Solve Save + Borr Dynamic Programming Problem (Vectorized)
back to Fan's Dynamic Assets Repository Table of Content.
Contents
- FF_ABZ_VF_VEC solve infinite horizon exo shock + endo asset problem
- Default
- Parse Parameters 1
- Parse Parameters 2
- Display Parameters
- Initialize Output Matrixes
- Initialize Convergence Conditions
- Iterate Value Function
- Solve Optimization Problem Current Iteration
- Check Tolerance and Continuation
- Process Optimal Choices
function result_map = ff_abz_vf_vec(varargin)
FF_ABZ_VF_VEC solve infinite horizon exo shock + endo asset problem
This program solves the infinite horizon dynamic single asset and single shock problem with vectorized codes. ff_abz_vf shows looped codes. The solution is the same.
The borrowing problem is similar to the savings problem. The main addition here in comparison to the savings only code ff_az_vf_vec is the ability to deal with default, as well as an additional shock to the borrowing interest rate.
The vectorization takes advantage of implicit parallization that modern computers have when same instructions are given for different blocks of data With vectorization, we face a tradeoff between memory and speed. Suppose we have many shock points and many states points, if we build all states and choices into one single matrix and compute consumption, utility, etc over that entire matrix, that might be more efficient than computing consumption, utility, etc by subset of that matrix over a loop, but there is time required for generating that large input matrix, and if there are too many states, a computer could run out of memory.
The design philosophy here is that we vectorize the endogenous states and choices into matrixes, but do not include the exogeous states (shocks). The exogenous shocks remain looped. This means we can potentially have multiple shock variables discretized over a large number of shock states, and the computer would not run into memory problems. The speed gain from vectoring the rest of the problem conditional on shocks is very large compared to the pure looped version of the problem. Even if more memory is available, including the exogenous states in the vectorization process might not be speed improving.
Note one key issue is whether a programming language is row or column major depending on which, states should be rows or columns.
Another programming issue is the idea of broadcasting vs matrix algebra, both are used here. Since Matlab R2016b, matrix broadcasting has been allowed, which means the sum of a N by 1 and 1 by M is N by M. This is unrelated to matrix algebra. Matrix array broadcasting is very useful because it reduces the dimensionality of our model input state and choice and shock vectors, offering greater code clarity.
@param param_map container parameter container
@param support_map container support container
@param armt_map container container with states, choices and shocks grids that are inputs for grid based solution algorithm
@param func_map container container with function handles for consumption cash-on-hand etc.
@return result_map container contains policy function matrix, value function matrix, iteration results, and policy function, value function and iteration results tables.
keys included in result_map:
- mt_val matrix states_n by shock_n matrix of converged value function grid
- mt_pol_a matrix states_n by shock_n matrix of converged policy function grid
- ar_val_diff_norm array if bl_post = true it_iter_last by 1 val function difference between iteration
- ar_pol_diff_norm array if bl_post = true it_iter_last by 1 policy function difference between iterations
- mt_pol_perc_change matrix if bl_post = true it_iter_last by shock_n the proportion of grid points at which policy function changed between current and last iteration for each element of shock
@example
% Get Default Parameters it_param_set = 2; [param_map, support_map] = ffs_abz_set_default_param(it_param_set); % Chnage param_map keys for borrowing param_map('fl_b_bd') = -20; % borrow bound param_map('bl_default') = false; % true if allow for default param_map('fl_c_min') = 0.0001; % u(c_min) when default % Change Keys in param_map param_map('it_a_n') = 500; param_map('fl_z_r_borr_n') = 5; param_map('it_z_wage_n') = 15; param_map('it_z_n') = param_map('it_z_wage_n') * param_map('fl_z_r_borr_n'); param_map('fl_a_max') = 100; param_map('fl_w') = 1.3; % Change Keys support_map support_map('bl_display') = false; support_map('bl_post') = true; support_map('bl_display_final') = false; % Call Program with external parameters that override defaults. ff_abz_vf_vec(param_map, support_map);
@include
@seealso
- save loop: ff_az_vf
- save vectorized: ff_az_vf_vec
- save optimized-vectorized: ff_az_vf_vecsv
- save + borr loop: ff_abz_vf
- save + borr vectorized: ff_abz_vf_vec
- save + borr optimized-vectorized: ff_abz_vf_vecsv
Default
- it_param_set = 1: quick test
- it_param_set = 2: benchmark run
- it_param_set = 3: benchmark profile
- it_param_set = 4: press publish button
it_param_set = 4; bl_input_override = true; [param_map, support_map] = ffs_abz_set_default_param(it_param_set); % Note: param_map and support_map can be adjusted here or outside to override defaults % param_map('it_a_n') = 750; % param_map('fl_z_r_borr_n') = 5; % param_map('it_z_wage_n') = 15; % param_map('it_z_n') = param_map('it_z_wage_n') * param_map('fl_z_r_borr_n'); % param_map('fl_r_save') = 0.025; % param_map('fl_z_r_borr_poiss_mean') = 1.75; [armt_map, func_map] = ffs_abz_get_funcgrid(param_map, support_map, bl_input_override); % 1 for override default_params = {param_map support_map armt_map func_map};
Parse Parameters 1
% if varargin only has param_map and support_map, params_len = length(varargin); [default_params{1:params_len}] = varargin{:}; param_map = [param_map; default_params{1}]; support_map = [support_map; default_params{2}]; if params_len >= 1 && params_len <= 2 % If override param_map, re-generate armt and func if they are not % provided bl_input_override = true; [armt_map, func_map] = ffs_abz_get_funcgrid(param_map, support_map, bl_input_override); else % Override all armt_map = [armt_map; default_params{3}]; func_map = [func_map; default_params{4}]; end % append function name st_func_name = 'ff_abz_vf_vec'; support_map('st_profile_name_main') = [st_func_name support_map('st_profile_name_main')]; support_map('st_mat_name_main') = [st_func_name support_map('st_mat_name_main')]; support_map('st_img_name_main') = [st_func_name support_map('st_img_name_main')];
Parse Parameters 2
% armt_map params_group = values(armt_map, {'ar_a', 'mt_z_trans', 'ar_z_r_borr_mesh_wage', 'ar_z_wage_mesh_r_borr'}); [ar_a, mt_z_trans, ar_z_r_borr_mesh_wage, ar_z_wage_mesh_r_borr] = params_group{:}; % func_map params_group = values(func_map, {'f_util_log', 'f_util_crra', 'f_cons_checkcmin', 'f_awithr_to_anor', 'f_coh', 'f_cons_coh'}); [f_util_log, f_util_crra, f_cons_checkcmin, f_awithr_to_anor, f_coh, f_cons_coh] = params_group{:}; % param_map params_group = values(param_map, {'it_a_n', 'it_z_n', 'fl_crra', 'fl_beta', 'fl_c_min',... 'fl_nan_replace', 'bl_default', 'fl_default_aprime'}); [it_a_n, it_z_n, fl_crra, fl_beta, fl_c_min, ... fl_nan_replace, bl_default, fl_default_aprime] = params_group{:}; params_group = values(param_map, {'it_maxiter_val', 'fl_tol_val', 'fl_tol_pol', 'it_tol_pol_nochange'}); [it_maxiter_val, fl_tol_val, fl_tol_pol, it_tol_pol_nochange] = params_group{:}; % support_map params_group = values(support_map, {'bl_profile', 'st_profile_path', ... 'st_profile_prefix', 'st_profile_name_main', 'st_profile_suffix',... 'bl_time', 'bl_display_defparam', 'bl_display', 'it_display_every', 'bl_post'}); [bl_profile, st_profile_path, ... st_profile_prefix, st_profile_name_main, st_profile_suffix, ... bl_time, bl_display_defparam, bl_display, it_display_every, bl_post] = params_group{:};
Display Parameters
if(bl_display_defparam) fft_container_map_display(param_map); fft_container_map_display(support_map); end
---------------------------------------- ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Begin: Show all key and value pairs from container CONTAINER NAME: PARAM_MAP ---------------------------------------- Map with properties: Count: 35 KeyType: char ValueType: any xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ---------------------------------------- ---------------------------------------- pos = 32 ; key = st_analytical_stationary_type ; val = eigenvector pos = 33 ; key = st_model ; val = abz pos = 34 ; key = st_z_r_borr_drv_ele_type ; val = unif pos = 35 ; key = st_z_r_borr_drv_prb_type ; val = poiss ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Scalars in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx value __ ___ ______ bl_b_is_principle 1 1 1 bl_default 2 2 1 bl_loglin 3 3 0 fl_a_max 4 4 50 fl_a_min 5 5 0 fl_b_bd 6 6 -20 fl_beta 7 7 0.94 fl_c_min 8 8 0.01 fl_crra 9 9 1.5 fl_default_aprime 10 10 0 fl_loglin_threshold 11 11 1 fl_nan_replace 12 12 -99999 fl_r_save 13 13 0.025 fl_tol_dist 14 14 1e-05 fl_tol_pol 15 15 1e-05 fl_tol_val 16 16 1e-05 fl_w 17 17 1.28 fl_z_r_borr_max 18 18 0.095 fl_z_r_borr_min 19 19 0.025 fl_z_r_borr_n 20 20 5 fl_z_r_borr_poiss_mean 21 21 10 fl_z_wage_mu 22 22 0 fl_z_wage_rho 23 23 0.8 fl_z_wage_sig 24 24 0.2 it_a_n 25 25 750 it_maxiter_dist 26 26 1000 it_maxiter_val 27 27 1000 it_tol_pol_nochange 28 28 25 it_trans_power_dist 29 29 1000 it_z_n 30 30 55 it_z_wage_n 31 31 11 ---------------------------------------- ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Begin: Show all key and value pairs from container CONTAINER NAME: SUPPORT_MAP ---------------------------------------- Map with properties: Count: 40 KeyType: char ValueType: any xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx ---------------------------------------- ---------------------------------------- pos = 26 ; key = st_img_name_main ; val = ff_abz_vf_vec_default pos = 27 ; key = st_img_path ; val = C:/Users/fan/CodeDynaAsset//m_abz//solve/img/ pos = 28 ; key = st_img_prefix ; val = pos = 29 ; key = st_img_suffix ; val = _p4.png pos = 30 ; key = st_mat_name_main ; val = ff_abz_vf_vec_default pos = 31 ; key = st_mat_path ; val = C:/Users/fan/CodeDynaAsset//m_abz//solve/mat/ pos = 32 ; key = st_mat_prefix ; val = pos = 33 ; key = st_mat_suffix ; val = _p4 pos = 34 ; key = st_mat_test_path ; val = C:/Users/fan/CodeDynaAsset//m_abz//test/ff_az_ds_vecsv/mat/ pos = 35 ; key = st_matimg_path_root ; val = C:/Users/fan/CodeDynaAsset//m_abz/ pos = 36 ; key = st_profile_name_main ; val = ff_abz_vf_vec_default pos = 37 ; key = st_profile_path ; val = C:/Users/fan/CodeDynaAsset//m_abz//solve/profile/ pos = 38 ; key = st_profile_prefix ; val = pos = 39 ; key = st_profile_suffix ; val = _p4 pos = 40 ; key = st_title_prefix ; val = ---------------------------------------- xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx Scalars in Container and Sizes and Basic Statistics xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx i idx value __ ___ _____ bl_display 1 1 0 bl_display_defparam 2 2 1 bl_display_dist 3 3 0 bl_display_final 4 4 1 bl_display_final_dist 5 5 0 bl_display_final_dist_detail 6 6 0 bl_display_funcgrids 7 7 0 bl_graph 8 8 1 bl_graph_coh_t_coh 9 9 1 bl_graph_funcgrids 10 10 0 bl_graph_onebyones 11 11 1 bl_graph_pol_lvl 12 12 1 bl_graph_pol_pct 13 13 1 bl_graph_val 14 14 1 bl_img_save 15 15 0 bl_mat 16 16 0 bl_post 17 17 1 bl_profile 18 18 0 bl_profile_dist 19 19 0 bl_time 20 20 1 it_display_every 21 21 5 it_display_final_colmax 22 22 15 it_display_final_rowmax 23 23 100 it_display_summmat_colmax 24 24 5 it_display_summmat_rowmax 25 25 5
Initialize Output Matrixes
include mt_pol_idx which we did not have in looped code
mt_val_cur = zeros(length(ar_a),it_z_n); mt_val = mt_val_cur - 1; mt_pol_a = zeros(length(ar_a),it_z_n); mt_pol_a_cur = mt_pol_a - 1; mt_pol_idx = zeros(length(ar_a),it_z_n);
Initialize Convergence Conditions
bl_vfi_continue = true; it_iter = 0; ar_val_diff_norm = zeros([it_maxiter_val, 1]); ar_pol_diff_norm = zeros([it_maxiter_val, 1]); mt_pol_perc_change = zeros([it_maxiter_val, it_z_n]);
Iterate Value Function
Loop solution with 4 nested loops
- loop 1: over exogenous states
- loop 2: over endogenous states
- loop 3: over choices
- loop 4: add future utility, integration--loop over future shocks
% Start Profile if (bl_profile) close all; profile off; profile on; end % Start Timer if (bl_time) tic; end % Value Function Iteration while bl_vfi_continue
it_iter = it_iter + 1;
Solve Optimization Problem Current Iteration
Only this segment of code differs between ff_abz_vf and ff_abz_vf_vec
% loop 1: over exogenous states % incorporating these shocks into vectorization has high memory burden % but insignificant speed gains. Keeping this loop allows for large % number of shocks without overwhelming memory for it_z_i = 1:it_z_n % Current Shock fl_z_r_borr = ar_z_r_borr_mesh_wage(it_z_i); fl_z_wage = ar_z_wage_mesh_r_borr(it_z_i); % cash-on-hand ar_coh = f_coh(fl_z_wage, ar_a); % Consumption: fl_z = 1 by 1, ar_a = 1 by N, ar_a' = N by 1 % mt_c is N by N: matrix broadcasting, expand to matrix from arrays mt_c = f_cons_coh(ar_coh, fl_z_r_borr, ar_a'); % EVAL current utility: N by N, f_util defined earlier if (fl_crra == 1) mt_utility = f_util_log(mt_c); fl_u_cmin = f_util_log(fl_c_min); else mt_utility = f_util_crra(mt_c); fl_u_cmin = f_util_crra(fl_c_min); end % f(z'|z) ar_z_trans_condi = mt_z_trans(it_z_i,:); % EVAL EV((A',K'),Z'|Z) = V((A',K'),Z') x p(z'|z)', (N by Z) x (Z by 1) = N by 1 % Note: transpose ar_z_trans_condi from 1 by Z to Z by 1 % Note: matrix multiply not dot multiply mt_evzp_condi_z = mt_val_cur * ar_z_trans_condi'; % EVAL add on future utility, N by N + N by 1, broadcast again mt_utility = mt_utility + fl_beta*mt_evzp_condi_z; if (bl_default) % if default: only today u(cmin), transition out next period, debt wiped out mt_utility(mt_c <= fl_c_min) = fl_u_cmin + fl_beta*mt_evzp_condi_z(ar_a == fl_default_aprime); else % if default is not allowed: v = u(cmin) mt_utility(mt_c <= fl_c_min) = fl_nan_replace; end % Optimization: remember matlab is column major, rows must be % choices, columns must be states % <https://en.wikipedia.org/wiki/Row-_and_column-major_order COLUMN-MAJOR> % mt_utility is N by N, rows are choices, cols are states. [ar_opti_val_z, ar_opti_idx_z] = max(mt_utility); ar_opti_aprime_z = ar_a(ar_opti_idx_z); ar_opti_c_z = f_cons_coh(ar_coh, fl_z_r_borr, ar_opti_aprime_z); % Handle Default is optimal or not if (bl_default) % if defaulting is optimal choice, at these states, not required % to default, non-default possible, but default could be optimal ar_opti_aprime_z(ar_opti_c_z <= fl_c_min) = fl_default_aprime; ar_opti_idx_z(ar_opti_c_z <= fl_c_min) = find(ar_a == fl_default_aprime); else % if default is not allowed, then next period same state as now % this is absorbing state, this is the limiting case, single % state space point, lowest a and lowest shock has this. ar_opti_aprime_z(ar_opti_c_z <= fl_c_min) = ar_a(ar_opti_c_z <= fl_c_min); end % store optimal values mt_val(:,it_z_i) = ar_opti_val_z; mt_pol_a(:,it_z_i) = ar_opti_aprime_z; if (it_iter == (it_maxiter_val + 1)) mt_pol_idx(:,it_z_i) = ar_opti_idx_z; end end
Check Tolerance and Continuation
% Difference across iterations ar_val_diff_norm(it_iter) = norm(mt_val - mt_val_cur); ar_pol_diff_norm(it_iter) = norm(mt_pol_a - mt_pol_a_cur); mt_pol_perc_change(it_iter, :) = sum((mt_pol_a ~= mt_pol_a_cur))/(it_a_n); % Update mt_val_cur = mt_val; mt_pol_a_cur = mt_pol_a; % Print Iteration Results if (bl_display && (rem(it_iter, it_display_every)==0)) fprintf('VAL it_iter:%d, fl_diff:%d, fl_diff_pol:%d\n', ... it_iter, ar_val_diff_norm(it_iter), ar_pol_diff_norm(it_iter)); tb_valpol_iter = array2table([mean(mt_val_cur,1); mean(mt_pol_a_cur,1); ... mt_val_cur(it_a_n,:); mt_pol_a_cur(it_a_n,:)]); tb_valpol_iter.Properties.VariableNames = strcat('z', string((1:size(mt_val_cur,2)))); tb_valpol_iter.Properties.RowNames = {'mval', 'map', 'Hval', 'Hap'}; disp('mval = mean(mt_val_cur,1), average value over a') disp('map = mean(mt_pol_a_cur,1), average choice over a') disp('Hval = mt_val_cur(it_a_n,:), highest a state val') disp('Hap = mt_pol_a_cur(it_a_n,:), highest a state choice') disp(tb_valpol_iter); end % Continuation Conditions: % 1. if value function convergence criteria reached % 2. if policy function variation over iterations is less than % threshold if (it_iter == (it_maxiter_val + 1)) bl_vfi_continue = false; elseif ((it_iter == it_maxiter_val) || ... (ar_val_diff_norm(it_iter) < fl_tol_val) || ... (sum(ar_pol_diff_norm(max(1, it_iter-it_tol_pol_nochange):it_iter)) < fl_tol_pol)) % Fix to max, run again to save results if needed it_iter_last = it_iter; it_iter = it_maxiter_val; end
end % End Timer if (bl_time) toc; end % End Profile if (bl_profile) profile off profile viewer st_file_name = [st_profile_prefix st_profile_name_main st_profile_suffix]; profsave(profile('info'), strcat(st_profile_path, st_file_name)); end
Elapsed time is 345.177068 seconds.
Process Optimal Choices
result_map = containers.Map('KeyType','char', 'ValueType','any'); result_map('mt_val') = mt_val; result_map('mt_pol_idx') = mt_pol_idx; result_map('cl_mt_val') = {mt_val, zeros(1)}; result_map('cl_mt_coh') = {f_coh(ar_z_wage_mesh_r_borr, ar_a'), zeros(1)}; result_map('cl_mt_pol_a') = {f_awithr_to_anor(ar_z_r_borr_mesh_wage, mt_pol_a), zeros(1)}; result_map('cl_mt_pol_c') = {f_cons_checkcmin(ar_z_r_borr_mesh_wage, ar_z_wage_mesh_r_borr, ar_a', mt_pol_a), zeros(1)}; result_map('ar_st_pol_names') = ["cl_mt_val", "cl_mt_pol_a", "cl_mt_coh", "cl_mt_pol_c"]; if (bl_post) bl_input_override = true; result_map('ar_val_diff_norm') = ar_val_diff_norm(1:it_iter_last); result_map('ar_pol_diff_norm') = ar_pol_diff_norm(1:it_iter_last); result_map('mt_pol_perc_change') = mt_pol_perc_change(1:it_iter_last, :); result_map = ff_az_vf_post(param_map, support_map, armt_map, func_map, result_map, bl_input_override); end
valgap = norm(mt_val - mt_val_cur): value function difference across iterations polgap = norm(mt_pol_a - mt_pol_a_cur): policy function difference across iterations z1 = z1 perc change: sum((mt_pol_a ~= mt_pol_a_cur))/(it_a_n): percentage of state space points conditional on shock where the policy function is changing across iterations valgap polgap zi1_zr_0_025_zw_0_34664 zi2_zr_0_025_zw_0_42338 zi3_zr_0_025_zw_0_51712 zi4_zr_0_025_zw_0_63162 zi5_zr_0_025_zw_0_77146 zi6_zr_0_025_zw_0_94226 zi7_zr_0_025_zw_1_1509 zi8_zr_0_025_zw_1_4057 zi48_zr_0_095_zw_0_63162 zi49_zr_0_095_zw_0_77146 zi50_zr_0_095_zw_0_94226 zi51_zr_0_095_zw_1_1509 zi52_zr_0_095_zw_1_4057 zi53_zr_0_095_zw_1_7169 zi54_zr_0_095_zw_2_097 zi55_zr_0_095_zw_2_5613 ________ _______ _______________________ _______________________ _______________________ _______________________ _______________________ _______________________ ______________________ ______________________ ________________________ ________________________ ________________________ _______________________ _______________________ _______________________ ______________________ _______________________ iter=1 352.78 3846.1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 iter=2 302.85 4213.3 0.99867 1 1 1 1 1 1 1 0.98533 0.988 0.99067 0.99333 0.99733 1 1 1 iter=3 247.45 1370.3 0.984 0.98667 0.99067 0.99467 0.99733 0.99867 0.99867 1 0.976 0.98 0.98667 0.99467 0.996 1 1 1 iter=4 205.06 685.13 0.97067 0.97467 0.98 0.98133 0.98667 0.99333 0.99467 0.99467 0.968 0.97067 0.97733 0.988 0.996 0.98933 0.99867 1 iter=5 173.89 403.37 0.95467 0.956 0.96533 0.96933 0.97733 0.98667 0.99467 0.996 0.95333 0.96267 0.96933 0.98267 0.99467 0.98667 0.996 1 iter=6 149.47 269.7 0.94133 0.94133 0.94933 0.96 0.97067 0.984 0.984 0.988 0.94 0.95067 0.96533 0.96933 0.98667 0.98 0.99467 0.99733 iter=7 129.24 193.98 0.92667 0.93733 0.94533 0.95733 0.95333 0.96533 0.97867 0.98533 0.93867 0.93867 0.95067 0.95733 0.97467 0.98533 0.98667 0.98667 iter=8 112.61 144.55 0.91467 0.92267 0.93333 0.94267 0.956 0.95867 0.976 0.98667 0.92667 0.94267 0.94533 0.95867 0.96133 0.984 0.976 0.972 iter=9 98.571 110.61 0.90933 0.91867 0.92267 0.93067 0.952 0.95467 0.964 0.98 0.92267 0.936 0.95067 0.95467 0.968 0.97333 0.968 0.98267 iter=10 86.561 88.402 0.90133 0.91333 0.92267 0.92667 0.94267 0.95467 0.95733 0.96267 0.912 0.91333 0.93333 0.94 0.95467 0.95733 0.976 0.96667 iter=11 76.23 71.99 0.88667 0.89467 0.908 0.92133 0.92133 0.936 0.94667 0.95733 0.89867 0.92 0.91467 0.94267 0.94 0.97067 0.972 0.956 iter=12 67.259 59.599 0.884 0.88267 0.88933 0.91867 0.90933 0.92267 0.94133 0.95333 0.89733 0.904 0.92 0.92267 0.94267 0.94667 0.94533 0.964 iter=13 59.469 49.538 0.86533 0.86667 0.87867 0.88267 0.896 0.90267 0.92533 0.93333 0.872 0.88533 0.90267 0.90933 0.924 0.92933 0.952 0.94533 iter=14 52.672 42.341 0.836 0.85333 0.86533 0.864 0.88133 0.89733 0.912 0.92 0.856 0.88 0.87067 0.89733 0.89733 0.92533 0.92533 0.924 iter=15 46.718 36.325 0.82 0.82667 0.83467 0.85067 0.85333 0.86933 0.86667 0.89867 0.844 0.84 0.86 0.87067 0.88533 0.89333 0.89067 0.92267 iter=16 41.497 31.568 0.788 0.81067 0.80667 0.812 0.83067 0.832 0.84267 0.84133 0.808 0.82133 0.832 0.83733 0.852 0.848 0.88133 0.88533 iter=17 36.931 27.298 0.76133 0.76267 0.784 0.78 0.80267 0.79867 0.8 0.81067 0.76267 0.78133 0.78133 0.80267 0.79867 0.83067 0.84 0.84133 iter=18 32.935 23.985 0.73733 0.74933 0.75467 0.74533 0.756 0.77067 0.77067 0.77333 0.76133 0.752 0.77467 0.78 0.77333 0.788 0.796 0.82533 iter=19 29.427 22.92 0.696 0.696 0.704 0.71467 0.70667 0.72267 0.724 0.74133 0.7 0.72133 0.71467 0.72 0.74667 0.74267 0.76133 0.78667 iter=20 26.354 18.398 0.648 0.656 0.66267 0.66533 0.66667 0.67333 0.69733 0.696 0.66933 0.64667 0.67867 0.67733 0.7 0.70667 0.74267 0.748 iter=21 23.673 20.326 0.62133 0.612 0.61333 0.628 0.62533 0.64133 0.64667 0.66667 0.59467 0.652 0.61067 0.65333 0.64 0.692 0.696 0.676 iter=22 21.331 14.676 0.57733 0.564 0.568 0.59467 0.58133 0.59733 0.61067 0.61733 0.61867 0.588 0.64133 0.624 0.632 0.63333 0.63067 0.70667 iter=23 19.278 13.103 0.52 0.51467 0.51867 0.52933 0.544 0.544 0.56267 0.57467 0.528 0.52133 0.536 0.54267 0.588 0.56267 0.616 0.636 iter=24 17.484 20.103 0.46267 0.468 0.48267 0.48667 0.49067 0.5 0.516 0.528 0.46533 0.46933 0.484 0.492 0.50933 0.55333 0.58133 0.56533 iter=25 15.919 10.708 0.41067 0.42267 0.43467 0.44133 0.45067 0.45333 0.46667 0.48533 0.42933 0.496 0.44267 0.496 0.46933 0.51733 0.49867 0.524 iter=26 14.549 9.6377 0.372 0.37067 0.38533 0.384 0.396 0.4 0.42533 0.43067 0.41867 0.38133 0.43867 0.42533 0.43733 0.44267 0.46133 0.52267 iter=27 13.349 8.7583 0.32933 0.34667 0.34133 0.34933 0.35733 0.364 0.37467 0.392 0.34933 0.35333 0.36267 0.36267 0.40933 0.39067 0.40533 0.444 iter=28 12.293 20.055 0.30667 0.30267 0.30933 0.30933 0.32533 0.336 0.34267 0.35333 0.304 0.31733 0.324 0.332 0.34667 0.35333 0.404 0.39867 iter=29 11.365 20.119 0.264 0.27467 0.28 0.28533 0.284 0.29733 0.30667 0.316 0.27733 0.27867 0.29067 0.296 0.31467 0.33067 0.348 0.35467 iter=30 10.546 20.046 0.244 0.25067 0.25467 0.25733 0.264 0.268 0.27867 0.28533 0.26133 0.28 0.26267 0.26933 0.27467 0.308 0.31467 0.31867 iter=31 9.8215 20.038 0.22 0.21733 0.22667 0.22533 0.236 0.24267 0.25067 0.26133 0.22133 0.24267 0.23733 0.26667 0.25733 0.27733 0.27733 0.28933 iter=32 9.177 6.0135 0.19867 0.20667 0.20933 0.20667 0.216 0.22 0.22933 0.22933 0.204 0.21733 0.21733 0.23333 0.22133 0.24667 0.25467 0.264 iter=33 8.601 5.4548 0.17733 0.17733 0.18 0.188 0.192 0.196 0.20267 0.22 0.19467 0.19067 0.21467 0.204 0.21733 0.22 0.23067 0.252 iter=34 8.0824 5.1358 0.16267 0.17067 0.168 0.17333 0.18 0.18133 0.19067 0.19333 0.17867 0.17733 0.184 0.188 0.196 0.204 0.21067 0.24267 iter=35 7.612 4.6977 0.144 0.14533 0.15467 0.152 0.152 0.16267 0.17067 0.176 0.15467 0.14933 0.16533 0.16933 0.18533 0.17467 0.19333 0.20267 iter=36 7.1826 4.3323 0.13867 0.14 0.13333 0.144 0.14933 0.14667 0.15467 0.16 0.144 0.148 0.14533 0.152 0.16267 0.168 0.172 0.18933 iter=37 6.7888 4.0273 0.112 0.12933 0.128 0.12267 0.13467 0.14133 0.14267 0.15067 0.12667 0.13467 0.14267 0.14 0.152 0.15333 0.15867 0.16933 iter=38 6.4262 3.6336 0.108 0.10933 0.112 0.11867 0.116 0.12133 0.128 0.13333 0.11333 0.11467 0.12267 0.12667 0.13467 0.13733 0.144 0.15867 iter=39 6.091 3.3142 0.097333 0.10267 0.1 0.1 0.1 0.112 0.112 0.116 0.10133 0.1 0.10933 0.112 0.11733 0.12667 0.136 0.136 iter=40 5.7799 3.1979 0.092 0.092 0.096 0.10133 0.11067 0.10267 0.11333 0.112 0.10267 0.10933 0.10133 0.11333 0.108 0.11867 0.11733 0.13467 iter=41 5.4897 3.0676 0.076 0.085333 0.088 0.085333 0.088 0.092 0.094667 0.10533 0.088 0.086667 0.092 0.094667 0.10667 0.1 0.11467 0.12133 iter=42 5.2179 2.8667 0.074667 0.070667 0.076 0.081333 0.077333 0.084 0.084 0.086667 0.08 0.078667 0.084 0.082667 0.088 0.094667 0.098667 0.104 iter=43 4.9625 2.6699 0.066667 0.066667 0.065333 0.069333 0.077333 0.070667 0.08 0.084 0.070667 0.073333 0.069333 0.077333 0.085333 0.090667 0.098667 0.1 iter=44 4.7217 2.5725 0.062667 0.065333 0.062667 0.06 0.068 0.072 0.076 0.08 0.06 0.068 0.072 0.076 0.077333 0.08 0.085333 0.093333 iter=45 4.494 2.4152 0.056 0.057333 0.056 0.065333 0.065333 0.068 0.070667 0.068 0.064 0.065333 0.066667 0.069333 0.066667 0.073333 0.070667 0.08 iter=46 4.2781 2.2213 0.053333 0.049333 0.054667 0.052 0.049333 0.057333 0.057333 0.068 0.050667 0.050667 0.057333 0.057333 0.068 0.065333 0.073333 0.08 iter=47 4.073 1.9928 0.04 0.048 0.049333 0.053333 0.048 0.052 0.052 0.062667 0.052 0.048 0.052 0.053333 0.061333 0.06 0.066667 0.066667 iter=48 3.8779 1.7916 0.04 0.04 0.048 0.042667 0.048 0.048 0.048 0.046667 0.042667 0.049333 0.048 0.048 0.048 0.054667 0.058667 0.06 iter=49 3.692 1.6427 0.036 0.033333 0.036 0.04 0.041333 0.042667 0.049333 0.045333 0.04 0.041333 0.042667 0.049333 0.045333 0.049333 0.056 0.056 iter=50 3.5146 1.5439 0.034667 0.036 0.034667 0.038667 0.036 0.038667 0.038667 0.041333 0.038667 0.036 0.037333 0.038667 0.042667 0.049333 0.045333 0.056 iter=79 0.73574 0.36196 0.0013333 0 0 0.0013333 0 0.0026667 0 0.0026667 0.0013333 0 0.0026667 0 0.0026667 0.0026667 0.004 0 iter=80 0.69375 0.29554 0.0013333 0.0013333 0 0.0013333 0 0 0.0013333 0.0026667 0.0013333 0 0 0.0013333 0.0026667 0.0013333 0 0.0026667 iter=81 0.65401 0.29554 0 0 0.0013333 0 0.0026667 0 0.0013333 0.0013333 0 0.0026667 0 0.0013333 0.0013333 0.0026667 0.0026667 0.0013333 iter=82 0.6164 0.41796 0 0.0053333 0.004 0.0013333 0.0013333 0 0.0026667 0.0013333 0.0013333 0.0013333 0 0.0026667 0.0013333 0.0013333 0.0026667 0.0013333 iter=83 0.58083 0.36196 0 0 0 0.0026667 0.0013333 0.0026667 0 0.0013333 0.0026667 0.0013333 0.0026667 0 0.0013333 0 0 0.004 iter=84 0.5472 0.29554 0 0 0 0 0 0 0.0026667 0 0 0 0 0.0026667 0 0 0.0026667 0.0013333 iter=85 0.51542 0.20898 0 0.0013333 0 0 0.0013333 0.0013333 0 0 0 0.0013333 0.0013333 0 0 0.0013333 0.0013333 0.0013333 iter=86 0.48541 0.20898 0 0 0.0013333 0.0013333 0.0013333 0 0 0 0.0013333 0.0013333 0 0 0 0 0 0.0013333 iter=87 0.45706 0.29554 0.0013333 0 0.0013333 0 0 0 0 0 0 0 0 0 0 0 0 0.0026667 iter=88 0.43031 0.20898 0 0 0 0 0 0.0013333 0 0.0013333 0 0 0.0013333 0 0.0013333 0.0013333 0 0.0013333 iter=89 0.40506 0.29554 0 0 0 0 0.0013333 0 0 0 0 0.0013333 0 0 0 0.0026667 0 0 iter=90 0.38125 0.20898 0 0 0 0 0 0.0013333 0 0.0013333 0 0 0.0013333 0 0.0013333 0.0013333 0.0013333 0 iter=91 0.35879 0.20898 0 0.0013333 0 0 0 0.0013333 0 0.0013333 0 0 0.0013333 0 0.0013333 0 0 0 iter=92 0.33762 0.20898 0 0 0 0.0013333 0 0 0 0.0013333 0.0013333 0 0 0 0.0013333 0 0.0013333 0 iter=93 0.31766 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=94 0.29886 0.20898 0 0 0 0 0 0 0 0.0013333 0 0 0 0 0.0013333 0 0 0 iter=95 0.28114 0.20898 0 0 0 0 0 0 0 0 0 0 0 0 0 0.0013333 0 0 iter=96 0.26446 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=97 0.24874 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=98 0.23395 0.20898 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0.0013333 iter=99 0.22002 0.20898 0.0013333 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=100 0.20692 0.20898 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0.0013333 iter=101 0.19458 0.20898 0 0 0 0.0013333 0 0 0 0 0.0013333 0 0 0 0 0 0 0 iter=102 0.18297 0.20898 0 0 0 0 0 0 0.0013333 0 0 0 0 0.0013333 0 0 0 0 iter=103 0.17205 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=104 0.16178 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=105 0.15211 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=106 0.14302 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=107 0.13446 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=108 0.12642 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=109 0.11886 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=110 0.11174 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=111 0.10505 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=112 0.098761 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=113 0.092846 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=114 0.087284 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=115 0.082055 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=116 0.077138 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=117 0.072516 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=118 0.06817 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=119 0.064083 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=120 0.060242 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=121 0.056631 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=122 0.053235 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=123 0.050043 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=124 0.047043 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=125 0.044222 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=126 0.04157 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=127 0.039077 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 iter=128 0.036733 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 tb_val: V(a,z) value at each state space point zi1_zr_0_025_zw_0_34664 zi2_zr_0_025_zw_0_42338 zi3_zr_0_025_zw_0_51712 zi4_zr_0_025_zw_0_63162 zi5_zr_0_025_zw_0_77146 zi6_zr_0_025_zw_0_94226 zi7_zr_0_025_zw_1_1509 zi8_zr_0_025_zw_1_4057 zi48_zr_0_095_zw_0_63162 zi49_zr_0_095_zw_0_77146 zi50_zr_0_095_zw_0_94226 zi51_zr_0_095_zw_1_1509 zi52_zr_0_095_zw_1_4057 zi53_zr_0_095_zw_1_7169 zi54_zr_0_095_zw_2_097 zi55_zr_0_095_zw_2_5613 _______________________ _______________________ _______________________ _______________________ _______________________ _______________________ ______________________ ______________________ ________________________ ________________________ ________________________ _______________________ _______________________ _______________________ ______________________ _______________________ a1=-20 -17.865 -17.407 -16.896 -16.363 -15.598 -14.718 -13.953 -13.232 -16.363 -15.822 -15.278 -14.738 -14.203 -13.672 -12.314 -10.919 a2=-19.9065 -17.865 -17.407 -16.896 -16.283 -15.365 -14.578 -13.863 -13.172 -16.363 -15.822 -15.278 -14.738 -14.203 -13.628 -12.22 -10.827 a3=-19.8131 -17.865 -17.407 -16.896 -15.982 -15.182 -14.46 -13.785 -13.119 -16.363 -15.822 -15.278 -14.738 -14.203 -13.427 -12.137 -10.738 a4=-19.7196 -17.865 -17.407 -16.542 -15.754 -15.033 -14.359 -13.716 -13.07 -16.363 -15.822 -15.278 -14.738 -14.203 -13.266 -12.042 -10.65 a5=-19.6262 -17.794 -17.019 -16.277 -15.575 -14.908 -14.272 -13.653 -13.026 -16.363 -15.822 -15.278 -14.738 -14.203 -13.132 -11.951 -10.565 a6=-19.5327 -17.385 -16.731 -16.073 -15.428 -14.802 -14.195 -13.597 -12.985 -16.363 -15.822 -15.278 -14.738 -14.203 -13.02 -11.862 -10.477 a7=-19.4393 -17.093 -16.512 -15.908 -15.305 -14.71 -14.126 -13.546 -12.907 -16.363 -15.822 -15.278 -14.738 -14.015 -12.923 -11.763 -10.388 a8=-19.3458 -16.871 -16.338 -15.773 -15.2 -14.63 -14.065 -13.5 -12.812 -16.363 -15.822 -15.278 -14.738 -13.845 -12.838 -11.666 -10.301 a9=-19.2523 -16.695 -16.195 -15.658 -15.11 -14.559 -14.01 -13.457 -12.715 -16.363 -15.822 -15.278 -14.738 -13.705 -12.763 -11.576 -10.214 a10=-19.1589 -16.551 -16.076 -15.56 -15.03 -14.495 -13.959 -13.418 -12.623 -16.363 -15.822 -15.278 -14.566 -13.587 -12.697 -11.484 -10.128 a11=-19.0654 -16.43 -15.973 -15.475 -14.96 -14.438 -13.913 -13.381 -12.536 -16.363 -15.822 -15.278 -14.377 -13.486 -12.636 -11.398 -10.043 a12=-18.972 -16.327 -15.884 -15.399 -14.897 -14.386 -13.871 -13.347 -12.456 -16.363 -15.822 -15.189 -14.223 -13.399 -12.56 -11.311 -9.958 a13=-18.8785 -16.237 -15.806 -15.332 -14.84 -14.339 -13.832 -13.266 -12.376 -16.363 -15.822 -14.956 -14.095 -13.322 -12.46 -11.221 -9.8713 a14=-18.785 -16.159 -15.737 -15.272 -14.789 -14.296 -13.796 -13.168 -12.302 -16.363 -15.694 -14.773 -13.987 -13.253 -12.37 -11.135 -9.7857 a15=-18.6916 -16.089 -15.675 -15.218 -14.742 -14.256 -13.763 -13.071 -12.231 -16.363 -15.439 -14.623 -13.893 -13.192 -12.27 -11.048 -9.7008 a16=-18.5981 -16.027 -15.619 -15.168 -14.698 -14.219 -13.731 -12.984 -12.16 -16.076 -15.241 -14.499 -13.811 -13.137 -12.176 -10.966 -9.6161 a17=-18.5047 -15.971 -15.568 -15.123 -14.659 -14.184 -13.7 -12.899 -12.094 -15.827 -15.081 -14.392 -13.739 -13.087 -12.084 -10.882 -9.5322 a18=-18.4112 -15.92 -15.522 -15.082 -14.622 -14.152 -13.589 -12.821 -12.025 -15.633 -14.949 -14.301 -13.674 -13.041 -11.992 -10.8 -9.4474 a19=-18.3178 -15.873 -15.48 -15.043 -14.588 -14.122 -13.491 -12.747 -11.954 -15.476 -14.837 -14.22 -13.616 -12.982 -11.906 -10.718 -9.3629 a20=-18.2243 -15.83 -15.44 -15.008 -14.556 -14.094 -13.396 -12.677 -11.884 -15.345 -14.741 -14.149 -13.563 -12.88 -11.823 -10.632 -9.2787 a21=-18.1308 -15.791 -15.404 -14.974 -14.526 -14.023 -13.312 -12.61 -11.815 -15.235 -14.657 -14.085 -13.515 -12.777 -11.741 -10.547 -9.195 a22=-18.0374 -15.754 -15.37 -14.943 -14.498 -13.915 -13.229 -12.544 -11.745 -15.14 -14.583 -14.028 -13.472 -12.677 -11.663 -10.463 -9.1116 a23=-17.9439 -15.721 -15.339 -14.914 -14.472 -13.815 -13.155 -12.478 -11.676 -15.057 -14.517 -13.976 -13.431 -12.584 -11.585 -10.38 -9.0269 a24=-17.8505 -15.689 -15.309 -14.887 -14.381 -13.723 -13.082 -12.415 -11.599 -14.983 -14.457 -13.929 -13.394 -12.497 -11.509 -10.296 -8.9423 a25=-17.757 -15.659 -15.281 -14.862 -14.267 -13.637 -13.013 -12.355 -11.522 -14.918 -14.404 -13.885 -13.325 -12.412 -11.427 -10.212 -8.8581 a26=-17.6636 -15.631 -15.255 -14.788 -14.167 -13.556 -12.948 -12.293 -11.448 -14.859 -14.355 -13.845 -13.218 -12.334 -11.344 -10.129 -8.7738 a27=-17.5701 -15.605 -15.231 -14.664 -14.07 -13.481 -12.886 -12.235 -11.376 -14.806 -14.31 -13.808 -13.115 -12.257 -11.262 -10.046 -8.689 a28=-17.4766 -15.581 -15.126 -14.558 -13.984 -13.41 -12.828 -12.167 -11.3 -14.757 -14.269 -13.774 -13.023 -12.184 -11.181 -9.9649 -8.6044 a29=-17.3832 -15.512 -15 -14.454 -13.899 -13.343 -12.77 -12.095 -11.225 -14.713 -14.231 -13.742 -12.933 -12.113 -11.102 -9.8823 -8.5199 a30=-17.2897 -15.381 -14.889 -14.362 -13.824 -13.279 -12.714 -12.026 -11.151 -14.672 -14.196 -13.632 -12.849 -12.037 -11.021 -9.7999 -8.4356 a31=-17.1963 -15.258 -14.784 -14.273 -13.75 -13.219 -12.66 -11.954 -11.078 -14.634 -14.163 -13.528 -12.772 -11.961 -10.937 -9.7177 -8.351 a32=-17.1028 -15.147 -14.689 -14.193 -13.684 -13.161 -12.605 -11.878 -11.006 -14.599 -14.132 -13.428 -12.697 -11.887 -10.855 -9.6361 -8.2659 a33=-17.0093 -15.043 -14.599 -14.116 -13.618 -13.107 -12.543 -11.804 -10.934 -14.567 -14.062 -13.338 -12.627 -11.813 -10.775 -9.5547 -8.1805 a34=-16.9159 -14.948 -14.516 -14.046 -13.558 -13.052 -12.484 -11.731 -10.862 -14.536 -13.947 -13.252 -12.555 -11.741 -10.694 -9.4719 -8.0949 a35=-16.8224 -14.859 -14.438 -13.977 -13.499 -13.001 -12.414 -11.657 -10.79 -14.508 -13.843 -13.172 -12.488 -11.659 -10.614 -9.389 -8.009 a36=-16.729 -14.776 -14.365 -13.914 -13.444 -12.951 -12.343 -11.585 -10.72 -14.409 -13.744 -13.097 -12.422 -11.577 -10.533 -9.3068 -7.923 a37=-16.6355 -14.699 -14.296 -13.853 -13.391 -12.899 -12.27 -11.514 -10.648 -14.291 -13.655 -13.024 -12.357 -11.498 -10.454 -9.2237 -7.837 a38=-16.5421 -14.626 -14.231 -13.797 -13.341 -12.848 -12.191 -11.443 -10.574 -14.185 -13.57 -12.957 -12.292 -11.422 -10.375 -9.1406 -7.751 a39=-16.4486 -14.558 -14.17 -13.742 -13.292 -12.787 -12.117 -11.374 -10.502 -14.084 -13.492 -12.892 -12.223 -11.34 -10.294 -9.0578 -7.6648 a40=-16.3551 -14.493 -14.112 -13.691 -13.243 -12.716 -12.044 -11.306 -10.43 -13.993 -13.417 -12.83 -12.146 -11.26 -10.214 -8.9755 -7.5784 a41=-16.2617 -14.432 -14.056 -13.64 -13.197 -12.642 -11.972 -11.237 -10.356 -13.907 -13.349 -12.77 -12.073 -11.181 -10.134 -8.8928 -7.491 a42=-16.1682 -14.374 -14.004 -13.593 -13.152 -12.562 -11.902 -11.168 -10.283 -13.828 -13.281 -12.712 -11.996 -11.104 -10.056 -8.8099 -7.4033 a43=-16.0748 -14.319 -13.953 -13.546 -13.095 -12.485 -11.833 -11.099 -10.209 -13.753 -13.219 -12.654 -11.915 -11.027 -9.9754 -8.7271 -7.3155 a44=-15.9813 -14.266 -13.905 -13.503 -13.02 -12.41 -11.765 -11.03 -10.135 -13.682 -13.159 -12.589 -11.836 -10.95 -9.8946 -8.6437 -7.2273 a45=-15.8879 -14.216 -13.859 -13.459 -12.938 -12.337 -11.698 -10.96 -10.061 -13.616 -13.102 -12.525 -11.758 -10.873 -9.8143 -8.5597 -7.139 a46=-15.7944 -14.169 -13.815 -13.414 -12.857 -12.265 -11.632 -10.89 -9.987 -13.552 -13.046 -12.45 -11.679 -10.797 -9.7333 -8.4757 -7.0507 a47=-15.7009 -14.123 -13.772 -13.338 -12.776 -12.196 -11.568 -10.818 -9.9136 -13.494 -12.993 -12.374 -11.602 -10.72 -9.6524 -8.3919 -6.962 a48=-15.6075 -14.079 -13.73 -13.251 -12.699 -12.129 -11.503 -10.746 -9.8396 -13.436 -12.937 -12.295 -11.527 -10.642 -9.5717 -8.3078 -6.8732 a49=-15.514 -14.036 -13.683 -13.164 -12.626 -12.064 -11.436 -10.674 -9.7655 -13.382 -12.883 -12.213 -11.452 -10.564 -9.4908 -8.2237 -6.7832 a50=-15.4206 -13.996 -13.588 -13.08 -12.555 -12 -11.37 -10.601 -9.6918 -13.33 -12.817 -12.134 -11.379 -10.487 -9.4101 -8.1396 -6.6928 a701=45.4206 13.169 13.248 13.338 13.439 13.55 13.671 13.805 13.952 13.439 13.55 13.671 13.805 13.952 14.113 14.287 14.467 a702=45.514 13.181 13.259 13.349 13.45 13.56 13.682 13.815 13.962 13.45 13.56 13.682 13.815 13.962 14.122 14.296 14.476 a703=45.6075 13.192 13.27 13.361 13.461 13.571 13.692 13.825 13.972 13.461 13.571 13.692 13.825 13.972 14.132 14.306 14.485 a704=45.7009 13.204 13.282 13.372 13.472 13.582 13.703 13.836 13.982 13.472 13.582 13.703 13.836 13.982 14.142 14.316 14.495 a705=45.7944 13.215 13.293 13.383 13.483 13.593 13.713 13.846 13.992 13.483 13.593 13.713 13.846 13.992 14.152 14.325 14.504 a706=45.8879 13.227 13.304 13.394 13.494 13.603 13.724 13.856 14.002 13.494 13.603 13.724 13.856 14.002 14.162 14.335 14.513 a707=45.9813 13.238 13.315 13.405 13.505 13.614 13.734 13.867 14.012 13.505 13.614 13.734 13.867 14.012 14.172 14.344 14.522 a708=46.0748 13.25 13.327 13.416 13.516 13.625 13.745 13.877 14.022 13.516 13.625 13.745 13.877 14.022 14.181 14.354 14.532 a709=46.1682 13.261 13.338 13.427 13.527 13.636 13.755 13.887 14.032 13.527 13.636 13.755 13.887 14.032 14.191 14.363 14.541 a710=46.2617 13.272 13.349 13.438 13.537 13.646 13.766 13.897 14.042 13.537 13.646 13.766 13.897 14.042 14.201 14.373 14.55 a711=46.3551 13.284 13.36 13.449 13.548 13.657 13.776 13.908 14.052 13.548 13.657 13.776 13.908 14.052 14.211 14.382 14.559 a712=46.4486 13.295 13.371 13.46 13.559 13.668 13.787 13.918 14.062 13.559 13.668 13.787 13.918 14.062 14.22 14.391 14.568 a713=46.5421 13.306 13.383 13.471 13.57 13.678 13.797 13.928 14.072 13.57 13.678 13.797 13.928 14.072 14.23 14.401 14.577 a714=46.6355 13.317 13.394 13.482 13.581 13.689 13.808 13.938 14.082 13.581 13.689 13.808 13.938 14.082 14.24 14.41 14.587 a715=46.729 13.329 13.405 13.493 13.591 13.699 13.818 13.948 14.092 13.591 13.699 13.818 13.948 14.092 14.249 14.42 14.596 a716=46.8224 13.34 13.416 13.504 13.602 13.71 13.828 13.959 14.102 13.602 13.71 13.828 13.959 14.102 14.259 14.429 14.605 a717=46.9159 13.351 13.427 13.515 13.613 13.72 13.839 13.969 14.112 13.613 13.72 13.839 13.969 14.112 14.269 14.438 14.614 a718=47.0093 13.362 13.438 13.526 13.624 13.731 13.849 13.979 14.122 13.624 13.731 13.849 13.979 14.122 14.278 14.448 14.623 a719=47.1028 13.373 13.449 13.537 13.634 13.742 13.859 13.989 14.131 13.634 13.742 13.859 13.989 14.131 14.288 14.457 14.632 a720=47.1963 13.385 13.46 13.548 13.645 13.752 13.87 13.999 14.141 13.645 13.752 13.87 13.999 14.141 14.297 14.466 14.641 a721=47.2897 13.396 13.471 13.558 13.656 13.762 13.88 14.009 14.151 13.656 13.762 13.88 14.009 14.151 14.307 14.476 14.65 a722=47.3832 13.407 13.482 13.569 13.666 13.773 13.89 14.019 14.161 13.666 13.773 13.89 14.019 14.161 14.316 14.485 14.659 a723=47.4766 13.418 13.493 13.58 13.677 13.783 13.9 14.029 14.171 13.677 13.783 13.9 14.029 14.171 14.326 14.494 14.668 a724=47.5701 13.429 13.504 13.591 13.687 13.794 13.911 14.039 14.18 13.687 13.794 13.911 14.039 14.18 14.335 14.503 14.677 a725=47.6636 13.44 13.515 13.602 13.698 13.804 13.921 14.049 14.19 13.698 13.804 13.921 14.049 14.19 14.345 14.513 14.686 a726=47.757 13.451 13.526 13.612 13.709 13.815 13.931 14.059 14.2 13.709 13.815 13.931 14.059 14.2 14.354 14.522 14.695 a727=47.8505 13.462 13.536 13.623 13.719 13.825 13.941 14.069 14.21 13.719 13.825 13.941 14.069 14.21 14.364 14.531 14.704 a728=47.9439 13.473 13.547 13.634 13.73 13.835 13.951 14.079 14.219 13.73 13.835 13.951 14.079 14.219 14.373 14.54 14.713 a729=48.0374 13.484 13.558 13.644 13.74 13.846 13.961 14.089 14.229 13.74 13.846 13.961 14.089 14.229 14.383 14.549 14.722 a730=48.1308 13.495 13.569 13.655 13.751 13.856 13.972 14.099 14.239 13.751 13.856 13.972 14.099 14.239 14.392 14.559 14.731 a731=48.2243 13.506 13.58 13.666 13.761 13.866 13.982 14.109 14.248 13.761 13.866 13.982 14.109 14.248 14.402 14.568 14.739 a732=48.3178 13.517 13.59 13.676 13.772 13.876 13.992 14.118 14.258 13.772 13.876 13.992 14.118 14.258 14.411 14.577 14.748 a733=48.4112 13.527 13.601 13.687 13.782 13.887 14.002 14.128 14.268 13.782 13.887 14.002 14.128 14.268 14.42 14.586 14.757 a734=48.5047 13.538 13.612 13.697 13.792 13.897 14.012 14.138 14.277 13.792 13.897 14.012 14.138 14.277 14.43 14.595 14.766 a735=48.5981 13.549 13.623 13.708 13.803 13.907 14.022 14.148 14.287 13.803 13.907 14.022 14.148 14.287 14.439 14.604 14.775 a736=48.6916 13.56 13.633 13.719 13.813 13.917 14.032 14.158 14.296 13.813 13.917 14.032 14.158 14.296 14.449 14.613 14.784 a737=48.785 13.571 13.644 13.729 13.824 13.928 14.042 14.168 14.306 13.824 13.928 14.042 14.168 14.306 14.458 14.622 14.792 a738=48.8785 13.582 13.655 13.74 13.834 13.938 14.052 14.177 14.316 13.834 13.938 14.052 14.177 14.316 14.467 14.631 14.801 a739=48.972 13.592 13.665 13.75 13.844 13.948 14.062 14.187 14.325 13.844 13.948 14.062 14.187 14.325 14.476 14.64 14.81 a740=49.0654 13.603 13.676 13.761 13.855 13.958 14.072 14.197 14.335 13.855 13.958 14.072 14.197 14.335 14.486 14.65 14.819 a741=49.1589 13.614 13.687 13.771 13.865 13.968 14.082 14.207 14.344 13.865 13.968 14.082 14.207 14.344 14.495 14.659 14.828 a742=49.2523 13.625 13.697 13.781 13.875 13.978 14.092 14.216 14.354 13.875 13.978 14.092 14.216 14.354 14.504 14.668 14.836 a743=49.3458 13.635 13.708 13.792 13.885 13.988 14.101 14.226 14.363 13.885 13.988 14.101 14.226 14.363 14.513 14.677 14.845 a744=49.4393 13.646 13.718 13.802 13.896 13.998 14.111 14.236 14.372 13.896 13.998 14.111 14.236 14.372 14.523 14.685 14.854 a745=49.5327 13.657 13.729 13.813 13.906 14.008 14.121 14.245 14.382 13.906 14.008 14.121 14.245 14.382 14.532 14.694 14.862 a746=49.6262 13.667 13.739 13.823 13.916 14.018 14.131 14.255 14.391 13.916 14.018 14.131 14.255 14.391 14.541 14.703 14.871 a747=49.7196 13.678 13.75 13.833 13.926 14.028 14.141 14.265 14.401 13.926 14.028 14.141 14.265 14.401 14.55 14.712 14.88 a748=49.8131 13.688 13.76 13.844 13.936 14.038 14.151 14.274 14.41 13.936 14.038 14.151 14.274 14.41 14.559 14.721 14.888 a749=49.9065 13.699 13.771 13.854 13.947 14.048 14.161 14.284 14.42 13.947 14.048 14.161 14.284 14.42 14.569 14.73 14.897 a750=50 13.709 13.781 13.864 13.957 14.058 14.17 14.293 14.429 13.957 14.058 14.17 14.293 14.429 14.578 14.739 14.906 tb_pol_a: optimal asset choice for each state space point zi1_zr_0_025_zw_0_34664 zi2_zr_0_025_zw_0_42338 zi3_zr_0_025_zw_0_51712 zi4_zr_0_025_zw_0_63162 zi5_zr_0_025_zw_0_77146 zi6_zr_0_025_zw_0_94226 zi7_zr_0_025_zw_1_1509 zi8_zr_0_025_zw_1_4057 zi48_zr_0_095_zw_0_63162 zi49_zr_0_095_zw_0_77146 zi50_zr_0_095_zw_0_94226 zi51_zr_0_095_zw_1_1509 zi52_zr_0_095_zw_1_4057 zi53_zr_0_095_zw_1_7169 zi54_zr_0_095_zw_2_097 zi55_zr_0_095_zw_2_5613 _______________________ _______________________ _______________________ _______________________ _______________________ _______________________ ______________________ ______________________ ________________________ ________________________ ________________________ _______________________ _______________________ _______________________ ______________________ _______________________ a1=-20 0 0 0 0 -19.512 -19.512 -19.512 -19.512 0 0 0 0 0 0 -18.265 -17.753 a2=-19.9065 0 0 0 -19.512 -19.512 -19.512 -19.512 -19.512 0 0 0 0 0 -18.265 -18.265 -17.667 a3=-19.8131 0 0 0 -19.512 -19.512 -19.512 -19.512 -19.512 0 0 0 0 0 -18.265 -18.265 -17.582 a4=-19.7196 0 0 -19.512 -19.512 -19.512 -19.512 -19.512 -19.512 0 0 0 0 0 -18.265 -18.009 -17.497 a5=-19.6262 -19.512 -19.512 -19.512 -19.512 -19.512 -19.512 -19.512 -19.512 0 0 0 0 0 -18.265 -17.923 -17.411 a6=-19.5327 -19.512 -19.512 -19.512 -19.512 -19.512 -19.512 -19.512 -19.512 0 0 0 0 0 -18.265 -17.923 -17.241 a7=-19.4393 -19.512 -19.512 -19.512 -19.512 -19.512 -19.512 -19.512 -18.6 0 0 0 0 -18.265 -18.265 -17.753 -17.241 a8=-19.3458 -19.512 -19.512 -19.512 -19.512 -19.512 -19.512 -19.512 -18.509 0 0 0 0 -18.265 -18.265 -17.667 -17.07 a9=-19.2523 -19.512 -19.512 -19.512 -19.512 -19.512 -19.512 -19.512 -18.418 0 0 0 0 -18.265 -18.265 -17.582 -17.07 a10=-19.1589 -19.512 -19.512 -19.512 -19.512 -19.512 -19.512 -19.512 -18.418 0 0 0 -18.265 -18.265 -18.265 -17.497 -16.899 a11=-19.0654 -19.512 -19.512 -19.512 -19.512 -19.512 -19.512 -19.512 -18.327 0 0 0 -18.265 -18.265 -18.009 -17.411 -16.814 a12=-18.972 -19.512 -19.512 -19.512 -19.512 -19.512 -19.512 -19.512 -18.236 0 0 -18.265 -18.265 -18.265 -17.753 -17.326 -16.729 a13=-18.8785 -19.512 -19.512 -19.512 -19.512 -19.512 -19.512 -18.327 -18.236 0 0 -18.265 -18.265 -18.265 -17.667 -17.241 -16.643 a14=-18.785 -19.512 -19.512 -19.512 -19.512 -19.512 -19.512 -18.327 -18.145 0 -18.265 -18.265 -18.265 -18.265 -17.497 -17.155 -16.558 a15=-18.6916 -19.512 -19.512 -19.512 -19.512 -19.512 -19.512 -18.236 -18.053 0 -18.265 -18.265 -18.265 -18.265 -17.497 -17.07 -16.472 a16=-18.5981 -19.512 -19.512 -19.512 -19.512 -19.512 -19.512 -18.145 -18.053 -18.265 -18.265 -18.265 -18.265 -18.265 -17.411 -16.985 -16.387 a17=-18.5047 -19.512 -19.512 -19.512 -19.512 -19.512 -18.145 -18.145 -17.962 -18.265 -18.265 -18.265 -18.265 -18.265 -17.326 -16.899 -16.302 a18=-18.4112 -19.512 -19.512 -19.512 -19.512 -19.512 -18.145 -18.053 -17.78 -18.265 -18.265 -18.265 -18.265 -18.265 -17.241 -16.814 -16.216 a19=-18.3178 -19.512 -19.512 -19.512 -19.512 -19.512 -18.053 -18.053 -17.689 -18.265 -18.265 -18.265 -18.265 -17.411 -17.155 -16.643 -16.131 a20=-18.2243 -19.512 -19.512 -19.512 -19.512 -19.512 -18.053 -17.962 -17.689 -18.265 -18.265 -18.265 -18.265 -17.411 -17.07 -16.643 -16.046 a21=-18.1308 -19.512 -19.512 -19.512 -19.512 -18.053 -17.962 -17.962 -17.506 -18.265 -18.265 -18.265 -18.265 -17.241 -17.07 -16.558 -15.96 a22=-18.0374 -19.512 -19.512 -19.512 -19.512 -17.962 -17.962 -17.78 -17.506 -18.265 -18.265 -18.265 -18.265 -17.241 -16.985 -16.472 -15.79 a23=-17.9439 -19.512 -19.512 -19.512 -19.512 -17.962 -17.871 -17.78 -17.324 -18.265 -18.265 -18.265 -18.265 -17.155 -16.899 -16.387 -15.704 a24=-17.8505 -19.512 -19.512 -19.512 -17.871 -17.871 -17.871 -17.689 -17.233 -18.265 -18.265 -18.265 -18.265 -17.07 -16.814 -16.216 -15.619 a25=-17.757 -19.512 -19.512 -19.512 -17.871 -17.871 -17.78 -17.597 -17.142 -18.265 -18.265 -18.265 -17.155 -17.07 -16.643 -16.131 -15.534 a26=-17.6636 -19.512 -19.512 -17.871 -17.78 -17.78 -17.78 -17.506 -17.05 -18.265 -18.265 -18.265 -17.155 -16.985 -16.558 -16.046 -15.448 a27=-17.5701 -19.512 -19.512 -17.78 -17.78 -17.78 -17.689 -17.506 -16.868 -18.265 -18.265 -18.265 -17.07 -16.899 -16.472 -15.96 -15.363 a28=-17.4766 -19.512 -17.78 -17.78 -17.689 -17.689 -17.689 -17.233 -16.868 -18.265 -18.265 -18.265 -16.985 -16.899 -16.387 -15.875 -15.278 a29=-17.3832 -17.689 -17.689 -17.689 -17.689 -17.689 -17.597 -17.142 -16.777 -18.265 -18.265 -18.265 -16.985 -16.814 -16.302 -15.79 -15.192 a30=-17.2897 -17.689 -17.689 -17.689 -17.689 -17.597 -17.506 -17.05 -16.686 -18.265 -18.265 -16.985 -16.899 -16.643 -16.131 -15.704 -15.107 a31=-17.1963 -17.597 -17.597 -17.597 -17.597 -17.597 -17.415 -16.868 -16.594 -18.265 -18.265 -16.899 -16.899 -16.558 -16.046 -15.619 -15.022 a32=-17.1028 -17.597 -17.597 -17.597 -17.597 -17.506 -17.233 -16.777 -16.503 -18.265 -18.265 -16.899 -16.814 -16.472 -16.046 -15.534 -14.851 a33=-17.0093 -17.506 -17.506 -17.506 -17.506 -17.506 -17.142 -16.686 -16.412 -18.265 -16.899 -16.814 -16.729 -16.387 -15.96 -15.448 -14.766 a34=-16.9159 -17.506 -17.506 -17.506 -17.415 -17.415 -17.05 -16.594 -16.321 -18.265 -16.814 -16.814 -16.643 -16.216 -15.79 -15.278 -14.68 a35=-16.8224 -17.415 -17.415 -17.415 -17.415 -17.324 -16.777 -16.503 -16.23 -18.265 -16.814 -16.729 -16.558 -16.131 -15.704 -15.192 -14.595 a36=-16.729 -17.415 -17.415 -17.415 -17.324 -17.233 -16.686 -16.412 -16.139 -16.729 -16.729 -16.729 -16.558 -16.046 -15.619 -15.107 -14.509 a37=-16.6355 -17.324 -17.324 -17.324 -17.324 -17.142 -16.594 -16.412 -16.047 -16.729 -16.729 -16.643 -16.472 -15.96 -15.534 -15.022 -14.424 a38=-16.5421 -17.324 -17.324 -17.324 -17.233 -17.05 -16.503 -16.321 -15.956 -16.643 -16.643 -16.558 -16.387 -15.875 -15.448 -14.936 -14.339 a39=-16.4486 -17.233 -17.233 -17.233 -17.233 -16.777 -16.412 -16.23 -15.865 -16.643 -16.643 -16.558 -16.131 -15.79 -15.363 -14.851 -14.253 a40=-16.3551 -17.233 -17.233 -17.233 -17.142 -16.503 -16.321 -16.139 -15.774 -16.558 -16.558 -16.472 -16.046 -15.704 -15.278 -14.766 -14.168 a41=-16.2617 -17.142 -17.142 -17.142 -17.05 -16.412 -16.23 -16.047 -15.592 -16.558 -16.472 -16.387 -15.96 -15.619 -15.192 -14.68 -13.997 a42=-16.1682 -17.142 -17.142 -17.05 -16.868 -16.321 -16.23 -15.956 -15.592 -16.472 -16.472 -16.387 -15.79 -15.534 -15.107 -14.595 -13.912 a43=-16.0748 -17.05 -17.05 -17.05 -16.503 -16.23 -16.139 -15.865 -15.409 -16.472 -16.387 -16.216 -15.704 -15.448 -14.936 -14.509 -13.827 a44=-15.9813 -17.05 -17.05 -16.959 -16.23 -16.139 -16.047 -15.683 -15.318 -16.387 -16.387 -16.046 -15.619 -15.278 -14.851 -14.339 -13.741 a45=-15.8879 -16.959 -16.959 -16.868 -16.139 -16.047 -15.956 -15.592 -15.227 -16.387 -16.302 -15.96 -15.534 -15.192 -14.766 -14.253 -13.656 a46=-15.7944 -16.959 -16.868 -16.777 -16.047 -16.047 -15.865 -15.5 -15.136 -16.302 -16.216 -15.704 -15.448 -15.192 -14.68 -14.168 -13.571 a47=-15.7009 -16.868 -16.868 -16.047 -16.047 -15.956 -15.774 -15.409 -15.044 -16.302 -16.131 -15.619 -15.363 -15.022 -14.595 -14.083 -13.485 a48=-15.6075 -16.868 -16.777 -15.956 -15.956 -15.865 -15.683 -15.318 -14.953 -16.216 -16.046 -15.448 -15.278 -14.936 -14.509 -13.997 -13.4 a49=-15.514 -16.777 -15.956 -15.956 -15.865 -15.774 -15.592 -15.227 -14.862 -16.131 -15.96 -15.448 -15.192 -14.851 -14.424 -13.912 -13.229 a50=-15.4206 -16.686 -15.865 -15.865 -15.865 -15.774 -15.409 -15.136 -14.771 -16.131 -15.704 -15.363 -15.107 -14.766 -14.339 -13.827 -13.144 a701=45.4206 41.851 41.851 41.942 42.033 42.216 42.398 42.58 42.854 42.033 42.216 42.398 42.58 42.854 43.127 43.583 44.039 a702=45.514 41.942 41.942 42.033 42.124 42.307 42.489 42.672 42.945 42.124 42.307 42.489 42.672 42.945 43.219 43.674 44.13 a703=45.6075 42.033 42.033 42.124 42.216 42.398 42.58 42.763 43.036 42.216 42.398 42.58 42.763 43.036 43.31 43.674 44.222 a704=45.7009 42.124 42.124 42.216 42.307 42.489 42.672 42.854 43.127 42.307 42.489 42.672 42.854 43.127 43.401 43.766 44.313 a705=45.7944 42.216 42.216 42.307 42.398 42.58 42.763 42.945 43.219 42.398 42.58 42.763 42.945 43.219 43.492 43.857 44.404 a706=45.8879 42.307 42.307 42.398 42.489 42.672 42.763 43.036 43.31 42.489 42.672 42.763 43.036 43.31 43.583 43.948 44.495 a707=45.9813 42.398 42.398 42.489 42.58 42.763 42.854 43.127 43.401 42.58 42.763 42.854 43.127 43.401 43.674 44.039 44.586 a708=46.0748 42.489 42.489 42.58 42.672 42.854 42.945 43.219 43.401 42.672 42.854 42.945 43.219 43.401 43.766 44.13 44.677 a709=46.1682 42.489 42.58 42.672 42.763 42.945 43.036 43.31 43.492 42.763 42.945 43.036 43.31 43.492 43.857 44.222 44.769 a710=46.2617 42.58 42.672 42.763 42.854 43.036 43.127 43.401 43.583 42.854 43.036 43.127 43.401 43.583 43.948 44.313 44.86 a711=46.3551 42.672 42.763 42.854 42.945 43.127 43.219 43.492 43.674 42.945 43.127 43.219 43.492 43.674 44.039 44.404 44.951 a712=46.4486 42.763 42.854 42.945 43.036 43.219 43.31 43.583 43.766 43.036 43.219 43.31 43.583 43.766 44.13 44.495 45.042 a713=46.5421 42.854 42.945 43.036 43.127 43.219 43.401 43.674 43.857 43.127 43.219 43.401 43.674 43.857 44.222 44.586 45.133 a714=46.6355 42.945 43.036 43.127 43.219 43.31 43.492 43.674 43.948 43.219 43.31 43.492 43.674 43.948 44.313 44.677 45.225 a715=46.729 43.036 43.127 43.219 43.31 43.401 43.583 43.766 44.039 43.31 43.401 43.583 43.766 44.039 44.404 44.769 45.316 a716=46.8224 43.127 43.219 43.31 43.401 43.492 43.674 43.857 44.13 43.401 43.492 43.674 43.857 44.13 44.495 44.86 45.407 a717=46.9159 43.219 43.31 43.401 43.492 43.583 43.766 43.948 44.222 43.492 43.583 43.766 43.948 44.222 44.586 44.951 45.498 a718=47.0093 43.31 43.401 43.492 43.583 43.674 43.857 44.039 44.313 43.583 43.674 43.857 44.039 44.313 44.677 45.042 45.589 a719=47.1028 43.401 43.492 43.583 43.674 43.766 43.948 44.13 44.404 43.674 43.766 43.948 44.13 44.404 44.769 45.133 45.68 a720=47.1963 43.492 43.583 43.674 43.766 43.857 44.039 44.222 44.495 43.766 43.857 44.039 44.222 44.495 44.86 45.225 45.68 a721=47.2897 43.583 43.674 43.766 43.857 43.948 44.13 44.313 44.586 43.857 43.948 44.13 44.313 44.586 44.951 45.316 45.772 a722=47.3832 43.674 43.766 43.857 43.948 44.039 44.222 44.404 44.677 43.948 44.039 44.222 44.404 44.677 45.042 45.407 45.863 a723=47.4766 43.766 43.857 43.948 44.039 44.13 44.313 44.495 44.769 44.039 44.13 44.313 44.495 44.769 45.133 45.498 45.954 a724=47.5701 43.857 43.948 44.039 44.13 44.222 44.404 44.586 44.86 44.13 44.222 44.404 44.586 44.86 45.225 45.589 46.045 a725=47.6636 43.948 44.039 44.13 44.222 44.313 44.495 44.677 44.951 44.222 44.313 44.495 44.677 44.951 45.225 45.68 46.136 a726=47.757 44.039 44.13 44.222 44.313 44.404 44.586 44.769 45.042 44.313 44.404 44.586 44.769 45.042 45.316 45.772 46.227 a727=47.8505 44.13 44.222 44.313 44.404 44.495 44.677 44.86 45.133 44.404 44.495 44.677 44.86 45.133 45.407 45.863 46.319 a728=47.9439 44.222 44.313 44.404 44.495 44.586 44.769 44.951 45.225 44.495 44.586 44.769 44.951 45.225 45.498 45.954 46.41 a729=48.0374 44.313 44.404 44.404 44.586 44.677 44.86 45.042 45.316 44.586 44.677 44.86 45.042 45.316 45.589 46.045 46.501 a730=48.1308 44.404 44.495 44.495 44.677 44.769 44.951 45.133 45.407 44.677 44.769 44.951 45.133 45.407 45.68 46.136 46.592 a731=48.2243 44.495 44.586 44.586 44.769 44.86 45.042 45.225 45.498 44.769 44.86 45.042 45.225 45.498 45.772 46.227 46.683 a732=48.3178 44.586 44.677 44.677 44.86 44.951 45.133 45.316 45.589 44.86 44.951 45.133 45.316 45.589 45.863 46.319 46.775 a733=48.4112 44.677 44.677 44.769 44.86 45.042 45.225 45.407 45.68 44.86 45.042 45.225 45.407 45.68 45.954 46.41 46.866 a734=48.5047 44.769 44.769 44.86 44.951 45.133 45.316 45.498 45.772 44.951 45.133 45.316 45.498 45.772 46.045 46.501 46.957 a735=48.5981 44.86 44.86 44.951 45.042 45.225 45.407 45.589 45.863 45.042 45.225 45.407 45.589 45.863 46.136 46.592 47.048 a736=48.6916 44.951 44.951 45.042 45.133 45.316 45.498 45.68 45.954 45.133 45.316 45.498 45.68 45.954 46.227 46.683 47.139 a737=48.785 45.042 45.042 45.133 45.225 45.407 45.589 45.772 46.045 45.225 45.407 45.589 45.772 46.045 46.319 46.775 47.23 a738=48.8785 45.133 45.133 45.225 45.316 45.498 45.68 45.863 46.136 45.316 45.498 45.68 45.863 46.136 46.41 46.775 47.322 a739=48.972 45.225 45.225 45.316 45.407 45.589 45.772 45.954 46.227 45.407 45.589 45.772 45.954 46.227 46.501 46.866 47.413 a740=49.0654 45.316 45.316 45.407 45.498 45.68 45.772 46.045 46.319 45.498 45.68 45.772 46.045 46.319 46.592 46.957 47.504 a741=49.1589 45.407 45.407 45.498 45.589 45.772 45.863 46.136 46.41 45.589 45.772 45.863 46.136 46.41 46.683 47.048 47.595 a742=49.2523 45.498 45.498 45.589 45.68 45.863 45.954 46.227 46.41 45.68 45.863 45.954 46.227 46.41 46.775 47.139 47.686 a743=49.3458 45.498 45.589 45.68 45.772 45.954 46.045 46.319 46.501 45.772 45.954 46.045 46.319 46.501 46.866 47.23 47.778 a744=49.4393 45.589 45.68 45.772 45.863 46.045 46.136 46.41 46.592 45.863 46.045 46.136 46.41 46.592 46.957 47.322 47.869 a745=49.5327 45.68 45.772 45.863 45.954 46.136 46.227 46.501 46.683 45.954 46.136 46.227 46.501 46.683 47.048 47.413 47.96 a746=49.6262 45.772 45.863 45.954 46.045 46.227 46.319 46.592 46.775 46.045 46.227 46.319 46.592 46.775 47.139 47.504 48.051 a747=49.7196 45.863 45.954 46.045 46.136 46.227 46.41 46.683 46.866 46.136 46.227 46.41 46.683 46.866 47.23 47.595 48.142 a748=49.8131 45.954 46.045 46.136 46.227 46.319 46.501 46.683 46.957 46.227 46.319 46.501 46.683 46.957 47.322 47.686 48.233 a749=49.9065 46.045 46.136 46.227 46.319 46.41 46.592 46.775 47.048 46.319 46.41 46.592 46.775 47.048 47.413 47.778 48.325 a750=50 46.136 46.227 46.319 46.41 46.501 46.683 46.866 47.139 46.41 46.501 46.683 46.866 47.139 47.504 47.869 48.416
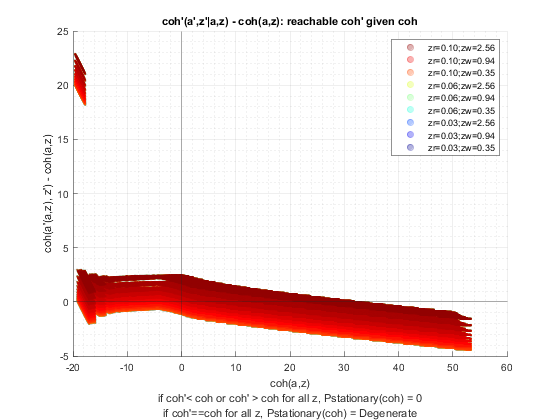
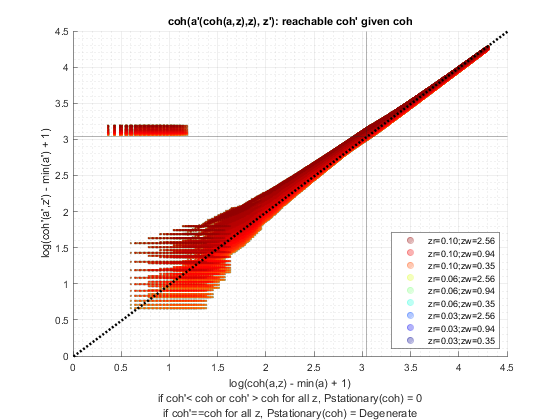
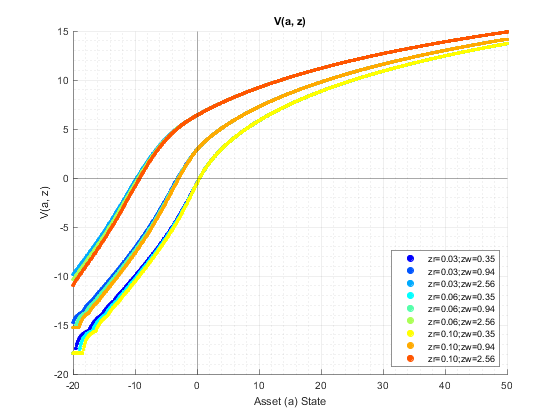
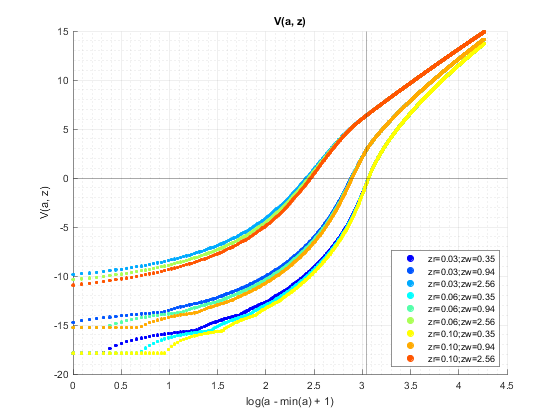
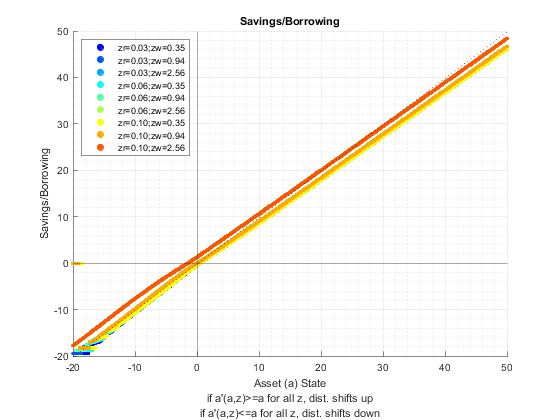
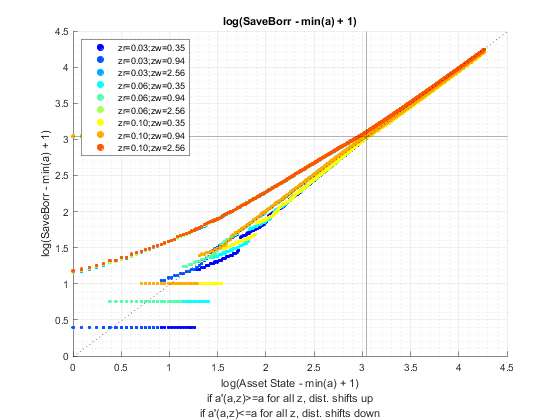
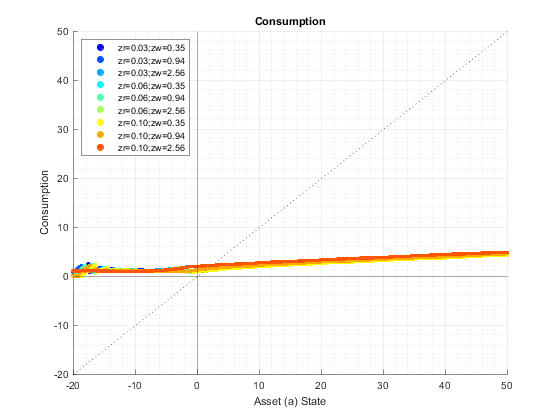
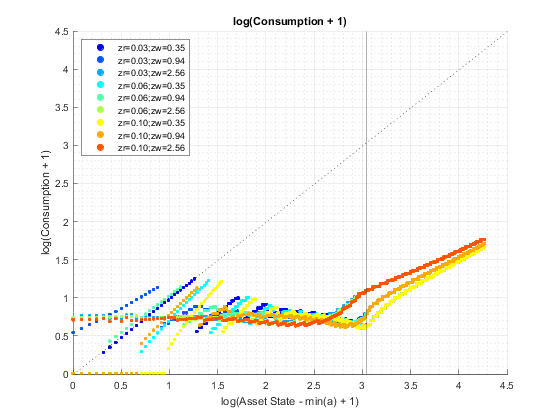
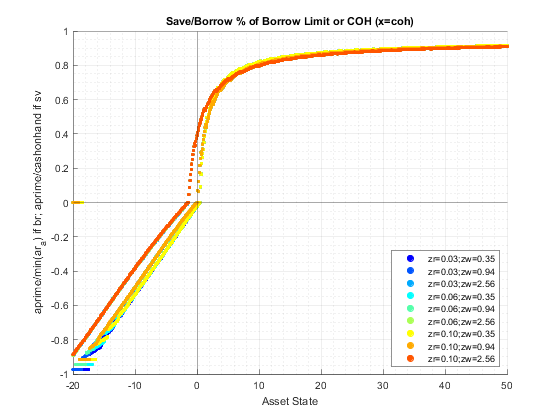
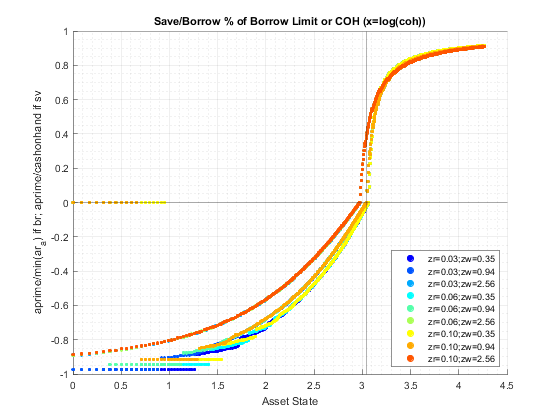
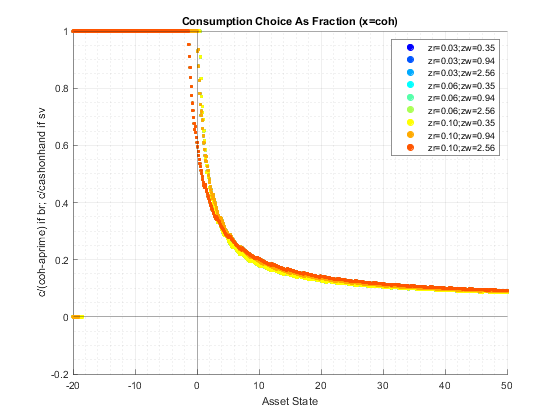
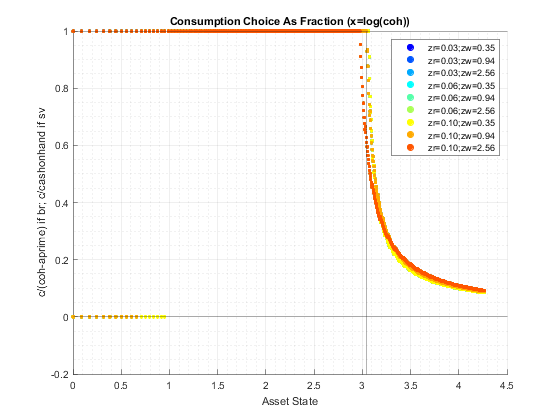
end
ans = Map with properties: Count: 13 KeyType: char ValueType: any