n 29 29 50 it_c_interp_grid_gap 30 30 0.0001 it_maxiter_dist 31 31 1000 it_maxiter_val 32 32 250 it_tol_pol_nochange 33 33 25 it_w_perc_n 34 34 50 it_z_n 35 35 55 it_z_wage_n 36 36 11 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Strings in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx _ ___ st_analytical_stationary_type 1 37 st_model 2 38 st_v_coh_z_interp_method 3 39 st_z_r_borr_drv_ele_type 4 40 st_z_r_borr_drv_prb_type 5 41 ---------------------------------------- ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Begin: Show all key and value pairs from container CONTAINER NAME: SUPPORT_MAP ---------------------------------------- Map with properties: Count: 43 KeyType: char ValueType: any xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ---------------------------------------- ---------------------------------------- pos = 1 ; key = bl_display ; val = false pos = 2 ; key = bl_display_defparam ; val = true pos = 3 ; key = bl_display_dist ; val = false pos = 4 ; key = bl_display_evf ; val = false pos = 5 ; key = bl_display_final ; val = true pos = 6 ; key = bl_display_final_dist ; val = false pos = 7 ; key = bl_display_final_dist_detail ; val = false pos = 8 ; key = bl_display_funcgrids ; val = false pos = 9 ; key = bl_graph ; val = true pos = 10 ; key = bl_graph_coh_t_coh ; val = true pos = 11 ; key = bl_graph_evf ; val = false pos = 12 ; key = bl_graph_funcgrids ; val = false pos = 13 ; key = bl_graph_funcgrids_detail ; val = false pos = 14 ; key = bl_graph_onebyones ; val = true pos = 15 ; key = bl_graph_pol_lvl ; val = true pos = 16 ; key = bl_graph_pol_pct ; val = true pos = 17 ; key = bl_graph_val ; val = true pos = 18 ; key = bl_img_save ; val = false pos = 19 ; key = bl_mat ; val = false pos = 20 ; key = bl_post ; val = true pos = 21 ; key = bl_profile ; val = false pos = 22 ; key = bl_profile_dist ; val = false pos = 23 ; key = bl_time ; val = false pos = 24 ; key = it_display_every ; val = 5 pos = 25 ; key = it_display_final_colmax ; val = 12 pos = 26 ; key = it_display_final_rowmax ; val = 100 pos = 27 ; key = it_display_summmat_colmax ; val = 7 pos = 28 ; key = it_display_summmat_rowmax ; val = 7 pos = 29 ; key = st_img_name_main ; val = _default pos = 30 ; key = st_img_path ; val = C:/Users/fan/CodeDynaAsset//m_ipwkbzr//solve/img/ pos = 31 ; key = st_img_prefix ; val = pos = 32 ; key = st_img_suffix ; val = _p4.png pos = 33 ; key = st_mat_name_main ; val = _default pos = 34 ; key = st_mat_path ; val = C:/Users/fan/CodeDynaAsset//m_ipwkbzr//solve/mat/ pos = 35 ; key = st_mat_prefix ; val = pos = 36 ; key = st_mat_suffix ; val = _p4 pos = 37 ; key = st_mat_test_path ; val = C:/Users/fan/CodeDynaAsset//m_ipwkbzr//test/ff_ipwkbzr_ds_vecsv/mat/ pos = 38 ; key = st_matimg_path_root ; val = C:/Users/fan/CodeDynaAsset//m_ipwkbzr/ pos = 39 ; key = st_profile_name_main ; val = _default pos = 40 ; key = st_profile_path ; val = C:/Users/fan/CodeDynaAsset//m_ipwkbzr//solve/profile/ pos = 41 ; key = st_profile_prefix ; val = pos = 42 ; key = st_profile_suffix ; val = _p4 pos = 43 ; key = st_title_prefix ; val = ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Scalars in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx value __ ___ _____ bl_display 1 1 0 bl_display_defparam 2 2 1 bl_display_dist 3 3 0 bl_display_evf 4 4 0 bl_display_final 5 5 1 bl_display_final_dist 6 6 0 bl_display_final_dist_detail 7 7 0 bl_display_funcgrids 8 8 0 bl_graph 9 9 1 bl_graph_coh_t_coh 10 10 1 bl_graph_evf 11 11 0 bl_graph_funcgrids 12 12 0 bl_graph_funcgrids_detail 13 13 0 bl_graph_onebyones 14 14 1 bl_graph_pol_lvl 15 15 1 bl_graph_pol_pct 16 16 1 bl_graph_val 17 17 1 bl_img_save 18 18 0 bl_mat 19 19 0 bl_post 20 20 1 bl_profile 21 21 0 bl_profile_dist 22 22 0 bl_time 23 23 0 it_display_every 24 24 5 it_display_final_colmax 25 25 12 it_display_final_rowmax 26 26 100 it_display_summmat_colmax 27 27 7 it_display_summmat_rowmax 28 28 7 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Strings in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx __ ___ st_img_name_main 1 29 st_img_path 2 30 st_img_prefix 3 31 st_img_suffix 4 32 st_mat_name_main 5 33 st_mat_path 6 34 st_mat_prefix 7 35 st_mat_suffix 8 36 st_mat_test_path 9 37 st_matimg_path_root 10 38 st_profile_name_main 11 39 st_profile_path 12 40 st_profile_prefix 13 41 st_profile_suffix 14 42 st_title_prefix 15 43 ---------------------------------------- ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Begin: Show all key and value pairs from container CONTAINER NAME: PARAM_MAP ---------------------------------------- Map with properties: Count: 42 KeyType: char ValueType: any xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ---------------------------------------- ---------------------------------------- pos = 1 ; key = bl_default ; val = false pos = 2 ; key = fl_Amean ; val = 1 pos = 3 ; key = fl_alpha ; val = 0.36 pos = 4 ; key = fl_b_bd ; val = -20 pos = 5 ; key = fl_beta ; val = 0.94 pos = 6 ; key = fl_c_min ; val = 0.02 pos = 7 ; key = fl_coh_interp_grid_gap ; val = 2 pos = 8 ; key = fl_crra ; val = 1.5 pos = 9 ; key = fl_default_aprime ; val = 0 pos = 10 ; key = fl_default_wprime ; val = 0 pos = 11 ; key = fl_delta ; val = 0.08 pos = 12 ; key = fl_k_max ; val = 70 pos = 13 ; key = fl_k_min ; val = 0 pos = 14 ; key = fl_nan_replace ; val = -9999 pos = 15 ; key = fl_r_save ; val = 0.025 pos = 16 ; key = fl_tol_dist ; val = 1e-05 pos = 17 ; key = fl_tol_pol ; val = 1e-05 pos = 18 ; key = fl_tol_val ; val = 1e-05 pos = 19 ; key = fl_w ; val = 0.44365 pos = 20 ; key = fl_w_interp_grid_gap ; val = 2 pos = 21 ; key = fl_w_max ; val = 50 pos = 22 ; key = fl_w_min ; val = -20 pos = 23 ; key = fl_z_r_borr_max ; val = 0.095 pos = 24 ; key = fl_z_r_borr_min ; val = 0.025 pos = 25 ; key = fl_z_r_borr_n ; val = 3 pos = 26 ; key = fl_z_r_borr_poiss_mean ; val = 20 pos = 27 ; key = fl_z_wage_mu ; val = 0 pos = 28 ; key = fl_z_wage_rho ; val = 0.8 pos = 29 ; key = fl_z_wage_sig ; val = 0.2 pos = 30 ; key = it_ak_perc_n ; val = 45 pos = 31 ; key = it_c_interp_grid_gap ; val = 0.0001 pos = 32 ; key = it_maxiter_dist ; val = 1000 pos = 33 ; key = it_maxiter_val ; val = 250 pos = 34 ; key = it_tol_pol_nochange ; val = 25 pos = 35 ; key = it_w_perc_n ; val = 25 pos = 36 ; key = it_z_n ; val = 33 pos = 37 ; key = it_z_wage_n ; val = 11 pos = 38 ; key = st_analytical_stationary_type ; val = eigenvector pos = 39 ; key = st_model ; val = ipwkbzr pos = 40 ; key = st_v_coh_z_interp_method ; val = method_cell pos = 41 ; key = st_z_r_borr_drv_ele_type ; val = unif pos = 42 ; key = st_z_r_borr_drv_prb_type ; val = poiss ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Scalars in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx value __ ___ _______ bl_default 1 1 0 fl_Amean 2 2 1 fl_alpha 3 3 0.36 fl_b_bd 4 4 -20 fl_beta 5 5 0.94 fl_c_min 6 6 0.02 fl_coh_interp_grid_gap 7 7 2 fl_crra 8 8 1.5 fl_default_aprime 9 9 0 fl_default_wprime 10 10 0 fl_delta 11 11 0.08 fl_k_max 12 12 70 fl_k_min 13 13 0 fl_nan_replace 14 14 -9999 fl_r_save 15 15 0.025 fl_tol_dist 16 16 1e-05 fl_tol_pol 17 17 1e-05 fl_tol_val 18 18 1e-05 fl_w 19 19 0.44365 fl_w_interp_grid_gap 20 20 2 fl_w_max 21 21 50 fl_w_min 22 22 -20 fl_z_r_borr_max 23 23 0.095 fl_z_r_borr_min 24 24 0.025 fl_z_r_borr_n 25 25 3 fl_z_r_borr_poiss_mean 26 26 20 fl_z_wage_mu 27 27 0 fl_z_wage_rho 28 28 0.8 fl_z_wage_sig 29 29 0.2 it_ak_perc_n 30 30 45 it_c_interp_grid_gap 31 31 0.0001 it_maxiter_dist 32 32 1000 it_maxiter_val 33 33 250 it_tol_pol_nochange 34 34 25 it_w_perc_n 35 35 25 it_z_n 36 36 33 it_z_wage_n 37 37 11 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Strings in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx _ ___ st_analytical_stationary_type 1 38 st_model 2 39 st_v_coh_z_interp_method 3 40 st_z_r_borr_drv_ele_type 4 41 st_z_r_borr_drv_prb_type 5 42 ---------------------------------------- ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Begin: Show all key and value pairs from container CONTAINER NAME: SUPPORT_MAP ---------------------------------------- Map with properties: Count: 43 KeyType: char ValueType: any xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ---------------------------------------- ---------------------------------------- pos = 1 ; key = bl_display ; val = false pos = 2 ; key = bl_display_defparam ; val = true pos = 3 ; key = bl_display_dist ; val = false pos = 4 ; key = bl_display_evf ; val = false pos = 5 ; key = bl_display_final ; val = true pos = 6 ; key = bl_display_final_dist ; val = false pos = 7 ; key = bl_display_final_dist_detail ; val = false pos = 8 ; key = bl_display_funcgrids ; val = true pos = 9 ; key = bl_graph ; val = true pos = 10 ; key = bl_graph_coh_t_coh ; val = true pos = 11 ; key = bl_graph_evf ; val = false pos = 12 ; key = bl_graph_funcgrids ; val = true pos = 13 ; key = bl_graph_funcgrids_detail ; val = true pos = 14 ; key = bl_graph_onebyones ; val = true pos = 15 ; key = bl_graph_pol_lvl ; val = true pos = 16 ; key = bl_graph_pol_pct ; val = true pos = 17 ; key = bl_graph_val ; val = true pos = 18 ; key = bl_img_save ; val = false pos = 19 ; key = bl_mat ; val = false pos = 20 ; key = bl_post ; val = true pos = 21 ; key = bl_profile ; val = false pos = 22 ; key = bl_profile_dist ; val = false pos = 23 ; key = bl_time ; val = false pos = 24 ; key = it_display_every ; val = 5 pos = 25 ; key = it_display_final_colmax ; val = 12 pos = 26 ; key = it_display_final_rowmax ; val = 100 pos = 27 ; key = it_display_summmat_colmax ; val = 7 pos = 28 ; key = it_display_summmat_rowmax ; val = 7 pos = 29 ; key = st_img_name_main ; val = _default pos = 30 ; key = st_img_path ; val = C:/Users/fan/CodeDynaAsset//m_ipwkbzr//solve/img/ pos = 31 ; key = st_img_prefix ; val = pos = 32 ; key = st_img_suffix ; val = _p4.png pos = 33 ; key = st_mat_name_main ; val = _default pos = 34 ; key = st_mat_path ; val = C:/Users/fan/CodeDynaAsset//m_ipwkbzr//solve/mat/ pos = 35 ; key = st_mat_prefix ; val = pos = 36 ; key = st_mat_suffix ; val = _p4 pos = 37 ; key = st_mat_test_path ; val = C:/Users/fan/CodeDynaAsset//m_ipwkbzr//test/ff_ipwkbzr_ds_vecsv/mat/ pos = 38 ; key = st_matimg_path_root ; val = C:/Users/fan/CodeDynaAsset//m_ipwkbzr/ pos = 39 ; key = st_profile_name_main ; val = _default pos = 40 ; key = st_profile_path ; val = C:/Users/fan/CodeDynaAsset//m_ipwkbzr//solve/profile/ pos = 41 ; key = st_profile_prefix ; val = pos = 42 ; key = st_profile_suffix ; val = _p4 pos = 43 ; key = st_title_prefix ; val = ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Scalars in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx value __ ___ _____ bl_display 1 1 0 bl_display_defparam 2 2 1 bl_display_dist 3 3 0 bl_display_evf 4 4 0 bl_display_final 5 5 1 bl_display_final_dist 6 6 0 bl_display_final_dist_detail 7 7 0 bl_display_funcgrids 8 8 1 bl_graph 9 9 1 bl_graph_coh_t_coh 10 10 1 bl_graph_evf 11 11 0 bl_graph_funcgrids 12 12 1 bl_graph_funcgrids_detail 13 13 1 bl_graph_onebyones 14 14 1 bl_graph_pol_lvl 15 15 1 bl_graph_pol_pct 16 16 1 bl_graph_val 17 17 1 bl_img_save 18 18 0 bl_mat 19 19 0 bl_post 20 20 1 bl_profile 21 21 0 bl_profile_dist 22 22 0 bl_time 23 23 0 it_display_every 24 24 5 it_display_final_colmax 25 25 12 it_display_final_rowmax 26 26 100 it_display_summmat_colmax 27 27 7 it_display_summmat_rowmax 28 28 7 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Strings in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx __ ___ st_img_name_main 1 29 st_img_path 2 30 st_img_prefix 3 31 st_img_suffix 4 32 st_mat_name_main 5 33 st_mat_path 6 34 st_mat_prefix 7 35 st_mat_suffix 8 36 st_mat_test_path 9 37 st_matimg_path_root 10 38 st_profile_name_main 11 39 st_profile_path 12 40 st_profile_prefix 13 41 st_profile_suffix 14 42 st_title_prefix 15 43
Parse Parameters 1a
params_group = values(param_map, {'bl_default', 'fl_b_bd', 'fl_w_min', 'fl_w_max'}); [bl_default, fl_b_bd, fl_w_min, fl_w_max] = params_group{:}; params_group = values(param_map, {'fl_crra', 'fl_c_min'}); [fl_crra, fl_c_min] = params_group{:}; params_group = values(param_map, {'fl_Amean', 'fl_alpha', 'fl_delta'}); [fl_Amean, fl_alpha, fl_delta] = params_group{:}; params_group = values(param_map, {'fl_r_save', 'fl_w'}); [fl_r_save, fl_w] = params_group{:};
Parse Parameters 1b
params_group = values(param_map, {... 'it_w_perc_n', 'it_ak_perc_n',... 'it_c_interp_grid_gap', 'fl_w_interp_grid_gap', 'fl_coh_interp_grid_gap'}); [it_w_perc_n, it_ak_perc_n,... it_c_interp_grid_gap, fl_w_interp_grid_gap, fl_coh_interp_grid_gap] = params_group{:}; params_group = values(param_map, {'st_v_coh_z_interp_method'}); [st_v_coh_z_interp_method] = params_group{:};
Parse Parameters 2
% param_map shock income params_group = values(param_map, {'it_z_wage_n', 'fl_z_wage_mu', 'fl_z_wage_rho', 'fl_z_wage_sig'}); [it_z_wage_n, fl_z_wage_mu, fl_z_wage_rho, fl_z_wage_sig] = params_group{:}; % param_map shock borrowing interest params_group = values(param_map, {'st_z_r_borr_drv_ele_type', 'st_z_r_borr_drv_prb_type', 'fl_z_r_borr_poiss_mean', ... 'fl_z_r_borr_max', 'fl_z_r_borr_min', 'fl_z_r_borr_n'}); [st_z_r_borr_drv_ele_type, st_z_r_borr_drv_prb_type, fl_z_r_borr_poiss_mean, ... fl_z_r_borr_max, fl_z_r_borr_min, fl_z_r_borr_n] = params_group{:}; % param_map shock income params_group = values(param_map, {'it_z_n'}); [it_z_n] = params_group{:};
Parse Parameters 3
params_group = values(support_map, {'bl_graph_funcgrids', 'bl_graph_funcgrids_detail', 'bl_display_funcgrids'}); [bl_graph_funcgrids, bl_graph_funcgrids_detail, bl_display_funcgrids] = params_group{:}; params_group = values(support_map, {'it_display_summmat_rowmax', 'it_display_summmat_colmax'}); [it_display_summmat_rowmax, it_display_summmat_colmax] = params_group{:};
F1: Get Shock: Income Shock (ar1)
[~, mt_z_wage_trans, ar_z_wage_prob, ar_z_wage] = ffto_gen_tauchen_jhl(fl_z_wage_mu,fl_z_wage_rho,fl_z_wage_sig,it_z_wage_n);
F2: Get Shock: Interest Rate Shock (iid)
% get borrowing grid and probabilities param_dsv_map = containers.Map('KeyType','char', 'ValueType','any'); param_dsv_map('st_drv_ele_type') = st_z_r_borr_drv_ele_type; param_dsv_map('st_drv_prb_type') = st_z_r_borr_drv_prb_type; param_dsv_map('fl_poiss_mean') = fl_z_r_borr_poiss_mean; param_dsv_map('fl_max') = fl_z_r_borr_max; param_dsv_map('fl_min') = fl_z_r_borr_min; param_dsv_map('fl_n') = fl_z_r_borr_n; [ar_z_r_borr, ar_z_r_borr_prob] = fft_gen_discrete_var(param_dsv_map, true);
G: Generate Asset and Choice Grid for 2nd stage Problem
This generate triangular choice structure. Household choose total aggregate savings, and within that how much to put into risky capital and how much to put into safe assets, in percentages. See ffs_ipwkbzr_set_default_param for details.
if (~bl_default) fl_r_borr_max = max(ar_z_r_borr); fl_w_min_use = max(fl_w_min, -(fl_w)/fl_r_borr_max); fl_b_bd = fl_w_min_use; else fl_w_min_use = fl_w_min; end % percentage grid for 1st stage choice problem, level grid for 2nd stage % solving optimal k given w and z. ar_w_perc = linspace(0, 1, it_w_perc_n); it_w_interp_n = ((fl_w_max-fl_w_min_use)/(fl_w_interp_grid_gap)); ar_w_level_full = fft_array_add_zero(linspace(fl_w_min_use, fl_w_max, it_w_interp_n), true); ar_w_level = ar_w_level_full; it_w_interp_n = length(ar_w_level_full); % max k given w, need to consider the possibility of borrowing. ar_k_max = ar_w_level_full - fl_b_bd; % k percentage choice grid ar_ak_perc = linspace(0, 1, it_ak_perc_n); % 2nd stage percentage choice matrixes % (ar_k_max') is it_w_interp_n by 1, and (ar_ak_perc) is 1 by it_ak_perc_n % mt_k is a it_w_interp_n by it_ak_perc_n matrix of choice points of k' % conditional on w, each column is a different w, each row for each col a % different k' value. mt_k = (ar_k_max'*ar_ak_perc)'; mt_a = (ar_w_level_full - mt_k); % can not have choice that are beyond feasible bound given the percentage % structure here. mt_bl_constrained = (mt_a < fl_b_bd); if (sum(mt_bl_constrained) > 0 ) error('at %s second stage choice points, percentage choice exceed bounds, can not happen',... num2str(sum(mt_bl_constrained))); end ar_a_meshk_full = mt_a(:); ar_k_mesha_full = mt_k(:); ar_a_meshk = ar_a_meshk_full; ar_k_mesha = ar_k_mesha_full; % iid transition matrix mt_z_r_borr_prob_trans = repmat(ar_z_r_borr_prob, [length(ar_z_r_borr_prob), 1]);
F3: Get Shock: Mesh Shocks Together
R is outter, W is Inner
% Kronecker product to get full transition matrix for the two shocks mt_z_trans = kron(mt_z_r_borr_prob_trans, mt_z_wage_trans); % mesh the shock vectors [mt_z_wage_mesh_r_borr_w1r2, mt_z_r_borr_mesh_wage_w1r2] = ndgrid(ar_z_wage, ar_z_r_borr); ar_z_wage_mesh_r_borr_w1r2 = mt_z_wage_mesh_r_borr_w1r2(:)'; ar_z_r_borr_mesh_wage_w1r2 = mt_z_r_borr_mesh_wage_w1r2(:)'; % mesh the shock vectors [mt_z_r_borr_mesh_wage_r1w2, mt_z_wage_mesh_r_borr_r1w2] = ndgrid(ar_z_r_borr, ar_z_wage); ar_z_wage_mesh_r_borr_r1w2 = mt_z_wage_mesh_r_borr_r1w2(:)'; ar_z_r_borr_mesh_wage_r1w2 = mt_z_r_borr_mesh_wage_r1w2(:)'; if (bl_display_funcgrids) disp('----------------------------------------'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp('Borrow R Shock: ar_z_r_borr_mesh_wage_w1r2'); disp('Prod/Wage Shock: ar_z_wage_mesh_r_borr_w1r2'); disp('show which shock is inner and which is outter'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); tb_two_shocks = array2table([ar_z_r_borr_mesh_wage_w1r2;... ar_z_wage_mesh_r_borr_w1r2]'); cl_col_names = ["Borrow R Shock (Meshed)", "Wage R Shock (Meshed)"]; cl_row_names = strcat('zi=', string((1:it_z_n))); tb_two_shocks.Properties.VariableNames = matlab.lang.makeValidName(cl_col_names); tb_two_shocks.Properties.RowNames = matlab.lang.makeValidName(cl_row_names); it_row_display = it_z_wage_n*2; disp(size(tb_two_shocks)); disp(head(tb_two_shocks, it_row_display)); disp(tail(tb_two_shocks, it_row_display)); disp('----------------------------------------'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp('Borrow R Shock: ar_z_wage_mesh_r_borr_r1w2'); disp('Prod/Wage Shock: ar_z_r_borr_mesh_wage_r1w2'); disp('show which shock is inner and which is outter'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); tb_two_shocks = array2table([ar_z_wage_mesh_r_borr_r1w2;... ar_z_r_borr_mesh_wage_r1w2]'); cl_col_names = ["Borrow R Shock (Meshed)", "Wage R Shock (Meshed)"]; cl_row_names = strcat('zi=', string((1:length(ar_z_r_borr_mesh_wage_r1w2)))); tb_two_shocks.Properties.VariableNames = matlab.lang.makeValidName(cl_col_names); tb_two_shocks.Properties.RowNames = matlab.lang.makeValidName(cl_row_names); it_row_display = fl_z_r_borr_n*2; disp(size(tb_two_shocks)); disp(head(tb_two_shocks, it_row_display)); disp(tail(tb_two_shocks, it_row_display)); disp('----------------------------------------'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp('Borrow Rate Transition Matrix: mt_z_r_borr_prob_trans'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); it_col_n_keep = 15; it_row_n_keep = 15; [it_row_n, it_col_n] = size(mt_z_r_borr_prob_trans); [ar_it_cols, ar_it_rows] = fft_row_col_subset(it_col_n, it_col_n_keep, it_row_n, it_row_n_keep); cl_st_full_rowscols = cellstr([num2str(ar_z_r_borr', 'r%3.2f')]); tb_z_r_borr_prob_trans = array2table(round(mt_z_r_borr_prob_trans(ar_it_rows, ar_it_cols), 6)); cl_col_names = strcat('zi=', num2str(ar_it_cols'), ':', cl_st_full_rowscols(ar_it_cols)); cl_row_names = strcat('zi=', num2str(ar_it_rows'), ':', cl_st_full_rowscols(ar_it_rows)); tb_z_r_borr_prob_trans.Properties.VariableNames = matlab.lang.makeValidName(cl_col_names); tb_z_r_borr_prob_trans.Properties.RowNames = matlab.lang.makeValidName(cl_row_names); disp(size(tb_z_r_borr_prob_trans)); disp(tb_z_r_borr_prob_trans); disp('----------------------------------------'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp('Wage Prod Shock Transition Matrix: mt_z_r_borr_prob_trans'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); it_col_n_keep = 15; it_row_n_keep = 15; [it_row_n, it_col_n] = size(mt_z_wage_trans); [ar_it_cols, ar_it_rows] = fft_row_col_subset(it_col_n, it_col_n_keep, it_row_n, it_row_n_keep); cl_st_full_rowscols = cellstr([num2str(ar_z_wage', 'w%3.2f')]); tb_z_wage_trans = array2table(round(mt_z_wage_trans(ar_it_rows, ar_it_cols),6)); cl_col_names = strcat('zi=', num2str(ar_it_cols'), ':', cl_st_full_rowscols(ar_it_cols)); cl_row_names = strcat('zi=', num2str(ar_it_rows'), ':', cl_st_full_rowscols(ar_it_rows)); tb_z_wage_trans.Properties.VariableNames = matlab.lang.makeValidName(cl_col_names); tb_z_wage_trans.Properties.RowNames = matlab.lang.makeValidName(cl_row_names); disp(size(tb_z_wage_trans)); disp(tb_z_wage_trans); disp('----------------------------------------'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp('Full Transition Matrix: mt_z_trans'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); it_col_n_keep = it_z_wage_n*3; it_row_n_keep = it_z_wage_n*3; [it_row_n, it_col_n] = size(mt_z_trans); [ar_it_cols, ar_it_rows] = fft_row_col_subset(it_col_n, it_col_n_keep, it_row_n, it_row_n_keep); cl_st_full_rowscols = cellstr([num2str(ar_z_r_borr_mesh_wage_w1r2', 'r%3.2f;'), ... num2str(ar_z_wage_mesh_r_borr_w1r2', 'w%3.2f')]); tb_mt_z_trans = array2table(round(mt_z_trans(ar_it_rows, ar_it_cols),6)); cl_col_names = strcat('i', num2str(ar_it_cols'), ':', cl_st_full_rowscols(ar_it_cols)); cl_row_names = strcat('i', num2str(ar_it_rows'), ':', cl_st_full_rowscols(ar_it_rows)); tb_mt_z_trans.Properties.VariableNames = matlab.lang.makeValidName(cl_col_names); tb_mt_z_trans.Properties.RowNames = matlab.lang.makeValidName(cl_row_names); disp(size(tb_mt_z_trans)); disp(tb_mt_z_trans); end
---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Borrow R Shock: ar_z_r_borr_mesh_wage_w1r2 Prod/Wage Shock: ar_z_wage_mesh_r_borr_w1r2 show which shock is inner and which is outter xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx 33 2 BorrowRShock_Meshed_ WageRShock_Meshed_ ____________________ __________________ zi_1 0.025 0.34664 zi_2 0.025 0.42338 zi_3 0.025 0.51712 zi_4 0.025 0.63162 zi_5 0.025 0.77146 zi_6 0.025 0.94226 zi_7 0.025 1.1509 zi_8 0.025 1.4057 zi_9 0.025 1.7169 zi_10 0.025 2.097 zi_11 0.025 2.5613 zi_12 0.06 0.34664 zi_13 0.06 0.42338 zi_14 0.06 0.51712 zi_15 0.06 0.63162 zi_16 0.06 0.77146 zi_17 0.06 0.94226 zi_18 0.06 1.1509 zi_19 0.06 1.4057 zi_20 0.06 1.7169 zi_21 0.06 2.097 zi_22 0.06 2.5613 BorrowRShock_Meshed_ WageRShock_Meshed_ ____________________ __________________ zi_12 0.06 0.34664 zi_13 0.06 0.42338 zi_14 0.06 0.51712 zi_15 0.06 0.63162 zi_16 0.06 0.77146 zi_17 0.06 0.94226 zi_18 0.06 1.1509 zi_19 0.06 1.4057 zi_20 0.06 1.7169 zi_21 0.06 2.097 zi_22 0.06 2.5613 zi_23 0.095 0.34664 zi_24 0.095 0.42338 zi_25 0.095 0.51712 zi_26 0.095 0.63162 zi_27 0.095 0.77146 zi_28 0.095 0.94226 zi_29 0.095 1.1509 zi_30 0.095 1.4057 zi_31 0.095 1.7169 zi_32 0.095 2.097 zi_33 0.095 2.5613 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Borrow R Shock: ar_z_wage_mesh_r_borr_r1w2 Prod/Wage Shock: ar_z_r_borr_mesh_wage_r1w2 show which shock is inner and which is outter xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx 33 2 BorrowRShock_Meshed_ WageRShock_Meshed_ ____________________ __________________ zi_1 0.34664 0.025 zi_2 0.34664 0.06 zi_3 0.34664 0.095 zi_4 0.42338 0.025 zi_5 0.42338 0.06 zi_6 0.42338 0.095 BorrowRShock_Meshed_ WageRShock_Meshed_ ____________________ __________________ zi_28 2.097 0.025 zi_29 2.097 0.06 zi_30 2.097 0.095 zi_31 2.5613 0.025 zi_32 2.5613 0.06 zi_33 2.5613 0.095 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Borrow Rate Transition Matrix: mt_z_r_borr_prob_trans xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx 3 3 zi_1_r0_03 zi_2_r0_06 zi_3_r0_10 __________ __________ __________ zi_1_r0_03 0.004525 0.090498 0.90498 zi_2_r0_06 0.004525 0.090498 0.90498 zi_3_r0_10 0.004525 0.090498 0.90498 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Wage Prod Shock Transition Matrix: mt_z_r_borr_prob_trans xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx 11 11 zi_1_w0_35 zi_2_w0_42 zi_3_w0_52 zi_4_w0_63 zi_5_w0_77 zi_6_w0_94 zi_7_w1_15 zi_8_w1_41 zi_9_w1_72 zi_10_w2_10 zi_11_w2_56 __________ __________ __________ __________ __________ __________ __________ __________ __________ ___________ ___________ zi_1_w0_35 0.30854 0.38293 0.24173 0.060598 0.005977 0.000229 3e-06 0 0 0 0 zi_2_w0_42 0.0968 0.28529 0.37595 0.1974 0.041098 0.003359 0.000106 1e-06 0 0 0 zi_3_w0_52 0.017864 0.1178 0.32451 0.35577 0.15534 0.026851 0.001818 4.8e-05 0 0 0 zi_4_w0_63 0.001866 0.026851 0.15534 0.35577 0.32451 0.1178 0.016897 0.000947 2e-05 0 0 zi_5_w0_77 0.000108 0.003359 0.041098 0.1974 0.37595 0.28529 0.086076 0.010241 0.000475 8e-06 0 zi_6_w0_94 3e-06 0.000229 0.005977 0.060598 0.24173 0.38293 0.24173 0.060598 0.005977 0.000229 3e-06 zi_7_w1_15 0 8e-06 0.000475 0.010241 0.086076 0.28529 0.37595 0.1974 0.041098 0.003359 0.000108 zi_8_w1_41 0 0 2e-05 0.000947 0.016897 0.1178 0.32451 0.35577 0.15534 0.026851 0.001866 zi_9_w1_72 0 0 0 4.8e-05 0.001818 0.026851 0.15534 0.35577 0.32451 0.1178 0.017864 zi_10_w2_10 0 0 0 1e-06 0.000106 0.003359 0.041098 0.1974 0.37595 0.28529 0.0968 zi_11_w2_56 0 0 0 0 3e-06 0.000229 0.005977 0.060598 0.24173 0.38293 0.30854 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Full Transition Matrix: mt_z_trans xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx 33 33 i1_r0_03_w0_35 i2_r0_03_w0_42 i3_r0_03_w0_52 i4_r0_03_w0_63 i5_r0_03_w0_77 i6_r0_03_w0_94 i7_r0_03_w1_15 i8_r0_03_w1_41 i9_r0_03_w1_72 i10_r0_03_w2_10 i11_r0_03_w2_56 i12_r0_06_w0_35 i13_r0_06_w0_42 i14_r0_06_w0_52 i15_r0_06_w0_63 i16_r0_06_w0_77 i17_r0_06_w0_94 i18_r0_06_w1_15 i19_r0_06_w1_41 i20_r0_06_w1_72 i21_r0_06_w2_10 i22_r0_06_w2_56 i23_r0_10_w0_35 i24_r0_10_w0_42 i25_r0_10_w0_52 i26_r0_10_w0_63 i27_r0_10_w0_77 i28_r0_10_w0_94 i29_r0_10_w1_15 i30_r0_10_w1_41 i31_r0_10_w1_72 i32_r0_10_w2_10 i33_r0_10_w2_56 ______________ ______________ ______________ ______________ ______________ ______________ ______________ ______________ ______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ i1_r0_03_w0_35 0.001396 0.001733 0.001094 0.000274 2.7e-05 1e-06 0 0 0 0 0 0.027922 0.034654 0.021876 0.005484 0.000541 2.1e-05 0 0 0 0 0 0.27922 0.34654 0.21876 0.054839 0.005409 0.000207 3e-06 0 0 0 0 i2_r0_03_w0_42 0.000438 0.001291 0.001701 0.000893 0.000186 1.5e-05 0 0 0 0 0 0.00876 0.025818 0.034022 0.017864 0.003719 0.000304 1e-05 0 0 0 0 0.087602 0.25818 0.34022 0.17864 0.037193 0.00304 9.6e-05 1e-06 0 0 0 i3_r0_03_w0_52 8.1e-05 0.000533 0.001468 0.00161 0.000703 0.000121 8e-06 0 0 0 0 0.001617 0.010661 0.029367 0.032196 0.014058 0.00243 0.000164 4e-06 0 0 0 0.016167 0.10661 0.29367 0.32196 0.14058 0.024299 0.001645 4.3e-05 0 0 0 i4_r0_03_w0_63 8e-06 0.000121 0.000703 0.00161 0.001468 0.000533 7.6e-05 4e-06 0 0 0 0.000169 0.00243 0.014058 0.032196 0.029367 0.010661 0.001529 8.6e-05 2e-06 0 0 0.001689 0.024299 0.14058 0.32196 0.29367 0.10661 0.015291 0.000857 1.9e-05 0 0 i5_r0_03_w0_77 0 1.5e-05 0.000186 0.000893 0.001701 0.001291 0.000389 4.6e-05 2e-06 0 0 1e-05 0.000304 0.003719 0.017864 0.034022 0.025818 0.00779 0.000927 4.3e-05 1e-06 0 9.8e-05 0.00304 0.037193 0.17864 0.34022 0.25818 0.077897 0.009268 0.00043 8e-06 0 i6_r0_03_w0_94 0 1e-06 2.7e-05 0.000274 0.001094 0.001733 0.001094 0.000274 2.7e-05 1e-06 0 0 2.1e-05 0.000541 0.005484 0.021876 0.034654 0.021876 0.005484 0.000541 2.1e-05 0 3e-06 0.000207 0.005409 0.054839 0.21876 0.34654 0.21876 0.054839 0.005409 0.000207 3e-06 i7_r0_03_w1_15 0 0 2e-06 4.6e-05 0.000389 0.001291 0.001701 0.000893 0.000186 1.5e-05 0 0 1e-06 4.3e-05 0.000927 0.00779 0.025818 0.034022 0.017864 0.003719 0.000304 1e-05 0 8e-06 0.00043 0.009268 0.077897 0.25818 0.34022 0.17864 0.037193 0.00304 9.8e-05 i8_r0_03_w1_41 0 0 0 4e-06 7.6e-05 0.000533 0.001468 0.00161 0.000703 0.000121 8e-06 0 0 2e-06 8.6e-05 0.001529 0.010661 0.029367 0.032196 0.014058 0.00243 0.000169 0 0 1.9e-05 0.000857 0.015291 0.10661 0.29367 0.32196 0.14058 0.024299 0.001689 i9_r0_03_w1_72 0 0 0 0 8e-06 0.000121 0.000703 0.00161 0.001468 0.000533 8.1e-05 0 0 0 4e-06 0.000164 0.00243 0.014058 0.032196 0.029367 0.010661 0.001617 0 0 0 4.3e-05 0.001645 0.024299 0.14058 0.32196 0.29367 0.10661 0.016167 i10_r0_03_w2_10 0 0 0 0 0 1.5e-05 0.000186 0.000893 0.001701 0.001291 0.000438 0 0 0 0 1e-05 0.000304 0.003719 0.017864 0.034022 0.025818 0.00876 0 0 0 1e-06 9.6e-05 0.00304 0.037193 0.17864 0.34022 0.25818 0.087602 i11_r0_03_w2_56 0 0 0 0 0 1e-06 2.7e-05 0.000274 0.001094 0.001733 0.001396 0 0 0 0 0 2.1e-05 0.000541 0.005484 0.021876 0.034654 0.027922 0 0 0 0 3e-06 0.000207 0.005409 0.054839 0.21876 0.34654 0.27922 i12_r0_06_w0_35 0.001396 0.001733 0.001094 0.000274 2.7e-05 1e-06 0 0 0 0 0 0.027922 0.034654 0.021876 0.005484 0.000541 2.1e-05 0 0 0 0 0 0.27922 0.34654 0.21876 0.054839 0.005409 0.000207 3e-06 0 0 0 0 i13_r0_06_w0_42 0.000438 0.001291 0.001701 0.000893 0.000186 1.5e-05 0 0 0 0 0 0.00876 0.025818 0.034022 0.017864 0.003719 0.000304 1e-05 0 0 0 0 0.087602 0.25818 0.34022 0.17864 0.037193 0.00304 9.6e-05 1e-06 0 0 0 i14_r0_06_w0_52 8.1e-05 0.000533 0.001468 0.00161 0.000703 0.000121 8e-06 0 0 0 0 0.001617 0.010661 0.029367 0.032196 0.014058 0.00243 0.000164 4e-06 0 0 0 0.016167 0.10661 0.29367 0.32196 0.14058 0.024299 0.001645 4.3e-05 0 0 0 i15_r0_06_w0_63 8e-06 0.000121 0.000703 0.00161 0.001468 0.000533 7.6e-05 4e-06 0 0 0 0.000169 0.00243 0.014058 0.032196 0.029367 0.010661 0.001529 8.6e-05 2e-06 0 0 0.001689 0.024299 0.14058 0.32196 0.29367 0.10661 0.015291 0.000857 1.9e-05 0 0 i16_r0_06_w0_77 0 1.5e-05 0.000186 0.000893 0.001701 0.001291 0.000389 4.6e-05 2e-06 0 0 1e-05 0.000304 0.003719 0.017864 0.034022 0.025818 0.00779 0.000927 4.3e-05 1e-06 0 9.8e-05 0.00304 0.037193 0.17864 0.34022 0.25818 0.077897 0.009268 0.00043 8e-06 0 i17_r0_06_w0_94 0 1e-06 2.7e-05 0.000274 0.001094 0.001733 0.001094 0.000274 2.7e-05 1e-06 0 0 2.1e-05 0.000541 0.005484 0.021876 0.034654 0.021876 0.005484 0.000541 2.1e-05 0 3e-06 0.000207 0.005409 0.054839 0.21876 0.34654 0.21876 0.054839 0.005409 0.000207 3e-06 i18_r0_06_w1_15 0 0 2e-06 4.6e-05 0.000389 0.001291 0.001701 0.000893 0.000186 1.5e-05 0 0 1e-06 4.3e-05 0.000927 0.00779 0.025818 0.034022 0.017864 0.003719 0.000304 1e-05 0 8e-06 0.00043 0.009268 0.077897 0.25818 0.34022 0.17864 0.037193 0.00304 9.8e-05 i19_r0_06_w1_41 0 0 0 4e-06 7.6e-05 0.000533 0.001468 0.00161 0.000703 0.000121 8e-06 0 0 2e-06 8.6e-05 0.001529 0.010661 0.029367 0.032196 0.014058 0.00243 0.000169 0 0 1.9e-05 0.000857 0.015291 0.10661 0.29367 0.32196 0.14058 0.024299 0.001689 i20_r0_06_w1_72 0 0 0 0 8e-06 0.000121 0.000703 0.00161 0.001468 0.000533 8.1e-05 0 0 0 4e-06 0.000164 0.00243 0.014058 0.032196 0.029367 0.010661 0.001617 0 0 0 4.3e-05 0.001645 0.024299 0.14058 0.32196 0.29367 0.10661 0.016167 i21_r0_06_w2_10 0 0 0 0 0 1.5e-05 0.000186 0.000893 0.001701 0.001291 0.000438 0 0 0 0 1e-05 0.000304 0.003719 0.017864 0.034022 0.025818 0.00876 0 0 0 1e-06 9.6e-05 0.00304 0.037193 0.17864 0.34022 0.25818 0.087602 i22_r0_06_w2_56 0 0 0 0 0 1e-06 2.7e-05 0.000274 0.001094 0.001733 0.001396 0 0 0 0 0 2.1e-05 0.000541 0.005484 0.021876 0.034654 0.027922 0 0 0 0 3e-06 0.000207 0.005409 0.054839 0.21876 0.34654 0.27922 i23_r0_10_w0_35 0.001396 0.001733 0.001094 0.000274 2.7e-05 1e-06 0 0 0 0 0 0.027922 0.034654 0.021876 0.005484 0.000541 2.1e-05 0 0 0 0 0 0.27922 0.34654 0.21876 0.054839 0.005409 0.000207 3e-06 0 0 0 0 i24_r0_10_w0_42 0.000438 0.001291 0.001701 0.000893 0.000186 1.5e-05 0 0 0 0 0 0.00876 0.025818 0.034022 0.017864 0.003719 0.000304 1e-05 0 0 0 0 0.087602 0.25818 0.34022 0.17864 0.037193 0.00304 9.6e-05 1e-06 0 0 0 i25_r0_10_w0_52 8.1e-05 0.000533 0.001468 0.00161 0.000703 0.000121 8e-06 0 0 0 0 0.001617 0.010661 0.029367 0.032196 0.014058 0.00243 0.000164 4e-06 0 0 0 0.016167 0.10661 0.29367 0.32196 0.14058 0.024299 0.001645 4.3e-05 0 0 0 i26_r0_10_w0_63 8e-06 0.000121 0.000703 0.00161 0.001468 0.000533 7.6e-05 4e-06 0 0 0 0.000169 0.00243 0.014058 0.032196 0.029367 0.010661 0.001529 8.6e-05 2e-06 0 0 0.001689 0.024299 0.14058 0.32196 0.29367 0.10661 0.015291 0.000857 1.9e-05 0 0 i27_r0_10_w0_77 0 1.5e-05 0.000186 0.000893 0.001701 0.001291 0.000389 4.6e-05 2e-06 0 0 1e-05 0.000304 0.003719 0.017864 0.034022 0.025818 0.00779 0.000927 4.3e-05 1e-06 0 9.8e-05 0.00304 0.037193 0.17864 0.34022 0.25818 0.077897 0.009268 0.00043 8e-06 0 i28_r0_10_w0_94 0 1e-06 2.7e-05 0.000274 0.001094 0.001733 0.001094 0.000274 2.7e-05 1e-06 0 0 2.1e-05 0.000541 0.005484 0.021876 0.034654 0.021876 0.005484 0.000541 2.1e-05 0 3e-06 0.000207 0.005409 0.054839 0.21876 0.34654 0.21876 0.054839 0.005409 0.000207 3e-06 i29_r0_10_w1_15 0 0 2e-06 4.6e-05 0.000389 0.001291 0.001701 0.000893 0.000186 1.5e-05 0 0 1e-06 4.3e-05 0.000927 0.00779 0.025818 0.034022 0.017864 0.003719 0.000304 1e-05 0 8e-06 0.00043 0.009268 0.077897 0.25818 0.34022 0.17864 0.037193 0.00304 9.8e-05 i30_r0_10_w1_41 0 0 0 4e-06 7.6e-05 0.000533 0.001468 0.00161 0.000703 0.000121 8e-06 0 0 2e-06 8.6e-05 0.001529 0.010661 0.029367 0.032196 0.014058 0.00243 0.000169 0 0 1.9e-05 0.000857 0.015291 0.10661 0.29367 0.32196 0.14058 0.024299 0.001689 i31_r0_10_w1_72 0 0 0 0 8e-06 0.000121 0.000703 0.00161 0.001468 0.000533 8.1e-05 0 0 0 4e-06 0.000164 0.00243 0.014058 0.032196 0.029367 0.010661 0.001617 0 0 0 4.3e-05 0.001645 0.024299 0.14058 0.32196 0.29367 0.10661 0.016167 i32_r0_10_w2_10 0 0 0 0 0 1.5e-05 0.000186 0.000893 0.001701 0.001291 0.000438 0 0 0 0 1e-05 0.000304 0.003719 0.017864 0.034022 0.025818 0.00876 0 0 0 1e-06 9.6e-05 0.00304 0.037193 0.17864 0.34022 0.25818 0.087602 i33_r0_10_w2_56 0 0 0 0 0 1e-06 2.7e-05 0.000274 0.001094 0.001733 0.001396 0 0 0 0 0 2.1e-05 0.000541 0.005484 0.021876 0.034654 0.027922 0 0 0 0 3e-06 0.000207 0.005409 0.054839 0.21876 0.34654 0.27922
Get Equations
[f_util_log, f_util_crra, f_util_standin, f_util_standin_coh, f_prod, f_inc, f_coh, f_cons] = ...
ffs_ipwkbzr_set_functions(fl_crra, fl_c_min, fl_b_bd, fl_Amean, fl_alpha, fl_delta, fl_r_save, fl_w);
Generate Cash-on-Hand/State Matrix
The endogenous state variable is cash-on-hand, it has it_z_n*it_a_n number of points, covering all reachable points when ar_a is the choice vector and ar_z is the shock vector. requires inputs from get Asset and choice grids, get shock grids, and get equations above.
- mt_coh_wkb_full: this is the (I^k x I^w) by (M^r x M^z) matrix, where rows = it_w_interp_n*it_ak_perc_n, and cols = fl_z_r_borr_n*it_z_wage_n.
- mt_coh_wkb_full: this is the (I^k x I^w x M^r) by (M^z) matrix, where rows = it_w_interp_n*it_ak_perc_n*fl_z_r_borr_n, and cols = it_z_wage_n.
mt_coh_wkb_full = f_coh(ar_z_r_borr_mesh_wage_r1w2, ar_z_wage_mesh_r_borr_r1w2, ... ar_a_meshk_full, ar_k_mesha_full); it_coh_wkb_reshape_rows = it_w_interp_n*it_ak_perc_n*fl_z_r_borr_n; it_coh_wkb_reshape_cols = it_z_wage_n; mt_coh_wkb_full = reshape(mt_coh_wkb_full, [it_coh_wkb_reshape_rows, it_coh_wkb_reshape_cols]); % Generate Aggregate Variables ar_aplusk_mesh = ar_a_meshk_full + ar_k_mesha_full; if (bl_display_funcgrids || bl_graph_funcgrids) % Genereate Table tab_ak_choices = array2table([ar_aplusk_mesh, ar_k_mesha_full, ar_a_meshk_full]); cl_col_names = {'ar_aplusk_mesh', 'ar_k_mesha_full', 'ar_a_meshk_full'}; tab_ak_choices.Properties.VariableNames = cl_col_names; % Label Table Variables tab_ak_choices.Properties.VariableDescriptions{'ar_aplusk_mesh'} = ... '*ar_aplusk_mesha*: ar_aplusk_mesha = ar_a_meshk_full + ar_k_mesha_full;'; tab_ak_choices.Properties.VariableDescriptions{'ar_a_meshk_full'} = ... '*ar_a_meshk_full*:'; tab_ak_choices.Properties.VariableDescriptions{'ar_k_mesha_full'} = ... '*ar_k_mesha_full*:'; cl_var_desc = tab_ak_choices.Properties.VariableDescriptions; for it_var_name = 1:length(cl_var_desc) disp(cl_var_desc{it_var_name}); end disp('----------------------------------------'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp('tab_ak_choices'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); it_rows_toshow = length(ar_w_level)*2; disp(size(tab_ak_choices)); disp(head(array2table(tab_ak_choices), it_rows_toshow)); disp(tail(array2table(tab_ak_choices), it_rows_toshow)); % Generate Shock Full Mat to See coh_wkb_full zr and zw mt_z_r_borr_mwge_makfull = zeros([length(ar_a_meshk_full), it_z_n]) + ar_z_r_borr_mesh_wage_r1w2; mt_z_wage_mesh_r_makfull = zeros([length(ar_a_meshk_full), it_z_n]) + ar_z_wage_mesh_r_borr_r1w2; % use the same reshape command as above mt_z_r_borr_mwge_makfull = reshape(mt_z_r_borr_mwge_makfull, [it_coh_wkb_reshape_rows, it_coh_wkb_reshape_cols]); mt_z_wage_mesh_r_makfull = reshape(mt_z_wage_mesh_r_makfull, [it_coh_wkb_reshape_rows, it_coh_wkb_reshape_cols]); % Generate W and K Choices Full Mat to see which w and k mt_aplusk_mesh = zeros([length(ar_a_meshk_full), it_z_n]) + ar_aplusk_mesh; mt_a_meshk_full = zeros([length(ar_a_meshk_full), it_z_n]) + ar_a_meshk_full; mt_k_mesha_full = zeros([length(ar_a_meshk_full), it_z_n]) + ar_k_mesha_full; % use the same reshape command as above mt_aplusk_mesh = reshape(mt_aplusk_mesh, [it_coh_wkb_reshape_rows, it_coh_wkb_reshape_cols]); mt_a_meshk_full = reshape(mt_a_meshk_full, [it_coh_wkb_reshape_rows, it_coh_wkb_reshape_cols]); mt_k_mesha_full = reshape(mt_k_mesha_full, [it_coh_wkb_reshape_rows, it_coh_wkb_reshape_cols]); disp('----------------------------------------'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp('mt_coh_wkb_full'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp(size(mt_coh_wkb_full)); disp(head(array2table(mt_coh_wkb_full), it_rows_toshow)); disp(tail(array2table(mt_coh_wkb_full), it_rows_toshow)); disp('----------------------------------------'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp('Shock Borrow R: mt_z_r_borr_mwge_makfull'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp(size(mt_z_r_borr_mwge_makfull)); disp(head(array2table(mt_z_r_borr_mwge_makfull), it_rows_toshow)); disp(tail(array2table(mt_z_r_borr_mwge_makfull), it_rows_toshow)); disp('----------------------------------------'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp('Shock Productivity: mt_z_wage_mesh_r_makfull'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp(size(mt_z_wage_mesh_r_makfull)); disp(head(array2table(mt_z_wage_mesh_r_makfull), it_rows_toshow)); disp(tail(array2table(mt_z_wage_mesh_r_makfull), it_rows_toshow)); disp('----------------------------------------'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp('W = Aprime + Kprime; mt_aplusk_mesh'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp(size(mt_aplusk_mesh)); disp(head(array2table(mt_aplusk_mesh), it_rows_toshow)); disp(tail(array2table(mt_aplusk_mesh), it_rows_toshow)); disp('----------------------------------------'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp('Kprime: mt_k_mesha_full'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp(size(mt_k_mesha_full)); disp(head(array2table(mt_k_mesha_full), it_rows_toshow)); disp(tail(array2table(mt_k_mesha_full), it_rows_toshow)); disp('----------------------------------------'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp('Aprime: mt_a_meshk_full'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp(size(mt_a_meshk_full)); disp(head(array2table(mt_a_meshk_full), it_rows_toshow)); disp(tail(array2table(mt_a_meshk_full), it_rows_toshow)); end
*ar_aplusk_mesha*: ar_aplusk_mesha = ar_a_meshk_full + ar_k_mesha_full; *ar_k_mesha_full*: *ar_a_meshk_full*: ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx tab_ak_choices xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx 1215 3 tab_ak_choices1 tab_ak_choices2 tab_ak_choices3 ar_aplusk_mesh ar_k_mesha_full ar_a_meshk_full _______________ _______________ _______________ -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -4.67 0 -4.67 -2.4832 0 -2.4832 -2.4832 0.0497 -2.5329 -2.4832 0.0994 -2.5826 -2.4832 0.1491 -2.6323 -2.4832 0.1988 -2.682 -2.4832 0.2485 -2.7317 -2.4832 0.2982 -2.7814 -2.4832 0.3479 -2.8311 -2.4832 0.3976 -2.8808 tab_ak_choices1 tab_ak_choices2 tab_ak_choices3 ar_aplusk_mesh ar_k_mesha_full ar_a_meshk_full _______________ _______________ _______________ 47.813 42.941 4.8724 47.813 44.134 3.6796 47.813 45.326 2.4868 47.813 46.519 1.294 47.813 47.712 0.10122 47.813 48.905 -1.0916 47.813 50.098 -2.2844 47.813 51.29 -3.4772 47.813 52.483 -4.67 50 0 50 50 1.2425 48.758 50 2.485 47.515 50 3.7275 46.273 50 4.97 45.03 50 6.2125 43.788 50 7.455 42.545 50 8.6975 41.303 50 9.94 40.06 50 11.182 38.818 50 12.425 37.575 50 13.667 36.333 50 14.91 35.09 50 16.152 33.848 50 17.395 32.605 50 18.637 31.363 50 19.88 30.12 50 21.122 28.878 50 22.365 27.635 50 23.607 26.393 50 24.85 25.15 50 26.092 23.908 50 27.335 22.665 50 28.577 21.423 50 29.82 20.18 50 31.062 18.938 50 32.305 17.695 50 33.547 16.453 50 34.79 15.21 50 36.032 13.968 50 37.275 12.725 50 38.517 11.483 50 39.76 10.24 50 41.002 8.9975 50 42.245 7.755 50 43.487 6.5125 50 44.73 5.27 50 45.972 4.0275 50 47.215 2.785 50 48.457 1.5425 50 49.7 0.30002 50 50.942 -0.94248 50 52.185 -2.185 50 53.427 -3.4275 50 54.67 -4.67 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx mt_coh_wkb_full xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx 3645 11 mt_coh_wkb_full1 mt_coh_wkb_full2 mt_coh_wkb_full3 mt_coh_wkb_full4 mt_coh_wkb_full5 mt_coh_wkb_full6 mt_coh_wkb_full7 mt_coh_wkb_full8 mt_coh_wkb_full9 mt_coh_wkb_full10 mt_coh_wkb_full11 ________________ ________________ ________________ ________________ ________________ ________________ ________________ ________________ ________________ _________________ _________________ -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -2.1016 -2.1016 -2.1016 -2.1016 -2.1016 -2.1016 -2.1016 -2.1016 -2.1016 -2.1016 -2.1016 -1.9892 -1.9631 -1.9313 -1.8925 -1.845 -1.787 -1.7162 -1.6298 -1.5241 -1.3951 -1.2376 -1.9611 -1.9276 -1.8868 -1.8369 -1.776 -1.7016 -1.6108 -1.4998 -1.3642 -1.1986 -0.99641 -1.9426 -1.9039 -1.8566 -1.7989 -1.7284 -1.6423 -1.5372 -1.4088 -1.2519 -1.0603 -0.82629 -1.9287 -1.8858 -1.8334 -1.7694 -1.6912 -1.5957 -1.4791 -1.3367 -1.1627 -0.95019 -0.69064 -1.9177 -1.8712 -1.8144 -1.7451 -1.6604 -1.5569 -1.4305 -1.2762 -1.0876 -0.85735 -0.57609 -1.9087 -1.859 -1.7984 -1.7243 -1.6339 -1.5234 -1.3884 -1.2236 -1.0223 -0.77639 -0.47605 -1.9011 -1.8486 -1.7845 -1.7062 -1.6106 -1.4938 -1.3512 -1.1769 -0.96413 -0.7042 -0.38673 -1.8947 -1.8396 -1.7723 -1.6902 -1.5899 -1.4673 -1.3176 -1.1348 -0.91154 -0.63881 -0.3057 mt_coh_wkb_full1 mt_coh_wkb_full2 mt_coh_wkb_full3 mt_coh_wkb_full4 mt_coh_wkb_full5 mt_coh_wkb_full6 mt_coh_wkb_full7 mt_coh_wkb_full8 mt_coh_wkb_full9 mt_coh_wkb_full10 mt_coh_wkb_full11 ________________ ________________ ________________ ________________ ________________ ________________ ________________ ________________ ________________ _________________ _________________ 46.285 46.582 46.945 47.388 47.93 48.591 49.399 50.385 51.59 53.061 54.859 46.173 46.473 46.84 47.287 47.834 48.502 49.317 50.314 51.53 53.016 54.832 46.061 46.364 46.734 47.186 47.738 48.412 49.236 50.241 51.47 52.97 54.803 45.949 46.255 46.628 47.084 47.641 48.322 49.153 50.168 51.408 52.923 54.773 45.836 46.145 46.522 46.982 47.544 48.231 49.07 50.094 51.346 52.874 54.741 45.647 45.958 46.339 46.803 47.37 48.063 48.909 49.943 51.206 52.748 54.631 45.45 45.765 46.148 46.617 47.189 47.888 48.741 49.784 51.058 52.613 54.513 45.254 45.571 45.957 46.43 47.007 47.712 48.573 49.624 50.909 52.477 54.393 45.057 45.376 45.766 46.243 46.825 47.535 48.403 49.464 50.759 52.341 54.272 51.694 51.694 51.694 51.694 51.694 51.694 51.694 51.694 51.694 51.694 51.694 51.938 52.021 52.122 52.246 52.397 52.582 52.808 53.083 53.42 53.831 54.333 51.914 52.02 52.15 52.309 52.503 52.74 53.03 53.383 53.815 54.343 54.987 51.859 51.982 52.133 52.317 52.541 52.815 53.15 53.56 54.059 54.67 55.415 51.789 51.926 52.093 52.297 52.546 52.85 53.222 53.675 54.23 54.907 55.734 51.71 51.859 52.039 52.26 52.53 52.86 53.263 53.754 54.355 55.089 55.985 51.625 51.783 51.977 52.213 52.501 52.853 53.283 53.808 54.449 55.233 56.19 51.536 51.703 51.907 52.156 52.461 52.833 53.288 53.843 54.521 55.349 56.361 51.442 51.618 51.832 52.094 52.413 52.804 53.281 53.863 54.575 55.444 56.505 51.346 51.529 51.753 52.026 52.359 52.767 53.264 53.872 54.614 55.521 56.628 51.248 51.438 51.67 51.954 52.3 52.723 53.24 53.871 54.642 55.584 56.734 51.147 51.344 51.584 51.878 52.236 52.674 53.209 53.862 54.66 55.634 56.825 51.045 51.248 51.496 51.799 52.169 52.621 53.172 53.846 54.67 55.675 56.903 50.941 51.15 51.405 51.717 52.098 52.563 53.131 53.825 54.672 55.707 56.971 50.836 51.051 51.313 51.633 52.024 52.502 53.085 53.798 54.668 55.731 57.029 50.73 50.95 51.219 51.547 51.948 52.438 53.036 53.766 54.658 55.748 57.079 50.623 50.848 51.123 51.459 51.87 52.371 52.983 53.73 54.643 55.759 57.121 50.515 50.745 51.026 51.37 51.789 52.301 52.927 53.691 54.624 55.764 57.156 50.406 50.641 50.928 51.279 51.707 52.229 52.868 53.648 54.601 55.764 57.185 50.297 50.536 50.829 51.186 51.623 52.156 52.807 53.602 54.573 55.76 57.209 50.186 50.43 50.728 51.092 51.537 52.08 52.743 53.553 54.543 55.751 57.227 50.075 50.324 50.627 50.998 51.45 52.003 52.678 53.502 54.509 55.739 57.241 49.964 50.216 50.525 50.902 51.362 51.924 52.61 53.448 54.472 55.723 57.251 49.852 50.108 50.422 50.805 51.272 51.843 52.541 53.393 54.433 55.704 57.256 49.739 50 50.318 50.707 51.182 51.761 52.47 53.335 54.391 55.682 57.258 49.626 49.891 50.214 50.608 51.09 51.678 52.397 53.275 54.347 55.657 57.256 49.513 49.781 50.109 50.509 50.997 51.594 52.323 53.213 54.301 55.629 57.251 49.399 49.671 50.003 50.408 50.904 51.509 52.247 53.15 54.252 55.599 57.243 49.285 49.56 49.896 50.307 50.809 51.422 52.171 53.085 54.202 55.566 57.232 49.17 49.449 49.79 50.206 50.714 51.335 52.093 53.019 54.15 55.531 57.219 49.055 49.337 49.682 50.103 50.618 51.246 52.014 52.951 54.096 55.494 57.202 48.94 49.225 49.574 50.001 50.521 51.157 51.933 52.882 54.041 55.456 57.184 48.824 49.113 49.466 49.897 50.424 51.067 51.852 52.812 53.984 55.415 57.163 48.708 49 49.357 49.793 50.326 50.976 51.77 52.74 53.925 55.372 57.14 48.592 48.887 49.248 49.689 50.227 50.884 51.687 52.668 53.865 55.328 57.115 48.475 48.774 49.138 49.584 50.127 50.792 51.603 52.594 53.804 55.282 57.088 48.359 48.66 49.028 49.478 50.028 50.699 51.518 52.519 53.742 55.235 57.059 48.242 48.546 48.918 49.372 49.927 50.605 51.432 52.443 53.678 55.186 57.028 48.125 48.432 48.807 49.266 49.826 50.51 51.346 52.367 53.613 55.136 56.996 48.007 48.317 48.696 49.159 49.725 50.415 51.259 52.289 53.547 55.084 56.962 47.89 48.203 48.585 49.052 49.623 50.32 51.171 52.211 53.481 55.032 56.926 47.706 48.022 48.408 48.879 49.455 50.158 51.017 52.065 53.347 54.912 56.823 47.501 47.819 48.209 48.684 49.265 49.974 50.84 51.898 53.191 54.769 56.697 47.296 47.617 48.009 48.489 49.075 49.79 50.664 51.731 53.034 54.626 56.57 47.09 47.414 47.81 48.294 48.884 49.605 50.486 51.562 52.876 54.482 56.442 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Shock Borrow R: mt_z_r_borr_mwge_makfull xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx 3645 11 mt_z_r_borr_mwge_makfull1 mt_z_r_borr_mwge_makfull2 mt_z_r_borr_mwge_makfull3 mt_z_r_borr_mwge_makfull4 mt_z_r_borr_mwge_makfull5 mt_z_r_borr_mwge_makfull6 mt_z_r_borr_mwge_makfull7 mt_z_r_borr_mwge_makfull8 mt_z_r_borr_mwge_makfull9 mt_z_r_borr_mwge_makfull10 mt_z_r_borr_mwge_makfull11 _________________________ _________________________ _________________________ _________________________ _________________________ _________________________ _________________________ _________________________ _________________________ __________________________ __________________________ 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 0.025 mt_z_r_borr_mwge_makfull1 mt_z_r_borr_mwge_makfull2 mt_z_r_borr_mwge_makfull3 mt_z_r_borr_mwge_makfull4 mt_z_r_borr_mwge_makfull5 mt_z_r_borr_mwge_makfull6 mt_z_r_borr_mwge_makfull7 mt_z_r_borr_mwge_makfull8 mt_z_r_borr_mwge_makfull9 mt_z_r_borr_mwge_makfull10 mt_z_r_borr_mwge_makfull11 _________________________ _________________________ _________________________ _________________________ _________________________ _________________________ _________________________ _________________________ _________________________ __________________________ __________________________ 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 0.095 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Shock Productivity: mt_z_wage_mesh_r_makfull xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx 3645 11 mt_z_wage_mesh_r_makfull1 mt_z_wage_mesh_r_makfull2 mt_z_wage_mesh_r_makfull3 mt_z_wage_mesh_r_makfull4 mt_z_wage_mesh_r_makfull5 mt_z_wage_mesh_r_makfull6 mt_z_wage_mesh_r_makfull7 mt_z_wage_mesh_r_makfull8 mt_z_wage_mesh_r_makfull9 mt_z_wage_mesh_r_makfull10 mt_z_wage_mesh_r_makfull11 _________________________ _________________________ _________________________ _________________________ _________________________ _________________________ _________________________ _________________________ _________________________ __________________________ __________________________ 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 mt_z_wage_mesh_r_makfull1 mt_z_wage_mesh_r_makfull2 mt_z_wage_mesh_r_makfull3 mt_z_wage_mesh_r_makfull4 mt_z_wage_mesh_r_makfull5 mt_z_wage_mesh_r_makfull6 mt_z_wage_mesh_r_makfull7 mt_z_wage_mesh_r_makfull8 mt_z_wage_mesh_r_makfull9 mt_z_wage_mesh_r_makfull10 mt_z_wage_mesh_r_makfull11 _________________________ _________________________ _________________________ _________________________ _________________________ _________________________ _________________________ _________________________ _________________________ __________________________ __________________________ 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 0.34664 0.42338 0.51712 0.63162 0.77146 0.94226 1.1509 1.4057 1.7169 2.097 2.5613 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx W = Aprime + Kprime; mt_aplusk_mesh xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx 3645 11 mt_aplusk_mesh1 mt_aplusk_mesh2 mt_aplusk_mesh3 mt_aplusk_mesh4 mt_aplusk_mesh5 mt_aplusk_mesh6 mt_aplusk_mesh7 mt_aplusk_mesh8 mt_aplusk_mesh9 mt_aplusk_mesh10 mt_aplusk_mesh11 _______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ ________________ ________________ -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 mt_aplusk_mesh1 mt_aplusk_mesh2 mt_aplusk_mesh3 mt_aplusk_mesh4 mt_aplusk_mesh5 mt_aplusk_mesh6 mt_aplusk_mesh7 mt_aplusk_mesh8 mt_aplusk_mesh9 mt_aplusk_mesh10 mt_aplusk_mesh11 _______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ _______________ ________________ ________________ 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 47.813 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 50 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Kprime: mt_k_mesha_full xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx 3645 11 mt_k_mesha_full1 mt_k_mesha_full2 mt_k_mesha_full3 mt_k_mesha_full4 mt_k_mesha_full5 mt_k_mesha_full6 mt_k_mesha_full7 mt_k_mesha_full8 mt_k_mesha_full9 mt_k_mesha_full10 mt_k_mesha_full11 ________________ ________________ ________________ ________________ ________________ ________________ ________________ ________________ ________________ _________________ _________________ 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0.0497 0.0497 0.0497 0.0497 0.0497 0.0497 0.0497 0.0497 0.0497 0.0497 0.0497 0.0994 0.0994 0.0994 0.0994 0.0994 0.0994 0.0994 0.0994 0.0994 0.0994 0.0994 0.1491 0.1491 0.1491 0.1491 0.1491 0.1491 0.1491 0.1491 0.1491 0.1491 0.1491 0.1988 0.1988 0.1988 0.1988 0.1988 0.1988 0.1988 0.1988 0.1988 0.1988 0.1988 0.2485 0.2485 0.2485 0.2485 0.2485 0.2485 0.2485 0.2485 0.2485 0.2485 0.2485 0.2982 0.2982 0.2982 0.2982 0.2982 0.2982 0.2982 0.2982 0.2982 0.2982 0.2982 0.3479 0.3479 0.3479 0.3479 0.3479 0.3479 0.3479 0.3479 0.3479 0.3479 0.3479 0.3976 0.3976 0.3976 0.3976 0.3976 0.3976 0.3976 0.3976 0.3976 0.3976 0.3976 mt_k_mesha_full1 mt_k_mesha_full2 mt_k_mesha_full3 mt_k_mesha_full4 mt_k_mesha_full5 mt_k_mesha_full6 mt_k_mesha_full7 mt_k_mesha_full8 mt_k_mesha_full9 mt_k_mesha_full10 mt_k_mesha_full11 ________________ ________________ ________________ ________________ ________________ ________________ ________________ ________________ ________________ _________________ _________________ 42.941 42.941 42.941 42.941 42.941 42.941 42.941 42.941 42.941 42.941 42.941 44.134 44.134 44.134 44.134 44.134 44.134 44.134 44.134 44.134 44.134 44.134 45.326 45.326 45.326 45.326 45.326 45.326 45.326 45.326 45.326 45.326 45.326 46.519 46.519 46.519 46.519 46.519 46.519 46.519 46.519 46.519 46.519 46.519 47.712 47.712 47.712 47.712 47.712 47.712 47.712 47.712 47.712 47.712 47.712 48.905 48.905 48.905 48.905 48.905 48.905 48.905 48.905 48.905 48.905 48.905 50.098 50.098 50.098 50.098 50.098 50.098 50.098 50.098 50.098 50.098 50.098 51.29 51.29 51.29 51.29 51.29 51.29 51.29 51.29 51.29 51.29 51.29 52.483 52.483 52.483 52.483 52.483 52.483 52.483 52.483 52.483 52.483 52.483 0 0 0 0 0 0 0 0 0 0 0 1.2425 1.2425 1.2425 1.2425 1.2425 1.2425 1.2425 1.2425 1.2425 1.2425 1.2425 2.485 2.485 2.485 2.485 2.485 2.485 2.485 2.485 2.485 2.485 2.485 3.7275 3.7275 3.7275 3.7275 3.7275 3.7275 3.7275 3.7275 3.7275 3.7275 3.7275 4.97 4.97 4.97 4.97 4.97 4.97 4.97 4.97 4.97 4.97 4.97 6.2125 6.2125 6.2125 6.2125 6.2125 6.2125 6.2125 6.2125 6.2125 6.2125 6.2125 7.455 7.455 7.455 7.455 7.455 7.455 7.455 7.455 7.455 7.455 7.455 8.6975 8.6975 8.6975 8.6975 8.6975 8.6975 8.6975 8.6975 8.6975 8.6975 8.6975 9.94 9.94 9.94 9.94 9.94 9.94 9.94 9.94 9.94 9.94 9.94 11.182 11.182 11.182 11.182 11.182 11.182 11.182 11.182 11.182 11.182 11.182 12.425 12.425 12.425 12.425 12.425 12.425 12.425 12.425 12.425 12.425 12.425 13.667 13.667 13.667 13.667 13.667 13.667 13.667 13.667 13.667 13.667 13.667 14.91 14.91 14.91 14.91 14.91 14.91 14.91 14.91 14.91 14.91 14.91 16.152 16.152 16.152 16.152 16.152 16.152 16.152 16.152 16.152 16.152 16.152 17.395 17.395 17.395 17.395 17.395 17.395 17.395 17.395 17.395 17.395 17.395 18.637 18.637 18.637 18.637 18.637 18.637 18.637 18.637 18.637 18.637 18.637 19.88 19.88 19.88 19.88 19.88 19.88 19.88 19.88 19.88 19.88 19.88 21.122 21.122 21.122 21.122 21.122 21.122 21.122 21.122 21.122 21.122 21.122 22.365 22.365 22.365 22.365 22.365 22.365 22.365 22.365 22.365 22.365 22.365 23.607 23.607 23.607 23.607 23.607 23.607 23.607 23.607 23.607 23.607 23.607 24.85 24.85 24.85 24.85 24.85 24.85 24.85 24.85 24.85 24.85 24.85 26.092 26.092 26.092 26.092 26.092 26.092 26.092 26.092 26.092 26.092 26.092 27.335 27.335 27.335 27.335 27.335 27.335 27.335 27.335 27.335 27.335 27.335 28.577 28.577 28.577 28.577 28.577 28.577 28.577 28.577 28.577 28.577 28.577 29.82 29.82 29.82 29.82 29.82 29.82 29.82 29.82 29.82 29.82 29.82 31.062 31.062 31.062 31.062 31.062 31.062 31.062 31.062 31.062 31.062 31.062 32.305 32.305 32.305 32.305 32.305 32.305 32.305 32.305 32.305 32.305 32.305 33.547 33.547 33.547 33.547 33.547 33.547 33.547 33.547 33.547 33.547 33.547 34.79 34.79 34.79 34.79 34.79 34.79 34.79 34.79 34.79 34.79 34.79 36.032 36.032 36.032 36.032 36.032 36.032 36.032 36.032 36.032 36.032 36.032 37.275 37.275 37.275 37.275 37.275 37.275 37.275 37.275 37.275 37.275 37.275 38.517 38.517 38.517 38.517 38.517 38.517 38.517 38.517 38.517 38.517 38.517 39.76 39.76 39.76 39.76 39.76 39.76 39.76 39.76 39.76 39.76 39.76 41.002 41.002 41.002 41.002 41.002 41.002 41.002 41.002 41.002 41.002 41.002 42.245 42.245 42.245 42.245 42.245 42.245 42.245 42.245 42.245 42.245 42.245 43.487 43.487 43.487 43.487 43.487 43.487 43.487 43.487 43.487 43.487 43.487 44.73 44.73 44.73 44.73 44.73 44.73 44.73 44.73 44.73 44.73 44.73 45.972 45.972 45.972 45.972 45.972 45.972 45.972 45.972 45.972 45.972 45.972 47.215 47.215 47.215 47.215 47.215 47.215 47.215 47.215 47.215 47.215 47.215 48.457 48.457 48.457 48.457 48.457 48.457 48.457 48.457 48.457 48.457 48.457 49.7 49.7 49.7 49.7 49.7 49.7 49.7 49.7 49.7 49.7 49.7 50.942 50.942 50.942 50.942 50.942 50.942 50.942 50.942 50.942 50.942 50.942 52.185 52.185 52.185 52.185 52.185 52.185 52.185 52.185 52.185 52.185 52.185 53.427 53.427 53.427 53.427 53.427 53.427 53.427 53.427 53.427 53.427 53.427 54.67 54.67 54.67 54.67 54.67 54.67 54.67 54.67 54.67 54.67 54.67 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Aprime: mt_a_meshk_full xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx 3645 11 mt_a_meshk_full1 mt_a_meshk_full2 mt_a_meshk_full3 mt_a_meshk_full4 mt_a_meshk_full5 mt_a_meshk_full6 mt_a_meshk_full7 mt_a_meshk_full8 mt_a_meshk_full9 mt_a_meshk_full10 mt_a_meshk_full11 ________________ ________________ ________________ ________________ ________________ ________________ ________________ ________________ ________________ _________________ _________________ -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.4832 -2.5329 -2.5329 -2.5329 -2.5329 -2.5329 -2.5329 -2.5329 -2.5329 -2.5329 -2.5329 -2.5329 -2.5826 -2.5826 -2.5826 -2.5826 -2.5826 -2.5826 -2.5826 -2.5826 -2.5826 -2.5826 -2.5826 -2.6323 -2.6323 -2.6323 -2.6323 -2.6323 -2.6323 -2.6323 -2.6323 -2.6323 -2.6323 -2.6323 -2.682 -2.682 -2.682 -2.682 -2.682 -2.682 -2.682 -2.682 -2.682 -2.682 -2.682 -2.7317 -2.7317 -2.7317 -2.7317 -2.7317 -2.7317 -2.7317 -2.7317 -2.7317 -2.7317 -2.7317 -2.7814 -2.7814 -2.7814 -2.7814 -2.7814 -2.7814 -2.7814 -2.7814 -2.7814 -2.7814 -2.7814 -2.8311 -2.8311 -2.8311 -2.8311 -2.8311 -2.8311 -2.8311 -2.8311 -2.8311 -2.8311 -2.8311 -2.8808 -2.8808 -2.8808 -2.8808 -2.8808 -2.8808 -2.8808 -2.8808 -2.8808 -2.8808 -2.8808 mt_a_meshk_full1 mt_a_meshk_full2 mt_a_meshk_full3 mt_a_meshk_full4 mt_a_meshk_full5 mt_a_meshk_full6 mt_a_meshk_full7 mt_a_meshk_full8 mt_a_meshk_full9 mt_a_meshk_full10 mt_a_meshk_full11 ________________ ________________ ________________ ________________ ________________ ________________ ________________ ________________ ________________ _________________ _________________ 4.8724 4.8724 4.8724 4.8724 4.8724 4.8724 4.8724 4.8724 4.8724 4.8724 4.8724 3.6796 3.6796 3.6796 3.6796 3.6796 3.6796 3.6796 3.6796 3.6796 3.6796 3.6796 2.4868 2.4868 2.4868 2.4868 2.4868 2.4868 2.4868 2.4868 2.4868 2.4868 2.4868 1.294 1.294 1.294 1.294 1.294 1.294 1.294 1.294 1.294 1.294 1.294 0.10122 0.10122 0.10122 0.10122 0.10122 0.10122 0.10122 0.10122 0.10122 0.10122 0.10122 -1.0916 -1.0916 -1.0916 -1.0916 -1.0916 -1.0916 -1.0916 -1.0916 -1.0916 -1.0916 -1.0916 -2.2844 -2.2844 -2.2844 -2.2844 -2.2844 -2.2844 -2.2844 -2.2844 -2.2844 -2.2844 -2.2844 -3.4772 -3.4772 -3.4772 -3.4772 -3.4772 -3.4772 -3.4772 -3.4772 -3.4772 -3.4772 -3.4772 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 50 50 50 50 50 50 50 50 50 50 50 48.758 48.758 48.758 48.758 48.758 48.758 48.758 48.758 48.758 48.758 48.758 47.515 47.515 47.515 47.515 47.515 47.515 47.515 47.515 47.515 47.515 47.515 46.273 46.273 46.273 46.273 46.273 46.273 46.273 46.273 46.273 46.273 46.273 45.03 45.03 45.03 45.03 45.03 45.03 45.03 45.03 45.03 45.03 45.03 43.788 43.788 43.788 43.788 43.788 43.788 43.788 43.788 43.788 43.788 43.788 42.545 42.545 42.545 42.545 42.545 42.545 42.545 42.545 42.545 42.545 42.545 41.303 41.303 41.303 41.303 41.303 41.303 41.303 41.303 41.303 41.303 41.303 40.06 40.06 40.06 40.06 40.06 40.06 40.06 40.06 40.06 40.06 40.06 38.818 38.818 38.818 38.818 38.818 38.818 38.818 38.818 38.818 38.818 38.818 37.575 37.575 37.575 37.575 37.575 37.575 37.575 37.575 37.575 37.575 37.575 36.333 36.333 36.333 36.333 36.333 36.333 36.333 36.333 36.333 36.333 36.333 35.09 35.09 35.09 35.09 35.09 35.09 35.09 35.09 35.09 35.09 35.09 33.848 33.848 33.848 33.848 33.848 33.848 33.848 33.848 33.848 33.848 33.848 32.605 32.605 32.605 32.605 32.605 32.605 32.605 32.605 32.605 32.605 32.605 31.363 31.363 31.363 31.363 31.363 31.363 31.363 31.363 31.363 31.363 31.363 30.12 30.12 30.12 30.12 30.12 30.12 30.12 30.12 30.12 30.12 30.12 28.878 28.878 28.878 28.878 28.878 28.878 28.878 28.878 28.878 28.878 28.878 27.635 27.635 27.635 27.635 27.635 27.635 27.635 27.635 27.635 27.635 27.635 26.393 26.393 26.393 26.393 26.393 26.393 26.393 26.393 26.393 26.393 26.393 25.15 25.15 25.15 25.15 25.15 25.15 25.15 25.15 25.15 25.15 25.15 23.908 23.908 23.908 23.908 23.908 23.908 23.908 23.908 23.908 23.908 23.908 22.665 22.665 22.665 22.665 22.665 22.665 22.665 22.665 22.665 22.665 22.665 21.423 21.423 21.423 21.423 21.423 21.423 21.423 21.423 21.423 21.423 21.423 20.18 20.18 20.18 20.18 20.18 20.18 20.18 20.18 20.18 20.18 20.18 18.938 18.938 18.938 18.938 18.938 18.938 18.938 18.938 18.938 18.938 18.938 17.695 17.695 17.695 17.695 17.695 17.695 17.695 17.695 17.695 17.695 17.695 16.453 16.453 16.453 16.453 16.453 16.453 16.453 16.453 16.453 16.453 16.453 15.21 15.21 15.21 15.21 15.21 15.21 15.21 15.21 15.21 15.21 15.21 13.968 13.968 13.968 13.968 13.968 13.968 13.968 13.968 13.968 13.968 13.968 12.725 12.725 12.725 12.725 12.725 12.725 12.725 12.725 12.725 12.725 12.725 11.483 11.483 11.483 11.483 11.483 11.483 11.483 11.483 11.483 11.483 11.483 10.24 10.24 10.24 10.24 10.24 10.24 10.24 10.24 10.24 10.24 10.24 8.9975 8.9975 8.9975 8.9975 8.9975 8.9975 8.9975 8.9975 8.9975 8.9975 8.9975 7.755 7.755 7.755 7.755 7.755 7.755 7.755 7.755 7.755 7.755 7.755 6.5125 6.5125 6.5125 6.5125 6.5125 6.5125 6.5125 6.5125 6.5125 6.5125 6.5125 5.27 5.27 5.27 5.27 5.27 5.27 5.27 5.27 5.27 5.27 5.27 4.0275 4.0275 4.0275 4.0275 4.0275 4.0275 4.0275 4.0275 4.0275 4.0275 4.0275 2.785 2.785 2.785 2.785 2.785 2.785 2.785 2.785 2.785 2.785 2.785 1.5425 1.5425 1.5425 1.5425 1.5425 1.5425 1.5425 1.5425 1.5425 1.5425 1.5425 0.30002 0.30002 0.30002 0.30002 0.30002 0.30002 0.30002 0.30002 0.30002 0.30002 0.30002 -0.94248 -0.94248 -0.94248 -0.94248 -0.94248 -0.94248 -0.94248 -0.94248 -0.94248 -0.94248 -0.94248 -2.185 -2.185 -2.185 -2.185 -2.185 -2.185 -2.185 -2.185 -2.185 -2.185 -2.185 -3.4275 -3.4275 -3.4275 -3.4275 -3.4275 -3.4275 -3.4275 -3.4275 -3.4275 -3.4275 -3.4275 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67
Check if COH is within Borrowing Bounds
some coh levels are below borrowing bound, can not borrow enough to pay debt
mt_bl_coh_wkb_invalid = (mt_coh_wkb_full < fl_b_bd); % (k,a) invalid if coh(k,a,z) < bd for any z ar_bl_wkb_invalid = max(mt_bl_coh_wkb_invalid,[], 2); mt_bl_wkb_invalid = reshape(ar_bl_wkb_invalid, [it_ak_perc_n, it_w_interp_n*fl_z_r_borr_n]); % find the first w_level choice where some k(w) percent choices are valid? ar_bl_w_level_invalid = min(mt_bl_wkb_invalid, [], 1); % w choices can not be lower than fl_w_level_min_valid. If w choices are % lower, given the current borrowing interest rate as well as the minimum % income level in the future, and the maximum borrowing level available % next period, and given the shock distribution, there exists some state in % the future when the household when making this choice will be unable to % borrow sufficiently to maintain positive consumption. ar_w_level_full_dup = repmat(ar_w_level_full, [1,fl_z_r_borr_n]); fl_w_level_min_valid = min(ar_w_level_full_dup(~ar_bl_w_level_invalid));
Update Valid 2nd stage choice matrix
ar_w_level = linspace(fl_w_level_min_valid, fl_w_max, it_w_interp_n); ar_k_max = ar_w_level - fl_b_bd; mt_k = (ar_k_max'*ar_ak_perc)'; mt_a = (ar_w_level - mt_k); ar_a_meshk = mt_a(:); ar_k_mesha = mt_k(:);
Generate 2nd Stage Cash-on-Hand Points for Interpolation
% it_ak_perc_n = length(ar_ak_perc); % it_w_interp_n = length(ar_w_level); it_wak_n = it_w_interp_n*it_ak_perc_n; % mt_coh_wkb = mt_coh_wkb_full(~ar_bl_wkb_invalid, :); mt_coh_wkb = mt_coh_wkb_full; mt_coh_wkb_mesh_z_r_borr = repmat(mt_coh_wkb_full, [1, fl_z_r_borr_n]); mt_z_r_borr_mesh_coh_wkb = repmat(ar_z_r_borr, [size(mt_coh_wkb,1),1]); mt_z_wage_mesh_coh_wkb = repmat(ar_z_wage, [size(mt_coh_wkb,1),1]); mt_z_mesh_coh_wkb = repmat((1:it_z_n), [size(mt_coh_wkb,1), 1]); mt_z_mesh_coh_wkb_seg = repmat((1:it_z_n), [it_wak_n, 1]); if (ismember(st_v_coh_z_interp_method, ["method_cell"])) cl_mt_coh_wkb_mesh_z_r_borr = cell([fl_z_r_borr_n, 1]); for it_z_r_borr_ctr = 1:1:fl_z_r_borr_n it_mt_val_row_start = it_wak_n*(it_z_r_borr_ctr-1) + 1; it_mt_val_row_end = it_mt_val_row_start + it_wak_n - 1; cl_mt_coh_wkb_mesh_z_r_borr{it_z_r_borr_ctr} = ... mt_coh_wkb_mesh_z_r_borr(it_mt_val_row_start:it_mt_val_row_end, :); end elseif (ismember(st_v_coh_z_interp_method, ["method_idx_a", "method_idx_b"])) % This is borrowing with default or not condition fl_min_mt_coh = fl_b_bd; cl_mt_coh_wkb_mesh_z_r_borr = cell([fl_z_r_borr_n, 1]); for it_z_r_borr_ctr = 1:1:fl_z_r_borr_n it_mt_val_row_start = it_wak_n*(it_z_r_borr_ctr-1) + 1; it_mt_val_row_end = it_mt_val_row_start + it_wak_n - 1; mt_coh_wkb_mesh_z_r_borr_seg = ... 1 + ((mt_coh_wkb_mesh_z_r_borr(it_mt_val_row_start:it_mt_val_row_end, :) - fl_min_mt_coh)/fl_coh_interp_grid_gap); cl_mt_coh_wkb_mesh_z_r_borr{it_z_r_borr_ctr} = mt_coh_wkb_mesh_z_r_borr_seg; end end
Generate 1st Stage States: Interpolation Cash-on-hand Interpolation Grid
For the iwkz problems, we solve the problem along a grid of cash-on-hand values, the interpolate to find v(k',b',z) at (k',b') choices. Crucially, we have to coh matrxies
fl_min_mt_coh = fl_b_bd; fl_max_mt_coh = max(max(mt_coh_wkb)); % This is savings only condition % fl_min_mt_coh = min(min(mt_coh_wkb)); % This could be condition if no defaults are allowed % fl_min_mt_coh = fl_w_level_min_valid; it_coh_interp_n = (fl_max_mt_coh-fl_min_mt_coh)/(fl_coh_interp_grid_gap); ar_interp_coh_grid = fft_array_add_zero(linspace(fl_min_mt_coh, fl_max_mt_coh, it_coh_interp_n), true); mt_interp_coh_grid_mesh_w_perc = repmat(ar_interp_coh_grid, [it_w_perc_n, 1]); it_coh_interp_n = length(ar_interp_coh_grid); [mt_interp_coh_grid_mesh_z_wage, mt_z_wage_mesh_interp_coh_grid] = ndgrid(ar_interp_coh_grid, ar_z_wage); mt_interp_coh_grid_mesh_z = repmat(ar_interp_coh_grid', [1, it_z_n]); % This is meshed index, 1:it_z_n index for, common value each column mt_z_mesh_interp_coh_grid = repmat((1:it_z_n), [it_coh_interp_n, 1]);
Generate 1st Stage Choices: Interpolation Cash-on-hand Interpolation Grid
previously, our ar_w was the first stage choice grid, the grid was the same for all coh levels. Now, for each coh level, there is a different ar_w. ar_interp_coh_grid is (1 by ar_interp_coh_grid) and ar_w_perc is ( 1 by it_w_perc_n). Conditional on z, each choice matrix is (it_w_perc_n by ar_interp_coh_grid). Here we are pre-computing the choice matrix. This could be a large matrix if the choice grid is large. This is the matrix of aggregate savings choices
if (fl_min_mt_coh < 0) % borrowing bound is below zero mt_w_perc_mesh_interp_coh_grid = ((ar_interp_coh_grid-fl_min_mt_coh)'*ar_w_perc)' + fl_min_mt_coh; else % savings only mt_w_perc_mesh_interp_coh_grid = ((ar_interp_coh_grid)'*ar_w_perc)'; end
Generate Interpolation Consumption Grid
We also interpolate over consumption to speed the program up. We only solve for u(c) at this grid for the iwkz problmes, and then interpolate other c values.
fl_c_max = max(max(mt_coh_wkb_full)) - fl_b_bd; it_interp_c_grid_n = (fl_c_max-fl_c_min)/(it_c_interp_grid_gap); ar_interp_c_grid = linspace(fl_c_min, fl_c_max, it_interp_c_grid_n);
Initialize armt_map to store, state, choice, shock matrixes
armt_map = containers.Map('KeyType','char', 'ValueType','any'); armtdesc_map = containers.Map('KeyType','char', 'ValueType','any');
Store armt_map (1): base arrays
Dimensions of Various Grids: I for level grid, M for shock grid, P for percent grid. Dimensions are:
- ar_interp_c_grid: 1 by I^c
- ar_interp_coh_grid: 1 by I^{coh}
- ar_w_level: 1 by I^{W=k+b}
- ar_w_perc: 1 by P^{W=k+b}
- ar_ak_perc: 1 by P^{k and b}
more descriptions:
- ar_interp_c_grid: 1 by I^c, 1st stage consumption interpolation
- ar_interp_coh_grid: 1 by I^{coh}, 1st stage value function V(coh,z)
- ar_w_level: 1 by I^{W=k+b}, 2nd stage k*(w,z) w grid. 2nd stage, level of w over which we solve the optimal percentage k' choices. Need to generate interpolant based on this so that we know optimal k* given ar_w_perc(coh) in the 1st stage
- ar_w_perc: 1 by P^{W=k+b}, 1st stage w \in {w_perc(coh)} choice set. 1st stage, percentage w choice given coh, at each coh level the number of choice points is the same for this problem with percentage grid points.
- ar_ak_perc: 1 by P^{k and b}, 2nd stage k \in {ask_perc(w,z)} set
armt_map('ar_interp_c_grid') = ar_interp_c_grid; armt_map('ar_interp_coh_grid') = ar_interp_coh_grid; armt_map('ar_w_level') = ar_w_level; armt_map('ar_w_perc') = ar_w_perc; armt_map('ar_ak_perc') = ar_ak_perc;
Store armt_map (2): 1st stage level coh on hand related arrays
Dimensions of Various Grids: I for level grid, M for shock grid, P for percent grid. Dimensions are:
- mt_interp_coh_grid_mesh_z_wage: I^{coh} by M^w
- mt_z_wage_mesh_interp_coh_grid: I^{coh} by M^w
- mt_interp_coh_grid_mesh_w_perc: I^{coh} by P^{LAM=k+b}
- mt_w_perc_mesh_interp_coh_grid: I^{coh} by P^{LAM=k+b}
more descriptions:
- mt_w_perc_mesh_interp_coh_grid 1st stage, generate w(coh, percent), meaning the level of w given coh and the percentage grid of ar_w_perc. Mesh this with the coh grid, Rows here correspond to percentage of w choices, columns correspond to cash-on-hand. The columns of cash-on-hand is determined by ar_interp_coh_grid, because we solve the 1st stage problem at that coh grid.
armt_map('mt_interp_coh_grid_mesh_z_wage') = mt_interp_coh_grid_mesh_z_wage; armt_map('mt_z_wage_mesh_interp_coh_grid') = mt_z_wage_mesh_interp_coh_grid; armt_map('mt_interp_coh_grid_mesh_w_perc') = mt_interp_coh_grid_mesh_w_perc; armt_map('mt_w_perc_mesh_interp_coh_grid') = mt_w_perc_mesh_interp_coh_grid; armt_map('mt_interp_coh_grid_mesh_z') = mt_interp_coh_grid_mesh_z; armt_map('mt_z_mesh_interp_coh_grid') = mt_z_mesh_interp_coh_grid;
Store armt_map (3): 2nd stage reachable coh(k(w), a(w,k), z', r)
Dimensions of Various Grids: I for level grid, M for shock grid, P for percent grid. These are grids for 1st stage solution
- mt_coh_wkb: (I^k x I^w x M^r) by (M^z)
- mt_z_wage_mesh_coh_wkb: (I^k x I^w x M^r) by (M^z)
armt_map('mt_coh_wkb') = mt_coh_wkb_full; armt_map('mt_coh_wkb_mesh_z_r_borr') = mt_coh_wkb_mesh_z_r_borr; armt_map('mt_z_mesh_coh_wkb') = mt_z_mesh_coh_wkb; armt_map('mt_z_wage_mesh_coh_wkb') = mt_z_wage_mesh_coh_wkb; armt_map('cl_mt_coh_wkb_mesh_z_r_borr') = cl_mt_coh_wkb_mesh_z_r_borr; armt_map('mt_z_mesh_coh_wkb_seg') = mt_z_mesh_coh_wkb_seg; % armt_map('mt_z_r_borr_mesh_coh_wkb') = mt_z_r_borr_mesh_coh_wkb;
Store armt_map (4): 2nd stage additional arrays
Dimensions of Various Grids: I for level grid, M for shock grid, P for percent grid. These are grids for 1st stage solution
- mt_k: (I^w) by (P^{k and b})
- ar_a_meshk: 1 by (I^w x P^{k and b})
- ar_k_mesha: 1 by (I^w x P^{k and b})
- ar_aplusk_mesh: 1 by (I^w x P^{k and b})
- it_ameshk_n: scalar
armt_map('mt_k') = mt_k; armt_map('ar_a_meshk') = ar_a_meshk; armt_map('ar_k_mesha') = ar_k_mesha; armt_map('ar_aplusk_mesh') = ar_aplusk_mesh; armt_map('it_ameshk_n') = length(ar_a_meshk);
Store armt_map (5): Shock Grids Arrays and Mesh
Dimensions of Various Grids: I for level grid, M for shock grid, P for percent grid. These are grids for 1st stage solution
- ar_z_r_borr: 1 by (M^r)
- ar_z_r_borr_prob: 1 by (M^r)
- ar_z_wage: 1 by (M^z)
- ar_z_wage_prob: 1 by (M^z)
- ar_z_r_borr_mesh_wage_w1r2: 1 by (M^z x M^r)
- ar_z_wage_mesh_r_borr_w1r2: 1 by (M^z x M^r)
- ar_z_r_borr_mesh_wage_r1w2: 1 by (M^r x M^z)
- ar_z_wage_mesh_r_borr_r1w2: 1 by (M^r x M^z)
armt_map('ar_z_r_borr') = ar_z_r_borr; armt_map('ar_z_r_borr_prob') = ar_z_r_borr_prob; armt_map('ar_z_wage') = ar_z_wage; armt_map('ar_z_wage_prob') = ar_z_wage_prob; armt_map('ar_z_r_borr_mesh_wage_w1r2') = ar_z_r_borr_mesh_wage_w1r2; armt_map('ar_z_wage_mesh_r_borr_w1r2') = ar_z_wage_mesh_r_borr_w1r2; armt_map('ar_z_r_borr_mesh_wage_r1w2') = ar_z_r_borr_mesh_wage_r1w2; armt_map('ar_z_wage_mesh_r_borr_r1w2') = ar_z_wage_mesh_r_borr_r1w2; armt_map('mt_z_trans') = mt_z_trans;
Store Function Map
func_map = containers.Map('KeyType','char', 'ValueType','any'); func_map('f_util_log') = f_util_log; func_map('f_util_crra') = f_util_crra; func_map('f_util_standin') = f_util_standin; func_map('f_util_standin_coh') = f_util_standin_coh; func_map('f_prod') = f_prod; func_map('f_inc') = f_inc; func_map('f_coh') = f_coh; func_map('f_cons') = f_cons;
Graph
if (bl_graph_funcgrids)
Generate Limited Legends
8 graph points, 2 levels of borrow rates, and 4 levels of rbr rates
ar_it_z_r_borr = ([1 round((fl_z_r_borr_n)/2) (fl_z_r_borr_n)]); ar_it_z_wage = ([1 round((it_z_wage_n)/2) (it_z_wage_n)]); % combine by index mt_it_z_graph = ar_it_z_wage' + it_z_wage_n*(ar_it_z_r_borr-1); ar_it_z_graph = mt_it_z_graph(:)'; ar_it_z_graph_zwage = ([1 round((it_z_wage_n)/4) 2*round((it_z_wage_n)/4) 3*round((it_z_wage_n)/4) (it_z_wage_n)]); % legends index final cl_st_legendCell = cellstr([num2str(ar_z_r_borr_mesh_wage_w1r2', 'zr=%3.2f;'), ... num2str(ar_z_wage_mesh_r_borr_w1r2', 'zw=%3.2f')]); % legends index final full mat wage only cl_st_legendCell_zwage = cellstr([num2str(ar_z_wage', 'zw=%3.2f')]);
Graph 1: a and k choice grid graphs
compare the figure here to the same figure in ffs_akz_get_funcgrid. there the grid points are on an even grid, half of the grid points have NA. for the grid here, the grid points get denser as we get closer to low w = k'+b' levels. This is what is different visually about percentage points based choice grid for the 2nd stage problem.
figure('PaperPosition', [0 0 7 4]); hold on; chart = plot(mt_a, mt_k, 'blue'); clr = jet(numel(chart)); for m = 1:numel(chart) set(chart(m),'Color',clr(m,:)) end % if (length(ar_w_level_full) <= 100) scatter(ar_a_meshk, ar_k_mesha, 3, 'filled', ... 'MarkerEdgeColor', 'b', 'MarkerFaceColor', 'b'); % end if (length(ar_w_level_full) <= 100) gf_invalid_scatter = scatter(mt_a_meshk_full(ar_bl_wkb_invalid),... mt_k_mesha_full(ar_bl_wkb_invalid),... 20, 'O', 'MarkerEdgeColor', 'black', 'MarkerFaceColor', 'black'); end xline(0); yline(0); title('Risky K Percentage Grids Given w=k+a (2nd Stage)') ylabel('Capital Choice (mt\_k)') xlabel({'Borrowing (<0) or Saving (>0) (mt\_a)'... 'Each Diagonal Line a Different w=k+a level'... 'Percentage for Risky K along Each Diagonal'}) legend2plot = fliplr([1 round(numel(chart)/3) round((2*numel(chart))/4) numel(chart)]); legendCell = cellstr(num2str(ar_w_level', 'k+a=%3.2f')); if (length(ar_w_level_full) <= 100) chart(length(chart)+1) = gf_invalid_scatter; legendCell{length(legendCell) + 1} = 'Invalid: COH(a,b,z)<bar(b) some z'; legend(chart([legend2plot length(legendCell)]), legendCell([legend2plot length(legendCell)]), 'Location', 'northeast'); else legend(chart([legend2plot]), legendCell([legend2plot]), 'Location', 'northeast'); end grid on;
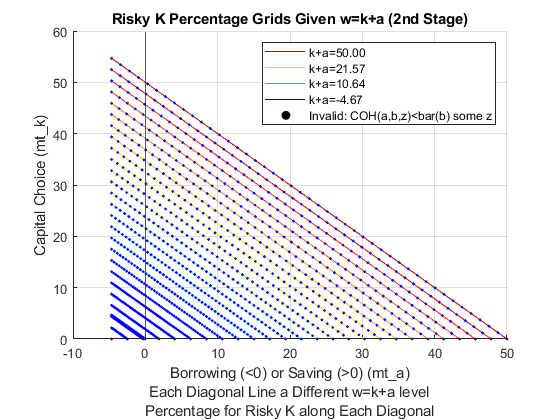
Graph 2: coh by shock
compare the figure here to the same figure in ffs_akz_get_funcgrid. there the grid points are on an even grid. Visually, one could see that the blue/red line segments here are always the same length, but in the ffs_akz_get_funcgrid figure, they are increasingly longer as we move towards the right. They are even because the number of percentage points available is constant regardless of w = k' + b' levels. But previously, the number of grid points available is increasing as w increases since choice grid is based on levels.
figure('PaperPosition', [0 0 7 4]); chart = plot(0:1:(size(mt_coh_wkb_full,1)-1), mt_coh_wkb_full); clr = jet(numel(chart)); for m = 1:numel(chart) set(chart(m),'Color',clr(m,:)) end % zero lines xline(0); yline(0); % invalid points separating lines yline_borrbound = yline(fl_b_bd); yline_borrbound.HandleVisibility = 'on'; yline_borrbound.LineStyle = '--'; yline_borrbound.Color = 'blue'; yline_borrbound.LineWidth = 2.5; title('Cash-on-Hand given w(k+b),k,z'); ylabel('Cash-on-Hand (mt\_coh\_wkb\_full)'); xlabel({'Index of Cash-on-Hand Discrete Point (0:1:(size(mt\_coh\_wkb\_full,1)-1))'... 'Super-Segment: borrow r; Sub-Segment: w=k+b; within seg increasing k'... 'For each w and z, coh maximizing k is different'}); cl_st_legendCell_here = cl_st_legendCell_zwage; cl_st_legendCell_here{length(cl_st_legendCell_here) + 1} = 'borrow-constraint'; chart(length(chart)+1) = yline_borrbound; legend(chart([ar_it_z_graph_zwage length(cl_st_legendCell_here)]), ... cl_st_legendCell_here([ar_it_z_graph_zwage length(cl_st_legendCell_here)]), 'Location', 'southeast'); grid on;
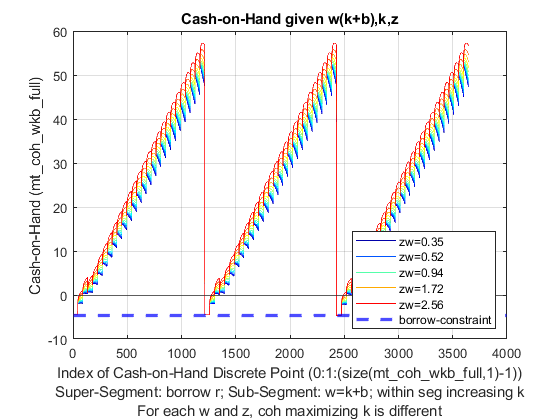
Graph 3: 1st State Aggregate Savings Choices by COH interpolation grids
figure('PaperPosition', [0 0 7 4]); hold on; chart = plot(ar_interp_coh_grid, mt_w_perc_mesh_interp_coh_grid'); clr = jet(numel(chart)); for m = 1:numel(chart) set(chart(m),'Color',clr(m,:)) end if (length(ar_interp_coh_grid) <= 100) [~, mt_interp_coh_grid_mesh_w_perc] = ndgrid(ar_w_perc, ar_interp_coh_grid); scatter(mt_interp_coh_grid_mesh_w_perc(:), mt_w_perc_mesh_interp_coh_grid(:), 3, 'filled', ... 'MarkerEdgeColor', 'b', 'MarkerFaceColor', 'b'); end % invalid points separating lines yline_borrbound = yline(fl_w_level_min_valid); yline_borrbound.HandleVisibility = 'on'; yline_borrbound.LineStyle = '--'; yline_borrbound.Color = 'red'; yline_borrbound.LineWidth = 2.5; xline0 = xline(0); xline0.HandleVisibility = 'off'; yline0 = yline(0); yline0.HandleVisibility = 'off'; title({'Aggregate Savings Percentage Grids (1st Stage)' ... 'y=mt\_w\_by\_interp\_coh\_interp\_grid, and, y=ar\_interp\_coh\_grid'}); ylabel('1st Stage Aggregate Savings Choices'); xlabel({'Cash-on-Hand Levels (Interpolation Points)'... 'w(coh)>min-agg-save, coh(k(w),w-k)>=bar(b)'}); legend2plot = fliplr([1 round(numel(chart)/3) round((2*numel(chart))/4) numel(chart)]); legendCell = cellstr(num2str(ar_w_perc', 'ar w perc=%3.2f')); legendCell{length(legendCell) + 1} = 'min-agg-save'; chart(length(chart)+1) = yline_borrbound; legend(chart([legend2plot length(legendCell)]), legendCell([legend2plot length(legendCell)]), 'Location', 'northwest'); grid on;
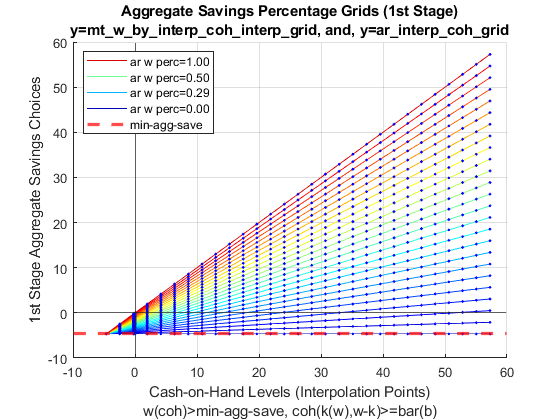
end
Graph Details, Generally do Not Run
if (bl_graph_funcgrids_detail)
Graph 1: 2nd stage coh reached by k' b' choices by index
figure('PaperPosition', [0 0 7 4]); ar_coh_kpzgrid_unique = unique(sort(mt_coh_wkb_full(:))); scatter(1:length(ar_coh_kpzgrid_unique), ar_coh_kpzgrid_unique); xline(0); yline(0); title('Cash-on-Hand given w(k+b),k,z'); ylabel('Cash-on-Hand (y=ar\_coh\_kpzgrid\_unique)'); xlabel({'Index of Cash-on-Hand Discrete Point' 'x = 1:length(ar\_coh\_kpzgrid\_unique)'}); grid on;
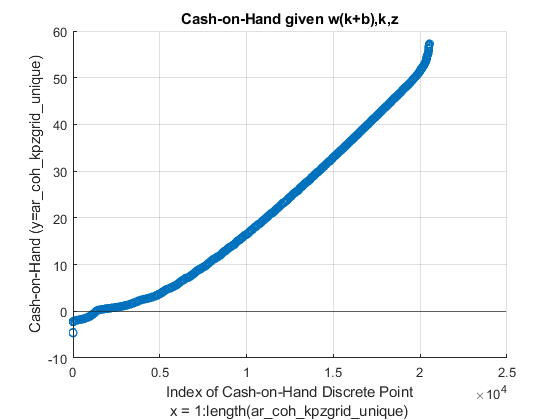
Graph 2: 2nd stage coh reached by k' b' choices by coh
figure('PaperPosition', [0 0 7 4]); ar_coh_kpzgrid_unique = unique(sort(mt_coh_wkb_full(:))); scatter(ar_coh_kpzgrid_unique, ar_coh_kpzgrid_unique, '.'); xline(0); yline(0); title('Cash-on-Hand given w(k+b),k,z; See Clearly Sparsity Density of Grid across Z'); ylabel('Cash-on-Hand (y = ar\_coh\_kpzgrid\_unique)'); xlabel({'Cash-on-Hand' 'x = ar\_coh\_kpzgrid\_unique'}); grid on;
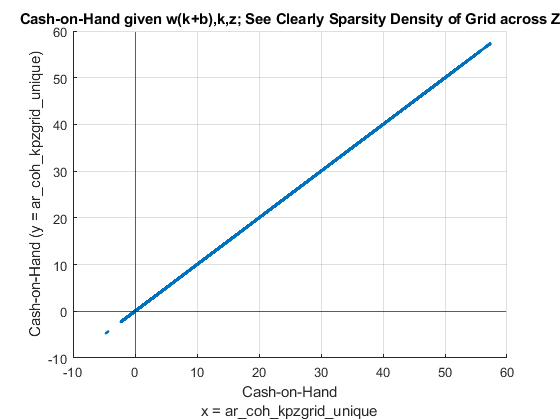
end
Display
if (bl_display_funcgrids) disp('----------------------------------------'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp('ar_z_wage'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp(size(ar_z_wage)); disp(ar_z_wage); disp('----------------------------------------'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp('ar_w_level_full'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp(size(ar_w_level_full)); disp(ar_w_level_full); disp('----------------------------------------'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp('mt_z_trans'); disp('xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx'); disp(size(mt_z_trans)); disp(head(array2table(mt_z_trans), 10)); disp(tail(array2table(mt_z_trans), 10)); end
---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ar_z_wage xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx 1 11 Columns 1 through 7 0.3466 0.4234 0.5171 0.6316 0.7715 0.9423 1.1509 Columns 8 through 11 1.4057 1.7169 2.0970 2.5613 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ar_w_level_full xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx 1 27 Columns 1 through 7 -4.6700 -2.4832 -0.2964 0 1.8904 4.0772 6.2640 Columns 8 through 14 8.4508 10.6376 12.8244 15.0112 17.1980 19.3848 21.5716 Columns 15 through 21 23.7584 25.9452 28.1320 30.3188 32.5056 34.6924 36.8792 Columns 22 through 27 39.0660 41.2528 43.4396 45.6264 47.8132 50.0000 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx mt_z_trans xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx 33 33 mt_z_trans1 mt_z_trans2 mt_z_trans3 mt_z_trans4 mt_z_trans5 mt_z_trans6 mt_z_trans7 mt_z_trans8 mt_z_trans9 mt_z_trans10 mt_z_trans11 mt_z_trans12 mt_z_trans13 mt_z_trans14 mt_z_trans15 mt_z_trans16 mt_z_trans17 mt_z_trans18 mt_z_trans19 mt_z_trans20 mt_z_trans21 mt_z_trans22 mt_z_trans23 mt_z_trans24 mt_z_trans25 mt_z_trans26 mt_z_trans27 mt_z_trans28 mt_z_trans29 mt_z_trans30 mt_z_trans31 mt_z_trans32 mt_z_trans33 ___________ ___________ ___________ ___________ ___________ ___________ ___________ ___________ ___________ ____________ ____________ ____________ ____________ ____________ ____________ ____________ ____________ ____________ ____________ ____________ ____________ ____________ ____________ ____________ ____________ ____________ ____________ ____________ ____________ ____________ ____________ ____________ ____________ 0.0013961 0.0017327 0.0010938 0.0002742 2.7045e-05 1.0372e-06 1.5288e-08 8.5744e-11 1.8158e-13 1.4418e-16 0 0.027922 0.034654 0.021876 0.0054839 0.00054091 2.0745e-05 3.0576e-07 1.7149e-09 3.6315e-12 2.8836e-15 0 0.27922 0.34654 0.21876 0.054839 0.0054091 0.00020745 3.0576e-06 1.7149e-08 3.6315e-11 2.8836e-14 0 0.00043801 0.0012909 0.0017011 0.0008932 0.00018597 1.52e-05 4.819e-07 5.8589e-09 2.7059e-11 4.7123e-14 3.0644e-17 0.0087602 0.025818 0.034022 0.017864 0.0037193 0.000304 9.6379e-06 1.1718e-07 5.4117e-10 9.4246e-13 6.1288e-16 0.087602 0.25818 0.34022 0.17864 0.037193 0.00304 9.6379e-05 1.1718e-06 5.4117e-09 9.4246e-12 6.1288e-15 8.0834e-05 0.00053304 0.0014684 0.0016098 0.00070291 0.0001215 8.225e-06 2.1546e-07 2.16e-09 8.2123e-12 1.1765e-14 0.0016167 0.010661 0.029367 0.032196 0.014058 0.0024299 0.0001645 4.3092e-06 4.3201e-08 1.6425e-10 2.3531e-13 0.016167 0.10661 0.29367 0.32196 0.14058 0.024299 0.001645 4.3092e-05 4.3201e-07 1.6425e-09 2.3531e-12 8.4426e-06 0.0001215 0.00070291 0.0016098 0.0014684 0.00053304 7.6456e-05 4.2848e-06 9.2704e-08 7.6605e-10 2.3997e-12 0.00016885 0.0024299 0.014058 0.032196 0.029367 0.010661 0.0015291 8.5696e-05 1.8541e-06 1.5321e-08 4.7995e-11 0.0016885 0.024299 0.14058 0.32196 0.29367 0.10661 0.015291 0.00085696 1.8541e-05 1.5321e-07 4.7995e-10 4.8778e-07 1.52e-05 0.00018597 0.0008932 0.0017011 0.0012909 0.00038949 4.6338e-05 2.1488e-06 3.838e-08 2.62e-10 9.7556e-06 0.000304 0.0037193 0.017864 0.034022 0.025818 0.0077897 0.00092676 4.2976e-05 7.676e-07 5.2399e-09 9.7556e-05 0.00304 0.037193 0.17864 0.34022 0.25818 0.077897 0.0092676 0.00042976 7.676e-06 5.2399e-08 1.5374e-08 1.0372e-06 2.7045e-05 0.0002742 0.0010938 0.0017327 0.0010938 0.0002742 2.7045e-05 1.0372e-06 1.5374e-08 3.0748e-07 2.0745e-05 0.00054091 0.0054839 0.021876 0.034654 0.021876 0.0054839 0.00054091 2.0745e-05 3.0748e-07 3.0748e-06 0.00020745 0.0054091 0.054839 0.21876 0.34654 0.21876 0.054839 0.0054091 0.00020745 3.0748e-06 2.62e-10 3.838e-08 2.1488e-06 4.6338e-05 0.00038949 0.0012909 0.0017011 0.0008932 0.00018597 1.52e-05 4.8778e-07 5.2399e-09 7.676e-07 4.2976e-05 0.00092676 0.0077897 0.025818 0.034022 0.017864 0.0037193 0.000304 9.7556e-06 5.2399e-08 7.676e-06 0.00042976 0.0092676 0.077897 0.25818 0.34022 0.17864 0.037193 0.00304 9.7556e-05 2.3997e-12 7.6605e-10 9.2704e-08 4.2848e-06 7.6456e-05 0.00053304 0.0014684 0.0016098 0.00070291 0.0001215 8.4426e-06 4.7995e-11 1.5321e-08 1.8541e-06 8.5696e-05 0.0015291 0.010661 0.029367 0.032196 0.014058 0.0024299 0.00016885 4.7995e-10 1.5321e-07 1.8541e-05 0.00085696 0.015291 0.10661 0.29367 0.32196 0.14058 0.024299 0.0016885 1.1765e-14 8.2123e-12 2.16e-09 2.1546e-07 8.225e-06 0.0001215 0.00070291 0.0016098 0.0014684 0.00053304 8.0834e-05 2.3531e-13 1.6425e-10 4.3201e-08 4.3092e-06 0.0001645 0.0024299 0.014058 0.032196 0.029367 0.010661 0.0016167 2.3531e-12 1.6425e-09 4.3201e-07 4.3092e-05 0.001645 0.024299 0.14058 0.32196 0.29367 0.10661 0.016167 3.0784e-17 4.7123e-14 2.7059e-11 5.8589e-09 4.819e-07 1.52e-05 0.00018597 0.0008932 0.0017011 0.0012909 0.00043801 6.1568e-16 9.4246e-13 5.4117e-10 1.1718e-07 9.6379e-06 0.000304 0.0037193 0.017864 0.034022 0.025818 0.0087602 6.1568e-15 9.4246e-12 5.4117e-09 1.1718e-06 9.6379e-05 0.00304 0.037193 0.17864 0.34022 0.25818 0.087602 mt_z_trans1 mt_z_trans2 mt_z_trans3 mt_z_trans4 mt_z_trans5 mt_z_trans6 mt_z_trans7 mt_z_trans8 mt_z_trans9 mt_z_trans10 mt_z_trans11 mt_z_trans12 mt_z_trans13 mt_z_trans14 mt_z_trans15 mt_z_trans16 mt_z_trans17 mt_z_trans18 mt_z_trans19 mt_z_trans20 mt_z_trans21 mt_z_trans22 mt_z_trans23 mt_z_trans24 mt_z_trans25 mt_z_trans26 mt_z_trans27 mt_z_trans28 mt_z_trans29 mt_z_trans30 mt_z_trans31 mt_z_trans32 mt_z_trans33 ___________ ___________ ___________ ___________ ___________ ___________ ___________ ___________ ___________ ____________ ____________ ____________ ____________ ____________ ____________ ____________ ____________ ____________ ____________ ____________ ____________ ____________ ____________ ____________ ____________ ____________ ____________ ____________ ____________ ____________ ____________ ____________ ____________ 0.00043801 0.0012909 0.0017011 0.0008932 0.00018597 1.52e-05 4.819e-07 5.8589e-09 2.7059e-11 4.7123e-14 3.0644e-17 0.0087602 0.025818 0.034022 0.017864 0.0037193 0.000304 9.6379e-06 1.1718e-07 5.4117e-10 9.4246e-13 6.1288e-16 0.087602 0.25818 0.34022 0.17864 0.037193 0.00304 9.6379e-05 1.1718e-06 5.4117e-09 9.4246e-12 6.1288e-15 8.0834e-05 0.00053304 0.0014684 0.0016098 0.00070291 0.0001215 8.225e-06 2.1546e-07 2.16e-09 8.2123e-12 1.1765e-14 0.0016167 0.010661 0.029367 0.032196 0.014058 0.0024299 0.0001645 4.3092e-06 4.3201e-08 1.6425e-10 2.3531e-13 0.016167 0.10661 0.29367 0.32196 0.14058 0.024299 0.001645 4.3092e-05 4.3201e-07 1.6425e-09 2.3531e-12 8.4426e-06 0.0001215 0.00070291 0.0016098 0.0014684 0.00053304 7.6456e-05 4.2848e-06 9.2704e-08 7.6605e-10 2.3997e-12 0.00016885 0.0024299 0.014058 0.032196 0.029367 0.010661 0.0015291 8.5696e-05 1.8541e-06 1.5321e-08 4.7995e-11 0.0016885 0.024299 0.14058 0.32196 0.29367 0.10661 0.015291 0.00085696 1.8541e-05 1.5321e-07 4.7995e-10 4.8778e-07 1.52e-05 0.00018597 0.0008932 0.0017011 0.0012909 0.00038949 4.6338e-05 2.1488e-06 3.838e-08 2.62e-10 9.7556e-06 0.000304 0.0037193 0.017864 0.034022 0.025818 0.0077897 0.00092676 4.2976e-05 7.676e-07 5.2399e-09 9.7556e-05 0.00304 0.037193 0.17864 0.34022 0.25818 0.077897 0.0092676 0.00042976 7.676e-06 5.2399e-08 1.5374e-08 1.0372e-06 2.7045e-05 0.0002742 0.0010938 0.0017327 0.0010938 0.0002742 2.7045e-05 1.0372e-06 1.5374e-08 3.0748e-07 2.0745e-05 0.00054091 0.0054839 0.021876 0.034654 0.021876 0.0054839 0.00054091 2.0745e-05 3.0748e-07 3.0748e-06 0.00020745 0.0054091 0.054839 0.21876 0.34654 0.21876 0.054839 0.0054091 0.00020745 3.0748e-06 2.62e-10 3.838e-08 2.1488e-06 4.6338e-05 0.00038949 0.0012909 0.0017011 0.0008932 0.00018597 1.52e-05 4.8778e-07 5.2399e-09 7.676e-07 4.2976e-05 0.00092676 0.0077897 0.025818 0.034022 0.017864 0.0037193 0.000304 9.7556e-06 5.2399e-08 7.676e-06 0.00042976 0.0092676 0.077897 0.25818 0.34022 0.17864 0.037193 0.00304 9.7556e-05 2.3997e-12 7.6605e-10 9.2704e-08 4.2848e-06 7.6456e-05 0.00053304 0.0014684 0.0016098 0.00070291 0.0001215 8.4426e-06 4.7995e-11 1.5321e-08 1.8541e-06 8.5696e-05 0.0015291 0.010661 0.029367 0.032196 0.014058 0.0024299 0.00016885 4.7995e-10 1.5321e-07 1.8541e-05 0.00085696 0.015291 0.10661 0.29367 0.32196 0.14058 0.024299 0.0016885 1.1765e-14 8.2123e-12 2.16e-09 2.1546e-07 8.225e-06 0.0001215 0.00070291 0.0016098 0.0014684 0.00053304 8.0834e-05 2.3531e-13 1.6425e-10 4.3201e-08 4.3092e-06 0.0001645 0.0024299 0.014058 0.032196 0.029367 0.010661 0.0016167 2.3531e-12 1.6425e-09 4.3201e-07 4.3092e-05 0.001645 0.024299 0.14058 0.32196 0.29367 0.10661 0.016167 3.0784e-17 4.7123e-14 2.7059e-11 5.8589e-09 4.819e-07 1.52e-05 0.00018597 0.0008932 0.0017011 0.0012909 0.00043801 6.1568e-16 9.4246e-13 5.4117e-10 1.1718e-07 9.6379e-06 0.000304 0.0037193 0.017864 0.034022 0.025818 0.0087602 6.1568e-15 9.4246e-12 5.4117e-09 1.1718e-06 9.6379e-05 0.00304 0.037193 0.17864 0.34022 0.25818 0.087602 4.2894e-20 1.4434e-16 1.8158e-13 8.5744e-11 1.5288e-08 1.0372e-06 2.7045e-05 0.0002742 0.0010938 0.0017327 0.0013961 8.5788e-19 2.8868e-15 3.6315e-12 1.7149e-09 3.0576e-07 2.0745e-05 0.00054091 0.0054839 0.021876 0.034654 0.027922 8.5788e-18 2.8868e-14 3.6315e-11 1.7149e-08 3.0576e-06 0.00020745 0.0054091 0.054839 0.21876 0.34654 0.27922
Display
if (bl_display_funcgrids) fft_container_map_display(armt_map, it_display_summmat_rowmax, it_display_summmat_colmax); fft_container_map_display(func_map, it_display_summmat_rowmax, it_display_summmat_colmax); end
---------------------------------------- ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Begin: Show all key and value pairs from container CONTAINER NAME: ARMT_MAP ---------------------------------------- Map with properties: Count: 31 KeyType: char ValueType: any xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ---------------------------------------- ---------------------------------------- pos = 1 ; key = ar_a_meshk ;rown= 1215 ,coln= 1 ar_a_meshk :mu= 8.5778 ,sd= 12.4414 ,min= -4.67 ,max= 50 zi_1_c1 _______ zi_1_R1 -4.67 zi_2_R2 -4.67 zi_3_R3 -4.67 zi_608_R608 8.4508 zi_1213_r1213 -2.185 zi_1214_r1214 -3.4275 zi_1215_r1215 -4.67 pos = 2 ; key = ar_ak_perc ;rown= 1 ,coln= 45 ar_ak_perc :mu= 0.5 ,sd= 0.2985 ,min= 0 ,max= 1 zi_1_C1 zi_2_C2 zi_3_C3 zi_23_c23 zi_43_c43 zi_44_c44 zi_45_c45 _______ ________ ________ _________ _________ _________ _________ zi_1_r1 0 0.022727 0.045455 0.5 0.95455 0.97727 1 pos = 3 ; key = ar_aplusk_mesh ;rown= 1215 ,coln= 1 ar_aplusk_mesh :mu= 21.8256 ,sd= 16.6607 ,min= -4.67 ,max= 50 zi_1_c1 _______ zi_1_R1 -4.67 zi_2_R2 -4.67 zi_3_R3 -4.67 zi_608_R608 21.572 zi_1213_r1213 50 zi_1214_r1214 50 zi_1215_r1215 50 pos = 4 ; key = ar_interp_c_grid ;rown= 1 ,coln= 619078 ar_interp_c_grid :mu= 30.9739 ,sd= 17.8713 ,min= 0.02 ,max= 61.9279 zi_1_C1 zi_2_C2 zi_3_C3 zi_309539_c309539 zi_619076_c619076 zi_619077_c619077 zi_619078_c619078 _______ _______ _______ _________________ _________________ _________________ _________________ zi_1_r1 0.02 0.0201 0.0202 30.974 61.928 61.928 61.928 pos = 5 ; key = ar_interp_coh_grid ;rown= 1 ,coln= 30 ar_interp_coh_grid :mu= 25.4175 ,sd= 19.1171 ,min= -4.67 ,max= 57.2579 zi_1_C1 zi_2_C2 zi_3_C3 zi_15_c15 zi_28_c28 zi_29_c29 zi_30_c30 _______ _______ ________ _________ _________ _________ _________ zi_1_r1 -4.67 -2.4583 -0.24656 24.082 52.834 55.046 57.258 pos = 6 ; key = ar_k_mesha ;rown= 1215 ,coln= 1 ar_k_mesha :mu= 13.2478 ,sd= 12.4414 ,min= 0 ,max= 54.67 zi_1_c1 _______ zi_1_R1 0 zi_2_R2 0 zi_3_R3 0 zi_608_R608 13.121 zi_1213_r1213 52.185 zi_1214_r1214 53.427 zi_1215_r1215 54.67 pos = 7 ; key = ar_w_level ;rown= 1 ,coln= 27 ar_w_level :mu= 21.8256 ,sd= 16.9711 ,min= -4.67 ,max= 50 zi_1_C1 zi_2_C2 zi_3_C3 zi_14_c14 zi_25_c25 zi_26_c26 zi_27_c27 _______ _______ ________ _________ _________ _________ _________ zi_1_r1 -4.67 -2.4832 -0.29638 21.572 45.626 47.813 50 pos = 8 ; key = ar_w_perc ;rown= 1 ,coln= 25 ar_w_perc :mu= 0.5 ,sd= 0.30666 ,min= 0 ,max= 1 zi_1_C1 zi_2_C2 zi_3_C3 zi_13_c13 zi_23_c23 zi_24_c24 zi_25_c25 _______ ________ ________ _________ _________ _________ _________ zi_1_r1 0 0.041667 0.083333 0.5 0.91667 0.95833 1 pos = 9 ; key = ar_z_r_borr ;rown= 1 ,coln= 3 ar_z_r_borr :mu= 0.06 ,sd= 0.035 ,min= 0.025 ,max= 0.095 zi_1_c1 zi_2_c2 zi_3_c3 _______ _______ _______ zi_1_r1 0.025 0.06 0.095 pos = 10 ; key = ar_z_r_borr_mesh_wage_r1w2 ;rown= 1 ,coln= 33 ar_z_r_borr_mesh_wage_r1w2 :mu= 0.06 ,sd= 0.02902 ,min= 0.025 ,max= 0.095 zi_1_C1 zi_2_C2 zi_3_C3 zi_17_c17 zi_31_c31 zi_32_c32 zi_33_c33 _______ _______ _______ _________ _________ _________ _________ zi_1_r1 0.025 0.06 0.095 0.06 0.025 0.06 0.095 pos = 11 ; key = ar_z_r_borr_mesh_wage_w1r2 ;rown= 1 ,coln= 33 ar_z_r_borr_mesh_wage_w1r2 :mu= 0.06 ,sd= 0.02902 ,min= 0.025 ,max= 0.095 zi_1_C1 zi_2_C2 zi_3_C3 zi_17_c17 zi_31_c31 zi_32_c32 zi_33_c33 _______ _______ _______ _________ _________ _________ _________ zi_1_r1 0.025 0.025 0.025 0.06 0.095 0.095 0.095 pos = 12 ; key = ar_z_r_borr_prob ;rown= 1 ,coln= 3 ar_z_r_borr_prob :mu= 0.33333 ,sd= 0.49692 ,min= 0.0045249 ,max= 0.90498 zi_1_c1 zi_2_c2 zi_3_c3 _________ ________ _______ zi_1_r1 0.0045249 0.090498 0.90498 pos = 13 ; key = ar_z_wage ;rown= 1 ,coln= 11 ar_z_wage :mu= 1.1422 ,sd= 0.72828 ,min= 0.34664 ,max= 2.5613 zi_1_C1 zi_2_C2 zi_3_C3 zi_6_C6 zi_9_C9 zi_10_c10 zi_11_c11 _______ _______ _______ _______ _______ _________ _________ zi_1_r1 0.34664 0.42338 0.51712 0.94226 1.7169 2.097 2.5613 pos = 14 ; key = ar_z_wage_mesh_r_borr_r1w2 ;rown= 1 ,coln= 33 ar_z_wage_mesh_r_borr_r1w2 :mu= 1.1422 ,sd= 0.70515 ,min= 0.34664 ,max= 2.5613 zi_1_C1 zi_2_C2 zi_3_C3 zi_17_c17 zi_31_c31 zi_32_c32 zi_33_c33 _______ _______ _______ _________ _________ _________ _________ zi_1_r1 0.34664 0.34664 0.34664 0.94226 2.5613 2.5613 2.5613 pos = 15 ; key = ar_z_wage_mesh_r_borr_w1r2 ;rown= 1 ,coln= 33 ar_z_wage_mesh_r_borr_w1r2 :mu= 1.1422 ,sd= 0.70515 ,min= 0.34664 ,max= 2.5613 zi_1_C1 zi_2_C2 zi_3_C3 zi_17_c17 zi_31_c31 zi_32_c32 zi_33_c33 _______ _______ _______ _________ _________ _________ _________ zi_1_r1 0.34664 0.42338 0.51712 0.94226 1.7169 2.097 2.5613 pos = 16 ; key = ar_z_wage_prob ;rown= 1 ,coln= 11 ar_z_wage_prob :mu= 0.090909 ,sd= 0.084822 ,min= 0.0039324 ,max= 0.23011 zi_1_C1 zi_2_C2 zi_3_C3 zi_6_C6 zi_9_C9 zi_10_c10 zi_11_c11 _________ ________ ________ _______ ________ _________ _________ zi_1_r1 0.0039324 0.016056 0.051615 0.23011 0.051615 0.016056 0.0039324 pos = 17 ; key = cl_mt_coh_wkb_mesh_z_r_borr ;rown= 1215 ,coln= 33 cl_mt_coh_wkb_mesh_z_r_borr :mu= 23.9244 ,sd= 17.0645 ,min= -4.3431 ,max= 57.2579 zi_1_C1 zi_2_C2 zi_3_C3 zi_17_c17 zi_31_c31 zi_32_c32 zi_33_c33 _______ _______ _______ _________ _________ _________ _________ zi_1_R1 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 zi_2_R2 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 zi_3_R3 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 zi_608_R608 22.053 22.246 22.483 23.557 25.514 26.474 27.647 zi_1213_r1213 47.654 47.972 48.362 50.127 53.344 54.922 56.85 zi_1214_r1214 47.535 47.857 48.249 50.03 53.274 54.866 56.81 zi_1215_r1215 47.417 47.741 48.137 49.932 53.203 54.808 56.769 pos = 18 ; key = it_ameshk_n ; val = 1215 pos = 19 ; key = mt_coh_wkb ;rown= 3645 ,coln= 11 mt_coh_wkb :mu= 23.8912 ,sd= 17.0935 ,min= -4.67 ,max= 57.2579 zi_1_C1 zi_2_C2 zi_3_C3 zi_6_C6 zi_9_C9 zi_10_c10 zi_11_c11 _______ _______ _______ _______ _______ _________ _________ zi_1_R1 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 zi_2_R2 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 zi_3_R3 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 zi_1823_r1823 22.053 22.246 22.483 23.557 25.514 26.474 27.647 zi_3643_r3643 47.501 47.819 48.209 49.974 53.191 54.769 56.697 zi_3644_r3644 47.296 47.617 48.009 49.79 53.034 54.626 56.57 zi_3645_r3645 47.09 47.414 47.81 49.605 52.876 54.482 56.442 pos = 20 ; key = mt_coh_wkb_mesh_z_r_borr ;rown= 3645 ,coln= 33 mt_coh_wkb_mesh_z_r_borr :mu= 23.8912 ,sd= 17.0933 ,min= -4.67 ,max= 57.2579 zi_1_C1 zi_2_C2 zi_3_C3 zi_17_c17 zi_31_c31 zi_32_c32 zi_33_c33 _______ _______ _______ _________ _________ _________ _________ zi_1_R1 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 zi_2_R2 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 zi_3_R3 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 -4.3431 zi_1823_r1823 22.053 22.246 22.483 23.557 25.514 26.474 27.647 zi_3643_r3643 47.501 47.819 48.209 49.974 53.191 54.769 56.697 zi_3644_r3644 47.296 47.617 48.009 49.79 53.034 54.626 56.57 zi_3645_r3645 47.09 47.414 47.81 49.605 52.876 54.482 56.442 pos = 21 ; key = mt_interp_coh_grid_mesh_w_perc ;rown= 25 ,coln= 30 mt_interp_coh_grid_mesh_w_perc :mu= 25.4175 ,sd= 18.8083 ,min= -4.67 ,max= 57.2579 zi_1_C1 zi_2_C2 zi_3_C3 zi_15_c15 zi_28_c28 zi_29_c29 zi_30_c30 _______ _______ ________ _________ _________ _________ _________ zi_1_R1 -4.67 -2.4583 -0.24656 24.082 52.834 55.046 57.258 zi_2_R2 -4.67 -2.4583 -0.24656 24.082 52.834 55.046 57.258 zi_3_R3 -4.67 -2.4583 -0.24656 24.082 52.834 55.046 57.258 zi_13_r13 -4.67 -2.4583 -0.24656 24.082 52.834 55.046 57.258 zi_23_r23 -4.67 -2.4583 -0.24656 24.082 52.834 55.046 57.258 zi_24_r24 -4.67 -2.4583 -0.24656 24.082 52.834 55.046 57.258 zi_25_r25 -4.67 -2.4583 -0.24656 24.082 52.834 55.046 57.258 pos = 22 ; key = mt_interp_coh_grid_mesh_z ;rown= 30 ,coln= 33 mt_interp_coh_grid_mesh_z :mu= 25.4175 ,sd= 18.8052 ,min= -4.67 ,max= 57.2579 zi_1_C1 zi_2_C2 zi_3_C3 zi_17_c17 zi_31_c31 zi_32_c32 zi_33_c33 ________ ________ ________ _________ _________ _________ _________ zi_1_R1 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 zi_2_R2 -2.4583 -2.4583 -2.4583 -2.4583 -2.4583 -2.4583 -2.4583 zi_3_R3 -0.24656 -0.24656 -0.24656 -0.24656 -0.24656 -0.24656 -0.24656 zi_15_r15 24.082 24.082 24.082 24.082 24.082 24.082 24.082 zi_28_r28 52.834 52.834 52.834 52.834 52.834 52.834 52.834 zi_29_r29 55.046 55.046 55.046 55.046 55.046 55.046 55.046 zi_30_r30 57.258 57.258 57.258 57.258 57.258 57.258 57.258 pos = 23 ; key = mt_interp_coh_grid_mesh_z_wage ;rown= 30 ,coln= 11 mt_interp_coh_grid_mesh_z_wage :mu= 25.4175 ,sd= 18.8243 ,min= -4.67 ,max= 57.2579 zi_1_C1 zi_2_C2 zi_3_C3 zi_6_C6 zi_9_C9 zi_10_c10 zi_11_c11 ________ ________ ________ ________ ________ _________ _________ zi_1_R1 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 zi_2_R2 -2.4583 -2.4583 -2.4583 -2.4583 -2.4583 -2.4583 -2.4583 zi_3_R3 -0.24656 -0.24656 -0.24656 -0.24656 -0.24656 -0.24656 -0.24656 zi_15_r15 24.082 24.082 24.082 24.082 24.082 24.082 24.082 zi_28_r28 52.834 52.834 52.834 52.834 52.834 52.834 52.834 zi_29_r29 55.046 55.046 55.046 55.046 55.046 55.046 55.046 zi_30_r30 57.258 57.258 57.258 57.258 57.258 57.258 57.258 pos = 24 ; key = mt_k ;rown= 45 ,coln= 27 mt_k :mu= 13.2478 ,sd= 12.4414 ,min= 0 ,max= 54.67 zi_1_C1 zi_2_C2 zi_3_C3 zi_14_c14 zi_25_c25 zi_26_c26 zi_27_c27 _______ _______ _______ _________ _________ _________ _________ zi_1_R1 0 0 0 0 0 0 0 zi_2_R2 0 0.0497 0.0994 0.5964 1.1431 1.1928 1.2425 zi_3_R3 0 0.0994 0.1988 1.1928 2.2862 2.3856 2.485 zi_23_r23 0 1.0934 2.1868 13.121 25.148 26.242 27.335 zi_43_r43 0 2.0874 4.1748 25.049 48.01 50.098 52.185 zi_44_r44 0 2.1371 4.2742 25.645 49.153 51.29 53.427 zi_45_r45 0 2.1868 4.3736 26.242 50.296 52.483 54.67 pos = 25 ; key = mt_w_perc_mesh_interp_coh_grid ;rown= 25 ,coln= 30 mt_w_perc_mesh_interp_coh_grid :mu= 10.3738 ,sd= 14.22 ,min= -4.67 ,max= 57.2579 zi_1_C1 zi_2_C2 zi_3_C3 zi_15_c15 zi_28_c28 zi_29_c29 zi_30_c30 _______ _______ ________ _________ _________ _________ _________ zi_1_R1 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 -4.67 zi_2_R2 -4.67 -4.5778 -4.4857 -3.472 -2.274 -2.1818 -2.0897 zi_3_R3 -4.67 -4.4857 -4.3014 -2.274 0.12206 0.30637 0.49068 zi_13_r13 -4.67 -3.5641 -2.4583 9.7061 24.082 25.188 26.294 zi_23_r23 -4.67 -2.6426 -0.61518 21.686 48.042 50.07 52.097 zi_24_r24 -4.67 -2.5504 -0.43087 22.884 50.438 52.558 54.678 zi_25_r25 -4.67 -2.4583 -0.24656 24.082 52.834 55.046 57.258 pos = 26 ; key = mt_z_mesh_coh_wkb ;rown= 3645 ,coln= 33 mt_z_mesh_coh_wkb :mu= 17 ,sd= 9.5219 ,min= 1 ,max= 33 zi_1_C1 zi_2_C2 zi_3_C3 zi_17_c17 zi_31_c31 zi_32_c32 zi_33_c33 _______ _______ _______ _________ _________ _________ _________ zi_1_R1 1 2 3 17 31 32 33 zi_2_R2 1 2 3 17 31 32 33 zi_3_R3 1 2 3 17 31 32 33 zi_1823_r1823 1 2 3 17 31 32 33 zi_3643_r3643 1 2 3 17 31 32 33 zi_3644_r3644 1 2 3 17 31 32 33 zi_3645_r3645 1 2 3 17 31 32 33 pos = 27 ; key = mt_z_mesh_coh_wkb_seg ;rown= 1215 ,coln= 33 mt_z_mesh_coh_wkb_seg :mu= 17 ,sd= 9.522 ,min= 1 ,max= 33 zi_1_C1 zi_2_C2 zi_3_C3 zi_17_c17 zi_31_c31 zi_32_c32 zi_33_c33 _______ _______ _______ _________ _________ _________ _________ zi_1_R1 1 2 3 17 31 32 33 zi_2_R2 1 2 3 17 31 32 33 zi_3_R3 1 2 3 17 31 32 33 zi_608_R608 1 2 3 17 31 32 33 zi_1213_r1213 1 2 3 17 31 32 33 zi_1214_r1214 1 2 3 17 31 32 33 zi_1215_r1215 1 2 3 17 31 32 33 pos = 28 ; key = mt_z_mesh_interp_coh_grid ;rown= 30 ,coln= 33 mt_z_mesh_interp_coh_grid :mu= 17 ,sd= 9.5267 ,min= 1 ,max= 33 zi_1_C1 zi_2_C2 zi_3_C3 zi_17_c17 zi_31_c31 zi_32_c32 zi_33_c33 _______ _______ _______ _________ _________ _________ _________ zi_1_R1 1 2 3 17 31 32 33 zi_2_R2 1 2 3 17 31 32 33 zi_3_R3 1 2 3 17 31 32 33 zi_15_r15 1 2 3 17 31 32 33 zi_28_r28 1 2 3 17 31 32 33 zi_29_r29 1 2 3 17 31 32 33 zi_30_r30 1 2 3 17 31 32 33 pos = 29 ; key = mt_z_trans ;rown= 33 ,coln= 33 mt_z_trans :mu= 0.030303 ,sd= 0.077699 ,min= 0 ,max= 0.34654 zi_1_C1 zi_2_C2 zi_3_C3 zi_17_c17 zi_31_c31 zi_32_c32 zi_33_c33 __________ __________ __________ __________ __________ __________ __________ zi_1_R1 0.0013961 0.0017327 0.0010938 2.0745e-05 3.6315e-11 2.8836e-14 0 zi_2_R2 0.00043801 0.0012909 0.0017011 0.000304 5.4117e-09 9.4246e-12 6.1288e-15 zi_3_R3 8.0834e-05 0.00053304 0.0014684 0.0024299 4.3201e-07 1.6425e-09 2.3531e-12 zi_17_r17 1.5374e-08 1.0372e-06 2.7045e-05 0.034654 0.0054091 0.00020745 3.0748e-06 zi_31_r31 1.1765e-14 8.2123e-12 2.16e-09 0.0024299 0.29367 0.10661 0.016167 zi_32_r32 3.0784e-17 4.7123e-14 2.7059e-11 0.000304 0.34022 0.25818 0.087602 zi_33_r33 4.2894e-20 1.4434e-16 1.8158e-13 2.0745e-05 0.21876 0.34654 0.27922 pos = 30 ; key = mt_z_wage_mesh_coh_wkb ;rown= 3645 ,coln= 11 mt_z_wage_mesh_coh_wkb :mu= 1.1422 ,sd= 0.69439 ,min= 0.34664 ,max= 2.5613 zi_1_C1 zi_2_C2 zi_3_C3 zi_6_C6 zi_9_C9 zi_10_c10 zi_11_c11 _______ _______ _______ _______ _______ _________ _________ zi_1_R1 0.34664 0.42338 0.51712 0.94226 1.7169 2.097 2.5613 zi_2_R2 0.34664 0.42338 0.51712 0.94226 1.7169 2.097 2.5613 zi_3_R3 0.34664 0.42338 0.51712 0.94226 1.7169 2.097 2.5613 zi_1823_r1823 0.34664 0.42338 0.51712 0.94226 1.7169 2.097 2.5613 zi_3643_r3643 0.34664 0.42338 0.51712 0.94226 1.7169 2.097 2.5613 zi_3644_r3644 0.34664 0.42338 0.51712 0.94226 1.7169 2.097 2.5613 zi_3645_r3645 0.34664 0.42338 0.51712 0.94226 1.7169 2.097 2.5613 pos = 31 ; key = mt_z_wage_mesh_interp_coh_grid ;rown= 30 ,coln= 11 mt_z_wage_mesh_interp_coh_grid :mu= 1.1422 ,sd= 0.69544 ,min= 0.34664 ,max= 2.5613 zi_1_C1 zi_2_C2 zi_3_C3 zi_6_C6 zi_9_C9 zi_10_c10 zi_11_c11 _______ _______ _______ _______ _______ _________ _________ zi_1_R1 0.34664 0.42338 0.51712 0.94226 1.7169 2.097 2.5613 zi_2_R2 0.34664 0.42338 0.51712 0.94226 1.7169 2.097 2.5613 zi_3_R3 0.34664 0.42338 0.51712 0.94226 1.7169 2.097 2.5613 zi_15_r15 0.34664 0.42338 0.51712 0.94226 1.7169 2.097 2.5613 zi_28_r28 0.34664 0.42338 0.51712 0.94226 1.7169 2.097 2.5613 zi_29_r29 0.34664 0.42338 0.51712 0.94226 1.7169 2.097 2.5613 zi_30_r30 0.34664 0.42338 0.51712 0.94226 1.7169 2.097 2.5613 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Matrix in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx rowN colN mean std min max __ ___ ____ __________ ________ ________ _________ _______ ar_a_meshk 1 1 1215 1 8.5778 12.441 -4.67 50 ar_ak_perc 2 2 1 45 0.5 0.2985 0 1 ar_aplusk_mesh 3 3 1215 1 21.826 16.661 -4.67 50 ar_interp_c_grid 4 4 1 6.1908e+05 30.974 17.871 0.02 61.928 ar_interp_coh_grid 5 5 1 30 25.418 19.117 -4.67 57.258 ar_k_mesha 6 6 1215 1 13.248 12.441 0 54.67 ar_w_level 7 7 1 27 21.826 16.971 -4.67 50 ar_w_perc 8 8 1 25 0.5 0.30666 0 1 ar_z_r_borr 9 9 1 3 0.06 0.035 0.025 0.095 ar_z_r_borr_mesh_wage_r1w2 10 10 1 33 0.06 0.02902 0.025 0.095 ar_z_r_borr_mesh_wage_w1r2 11 11 1 33 0.06 0.02902 0.025 0.095 ar_z_r_borr_prob 12 12 1 3 0.33333 0.49692 0.0045249 0.90498 ar_z_wage 13 13 1 11 1.1422 0.72828 0.34664 2.5613 ar_z_wage_mesh_r_borr_r1w2 14 14 1 33 1.1422 0.70515 0.34664 2.5613 ar_z_wage_mesh_r_borr_w1r2 15 15 1 33 1.1422 0.70515 0.34664 2.5613 ar_z_wage_prob 16 16 1 11 0.090909 0.084822 0.0039324 0.23011 cl_mt_coh_wkb_mesh_z_r_borr 17 17 1215 33 23.924 17.064 -4.3431 57.258 mt_coh_wkb 18 19 3645 11 23.891 17.093 -4.67 57.258 mt_coh_wkb_mesh_z_r_borr 19 20 3645 33 23.891 17.093 -4.67 57.258 mt_interp_coh_grid_mesh_w_perc 20 21 25 30 25.418 18.808 -4.67 57.258 mt_interp_coh_grid_mesh_z 21 22 30 33 25.418 18.805 -4.67 57.258 mt_interp_coh_grid_mesh_z_wage 22 23 30 11 25.418 18.824 -4.67 57.258 mt_k 23 24 45 27 13.248 12.441 0 54.67 mt_w_perc_mesh_interp_coh_grid 24 25 25 30 10.374 14.22 -4.67 57.258 mt_z_mesh_coh_wkb 25 26 3645 33 17 9.5219 1 33 mt_z_mesh_coh_wkb_seg 26 27 1215 33 17 9.522 1 33 mt_z_mesh_interp_coh_grid 27 28 30 33 17 9.5267 1 33 mt_z_trans 28 29 33 33 0.030303 0.077699 0 0.34654 mt_z_wage_mesh_coh_wkb 29 30 3645 11 1.1422 0.69439 0.34664 2.5613 mt_z_wage_mesh_interp_coh_grid 30 31 30 11 1.1422 0.69544 0.34664 2.5613 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Scalars in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx value _ ___ _____ it_ameshk_n 1 18 1215 ---------------------------------------- ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Begin: Show all key and value pairs from container CONTAINER NAME: FUNC_MAP ---------------------------------------- Map with properties: Count: 8 KeyType: char ValueType: any xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ---------------------------------------- ---------------------------------------- pos = 1 ; key = f_coh ; val = @(fl_r_borr,z,b,k)(f_prod(z,k)+k*(1-fl_delta)+fl_w+b.*(1+fl_r_save).*(b>0)+b.*(1+fl_r_borr).*(b<=0)) pos = 2 ; key = f_cons ; val = @(coh,bprime,kprime)(coh-kprime-bprime) pos = 3 ; key = f_inc ; val = @(fl_r_borr,z,b,k)(f_prod(z,k)-(fl_delta)*k+fl_w+b.*(fl_r_save).*(b>0)+b.*(fl_r_borr).*(b<=0)) pos = 4 ; key = f_prod ; val = @(z,k)((fl_Amean.*(z)).*(k.^(fl_alpha))) pos = 5 ; key = f_util_crra ; val = @(c)(((c).^(1-fl_crra)-1)./(1-fl_crra)) pos = 6 ; key = f_util_log ; val = @(c)log(c) pos = 7 ; key = f_util_standin ; val = @(fl_r_borr,z,b,k)f_util_log((f_coh(fl_r_borr,z,b,k)-fl_b_bd).*((f_coh(fl_r_borr,z,b,k)-fl_b_bd)>fl_c_min)+fl_c_min.*((f_coh(fl_r_borr,z,b,k)-fl_b_bd)<=fl_c_min)) pos = 8 ; key = f_util_standin_coh ; val = @(coh,fl_r_borr)f_util_log((coh-fl_b_bd).*((coh>0)&(((coh-fl_b_bd)./(1))>fl_c_min))+((coh-fl_b_bd)./(1)).*((coh<=0)&(((coh-fl_b_bd)./(1))>fl_c_min))+(fl_c_min./(1+fl_r_borr)).*((((coh-fl_b_bd)./(1))<=fl_c_min))) ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Scalars in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx xFunction _ ___ _________ f_coh 1 1 1 f_cons 2 2 2 f_inc 3 3 3 f_prod 4 4 4 f_util_crra 5 5 5 f_util_log 6 6 6 f_util_standin 7 7 7 f_util_standin_coh 8 8 8
end
ans = Map with properties: Count: 31 KeyType: char ValueType: any