Solve Save + Borr Dynamic Programming Problem (Loop)
back to Fan's Dynamic Assets Repository Table of Content.
Contents
- FF_ABZ_VF solve infinite horizon exo shock + endo asset problem
- Default
- Parse Parameters 1
- Parse Parameters 2
- Display Parameters
- Initialize Output Matrixes
- Initialize Convergence Conditions
- Iterate Value Function
- Iterate over a and z states
- Store Optimal Choices and Value Given(a,z)
- Check Tolerance and Continuation
- Process Optimal Choices
function result_map = ff_abz_vf(varargin)
FF_ABZ_VF solve infinite horizon exo shock + endo asset problem
This program solves the infinite horizon dynamic single asset and single shock problem with loops. This file contains codes that processes borrowing.
The borrowing problem is very similar to the savings problem. The code could be identical if one does not have to deal with default. The main addition here in comparison to the savings only code ff_az_vf is the ability to deal with default.
@param param_map container parameter container
@param support_map container support container
@param armt_map container container with states, choices and shocks grids that are inputs for grid based solution algorithm
@param func_map container container with function handles for consumption cash-on-hand etc.
@return result_map container contains policy function matrix, value function matrix, iteration results, and policy function, value function and iteration results tables.
keys included in result_map:
- mt_val matrix states_n by shock_n matrix of converged value function grid
- mt_pol_a matrix states_n by shock_n matrix of converged policy function grid
- ar_val_diff_norm array if bl_post = true it_iter_last by 1 val function difference between iteration
- ar_pol_diff_norm array if bl_post = true it_iter_last by 1 policy function difference between iterations
- mt_pol_perc_change matrix if bl_post = true it_iter_last by shock_n the proportion of grid points at which policy function changed between current and last iteration for each element of shock
@example
% Get Default Parameters it_param_set = 2; [param_map, support_map] = ffs_abz_set_default_param(it_param_set); % Chnage param_map keys for borrowing param_map('fl_b_bd') = -20; % borrow bound param_map('bl_default') = false; % true if allow for default param_map('fl_c_min') = 0.0001; % u(c_min) when default % Change Keys in param_map param_map('it_a_n') = 500; param_map('fl_z_r_borr_n') = 5; param_map('it_z_wage_n') = 15; param_map('it_z_n') = param_map('it_z_wage_n') * param_map('fl_z_r_borr_n'); param_map('fl_a_max') = 100; param_map('fl_w') = 1.3; % Change Keys support_map support_map('bl_display') = false; support_map('bl_post') = true; support_map('bl_display_final') = false; % Call Program with external parameters that override defaults. ff_abz_vf(param_map, support_map);
@include
@seealso
- save loop: ff_az_vf
- save vectorized: ff_az_vf_vec
- save optimized-vectorized: ff_az_vf_vecsv
- save + borr loop: ff_abz_vf
- save + borr vectorized: ff_abz_vf_vec
- save + borr optimized-vectorized: ff_abz_vf_vecsv
Default
- it_param_set = 1: quick test
- it_param_set = 2: benchmark run
- it_param_set = 3: benchmark profile
- it_param_set = 4: press publish button
it_param_set = 4; bl_input_override = true; [param_map, support_map] = ffs_abz_set_default_param(it_param_set); % Note: param_map and support_map can be adjusted here or outside to override defaults param_map('it_a_n') = 75; param_map('fl_z_r_borr_n') = 3; param_map('it_z_wage_n') = 5; param_map('it_z_n') = param_map('it_z_wage_n') * param_map('fl_z_r_borr_n'); % param_map('fl_r_save') = 0.025; % param_map('fl_r_borr') = 0.035; [armt_map, func_map] = ffs_abz_get_funcgrid(param_map, support_map, bl_input_override); % 1 for override default_params = {param_map support_map armt_map func_map};
Parse Parameters 1
% if varargin only has param_map and support_map, params_len = length(varargin); [default_params{1:params_len}] = varargin{:}; param_map = [param_map; default_params{1}]; support_map = [support_map; default_params{2}]; if params_len >= 1 && params_len <= 2 % If override param_map, re-generate armt and func if they are not % provided bl_input_override = true; [armt_map, func_map] = ffs_abz_get_funcgrid(param_map, support_map, bl_input_override); else % Override all armt_map = [armt_map; default_params{3}]; func_map = [func_map; default_params{4}]; end % append function name st_func_name = 'ff_abz_vf'; support_map('st_profile_name_main') = [st_func_name support_map('st_profile_name_main')]; support_map('st_mat_name_main') = [st_func_name support_map('st_mat_name_main')]; support_map('st_img_name_main') = [st_func_name support_map('st_img_name_main')];
Parse Parameters 2
% armt_map params_group = values(armt_map, {'ar_a', 'mt_z_trans', 'ar_z_r_borr_mesh_wage', 'ar_z_wage_mesh_r_borr'}); [ar_a, mt_z_trans, ar_z_r_borr_mesh_wage, ar_z_wage_mesh_r_borr] = params_group{:}; % func_map params_group = values(func_map, {'f_util_log', 'f_util_crra', 'f_cons_checkcmin', 'f_awithr_to_anor', 'f_coh', 'f_cons_coh'}); [f_util_log, f_util_crra, f_cons_checkcmin, f_awithr_to_anor, f_coh, f_cons_coh] = params_group{:}; % param_map params_group = values(param_map, {'it_a_n', 'it_z_n', 'fl_crra', 'fl_beta', 'fl_c_min',... 'fl_nan_replace', 'bl_default', 'fl_default_aprime'}); [it_a_n, it_z_n, fl_crra, fl_beta, fl_c_min, ... fl_nan_replace, bl_default, fl_default_aprime] = params_group{:}; params_group = values(param_map, {'it_maxiter_val', 'fl_tol_val', 'fl_tol_pol', 'it_tol_pol_nochange'}); [it_maxiter_val, fl_tol_val, fl_tol_pol, it_tol_pol_nochange] = params_group{:}; % support_map params_group = values(support_map, {'bl_profile', 'st_profile_path', ... 'st_profile_prefix', 'st_profile_name_main', 'st_profile_suffix',... 'bl_time', 'bl_display_defparam', 'bl_display', 'it_display_every', 'bl_post'}); [bl_profile, st_profile_path, ... st_profile_prefix, st_profile_name_main, st_profile_suffix, ... bl_time, bl_display_defparam, bl_display, it_display_every, bl_post] = params_group{:};
Display Parameters
if(bl_display_defparam) fft_container_map_display(param_map); fft_container_map_display(support_map); end
---------------------------------------- ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Begin: Show all key and value pairs from container CONTAINER NAME: PARAM_MAP ---------------------------------------- Map with properties: Count: 35 KeyType: char ValueType: any xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ---------------------------------------- ---------------------------------------- pos = 32 ; key = st_analytical_stationary_type ; val = eigenvector pos = 33 ; key = st_model ; val = abz pos = 34 ; key = st_z_r_borr_drv_ele_type ; val = unif pos = 35 ; key = st_z_r_borr_drv_prb_type ; val = poiss ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Scalars in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx value __ ___ ______ bl_b_is_principle 1 1 1 bl_default 2 2 1 bl_loglin 3 3 0 fl_a_max 4 4 50 fl_a_min 5 5 0 fl_b_bd 6 6 -20 fl_beta 7 7 0.94 fl_c_min 8 8 0.01 fl_crra 9 9 1.5 fl_default_aprime 10 10 0 fl_loglin_threshold 11 11 1 fl_nan_replace 12 12 -99999 fl_r_save 13 13 0.025 fl_tol_dist 14 14 1e-05 fl_tol_pol 15 15 1e-05 fl_tol_val 16 16 1e-05 fl_w 17 17 1.28 fl_z_r_borr_max 18 18 0.095 fl_z_r_borr_min 19 19 0.025 fl_z_r_borr_n 20 20 3 fl_z_r_borr_poiss_mean 21 21 10 fl_z_wage_mu 22 22 0 fl_z_wage_rho 23 23 0.8 fl_z_wage_sig 24 24 0.2 it_a_n 25 25 75 it_maxiter_dist 26 26 1000 it_maxiter_val 27 27 1000 it_tol_pol_nochange 28 28 25 it_trans_power_dist 29 29 1000 it_z_n 30 30 15 it_z_wage_n 31 31 5 ---------------------------------------- ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Begin: Show all key and value pairs from container CONTAINER NAME: SUPPORT_MAP ---------------------------------------- Map with properties: Count: 40 KeyType: char ValueType: any xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ---------------------------------------- ---------------------------------------- pos = 26 ; key = st_img_name_main ; val = ff_abz_vf_default pos = 27 ; key = st_img_path ; val = C:/Users/fan/CodeDynaAsset//m_abz//solve/img/ pos = 28 ; key = st_img_prefix ; val = pos = 29 ; key = st_img_suffix ; val = _p4.png pos = 30 ; key = st_mat_name_main ; val = ff_abz_vf_default pos = 31 ; key = st_mat_path ; val = C:/Users/fan/CodeDynaAsset//m_abz//solve/mat/ pos = 32 ; key = st_mat_prefix ; val = pos = 33 ; key = st_mat_suffix ; val = _p4 pos = 34 ; key = st_mat_test_path ; val = C:/Users/fan/CodeDynaAsset//m_abz//test/ff_az_ds_vecsv/mat/ pos = 35 ; key = st_matimg_path_root ; val = C:/Users/fan/CodeDynaAsset//m_abz/ pos = 36 ; key = st_profile_name_main ; val = ff_abz_vf_default pos = 37 ; key = st_profile_path ; val = C:/Users/fan/CodeDynaAsset//m_abz//solve/profile/ pos = 38 ; key = st_profile_prefix ; val = pos = 39 ; key = st_profile_suffix ; val = _p4 pos = 40 ; key = st_title_prefix ; val = ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Scalars in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx value __ ___ _____ bl_display 1 1 0 bl_display_defparam 2 2 1 bl_display_dist 3 3 0 bl_display_final 4 4 1 bl_display_final_dist 5 5 0 bl_display_final_dist_detail 6 6 0 bl_display_funcgrids 7 7 0 bl_graph 8 8 1 bl_graph_coh_t_coh 9 9 1 bl_graph_funcgrids 10 10 0 bl_graph_onebyones 11 11 1 bl_graph_pol_lvl 12 12 1 bl_graph_pol_pct 13 13 1 bl_graph_val 14 14 1 bl_img_save 15 15 0 bl_mat 16 16 0 bl_post 17 17 1 bl_profile 18 18 0 bl_profile_dist 19 19 0 bl_time 20 20 1 it_display_every 21 21 5 it_display_final_colmax 22 22 15 it_display_final_rowmax 23 23 100 it_display_summmat_colmax 24 24 5 it_display_summmat_rowmax 25 25 5
Initialize Output Matrixes
mt_val_cur = zeros(length(ar_a),it_z_n); mt_val = mt_val_cur - 1; mt_pol_a = zeros(length(ar_a),it_z_n); mt_pol_a_cur = mt_pol_a - 1;
Initialize Convergence Conditions
bl_vfi_continue = true; it_iter = 0; ar_val_diff_norm = zeros([it_maxiter_val, 1]); ar_pol_diff_norm = zeros([it_maxiter_val, 1]); mt_pol_perc_change = zeros([it_maxiter_val, it_z_n]);
Iterate Value Function
Loop solution with 4 nested loops
- loop 1: over exogenous states
- loop 2: over endogenous states
- loop 3: over choices
- loop 4: add future utility, integration--loop over future shocks
% Start Profile if (bl_profile) close all; profile off; profile on; end % Start Timer if (bl_time) tic; end % Utility at-Default/at-limiting-case-when-nodefault if (fl_crra == 1) fl_u_cmin = f_util_log(fl_c_min); else fl_u_cmin = f_util_crra(fl_c_min); end % Value Function Iteration while bl_vfi_continue
it_iter = it_iter + 1;
Iterate over a and z states
Solve Problem if Default: coh < borr_bound, then: u(c_min) + EV(a'=0,z') these are the values for defaulter if do not borrow enough to pay debt: a'=0 if save more than what you have: a'=0 if borrowing, possible that all ar_val_cur values are u(cmin), when that is the case, utility equal to c_min, this means given current choice set, can only default, get utility at c_min level, and a' go to 0. See maximization over loop 3 choices for loop 1+2 states. see README_cminymin_borrsave for additional discussions.
when default is not allowed, there should be exactly one element of the state space where we enter this condition. That is at a_min and z_min. At this point, the natural borrowing constraint when fully used up leads to c = 0. When default is not allowed, this is the limiting case. We solve the limiting case as if default is possible. see the figure here: ffs_abz_get_funcgrid_nodefault. For the figure under section Generate Borrowing A Grid with Default, there are no points to the lower right of the red horizontal and verticle lines, because no default is allowed. But the intersection of the two red lines is this limiting case where c = 0.
when default is allowed. See again ffs_abz_get_funcgrid_nodefault. Again under section Generate Borrowing A Grid with Default, see that there is an area to the right bottom of the two blue horizontal and vertical lines. For all points in that area, the household has to default, they can not borrow even at max to get positive consumption. At other points higher than the blue horizontal line, it is also possible for defaulting to be the optimal choice.
% loop 1: over exogenous states for it_z_i = 1:it_z_n % Current Shock fl_z_r_borr = ar_z_r_borr_mesh_wage(it_z_i); fl_z_wage = ar_z_wage_mesh_r_borr(it_z_i); % loop 2: over endogenous states for it_a_j = 1:length(ar_a)
fl_a = ar_a(it_a_j); ar_val_cur = zeros(size(ar_a)); % calculate cash on hand fl_coh = f_coh(fl_z_wage, fl_a); % loop 3: over choices for it_ap_k = 1:length(ar_a) % get next period asset choice fl_ap = ar_a(it_ap_k); % calculate consumption fl_c = f_cons_coh(fl_coh, fl_z_r_borr, fl_ap); % assign u(c) if (fl_c <= fl_c_min) if (bl_default) % defaults % current utility: only today u(cmin) ar_val_cur(it_ap_k) = fl_u_cmin; % transition out next period, debt wiped out for it_az_q = 1:it_z_n ar_val_cur(it_ap_k) = ar_val_cur(it_ap_k) + ... fl_beta*mt_z_trans(it_z_i, it_az_q)*mt_val_cur((ar_a == fl_default_aprime), it_az_q); end else % if default is not allowed: v = fl_nan_replace ar_val_cur(it_ap_k) = fl_nan_replace; end else % Solve Optimization Problem: max_{a'} u(c(a,a',z)) + beta*EV(a',z') % borrowed enough to pay debt (and borrowing limit not exceeded) % saved only the coh available. % current utility if (fl_crra == 1) ar_val_cur(it_ap_k) = f_util_log(fl_c); else ar_val_cur(it_ap_k) = f_util_crra(fl_c); end % loop 4: add future utility, integration--loop over future shocks for it_az_q = 1:it_z_n ar_val_cur(it_ap_k) = ar_val_cur(it_ap_k) + ... fl_beta*mt_z_trans(it_z_i, it_az_q)*mt_val_cur(it_ap_k, it_az_q); end end end % optimal choice value [fl_opti_val_z, fl_opti_idx_z] = max(ar_val_cur); fl_opti_aprime_z = ar_a(fl_opti_idx_z); fl_opti_c_z = f_cons_coh(fl_coh, fl_z_r_borr, fl_opti_aprime_z); % Handle Default is optimal or not if (fl_opti_c_z <= fl_c_min) if (bl_default) % if defaulting is optimal choice, at these states, not required % to default, non-default possible, but default could be optimal fl_opti_aprime_z = fl_default_aprime; else % if default is not allowed, then next period same state as now % this is absorbing state, this is the limiting case, single % state space point, lowest a and lowest shock has this. fl_opti_aprime_z = fl_a; end end
Store Optimal Choices and Value Given(a,z)
mt_val(it_a_j,it_z_i) = fl_opti_val_z; mt_pol_a(it_a_j,it_z_i) = fl_opti_aprime_z;
end end
Check Tolerance and Continuation
% Difference across iterations ar_val_diff_norm(it_iter) = norm(mt_val - mt_val_cur); ar_pol_diff_norm(it_iter) = norm(mt_pol_a - mt_pol_a_cur); mt_pol_perc_change(it_iter, :) = sum((mt_pol_a ~= mt_pol_a_cur))/(it_a_n); % Update mt_val_cur = mt_val; mt_pol_a_cur = mt_pol_a; % Print Iteration Results if (bl_display && (rem(it_iter, it_display_every)==0)) fprintf('VAL it_iter:%d, fl_diff:%d, fl_diff_pol:%d\n', ... it_iter, ar_val_diff_norm(it_iter), ar_pol_diff_norm(it_iter)); tb_valpol_iter = array2table([mean(mt_val_cur,1); mean(mt_pol_a_cur,1); ... mt_val_cur(it_a_n,:); mt_pol_a_cur(it_a_n,:)]); tb_valpol_iter.Properties.VariableNames = strcat('z', string((1:size(mt_val_cur,2)))); tb_valpol_iter.Properties.RowNames = {'mval', 'map', 'Hval', 'Hap'}; disp('mval = mean(mt_val_cur,1), average value over a') disp('map = mean(mt_pol_a_cur,1), average choice over a') disp('Hval = mt_val_cur(it_a_n,:), highest a state val') disp('Hap = mt_pol_a_cur(it_a_n,:), highest a state choice') disp(tb_valpol_iter); end % Continuation Conditions: % 1. if value function convergence criteria reached % 2. if policy function variation over iterations is less than % threshold if (it_iter == (it_maxiter_val + 1)) bl_vfi_continue = false; elseif ((it_iter == it_maxiter_val) || ... (ar_val_diff_norm(it_iter) < fl_tol_val) || ... (sum(ar_pol_diff_norm(max(1, it_iter-it_tol_pol_nochange):it_iter)) < fl_tol_pol)) % Fix to max, run again to save results if needed it_iter_last = it_iter; it_iter = it_maxiter_val; end
end % End Timer if (bl_time) toc; end % End Profile if (bl_profile) profile off profile viewer st_file_name = [st_profile_prefix st_profile_name_main st_profile_suffix]; profsave(profile('info'), strcat(st_profile_path, st_file_name)); end
Elapsed time is 612.536238 seconds.
Process Optimal Choices
result_map = containers.Map('KeyType','char', 'ValueType','any'); result_map('mt_val') = mt_val; result_map('cl_mt_val') = {mt_val, zeros(1)}; result_map('cl_mt_coh') = {f_coh(ar_z_wage_mesh_r_borr, ar_a'), zeros(1)}; result_map('cl_mt_pol_a') = {f_awithr_to_anor(ar_z_r_borr_mesh_wage, mt_pol_a), zeros(1)}; result_map('cl_mt_pol_c') = {f_cons_checkcmin(ar_z_r_borr_mesh_wage, ar_z_wage_mesh_r_borr, ar_a', mt_pol_a), zeros(1)}; result_map('ar_st_pol_names') = ["cl_mt_val", "cl_mt_pol_a", "cl_mt_coh", "cl_mt_pol_c"]; if (bl_post) bl_input_override = true; result_map('ar_val_diff_norm') = ar_val_diff_norm(1:it_iter_last); result_map('ar_pol_diff_norm') = ar_pol_diff_norm(1:it_iter_last); result_map('mt_pol_perc_change') = mt_pol_perc_change(1:it_iter_last, :); result_map = ff_az_vf_post(param_map, support_map, armt_map, func_map, result_map, bl_input_override); end
valgap = norm(mt_val - mt_val_cur): value function difference across iterations polgap = norm(mt_pol_a - mt_pol_a_cur): policy function difference across iterations z1 = z1 perc change: sum((mt_pol_a ~= mt_pol_a_cur))/(it_a_n): percentage of state space points conditional on shock where the policy function is changing across iterations valgap polgap zi1_zr_0_025_zw_0_33942 zi2_zr_0_025_zw_0_5596 zi3_zr_0_025_zw_0_92263 zi4_zr_0_025_zw_1_5212 zi5_zr_0_025_zw_2_508 zi6_zr_0_06_zw_0_33942 zi7_zr_0_06_zw_0_5596 zi8_zr_0_06_zw_0_92263 zi9_zr_0_06_zw_1_5212 zi10_zr_0_06_zw_2_508 zi11_zr_0_095_zw_0_33942 zi12_zr_0_095_zw_0_5596 zi13_zr_0_095_zw_0_92263 zi14_zr_0_095_zw_1_5212 zi15_zr_0_095_zw_2_508 ________ ______ _______________________ ______________________ _______________________ ______________________ _____________________ ______________________ _____________________ ______________________ _____________________ _____________________ ________________________ _______________________ ________________________ _______________________ ______________________ iter=1 65.172 633.35 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 iter=2 55.481 697.53 0.98667 1 0.98667 1 1 0.98667 0.98667 1 0.98667 1 0.97333 0.96 0.97333 0.98667 1 iter=3 42.718 222.16 0.96 0.97333 0.96 0.97333 1 0.97333 0.96 0.98667 0.97333 0.97333 0.94667 0.97333 0.96 0.96 0.98667 iter=4 34.252 114.2 0.92 0.94667 0.93333 0.94667 0.96 0.92 0.92 0.93333 0.93333 0.96 0.90667 0.94667 0.92 0.94667 0.96 iter=5 28.491 65.961 0.86667 0.88 0.84 0.88 0.89333 0.85333 0.85333 0.88 0.88 0.90667 0.86667 0.88 0.85333 0.88 0.92 iter=6 24.283 45.62 0.82667 0.77333 0.77333 0.81333 0.85333 0.82667 0.77333 0.78667 0.81333 0.86667 0.8 0.77333 0.76 0.82667 0.82667 iter=7 20.984 33.283 0.72 0.70667 0.70667 0.72 0.78667 0.72 0.74667 0.70667 0.69333 0.77333 0.74667 0.72 0.72 0.72 0.76 iter=8 18.295 24.642 0.61333 0.62667 0.6 0.64 0.65333 0.6 0.6 0.62667 0.61333 0.69333 0.6 0.61333 0.6 0.61333 0.66667 iter=9 16.057 22.22 0.44 0.49333 0.48 0.50667 0.56 0.44 0.48 0.49333 0.49333 0.56 0.45333 0.48 0.48 0.50667 0.54667 iter=10 14.16 16.068 0.36 0.42667 0.42667 0.41333 0.45333 0.38667 0.41333 0.4 0.38667 0.48 0.38667 0.41333 0.4 0.41333 0.45333 iter=11 12.527 12.742 0.28 0.28 0.29333 0.32 0.37333 0.30667 0.26667 0.30667 0.33333 0.38667 0.29333 0.28 0.30667 0.34667 0.38667 iter=12 11.082 11.61 0.25333 0.26667 0.26667 0.26667 0.29333 0.24 0.24 0.28 0.22667 0.29333 0.24 0.24 0.28 0.22667 0.26667 iter=13 9.7712 10.41 0.22667 0.21333 0.22667 0.24 0.24 0.22667 0.2 0.21333 0.22667 0.25333 0.22667 0.21333 0.21333 0.22667 0.22667 iter=14 8.6441 9.1353 0.18667 0.21333 0.2 0.17333 0.22667 0.13333 0.21333 0.21333 0.17333 0.22667 0.14667 0.18667 0.22667 0.16 0.21333 iter=15 7.6535 9.3668 0.18667 0.13333 0.16 0.18667 0.18667 0.17333 0.14667 0.18667 0.18667 0.2 0.17333 0.13333 0.18667 0.18667 0.17333 iter=16 6.7818 7.5957 0.12 0.13333 0.16 0.13333 0.17333 0.093333 0.13333 0.14667 0.14667 0.18667 0.093333 0.10667 0.14667 0.12 0.16 iter=17 6.0432 7.3872 0.12 0.10667 0.12 0.13333 0.12 0.10667 0.13333 0.12 0.12 0.12 0.10667 0.10667 0.13333 0.10667 0.12 iter=18 5.4139 7.8713 0.093333 0.14667 0.093333 0.10667 0.14667 0.093333 0.14667 0.08 0.12 0.16 0.10667 0.13333 0.08 0.12 0.14667 iter=19 4.8819 6.9847 0.10667 0.08 0.08 0.10667 0.08 0.10667 0.093333 0.08 0.12 0.093333 0.12 0.066667 0.08 0.093333 0.12 iter=20 4.4358 6.0895 0.053333 0.08 0.066667 0.10667 0.093333 0.053333 0.093333 0.066667 0.093333 0.093333 0.053333 0.093333 0.08 0.093333 0.10667 iter=21 4.038 4.3313 0.026667 0.08 0.093333 0.053333 0.053333 0.04 0.08 0.093333 0.066667 0.053333 0.04 0.066667 0.093333 0.04 0.066667 iter=22 3.676 5.8123 0.093333 0.053333 0.066667 0.066667 0.093333 0.066667 0.066667 0.08 0.066667 0.10667 0.08 0.053333 0.066667 0.10667 0.093333 iter=23 3.35 4.5998 0.04 0.053333 0.093333 0.053333 0.066667 0.053333 0.04 0.093333 0.066667 0.066667 0.04 0.04 0.093333 0.066667 0.08 iter=24 3.0579 4.0683 0.04 0.053333 0.04 0.04 0.053333 0.04 0.053333 0.04 0.04 0.053333 0.04 0.053333 0.04 0.04 0.053333 iter=25 2.8022 4.4267 0.04 0.053333 0.026667 0.053333 0.053333 0.053333 0.053333 0.026667 0.053333 0.066667 0.04 0.066667 0.026667 0.053333 0.053333 iter=26 2.5729 3.5821 0.04 0.04 0.026667 0.04 0.053333 0.04 0.053333 0.026667 0.04 0.053333 0.04 0.04 0.04 0.053333 0.053333 iter=27 2.3659 3.3645 0.04 0.04 0.026667 0.04 0.053333 0.04 0.04 0.026667 0.04 0.04 0.04 0.04 0.026667 0.04 0.053333 iter=28 2.1864 3.4895 0.026667 0 0.04 0.053333 0.013333 0.026667 0 0.04 0.053333 0.013333 0.026667 0.013333 0.04 0.053333 0.013333 iter=29 2.0277 2.9335 0.04 0.026667 0.026667 0.013333 0.026667 0.04 0.026667 0.026667 0.013333 0.026667 0.053333 0.026667 0.026667 0.013333 0.026667 iter=30 1.8867 2.3488 0.013333 0.013333 0.026667 0.026667 0.026667 0.013333 0.013333 0.026667 0.026667 0.026667 0.013333 0.013333 0.026667 0.026667 0.026667 iter=31 1.7625 2.3488 0.026667 0.026667 0.026667 0.013333 0.013333 0.026667 0.026667 0.026667 0.013333 0.013333 0.026667 0.026667 0.026667 0.013333 0.013333 iter=32 1.6519 2.3488 0 0.013333 0.013333 0.013333 0.026667 0 0.013333 0.013333 0.013333 0.026667 0 0.013333 0.013333 0.013333 0.026667 iter=33 1.5532 2.8767 0.013333 0.013333 0.04 0.013333 0.026667 0.013333 0.013333 0.04 0.013333 0.026667 0.013333 0.013333 0.04 0.013333 0.026667 iter=34 1.4635 2.3488 0.026667 0.013333 0.026667 0.026667 0.013333 0.026667 0.013333 0.026667 0.026667 0.013333 0.026667 0.013333 0.026667 0.026667 0.013333 iter=35 1.3818 2.6873 0 0.026667 0.013333 0.013333 0.013333 0 0.026667 0.013333 0.013333 0.013333 0 0.026667 0.013333 0.013333 0.013333 iter=36 1.3067 1.6609 0.013333 0.013333 0.013333 0 0.013333 0.013333 0.013333 0.013333 0 0.013333 0.013333 0.013333 0.013333 0 0.013333 iter=37 1.2375 2.3488 0.013333 0 0.013333 0.026667 0.026667 0.013333 0 0.013333 0.026667 0.026667 0.013333 0 0.013333 0.026667 0.026667 iter=38 1.1731 2.3488 0 0.026667 0.026667 0.013333 0 0 0.026667 0.026667 0.013333 0 0 0.026667 0.026667 0.013333 0 iter=39 1.1133 2.3488 0.013333 0 0 0 0.026667 0.013333 0 0 0 0.026667 0.013333 0 0 0 0.026667 iter=40 1.0572 2.3488 0 0.026667 0.026667 0.013333 0.013333 0 0.013333 0.026667 0.013333 0.013333 0 0.013333 0.026667 0.013333 0.013333 iter=41 1.0048 2.3488 0.013333 0 0.013333 0.026667 0 0.013333 0 0.013333 0.026667 0 0.013333 0 0.013333 0.026667 0 iter=42 0.95538 2.3488 0.013333 0.013333 0.013333 0 0.026667 0.013333 0.013333 0.013333 0 0.026667 0.013333 0.013333 0.013333 0 0.026667 iter=43 0.90874 2.3488 0.013333 0.026667 0 0.013333 0 0.013333 0.026667 0 0.013333 0 0.013333 0.026667 0 0.013333 0 iter=44 0.86469 1.6609 0 0 0.013333 0 0.013333 0 0 0.013333 0 0.013333 0 0 0.013333 0 0.013333 iter=45 0.82291 1.6609 0.013333 0.013333 0 0.013333 0.013333 0.013333 0.013333 0 0.013333 0.013333 0.013333 0.013333 0 0.013333 0.013333 iter=46 0.78326 1.6609 0 0 0 0 0.013333 0 0 0 0 0.013333 0 0 0 0 0.013333 iter=47 0.74564 1.6609 0 0.013333 0 0.013333 0 0 0.013333 0 0.013333 0 0 0.013333 0 0.013333 0 iter=48 0.70974 1.6609 0.013333 0 0.013333 0 0.013333 0.013333 0 0.013333 0 0.013333 0.013333 0 0.013333 0 0.013333 iter=49 0.67549 1.6609 0 0 0 0.013333 0 0 0 0 0.013333 0 0 0 0 0.013333 0 iter=50 0.64274 1.6609 0 0.013333 0 0 0.013333 0 0.013333 0 0 0.013333 0 0.013333 0 0 0.013333 iter=51 0.61134 1.6609 0.013333 0 0 0.013333 0.013333 0.013333 0 0 0.013333 0.013333 0.013333 0 0 0.013333 0.013333 iter=52 0.58127 1.6609 0 0 0 0 0.013333 0 0 0 0 0.013333 0 0 0 0 0.013333 iter=53 0.55243 1.6609 0 0.013333 0 0 0 0 0.013333 0 0 0 0 0.013333 0 0 0 iter=54 0.52475 1.6609 0 0 0 0.013333 0 0 0 0 0.013333 0 0 0 0 0.013333 0 iter=55 0.49815 1.6609 0.013333 0 0 0 0.013333 0.013333 0 0 0 0.013333 0.013333 0 0 0 0.013333 iter=56 0.47262 1.6609 0 0.013333 0 0 0 0 0.013333 0 0 0 0 0.013333 0 0 0 iter=57 0.44812 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=58 0.42461 1.6609 0 0 0.013333 0.013333 0 0 0 0.013333 0.013333 0 0 0 0.013333 0.013333 0 iter=59 0.40209 1.6609 0 0 0 0 0.013333 0 0 0 0 0.013333 0 0 0 0 0.013333 iter=60 0.38054 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=61 0.35996 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=62 0.3403 1.6609 0 0.013333 0 0 0 0 0.013333 0 0 0 0 0.013333 0 0 0 iter=63 0.32156 2.3488 0 0 0 0.026667 0 0 0 0 0.026667 0 0 0 0 0.026667 0 iter=64 0.30371 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=65 0.28674 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=66 0.27061 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=67 0.25531 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=68 0.2408 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=69 0.22704 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=70 0.21402 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=71 0.2017 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=72 0.19006 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=73 0.17904 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=74 0.16864 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=75 0.15882 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=76 0.14955 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=77 0.1408 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=78 0.13254 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=79 0.12476 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=80 0.11742 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=81 0.1105 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=82 0.10398 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=83 0.097838 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=84 0.092051 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=85 0.086601 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=86 0.081469 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=87 0.076637 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=88 0.072088 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=89 0.067805 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 tb_val: V(a,z) value at each state space point zi1_zr_0_025_zw_0_33942 zi2_zr_0_025_zw_0_5596 zi3_zr_0_025_zw_0_92263 zi4_zr_0_025_zw_1_5212 zi5_zr_0_025_zw_2_508 zi6_zr_0_06_zw_0_33942 zi7_zr_0_06_zw_0_5596 zi8_zr_0_06_zw_0_92263 zi9_zr_0_06_zw_1_5212 zi10_zr_0_06_zw_2_508 zi11_zr_0_095_zw_0_33942 zi12_zr_0_095_zw_0_5596 zi13_zr_0_095_zw_0_92263 zi14_zr_0_095_zw_1_5212 zi15_zr_0_095_zw_2_508 _______________________ ______________________ _______________________ ______________________ _____________________ ______________________ _____________________ ______________________ _____________________ _____________________ ________________________ _______________________ ________________________ _______________________ ______________________ a1=-20 -18.809 -17.407 -15.294 -13.317 -10.304 -18.809 -17.407 -15.849 -13.876 -10.785 -18.809 -17.407 -15.849 -14.415 -11.392 a2=-19.0411 -17.247 -15.914 -14.447 -12.632 -9.4616 -18.809 -16.793 -14.884 -13.134 -9.9416 -18.809 -17.407 -15.849 -13.509 -10.541 a3=-18.0822 -16.61 -15.444 -13.965 -11.825 -8.6469 -16.956 -15.711 -14.318 -12.276 -9.0896 -17.691 -16.188 -14.604 -12.775 -9.6088 a4=-17.1233 -15.994 -14.654 -13.175 -11.103 -7.8244 -16.5 -15.331 -13.533 -11.508 -8.2666 -16.738 -15.546 -14.204 -11.925 -8.7072 a5=-16.1644 -15.256 -14.09 -12.604 -10.343 -6.9898 -15.68 -14.429 -12.998 -10.706 -7.4369 -16.204 -14.791 -13.257 -11.164 -7.8125 a6=-15.2055 -14.61 -13.33 -11.863 -9.6209 -6.103 -14.932 -13.856 -12.156 -9.9467 -6.5962 -15.379 -14.172 -12.678 -10.369 -6.9173 a7=-14.2466 -13.9 -12.728 -11.215 -8.8908 -5.1663 -14.277 -13.089 -11.515 -9.1821 -5.704 -14.661 -13.365 -11.885 -9.6156 -5.9788 a8=-13.2877 -13.179 -11.973 -10.477 -8.081 -4.2372 -13.56 -12.383 -10.756 -8.3719 -4.7351 -13.89 -12.721 -11.128 -8.7864 -4.9977 a9=-12.3288 -12.481 -11.315 -9.6894 -7.1681 -3.31 -12.832 -11.676 -10.014 -7.4583 -3.7326 -13.116 -11.929 -10.329 -7.804 -4.0304 a10=-11.3699 -11.737 -10.569 -8.8809 -6.2673 -2.3639 -12.128 -10.886 -9.2232 -6.5477 -2.7212 -12.373 -11.238 -9.491 -6.8365 -3.0699 a11=-10.411 -11.034 -9.8619 -8.0584 -5.3751 -1.4136 -11.378 -10.139 -8.412 -5.6496 -1.7138 -11.589 -10.462 -8.6392 -5.8908 -2.0948 a12=-9.45205 -10.287 -9.1078 -7.233 -4.4969 -0.46125 -10.644 -9.3495 -7.5873 -4.7604 -0.71103 -10.853 -9.7065 -7.7797 -4.9613 -1.0642 a13=-8.49315 -9.5665 -8.3601 -6.404 -3.6155 0.47175 -9.8705 -8.5691 -6.7602 -3.8855 0.26661 -10.075 -8.8589 -6.9224 -4.0518 -0.0023497 a14=-7.53425 -8.7964 -7.5605 -5.5635 -2.6411 1.4272 -9.0536 -7.7398 -5.8632 -2.9911 1.2618 -9.3275 -7.9745 -6.0658 -3.1441 1.0589 a15=-6.57534 -8.0216 -6.7326 -4.7003 -1.479 2.2706 -8.237 -6.8847 -4.9444 -1.7541 2.1408 -8.5285 -7.0737 -5.2012 -2.1371 1.9907 a16=-5.61644 -7.2167 -5.8886 -3.6317 -0.38738 3.0307 -7.3947 -6.0157 -3.8272 -0.59839 2.933 -7.6203 -6.1665 -4.0756 -0.86567 2.8257 a17=-4.65753 -6.3557 -4.9828 -2.4737 0.60261 3.6916 -6.5 -5.0869 -2.626 0.44692 3.6228 -6.6725 -5.2052 -2.8069 0.26485 3.5507 a18=-3.69863 -5.4505 -3.9496 -1.3854 1.5011 4.2901 -5.5643 -4.0925 -1.4994 1.3935 4.2476 -5.6934 -4.1831 -1.6269 1.276 4.2048 a19=-2.73973 -4.5039 -2.9184 -0.36632 2.3172 4.7864 -4.5899 -3.0739 -0.44614 2.2517 4.7676 -4.6831 -3.1405 -0.5308 2.1844 4.7495 a20=-1.78082 -3.5319 -1.9048 0.58667 3.0549 5.2448 -3.5927 -2.0159 0.53752 3.0266 5.2448 -3.6558 -2.1048 0.48783 2.9989 5.2448 a21=-0.821918 -2.55 -0.72707 1.5625 3.6999 5.6293 -2.5878 -0.77398 1.5409 3.6999 5.6293 -2.6255 -0.82049 1.5201 3.6999 5.6293 a22=0 -1.5699 0.22988 2.3102 4.1611 5.955 -1.5894 0.22988 2.3102 4.1611 5.955 -1.6083 0.22988 2.3102 4.1611 5.955 a23=0.136986 -1.4544 0.42786 2.4167 4.2142 5.9967 -1.4544 0.42786 2.4167 4.2142 5.9967 -1.4544 0.42786 2.4167 4.2142 5.9967 a24=1.09589 -0.34206 1.2141 2.9567 4.6541 6.3419 -0.34206 1.2141 2.9567 4.6541 6.3419 -0.34206 1.2141 2.9567 4.6541 6.3419 a25=2.05479 0.59383 1.9235 3.4527 5.0613 6.6701 0.59383 1.9235 3.4527 5.0613 6.6701 0.59383 1.9235 3.4527 5.0613 6.6701 a26=3.0137 1.4012 2.5638 3.9091 5.4394 6.9875 1.4012 2.5638 3.9091 5.4394 6.9875 1.4012 2.5638 3.9091 5.4394 6.9875 a27=3.9726 2.1099 3.143 4.3505 5.8055 7.2941 2.1099 3.143 4.3505 5.8055 7.2941 2.1099 3.143 4.3505 5.8055 7.2941 a28=4.93151 2.7399 3.673 4.7786 6.1605 7.5891 2.7399 3.673 4.7786 6.1605 7.5891 2.7399 3.673 4.7786 6.1605 7.5891 a29=5.89041 3.3067 4.1629 5.1908 6.5027 7.8724 3.3067 4.1629 5.1908 6.5027 7.8724 3.3067 4.1629 5.1908 6.5027 7.8724 a30=6.84932 3.8228 4.6194 5.5859 6.8313 8.1439 3.8228 4.6194 5.5859 6.8313 8.1439 3.8228 4.6194 5.5859 6.8313 8.1439 a31=7.80822 4.2974 5.0471 5.9635 7.1462 8.4041 4.2974 5.0471 5.9635 7.1462 8.4041 4.2974 5.0471 5.9635 7.1462 8.4041 a32=8.76712 4.7375 5.4495 6.3238 7.4474 8.6534 4.7375 5.4495 6.3238 7.4474 8.6534 4.7375 5.4495 6.3238 7.4474 8.6534 a33=9.72603 5.1485 5.8292 6.6674 7.7355 8.8924 5.1485 5.8292 6.6674 7.7355 8.8924 5.1485 5.8292 6.6674 7.7355 8.8924 a34=10.6849 5.5343 6.1885 6.9948 8.011 9.1216 5.5343 6.1885 6.9948 8.011 9.1216 5.5343 6.1885 6.9948 8.011 9.1216 a35=11.6438 5.8979 6.5292 7.3069 8.2745 9.3417 5.8979 6.5292 7.3069 8.2745 9.3417 5.8979 6.5292 7.3069 8.2745 9.3417 a36=12.6027 6.2419 6.8528 7.6046 8.5267 9.5533 6.2419 6.8528 7.6046 8.5267 9.5533 6.2419 6.8528 7.6046 8.5267 9.5533 a37=13.5616 6.5681 7.1607 7.8886 8.7682 9.757 6.5681 7.1607 7.8886 8.7682 9.757 6.5681 7.1607 7.8886 8.7682 9.757 a38=14.5205 6.8782 7.454 8.1597 8.9997 9.9537 6.8782 7.454 8.1597 8.9997 9.9537 6.8782 7.454 8.1597 8.9997 9.9537 a39=15.4795 7.1804 7.7337 8.4189 9.222 10.144 7.1804 7.7337 8.4189 9.222 10.144 7.1804 7.7337 8.4189 9.222 10.144 a40=16.4384 7.4708 8.0011 8.6668 9.4355 10.33 7.4708 8.0011 8.6668 9.4355 10.33 7.4708 8.0011 8.6668 9.4355 10.33 a41=17.3973 7.7515 8.257 8.9041 9.6412 10.512 7.7515 8.257 8.9041 9.6412 10.512 7.7515 8.257 8.9041 9.6412 10.512 a42=18.3562 8.0209 8.5023 9.1316 9.844 10.691 8.0209 8.5023 9.1316 9.844 10.691 8.0209 8.5023 9.1316 9.844 10.691 a43=19.3151 8.2805 8.739 9.3505 10.043 10.865 8.2805 8.739 9.3505 10.043 10.865 8.2805 8.739 9.3505 10.043 10.865 a44=20.274 8.5296 8.971 9.5616 10.237 11.035 8.5296 8.971 9.5616 10.237 11.035 8.5296 8.971 9.5616 10.237 11.035 a45=21.2329 8.7698 9.1949 9.7659 10.426 11.202 8.7698 9.1949 9.7659 10.426 11.202 8.7698 9.1949 9.7659 10.426 11.202 a46=22.1918 9.0024 9.4134 9.9638 10.609 11.366 9.0024 9.4134 9.9638 10.609 11.366 9.0024 9.4134 9.9638 10.609 11.366 a47=23.1507 9.2268 9.6244 10.156 10.788 11.528 9.2268 9.6244 10.156 10.788 11.528 9.2268 9.6244 10.156 10.788 11.528 a48=24.1096 9.4449 9.8301 10.342 10.962 11.685 9.4449 9.8301 10.342 10.962 11.685 9.4449 9.8301 10.342 10.962 11.685 a49=25.0685 9.6555 10.029 10.523 11.131 11.838 9.6555 10.029 10.523 11.131 11.838 9.6555 10.029 10.523 11.131 11.838 a50=26.0274 9.8604 10.223 10.699 11.295 11.987 9.8604 10.223 10.699 11.295 11.987 9.8604 10.223 10.699 11.295 11.987 a51=26.9863 10.058 10.41 10.87 11.455 12.132 10.058 10.41 10.87 11.455 12.132 10.058 10.41 10.87 11.455 12.132 a52=27.9452 10.251 10.593 11.037 11.611 12.274 10.251 10.593 11.037 11.611 12.274 10.251 10.593 11.037 11.611 12.274 a53=28.9041 10.438 10.77 11.2 11.763 12.411 10.438 10.77 11.2 11.763 12.411 10.438 10.77 11.2 11.763 12.411 a54=29.863 10.62 10.943 11.361 11.911 12.546 10.62 10.943 11.361 11.911 12.546 10.62 10.943 11.361 11.911 12.546 a55=30.8219 10.796 11.111 11.518 12.055 12.678 10.796 11.111 11.518 12.055 12.678 10.796 11.111 11.518 12.055 12.678 a56=31.7808 10.968 11.275 11.672 12.197 12.807 10.968 11.275 11.672 12.197 12.807 10.968 11.275 11.672 12.197 12.807 a57=32.7397 11.135 11.434 11.822 12.335 12.933 11.135 11.434 11.822 12.335 12.933 11.135 11.434 11.822 12.335 12.933 a58=33.6986 11.298 11.59 11.97 12.471 13.056 11.298 11.59 11.97 12.471 13.056 11.298 11.59 11.97 12.471 13.056 a59=34.6575 11.456 11.742 12.114 12.603 13.178 11.456 11.742 12.114 12.603 13.178 11.456 11.742 12.114 12.603 13.178 a60=35.6164 11.61 11.891 12.255 12.734 13.297 11.61 11.891 12.255 12.734 13.297 11.61 11.891 12.255 12.734 13.297 a61=36.5753 11.761 12.036 12.394 12.861 13.413 11.761 12.036 12.394 12.861 13.413 11.761 12.036 12.394 12.861 13.413 a62=37.5342 11.908 12.178 12.529 12.986 13.527 11.908 12.178 12.529 12.986 13.527 11.908 12.178 12.529 12.986 13.527 a63=38.4932 12.051 12.317 12.662 13.108 13.639 12.051 12.317 12.662 13.108 13.639 12.051 12.317 12.662 13.108 13.639 a64=39.4521 12.192 12.452 12.791 13.228 13.749 12.192 12.452 12.791 13.228 13.749 12.192 12.452 12.791 13.228 13.749 a65=40.411 12.329 12.585 12.918 13.346 13.857 12.329 12.585 12.918 13.346 13.857 12.329 12.585 12.918 13.346 13.857 a66=41.3699 12.463 12.715 13.043 13.461 13.962 12.463 12.715 13.043 13.461 13.962 12.463 12.715 13.043 13.461 13.962 a67=42.3288 12.594 12.842 13.165 13.574 14.066 12.594 12.842 13.165 13.574 14.066 12.594 12.842 13.165 13.574 14.066 a68=43.2877 12.723 12.967 13.284 13.685 14.168 12.723 12.967 13.284 13.685 14.168 12.723 12.967 13.284 13.685 14.168 a69=44.2466 12.85 13.088 13.401 13.793 14.268 12.85 13.088 13.401 13.793 14.268 12.85 13.088 13.401 13.793 14.268 a70=45.2055 12.975 13.208 13.516 13.9 14.365 12.975 13.208 13.516 13.9 14.365 12.975 13.208 13.516 13.9 14.365 a71=46.1644 13.097 13.325 13.628 14.004 14.462 13.097 13.325 13.628 14.004 14.462 13.097 13.325 13.628 14.004 14.462 a72=47.1233 13.217 13.44 13.739 14.107 14.556 13.217 13.44 13.739 14.107 14.556 13.217 13.44 13.739 14.107 14.556 a73=48.0822 13.335 13.552 13.847 14.207 14.649 13.335 13.552 13.847 14.207 14.649 13.335 13.552 13.847 14.207 14.649 a74=49.0411 13.45 13.663 13.953 14.307 14.741 13.45 13.663 13.953 14.307 14.741 13.45 13.663 13.953 14.307 14.741 a75=50 13.564 13.772 14.056 14.405 14.831 13.564 13.772 14.056 14.405 14.831 13.564 13.772 14.056 14.405 14.831 tb_pol_a: optimal asset choice for each state space point zi1_zr_0_025_zw_0_33942 zi2_zr_0_025_zw_0_5596 zi3_zr_0_025_zw_0_92263 zi4_zr_0_025_zw_1_5212 zi5_zr_0_025_zw_2_508 zi6_zr_0_06_zw_0_33942 zi7_zr_0_06_zw_0_5596 zi8_zr_0_06_zw_0_92263 zi9_zr_0_06_zw_1_5212 zi10_zr_0_06_zw_2_508 zi11_zr_0_095_zw_0_33942 zi12_zr_0_095_zw_0_5596 zi13_zr_0_095_zw_0_92263 zi14_zr_0_095_zw_1_5212 zi15_zr_0_095_zw_2_508 _______________________ ______________________ _______________________ ______________________ _____________________ ______________________ _____________________ ______________________ _____________________ _____________________ ________________________ _______________________ ________________________ _______________________ ______________________ a1=-20 0 0 -19.512 -19.512 -17.641 0 0 0 -18.868 -17.963 0 0 0 0 -18.265 a2=-19.0411 -19.512 -19.512 -19.512 -18.577 -16.706 0 -18.868 -18.868 -17.963 -17.059 0 0 0 -18.265 -17.389 a3=-18.0822 -19.512 -19.512 -17.641 -17.641 -15.77 -18.868 -18.868 -18.868 -17.059 -16.154 -18.265 -18.265 -18.265 -17.389 -15.638 a4=-17.1233 -17.641 -17.641 -17.641 -16.706 -14.835 -18.868 -17.059 -17.059 -16.154 -15.249 -18.265 -18.265 -18.265 -16.513 -14.762 a5=-16.1644 -16.706 -16.706 -15.77 -15.77 -13.899 -17.059 -17.059 -16.154 -15.249 -14.345 -16.513 -16.513 -16.513 -15.638 -13.886 a6=-15.2055 -15.77 -15.77 -15.77 -14.835 -12.964 -16.154 -16.154 -15.249 -14.345 -13.44 -15.638 -15.638 -14.762 -14.762 -13.011 a7=-14.2466 -14.835 -14.835 -13.899 -13.899 -12.028 -15.249 -15.249 -14.345 -13.44 -12.536 -14.762 -14.762 -14.762 -13.886 -12.135 a8=-13.2877 -13.899 -13.899 -12.964 -12.028 -11.093 -14.345 -13.44 -13.44 -12.536 -10.726 -13.886 -13.886 -13.011 -12.135 -11.259 a9=-12.3288 -12.964 -12.964 -12.028 -11.093 -10.157 -13.44 -12.536 -12.536 -11.631 -9.8217 -13.011 -13.011 -12.135 -11.259 -10.383 a10=-11.3699 -12.028 -12.028 -11.093 -10.157 -9.2215 -12.536 -11.631 -11.631 -10.726 -8.917 -12.135 -12.135 -11.259 -10.383 -9.5077 a11=-10.411 -11.093 -11.093 -10.157 -9.2215 -8.286 -11.631 -10.726 -10.726 -9.8217 -8.0124 -11.259 -11.259 -10.383 -9.5077 -8.632 a12=-9.45205 -10.157 -10.157 -9.2215 -8.286 -7.3505 -9.8217 -9.8217 -9.8217 -8.917 -7.1078 -10.383 -9.5077 -9.5077 -8.632 -6.8806 a13=-8.49315 -9.2215 -9.2215 -8.286 -7.3505 -6.415 -8.917 -8.917 -8.917 -8.0124 -6.2032 -9.5077 -8.632 -8.632 -7.7563 -6.0049 a14=-7.53425 -8.286 -8.286 -7.3505 -6.415 -5.4795 -8.0124 -8.0124 -7.1078 -6.2032 -5.2985 -8.632 -7.7563 -7.7563 -6.8806 -5.1292 a15=-6.57534 -7.3505 -7.3505 -6.415 -5.4795 -4.5439 -7.1078 -7.1078 -6.2032 -5.2985 -4.3939 -6.8806 -6.8806 -6.8806 -5.1292 -4.2535 a16=-5.61644 -6.415 -6.415 -5.4795 -4.5439 -3.6084 -6.2032 -6.2032 -5.2985 -4.3939 -3.4893 -6.0049 -6.0049 -5.1292 -4.2535 -3.3777 a17=-4.65753 -5.4795 -5.4795 -4.5439 -3.6084 -2.6729 -5.2985 -5.2985 -4.3939 -3.4893 -2.5846 -5.1292 -5.1292 -4.2535 -3.3777 -2.502 a18=-3.69863 -4.5439 -3.6084 -3.6084 -2.6729 -1.7374 -4.3939 -4.3939 -3.4893 -2.5846 -1.68 -4.2535 -4.2535 -3.3777 -2.502 -1.6263 a19=-2.73973 -3.6084 -2.6729 -2.6729 -1.7374 -0.80187 -3.4893 -3.4893 -2.5846 -1.68 -0.77539 -3.3777 -3.3777 -2.502 -1.6263 -0.75061 a20=-1.78082 -2.6729 -1.7374 -1.7374 -0.80187 0 -2.5846 -1.68 -1.68 -0.77539 0 -2.502 -2.502 -1.6263 -0.75061 0 a21=-0.821918 -1.7374 -0.80187 -0.80187 0 0.13365 -1.68 -0.77539 -0.77539 0 0.13365 -1.6263 -0.75061 -0.75061 0 0.13365 a22=0 -0.80187 0 0 0.13365 1.0692 -0.77539 0 0 0.13365 1.0692 -0.75061 0 0 0.13365 1.0692 a23=0.136986 0 0 0.13365 0.13365 1.0692 0 0 0.13365 0.13365 1.0692 0 0 0.13365 0.13365 1.0692 a24=1.09589 0.13365 0.13365 1.0692 1.0692 2.0047 0.13365 0.13365 1.0692 1.0692 2.0047 0.13365 0.13365 1.0692 1.0692 2.0047 a25=2.05479 1.0692 1.0692 2.0047 2.0047 2.9402 1.0692 1.0692 2.0047 2.0047 2.9402 1.0692 1.0692 2.0047 2.0047 2.9402 a26=3.0137 2.0047 2.0047 2.9402 2.9402 3.8757 2.0047 2.0047 2.9402 2.9402 3.8757 2.0047 2.0047 2.9402 2.9402 3.8757 a27=3.9726 2.9402 2.9402 2.9402 3.8757 4.8112 2.9402 2.9402 2.9402 3.8757 4.8112 2.9402 2.9402 2.9402 3.8757 4.8112 a28=4.93151 3.8757 3.8757 3.8757 4.8112 5.7467 3.8757 3.8757 3.8757 4.8112 5.7467 3.8757 3.8757 3.8757 4.8112 5.7467 a29=5.89041 4.8112 4.8112 4.8112 5.7467 6.6823 4.8112 4.8112 4.8112 5.7467 6.6823 4.8112 4.8112 4.8112 5.7467 6.6823 a30=6.84932 5.7467 5.7467 5.7467 6.6823 7.6178 5.7467 5.7467 5.7467 6.6823 7.6178 5.7467 5.7467 5.7467 6.6823 7.6178 a31=7.80822 6.6823 6.6823 6.6823 7.6178 8.5533 6.6823 6.6823 6.6823 7.6178 8.5533 6.6823 6.6823 6.6823 7.6178 8.5533 a32=8.76712 7.6178 7.6178 7.6178 8.5533 9.4888 7.6178 7.6178 7.6178 8.5533 9.4888 7.6178 7.6178 7.6178 8.5533 9.4888 a33=9.72603 8.5533 8.5533 8.5533 9.4888 10.424 8.5533 8.5533 8.5533 9.4888 10.424 8.5533 8.5533 8.5533 9.4888 10.424 a34=10.6849 9.4888 9.4888 9.4888 10.424 11.36 9.4888 9.4888 9.4888 10.424 11.36 9.4888 9.4888 9.4888 10.424 11.36 a35=11.6438 10.424 10.424 10.424 11.36 12.295 10.424 10.424 10.424 11.36 12.295 10.424 10.424 10.424 11.36 12.295 a36=12.6027 11.36 11.36 11.36 12.295 13.231 11.36 11.36 11.36 12.295 13.231 11.36 11.36 11.36 12.295 13.231 a37=13.5616 12.295 12.295 12.295 13.231 14.166 12.295 12.295 12.295 13.231 14.166 12.295 12.295 12.295 13.231 14.166 a38=14.5205 13.231 13.231 13.231 14.166 15.102 13.231 13.231 13.231 14.166 15.102 13.231 13.231 13.231 14.166 15.102 a39=15.4795 13.231 14.166 14.166 15.102 16.037 13.231 14.166 14.166 15.102 16.037 13.231 14.166 14.166 15.102 16.037 a40=16.4384 14.166 15.102 15.102 16.037 16.973 14.166 15.102 15.102 16.037 16.973 14.166 15.102 15.102 16.037 16.973 a41=17.3973 15.102 16.037 16.037 16.973 17.908 15.102 16.037 16.037 16.973 17.908 15.102 16.037 16.037 16.973 17.908 a42=18.3562 16.037 16.973 16.973 16.973 18.844 16.037 16.973 16.973 16.973 18.844 16.037 16.973 16.973 16.973 18.844 a43=19.3151 16.973 16.973 17.908 17.908 19.779 16.973 16.973 17.908 17.908 19.779 16.973 16.973 17.908 17.908 19.779 a44=20.274 17.908 17.908 18.844 18.844 20.715 17.908 17.908 18.844 18.844 20.715 17.908 17.908 18.844 18.844 20.715 a45=21.2329 18.844 18.844 19.779 19.779 21.651 18.844 18.844 19.779 19.779 21.651 18.844 18.844 19.779 19.779 21.651 a46=22.1918 19.779 19.779 20.715 20.715 21.651 19.779 19.779 20.715 20.715 21.651 19.779 19.779 20.715 20.715 21.651 a47=23.1507 20.715 20.715 21.651 21.651 22.586 20.715 20.715 21.651 21.651 22.586 20.715 20.715 21.651 21.651 22.586 a48=24.1096 21.651 21.651 22.586 22.586 23.522 21.651 21.651 22.586 22.586 23.522 21.651 21.651 22.586 22.586 23.522 a49=25.0685 22.586 22.586 23.522 23.522 24.457 22.586 22.586 23.522 23.522 24.457 22.586 22.586 23.522 23.522 24.457 a50=26.0274 23.522 23.522 24.457 24.457 25.393 23.522 23.522 24.457 24.457 25.393 23.522 23.522 24.457 24.457 25.393 a51=26.9863 24.457 24.457 25.393 25.393 26.328 24.457 24.457 25.393 25.393 26.328 24.457 24.457 25.393 25.393 26.328 a52=27.9452 25.393 25.393 26.328 26.328 27.264 25.393 25.393 26.328 26.328 27.264 25.393 25.393 26.328 26.328 27.264 a53=28.9041 26.328 26.328 26.328 27.264 28.199 26.328 26.328 26.328 27.264 28.199 26.328 26.328 26.328 27.264 28.199 a54=29.863 27.264 27.264 27.264 28.199 29.135 27.264 27.264 27.264 28.199 29.135 27.264 27.264 27.264 28.199 29.135 a55=30.8219 28.199 28.199 28.199 29.135 30.07 28.199 28.199 28.199 29.135 30.07 28.199 28.199 28.199 29.135 30.07 a56=31.7808 29.135 29.135 29.135 30.07 31.006 29.135 29.135 29.135 30.07 31.006 29.135 29.135 29.135 30.07 31.006 a57=32.7397 30.07 30.07 30.07 31.006 31.941 30.07 30.07 30.07 31.006 31.941 30.07 30.07 30.07 31.006 31.941 a58=33.6986 31.006 31.006 31.006 31.941 32.877 31.006 31.006 31.006 31.941 32.877 31.006 31.006 31.006 31.941 32.877 a59=34.6575 31.941 31.941 31.941 32.877 33.812 31.941 31.941 31.941 32.877 33.812 31.941 31.941 31.941 32.877 33.812 a60=35.6164 32.877 32.877 32.877 33.812 34.748 32.877 32.877 32.877 33.812 34.748 32.877 32.877 32.877 33.812 34.748 a61=36.5753 33.812 33.812 33.812 34.748 35.683 33.812 33.812 33.812 34.748 35.683 33.812 33.812 33.812 34.748 35.683 a62=37.5342 34.748 34.748 34.748 35.683 36.619 34.748 34.748 34.748 35.683 36.619 34.748 34.748 34.748 35.683 36.619 a63=38.4932 35.683 35.683 35.683 36.619 37.554 35.683 35.683 35.683 36.619 37.554 35.683 35.683 35.683 36.619 37.554 a64=39.4521 36.619 36.619 36.619 37.554 38.49 36.619 36.619 36.619 37.554 38.49 36.619 36.619 36.619 37.554 38.49 a65=40.411 37.554 37.554 37.554 38.49 39.425 37.554 37.554 37.554 38.49 39.425 37.554 37.554 37.554 38.49 39.425 a66=41.3699 38.49 38.49 38.49 39.425 40.361 38.49 38.49 38.49 39.425 40.361 38.49 38.49 38.49 39.425 40.361 a67=42.3288 38.49 39.425 39.425 40.361 41.296 38.49 39.425 39.425 40.361 41.296 38.49 39.425 39.425 40.361 41.296 a68=43.2877 39.425 40.361 40.361 41.296 42.232 39.425 40.361 40.361 41.296 42.232 39.425 40.361 40.361 41.296 42.232 a69=44.2466 40.361 41.296 41.296 42.232 43.167 40.361 41.296 41.296 42.232 43.167 40.361 41.296 41.296 42.232 43.167 a70=45.2055 41.296 42.232 42.232 43.167 44.103 41.296 42.232 42.232 43.167 44.103 41.296 42.232 42.232 43.167 44.103 a71=46.1644 42.232 43.167 43.167 44.103 45.038 42.232 43.167 43.167 44.103 45.038 42.232 43.167 43.167 44.103 45.038 a72=47.1233 43.167 44.103 44.103 45.038 45.974 43.167 44.103 44.103 45.038 45.974 43.167 44.103 44.103 45.038 45.974 a73=48.0822 44.103 45.038 45.038 45.038 46.909 44.103 45.038 45.038 45.038 46.909 44.103 45.038 45.038 45.038 46.909 a74=49.0411 45.038 45.038 45.974 45.974 47.845 45.038 45.038 45.974 45.974 47.845 45.038 45.038 45.974 45.974 47.845 a75=50 45.974 45.974 46.909 46.909 48.78 45.974 45.974 46.909 46.909 48.78 45.974 45.974 46.909 46.909 48.78
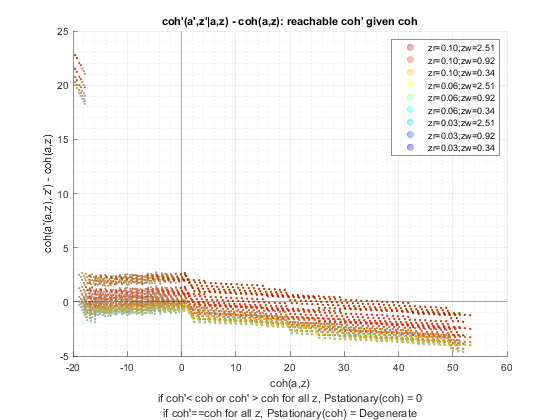
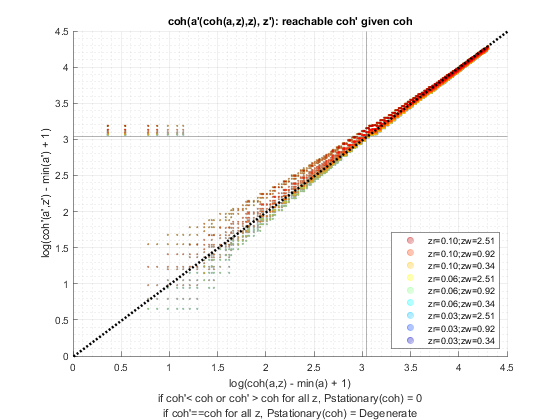
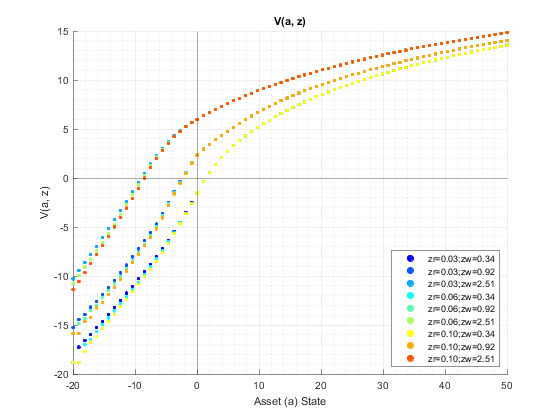
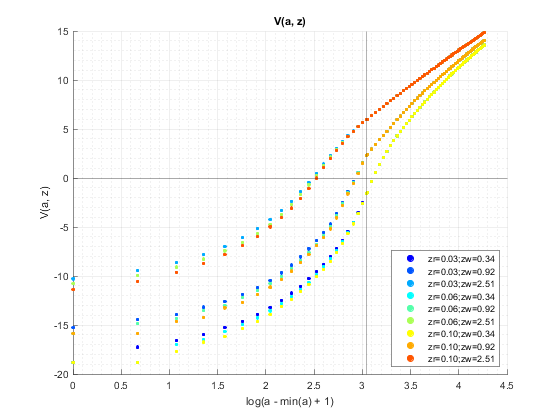
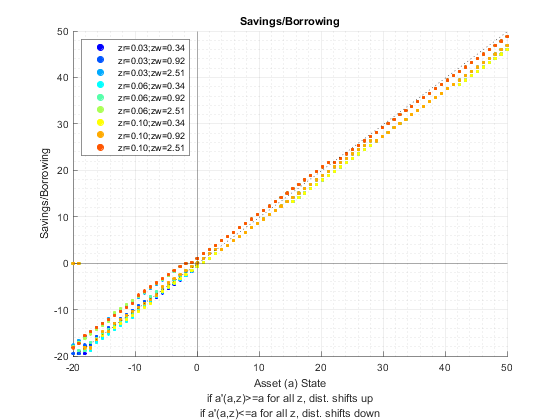
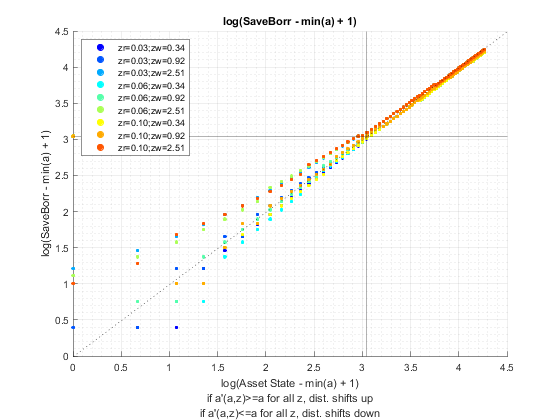
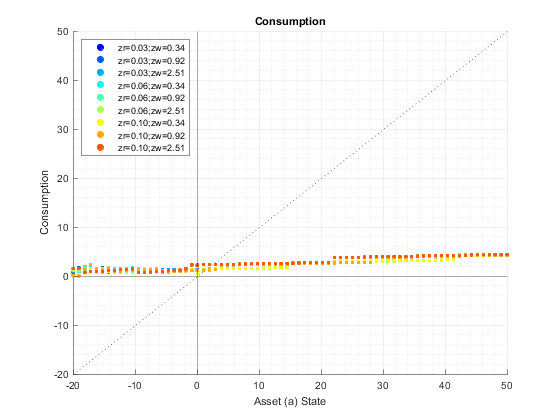
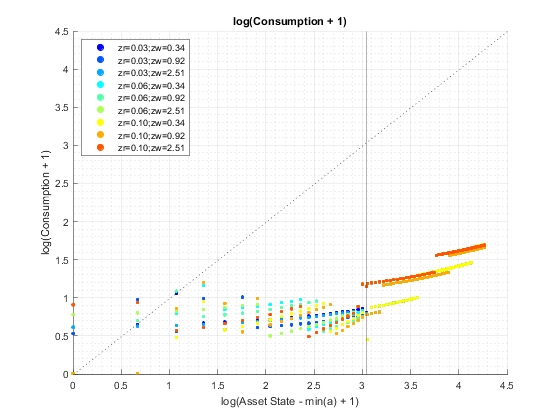
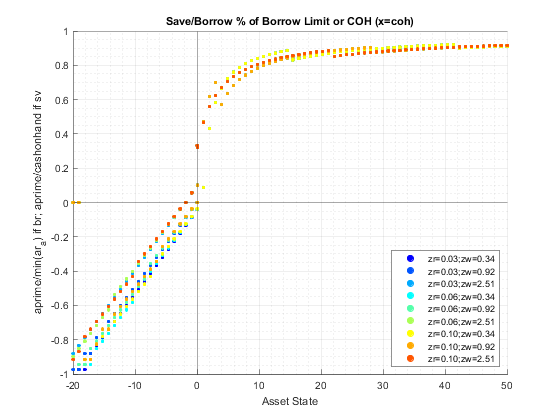
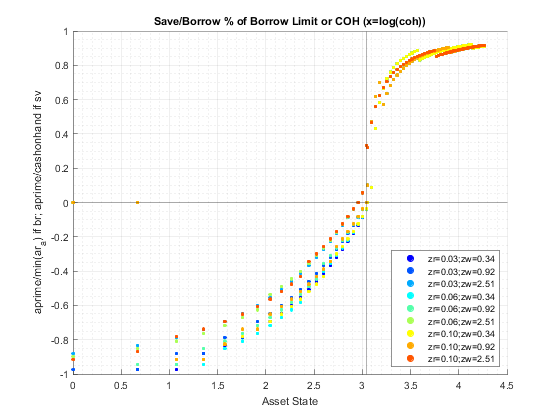
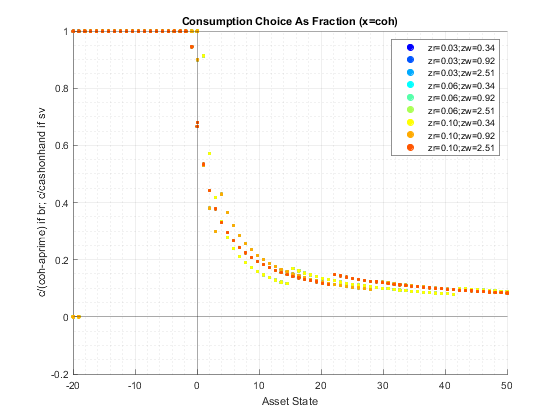
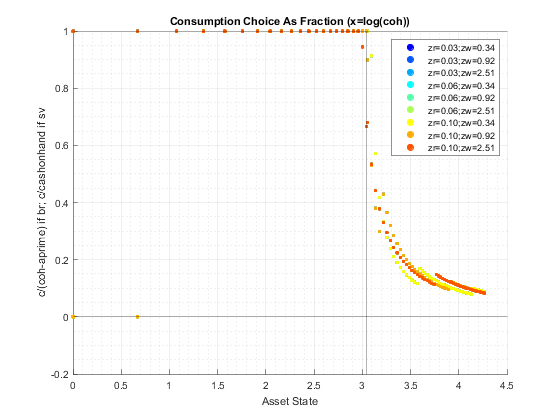
end
ans = Map with properties: Count: 12 KeyType: char ValueType: any