Solve For+Inf+Borr+Save Dynamic Programming Problem (Optimized-Vectorized)
back to Fan's Dynamic Assets Repository Table of Content.
Contents
- FF_ABZ_FIBS_VF_VECSV solve infinite horizon exo shock + endo asset problem
- Default
- Parse Parameters 1
- Parse Parameters 2
- Initialize Output Matrixes
- Initialize Convergence Conditions
- Iterate Value Function
- Solve Optimization Problem Current Iteration
- Solve the Formal Informal Problem for each a' and coh: c_forinf(a')
- Update Consumption Matrix CASE A + B + C Consumptions
- Evaluate u(c) and store
- Solve Optimization Problem: max_{a'} (u(c_forinf(a')) + EV(a',z'))
- Check Tolerance and Continuation
- Process Optimal Choices
- Post Solution Graph and Table Generation
- Display Various Containers
- Display 1 support_map
- Display 2 armt_map
- Display 3 param_map
- Display 4 func_map
- Display 5 result_map
function result_map = ff_abz_fibs_vf_vecsv(varargin)
FF_ABZ_FIBS_VF_VECSV solve infinite horizon exo shock + endo asset problem
The model could be invoked mainly in sveral ways:
- param_map('bl_default') = true; param_map('bl_bridge') = false; param_map('bl_rollover') = true; Given these, default is possible, bridge loans are not needed because rollover is allowed for formal loans (or informal loans)
- we change param_map('bl_bridge') = true, that means rollover is still allowed, but only allowed using informal sources, formal loans no longer allow for roll-over. Furthermore, if both bl_bridge and bl_rollover are false, that means we are not allowing for rollover at all, so households can not borrow such that they end up with negative cash-on-hand.
Default simulation bl_bridge = false.
@param param_map container parameter container
@param support_map container support container
@param armt_map container container with states, choices and shocks grids that are inputs for grid based solution algorithm
@param func_map container container with function handles for consumption cash-on-hand etc.
@return result_map container contains policy function matrix, value function matrix, iteration results, and policy function, value function and iteration results tables.
@example
% Get Default Parameters it_param_set = 4; [param_map, support_map] = ffs_abz_fibs_set_default_param(it_param_set); % Chnage param_map keys for borrowing param_map('fl_b_bd') = -20; % borrow bound param_map('bl_default') = false; % true if allow for default param_map('fl_c_min') = 0.0001; % u(c_min) when default % Change Keys in param_map param_map('it_a_n') = 500; param_map('it_z_n') = 11; param_map('fl_a_max') = 100; param_map('fl_w') = 1.3; param_map('fl_r_inf') = 0.06; param_map('fl_r_fsv') = 0.01; param_map('fl_r_fbr') = 0.035; param_map('bl_b_is_principle') = false; % see: ffs_for_br_block.m param_map('st_forbrblk_type') = 'seg3'; param_map('fl_forbrblk_brmost') = -10; param_map('fl_forbrblk_brleast') = -1; param_map('fl_forbrblk_gap') = -1; % Change Keys support_map support_map('bl_display') = false; support_map('bl_post') = true; support_map('bl_display_final') = false; % Call Program with external parameters that override defaults. ff_abz_fibs_vf_vecsv(param_map, support_map);
@include
@seealso
- for/inf + save + borr loop: ff_abz_fibs_vf
- for/inf + borr vectorized: ff_abz_fibs_vf_vec
- for/inf + borr optimized-vectorized: ff_abz_fibs_vf_vecsv
Default
- it_param_set = 1: quick test
- it_param_set = 2: benchmark run
- it_param_set = 3: benchmark profile
- it_param_set = 4: press publish button
go to ffs_abz_fibs_fibs_set_default_param to change parameters in param_map container. The parameters can also be updated here directly after obtaining them from ffs_abz_fibs_set_default_param as we possibly change it_a_n and it_z_n here.
it_param_set = 4; bl_input_override = true; [param_map, support_map] = ffs_abz_fibs_set_default_param(it_param_set); % Note: param_map and support_map can be adjusted here or outside to override defaults % To generate results as if formal informal do not matter % param_map('fl_r_fsv') = 0.025; % param_map('fl_r_inf') = 0.035; % param_map('fl_r_inf_bridge') = 0.035; % param_map('fl_r_fbr') = 0.035; % param_map('bl_b_is_principle') = false; % param_map('st_forbrblk_type') = 'seg3'; % param_map('fl_forbrblk_brmost') = -19; % param_map('fl_forbrblk_brleast') = -1; % param_map('fl_forbrblk_gap') = -1.5; % param_map('bl_b_is_principle') = false; % param_map('it_a_n') = 750; % param_map('it_z_n') = 15; [armt_map, func_map] = ffs_abz_fibs_get_funcgrid(param_map, support_map, bl_input_override); % 1 for override default_params = {param_map support_map armt_map func_map};
Parse Parameters 1
% if varargin only has param_map and support_map, params_len = length(varargin); [default_params{1:params_len}] = varargin{:}; param_map = [param_map; default_params{1}]; support_map = [support_map; default_params{2}]; if params_len >= 1 && params_len <= 2 % If override param_map, re-generate armt and func if they are not % provided bl_input_override = true; [armt_map, func_map] = ffs_abz_fibs_get_funcgrid(param_map, support_map, bl_input_override); else % Override all armt_map = [armt_map; default_params{3}]; func_map = [func_map; default_params{4}]; end % append function name st_func_name = 'ff_abz_fibs_vf_vecsv'; support_map('st_profile_name_main') = [st_func_name support_map('st_profile_name_main')]; support_map('st_mat_name_main') = [st_func_name support_map('st_mat_name_main')]; support_map('st_img_name_main') = [st_func_name support_map('st_img_name_main')];
Parse Parameters 2
% armt_map params_group = values(armt_map, {'ar_a', 'mt_z_trans', 'ar_z'}); [ar_a, mt_z_trans, ar_z] = params_group{:}; % Formal choice Menu/Grid and Interest Rate Menu/Grid params_group = values(armt_map, {'ar_forbrblk_r', 'ar_forbrblk'}); [ar_forbrblk_r, ar_forbrblk] = params_group{:}; % func_map params_group = values(func_map, {'f_util_log', 'f_util_crra', 'f_coh', 'f_cons_coh_fbis', 'f_cons_coh_save'}); [f_util_log, f_util_crra, f_coh, f_cons_coh_fbis, f_cons_coh_save] = params_group{:}; % param_map params_group = values(param_map, {'it_a_n', 'it_z_n', 'fl_crra', 'fl_beta', 'fl_c_min',... 'fl_nan_replace', 'bl_default', 'bl_bridge', 'bl_rollover', 'fl_default_aprime'}); [it_a_n, it_z_n, fl_crra, fl_beta, fl_c_min, ... fl_nan_replace, bl_default, bl_bridge, bl_rollover, fl_default_aprime] = params_group{:}; params_group = values(param_map, {'it_maxiter_val', 'fl_tol_val', 'fl_tol_pol', 'it_tol_pol_nochange'}); [it_maxiter_val, fl_tol_val, fl_tol_pol, it_tol_pol_nochange] = params_group{:}; % param_map, Formal informal params_group = values(param_map, {'fl_r_inf', 'fl_r_fsv', 'bl_b_is_principle'}); [fl_r_inf, fl_r_fsv, bl_b_is_principle] = params_group{:}; % support_map params_group = values(support_map, {'bl_profile', 'st_profile_path', ... 'st_profile_prefix', 'st_profile_name_main', 'st_profile_suffix',... 'bl_display_minccost', 'bl_display_infbridge', ... 'bl_time', 'bl_display', 'it_display_every', 'bl_post'}); [bl_profile, st_profile_path, ... st_profile_prefix, st_profile_name_main, st_profile_suffix, ... bl_display_minccost, bl_display_infbridge, ... bl_time, bl_display, it_display_every, bl_post] = params_group{:};
Initialize Output Matrixes
include mt_pol_idx which we did not have in looped code
mt_val_cur = zeros(length(ar_a),length(ar_z)); mt_val = mt_val_cur - 1; mt_pol_a = zeros(length(ar_a),length(ar_z)); mt_pol_a_cur = mt_pol_a - 1; mt_pol_idx = zeros(length(ar_a),length(ar_z)); mt_pol_cons = zeros(length(ar_a),length(ar_z)); % collect optimal borrowing formal and informal choices mt_pol_b_bridge = zeros(length(ar_a),length(ar_z)); mt_pol_inf_borr_nobridge = zeros(length(ar_a),length(ar_z)); mt_pol_for_borr = zeros(length(ar_a),length(ar_z)); mt_pol_for_save = zeros(length(ar_a),length(ar_z)); % We did not need these in ff_abz_vf or ff_abz_vf_vec % see % <https://fanwangecon.github.io/M4Econ/support/speed/partupdate/fs_u_c_partrepeat_main.html % fs_u_c_partrepeat_main> for why store using cells. cl_u_c_store = cell([it_z_n, 1]); cl_c_store = cell([it_z_n, 1]); cl_c_valid_idx = cell([it_z_n, 1]);
Initialize Convergence Conditions
bl_vfi_continue = true; it_iter = 0; ar_val_diff_norm = zeros([it_maxiter_val, 1]); ar_pol_diff_norm = zeros([it_maxiter_val, 1]); mt_pol_perc_change = zeros([it_maxiter_val, it_z_n]);
Iterate Value Function
Loop solution with 4 nested loops
- loop 1: over exogenous states
- loop 2: over endogenous states
- loop 3: over choices
- loop 4: add future utility, integration--loop over future shocks
% Start Profile if (bl_profile) close all; profile off; profile on; end % Start Timer if (bl_time) tic; end % Value Function Iteration while bl_vfi_continue
it_iter = it_iter + 1;
Solve Optimization Problem Current Iteration
% loop 1: over exogenous states for it_z_i = 1:length(ar_z)
Solve the Formal Informal Problem for each a' and coh: c_forinf(a')
find the today's consumption maximizing formal and informal choices given a' and coh. The formal and informal choices need to generate exactly a', but depending on which formal and informal joint choice is used, the consumption cost today a' is different. Note here, a is principle + interests. Three areas:
- CASE A a' > 0: savings, do not need to optimize over formal and informal choices
- CASE B a' < 0 & coh < 0: need bridge loan to pay for unpaid debt, and borrowing over-all, need to first pick bridge loan to pay for debt, if bridge loan is insufficient, go into default. After bridge loan, optimize over formal+informal, borrow+save joint choices.
- CASE C a' < 0 & coh > 0: do not need to get informal bridge loans, optimize over for+inf save, for+save+borr, inf+borr only, for borrow only.
% 1. Current Shock fl_z = ar_z(it_z_i); % 2. cash-on-hand ar_coh = f_coh(fl_z, ar_a); % Consumption and u(c) only need to be evaluated once if (it_iter == 1)
% 3. *CASE A* initiate consumption matrix as if all save mt_c = f_cons_coh_save(ar_coh, ar_a'); % 4. *CASE B+C* get negative coh index and get borrowing choices index ar_coh_neg_idx = (ar_coh <= 0); ar_a_neg_idx = (ar_a < 0); ar_coh_neg = ar_coh(ar_coh_neg_idx); ar_a_neg = ar_a(ar_a_neg_idx); % 5. if coh > 0 and ap < 0, can allow same for+inf result to all coh. % The procedure below works regardless of how ar_coh is sorted. get % the index of all negative coh elements as well as first % non-negative element. We solve the formal and informal problem at % these points, note that we only need to solve the formal and % informal problem for positive coh level once. ar_coh_first_pos_idx = (cumsum(ar_coh_neg_idx == 0) == 1); ar_coh_forinfsolve_idx = (ar_coh_first_pos_idx | ar_coh_neg_idx); ar_coh_forinfsolve_a_neg_idx = (ar_coh(ar_coh_forinfsolve_idx) <= 0); % 6. *CASE B + C* Negative asset choices (borrowing), 1 col Case C % negp1: negative coh + 1, 1 meaning 1 positive coh, first positive % coh column index element grabbed. mt_coh_negp1_mesh_neg_aprime = zeros(size(ar_a_neg')) + ar_coh(ar_coh_forinfsolve_idx); mt_neg_aprime_mesh_coh_negp1 = zeros(size(mt_coh_negp1_mesh_neg_aprime)) + ar_a_neg'; if (bl_bridge) % mt_neg_aprime_mesh_coh_1col4poscoh = zeros([length(ar_a_neg), (length(ar_coh_neg)+1)]) + ar_a_neg'; % ar_coh_neg_idx_1col4poscoh = ar_coh_neg_idx(1:(length(ar_coh_neg)+1)); % 6. *CASE B* Solve for: if (fl_ap < 0) and if (fl_coh < 0) [mt_aprime_nobridge_negcoh, ~, mt_c_bridge_negcoh] = ffs_fibs_inf_bridge(... bl_b_is_principle, fl_r_inf, ... mt_neg_aprime_mesh_coh_negp1(:,ar_coh_forinfsolve_a_neg_idx), ... mt_coh_negp1_mesh_neg_aprime(:,ar_coh_forinfsolve_a_neg_idx), ... bl_display_infbridge, bl_input_override); % generate mt_aprime_nobridge mt_neg_aprime_mesh_coh_negp1(:, ar_coh_forinfsolve_a_neg_idx) = mt_aprime_nobridge_negcoh; else % no bridge loan needed means roll over is allowed. mt_neg_aprime_mesh_coh_negp1 = ar_a_neg'; end % 7. *CASE B + C* formal and informal joint choices, 1 col Case C bl_input_override = true; [ar_max_c_nobridge, ~, ~, ~] = ... ffs_fibs_min_c_cost(... bl_b_is_principle, fl_r_inf, fl_r_fsv, ... ar_forbrblk_r, ar_forbrblk, ... mt_neg_aprime_mesh_coh_negp1(:), ... bl_display_minccost, bl_input_override);
Update Consumption Matrix CASE A + B + C Consumptions
Current mt_c is assuming all to be case A
- Update Columns for case B (negative coh)
- Update Columns for case C (1 column): ar_coh_first_pos_idx, included in ar_coh_forinfsolve_idx
- Update Columns for all case C: ~ar_coh_neg_idx using 1 column result
% 1. Initalize all Neg Aprime consumption cost of aprime inputs % Initialize mt_max_c_nobridge_a_neg = zeros([length(ar_a_neg), length(ar_coh)]) + 0; mt_c_bridge_coh_a_neg = zeros(size(mt_max_c_nobridge_a_neg)) + 0; % 2. Fill in *Case B* and *Case C* (one column) Other C-cost mt_max_c_nobridge_negcohp1 = reshape(ar_max_c_nobridge, [size(mt_neg_aprime_mesh_coh_negp1)]); if (bl_bridge) % 2. Fill in *Case B* Bridge C-cost mt_c_bridge_coh_a_neg(:, ar_coh_neg_idx) = mt_c_bridge_negcoh; % 2. Fill in *Case B* and *Case C* (one column) Other C-cost mt_max_c_nobridge_a_neg(:, ar_coh_forinfsolve_idx) = mt_max_c_nobridge_negcohp1; mt_max_c_nobridge_a_neg(:, ~ar_coh_forinfsolve_idx) = ... zeros(size(mt_c(ar_a_neg_idx, ~ar_coh_forinfsolve_idx))) ... + mt_max_c_nobridge_negcohp1(:, ~ar_coh_forinfsolve_a_neg_idx); else mt_max_c_nobridge_a_neg = zeros([length(ar_a_neg), length(ar_coh)]) + mt_max_c_nobridge_negcohp1; end % 3. Consumption for B + C Cases % note, the c cost of aprime is the same for all coh > 0, but mt_c % is different still for each coh and aprime. mt_c_forinfsolve = f_cons_coh_fbis(ar_coh, mt_c_bridge_coh_a_neg + mt_max_c_nobridge_a_neg); % 4. Update with Case B and C mt_c(ar_a_neg_idx, :) = mt_c_forinfsolve;
Evaluate u(c) and store
1. EVAL current utility: N by N, f_util defined earlier
if (fl_crra == 1) mt_utility = log(mt_c); fl_u_cmin = f_util_log(fl_c_min); else mt_utility = f_util_crra(mt_c); fl_u_cmin = f_util_crra(fl_c_min); end % 2. Invliad consumption points mt_it_c_valid_idx = (mt_c <= fl_c_min); % Set below threshold c to c_min mt_c(mt_c < fl_c_min) = fl_c_min; % 3. Invalid points if bridge not possible also no rollover if( ~bl_rollover && ~bl_bridge) mt_it_c_valid_idx(:, ar_coh_neg_idx) = 1; end % 4. Eliminate Complex Numbers mt_utility(mt_it_c_valid_idx) = fl_u_cmin; % 5. Store in cells cl_c_store{it_z_i} = mt_c; cl_u_c_store{it_z_i} = mt_utility; cl_c_valid_idx{it_z_i} = mt_it_c_valid_idx;
end
Solve Optimization Problem: max_{a'} (u(c_forinf(a')) + EV(a',z'))
% 1. f(z'|z) ar_z_trans_condi = mt_z_trans(it_z_i,:); % 2. EVAL EV((A',K'),Z'|Z) = V((A',K'),Z') x p(z'|z)', (N by Z) x (Z by 1) = N by 1 % Note: transpose ar_z_trans_condi from 1 by Z to Z by 1 % Note: matrix multiply not dot multiply mt_evzp_condi_z = mt_val_cur * ar_z_trans_condi'; % 3. EVAL add on future utility, N by N + N by 1, broadcast again mt_utility = cl_u_c_store{it_z_i} + fl_beta*mt_evzp_condi_z; % 4. Index update % using the method below is much faster than index replace % see <https://fanwangecon.github.io/M4Econ/support/speed/index/fs_subscript.html fs_subscript> mt_it_c_valid_idx = cl_c_valid_idx{it_z_i}; % Default or Not Utility Handling if (bl_default) % if default: only today u(cmin), transition out next period, debt wiped out fl_v_default = fl_u_cmin + fl_beta*mt_evzp_condi_z(ar_a == fl_default_aprime); mt_utility = mt_utility.*(~mt_it_c_valid_idx) + fl_v_default*(mt_it_c_valid_idx); else % if default is not allowed: v = u(cmin) mt_utility = mt_utility.*(~mt_it_c_valid_idx) + fl_nan_replace*(mt_it_c_valid_idx); end % Optimization: remember matlab is column major, rows must be % choices, columns must be states % <https://en.wikipedia.org/wiki/Row-_and_column-major_order COLUMN-MAJOR> % mt_utility is N by N, rows are choices, cols are states. [ar_opti_val_z, ar_opti_idx_z] = max(mt_utility); [it_choies_n, it_states_n] = size(mt_utility); ar_add_grid = linspace(0, it_choies_n*(it_states_n-1), it_states_n); ar_opti_linear_idx_z = ar_opti_idx_z + ar_add_grid; ar_opti_aprime_z = ar_a(ar_opti_idx_z); ar_opti_c_z = cl_c_store{it_z_i}(ar_opti_linear_idx_z); % Handle Default is optimal or not if (bl_default) % if defaulting is optimal choice, at these states, not required % to default, non-default possible, but default could be optimal ar_opti_aprime_z(ar_opti_c_z <= fl_c_min) = fl_default_aprime; ar_opti_idx_z(ar_opti_c_z <= fl_c_min) = find(ar_a == fl_default_aprime); else % if default is not allowed, then next period same state as now % this is absorbing state, this is the limiting case, single % state space point, lowest a and lowest shock has this. ar_opti_aprime_z(ar_opti_c_z <= fl_c_min) = min(ar_a); end % 6. no bridge and no rollover allowed if( ~bl_rollover && ~bl_bridge) if (bl_default) % if default: only today u(cmin), transition out next period, debt wiped out ar_opti_aprime_z(ar_coh_neg_idx) = fl_default_aprime; else % if default is not allowed: v = fl_nan_replace ar_opti_aprime_z(ar_coh_neg_idx) = ar_a(fl_nan_replace); end end % store optimal values mt_val(:,it_z_i) = ar_opti_val_z; mt_pol_a(:,it_z_i) = ar_opti_aprime_z; mt_pol_cons(:,it_z_i) = ar_opti_c_z; if (it_iter == (it_maxiter_val + 1)) mt_pol_idx(:,it_z_i) = ar_opti_idx_z; end
end
Check Tolerance and Continuation
% Difference across iterations ar_val_diff_norm(it_iter) = norm(mt_val - mt_val_cur); ar_pol_diff_norm(it_iter) = norm(mt_pol_a - mt_pol_a_cur); mt_pol_perc_change(it_iter, :) = sum((mt_pol_a ~= mt_pol_a_cur))/(it_a_n); % Update mt_val_cur = mt_val; mt_pol_a_cur = mt_pol_a; % Print Iteration Results if (bl_display && (rem(it_iter, it_display_every)==0)) fprintf('VAL it_iter:%d, fl_diff:%d, fl_diff_pol:%d\n', ... it_iter, ar_val_diff_norm(it_iter), ar_pol_diff_norm(it_iter)); tb_valpol_iter = array2table([mean(mt_val_cur,1); mean(mt_pol_a_cur,1); ... mt_val_cur(it_a_n,:); mt_pol_a_cur(it_a_n,:)]); tb_valpol_iter.Properties.VariableNames = strcat('z', string((1:size(mt_val_cur,2)))); tb_valpol_iter.Properties.RowNames = {'mval', 'map', 'Hval', 'Hap'}; disp('mval = mean(mt_val_cur,1), average value over a') disp('map = mean(mt_pol_a_cur,1), average choice over a') disp('Hval = mt_val_cur(it_a_n,:), highest a state val') disp('Hap = mt_pol_a_cur(it_a_n,:), highest a state choice') disp(tb_valpol_iter); end % Continuation Conditions: % 1. if value function convergence criteria reached % 2. if policy function variation over iterations is less than % threshold if (it_iter == (it_maxiter_val + 1)) bl_vfi_continue = false; elseif ((it_iter == it_maxiter_val) || ... (ar_val_diff_norm(it_iter) < fl_tol_val) || ... (sum(ar_pol_diff_norm(max(1, it_iter-it_tol_pol_nochange):it_iter)) < fl_tol_pol)) % Fix to max, run again to save results if needed it_iter_last = it_iter; it_iter = it_maxiter_val; end
end % End Timer if (bl_time) toc; end % End Profile if (bl_profile) profile off profile viewer st_file_name = [st_profile_prefix st_profile_name_main st_profile_suffix]; profsave(profile('info'), strcat(st_profile_path, st_file_name)); end
Process Optimal Choices
result_map = containers.Map('KeyType','char', 'ValueType','any'); result_map('mt_val') = mt_val; result_map('mt_pol_idx') = mt_pol_idx; % Find optimal Formal Informal Choices. Could have saved earlier, but was % wasteful of resources for it_z_i = 1:length(ar_z) for it_a_j = 1:length(ar_a) fl_z = ar_z(it_z_i); fl_a = ar_a(it_a_j); fl_coh = f_coh(fl_z, fl_a); fl_a_opti = mt_pol_a(it_a_j, it_z_i); % call formal and informal function. [~, fl_opti_b_bridge, fl_opti_inf_borr_nobridge, fl_opti_for_borr, fl_opti_for_save] = ... ffs_fibs_min_c_cost_bridge(fl_a_opti, fl_coh, ... param_map, support_map, armt_map, func_map, bl_input_override); % store savings and borrowing formal and inf optimal choices mt_pol_b_bridge(it_a_j,it_z_i) = fl_opti_b_bridge; mt_pol_inf_borr_nobridge(it_a_j,it_z_i) = fl_opti_inf_borr_nobridge; mt_pol_for_borr(it_a_j,it_z_i) = fl_opti_for_borr; mt_pol_for_save(it_a_j,it_z_i) = fl_opti_for_save; end end result_map('cl_mt_pol_a') = {mt_pol_a, zeros(1)}; result_map('cl_mt_coh') = {f_coh(ar_z, ar_a'), zeros(1)}; result_map('cl_mt_pol_c') = {mt_pol_cons, zeros(1)}; result_map('cl_mt_pol_b_bridge') = {mt_pol_b_bridge, zeros(1)}; result_map('cl_mt_pol_inf_borr_nobridge') = {mt_pol_inf_borr_nobridge, zeros(1)}; result_map('cl_mt_pol_for_borr') = {mt_pol_for_borr, zeros(1)}; result_map('cl_mt_pol_for_save') = {mt_pol_for_save, zeros(1)}; result_map('ar_st_pol_names') = ["cl_mt_pol_a", "cl_mt_coh", "cl_mt_pol_c", ... "cl_mt_pol_b_bridge", "cl_mt_pol_inf_borr_nobridge", "cl_mt_pol_for_borr", "cl_mt_pol_for_save"]; % Get Discrete Choice Outcomes result_map = ffs_fibs_identify_discrete(result_map, bl_input_override);
Post Solution Graph and Table Generation
Note in comparison with abz, results here, even when using identical parameters would differ because in abz solved where choices are principle. Here choices are principle + interests in order to facilitate using the informal choice functions.
Note that this means two things are different, on the one hand, the value of asset for to coh is different based on the grid of assets. If the asset grid is negative, now per grid point, there is more coh because that grid point of asset no longer has interest rates. On the other hand, if one has positive asset grid point on arrival, that is worth less to coh. Additionally, when making choices for the next period, now choices aprime includes interests. What these mean is that the a grid no longer has the same meaning. We should expect at higher savings levels, for the same grid points, if optimal grid choices are the same as before, consumption should be lower when b includes interest rates and principle. This is however, not true when arriving in a period with negative a levels, for the same negative a level and same a prime negative choice, could have higher consumption here becasue have to pay less interests on debt. This tends to happen for smaller levels of borrowing choices.
Graphically, when using interest + principle, big difference in consumption as a fraction of (coh - aprime) figure. In those figures, when counting in principles only, the gap in coh and aprime is consumption, but now, as more is borrowed only a small fraction of coh and aprime gap is consumption, becuase aprime/(1+r) is put into consumption.
if (bl_post) bl_input_override = true; result_map('ar_val_diff_norm') = ar_val_diff_norm(1:it_iter_last); result_map('ar_pol_diff_norm') = ar_pol_diff_norm(1:it_iter_last); result_map('mt_pol_perc_change') = mt_pol_perc_change(1:it_iter_last, :); % Standard AZ graphs result_map = ff_az_vf_post(param_map, support_map, armt_map, func_map, result_map, bl_input_override); % Graphs for results_map with FIBS contents result_map = ff_az_fibs_vf_post(param_map, support_map, armt_map, func_map, result_map, bl_input_override); end
valgap = norm(mt_val - mt_val_cur): value function difference across iterations polgap = norm(mt_pol_a - mt_pol_a_cur): policy function difference across iterations z1 = z1 perc change: sum((mt_pol_a ~= mt_pol_a_cur))/(it_a_n): percentage of state space points conditional on shock where the policy function is changing across iterations valgap polgap z1_0_34741 z2_0_40076 z3_0_4623 z4_0_5333 z5_0_61519 z6_0_70966 z10_1_2567 z11_1_4496 z12_1_6723 z13_1_9291 z14_2_2253 z15_2_567 ________ ________ __________ __________ _________ _________ __________ __________ __________ __________ __________ __________ __________ _________ iter=1 193.16 2002 1 1 1 1 1 1 1 1 1 1 1 1 iter=2 147.46 2189.9 0.98 0.98133 0.98267 0.984 0.98533 0.98667 0.99733 0.99867 1 1 1 1 iter=3 119.38 721.95 0.96267 0.96533 0.96667 0.96933 0.972 0.97467 0.992 0.99467 1 1 1 1 iter=4 98.645 364.17 0.94933 0.95333 0.956 0.96 0.964 0.968 0.98267 0.99467 0.99467 0.99467 1 1 iter=5 83.341 214.04 0.932 0.93467 0.93733 0.94133 0.94667 0.948 0.97333 0.988 0.99067 0.99067 0.99867 0.99733 iter=6 71.328 142.53 0.916 0.91733 0.92 0.92533 0.92933 0.93467 0.96667 0.97733 0.98133 0.99333 0.98933 0.98533 iter=7 61.306 103.17 0.90133 0.90533 0.912 0.91467 0.92533 0.92933 0.956 0.968 0.96933 0.98267 0.98933 0.98533 iter=8 53.057 77.485 0.884 0.89067 0.89733 0.896 0.904 0.91733 0.94667 0.95333 0.96533 0.97467 0.996 0.99467 iter=9 46.053 59.056 0.87867 0.88133 0.88667 0.892 0.89867 0.904 0.94133 0.94933 0.96533 0.97733 0.98 0.98267 iter=10 40.105 47.942 0.85867 0.86267 0.87467 0.87867 0.88267 0.89467 0.93733 0.948 0.94533 0.96667 0.968 0.96533 iter=11 34.971 39.556 0.85867 0.86267 0.86 0.86533 0.87467 0.88533 0.91867 0.92667 0.952 0.96 0.972 0.98667 iter=12 30.532 32.316 0.83467 0.848 0.85467 0.86 0.864 0.86933 0.91333 0.932 0.92 0.93733 0.952 0.96 iter=13 26.721 27.698 0.82267 0.828 0.82667 0.852 0.84 0.86267 0.89467 0.90533 0.932 0.93867 0.94 0.96 iter=14 23.418 24.581 0.80133 0.80667 0.82533 0.808 0.84 0.85733 0.888 0.89733 0.88933 0.908 0.91867 0.92667 iter=15 20.561 21.804 0.77733 0.772 0.78267 0.81333 0.81733 0.80933 0.85067 0.852 0.87467 0.88267 0.904 0.92 iter=16 18.084 22.136 0.74 0.796 0.776 0.78933 0.77733 0.804 0.82533 0.84267 0.832 0.85467 0.85333 0.86667 iter=17 15.95 21 0.752 0.724 0.75733 0.75733 0.74933 0.77067 0.796 0.79333 0.80533 0.81067 0.84933 0.86667 iter=18 14.109 12.329 0.692 0.74133 0.70267 0.72 0.76 0.748 0.75333 0.76 0.78667 0.792 0.792 0.81067 iter=19 12.519 10.837 0.68667 0.668 0.71733 0.68267 0.676 0.704 0.73467 0.74133 0.72667 0.75067 0.75333 0.76133 iter=20 11.152 20.229 0.64 0.65467 0.636 0.67733 0.668 0.65333 0.69067 0.68533 0.71467 0.69333 0.74533 0.764 iter=21 9.9781 20.201 0.60667 0.59333 0.60533 0.60267 0.612 0.636 0.62267 0.63067 0.668 0.70133 0.66933 0.68933 iter=22 8.973 7.6088 0.55067 0.56667 0.55867 0.568 0.56667 0.568 0.61733 0.644 0.608 0.62533 0.63467 0.644 iter=23 8.1119 6.8177 0.51867 0.50533 0.52133 0.51867 0.52933 0.524 0.56133 0.55867 0.568 0.58133 0.61333 0.63467 iter=24 7.3741 6.1377 0.46533 0.46 0.46533 0.47333 0.47733 0.47867 0.49733 0.51067 0.54 0.53333 0.55867 0.57733 iter=25 6.739 5.548 0.408 0.42 0.424 0.41467 0.424 0.42933 0.452 0.472 0.492 0.51067 0.50667 0.52 iter=26 6.1882 5.0877 0.36133 0.37467 0.37067 0.37867 0.37467 0.38533 0.41467 0.41867 0.43333 0.44667 0.45867 0.47733 iter=27 5.7054 4.6636 0.32667 0.32667 0.336 0.34267 0.34267 0.34267 0.368 0.37333 0.388 0.40667 0.41333 0.424 iter=28 5.2784 4.273 0.304 0.29867 0.30267 0.292 0.30267 0.31733 0.32933 0.348 0.35333 0.36133 0.37067 0.38667 iter=29 4.8977 3.8881 0.26133 0.26267 0.272 0.28 0.27733 0.28133 0.30267 0.31467 0.316 0.32667 0.336 0.344 iter=30 4.557 3.5318 0.22667 0.236 0.248 0.244 0.24667 0.248 0.284 0.28 0.28133 0.304 0.30133 0.32 iter=31 4.2503 3.1787 0.216 0.21333 0.212 0.216 0.22667 0.228 0.23867 0.252 0.26267 0.26267 0.28667 0.29067 iter=32 3.9739 2.8897 0.18533 0.204 0.19333 0.204 0.19467 0.20533 0.22533 0.23333 0.23733 0.248 0.24933 0.26267 iter=33 3.7244 2.664 0.17867 0.176 0.184 0.17333 0.18667 0.19067 0.20267 0.212 0.21867 0.22267 0.23067 0.24533 iter=34 3.4987 2.4205 0.14933 0.15467 0.15333 0.16133 0.15467 0.15867 0.192 0.18933 0.192 0.2 0.20267 0.216 iter=35 3.2939 2.2838 0.13733 0.13867 0.13867 0.144 0.15333 0.152 0.15733 0.16933 0.18267 0.18133 0.19467 0.20133 iter=36 3.1074 2.1165 0.128 0.13067 0.13467 0.13067 0.128 0.13467 0.14933 0.15867 0.16533 0.16933 0.172 0.18 iter=37 2.9368 1.9272 0.10533 0.11333 0.11733 0.12133 0.12133 0.12133 0.14 0.14667 0.13867 0.14667 0.16 0.16 iter=38 2.7802 1.8469 0.10267 0.10267 0.10933 0.098667 0.11333 0.11867 0.12 0.12533 0.136 0.14133 0.14133 0.15067 iter=39 2.6359 1.6291 0.090667 0.092 0.089333 0.093333 0.092 0.097333 0.11333 0.12133 0.11733 0.128 0.12533 0.13333 iter=40 2.5024 1.5268 0.082667 0.084 0.085333 0.092 0.090667 0.084 0.10667 0.10667 0.10933 0.11867 0.124 0.124 iter=41 2.3784 1.4197 0.074667 0.08 0.076 0.082667 0.077333 0.085333 0.085333 0.090667 0.096 0.1 0.108 0.11733 iter=42 2.2626 1.3113 0.065333 0.068 0.069333 0.062667 0.077333 0.077333 0.086667 0.088 0.096 0.098667 0.094667 0.10267 iter=43 2.1541 1.2028 0.064 0.062667 0.062667 0.066667 0.065333 0.066667 0.076 0.078667 0.077333 0.081333 0.089333 0.089333 iter=44 2.0521 1.1451 0.06 0.052 0.064 0.058667 0.057333 0.058667 0.070667 0.073333 0.08 0.077333 0.088 0.090667 iter=45 1.9556 1.0413 0.042667 0.052 0.050667 0.049333 0.052 0.050667 0.062667 0.06 0.066667 0.065333 0.070667 0.076 iter=46 1.8643 0.99246 0.042667 0.048 0.038667 0.054667 0.049333 0.053333 0.056 0.058667 0.065333 0.068 0.066667 0.069333 iter=47 1.7774 0.92978 0.037333 0.04 0.044 0.042667 0.045333 0.042667 0.049333 0.052 0.056 0.06 0.057333 0.062667 iter=48 1.6945 0.86419 0.036 0.038667 0.038667 0.034667 0.041333 0.042667 0.044 0.050667 0.050667 0.056 0.058667 0.058667 iter=49 1.6154 0.78442 0.032 0.030667 0.030667 0.036 0.034667 0.041333 0.049333 0.044 0.041333 0.044 0.049333 0.052 iter=50 1.5396 0.76102 0.029333 0.026667 0.033333 0.028 0.032 0.028 0.036 0.042667 0.044 0.044 0.045333 0.048 iter=69 0.56255 0.23338 0 0.0013333 0 0 0.0026667 0.004 0.004 0.0053333 0.0026667 0.0026667 0.0066667 0.0053333 iter=70 0.53064 0.20898 0.0013333 0.0013333 0.0013333 0.0026667 0.0026667 0.0013333 0.0026667 0 0.0013333 0.004 0.004 0.0066667 iter=71 0.50032 0.20898 0.0066667 0.0013333 0.0026667 0.0013333 0 0.0013333 0.0026667 0.0013333 0.0053333 0 0.004 0.0026667 iter=72 0.47154 0.16187 0 0 0.0013333 0 0 0.0026667 0 0.004 0 0.004 0.004 0.0026667 iter=73 0.44427 0.13217 0 0 0 0 0.0013333 0 0 0.0013333 0.0013333 0.0026667 0.0013333 0.0026667 iter=74 0.41843 0.16187 0 0 0 0 0 0 0.0026667 0 0.0026667 0 0.004 0.0013333 iter=75 0.39399 0.13217 0.0013333 0 0 0.0013333 0 0.0026667 0.0013333 0.0013333 0.0026667 0.0026667 0 0 iter=76 0.37088 0.13217 0 0.0013333 0.0013333 0.0013333 0 0 0 0.0013333 0 0 0.0013333 0.0026667 iter=77 0.34905 0.093458 0 0 0 0 0 0 0.0013333 0 0 0.0013333 0.0013333 0.0013333 iter=78 0.32844 0.093458 0 0.0013333 0 0 0.0013333 0 0.0013333 0 0 0.0013333 0 0 iter=79 0.30901 0.16187 0 0.0013333 0 0.0013333 0 0.0013333 0 0 0 0.004 0.0013333 0 iter=80 0.29068 0.13217 0 0 0 0 0 0 0 0 0.0013333 0 0.0013333 0.0026667 iter=81 0.27341 0.093458 0 0 0 0 0 0 0.0013333 0 0 0 0 0 iter=82 0.25715 0.093458 0 0 0 0 0 0 0 0 0 0 0 0.0013333 iter=83 0.24183 0.093458 0 0 0 0 0 0.0013333 0 0 0 0 0 0 iter=84 0.22742 0.093458 0.0013333 0 0 0 0 0 0 0 0 0.0013333 0 0 iter=85 0.21385 0.093458 0 0 0 0 0 0 0 0 0.0013333 0 0 0 iter=86 0.20109 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=87 0.18908 0.093458 0 0.0013333 0 0 0.0013333 0 0 0 0 0 0 0 iter=88 0.17778 0.093458 0 0 0 0 0 0 0 0 0 0 0 0.0013333 iter=89 0.16716 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=90 0.15717 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=91 0.14777 0.093458 0 0 0 0.0013333 0 0 0 0 0 0 0 0 iter=92 0.13893 0.093458 0 0 0 0 0 0 0 0 0 0.0013333 0 0 iter=93 0.13062 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=94 0.1228 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=95 0.11545 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=96 0.10854 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=97 0.10204 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=98 0.09593 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=99 0.090183 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=100 0.08478 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=101 0.0797 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=102 0.074923 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=103 0.070433 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=104 0.06621 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=105 0.062241 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=106 0.058509 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=107 0.055 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=108 0.051702 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=109 0.048601 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=110 0.045686 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=111 0.042946 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=112 0.04037 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=113 0.037949 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=114 0.035672 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=115 0.033532 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=116 0.031521 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=117 0.02963 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=118 0.027852 0 0 0 0 0 0 0 0 0 0 0 0 0 tb_val: V(a,z) value at each state space point z1_0_34741 z2_0_40076 z3_0_4623 z4_0_5333 z5_0_61519 z6_0_70966 z10_1_2567 z11_1_4496 z12_1_6723 z13_1_9291 z14_2_2253 z15_2_567 __________ __________ _________ _________ __________ __________ __________ __________ __________ __________ __________ _________ a1=-20 -11.41 -11.157 -10.871 -10.562 -10.237 -9.8987 -8.4686 -8.1039 -7.7396 -7.378 -6.7979 -6.1925 a2=-19.9065 -11.41 -11.157 -10.871 -10.562 -10.237 -9.8987 -8.4686 -8.1039 -7.7396 -7.378 -6.7256 -6.1474 a3=-19.8131 -11.41 -11.157 -10.871 -10.562 -10.237 -9.8987 -8.4686 -8.1039 -7.7396 -7.2987 -6.6611 -6.0954 a4=-19.7196 -11.41 -11.157 -10.871 -10.562 -10.237 -9.8987 -8.4686 -8.1039 -7.7396 -7.2005 -6.603 -6.0336 a5=-19.6262 -11.41 -11.157 -10.871 -10.562 -10.237 -9.8987 -8.4686 -8.1039 -7.7396 -7.1182 -6.5504 -5.9757 a6=-19.5327 -11.41 -11.157 -10.871 -10.562 -10.237 -9.8987 -8.4686 -8.1039 -7.6537 -7.0454 -6.5025 -5.9169 a7=-19.4393 -11.41 -11.157 -10.871 -10.562 -10.237 -9.8987 -8.4686 -8.1039 -7.5445 -6.9804 -6.4586 -5.8571 a8=-19.3458 -11.41 -11.157 -10.871 -10.562 -10.237 -9.8987 -8.4686 -8.072 -7.4558 -6.922 -6.4181 -5.7977 a9=-19.2523 -11.41 -11.157 -10.871 -10.562 -10.237 -9.8987 -8.4686 -7.9417 -7.3783 -6.8691 -6.3799 -5.7408 a10=-19.1589 -11.41 -11.157 -10.871 -10.562 -10.237 -9.8987 -8.4451 -7.8316 -7.3095 -6.821 -6.3228 -5.6863 a11=-19.0654 -11.41 -11.157 -10.871 -10.562 -10.237 -9.8987 -8.2981 -7.742 -7.2479 -6.7768 -6.2592 -5.6334 a12=-18.972 -11.41 -11.157 -10.871 -10.562 -10.237 -9.8987 -8.1757 -7.6641 -7.1922 -6.7362 -6.1948 -5.5813 a13=-18.8785 -11.41 -11.157 -10.871 -10.562 -10.237 -9.8987 -8.0731 -7.5949 -7.1417 -6.6979 -6.1346 -5.5317 a14=-18.785 -11.41 -11.157 -10.871 -10.562 -10.237 -9.8987 -7.9884 -7.5329 -7.0956 -6.6608 -6.0763 -5.4772 a15=-18.6916 -11.41 -11.157 -10.871 -10.562 -10.237 -9.8987 -7.9138 -7.477 -7.0532 -6.6264 -6.0205 -5.4244 a16=-18.5981 -11.41 -11.157 -10.871 -10.562 -10.237 -9.8022 -7.8473 -7.4263 -7.014 -6.5712 -5.9675 -5.3716 a17=-18.5047 -11.41 -11.157 -10.871 -10.562 -10.124 -9.5994 -7.7877 -7.3799 -6.976 -6.5059 -5.9156 -5.3202 a18=-18.4112 -11.41 -11.157 -10.871 -10.402 -9.9105 -9.4373 -7.7337 -7.3373 -6.9402 -6.443 -5.8655 -5.2693 a19=-18.3178 -11.41 -11.083 -10.634 -10.183 -9.7379 -9.3037 -7.6846 -7.2981 -6.907 -6.3846 -5.8118 -5.2149 a20=-18.2243 -11.205 -10.822 -10.417 -10.006 -9.5968 -9.1912 -7.6396 -7.26 -6.876 -6.327 -5.7605 -5.1618 a21=-18.1308 -10.962 -10.611 -10.241 -9.8616 -9.4788 -9.0991 -7.5983 -7.2241 -6.8369 -6.2739 -5.7102 -5.1086 a22=-18.0374 -10.761 -10.44 -10.097 -9.7411 -9.3808 -9.0198 -7.56 -7.1908 -6.7691 -6.2202 -5.6607 -5.056 a23=-17.9439 -10.601 -10.301 -9.9771 -9.6405 -9.2984 -8.9495 -7.5222 -7.1597 -6.7066 -6.1669 -5.6129 -5.0034 a24=-17.8505 -10.468 -10.184 -9.8771 -9.5569 -9.2257 -8.8867 -7.4873 -7.1306 -6.6464 -6.1159 -5.56 -4.9511 a25=-17.757 -10.356 -10.088 -9.7938 -9.4831 -9.1608 -8.83 -7.4548 -7.1034 -6.5876 -6.0661 -5.5078 -4.8981 a26=-17.6636 -10.265 -10.006 -9.7202 -9.4173 -9.1024 -8.7786 -7.4244 -7.0479 -6.5318 -6.0189 -5.4563 -4.8456 a27=-17.5701 -10.186 -9.9337 -9.6546 -9.3582 -9.0496 -8.7317 -7.396 -6.981 -6.4783 -5.9731 -5.4054 -4.7937 a28=-17.4766 -10.116 -9.8693 -9.5957 -9.3047 -9.0014 -8.6886 -7.3694 -6.9178 -6.4271 -5.9253 -5.3526 -4.7414 a29=-17.3832 -10.053 -9.8113 -9.5424 -9.256 -8.9573 -8.649 -7.3453 -6.8583 -6.3788 -5.8754 -5.3 -4.6888 a30=-17.2897 -9.9968 -9.7587 -9.4938 -9.2114 -8.9166 -8.6108 -7.2796 -6.8017 -6.3316 -5.8268 -5.248 -4.6364 a31=-17.1963 -9.9456 -9.7108 -9.4493 -9.1704 -8.8784 -8.5746 -7.2127 -6.7483 -6.2869 -5.7787 -5.1956 -4.5844 a32=-17.1028 -9.8989 -9.667 -9.4084 -9.1321 -8.8413 -8.5409 -7.1496 -6.697 -6.2411 -5.7278 -5.1443 -4.5322 a33=-17.0093 -9.8559 -9.6265 -9.3701 -9.0946 -8.8069 -8.5095 -7.09 -6.6488 -6.1942 -5.6761 -5.0926 -4.4797 a34=-16.9159 -9.8164 -9.5883 -9.3327 -9.0599 -8.7749 -8.4802 -7.0337 -6.6014 -6.1479 -5.625 -5.0406 -4.4273 a35=-16.8224 -9.7783 -9.5514 -9.2981 -9.0276 -8.745 -8.4527 -6.9799 -6.5561 -6.1037 -5.5729 -4.9891 -4.3747 a36=-16.729 -9.7421 -9.5171 -9.2658 -8.9975 -8.717 -8.4271 -6.9291 -6.5094 -6.0574 -5.5214 -4.9382 -4.3217 a37=-16.6355 -9.7085 -9.4853 -9.2358 -8.9693 -8.6907 -8.4043 -6.8796 -6.4652 -6.0081 -5.4699 -4.887 -4.2685 a38=-16.5421 -9.6772 -9.4555 -9.2076 -8.9428 -8.6672 -8.3827 -6.8318 -6.4223 -5.9597 -5.418 -4.8356 -4.2154 a39=-16.4486 -9.648 -9.4276 -9.1811 -8.9189 -8.6452 -8.3621 -6.7862 -6.381 -5.9088 -5.3664 -4.7843 -4.1623 a40=-16.3551 -9.6206 -9.4014 -9.1574 -8.8968 -8.6244 -8.287 -6.7417 -6.3383 -5.8573 -5.3162 -4.7327 -4.1089 a41=-16.2617 -9.5951 -9.3781 -9.1353 -8.8758 -8.5869 -8.2075 -6.7001 -6.293 -5.8062 -5.2654 -4.6809 -4.0558 a42=-16.1682 -9.5723 -9.3562 -9.1144 -8.8559 -8.5026 -8.1358 -6.6589 -6.2491 -5.7536 -5.2146 -4.6289 -4.0023 a43=-16.0748 -9.5507 -9.3355 -9.0945 -8.7765 -8.4227 -8.0662 -6.6192 -6.1998 -5.7024 -5.1645 -4.5768 -3.9487 a44=-15.9813 -9.5302 -9.3157 -9.0279 -8.6918 -8.3492 -8.0027 -6.5773 -6.1511 -5.6511 -5.1145 -4.5248 -3.8948 a45=-15.8879 -9.5107 -9.2547 -8.9386 -8.6127 -8.2792 -7.9408 -6.5368 -6.0976 -5.6001 -5.0645 -4.4723 -3.8407 a46=-15.7944 -9.4378 -9.1579 -8.8552 -8.5389 -8.2143 -7.8841 -6.4969 -6.0462 -5.55 -5.0143 -4.4203 -3.7865 a47=-15.7009 -9.3428 -9.0722 -8.778 -8.4694 -8.152 -7.8283 -6.4516 -5.9927 -5.5004 -4.9635 -4.3673 -3.7323 a48=-15.6075 -9.253 -8.9914 -8.7051 -8.4042 -8.0941 -7.7772 -6.4022 -5.9406 -5.4512 -4.9126 -4.3142 -3.6781 a49=-15.514 -9.1724 -8.917 -8.637 -8.3423 -8.038 -7.7266 -6.3504 -5.8891 -5.4021 -4.861 -4.2615 -3.6236 a50=-15.4206 -9.0952 -8.8462 -8.5726 -8.2842 -7.986 -7.6797 -6.2966 -5.839 -5.3533 -4.8101 -4.2084 -3.5689 a701=45.4206 13.19 13.244 13.305 13.371 13.443 13.52 13.884 13.991 14.106 14.228 14.355 14.482 a702=45.514 13.201 13.255 13.316 13.383 13.454 13.531 13.894 14.001 14.116 14.237 14.364 14.491 a703=45.6075 13.213 13.266 13.327 13.394 13.465 13.541 13.904 14.011 14.126 14.247 14.373 14.501 a704=45.7009 13.224 13.278 13.338 13.405 13.476 13.552 13.914 14.021 14.136 14.257 14.383 14.51 a705=45.7944 13.236 13.289 13.35 13.416 13.487 13.563 13.925 14.031 14.145 14.266 14.392 14.519 a706=45.8879 13.247 13.3 13.361 13.427 13.498 13.574 13.935 14.041 14.155 14.276 14.402 14.528 a707=45.9813 13.258 13.312 13.372 13.438 13.509 13.585 13.945 14.051 14.165 14.285 14.411 14.538 a708=46.0748 13.27 13.323 13.383 13.449 13.52 13.595 13.955 14.061 14.175 14.295 14.42 14.547 a709=46.1682 13.281 13.334 13.394 13.46 13.531 13.606 13.965 14.071 14.184 14.305 14.43 14.556 a710=46.2617 13.292 13.345 13.405 13.471 13.541 13.617 13.975 14.081 14.194 14.314 14.439 14.565 a711=46.3551 13.303 13.356 13.416 13.482 13.552 13.628 13.985 14.091 14.204 14.324 14.449 14.574 a712=46.4486 13.315 13.368 13.427 13.493 13.563 13.638 13.995 14.101 14.214 14.333 14.458 14.583 a713=46.5421 13.326 13.379 13.438 13.504 13.574 13.649 14.005 14.111 14.223 14.343 14.467 14.592 a714=46.6355 13.337 13.39 13.449 13.515 13.585 13.659 14.016 14.121 14.233 14.352 14.476 14.601 a715=46.729 13.348 13.401 13.46 13.525 13.595 13.67 14.026 14.131 14.243 14.362 14.486 14.611 a716=46.8224 13.36 13.412 13.471 13.536 13.606 13.681 14.036 14.14 14.252 14.371 14.495 14.62 a717=46.9159 13.371 13.423 13.482 13.547 13.617 13.691 14.046 14.15 14.262 14.38 14.504 14.629 a718=47.0093 13.382 13.434 13.493 13.558 13.627 13.702 14.055 14.16 14.272 14.39 14.513 14.638 a719=47.1028 13.393 13.445 13.504 13.569 13.638 13.712 14.065 14.17 14.281 14.399 14.523 14.647 a720=47.1963 13.404 13.456 13.515 13.579 13.649 13.723 14.075 14.179 14.291 14.409 14.532 14.656 a721=47.2897 13.415 13.467 13.526 13.59 13.659 13.733 14.085 14.189 14.3 14.418 14.541 14.665 a722=47.3832 13.426 13.478 13.537 13.601 13.67 13.744 14.095 14.199 14.31 14.427 14.55 14.674 a723=47.4766 13.437 13.489 13.548 13.612 13.681 13.754 14.105 14.209 14.319 14.437 14.559 14.683 a724=47.5701 13.448 13.5 13.558 13.622 13.691 13.765 14.115 14.218 14.329 14.446 14.568 14.692 a725=47.6636 13.459 13.511 13.569 13.633 13.702 13.775 14.125 14.228 14.338 14.455 14.578 14.701 a726=47.757 13.47 13.522 13.58 13.644 13.712 13.786 14.135 14.238 14.348 14.465 14.587 14.709 a727=47.8505 13.481 13.532 13.591 13.654 13.723 13.796 14.144 14.247 14.357 14.474 14.596 14.718 a728=47.9439 13.492 13.543 13.601 13.665 13.733 13.806 14.154 14.257 14.367 14.483 14.605 14.727 a729=48.0374 13.503 13.554 13.612 13.676 13.744 13.817 14.164 14.267 14.376 14.493 14.614 14.736 a730=48.1308 13.514 13.565 13.623 13.686 13.754 13.827 14.174 14.276 14.386 14.502 14.623 14.745 a731=48.2243 13.525 13.576 13.634 13.697 13.765 13.838 14.184 14.286 14.395 14.511 14.632 14.754 a732=48.3178 13.535 13.586 13.644 13.707 13.775 13.848 14.193 14.295 14.404 14.52 14.641 14.763 a733=48.4112 13.546 13.597 13.655 13.718 13.786 13.858 14.203 14.305 14.414 14.529 14.65 14.772 a734=48.5047 13.557 13.608 13.665 13.728 13.796 13.868 14.213 14.314 14.423 14.539 14.659 14.78 a735=48.5981 13.568 13.619 13.676 13.739 13.806 13.879 14.222 14.324 14.433 14.548 14.668 14.789 a736=48.6916 13.579 13.629 13.687 13.749 13.817 13.889 14.232 14.333 14.442 14.557 14.677 14.798 a737=48.785 13.589 13.64 13.697 13.76 13.827 13.899 14.242 14.343 14.451 14.566 14.686 14.807 a738=48.8785 13.6 13.651 13.708 13.77 13.837 13.909 14.251 14.352 14.46 14.575 14.695 14.815 a739=48.972 13.611 13.661 13.718 13.781 13.848 13.919 14.261 14.362 14.47 14.584 14.704 14.824 a740=49.0654 13.622 13.672 13.729 13.791 13.858 13.93 14.271 14.371 14.479 14.593 14.713 14.833 a741=49.1589 13.632 13.682 13.739 13.801 13.868 13.94 14.28 14.381 14.488 14.603 14.722 14.842 a742=49.2523 13.643 13.693 13.75 13.812 13.878 13.95 14.29 14.39 14.498 14.612 14.731 14.85 a743=49.3458 13.654 13.704 13.76 13.822 13.889 13.96 14.299 14.4 14.507 14.621 14.739 14.859 a744=49.4393 13.664 13.714 13.771 13.832 13.899 13.97 14.309 14.409 14.516 14.63 14.748 14.868 a745=49.5327 13.675 13.725 13.781 13.843 13.909 13.98 14.318 14.418 14.525 14.639 14.757 14.876 a746=49.6262 13.685 13.735 13.791 13.853 13.919 13.99 14.328 14.428 14.534 14.648 14.766 14.885 a747=49.7196 13.696 13.746 13.802 13.863 13.929 14 14.337 14.437 14.544 14.657 14.775 14.894 a748=49.8131 13.706 13.756 13.812 13.874 13.94 14.01 14.347 14.446 14.553 14.666 14.784 14.902 a749=49.9065 13.717 13.766 13.823 13.884 13.95 14.02 14.356 14.456 14.562 14.675 14.792 14.911 a750=50 13.728 13.777 13.833 13.894 13.96 14.03 14.366 14.465 14.571 14.684 14.801 14.92 tb_pol_a: optimal asset choice for each state space point z1_0_34741 z2_0_40076 z3_0_4623 z4_0_5333 z5_0_61519 z6_0_70966 z10_1_2567 z11_1_4496 z12_1_6723 z13_1_9291 z14_2_2253 z15_2_567 __________ __________ _________ _________ __________ __________ __________ __________ __________ __________ __________ _________ a1=-20 0 0 0 0 0 0 0 0 0 0 -20 -20 a2=-19.9065 0 0 0 0 0 0 0 0 0 0 -20 -20 a3=-19.8131 0 0 0 0 0 0 0 0 0 -20 -20 -19.533 a4=-19.7196 0 0 0 0 0 0 0 0 0 -20 -20 -19.439 a5=-19.6262 0 0 0 0 0 0 0 0 0 -20 -20 -19.346 a6=-19.5327 0 0 0 0 0 0 0 0 -20 -20 -20 -19.252 a7=-19.4393 0 0 0 0 0 0 0 0 -20 -20 -20 -19.159 a8=-19.3458 0 0 0 0 0 0 0 -20 -20 -20 -20 -19.065 a9=-19.2523 0 0 0 0 0 0 0 -20 -20 -20 -20 -18.972 a10=-19.1589 0 0 0 0 0 0 -20 -20 -20 -20 -19.252 -18.972 a11=-19.0654 0 0 0 0 0 0 -20 -20 -20 -20 -19.159 -18.879 a12=-18.972 0 0 0 0 0 0 -20 -20 -20 -20 -19.065 -18.785 a13=-18.8785 0 0 0 0 0 0 -20 -20 -20 -20 -18.972 -18.598 a14=-18.785 0 0 0 0 0 0 -20 -20 -20 -20 -18.972 -18.505 a15=-18.6916 0 0 0 0 0 0 -20 -20 -20 -20 -18.879 -18.411 a16=-18.5981 0 0 0 0 0 -20 -20 -20 -20 -18.972 -18.785 -18.318 a17=-18.5047 0 0 0 0 -20 -20 -20 -20 -20 -18.972 -18.785 -18.318 a18=-18.4112 0 0 0 -20 -20 -20 -20 -20 -20 -18.879 -18.598 -18.224 a19=-18.3178 0 -20 -20 -20 -20 -20 -20 -20 -20 -18.785 -18.505 -18.037 a20=-18.2243 -20 -20 -20 -20 -20 -20 -20 -20 -20 -18.785 -18.411 -17.944 a21=-18.1308 -20 -20 -20 -20 -20 -20 -20 -20 -18.785 -18.692 -18.411 -17.85 a22=-18.0374 -20 -20 -20 -20 -20 -20 -20 -20 -18.785 -18.598 -18.318 -17.757 a23=-17.9439 -20 -20 -20 -20 -20 -20 -20 -20 -18.692 -18.505 -18.224 -17.664 a24=-17.8505 -20 -20 -20 -20 -20 -20 -20 -20 -18.692 -18.505 -18.037 -17.477 a25=-17.757 -20 -20 -20 -20 -20 -20 -20 -20 -18.598 -18.411 -17.944 -17.383 a26=-17.6636 -20 -20 -20 -20 -20 -20 -20 -18.598 -18.505 -18.318 -17.85 -17.29 a27=-17.5701 -20 -20 -20 -20 -20 -20 -20 -18.598 -18.505 -18.318 -17.664 -17.196 a28=-17.4766 -20 -20 -20 -20 -20 -20 -20 -18.505 -18.411 -18.037 -17.57 -17.103 a29=-17.3832 -20 -20 -20 -20 -20 -20 -20 -18.505 -18.411 -17.944 -17.477 -17.009 a30=-17.2897 -20 -20 -20 -20 -20 -20 -18.505 -18.411 -18.318 -17.944 -17.383 -16.916 a31=-17.1963 -20 -20 -20 -20 -20 -20 -18.411 -18.411 -18.224 -17.85 -17.29 -16.822 a32=-17.1028 -20 -20 -20 -20 -20 -20 -18.411 -18.318 -18.037 -17.57 -17.196 -16.729 a33=-17.0093 -20 -20 -20 -20 -20 -20 -18.318 -18.318 -18.037 -17.477 -17.103 -16.636 a34=-16.9159 -20 -20 -20 -20 -20 -20 -18.318 -18.224 -17.944 -17.477 -17.009 -16.542 a35=-16.8224 -20 -20 -20 -20 -20 -20 -18.224 -18.131 -17.85 -17.29 -16.916 -16.355 a36=-16.729 -20 -20 -20 -20 -20 -20 -18.224 -18.037 -17.57 -17.196 -16.822 -16.262 a37=-16.6355 -20 -20 -20 -20 -20 -20 -18.131 -17.944 -17.57 -17.103 -16.729 -16.168 a38=-16.5421 -20 -20 -20 -20 -20 -20 -18.037 -17.944 -17.477 -17.009 -16.636 -16.075 a39=-16.4486 -20 -20 -20 -20 -20 -20 -18.037 -17.85 -17.29 -16.916 -16.449 -15.981 a40=-16.3551 -20 -20 -20 -20 -20 -18.131 -17.944 -17.57 -17.196 -16.822 -16.355 -15.888 a41=-16.2617 -20 -20 -20 -20 -18.037 -18.037 -17.944 -17.57 -17.009 -16.729 -16.262 -15.794 a42=-16.1682 -20 -20 -20 -20 -18.037 -18.037 -17.85 -17.477 -16.916 -16.636 -16.168 -15.701 a43=-16.0748 -20 -20 -20 -17.944 -17.944 -17.944 -17.664 -17.196 -16.916 -16.542 -16.075 -15.514 a44=-15.9813 -20 -20 -17.944 -17.944 -17.944 -17.944 -17.57 -17.009 -16.729 -16.449 -15.981 -15.421 a45=-15.8879 -20 -17.85 -17.85 -17.85 -17.85 -17.85 -17.57 -16.916 -16.636 -16.355 -15.888 -15.327 a46=-15.7944 -17.85 -17.85 -17.85 -17.85 -17.85 -17.85 -17.29 -16.916 -16.542 -16.262 -15.794 -15.234 a47=-15.7009 -17.757 -17.757 -17.757 -17.757 -17.757 -17.757 -17.196 -16.729 -16.542 -16.075 -15.607 -15.14 a48=-15.6075 -17.757 -17.757 -17.757 -17.757 -17.757 -17.664 -16.916 -16.636 -16.355 -15.981 -15.514 -15.047 a49=-15.514 -17.664 -17.664 -17.664 -17.664 -17.664 -17.664 -16.729 -16.542 -16.262 -15.888 -15.421 -14.953 a50=-15.4206 -17.664 -17.664 -17.664 -17.664 -17.664 -17.57 -16.636 -16.449 -16.168 -15.794 -15.327 -14.86 a701=45.4206 42.897 42.897 42.991 42.991 43.084 43.178 43.738 43.925 44.206 44.393 44.766 45.14 a702=45.514 42.991 42.991 43.084 43.084 43.178 43.271 43.832 44.019 44.299 44.486 44.86 45.234 a703=45.6075 43.084 43.084 43.178 43.178 43.271 43.364 43.925 44.112 44.299 44.579 44.953 45.327 a704=45.7009 43.178 43.178 43.271 43.271 43.364 43.458 44.019 44.206 44.393 44.673 45.047 45.421 a705=45.7944 43.178 43.271 43.364 43.364 43.458 43.551 44.112 44.299 44.486 44.766 45.14 45.514 a706=45.8879 43.271 43.364 43.458 43.458 43.551 43.645 44.206 44.393 44.579 44.86 45.234 45.607 a707=45.9813 43.364 43.458 43.458 43.551 43.645 43.738 44.299 44.486 44.673 44.953 45.327 45.701 a708=46.0748 43.458 43.551 43.551 43.645 43.738 43.832 44.393 44.579 44.766 45.047 45.421 45.794 a709=46.1682 43.551 43.645 43.645 43.738 43.832 43.925 44.486 44.673 44.86 45.14 45.514 45.888 a710=46.2617 43.645 43.738 43.738 43.832 43.925 44.019 44.579 44.766 44.953 45.234 45.607 45.981 a711=46.3551 43.738 43.832 43.832 43.925 44.019 44.112 44.673 44.86 45.047 45.327 45.701 46.075 a712=46.4486 43.832 43.925 43.925 44.019 44.112 44.206 44.766 44.953 45.14 45.421 45.794 46.168 a713=46.5421 43.925 44.019 44.019 44.112 44.206 44.299 44.86 45.047 45.234 45.514 45.888 46.262 a714=46.6355 44.019 44.112 44.112 44.206 44.299 44.393 44.953 45.14 45.327 45.607 45.981 46.355 a715=46.729 44.112 44.206 44.206 44.299 44.393 44.486 44.953 45.234 45.421 45.701 46.075 46.449 a716=46.8224 44.206 44.299 44.299 44.393 44.486 44.579 45.047 45.327 45.514 45.794 46.075 46.542 a717=46.9159 44.299 44.393 44.393 44.486 44.579 44.673 45.14 45.421 45.607 45.888 46.168 46.636 a718=47.0093 44.393 44.486 44.486 44.579 44.673 44.766 45.234 45.514 45.701 45.981 46.262 46.729 a719=47.1028 44.486 44.579 44.579 44.673 44.766 44.86 45.327 45.607 45.794 46.075 46.355 46.729 a720=47.1963 44.579 44.673 44.673 44.766 44.86 44.953 45.421 45.607 45.888 46.168 46.449 46.822 a721=47.2897 44.673 44.766 44.766 44.86 44.953 45.047 45.514 45.701 45.981 46.262 46.542 46.916 a722=47.3832 44.766 44.766 44.86 44.953 45.047 45.047 45.607 45.794 46.075 46.355 46.636 47.009 a723=47.4766 44.86 44.86 44.953 45.047 45.047 45.14 45.701 45.888 46.168 46.449 46.729 47.103 a724=47.5701 44.953 44.953 45.047 45.14 45.14 45.234 45.794 45.981 46.262 46.542 46.822 47.196 a725=47.6636 45.047 45.047 45.14 45.234 45.234 45.327 45.888 46.075 46.355 46.636 46.916 47.29 a726=47.757 45.14 45.14 45.234 45.327 45.327 45.421 45.981 46.168 46.449 46.729 47.009 47.383 a727=47.8505 45.234 45.234 45.327 45.421 45.421 45.514 46.075 46.262 46.542 46.822 47.103 47.477 a728=47.9439 45.327 45.327 45.421 45.514 45.514 45.607 46.168 46.355 46.636 46.916 47.196 47.57 a729=48.0374 45.421 45.421 45.514 45.607 45.607 45.701 46.262 46.449 46.729 47.009 47.29 47.664 a730=48.1308 45.514 45.514 45.607 45.607 45.701 45.794 46.355 46.542 46.822 47.103 47.383 47.757 a731=48.2243 45.607 45.607 45.701 45.701 45.794 45.888 46.449 46.636 46.916 47.196 47.477 47.85 a732=48.3178 45.701 45.701 45.794 45.794 45.888 45.981 46.542 46.729 47.009 47.29 47.57 47.944 a733=48.4112 45.794 45.794 45.888 45.888 45.981 46.075 46.636 46.822 47.103 47.383 47.664 48.037 a734=48.5047 45.888 45.888 45.981 45.981 46.075 46.168 46.729 46.916 47.196 47.477 47.757 48.131 a735=48.5981 45.981 45.981 46.075 46.075 46.168 46.262 46.822 47.009 47.29 47.57 47.85 48.224 a736=48.6916 46.075 46.075 46.168 46.168 46.262 46.355 46.916 47.103 47.383 47.57 47.944 48.318 a737=48.785 46.168 46.168 46.262 46.262 46.355 46.449 47.009 47.196 47.477 47.664 48.037 48.411 a738=48.8785 46.262 46.262 46.355 46.355 46.449 46.542 47.103 47.29 47.477 47.757 48.131 48.505 a739=48.972 46.262 46.355 46.449 46.449 46.542 46.636 47.196 47.383 47.57 47.85 48.224 48.598 a740=49.0654 46.355 46.449 46.542 46.542 46.636 46.729 47.29 47.477 47.664 47.944 48.318 48.692 a741=49.1589 46.449 46.542 46.542 46.636 46.729 46.822 47.383 47.57 47.757 48.037 48.411 48.785 a742=49.2523 46.542 46.636 46.636 46.729 46.822 46.916 47.477 47.664 47.85 48.131 48.505 48.879 a743=49.3458 46.636 46.729 46.729 46.822 46.916 47.009 47.57 47.757 47.944 48.224 48.598 48.972 a744=49.4393 46.729 46.822 46.822 46.916 47.009 47.103 47.664 47.85 48.037 48.318 48.692 49.065 a745=49.5327 46.822 46.916 46.916 47.009 47.103 47.196 47.757 47.944 48.131 48.411 48.785 49.159 a746=49.6262 46.916 47.009 47.009 47.103 47.196 47.29 47.85 48.037 48.224 48.505 48.879 49.252 a747=49.7196 47.009 47.103 47.103 47.196 47.29 47.383 47.944 48.131 48.318 48.598 48.972 49.346 a748=49.8131 47.103 47.196 47.196 47.29 47.383 47.477 48.037 48.224 48.411 48.692 49.065 49.439 a749=49.9065 47.196 47.29 47.29 47.383 47.477 47.57 48.131 48.318 48.505 48.785 49.159 49.533 a750=50 47.29 47.383 47.383 47.477 47.57 47.664 48.131 48.411 48.598 48.879 49.252 49.626 mt_pol_b_bridge_print: bridge loans z1_0_34741 z2_0_40076 z3_0_4623 z4_0_5333 z5_0_61519 z6_0_70966 z10_1_2567 z11_1_4496 z12_1_6723 z13_1_9291 z14_2_2253 z15_2_567 __________ __________ _________ _________ __________ __________ __________ __________ __________ __________ __________ _________ coh1=-19.5553 0 0 0 0 0 0 0 0 0 0 -18.781 -18.302 coh2=-19.4619 0 0 0 0 0 0 0 0 0 0 -18.679 -18.2 coh3=-19.3684 0 0 0 0 0 0 0 0 0 -18.992 -18.576 -18.097 coh4=-19.2749 0 0 0 0 0 0 0 0 0 -18.889 -18.474 -17.995 coh5=-19.1815 0 0 0 0 0 0 0 0 0 -18.787 -18.372 -17.893 coh6=-19.088 0 0 0 0 0 0 0 0 -19.044 -18.685 -18.269 -17.79 coh7=-18.9946 0 0 0 0 0 0 0 0 -18.942 -18.582 -18.167 -17.688 coh8=-18.9011 0 0 0 0 0 0 0 -19.152 -18.84 -18.48 -18.065 -17.586 coh9=-18.8077 0 0 0 0 0 0 0 -19.049 -18.737 -18.378 -17.962 -17.483 coh10=-18.7142 0 0 0 0 0 0 -19.218 -18.947 -18.635 -18.275 -17.86 -17.381 coh11=-18.6207 0 0 0 0 0 0 -19.115 -18.845 -18.533 -18.173 -17.758 -17.279 coh12=-18.5273 0 0 0 0 0 0 -19.013 -18.742 -18.43 -18.071 -17.655 -17.176 coh13=-18.4338 0 0 0 0 0 0 -18.911 -18.64 -18.328 -17.968 -17.553 -17.074 coh14=-18.3404 0 0 0 0 0 0 -18.808 -18.538 -18.226 -17.866 -17.451 -16.972 coh15=-18.2469 0 0 0 0 0 0 -18.706 -18.435 -18.123 -17.764 -17.348 -16.869 coh16=-18.1534 0 0 0 0 0 -19.37 -18.604 -18.333 -18.021 -17.661 -17.246 -16.767 coh17=-18.06 0 0 0 0 -19.4 -19.268 -18.501 -18.231 -17.919 -17.559 -17.144 -16.665 coh18=-17.9665 0 0 0 -19.413 -19.298 -19.166 -18.399 -18.128 -17.816 -17.457 -17.041 -16.562 coh19=-17.8731 0 -19.496 -19.41 -19.31 -19.196 -19.063 -18.297 -18.026 -17.714 -17.354 -16.939 -16.46 coh20=-17.7796 -19.469 -19.394 -19.308 -19.208 -19.093 -18.961 -18.194 -17.924 -17.612 -17.252 -16.837 -16.358 coh21=-17.6862 -19.366 -19.292 -19.205 -19.106 -18.991 -18.859 -18.092 -17.821 -17.509 -17.149 -16.734 -16.255 coh22=-17.5927 -19.264 -19.189 -19.103 -19.003 -18.889 -18.756 -17.99 -17.719 -17.407 -17.047 -16.632 -16.153 coh23=-17.4992 -19.162 -19.087 -19.001 -18.901 -18.786 -18.654 -17.887 -17.617 -17.305 -16.945 -16.53 -16.051 coh24=-17.4058 -19.059 -18.985 -18.898 -18.799 -18.684 -18.552 -17.785 -17.514 -17.202 -16.842 -16.427 -15.948 coh25=-17.3123 -18.957 -18.882 -18.796 -18.696 -18.582 -18.449 -17.683 -17.412 -17.1 -16.74 -16.325 -15.846 coh26=-17.2189 -18.855 -18.78 -18.694 -18.594 -18.479 -18.347 -17.58 -17.31 -16.998 -16.638 -16.223 -15.744 coh27=-17.1254 -18.752 -18.678 -18.591 -18.492 -18.377 -18.245 -17.478 -17.207 -16.895 -16.535 -16.12 -15.641 coh28=-17.032 -18.65 -18.575 -18.489 -18.389 -18.275 -18.142 -17.376 -17.105 -16.793 -16.433 -16.018 -15.539 coh29=-16.9385 -18.548 -18.473 -18.387 -18.287 -18.172 -18.04 -17.273 -17.003 -16.691 -16.331 -15.916 -15.437 coh30=-16.845 -18.445 -18.371 -18.284 -18.185 -18.07 -17.938 -17.171 -16.9 -16.588 -16.228 -15.813 -15.334 coh31=-16.7516 -18.343 -18.268 -18.182 -18.082 -17.968 -17.835 -17.069 -16.798 -16.486 -16.126 -15.711 -15.232 coh32=-16.6581 -18.241 -18.166 -18.08 -17.98 -17.865 -17.733 -16.966 -16.696 -16.384 -16.024 -15.609 -15.13 coh33=-16.5647 -18.138 -18.064 -17.977 -17.878 -17.763 -17.631 -16.864 -16.593 -16.281 -15.921 -15.506 -15.027 coh34=-16.4712 -18.036 -17.961 -17.875 -17.775 -17.661 -17.528 -16.762 -16.491 -16.179 -15.819 -15.404 -14.925 coh35=-16.3777 -17.934 -17.859 -17.773 -17.673 -17.558 -17.426 -16.659 -16.389 -16.077 -15.717 -15.302 -14.823 coh36=-16.2843 -17.831 -17.757 -17.67 -17.571 -17.456 -17.324 -16.557 -16.286 -15.974 -15.614 -15.199 -14.72 coh37=-16.1908 -17.729 -17.654 -17.568 -17.468 -17.354 -17.221 -16.455 -16.184 -15.872 -15.512 -15.097 -14.618 coh38=-16.0974 -17.627 -17.552 -17.466 -17.366 -17.251 -17.119 -16.352 -16.082 -15.77 -15.41 -14.995 -14.516 coh39=-16.0039 -17.524 -17.45 -17.363 -17.264 -17.149 -17.017 -16.25 -15.979 -15.667 -15.307 -14.892 -14.413 coh40=-15.9105 -17.422 -17.347 -17.261 -17.161 -17.047 -16.914 -16.148 -15.877 -15.565 -15.205 -14.79 -14.311 coh41=-15.817 -17.32 -17.245 -17.159 -17.059 -16.944 -16.812 -16.045 -15.775 -15.463 -15.103 -14.688 -14.209 coh42=-15.7235 -17.217 -17.143 -17.056 -16.957 -16.842 -16.71 -15.943 -15.672 -15.36 -15 -14.585 -14.106 coh43=-15.6301 -17.115 -17.04 -16.954 -16.854 -16.74 -16.607 -15.841 -15.57 -15.258 -14.898 -14.483 -14.004 coh44=-15.5366 -17.013 -16.938 -16.852 -16.752 -16.637 -16.505 -15.738 -15.468 -15.156 -14.796 -14.381 -13.902 coh45=-15.4432 -16.91 -16.835 -16.749 -16.65 -16.535 -16.403 -15.636 -15.365 -15.053 -14.693 -14.278 -13.799 coh46=-15.3497 -16.808 -16.733 -16.647 -16.547 -16.433 -16.3 -15.534 -15.263 -14.951 -14.591 -14.176 -13.697 coh47=-15.2563 -16.706 -16.631 -16.545 -16.445 -16.33 -16.198 -15.431 -15.161 -14.849 -14.489 -14.074 -13.595 coh48=-15.1628 -16.603 -16.528 -16.442 -16.343 -16.228 -16.096 -15.329 -15.058 -14.746 -14.386 -13.971 -13.492 coh49=-15.0693 -16.501 -16.426 -16.34 -16.24 -16.126 -15.993 -15.227 -14.956 -14.644 -14.284 -13.869 -13.39 coh50=-14.9759 -16.399 -16.324 -16.238 -16.138 -16.023 -15.891 -15.124 -14.854 -14.542 -14.182 -13.767 -13.288 coh701=45.8652 0 0 0 0 0 0 0 0 0 0 0 0 coh702=45.9587 0 0 0 0 0 0 0 0 0 0 0 0 coh703=46.0522 0 0 0 0 0 0 0 0 0 0 0 0 coh704=46.1456 0 0 0 0 0 0 0 0 0 0 0 0 coh705=46.2391 0 0 0 0 0 0 0 0 0 0 0 0 coh706=46.3325 0 0 0 0 0 0 0 0 0 0 0 0 coh707=46.426 0 0 0 0 0 0 0 0 0 0 0 0 coh708=46.5195 0 0 0 0 0 0 0 0 0 0 0 0 coh709=46.6129 0 0 0 0 0 0 0 0 0 0 0 0 coh710=46.7064 0 0 0 0 0 0 0 0 0 0 0 0 coh711=46.7998 0 0 0 0 0 0 0 0 0 0 0 0 coh712=46.8933 0 0 0 0 0 0 0 0 0 0 0 0 coh713=46.9867 0 0 0 0 0 0 0 0 0 0 0 0 coh714=47.0802 0 0 0 0 0 0 0 0 0 0 0 0 coh715=47.1737 0 0 0 0 0 0 0 0 0 0 0 0 coh716=47.2671 0 0 0 0 0 0 0 0 0 0 0 0 coh717=47.3606 0 0 0 0 0 0 0 0 0 0 0 0 coh718=47.454 0 0 0 0 0 0 0 0 0 0 0 0 coh719=47.5475 0 0 0 0 0 0 0 0 0 0 0 0 coh720=47.6409 0 0 0 0 0 0 0 0 0 0 0 0 coh721=47.7344 0 0 0 0 0 0 0 0 0 0 0 0 coh722=47.8279 0 0 0 0 0 0 0 0 0 0 0 0 coh723=47.9213 0 0 0 0 0 0 0 0 0 0 0 0 coh724=48.0148 0 0 0 0 0 0 0 0 0 0 0 0 coh725=48.1082 0 0 0 0 0 0 0 0 0 0 0 0 coh726=48.2017 0 0 0 0 0 0 0 0 0 0 0 0 coh727=48.2952 0 0 0 0 0 0 0 0 0 0 0 0 coh728=48.3886 0 0 0 0 0 0 0 0 0 0 0 0 coh729=48.4821 0 0 0 0 0 0 0 0 0 0 0 0 coh730=48.5755 0 0 0 0 0 0 0 0 0 0 0 0 coh731=48.669 0 0 0 0 0 0 0 0 0 0 0 0 coh732=48.7624 0 0 0 0 0 0 0 0 0 0 0 0 coh733=48.8559 0 0 0 0 0 0 0 0 0 0 0 0 coh734=48.9494 0 0 0 0 0 0 0 0 0 0 0 0 coh735=49.0428 0 0 0 0 0 0 0 0 0 0 0 0 coh736=49.1363 0 0 0 0 0 0 0 0 0 0 0 0 coh737=49.2297 0 0 0 0 0 0 0 0 0 0 0 0 coh738=49.3232 0 0 0 0 0 0 0 0 0 0 0 0 coh739=49.4166 0 0 0 0 0 0 0 0 0 0 0 0 coh740=49.5101 0 0 0 0 0 0 0 0 0 0 0 0 coh741=49.6036 0 0 0 0 0 0 0 0 0 0 0 0 coh742=49.697 0 0 0 0 0 0 0 0 0 0 0 0 coh743=49.7905 0 0 0 0 0 0 0 0 0 0 0 0 coh744=49.8839 0 0 0 0 0 0 0 0 0 0 0 0 coh745=49.9774 0 0 0 0 0 0 0 0 0 0 0 0 coh746=50.0709 0 0 0 0 0 0 0 0 0 0 0 0 coh747=50.1643 0 0 0 0 0 0 0 0 0 0 0 0 coh748=50.2578 0 0 0 0 0 0 0 0 0 0 0 0 coh749=50.3512 0 0 0 0 0 0 0 0 0 0 0 0 coh750=50.4447 0 0 0 0 0 0 0 0 0 0 0 0 mt_pol_inf_borr_nobridge_print: Informal loans that is not bridge loan z1_0_34741 z2_0_40076 z3_0_4623 z4_0_5333 z5_0_61519 z6_0_70966 z10_1_2567 z11_1_4496 z12_1_6723 z13_1_9291 z14_2_2253 z15_2_567 __________ __________ _________ _________ __________ __________ __________ __________ __________ __________ __________ _________ coh1=-19.5553 0 0 0 0 0 0 0 0 0 0 -0.15398 -0.63294 coh2=-19.4619 0 0 0 0 0 0 0 0 0 0 -0.25631 -0.73528 coh3=-19.3684 0 0 0 0 0 0 0 0 0 0 -0.35865 -0.37033 coh4=-19.2749 0 0 0 0 0 0 0 0 0 -0.045783 -0.46099 -0.37921 coh5=-19.1815 0 0 0 0 0 0 0 0 0 -0.14812 -0.56332 -0.38808 coh6=-19.088 0 0 0 0 0 0 0 0 0 -0.25046 -0.66566 -0.39696 coh7=-18.9946 0 0 0 0 0 0 0 0 0 -0.35279 -0.768 -0.40584 coh8=-18.9011 0 0 0 0 0 0 0 0 -0.095197 -0.45513 -0.87033 -0.41472 coh9=-18.8077 0 0 0 0 0 0 0 0 -0.19753 -0.55747 0 -0.4236 coh10=-18.7142 0 0 0 0 0 0 0 0 -0.29987 -0.6598 -0.32734 -0.52594 coh11=-18.6207 0 0 0 0 0 0 0 -0.090189 -0.40221 -0.76214 -0.33622 -0.53481 coh12=-18.5273 0 0 0 0 0 0 0 -0.19253 -0.50454 -0.86447 -0.3451 -0.54369 coh13=-18.4338 0 0 0 0 0 0 -0.024381 -0.29486 -0.60688 0 -0.35398 -0.45911 coh14=-18.3404 0 0 0 0 0 0 -0.12672 -0.3972 -0.70922 0 -0.45631 -0.46799 coh15=-18.2469 0 0 0 0 0 0 -0.22905 -0.49953 -0.81155 0 -0.46519 -0.47687 coh16=-18.1534 0 0 0 0 0 0 -0.33139 -0.60187 -0.91389 -0.24578 -0.47407 -0.48575 coh17=-18.06 0 0 0 0 -0.59963 0 -0.43373 -0.70421 0 -0.34812 -0.57641 -0.58808 coh18=-17.9665 0 0 0 -0.58719 0 0 -0.53606 -0.80654 0 -0.357 -0.49183 -0.59696 coh19=-17.8731 0 -0.50376 -0.59002 0 0 0 -0.6384 -0.90888 0 -0.36588 -0.50071 -0.51238 coh20=-17.7796 -0.53132 -0.6061 0 0 0 0 -0.74074 0 0 -0.46821 -0.50959 -0.52126 coh21=-17.6862 0 0 0 0 0 -0.076392 -0.84307 0 -0.21062 -0.47709 -0.61192 -0.53014 coh22=-17.5927 0 0 0 0 -0.046317 -0.17873 0 0 -0.31295 -0.48597 -0.6208 -0.53902 coh23=-17.4992 0 0 0 -0.033868 -0.14865 -0.28106 0 0 -0.32183 -0.49485 -0.62968 -0.5479 coh24=-17.4058 0 0 -0.0367 -0.1362 -0.25099 -0.3834 0 0 -0.42417 -0.59718 -0.5451 -0.46332 coh25=-17.3123 0 -0.052779 -0.13904 -0.23854 -0.35333 -0.48574 0 0 -0.43305 -0.60606 -0.55398 -0.4722 coh26=-17.2189 -0.08034 -0.15512 -0.24137 -0.34088 -0.45566 -0.58807 0 -0.22337 -0.44193 -0.61494 -0.56286 -0.48108 coh27=-17.1254 -0.18268 -0.25745 -0.34371 -0.44321 -0.558 -0.69041 0 -0.3257 -0.54426 -0.71728 -0.47828 -0.48995 coh28=-17.032 -0.28501 -0.35979 -0.44605 -0.54555 -0.66034 -0.79275 0 -0.33458 -0.55314 -0.53924 -0.48716 -0.49883 coh29=-16.9385 -0.38735 -0.46212 -0.54838 -0.64789 -0.76267 -0.89508 -0.064264 -0.43692 -0.65548 -0.54812 -0.49603 -0.50771 coh30=-16.845 -0.48969 -0.56446 -0.65072 -0.75022 -0.86501 0 -0.26877 -0.4458 -0.66436 -0.65046 -0.50491 -0.51659 coh31=-16.7516 -0.59202 -0.6668 -0.75306 -0.85256 0 0 -0.27765 -0.54813 -0.67323 -0.65933 -0.51379 -0.52547 coh32=-16.6581 -0.69436 -0.76913 -0.85539 0 0 0 -0.37999 -0.55701 -0.58865 -0.4813 -0.52267 -0.53435 coh33=-16.5647 -0.7967 -0.87147 0 0 0 0 -0.38887 -0.65935 -0.69099 -0.49018 -0.53155 -0.54322 coh34=-16.4712 -0.89903 0 0 0 0 0 -0.4912 -0.66823 -0.69987 -0.59251 -0.54043 -0.5521 coh35=-16.3777 0 0 0 0 0 0 -0.50008 -0.6771 -0.70875 -0.50793 -0.54931 -0.46752 coh36=-16.2843 0 0 0 0 0 -0.013938 -0.60242 -0.68598 -0.53071 -0.51681 -0.55818 -0.4764 coh37=-16.1908 0 0 0 0 0 -0.11627 -0.6113 -0.69486 -0.63305 -0.52569 -0.56706 -0.48528 coh38=-16.0974 0 0 0 0 -0.0862 -0.21861 -0.62018 -0.7972 -0.64193 -0.53457 -0.57594 -0.49416 coh39=-16.0039 0 0 0 -0.073751 -0.18854 -0.32095 -0.72251 -0.80608 -0.55735 -0.54345 -0.49136 -0.50304 coh40=-15.9105 0 0 -0.076584 -0.17609 -0.29087 -0.15163 -0.73139 -0.62804 -0.56622 -0.55232 -0.50024 -0.51192 coh41=-15.817 -0.017887 -0.092662 -0.17892 -0.27842 -0.028092 -0.1605 -0.83373 -0.73038 -0.48165 -0.5612 -0.50912 -0.52079 coh42=-15.7235 -0.12022 -0.195 -0.28126 -0.38076 -0.13043 -0.26284 -0.84261 -0.73925 -0.49052 -0.57008 -0.518 -0.52967 coh43=-15.6301 -0.22256 -0.29733 -0.38359 -0.024522 -0.13931 -0.27172 -0.75803 -0.56122 -0.59286 -0.57896 -0.52688 -0.44509 coh44=-15.5366 -0.3249 -0.39967 -0.027355 -0.12686 -0.24164 -0.37406 -0.7669 -0.47664 -0.50828 -0.58784 -0.53575 -0.45397 coh45=-15.4432 -0.42723 0 -0.036233 -0.13574 -0.25052 -0.38293 -0.86924 -0.48552 -0.51716 -0.59672 -0.54463 -0.46285 coh46=-15.3497 0 -0.052312 -0.13857 -0.23807 -0.35286 -0.48527 -0.6912 -0.58785 -0.52604 -0.6056 -0.55351 -0.47173 coh47=-15.2563 0 -0.06119 -0.14745 -0.24695 -0.36174 -0.49415 -0.70008 -0.50327 -0.62837 -0.52102 -0.46893 -0.48061 coh48=-15.1628 -0.088751 -0.16353 -0.24978 -0.34929 -0.46407 -0.50303 -0.52204 -0.51215 -0.54379 -0.5299 -0.47781 -0.48949 coh49=-15.0693 -0.09763 -0.1724 -0.25866 -0.35817 -0.47295 -0.60536 -0.43747 -0.52103 -0.55267 -0.53877 -0.48669 -0.49836 coh50=-14.9759 -0.19997 -0.27474 -0.361 -0.4605 -0.57529 -0.61424 -0.44634 -0.52991 -0.56155 -0.54765 -0.49557 -0.50724 coh701=45.8652 0 0 0 0 0 0 0 0 0 0 0 0 coh702=45.9587 0 0 0 0 0 0 0 0 0 0 0 0 coh703=46.0522 0 0 0 0 0 0 0 0 0 0 0 0 coh704=46.1456 0 0 0 0 0 0 0 0 0 0 0 0 coh705=46.2391 0 0 0 0 0 0 0 0 0 0 0 0 coh706=46.3325 0 0 0 0 0 0 0 0 0 0 0 0 coh707=46.426 0 0 0 0 0 0 0 0 0 0 0 0 coh708=46.5195 0 0 0 0 0 0 0 0 0 0 0 0 coh709=46.6129 0 0 0 0 0 0 0 0 0 0 0 0 coh710=46.7064 0 0 0 0 0 0 0 0 0 0 0 0 coh711=46.7998 0 0 0 0 0 0 0 0 0 0 0 0 coh712=46.8933 0 0 0 0 0 0 0 0 0 0 0 0 coh713=46.9867 0 0 0 0 0 0 0 0 0 0 0 0 coh714=47.0802 0 0 0 0 0 0 0 0 0 0 0 0 coh715=47.1737 0 0 0 0 0 0 0 0 0 0 0 0 coh716=47.2671 0 0 0 0 0 0 0 0 0 0 0 0 coh717=47.3606 0 0 0 0 0 0 0 0 0 0 0 0 coh718=47.454 0 0 0 0 0 0 0 0 0 0 0 0 coh719=47.5475 0 0 0 0 0 0 0 0 0 0 0 0 coh720=47.6409 0 0 0 0 0 0 0 0 0 0 0 0 coh721=47.7344 0 0 0 0 0 0 0 0 0 0 0 0 coh722=47.8279 0 0 0 0 0 0 0 0 0 0 0 0 coh723=47.9213 0 0 0 0 0 0 0 0 0 0 0 0 coh724=48.0148 0 0 0 0 0 0 0 0 0 0 0 0 coh725=48.1082 0 0 0 0 0 0 0 0 0 0 0 0 coh726=48.2017 0 0 0 0 0 0 0 0 0 0 0 0 coh727=48.2952 0 0 0 0 0 0 0 0 0 0 0 0 coh728=48.3886 0 0 0 0 0 0 0 0 0 0 0 0 coh729=48.4821 0 0 0 0 0 0 0 0 0 0 0 0 coh730=48.5755 0 0 0 0 0 0 0 0 0 0 0 0 coh731=48.669 0 0 0 0 0 0 0 0 0 0 0 0 coh732=48.7624 0 0 0 0 0 0 0 0 0 0 0 0 coh733=48.8559 0 0 0 0 0 0 0 0 0 0 0 0 coh734=48.9494 0 0 0 0 0 0 0 0 0 0 0 0 coh735=49.0428 0 0 0 0 0 0 0 0 0 0 0 0 coh736=49.1363 0 0 0 0 0 0 0 0 0 0 0 0 coh737=49.2297 0 0 0 0 0 0 0 0 0 0 0 0 coh738=49.3232 0 0 0 0 0 0 0 0 0 0 0 0 coh739=49.4166 0 0 0 0 0 0 0 0 0 0 0 0 coh740=49.5101 0 0 0 0 0 0 0 0 0 0 0 0 coh741=49.6036 0 0 0 0 0 0 0 0 0 0 0 0 coh742=49.697 0 0 0 0 0 0 0 0 0 0 0 0 coh743=49.7905 0 0 0 0 0 0 0 0 0 0 0 0 coh744=49.8839 0 0 0 0 0 0 0 0 0 0 0 0 coh745=49.9774 0 0 0 0 0 0 0 0 0 0 0 0 coh746=50.0709 0 0 0 0 0 0 0 0 0 0 0 0 coh747=50.1643 0 0 0 0 0 0 0 0 0 0 0 0 coh748=50.2578 0 0 0 0 0 0 0 0 0 0 0 0 coh749=50.3512 0 0 0 0 0 0 0 0 0 0 0 0 coh750=50.4447 0 0 0 0 0 0 0 0 0 0 0 0 mt_pol_for_borr_print: formal borrowing z1_0_34741 z2_0_40076 z3_0_4623 z4_0_5333 z5_0_61519 z6_0_70966 z10_1_2567 z11_1_4496 z12_1_6723 z13_1_9291 z14_2_2253 z15_2_567 __________ __________ _________ _________ __________ __________ __________ __________ __________ __________ __________ _________ coh1=-19.5553 0 0 0 0 0 0 0 0 0 0 -1.065 -1.065 coh2=-19.4619 0 0 0 0 0 0 0 0 0 0 -1.065 -1.065 coh3=-19.3684 0 0 0 0 0 0 0 0 0 -1.065 -1.065 -1.065 coh4=-19.2749 0 0 0 0 0 0 0 0 0 -1.065 -1.065 -1.065 coh5=-19.1815 0 0 0 0 0 0 0 0 0 -1.065 -1.065 -1.065 coh6=-19.088 0 0 0 0 0 0 0 0 -1.065 -1.065 -1.065 -1.065 coh7=-18.9946 0 0 0 0 0 0 0 0 -1.065 -1.065 -1.065 -1.065 coh8=-18.9011 0 0 0 0 0 0 0 -1.065 -1.065 -1.065 -1.065 -1.065 coh9=-18.8077 0 0 0 0 0 0 0 -1.065 -1.065 -1.065 -2.6625 -1.065 coh10=-18.7142 0 0 0 0 0 0 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 coh11=-18.6207 0 0 0 0 0 0 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 coh12=-18.5273 0 0 0 0 0 0 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 coh13=-18.4338 0 0 0 0 0 0 -1.065 -1.065 -1.065 -2.6625 -1.065 -1.065 coh14=-18.3404 0 0 0 0 0 0 -1.065 -1.065 -1.065 -2.6625 -1.065 -1.065 coh15=-18.2469 0 0 0 0 0 0 -1.065 -1.065 -1.065 -2.6625 -1.065 -1.065 coh16=-18.1534 0 0 0 0 0 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 coh17=-18.06 0 0 0 0 0 -1.065 -1.065 -1.065 -2.6625 -1.065 -1.065 -1.065 coh18=-17.9665 0 0 0 0 -1.065 -1.065 -1.065 -1.065 -2.6625 -1.065 -1.065 -1.065 coh19=-17.8731 0 0 0 -1.065 -1.065 -1.065 -1.065 -1.065 -2.6625 -1.065 -1.065 -1.065 coh20=-17.7796 0 0 -1.065 -1.065 -1.065 -1.065 -1.065 -2.6625 -2.6625 -1.065 -1.065 -1.065 coh21=-17.6862 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 -2.6625 -1.065 -1.065 -1.065 -1.065 coh22=-17.5927 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 -2.6625 -2.6625 -1.065 -1.065 -1.065 -1.065 coh23=-17.4992 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 -2.6625 -2.6625 -1.065 -1.065 -1.065 -1.065 coh24=-17.4058 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 -2.6625 -2.6625 -1.065 -1.065 -1.065 -1.065 coh25=-17.3123 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 -2.6625 -2.6625 -1.065 -1.065 -1.065 -1.065 coh26=-17.2189 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 -2.6625 -1.065 -1.065 -1.065 -1.065 -1.065 coh27=-17.1254 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 -2.6625 -1.065 -1.065 -1.065 -1.065 -1.065 coh28=-17.032 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 -2.6625 -1.065 -1.065 -1.065 -1.065 -1.065 coh29=-16.9385 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 -2.6625 -1.065 -1.065 -1.065 -1.065 -1.065 coh30=-16.845 -1.065 -1.065 -1.065 -1.065 -1.065 -2.6625 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 coh31=-16.7516 -1.065 -1.065 -1.065 -1.065 -2.6625 -2.6625 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 coh32=-16.6581 -1.065 -1.065 -1.065 -2.6625 -2.6625 -2.6625 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 coh33=-16.5647 -1.065 -1.065 -2.6625 -2.6625 -2.6625 -2.6625 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 coh34=-16.4712 -1.065 -2.6625 -2.6625 -2.6625 -2.6625 -2.6625 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 coh35=-16.3777 -2.6625 -2.6625 -2.6625 -2.6625 -2.6625 -2.6625 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 coh36=-16.2843 -2.6625 -2.6625 -2.6625 -2.6625 -2.6625 -2.6625 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 coh37=-16.1908 -2.6625 -2.6625 -2.6625 -2.6625 -2.6625 -2.6625 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 coh38=-16.0974 -2.6625 -2.6625 -2.6625 -2.6625 -2.6625 -2.6625 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 coh39=-16.0039 -2.6625 -2.6625 -2.6625 -2.6625 -2.6625 -2.6625 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 coh40=-15.9105 -2.6625 -2.6625 -2.6625 -2.6625 -2.6625 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 coh41=-15.817 -2.6625 -2.6625 -2.6625 -2.6625 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 coh42=-15.7235 -2.6625 -2.6625 -2.6625 -2.6625 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 coh43=-15.6301 -2.6625 -2.6625 -2.6625 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 coh44=-15.5366 -2.6625 -2.6625 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 coh45=-15.4432 -2.6625 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 coh46=-15.3497 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 coh47=-15.2563 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 coh48=-15.1628 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 coh49=-15.0693 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 coh50=-14.9759 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 -1.065 coh701=45.8652 0 0 0 0 0 0 0 0 0 0 0 0 coh702=45.9587 0 0 0 0 0 0 0 0 0 0 0 0 coh703=46.0522 0 0 0 0 0 0 0 0 0 0 0 0 coh704=46.1456 0 0 0 0 0 0 0 0 0 0 0 0 coh705=46.2391 0 0 0 0 0 0 0 0 0 0 0 0 coh706=46.3325 0 0 0 0 0 0 0 0 0 0 0 0 coh707=46.426 0 0 0 0 0 0 0 0 0 0 0 0 coh708=46.5195 0 0 0 0 0 0 0 0 0 0 0 0 coh709=46.6129 0 0 0 0 0 0 0 0 0 0 0 0 coh710=46.7064 0 0 0 0 0 0 0 0 0 0 0 0 coh711=46.7998 0 0 0 0 0 0 0 0 0 0 0 0 coh712=46.8933 0 0 0 0 0 0 0 0 0 0 0 0 coh713=46.9867 0 0 0 0 0 0 0 0 0 0 0 0 coh714=47.0802 0 0 0 0 0 0 0 0 0 0 0 0 coh715=47.1737 0 0 0 0 0 0 0 0 0 0 0 0 coh716=47.2671 0 0 0 0 0 0 0 0 0 0 0 0 coh717=47.3606 0 0 0 0 0 0 0 0 0 0 0 0 coh718=47.454 0 0 0 0 0 0 0 0 0 0 0 0 coh719=47.5475 0 0 0 0 0 0 0 0 0 0 0 0 coh720=47.6409 0 0 0 0 0 0 0 0 0 0 0 0 coh721=47.7344 0 0 0 0 0 0 0 0 0 0 0 0 coh722=47.8279 0 0 0 0 0 0 0 0 0 0 0 0 coh723=47.9213 0 0 0 0 0 0 0 0 0 0 0 0 coh724=48.0148 0 0 0 0 0 0 0 0 0 0 0 0 coh725=48.1082 0 0 0 0 0 0 0 0 0 0 0 0 coh726=48.2017 0 0 0 0 0 0 0 0 0 0 0 0 coh727=48.2952 0 0 0 0 0 0 0 0 0 0 0 0 coh728=48.3886 0 0 0 0 0 0 0 0 0 0 0 0 coh729=48.4821 0 0 0 0 0 0 0 0 0 0 0 0 coh730=48.5755 0 0 0 0 0 0 0 0 0 0 0 0 coh731=48.669 0 0 0 0 0 0 0 0 0 0 0 0 coh732=48.7624 0 0 0 0 0 0 0 0 0 0 0 0 coh733=48.8559 0 0 0 0 0 0 0 0 0 0 0 0 coh734=48.9494 0 0 0 0 0 0 0 0 0 0 0 0 coh735=49.0428 0 0 0 0 0 0 0 0 0 0 0 0 coh736=49.1363 0 0 0 0 0 0 0 0 0 0 0 0 coh737=49.2297 0 0 0 0 0 0 0 0 0 0 0 0 coh738=49.3232 0 0 0 0 0 0 0 0 0 0 0 0 coh739=49.4166 0 0 0 0 0 0 0 0 0 0 0 0 coh740=49.5101 0 0 0 0 0 0 0 0 0 0 0 0 coh741=49.6036 0 0 0 0 0 0 0 0 0 0 0 0 coh742=49.697 0 0 0 0 0 0 0 0 0 0 0 0 coh743=49.7905 0 0 0 0 0 0 0 0 0 0 0 0 coh744=49.8839 0 0 0 0 0 0 0 0 0 0 0 0 coh745=49.9774 0 0 0 0 0 0 0 0 0 0 0 0 coh746=50.0709 0 0 0 0 0 0 0 0 0 0 0 0 coh747=50.1643 0 0 0 0 0 0 0 0 0 0 0 0 coh748=50.2578 0 0 0 0 0 0 0 0 0 0 0 0 coh749=50.3512 0 0 0 0 0 0 0 0 0 0 0 0 coh750=50.4447 0 0 0 0 0 0 0 0 0 0 0 0 mt_pol_for_save_print: formal savings z1_0_34741 z2_0_40076 z3_0_4623 z4_0_5333 z5_0_61519 z6_0_70966 z10_1_2567 z11_1_4496 z12_1_6723 z13_1_9291 z14_2_2253 z15_2_567 __________ __________ _________ _________ __________ __________ __________ __________ __________ __________ __________ _________ coh1=-19.5553 0 0 0 0 0 0 0 0 0 0 0 0 coh2=-19.4619 0 0 0 0 0 0 0 0 0 0 0 0 coh3=-19.3684 0 0 0 0 0 0 0 0 0 0.056554 0 0 coh4=-19.2749 0 0 0 0 0 0 0 0 0 0 0 0 coh5=-19.1815 0 0 0 0 0 0 0 0 0 0 0 0 coh6=-19.088 0 0 0 0 0 0 0 0 0.10948 0 0 0 coh7=-18.9946 0 0 0 0 0 0 0 0 0.0071398 0 0 0 coh8=-18.9011 0 0 0 0 0 0 0 0.21682 0 0 0 0 coh9=-18.8077 0 0 0 0 0 0 0 0.11448 0 0 0.62483 0 coh10=-18.7142 0 0 0 0 0 0 0.28263 0.012148 0 0 0 0 coh11=-18.6207 0 0 0 0 0 0 0.18029 0 0 0 0 0 coh12=-18.5273 0 0 0 0 0 0 0.077955 0 0 0 0 0 coh13=-18.4338 0 0 0 0 0 0 0 0 0 0.63069 0 0 coh14=-18.3404 0 0 0 0 0 0 0 0 0 0.52835 0 0 coh15=-18.2469 0 0 0 0 0 0 0 0 0 0.42602 0 0 coh16=-18.1534 0 0 0 0 0 0.43529 0 0 0 0 0 0 coh17=-18.06 0 0 0 0 0 0.33295 0 0 0.58128 0 0 0 coh18=-17.9665 0 0 0 0 0.36303 0.23062 0 0 0.47894 0 0 0 coh19=-17.8731 0 0 0 0.37548 0.26069 0.12828 0 0 0.3766 0 0 0 coh20=-17.7796 0 0 0.37265 0.27314 0.15836 0.025945 0 0.58628 0.27427 0 0 0 coh21=-17.6862 0.43134 0.35657 0.27031 0.1708 0.05602 0 0 0.48395 0 0 0 0 coh22=-17.5927 0.32901 0.25423 0.16797 0.068468 0 0 0.65209 0.38161 0 0 0 0 coh23=-17.4992 0.22667 0.15189 0.065636 0 0 0 0.54975 0.27927 0 0 0 0 coh24=-17.4058 0.12433 0.049558 0 0 0 0 0.44742 0.17694 0 0 0 0 coh25=-17.3123 0.021996 0 0 0 0 0 0.34508 0.074601 0 0 0 0 coh26=-17.2189 0 0 0 0 0 0 0.24275 0 0 0 0 0 coh27=-17.1254 0 0 0 0 0 0 0.14041 0 0 0 0 0 coh28=-17.032 0 0 0 0 0 0 0.038072 0 0 0 0 0 coh29=-16.9385 0 0 0 0 0 0 0 0 0 0 0 0 coh30=-16.845 0 0 0 0 0 0.60008 0 0 0 0 0 0 coh31=-16.7516 0 0 0 0 0.63016 0.49774 0 0 0 0 0 0 coh32=-16.6581 0 0 0 0.6426 0.52782 0.39541 0 0 0 0 0 0 coh33=-16.5647 0 0 0.63977 0.54027 0.42548 0.29307 0 0 0 0 0 0 coh34=-16.4712 0 0.62369 0.53744 0.43793 0.32315 0.19073 0 0 0 0 0 0 coh35=-16.3777 0.59613 0.52136 0.4351 0.33559 0.22081 0.088398 0 0 0 0 0 0 coh36=-16.2843 0.4938 0.41902 0.33276 0.23326 0.11847 0 0 0 0 0 0 0 coh37=-16.1908 0.39146 0.31668 0.23043 0.13092 0.016137 0 0 0 0 0 0 0 coh38=-16.0974 0.28912 0.21435 0.12809 0.028585 0 0 0 0 0 0 0 0 coh39=-16.0039 0.18679 0.11201 0.025753 0 0 0 0 0 0 0 0 0 coh40=-15.9105 0.08445 0.0096745 0 0 0 0 0 0 0 0 0 0 coh41=-15.817 0 0 0 0 0 0 0 0 0 0 0 0 coh42=-15.7235 0 0 0 0 0 0 0 0 0 0 0 0 coh43=-15.6301 0 0 0 0 0 0 0 0 0 0 0 0 coh44=-15.5366 0 0 0 0 0 0 0 0 0 0 0 0 coh45=-15.4432 0 0.050025 0 0 0 0 0 0 0 0 0 0 coh46=-15.3497 0.022464 0 0 0 0 0 0 0 0 0 0 0 coh47=-15.2563 0.013585 0 0 0 0 0 0 0 0 0 0 0 coh48=-15.1628 0 0 0 0 0 0 0 0 0 0 0 0 coh49=-15.0693 0 0 0 0 0 0 0 0 0 0 0 0 coh50=-14.9759 0 0 0 0 0 0 0 0 0 0 0 0 coh701=45.8652 42.897 42.897 42.991 42.991 43.084 43.178 43.738 43.925 44.206 44.393 44.766 45.14 coh702=45.9587 42.991 42.991 43.084 43.084 43.178 43.271 43.832 44.019 44.299 44.486 44.86 45.234 coh703=46.0522 43.084 43.084 43.178 43.178 43.271 43.364 43.925 44.112 44.299 44.579 44.953 45.327 coh704=46.1456 43.178 43.178 43.271 43.271 43.364 43.458 44.019 44.206 44.393 44.673 45.047 45.421 coh705=46.2391 43.178 43.271 43.364 43.364 43.458 43.551 44.112 44.299 44.486 44.766 45.14 45.514 coh706=46.3325 43.271 43.364 43.458 43.458 43.551 43.645 44.206 44.393 44.579 44.86 45.234 45.607 coh707=46.426 43.364 43.458 43.458 43.551 43.645 43.738 44.299 44.486 44.673 44.953 45.327 45.701 coh708=46.5195 43.458 43.551 43.551 43.645 43.738 43.832 44.393 44.579 44.766 45.047 45.421 45.794 coh709=46.6129 43.551 43.645 43.645 43.738 43.832 43.925 44.486 44.673 44.86 45.14 45.514 45.888 coh710=46.7064 43.645 43.738 43.738 43.832 43.925 44.019 44.579 44.766 44.953 45.234 45.607 45.981 coh711=46.7998 43.738 43.832 43.832 43.925 44.019 44.112 44.673 44.86 45.047 45.327 45.701 46.075 coh712=46.8933 43.832 43.925 43.925 44.019 44.112 44.206 44.766 44.953 45.14 45.421 45.794 46.168 coh713=46.9867 43.925 44.019 44.019 44.112 44.206 44.299 44.86 45.047 45.234 45.514 45.888 46.262 coh714=47.0802 44.019 44.112 44.112 44.206 44.299 44.393 44.953 45.14 45.327 45.607 45.981 46.355 coh715=47.1737 44.112 44.206 44.206 44.299 44.393 44.486 44.953 45.234 45.421 45.701 46.075 46.449 coh716=47.2671 44.206 44.299 44.299 44.393 44.486 44.579 45.047 45.327 45.514 45.794 46.075 46.542 coh717=47.3606 44.299 44.393 44.393 44.486 44.579 44.673 45.14 45.421 45.607 45.888 46.168 46.636 coh718=47.454 44.393 44.486 44.486 44.579 44.673 44.766 45.234 45.514 45.701 45.981 46.262 46.729 coh719=47.5475 44.486 44.579 44.579 44.673 44.766 44.86 45.327 45.607 45.794 46.075 46.355 46.729 coh720=47.6409 44.579 44.673 44.673 44.766 44.86 44.953 45.421 45.607 45.888 46.168 46.449 46.822 coh721=47.7344 44.673 44.766 44.766 44.86 44.953 45.047 45.514 45.701 45.981 46.262 46.542 46.916 coh722=47.8279 44.766 44.766 44.86 44.953 45.047 45.047 45.607 45.794 46.075 46.355 46.636 47.009 coh723=47.9213 44.86 44.86 44.953 45.047 45.047 45.14 45.701 45.888 46.168 46.449 46.729 47.103 coh724=48.0148 44.953 44.953 45.047 45.14 45.14 45.234 45.794 45.981 46.262 46.542 46.822 47.196 coh725=48.1082 45.047 45.047 45.14 45.234 45.234 45.327 45.888 46.075 46.355 46.636 46.916 47.29 coh726=48.2017 45.14 45.14 45.234 45.327 45.327 45.421 45.981 46.168 46.449 46.729 47.009 47.383 coh727=48.2952 45.234 45.234 45.327 45.421 45.421 45.514 46.075 46.262 46.542 46.822 47.103 47.477 coh728=48.3886 45.327 45.327 45.421 45.514 45.514 45.607 46.168 46.355 46.636 46.916 47.196 47.57 coh729=48.4821 45.421 45.421 45.514 45.607 45.607 45.701 46.262 46.449 46.729 47.009 47.29 47.664 coh730=48.5755 45.514 45.514 45.607 45.607 45.701 45.794 46.355 46.542 46.822 47.103 47.383 47.757 coh731=48.669 45.607 45.607 45.701 45.701 45.794 45.888 46.449 46.636 46.916 47.196 47.477 47.85 coh732=48.7624 45.701 45.701 45.794 45.794 45.888 45.981 46.542 46.729 47.009 47.29 47.57 47.944 coh733=48.8559 45.794 45.794 45.888 45.888 45.981 46.075 46.636 46.822 47.103 47.383 47.664 48.037 coh734=48.9494 45.888 45.888 45.981 45.981 46.075 46.168 46.729 46.916 47.196 47.477 47.757 48.131 coh735=49.0428 45.981 45.981 46.075 46.075 46.168 46.262 46.822 47.009 47.29 47.57 47.85 48.224 coh736=49.1363 46.075 46.075 46.168 46.168 46.262 46.355 46.916 47.103 47.383 47.57 47.944 48.318 coh737=49.2297 46.168 46.168 46.262 46.262 46.355 46.449 47.009 47.196 47.477 47.664 48.037 48.411 coh738=49.3232 46.262 46.262 46.355 46.355 46.449 46.542 47.103 47.29 47.477 47.757 48.131 48.505 coh739=49.4166 46.262 46.355 46.449 46.449 46.542 46.636 47.196 47.383 47.57 47.85 48.224 48.598 coh740=49.5101 46.355 46.449 46.542 46.542 46.636 46.729 47.29 47.477 47.664 47.944 48.318 48.692 coh741=49.6036 46.449 46.542 46.542 46.636 46.729 46.822 47.383 47.57 47.757 48.037 48.411 48.785 coh742=49.697 46.542 46.636 46.636 46.729 46.822 46.916 47.477 47.664 47.85 48.131 48.505 48.879 coh743=49.7905 46.636 46.729 46.729 46.822 46.916 47.009 47.57 47.757 47.944 48.224 48.598 48.972 coh744=49.8839 46.729 46.822 46.822 46.916 47.009 47.103 47.664 47.85 48.037 48.318 48.692 49.065 coh745=49.9774 46.822 46.916 46.916 47.009 47.103 47.196 47.757 47.944 48.131 48.411 48.785 49.159 coh746=50.0709 46.916 47.009 47.009 47.103 47.196 47.29 47.85 48.037 48.224 48.505 48.879 49.252 coh747=50.1643 47.009 47.103 47.103 47.196 47.29 47.383 47.944 48.131 48.318 48.598 48.972 49.346 coh748=50.2578 47.103 47.196 47.196 47.29 47.383 47.477 48.037 48.224 48.411 48.692 49.065 49.439 coh749=50.3512 47.196 47.29 47.29 47.383 47.477 47.57 48.131 48.318 48.505 48.785 49.159 49.533 coh750=50.4447 47.29 47.383 47.383 47.477 47.57 47.664 48.131 48.411 48.598 48.879 49.252 49.626
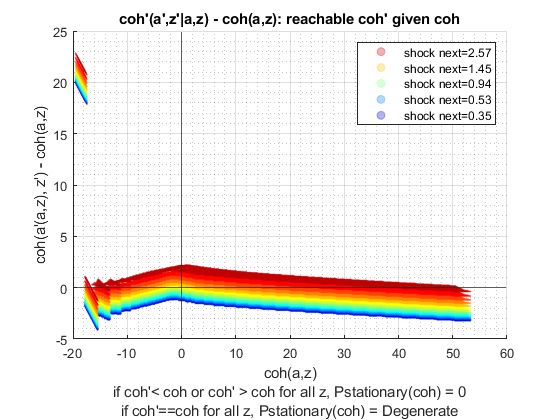
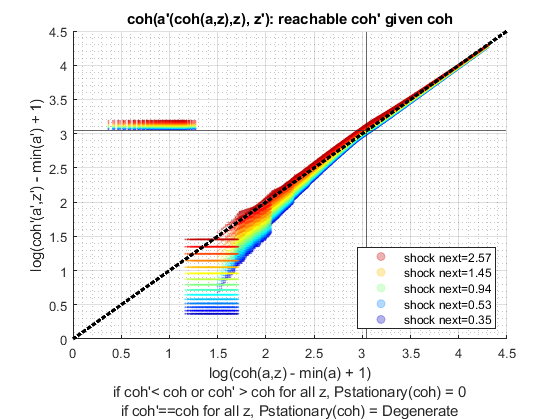
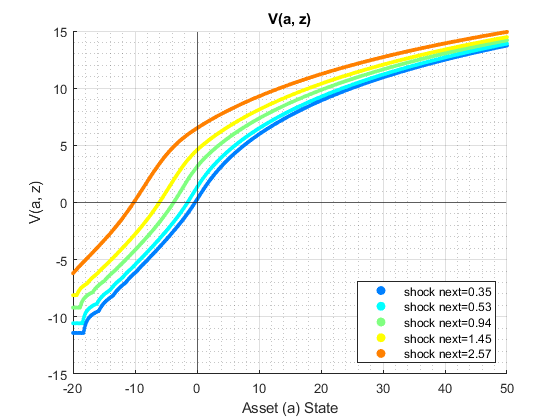
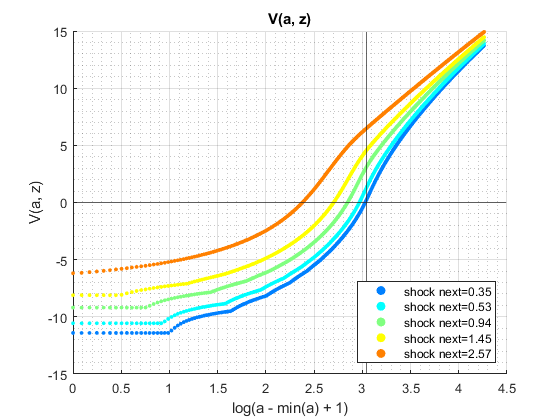
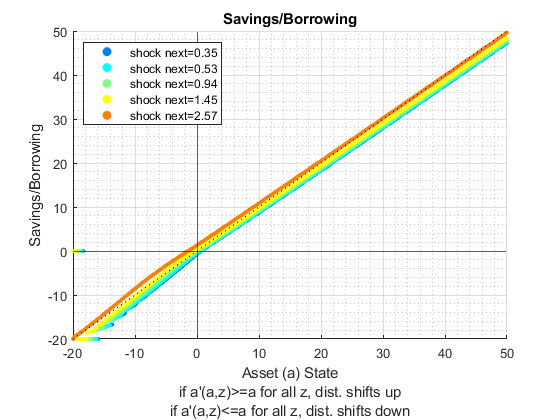
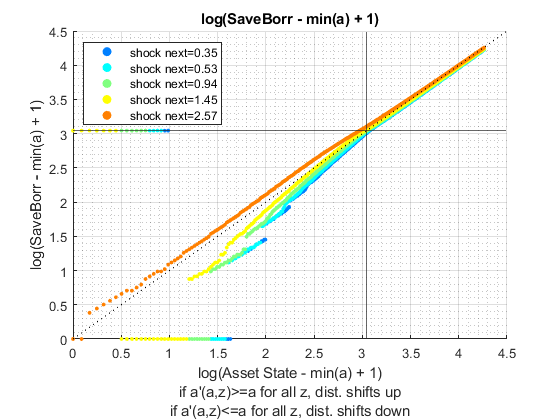
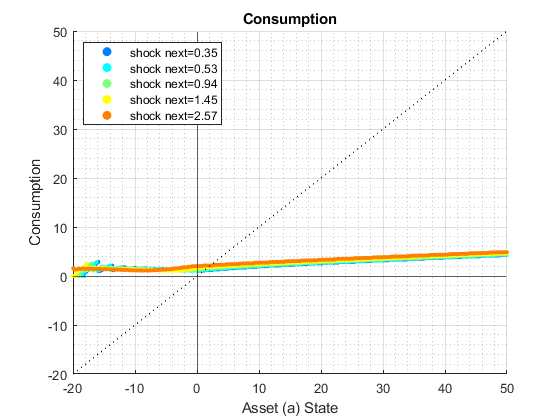
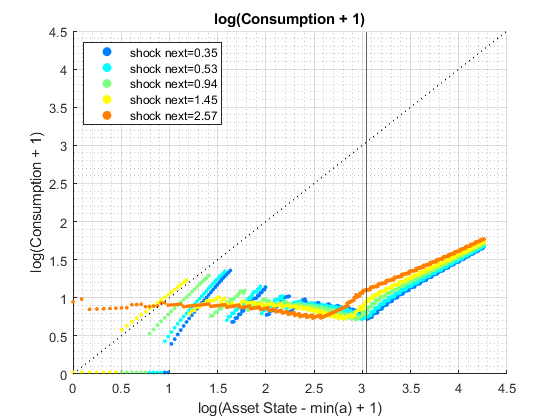
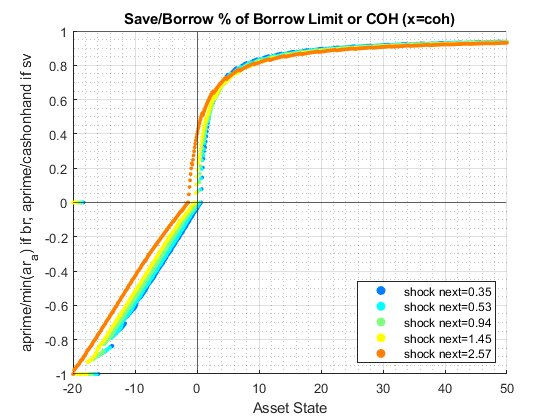
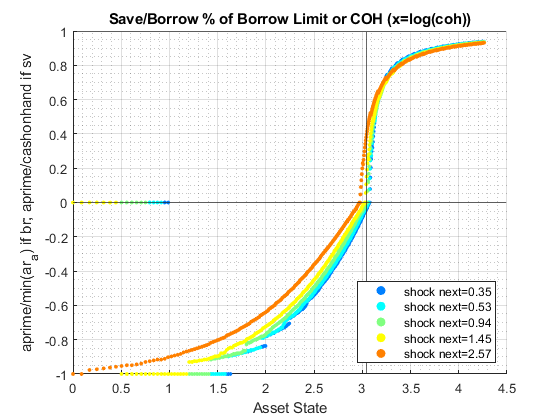
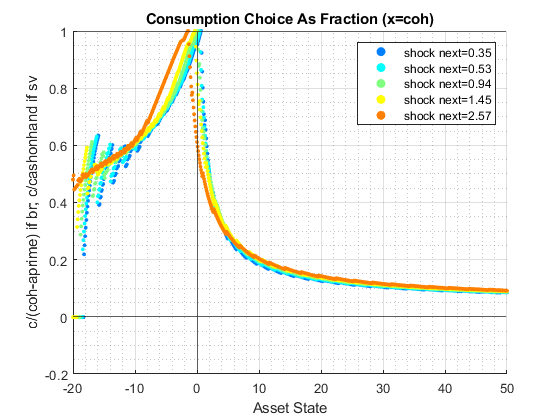
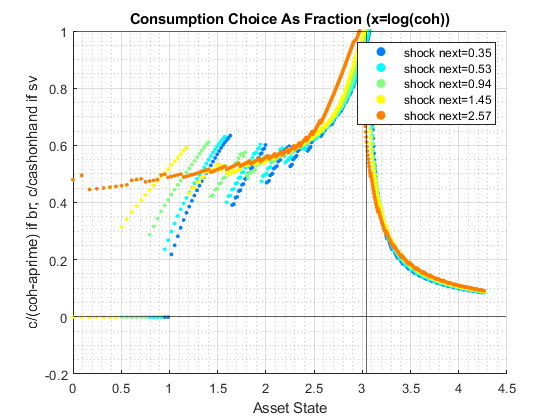
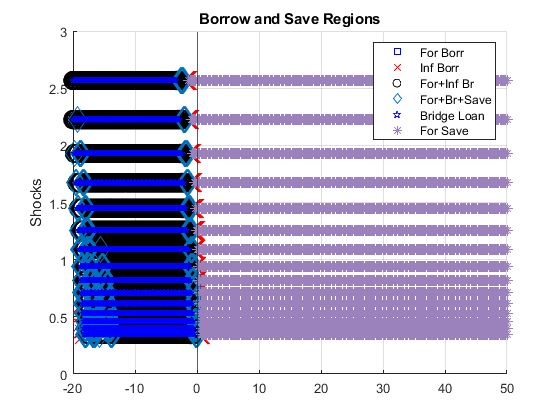
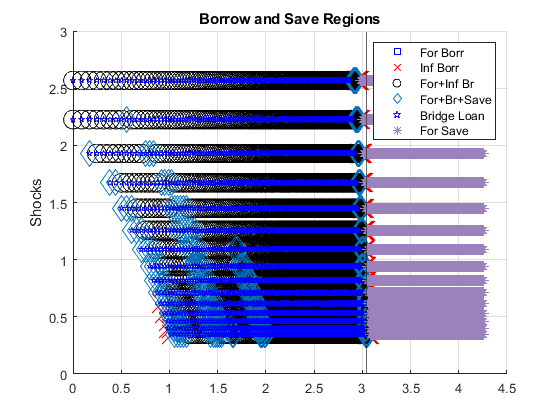
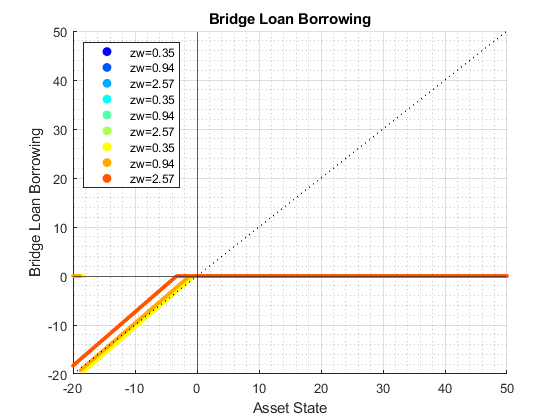
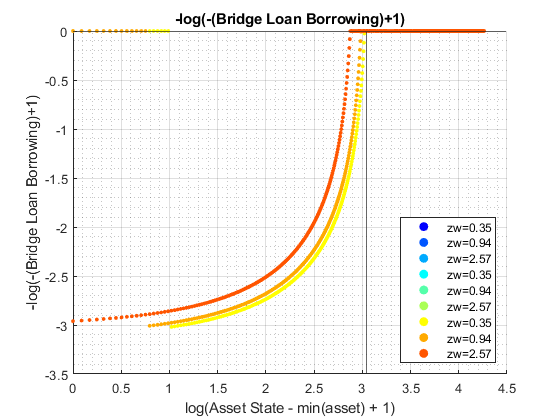
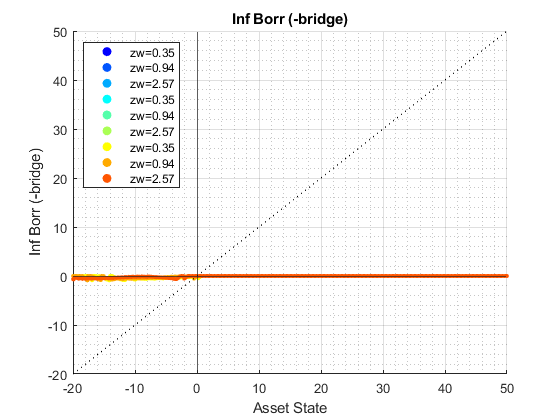
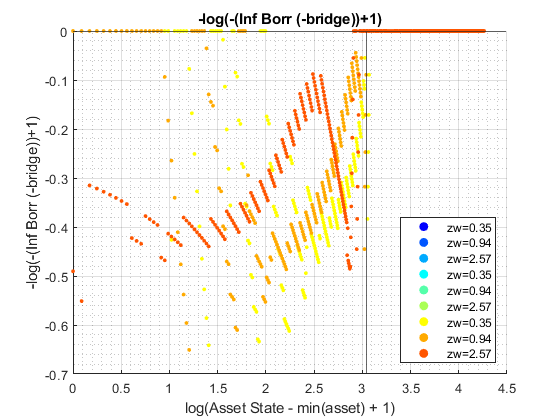
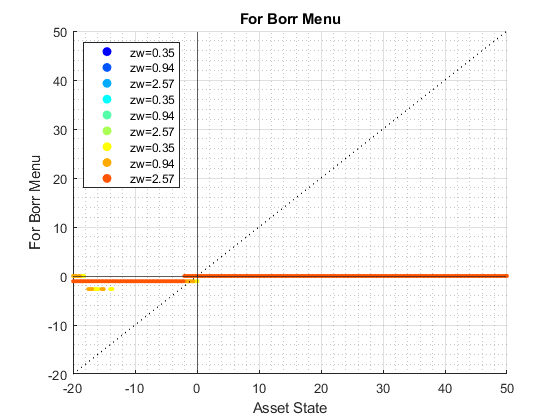
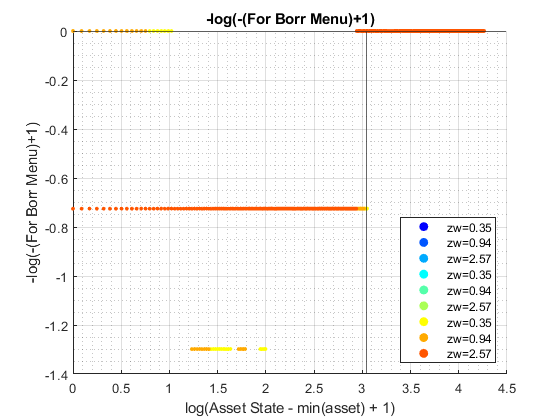
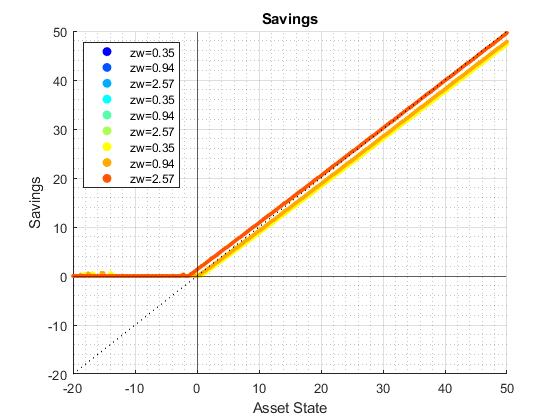
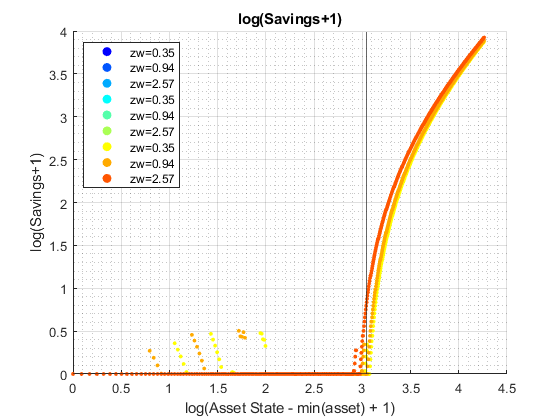
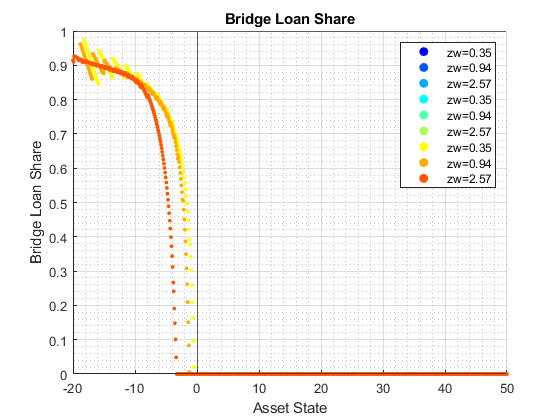
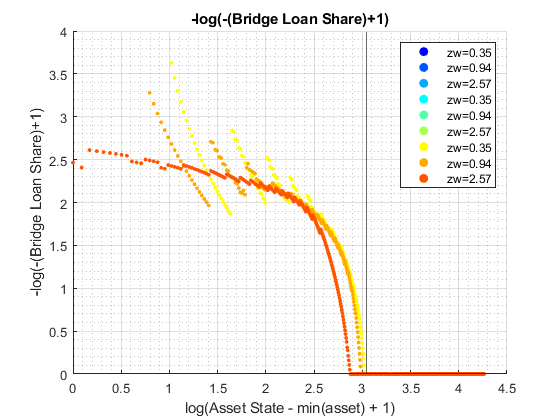
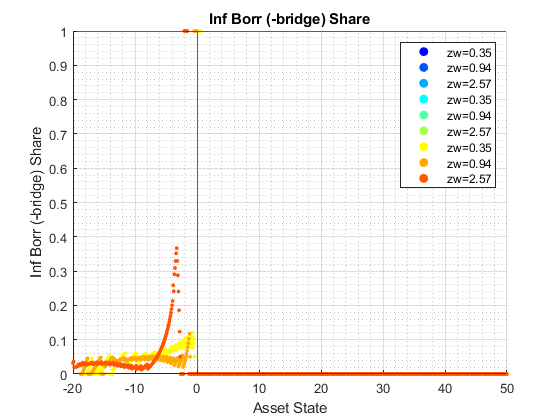
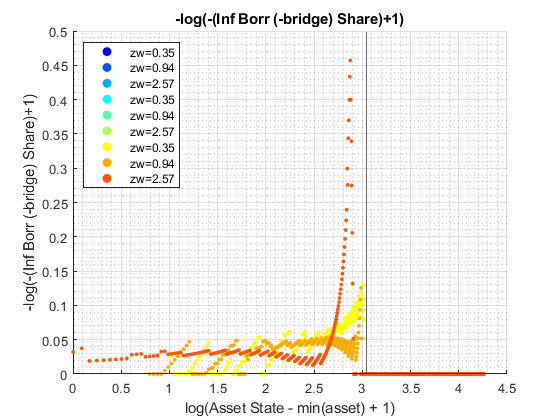
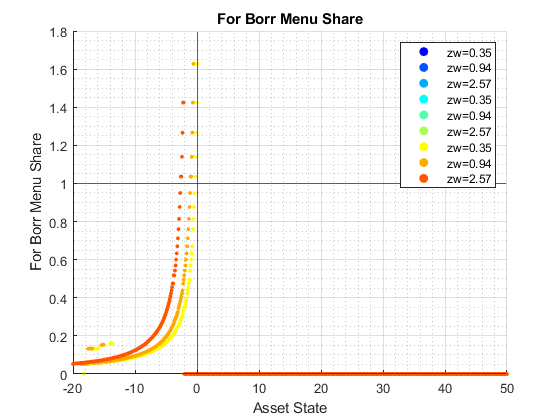
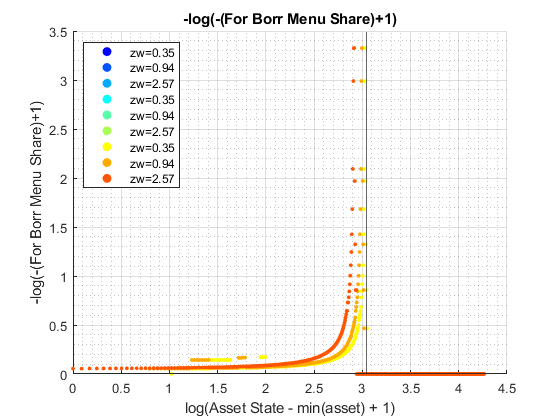
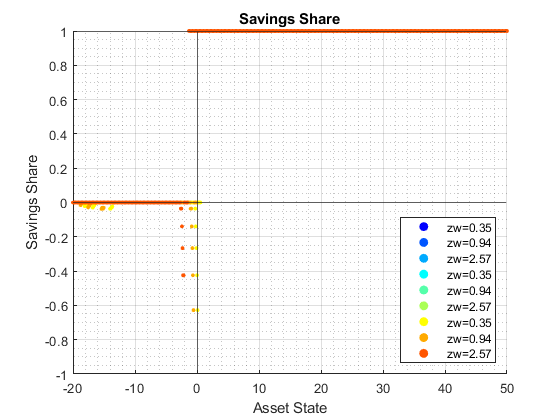
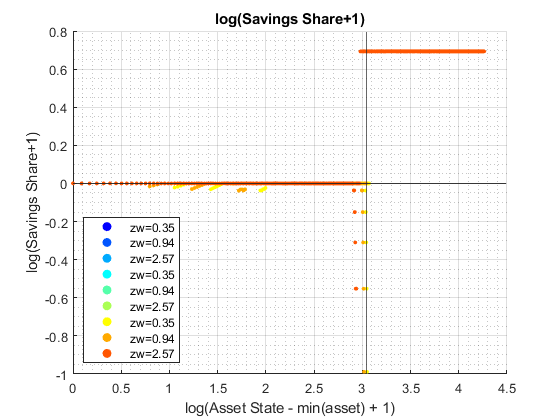
Display Various Containers
bl_display_defparam = true;
if (bl_display_defparam)
Display 1 support_map
fft_container_map_display(support_map);
---------------------------------------- ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Begin: Show all key and value pairs from container CONTAINER NAME: SUPPORT_MAP ---------------------------------------- Map with properties: Count: 42 KeyType: char ValueType: any xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ---------------------------------------- ---------------------------------------- pos = 1 ; key = bl_display ; val = false pos = 2 ; key = bl_display_dist ; val = false pos = 3 ; key = bl_display_final ; val = true pos = 4 ; key = bl_display_final_dist ; val = false pos = 5 ; key = bl_display_final_dist_detail ; val = false pos = 6 ; key = bl_display_funcgrids ; val = false pos = 7 ; key = bl_display_infbridge ; val = false pos = 8 ; key = bl_display_minccost ; val = false pos = 9 ; key = bl_graph ; val = true pos = 10 ; key = bl_graph_coh_t_coh ; val = true pos = 11 ; key = bl_graph_discrete ; val = true pos = 12 ; key = bl_graph_forinf_discrete ; val = true pos = 13 ; key = bl_graph_forinf_pol_lvl ; val = true pos = 14 ; key = bl_graph_forinf_pol_pct ; val = true pos = 15 ; key = bl_graph_funcgrids ; val = false pos = 16 ; key = bl_graph_onebyones ; val = true pos = 17 ; key = bl_graph_pol_lvl ; val = true pos = 18 ; key = bl_graph_pol_pct ; val = true pos = 19 ; key = bl_graph_val ; val = true pos = 20 ; key = bl_img_save ; val = false pos = 21 ; key = bl_mat ; val = false pos = 22 ; key = bl_post ; val = true pos = 23 ; key = bl_profile ; val = false pos = 24 ; key = bl_profile_dist ; val = false pos = 25 ; key = bl_time ; val = false pos = 26 ; key = it_display_every ; val = 5 pos = 27 ; key = it_display_final_colmax ; val = 12 pos = 28 ; key = it_display_final_rowmax ; val = 100 pos = 29 ; key = st_img_name_main ; val = ff_abz_fibs_vf_vecsv_default pos = 30 ; key = st_img_path ; val = C:/Users/fan/CodeDynaAsset//m_fibs//m_abz_solve/img/ pos = 31 ; key = st_img_prefix ; val = pos = 32 ; key = st_img_suffix ; val = _p4.png pos = 33 ; key = st_mat_name_main ; val = ff_abz_fibs_vf_vecsv_default pos = 34 ; key = st_mat_path ; val = C:/Users/fan/CodeDynaAsset//m_fibs//m_abz_solve/mat/ pos = 35 ; key = st_mat_prefix ; val = pos = 36 ; key = st_mat_suffix ; val = _p4 pos = 37 ; key = st_matimg_path_root ; val = C:/Users/fan/CodeDynaAsset//m_fibs/ pos = 38 ; key = st_profile_name_main ; val = ff_abz_fibs_vf_vecsv_default pos = 39 ; key = st_profile_path ; val = C:/Users/fan/CodeDynaAsset//m_fibs//m_abz_solve/profile/ pos = 40 ; key = st_profile_prefix ; val = pos = 41 ; key = st_profile_suffix ; val = _p4 pos = 42 ; key = st_title_prefix ; val = ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Scalars in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx value __ ___ _____ bl_display 1 1 0 bl_display_dist 2 2 0 bl_display_final 3 3 1 bl_display_final_dist 4 4 0 bl_display_final_dist_detail 5 5 0 bl_display_funcgrids 6 6 0 bl_display_infbridge 7 7 0 bl_display_minccost 8 8 0 bl_graph 9 9 1 bl_graph_coh_t_coh 10 10 1 bl_graph_discrete 11 11 1 bl_graph_forinf_discrete 12 12 1 bl_graph_forinf_pol_lvl 13 13 1 bl_graph_forinf_pol_pct 14 14 1 bl_graph_funcgrids 15 15 0 bl_graph_onebyones 16 16 1 bl_graph_pol_lvl 17 17 1 bl_graph_pol_pct 18 18 1 bl_graph_val 19 19 1 bl_img_save 20 20 0 bl_mat 21 21 0 bl_post 22 22 1 bl_profile 23 23 0 bl_profile_dist 24 24 0 bl_time 25 25 0 it_display_every 26 26 5 it_display_final_colmax 27 27 12 it_display_final_rowmax 28 28 100 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Strings in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx __ ___ st_img_name_main 1 29 st_img_path 2 30 st_img_prefix 3 31 st_img_suffix 4 32 st_mat_name_main 5 33 st_mat_path 6 34 st_mat_prefix 7 35 st_mat_suffix 8 36 st_matimg_path_root 9 37 st_profile_name_main 10 38 st_profile_path 11 39 st_profile_prefix 12 40 st_profile_suffix 13 41 st_title_prefix 14 42
Display 2 armt_map
fft_container_map_display(armt_map);
---------------------------------------- ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Begin: Show all key and value pairs from container CONTAINER NAME: ARMT_MAP ---------------------------------------- Map with properties: Count: 6 KeyType: char ValueType: any xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ---------------------------------------- ---------------------------------------- pos = 1 ; key = ar_a ;rown= 1 ,coln= 750 ar_a :mu= 15 ,sd= 20.2477 ,min= -20 ,max= 50 zi_1_C1 zi_2_C2 zi_3_C3 zi_375_c375 zi_748_c748 zi_749_c749 zi_750_c750 _______ _______ _______ ___________ ___________ ___________ ___________ zi_1_r1 -20 -19.907 -19.813 14.953 49.813 49.907 50 pos = 2 ; key = ar_forbrblk ;rown= 1 ,coln= 9 ar_forbrblk :mu= -7.0556 ,sd= 6.3809 ,min= -19 ,max= 0 zi_1_c1 zi_2_c2 zi_3_c3 zi_5_c5 zi_7_c7 zi_8_c8 zi_9_c9 _______ _______ _______ _______ _______ _______ _______ zi_1_r1 -19 -14.5 -10 -5.5 -2.5 -1 0 pos = 3 ; key = ar_forbrblk_r ;rown= 1 ,coln= 9 ar_forbrblk_r :mu= 0.065 ,sd= 0 ,min= 0.065 ,max= 0.065 zi_1_c1 zi_2_c2 zi_3_c3 zi_5_c5 zi_7_c7 zi_8_c8 zi_9_c9 _______ _______ _______ _______ _______ _______ _______ zi_1_r1 0.065 0.065 0.065 0.065 0.065 0.065 0.065 pos = 4 ; key = ar_stationary ;rown= 1 ,coln= 15 ar_stationary :mu= 0.066667 ,sd= 0.060897 ,min= 0.0027089 ,max= 0.16757 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 _________ _________ ________ _______ _________ _________ _________ zi_1_r1 0.0027089 0.0069499 0.018507 0.16757 0.018507 0.0069499 0.0027089 pos = 5 ; key = ar_z ;rown= 1 ,coln= 15 ar_z :mu= 1.1347 ,sd= 0.69878 ,min= 0.34741 ,max= 2.567 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 _______ _______ _______ _______ _________ _________ _________ zi_1_r1 0.34741 0.40076 0.4623 0.94436 1.9291 2.2253 2.567 pos = 6 ; key = mt_z_trans ;rown= 15 ,coln= 15 mt_z_trans :mu= 0.066667 ,sd= 0.095337 ,min= 0 ,max= 0.27902 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 __________ __________ __________ __________ __________ __________ __________ zi_1_R1 0.26016 0.26831 0.25551 0.00012823 2.7001e-13 1.1102e-15 0 zi_2_R2 0.11232 0.19622 0.2763 0.00098855 1.5289e-11 9.3592e-14 3.3307e-16 zi_3_R3 0.037073 0.10492 0.2185 0.0055558 6.2811e-10 5.7438e-12 3.1863e-14 zi_4_R4 0.0092081 0.040998 0.12635 0.022782 1.8732e-08 2.5567e-10 2.1255e-12 zi_5_R5 0.0017026 0.011702 0.053403 0.068209 4.058e-07 8.2598e-09 1.0277e-10 zi_11_r11 1.0277e-10 8.2598e-09 4.058e-07 0.068209 0.053403 0.011702 0.0017026 zi_12_r12 2.1256e-12 2.5567e-10 1.8732e-08 0.022782 0.12635 0.040998 0.0092081 zi_13_r13 3.1909e-14 5.7438e-12 6.2811e-10 0.0055558 0.2185 0.10492 0.037073 zi_14_r14 3.474e-16 9.3597e-14 1.5289e-11 0.00098855 0.2763 0.19622 0.11232 zi_15_r15 2.7412e-18 1.1057e-15 2.6998e-13 0.00012823 0.25551 0.26831 0.26016 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Matrix in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx rowN colN mean std min max _ ___ ____ ____ ________ ________ _________ _______ ar_a 1 1 1 750 15 20.248 -20 50 ar_forbrblk 2 2 1 9 -7.0556 6.3809 -19 0 ar_forbrblk_r 3 3 1 9 0.065 0 0.065 0.065 ar_stationary 4 4 1 15 0.066667 0.060897 0.0027089 0.16757 ar_z 5 5 1 15 1.1347 0.69878 0.34741 2.567 mt_z_trans 6 6 15 15 0.066667 0.095337 0 0.27902
Display 3 param_map
fft_container_map_display(param_map);
---------------------------------------- ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Begin: Show all key and value pairs from container CONTAINER NAME: PARAM_MAP ---------------------------------------- Map with properties: Count: 37 KeyType: char ValueType: any xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ---------------------------------------- ---------------------------------------- pos = 1 ; key = bl_b_is_principle ; val = false pos = 2 ; key = bl_bridge ; val = true pos = 3 ; key = bl_default ; val = true pos = 4 ; key = bl_loglin ; val = false pos = 5 ; key = bl_rollover ; val = true pos = 6 ; key = fl_a_max ; val = 50 pos = 7 ; key = fl_a_min ; val = 0 pos = 8 ; key = fl_b_bd ; val = -20 pos = 9 ; key = fl_beta ; val = 0.94 pos = 10 ; key = fl_c_min ; val = 0.02 pos = 11 ; key = fl_crra ; val = 1.5 pos = 12 ; key = fl_default_aprime ; val = 0 pos = 13 ; key = fl_forbrblk_brleast ; val = -1 pos = 14 ; key = fl_forbrblk_brmost ; val = -19 pos = 15 ; key = fl_forbrblk_gap ; val = -1.5 pos = 16 ; key = fl_loglin_threshold ; val = 1 pos = 17 ; key = fl_nan_replace ; val = -99999 pos = 18 ; key = fl_r_fbr ; val = 0.065 pos = 19 ; key = fl_r_fsv ; val = 0.025 pos = 20 ; key = fl_r_inf ; val = 0.095 pos = 21 ; key = fl_r_inf_bridge ; val = 0.095 pos = 22 ; key = fl_tol_dist ; val = 1e-05 pos = 23 ; key = fl_tol_pol ; val = 1e-05 pos = 24 ; key = fl_tol_val ; val = 1e-05 pos = 25 ; key = fl_w ; val = 1.28 pos = 26 ; key = fl_z_mu ; val = 0 pos = 27 ; key = fl_z_rho ; val = 0.8 pos = 28 ; key = fl_z_sig ; val = 0.2 pos = 29 ; key = it_a_n ; val = 750 pos = 30 ; key = it_maxiter_dist ; val = 1000 pos = 31 ; key = it_maxiter_val ; val = 1000 pos = 32 ; key = it_tol_pol_nochange ; val = 25 pos = 33 ; key = it_trans_power_dist ; val = 1000 pos = 34 ; key = it_z_n ; val = 15 pos = 35 ; key = st_analytical_stationary_type ; val = eigenvector pos = 36 ; key = st_forbrblk_type ; val = seg3 pos = 37 ; key = st_model ; val = abz_fibs ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Scalars in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx value __ ___ ______ bl_b_is_principle 1 1 0 bl_bridge 2 2 1 bl_default 3 3 1 bl_loglin 4 4 0 bl_rollover 5 5 1 fl_a_max 6 6 50 fl_a_min 7 7 0 fl_b_bd 8 8 -20 fl_beta 9 9 0.94 fl_c_min 10 10 0.02 fl_crra 11 11 1.5 fl_default_aprime 12 12 0 fl_forbrblk_brleast 13 13 -1 fl_forbrblk_brmost 14 14 -19 fl_forbrblk_gap 15 15 -1.5 fl_loglin_threshold 16 16 1 fl_nan_replace 17 17 -99999 fl_r_fbr 18 18 0.065 fl_r_fsv 19 19 0.025 fl_r_inf 20 20 0.095 fl_r_inf_bridge 21 21 0.095 fl_tol_dist 22 22 1e-05 fl_tol_pol 23 23 1e-05 fl_tol_val 24 24 1e-05 fl_w 25 25 1.28 fl_z_mu 26 26 0 fl_z_rho 27 27 0.8 fl_z_sig 28 28 0.2 it_a_n 29 29 750 it_maxiter_dist 30 30 1000 it_maxiter_val 31 31 1000 it_tol_pol_nochange 32 32 25 it_trans_power_dist 33 33 1000 it_z_n 34 34 15 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Strings in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx _ ___ st_analytical_stationary_type 1 35 st_forbrblk_type 2 36 st_model 3 37
Display 4 func_map
fft_container_map_display(func_map);
---------------------------------------- ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Begin: Show all key and value pairs from container CONTAINER NAME: FUNC_MAP ---------------------------------------- Map with properties: Count: 8 KeyType: char ValueType: any xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ---------------------------------------- ---------------------------------------- pos = 1 ; key = f_bprime ; val = @(fl_r_inf,ar_for_borr,ar_inf_borr,ar_for_save)(ar_for_borr./(1+fl_r_fbr)+ar_inf_borr./(1+fl_r_inf)+ar_for_save./(1+fl_r_fsv)) pos = 2 ; key = f_coh ; val = @(ar_z,ar_b)(ar_z*fl_w+ar_b) pos = 3 ; key = f_cons_coh_fbis ; val = @(coh,ar_bprime_in_c)(coh+ar_bprime_in_c) pos = 4 ; key = f_cons_coh_save ; val = @(coh,ar_for_save)(coh-ar_for_save./(1+fl_r_fsv)) pos = 5 ; key = f_inc ; val = @(ar_z,fl_r_inf,ar_for_borr,ar_inf_borr,ar_for_save)(ar_z*fl_w+((ar_for_borr./(1+fl_r_fbr))*fl_r_fbr+(ar_inf_borr./(1+fl_r_inf))*fl_r_inf+(ar_for_save./(1+fl_r_fsv))*fl_r_fsv)) pos = 6 ; key = f_util_crra ; val = @(c)(((c).^(1-fl_crra)-1)./(1-fl_crra)) pos = 7 ; key = f_util_log ; val = @(c)log(c) pos = 8 ; key = f_util_standin ; val = @(z,b)f_util_log(f_coh_simple(z,b).*(f_coh_simple(z,b)>0)+fl_c_min.*(f_coh_simple(z,b)<=0)) ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Scalars in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx xFunction _ ___ _________ f_bprime 1 1 1 f_coh 2 2 2 f_cons_coh_fbis 3 3 3 f_cons_coh_save 4 4 4 f_inc 5 5 5 f_util_crra 6 6 6 f_util_log 7 7 7 f_util_standin 8 8 8
Display 5 result_map
fft_container_map_display(result_map);
---------------------------------------- ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Begin: Show all key and value pairs from container CONTAINER NAME: RESULT_MAP ---------------------------------------- Map with properties: Count: 22 KeyType: char ValueType: any xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ---------------------------------------- ---------------------------------------- pos = 1 ; key = ar_pol_diff_norm ;rown= 118 ,coln= 1 ar_pol_diff_norm :mu= 53.0996 ,sd= 280.7213 ,min= 0 ,max= 2189.8514 zi_1_c1 _______ zi_1_R1 2002 zi_2_R2 2189.9 zi_3_R3 721.95 zi_4_R4 364.17 zi_5_R5 214.04 zi_114_r114 0 zi_115_r115 0 zi_116_r116 0 zi_117_r117 0 zi_118_r118 0 pos = 2 ; key = ar_st_pol_names ; val = cl_mt_pol_a cl_mt_coh cl_mt_pol_c cl_mt_pol_b_bridge cl_mt_pol_inf_borr_nobridge cl_mt_pol_for_borr cl_mt_pol_for_save pos = 3 ; key = ar_val_diff_norm ;rown= 118 ,coln= 1 ar_val_diff_norm :mu= 10.7496 ,sd= 28.8376 ,min= 0.027852 ,max= 193.1614 zi_1_c1 ________ zi_1_R1 193.16 zi_2_R2 147.46 zi_3_R3 119.38 zi_4_R4 98.645 zi_5_R5 83.341 zi_114_r114 0.035672 zi_115_r115 0.033532 zi_116_r116 0.031521 zi_117_r117 0.02963 zi_118_r118 0.027852 pos = 4 ; key = cl_mt_coh ;rown= 750 ,coln= 15 cl_mt_coh :mu= 16.4525 ,sd= 20.2536 ,min= -19.5553 ,max= 53.2858 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 _______ _______ _______ _______ _________ _________ _________ zi_1_R1 -19.555 -19.487 -19.408 -18.791 -17.531 -17.152 -16.714 zi_2_R2 -19.462 -19.394 -19.315 -18.698 -17.437 -17.058 -16.621 zi_3_R3 -19.368 -19.3 -19.221 -18.604 -17.344 -16.965 -16.527 zi_4_R4 -19.275 -19.207 -19.128 -18.511 -17.25 -16.871 -16.434 zi_5_R5 -19.181 -19.113 -19.034 -18.417 -17.157 -16.778 -16.34 zi_746_r746 50.071 50.139 50.218 50.835 52.095 52.475 52.912 zi_747_r747 50.164 50.233 50.311 50.928 52.189 52.568 53.005 zi_748_r748 50.258 50.326 50.405 51.022 52.282 52.661 53.099 zi_749_r749 50.351 50.42 50.498 51.115 52.376 52.755 53.192 zi_750_r750 50.445 50.513 50.592 51.209 52.469 52.848 53.286 pos = 5 ; key = cl_mt_pol_a ;rown= 750 ,coln= 15 cl_mt_pol_a :mu= 14.2841 ,sd= 19.5603 ,min= -20 ,max= 49.6262 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 _______ _______ _______ _______ _________ _________ _________ zi_1_R1 0 0 0 0 0 -20 -20 zi_2_R2 0 0 0 0 0 -20 -20 zi_3_R3 0 0 0 0 -20 -20 -19.533 zi_4_R4 0 0 0 0 -20 -20 -19.439 zi_5_R5 0 0 0 0 -20 -20 -19.346 zi_746_r746 46.916 47.009 47.009 47.477 48.505 48.879 49.252 zi_747_r747 47.009 47.103 47.103 47.57 48.598 48.972 49.346 zi_748_r748 47.103 47.196 47.196 47.664 48.692 49.065 49.439 zi_749_r749 47.196 47.29 47.29 47.757 48.785 49.159 49.533 zi_750_r750 47.29 47.383 47.383 47.85 48.879 49.252 49.626 pos = 6 ; key = cl_mt_pol_b_bridge ;rown= 750 ,coln= 15 cl_mt_pol_b_bridge :mu= -2.4126 ,sd= 5.0145 ,min= -19.4962 ,max= 0 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 _______ _______ _______ _______ _________ _________ _________ zi_1_R1 0 0 0 0 0 -18.781 -18.302 zi_2_R2 0 0 0 0 0 -18.679 -18.2 zi_3_R3 0 0 0 0 -18.992 -18.576 -18.097 zi_4_R4 0 0 0 0 -18.889 -18.474 -17.995 zi_5_R5 0 0 0 0 -18.787 -18.372 -17.893 zi_746_r746 0 0 0 0 0 0 0 zi_747_r747 0 0 0 0 0 0 0 zi_748_r748 0 0 0 0 0 0 0 zi_749_r749 0 0 0 0 0 0 0 zi_750_r750 0 0 0 0 0 0 0 pos = 7 ; key = cl_mt_pol_c ;rown= 750 ,coln= 15 cl_mt_pol_c :mu= 2.618 ,sd= 1.1332 ,min= 0.02 ,max= 4.87 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 _______ _______ _______ _______ _________ _________ _________ zi_1_R1 0.02 0.02 0.02 0.02 0.02 1.1406 1.578 zi_2_R2 0.02 0.02 0.02 0.02 0.02 1.2341 1.6715 zi_3_R3 0.02 0.02 0.02 0.02 0.94483 1.3275 1.3382 zi_4_R4 0.02 0.02 0.02 0.02 1.0418 1.421 1.3463 zi_5_R5 0.02 0.02 0.02 0.02 1.1353 1.5145 1.3544 zi_746_r746 4.2993 4.2764 4.3551 4.5163 4.7737 4.7882 4.8609 zi_747_r747 4.3015 4.2786 4.3574 4.5186 4.776 4.7905 4.8632 zi_748_r748 4.3038 4.2809 4.3597 4.5208 4.7783 4.7928 4.8655 zi_749_r749 4.3061 4.2832 4.362 4.5231 4.7806 4.795 4.8677 zi_750_r750 4.3084 4.2855 4.3643 4.5254 4.7829 4.7973 4.87 pos = 8 ; key = cl_mt_pol_for_borr ;rown= 750 ,coln= 15 cl_mt_pol_for_borr :mu= -0.30038 ,sd= 0.5387 ,min= -2.6625 ,max= 0 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 _______ _______ _______ _______ _________ _________ _________ zi_1_R1 0 0 0 0 0 -1.065 -1.065 zi_2_R2 0 0 0 0 0 -1.065 -1.065 zi_3_R3 0 0 0 0 -1.065 -1.065 -1.065 zi_4_R4 0 0 0 0 -1.065 -1.065 -1.065 zi_5_R5 0 0 0 0 -1.065 -1.065 -1.065 zi_746_r746 0 0 0 0 0 0 0 zi_747_r747 0 0 0 0 0 0 0 zi_748_r748 0 0 0 0 0 0 0 zi_749_r749 0 0 0 0 0 0 0 zi_750_r750 0 0 0 0 0 0 0 pos = 9 ; key = cl_mt_pol_for_save ;rown= 750 ,coln= 15 cl_mt_pol_for_save :mu= 17.0926 ,sd= 15.977 ,min= 0 ,max= 49.6262 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 _______ _______ _______ _______ _________ _________ _________ zi_1_R1 0 0 0 0 0 0 0 zi_2_R2 0 0 0 0 0 0 0 zi_3_R3 0 0 0 0 0.056554 0 0 zi_4_R4 0 0 0 0 0 0 0 zi_5_R5 0 0 0 0 0 0 0 zi_746_r746 46.916 47.009 47.009 47.477 48.505 48.879 49.252 zi_747_r747 47.009 47.103 47.103 47.57 48.598 48.972 49.346 zi_748_r748 47.103 47.196 47.196 47.664 48.692 49.065 49.439 zi_749_r749 47.196 47.29 47.29 47.757 48.785 49.159 49.533 zi_750_r750 47.29 47.383 47.383 47.85 48.879 49.252 49.626 pos = 10 ; key = cl_mt_pol_inf_borr_nobridge ;rown= 750 ,coln= 15 cl_mt_pol_inf_borr_nobridge :mu= -0.095582 ,sd= 0.19098 ,min= -0.92595 ,max= 0 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 _______ _______ _______ _______ _________ _________ _________ zi_1_R1 0 0 0 0 0 -0.15398 -0.63294 zi_2_R2 0 0 0 0 0 -0.25631 -0.73528 zi_3_R3 0 0 0 0 0 -0.35865 -0.37033 zi_4_R4 0 0 0 0 -0.045783 -0.46099 -0.37921 zi_5_R5 0 0 0 0 -0.14812 -0.56332 -0.38808 zi_746_r746 0 0 0 0 0 0 0 zi_747_r747 0 0 0 0 0 0 0 zi_748_r748 0 0 0 0 0 0 0 zi_749_r749 0 0 0 0 0 0 0 zi_750_r750 0 0 0 0 0 0 0 pos = 11 ; key = mt_it_b_bridge_idx ;rown= 750 ,coln= 15 mt_it_b_bridge_idx :mu= 0.25076 ,sd= 0.43347 ,min= 0 ,max= 1 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 _______ _______ _______ _______ _________ _________ _________ zi_1_R1 false false false false false true true zi_2_R2 false false false false false true true zi_3_R3 false false false false true true true zi_4_R4 false false false false true true true zi_5_R5 false false false false true true true zi_746_r746 false false false false false false false zi_747_r747 false false false false false false false zi_748_r748 false false false false false false false zi_749_r749 false false false false false false false zi_750_r750 false false false false false false false pos = 12 ; key = mt_it_for_only_nbdg ;rown= 750 ,coln= 15 mt_it_for_only_nbdg :mu= 0 ,sd= 0 ,min= 0 ,max= 0 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 _______ _______ _______ _______ _________ _________ _________ zi_1_R1 false false false false false false false zi_2_R2 false false false false false false false zi_3_R3 false false false false false false false zi_4_R4 false false false false false false false zi_5_R5 false false false false false false false zi_746_r746 false false false false false false false zi_747_r747 false false false false false false false zi_748_r748 false false false false false false false zi_749_r749 false false false false false false false zi_750_r750 false false false false false false false pos = 13 ; key = mt_it_fr_brrsv_nbdg ;rown= 750 ,coln= 15 mt_it_fr_brrsv_nbdg :mu= 0.022844 ,sd= 0.14941 ,min= 0 ,max= 1 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 _______ _______ _______ _______ _________ _________ _________ zi_1_R1 false false false false false false false zi_2_R2 false false false false false false false zi_3_R3 false false false false true false false zi_4_R4 false false false false false false false zi_5_R5 false false false false false false false zi_746_r746 false false false false false false false zi_747_r747 false false false false false false false zi_748_r748 false false false false false false false zi_749_r749 false false false false false false false zi_750_r750 false false false false false false false pos = 14 ; key = mt_it_frin_brr_nbdg ;rown= 750 ,coln= 15 mt_it_frin_brr_nbdg :mu= 0.23787 ,sd= 0.4258 ,min= 0 ,max= 1 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 _______ _______ _______ _______ _________ _________ _________ zi_1_R1 false false false false false true true zi_2_R2 false false false false false true true zi_3_R3 false false false false false true true zi_4_R4 false false false false true true true zi_5_R5 false false false false true true true zi_746_r746 false false false false false false false zi_747_r747 false false false false false false false zi_748_r748 false false false false false false false zi_749_r749 false false false false false false false zi_750_r750 false false false false false false false pos = 15 ; key = mt_it_frmsavng_only ;rown= 750 ,coln= 15 mt_it_frmsavng_only :mu= 0.71413 ,sd= 0.45185 ,min= 0 ,max= 1 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 _______ _______ _______ _______ _________ _________ _________ zi_1_R1 false false false false false false false zi_2_R2 false false false false false false false zi_3_R3 false false false false false false false zi_4_R4 false false false false false false false zi_5_R5 false false false false false false false zi_746_r746 true true true true true true true zi_747_r747 true true true true true true true zi_748_r748 true true true true true true true zi_749_r749 true true true true true true true zi_750_r750 true true true true true true true pos = 16 ; key = mt_it_inf_only_nbdg ;rown= 750 ,coln= 15 mt_it_inf_only_nbdg :mu= 0.0085333 ,sd= 0.091985 ,min= 0 ,max= 1 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 _______ _______ _______ _______ _________ _________ _________ zi_1_R1 false false false false false false false zi_2_R2 false false false false false false false zi_3_R3 false false false false false false false zi_4_R4 false false false false false false false zi_5_R5 false false false false false false false zi_746_r746 false false false false false false false zi_747_r747 false false false false false false false zi_748_r748 false false false false false false false zi_749_r749 false false false false false false false zi_750_r750 false false false false false false false pos = 17 ; key = mt_pol_idx ;rown= 750 ,coln= 15 mt_pol_idx :mu= 367.8396 ,sd= 209.2957 ,min= 1 ,max= 746 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 _______ _______ _______ _______ _________ _________ _________ zi_1_R1 215 215 215 215 215 1 1 zi_2_R2 215 215 215 215 215 1 1 zi_3_R3 215 215 215 215 1 1 6 zi_4_R4 215 215 215 215 1 1 7 zi_5_R5 215 215 215 215 1 1 8 zi_746_r746 717 718 718 723 734 738 742 zi_747_r747 718 719 719 724 735 739 743 zi_748_r748 719 720 720 725 736 740 744 zi_749_r749 720 721 721 726 737 741 745 zi_750_r750 721 722 722 727 738 742 746 pos = 18 ; key = mt_pol_perc_change ;rown= 118 ,coln= 15 mt_pol_perc_change :mu= 0.20847 ,sd= 0.33438 ,min= 0 ,max= 1 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 _______ _______ _______ _______ _________ _________ _________ zi_1_R1 1 1 1 1 1 1 1 zi_2_R2 0.98 0.98133 0.98267 0.992 1 1 1 zi_3_R3 0.96267 0.96533 0.96667 0.98133 1 1 1 zi_4_R4 0.94933 0.95333 0.956 0.972 0.99467 1 1 zi_5_R5 0.932 0.93467 0.93733 0.964 0.99067 0.99867 0.99733 zi_114_r114 0 0 0 0 0 0 0 zi_115_r115 0 0 0 0 0 0 0 zi_116_r116 0 0 0 0 0 0 0 zi_117_r117 0 0 0 0 0 0 0 zi_118_r118 0 0 0 0 0 0 0 pos = 19 ; key = mt_val ;rown= 750 ,coln= 15 mt_val :mu= 6.3492 ,sd= 7.0755 ,min= -11.4101 ,max= 14.9196 zi_1_C1 zi_2_C2 zi_3_C3 zi_8_C8 zi_13_c13 zi_14_c14 zi_15_c15 _______ _______ _______ _______ _________ _________ _________ zi_1_R1 -11.41 -11.157 -10.871 -9.1936 -7.378 -6.7979 -6.1925 zi_2_R2 -11.41 -11.157 -10.871 -9.1936 -7.378 -6.7256 -6.1474 zi_3_R3 -11.41 -11.157 -10.871 -9.1936 -7.2987 -6.6611 -6.0954 zi_4_R4 -11.41 -11.157 -10.871 -9.1936 -7.2005 -6.603 -6.0336 zi_5_R5 -11.41 -11.157 -10.871 -9.1936 -7.1182 -6.5504 -5.9757 zi_746_r746 13.685 13.735 13.791 14.148 14.648 14.766 14.885 zi_747_r747 13.696 13.746 13.802 14.157 14.657 14.775 14.894 zi_748_r748 13.706 13.756 13.812 14.167 14.666 14.784 14.902 zi_749_r749 13.717 13.766 13.823 14.177 14.675 14.792 14.911 zi_750_r750 13.728 13.777 13.833 14.187 14.684 14.801 14.92 pos = 20 ; key = tb_pol_a ;rown= 100 ,coln= 12 tb_pol_a :mu= 15.5562 ,sd= 30.8702 ,min= -20 ,max= 49.6262 zi_1_C1 zi_2_C2 zi_3_C3 zi_6_C6 zi_10_c10 zi_11_c11 zi_12_c12 _______ _______ _______ _______ _________ _________ _________ zi_1_R1 0 0 0 0 0 -20 -20 zi_2_R2 0 0 0 0 0 -20 -20 zi_3_R3 0 0 0 0 -20 -20 -19.533 zi_4_R4 0 0 0 0 -20 -20 -19.439 zi_5_R5 0 0 0 0 -20 -20 -19.346 zi_96_R96 46.916 47.009 47.009 47.29 48.505 48.879 49.252 zi_97_R97 47.009 47.103 47.103 47.383 48.598 48.972 49.346 zi_98_R98 47.103 47.196 47.196 47.477 48.692 49.065 49.439 zi_99_R99 47.196 47.29 47.29 47.57 48.785 49.159 49.533 zi_100_r100 47.29 47.383 47.383 47.664 48.879 49.252 49.626 pos = 21 ; key = tb_val ;rown= 100 ,coln= 12 tb_val :mu= 3.0056 ,sd= 11.1075 ,min= -11.4101 ,max= 14.9196 zi_1_C1 zi_2_C2 zi_3_C3 zi_6_C6 zi_10_c10 zi_11_c11 zi_12_c12 _______ _______ _______ _______ _________ _________ _________ zi_1_R1 -11.41 -11.157 -10.871 -9.8987 -7.378 -6.7979 -6.1925 zi_2_R2 -11.41 -11.157 -10.871 -9.8987 -7.378 -6.7256 -6.1474 zi_3_R3 -11.41 -11.157 -10.871 -9.8987 -7.2987 -6.6611 -6.0954 zi_4_R4 -11.41 -11.157 -10.871 -9.8987 -7.2005 -6.603 -6.0336 zi_5_R5 -11.41 -11.157 -10.871 -9.8987 -7.1182 -6.5504 -5.9757 zi_96_R96 13.685 13.735 13.791 13.99 14.648 14.766 14.885 zi_97_R97 13.696 13.746 13.802 14 14.657 14.775 14.894 zi_98_R98 13.706 13.756 13.812 14.01 14.666 14.784 14.902 zi_99_R99 13.717 13.766 13.823 14.02 14.675 14.792 14.911 zi_100_r100 13.728 13.777 13.833 14.03 14.684 14.801 14.92 pos = 22 ; key = tb_valpol_alliter ;rown= 100 ,coln= 14 tb_valpol_alliter :mu= 5.5725 ,sd= 82.9258 ,min= 0 ,max= 2189.8514 zi_1_C1 zi_2_C2 zi_3_C3 zi_7_C7 zi_12_c12 zi_13_c13 zi_14_c14 ________ _______ _______ _______ _________ _________ _________ zi_1_R1 193.16 2002 1 1 1 1 1 zi_2_R2 147.46 2189.9 0.98 0.98533 1 1 1 zi_3_R3 119.38 721.95 0.96267 0.972 1 1 1 zi_4_R4 98.645 364.17 0.94933 0.964 0.99467 1 1 zi_5_R5 83.341 214.04 0.932 0.94667 0.99067 0.99867 0.99733 zi_96_R96 0.035672 0 0 0 0 0 0 zi_97_R97 0.033532 0 0 0 0 0 0 zi_98_R98 0.031521 0 0 0 0 0 0 zi_99_R99 0.02963 0 0 0 0 0 0 zi_100_r100 0.027852 0 0 0 0 0 0 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Matrix in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx rowN colN mean std min max __ ___ ____ ____ _________ ________ ________ ______ ar_pol_diff_norm 1 1 118 1 53.1 280.72 0 2189.9 ar_val_diff_norm 2 3 118 1 10.75 28.838 0.027852 193.16 cl_mt_coh 3 4 750 15 16.452 20.254 -19.555 53.286 cl_mt_pol_a 4 5 750 15 14.284 19.56 -20 49.626 cl_mt_pol_b_bridge 5 6 750 15 -2.4126 5.0145 -19.496 0 cl_mt_pol_c 6 7 750 15 2.618 1.1332 0.02 4.87 cl_mt_pol_for_borr 7 8 750 15 -0.30038 0.5387 -2.6625 0 cl_mt_pol_for_save 8 9 750 15 17.093 15.977 0 49.626 cl_mt_pol_inf_borr_nobridge 9 10 750 15 -0.095582 0.19098 -0.92595 0 mt_it_b_bridge_idx 10 11 750 15 0.25076 0.43347 0 1 mt_it_for_only_nbdg 11 12 750 15 0 0 0 0 mt_it_fr_brrsv_nbdg 12 13 750 15 0.022844 0.14941 0 1 mt_it_frin_brr_nbdg 13 14 750 15 0.23787 0.4258 0 1 mt_it_frmsavng_only 14 15 750 15 0.71413 0.45185 0 1 mt_it_inf_only_nbdg 15 16 750 15 0.0085333 0.091985 0 1 mt_pol_idx 16 17 750 15 367.84 209.3 1 746 mt_pol_perc_change 17 18 118 15 0.20847 0.33438 0 1 mt_val 18 19 750 15 6.3492 7.0755 -11.41 14.92 tb_pol_a 19 20 100 12 15.556 30.87 -20 49.626 tb_val 20 21 100 12 3.0056 11.108 -11.41 14.92 tb_valpol_alliter 21 22 100 14 5.5725 82.926 0 2189.9 ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Strings in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx _ ___ ar_st_pol_names 1 2
end
end
ans = Map with properties: Count: 22 KeyType: char ValueType: any